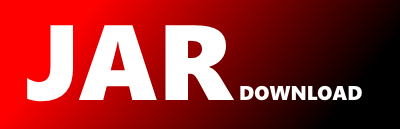
dl2-core.adl-parser.1.3.3.source-code.adlVisitor Maven / Gradle / Ivy
// Generated from adl.g4 by ANTLR 4.5.1
package org.openehr.adl.antlr4.generated;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link adlParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface adlVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link adlParser#adl}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAdl(adlParser.AdlContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#alphanumeric}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAlphanumeric(adlParser.AlphanumericContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypeId}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypeId(adlParser.ArchetypeIdContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#headerTag}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeaderTag(adlParser.HeaderTagContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#header}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeader(adlParser.HeaderContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypePropertyList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypePropertyList(adlParser.ArchetypePropertyListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypeProperty}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypeProperty(adlParser.ArchetypePropertyContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypePropertyValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypePropertyValue(adlParser.ArchetypePropertyValueContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypePropertyValuePart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypePropertyValuePart(adlParser.ArchetypePropertyValuePartContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#atCode}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAtCode(adlParser.AtCodeContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#specializeTag}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSpecializeTag(adlParser.SpecializeTagContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#specialize}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSpecialize(adlParser.SpecializeContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#concept}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConcept(adlParser.ConceptContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#language}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLanguage(adlParser.LanguageContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#description}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDescription(adlParser.DescriptionContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#definition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDefinition(adlParser.DefinitionContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#typeConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeConstraint(adlParser.TypeConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#complexObjectConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComplexObjectConstraint(adlParser.ComplexObjectConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#occurrences}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurrences(adlParser.OccurrencesContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#occurrenceRange}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurrenceRange(adlParser.OccurrenceRangeContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#attributeListMatcher}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAttributeListMatcher(adlParser.AttributeListMatcherContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#orderConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrderConstraint(adlParser.OrderConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#multiValueConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultiValueConstraint(adlParser.MultiValueConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#valueConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueConstraint(adlParser.ValueConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#ordinalConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrdinalConstraint(adlParser.OrdinalConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#ordinalItemList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrdinalItemList(adlParser.OrdinalItemListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#ordinalItem}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrdinalItem(adlParser.OrdinalItemContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#primitiveValueConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrimitiveValueConstraint(adlParser.PrimitiveValueConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#terminologyCodeConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTerminologyCodeConstraint(adlParser.TerminologyCodeConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypeSlotConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypeSlotConstraint(adlParser.ArchetypeSlotConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypeSlotValueConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypeSlotValueConstraint(adlParser.ArchetypeSlotValueConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypeSlotSingleConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypeSlotSingleConstraint(adlParser.ArchetypeSlotSingleConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#archetypeReferenceConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArchetypeReferenceConstraint(adlParser.ArchetypeReferenceConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#stringConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringConstraint(adlParser.StringConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#numberConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumberConstraint(adlParser.NumberConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#dateTimeConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTimeConstraint(adlParser.DateTimeConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#dateConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateConstraint(adlParser.DateConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#timeConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeConstraint(adlParser.TimeConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#durationConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDurationConstraint(adlParser.DurationConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#codeIdentifierList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCodeIdentifierList(adlParser.CodeIdentifierListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#regularExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRegularExpression(adlParser.RegularExpressionContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#regularExpressionInner1}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRegularExpressionInner1(adlParser.RegularExpressionInner1Context ctx);
/**
* Visit a parse tree produced by {@link adlParser#regularExpressionInner2}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRegularExpressionInner2(adlParser.RegularExpressionInner2Context ctx);
/**
* Visit a parse tree produced by {@link adlParser#attributeConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAttributeConstraint(adlParser.AttributeConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#tupleAttributeIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleAttributeIdentifier(adlParser.TupleAttributeIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#tupleChildConstraints}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleChildConstraints(adlParser.TupleChildConstraintsContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#tupleChildConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleChildConstraint(adlParser.TupleChildConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#attributeIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAttributeIdentifier(adlParser.AttributeIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#existence}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExistence(adlParser.ExistenceContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#cardinality}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCardinality(adlParser.CardinalityContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#numberIntervalConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumberIntervalConstraint(adlParser.NumberIntervalConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#dateIntervalConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateIntervalConstraint(adlParser.DateIntervalConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#timeIntervalConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeIntervalConstraint(adlParser.TimeIntervalConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#dateTimeIntervalConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTimeIntervalConstraint(adlParser.DateTimeIntervalConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#durationIntervalConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDurationIntervalConstraint(adlParser.DurationIntervalConstraintContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#terminology}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTerminology(adlParser.TerminologyContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#annotations}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAnnotations(adlParser.AnnotationsContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinValue(adlParser.OdinValueContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinObjectValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinObjectValue(adlParser.OdinObjectValueContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinObjectProperty}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinObjectProperty(adlParser.OdinObjectPropertyContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinCodePhraseValueList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinCodePhraseValueList(adlParser.OdinCodePhraseValueListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinCodePhraseValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinCodePhraseValue(adlParser.OdinCodePhraseValueContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinMapValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinMapValue(adlParser.OdinMapValueContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#odinMapValueEntry}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOdinMapValueEntry(adlParser.OdinMapValueEntryContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#openStringList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOpenStringList(adlParser.OpenStringListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#stringList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringList(adlParser.StringListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#numberList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumberList(adlParser.NumberListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#booleanList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanList(adlParser.BooleanListContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#codeIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCodeIdentifier(adlParser.CodeIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#codeIdentifierPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCodeIdentifierPart(adlParser.CodeIdentifierPartContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#identifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdentifier(adlParser.IdentifierContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#bool}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBool(adlParser.BoolContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#nameIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNameIdentifier(adlParser.NameIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#typeIdentifierWithGenerics}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeIdentifierWithGenerics(adlParser.TypeIdentifierWithGenericsContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#typeIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeIdentifier(adlParser.TypeIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#keyword}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKeyword(adlParser.KeywordContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#pathSegment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathSegment(adlParser.PathSegmentContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#rmPath}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRmPath(adlParser.RmPathContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#url}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUrl(adlParser.UrlContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#numberOrStar}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumberOrStar(adlParser.NumberOrStarContext ctx);
/**
* Visit a parse tree produced by {@link adlParser#number}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumber(adlParser.NumberContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy