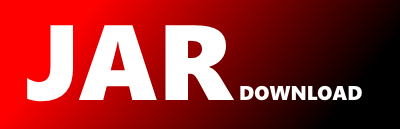
package.lib.esm.index.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of uncontrollable Show documentation
Show all versions of uncontrollable Show documentation
Wrap a controlled react component, to allow specific prop/handler pairs to be uncontrolled
The newest version!
function _objectWithoutPropertiesLoose(source, excluded) { if (source == null) return {}; var target = {}; var sourceKeys = Object.keys(source); var key, i; for (i = 0; i < sourceKeys.length; i++) { key = sourceKeys[i]; if (excluded.indexOf(key) >= 0) continue; target[key] = source[key]; } return target; }
function _toPropertyKey(arg) { var key = _toPrimitive(arg, "string"); return typeof key === "symbol" ? key : String(key); }
function _toPrimitive(input, hint) { if (typeof input !== "object" || input === null) return input; var prim = input[Symbol.toPrimitive]; if (prim !== undefined) { var res = prim.call(input, hint || "default"); if (typeof res !== "object") return res; throw new TypeError("@@toPrimitive must return a primitive value."); } return (hint === "string" ? String : Number)(input); }
import { useCallback, useRef, useState } from 'react';
export function defaultKey(key) {
return 'default' + key.charAt(0).toUpperCase() + key.substr(1);
}
function useUncontrolledProp(propValue, defaultValue, handler) {
const wasPropRef = useRef(propValue !== undefined);
const [stateValue, setState] = useState(defaultValue);
const isProp = propValue !== undefined;
const wasProp = wasPropRef.current;
wasPropRef.current = isProp;
/**
* If a prop switches from controlled to Uncontrolled
* reset its value to the defaultValue
*/
if (!isProp && wasProp && stateValue !== defaultValue) {
setState(defaultValue);
}
return [isProp ? propValue : stateValue, useCallback((...args) => {
const [value, ...rest] = args;
let returnValue = handler == null ? void 0 : handler(value, ...rest);
setState(value);
return returnValue;
}, [handler])];
}
export { useUncontrolledProp };
export function useUncontrolled(props, config) {
return Object.keys(config).reduce((result, fieldName) => {
const _ref = result,
_defaultKey = defaultKey(fieldName),
{
[_defaultKey]: defaultValue,
[fieldName]: propsValue
} = _ref,
rest = _objectWithoutPropertiesLoose(_ref, [_defaultKey, fieldName].map(_toPropertyKey));
const handlerName = config[fieldName];
const [value, handler] = useUncontrolledProp(propsValue, defaultValue, props[handlerName]);
return Object.assign({}, rest, {
[fieldName]: value,
[handlerName]: handler
});
}, props);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy