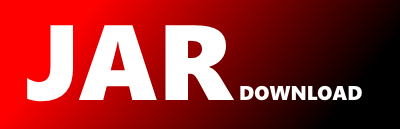
package.test.test.js Maven / Gradle / Ivy
import { render, fireEvent } from '@testing-library/react';
import React from 'react';
import { useUncontrolled } from '../src';
describe('uncontrollable', () => {
it('should track internally if not specified', () => {
let ref = {};
let Control = (props) => {
props = useUncontrolled(props, { value: 'onChange' });
ref.current = props;
return (
props.onChange(+e.target.value)}
/>
);
};
let inst = render( );
fireEvent.change(inst.getByTestId('input'), { target: { value: 42 } });
expect(ref.current.value).toEqual(42);
});
it('should allow for defaultProp', () => {
let ref = {};
let Control = (props) => {
props = useUncontrolled(props, {
value: 'onChange',
open: 'onToggle',
});
ref.current = props;
return (
props.onChange(+e.target.value)}
/>
);
};
let inst = render( );
expect(inst.container.querySelectorAll('.open')).toHaveLength(1);
expect(ref.current.defaultValue).not.toBeDefined();
expect(ref.current.defaultOpen).not.toBeDefined();
let input = inst.container.querySelector('input');
expect(input.value).toEqual('10');
fireEvent.change(inst.getByTestId('input'), { target: { value: 42 } });
expect(ref.current.value).toEqual(42);
});
it('should revert to defaultProp when switched to uncontrolled', () => {
let ref = {};
let Control = (props) => {
props = useUncontrolled(props, { value: 'onChange' });
ref.current = props;
return (
props.onChange(e.value)}
/>
);
};
let inst = render(
{}} />
);
expect(ref.current.value).toEqual('bar');
inst.rerender( {}} />);
expect(ref.current.value).toEqual('foo');
inst.rerender(
{}} />
);
expect(ref.current.value).toEqual('bar');
inst.rerender(
{}} />
);
expect(ref.current.value).toEqual('baz');
});
});
© 2015 - 2025 Weber Informatics LLC | Privacy Policy