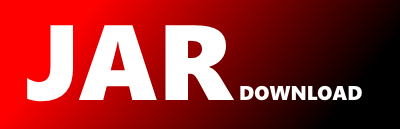
ght.yangtools.yang-parser-impl.1.0.2-Boron-SR2.source-code.YangStatementParser Maven / Gradle / Ivy
// Generated from YangStatementParser.g4 by ANTLR 4.5.1
package org.opendaylight.yangtools.antlrv4.code.gen;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class YangStatementParser extends Parser {
static { RuntimeMetaData.checkVersion("4.5.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
SEMICOLON=1, LEFT_BRACE=2, RIGHT_BRACE=3, SEP=4, IDENTIFIER=5, COLON=6,
PLUS=7, LINE_COMMENT=8, START_BLOCK_COMMENT=9, STRING=10, END_BLOCK_COMMENT=11;
public static final int
RULE_statement = 0, RULE_keyword = 1, RULE_argument = 2;
public static final String[] ruleNames = {
"statement", "keyword", "argument"
};
private static final String[] _LITERAL_NAMES = {
null, "';'", "'{'", "'}'", null, null, "':'", "'+'", null, "'/*'", null,
"'*/'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, "SEMICOLON", "LEFT_BRACE", "RIGHT_BRACE", "SEP", "IDENTIFIER", "COLON",
"PLUS", "LINE_COMMENT", "START_BLOCK_COMMENT", "STRING", "END_BLOCK_COMMENT"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "YangStatementParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public YangStatementParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class StatementContext extends ParserRuleContext {
public KeywordContext keyword() {
return getRuleContext(KeywordContext.class,0);
}
public TerminalNode SEMICOLON() { return getToken(YangStatementParser.SEMICOLON, 0); }
public TerminalNode LEFT_BRACE() { return getToken(YangStatementParser.LEFT_BRACE, 0); }
public TerminalNode RIGHT_BRACE() { return getToken(YangStatementParser.RIGHT_BRACE, 0); }
public List SEP() { return getTokens(YangStatementParser.SEP); }
public TerminalNode SEP(int i) {
return getToken(YangStatementParser.SEP, i);
}
public ArgumentContext argument() {
return getRuleContext(ArgumentContext.class,0);
}
public List statement() {
return getRuleContexts(StatementContext.class);
}
public StatementContext statement(int i) {
return getRuleContext(StatementContext.class,i);
}
public StatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_statement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YangStatementParserListener ) ((YangStatementParserListener)listener).enterStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YangStatementParserListener ) ((YangStatementParserListener)listener).exitStatement(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof YangStatementParserVisitor ) return ((YangStatementParserVisitor extends T>)visitor).visitStatement(this);
else return visitor.visitChildren(this);
}
}
public final StatementContext statement() throws RecognitionException {
StatementContext _localctx = new StatementContext(_ctx, getState());
enterRule(_localctx, 0, RULE_statement);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(9);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SEP) {
{
{
setState(6);
match(SEP);
}
}
setState(11);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(12);
keyword();
setState(16);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,1,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(13);
match(SEP);
}
}
}
setState(18);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,1,_ctx);
}
setState(20);
_la = _input.LA(1);
if (_la==IDENTIFIER || _la==STRING) {
{
setState(19);
argument();
}
}
setState(25);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SEP) {
{
{
setState(22);
match(SEP);
}
}
setState(27);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(55);
switch (_input.LA(1)) {
case SEMICOLON:
{
setState(28);
match(SEMICOLON);
}
break;
case LEFT_BRACE:
{
setState(29);
match(LEFT_BRACE);
setState(33);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(30);
match(SEP);
}
}
}
setState(35);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
}
setState(39);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,5,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(36);
statement();
}
}
}
setState(41);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,5,_ctx);
}
setState(45);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SEP) {
{
{
setState(42);
match(SEP);
}
}
setState(47);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(48);
match(RIGHT_BRACE);
setState(52);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,7,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(49);
match(SEP);
}
}
}
setState(54);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,7,_ctx);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(60);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,9,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(57);
match(SEP);
}
}
}
setState(62);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,9,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class KeywordContext extends ParserRuleContext {
public List IDENTIFIER() { return getTokens(YangStatementParser.IDENTIFIER); }
public TerminalNode IDENTIFIER(int i) {
return getToken(YangStatementParser.IDENTIFIER, i);
}
public TerminalNode COLON() { return getToken(YangStatementParser.COLON, 0); }
public KeywordContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_keyword; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YangStatementParserListener ) ((YangStatementParserListener)listener).enterKeyword(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YangStatementParserListener ) ((YangStatementParserListener)listener).exitKeyword(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof YangStatementParserVisitor ) return ((YangStatementParserVisitor extends T>)visitor).visitKeyword(this);
else return visitor.visitChildren(this);
}
}
public final KeywordContext keyword() throws RecognitionException {
KeywordContext _localctx = new KeywordContext(_ctx, getState());
enterRule(_localctx, 2, RULE_keyword);
try {
enterOuterAlt(_localctx, 1);
{
setState(65);
switch ( getInterpreter().adaptivePredict(_input,10,_ctx) ) {
case 1:
{
setState(63);
match(IDENTIFIER);
setState(64);
match(COLON);
}
break;
}
setState(67);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArgumentContext extends ParserRuleContext {
public List STRING() { return getTokens(YangStatementParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(YangStatementParser.STRING, i);
}
public List PLUS() { return getTokens(YangStatementParser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(YangStatementParser.PLUS, i);
}
public List SEP() { return getTokens(YangStatementParser.SEP); }
public TerminalNode SEP(int i) {
return getToken(YangStatementParser.SEP, i);
}
public TerminalNode IDENTIFIER() { return getToken(YangStatementParser.IDENTIFIER, 0); }
public ArgumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_argument; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YangStatementParserListener ) ((YangStatementParserListener)listener).enterArgument(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YangStatementParserListener ) ((YangStatementParserListener)listener).exitArgument(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof YangStatementParserVisitor ) return ((YangStatementParserVisitor extends T>)visitor).visitArgument(this);
else return visitor.visitChildren(this);
}
}
public final ArgumentContext argument() throws RecognitionException {
ArgumentContext _localctx = new ArgumentContext(_ctx, getState());
enterRule(_localctx, 4, RULE_argument);
int _la;
try {
int _alt;
setState(90);
switch (_input.LA(1)) {
case STRING:
enterOuterAlt(_localctx, 1);
{
setState(69);
match(STRING);
setState(86);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,13,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(73);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SEP) {
{
{
setState(70);
match(SEP);
}
}
setState(75);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(76);
match(PLUS);
setState(80);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SEP) {
{
{
setState(77);
match(SEP);
}
}
setState(82);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(83);
match(STRING);
}
}
}
setState(88);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,13,_ctx);
}
}
break;
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(89);
match(IDENTIFIER);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u0430\ud6d1\u8206\uad2d\u4417\uaef1\u8d80\uaadd\3\r_\4\2\t\2\4\3\t"+
"\3\4\4\t\4\3\2\7\2\n\n\2\f\2\16\2\r\13\2\3\2\3\2\7\2\21\n\2\f\2\16\2\24"+
"\13\2\3\2\5\2\27\n\2\3\2\7\2\32\n\2\f\2\16\2\35\13\2\3\2\3\2\3\2\7\2\""+
"\n\2\f\2\16\2%\13\2\3\2\7\2(\n\2\f\2\16\2+\13\2\3\2\7\2.\n\2\f\2\16\2"+
"\61\13\2\3\2\3\2\7\2\65\n\2\f\2\16\28\13\2\5\2:\n\2\3\2\7\2=\n\2\f\2\16"+
"\2@\13\2\3\3\3\3\5\3D\n\3\3\3\3\3\3\4\3\4\7\4J\n\4\f\4\16\4M\13\4\3\4"+
"\3\4\7\4Q\n\4\f\4\16\4T\13\4\3\4\7\4W\n\4\f\4\16\4Z\13\4\3\4\5\4]\n\4"+
"\3\4\2\2\5\2\4\6\2\2j\2\13\3\2\2\2\4C\3\2\2\2\6\\\3\2\2\2\b\n\7\6\2\2"+
"\t\b\3\2\2\2\n\r\3\2\2\2\13\t\3\2\2\2\13\f\3\2\2\2\f\16\3\2\2\2\r\13\3"+
"\2\2\2\16\22\5\4\3\2\17\21\7\6\2\2\20\17\3\2\2\2\21\24\3\2\2\2\22\20\3"+
"\2\2\2\22\23\3\2\2\2\23\26\3\2\2\2\24\22\3\2\2\2\25\27\5\6\4\2\26\25\3"+
"\2\2\2\26\27\3\2\2\2\27\33\3\2\2\2\30\32\7\6\2\2\31\30\3\2\2\2\32\35\3"+
"\2\2\2\33\31\3\2\2\2\33\34\3\2\2\2\349\3\2\2\2\35\33\3\2\2\2\36:\7\3\2"+
"\2\37#\7\4\2\2 \"\7\6\2\2! \3\2\2\2\"%\3\2\2\2#!\3\2\2\2#$\3\2\2\2$)\3"+
"\2\2\2%#\3\2\2\2&(\5\2\2\2\'&\3\2\2\2(+\3\2\2\2)\'\3\2\2\2)*\3\2\2\2*"+
"/\3\2\2\2+)\3\2\2\2,.\7\6\2\2-,\3\2\2\2.\61\3\2\2\2/-\3\2\2\2/\60\3\2"+
"\2\2\60\62\3\2\2\2\61/\3\2\2\2\62\66\7\5\2\2\63\65\7\6\2\2\64\63\3\2\2"+
"\2\658\3\2\2\2\66\64\3\2\2\2\66\67\3\2\2\2\67:\3\2\2\28\66\3\2\2\29\36"+
"\3\2\2\29\37\3\2\2\2:>\3\2\2\2;=\7\6\2\2<;\3\2\2\2=@\3\2\2\2><\3\2\2\2"+
">?\3\2\2\2?\3\3\2\2\2@>\3\2\2\2AB\7\7\2\2BD\7\b\2\2CA\3\2\2\2CD\3\2\2"+
"\2DE\3\2\2\2EF\7\7\2\2F\5\3\2\2\2GX\7\f\2\2HJ\7\6\2\2IH\3\2\2\2JM\3\2"+
"\2\2KI\3\2\2\2KL\3\2\2\2LN\3\2\2\2MK\3\2\2\2NR\7\t\2\2OQ\7\6\2\2PO\3\2"+
"\2\2QT\3\2\2\2RP\3\2\2\2RS\3\2\2\2SU\3\2\2\2TR\3\2\2\2UW\7\f\2\2VK\3\2"+
"\2\2WZ\3\2\2\2XV\3\2\2\2XY\3\2\2\2Y]\3\2\2\2ZX\3\2\2\2[]\7\7\2\2\\G\3"+
"\2\2\2\\[\3\2\2\2]\7\3\2\2\2\21\13\22\26\33#)/\669>CKRX\\";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy