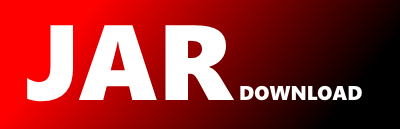
client.js.otp.modules.planner.ItinerariesWidget.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
/* This program is free software: you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public License
as published by the Free Software Foundation, either version 3 of
the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see .
*/
otp.namespace("otp.widgets");
otp.widgets.ItinerariesWidget =
otp.Class(otp.widgets.Widget, {
module : null,
itinsAccord : null,
footer : null,
itineraries : null,
activeIndex : 0,
previousPageCursor: null,
nextPageCursor: null,
// set to true by next/previous/etc. to indicate to only refresh the currently active itinerary
refreshActiveOnly : false,
showButtonRow : true,
showItineraryLink : true,
showPrintLink : true,
showEmailLink : true,
showSearchLink : false,
initialize : function(id, module) {
this.module = module;
otp.widgets.Widget.prototype.initialize.call(this, id, module, {
//TRANSLATORS: Widget title
title : _tr("Itineraries"),
cssClass : module.itinerariesWidgetCssClass || 'otp-defaultItinsWidget',
resizable : true,
closeable : true,
persistOnClose : true
});
//this.$().addClass('otp-itinsWidget');
//this.$().resizable();
//this.minimizable = true;
//this.addHeader("X Itineraries Returned");
},
activeItin : function() {
return this.itineraries[this.activeIndex];
},
updatePlan : function(plan) {
this.updateItineraries(
plan.itineraries,
plan.queryParams,
undefined,
plan.previousPageCursor,
plan.nextPageCursor
);
},
updateItineraries : function(
itineraries,
queryParams,
itinIndex,
previousPageCursor,
nextPageCursor
) {
var this_ = this;
var divId = this.id+"-itinsAccord";
if(this.minimized) this.unminimize();
if(this.refreshActiveOnly == true) { // if only refreshing one itinerary; e.g. if next/prev was used
// swap the old itinerary for the new one in both the TripPlan object and the local array
var newItin = itineraries[0];
var oldItin = this.itineraries[this.activeIndex];
oldItin.tripPlan.replaceItinerary(this.activeIndex, newItin);
this.itineraries[this.activeIndex] = newItin;
// create an alert if we moved to another day
var alerts = null;
if(newItin.differentServiceDayFrom(oldItin)) {
alerts = [ _tr("This itinerary departs on a different day from the previous one") ];
}
// refresh all itinerary headers
this.renderHeaders();
// refresh the main itinerary content
var itinContainer = $('#'+divId+'-'+this.activeIndex);
itinContainer.empty();
this.renderItinerary(newItin, this.activeIndex, alerts).appendTo(itinContainer);
this.refreshActiveOnly = false;
return;
}
this.itineraries = itineraries;
this.previousPageCursor = previousPageCursor;
this.nextPageCursor = nextPageCursor;
this.clear();
//TRANSLATORS: widget title
this.setTitle(ngettext("%d Itinerary Returned", "%d Itineraries Returned", this.itineraries.length));
var html = "";
this.itinsAccord = $(html).appendTo(this.$());
this.footer = $('').appendTo(this.$());
if(this.showButtonRow && queryParams.mode !== "WALK" && queryParams.mode !== "BICYCLE") {
this.renderButtonRow();
}
if(this.showSearchLink) {
var link = this.constructLink(queryParams,
jQuery.isFunction(this.module.getAdditionalUrlParams) ?
this.module.getAdditionalUrlParams() : null);
//TODO: Where does this link?
$('').appendTo(this.footer);
}
for(var i=0; i'+this.headerContent(itin, i)+'').appendTo(this.itinsAccord).click(function(evt) {
//$(''+this.headerContent(itin, i)+'
').appendTo(this.itinsAccord).click(function(evt) {
var headerDivId = divId+'-headerContent-'+i;
var itinSyle = "";
if(itin.itinData.systemNotices != null) {
itinSyle = "class=\"sysNotice\"";
}
$('
')
.appendTo(this.itinsAccord)
.data('itin', itin)
.data('index', i)
.click(function(evt) {
var itin = $(this).data('itin');
this_.module.drawItinerary(itin);
this_.activeIndex = $(this).data('index');
// Wait for the SVG element to be resized, before re-rendering
setTimeout(function () {
this_.renderElevationGraphContent(itin, this_.activeIndex);
}, 0);
});
$('')
.appendTo(this.itinsAccord)
.append(this.renderItinerary(itin, i));
}
this.activeIndex = parseInt(itinIndex) || 0;
this.itinsAccord.accordion({
active: this.activeIndex,
heightStyle: "fill"
});
// headers must be rendered after accordion is laid out to work around chrome layout bug
/*for(var i=0; i
'+(index+1)+'.
';
/*
// show iconographic trip leg summary
html += ' ';
// show trip duration
html += '('+itin.getDurationStr()+')
';
if(itin.groupSize) {
html += '[Group size: '+itin.groupSize+']
';
} */
var div = $('');
div.append(''+(index+1)+'
');
console.log("header div width: "+div.width());
// clear div
//html += '';
return div;
},
renderHeaders : function() {
for(var i=0; i