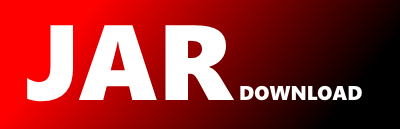
client.js.otp.modules.planner.Itinerary.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
/* This program is free software: you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public License
as published by the Free Software Foundation, either version 3 of
the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see .
*/
otp.namespace("otp.modules.planner");
otp.modules.planner.Itinerary = otp.Class({
itinData : null,
tripPlan : null,
firstStopIDs : null,
hasTransit : false,
totalWalk : 0,
initialize : function(itinData, tripPlan) {
this.itinData = itinData;
this.tripPlan = tripPlan;
this.firstStopIDs = [ ];
for(var l=0; l ';
if(i < this.itinData.legs.length-1)
html += '
';
}
return html;
},
getStartTime : function() {
return this.itinData.legs[0].startTime;
},
getEndTime : function() {
return this.itinData.legs[this.itinData.legs.length-1].endTime;
},
getStartTimeStr : function() {
return otp.util.Time.formatItinTime(this.getStartTime());
},
getEndTimeStr : function() {
return otp.util.Time.formatItinTime(this.getEndTime());
},
getStartLocationStr : function() {
var from = this.itinData.legs[0].from;
return from.name || "(" + from.lat.toFixed(5) + ", " + from.lon.toFixed(5) + ")";
},
getEndLocationStr : function() {
var to = this.itinData.legs[this.itinData.legs.length-1].to;
return to.name || "(" + to.lat.toFixed(5) + ", " + to.lon.toFixed(5)+ ")";
},
getDurationStr : function() {
// even though the API communicates in seconds and timestamps, the timestamps are converted to
// epoch milliseconds for internal representation.
return otp.util.Time.secsToHrMin( (this.getEndTime() - this.getStartTime())/1000.0 );
},
getGeneralizedCost : function() {
return this.itinData.generalizedCost;
},
buildFaresTable: function (products) {
const table = document.createElement("table");
table.classList.add("fares")
products.forEach(p => {
const tr = document.createElement("tr");
table.appendChild(tr);
const nameCell = document.createElement("td");
nameCell.classList.add("name");
nameCell.innerText = p.name || "";
const catCell = document.createElement("td");
if(p.category) {
catCell.innerText = p.category.name || "";
}
const containerCell = document.createElement("td");
if(p.container){
containerCell.innerText = p.container.name || "";
}
tr.appendChild(nameCell);
tr.appendChild(catCell);
tr.appendChild(containerCell);
const decimalPlaces = p.amount.currency.defaultFractionDigits;
const amount = (p.amount.cents / Math.pow(10, decimalPlaces)).toFixed(decimalPlaces);
const price = new Intl.NumberFormat(otp.config.locale.config.locale_short,
{
style: 'currency',
currency: p.amount.currency.currencyCode,
maximumFractionDigits: p.amount.currency.defaultFractionDigits,
}
).format(amount);
const priceCell = document.createElement("td");
priceCell.textContent = price;
tr.appendChild(priceCell);
table.appendChild(tr);
});
return table;
},
formatFaresV2: function (fare, legs) {
const allFares = document.createElement("div");
if (fare.coveringItinerary && fare.coveringItinerary.length > 0) {
const title = document.createElement("strong");
title.innerText = "Covering entire itinerary";
allFares.appendChild(title);
allFares.appendChild(this.buildFaresTable(this.itinData.fare.coveringItinerary));
}
if (fare.legProducts) {
fare.legProducts.forEach(legProducts => {
const name = legProducts.legIndices.map(index => `${legs[index].agencyName} ${legs[index].routeLongName}`).join(",");
const title = document.createElement("strong");
title.innerText = `Covering ${name}`;
allFares.appendChild(title);
allFares.appendChild(this.buildFaresTable(legProducts.products));
})
}
return allFares.outerHTML;
},
getFareStr : function() {
if(this.fareDisplayOverride) return this.fareDisplayOverride;
if(otp.config.fareDisplayOverride) return otp.config.fareDisplayOverride;
const fare = this.itinData.fare;
if(fare && (fare.coveringItinerary || fare.legProducts)) {
return this.formatFaresV2(fare, this.itinData.legs);
} else if(this.itinData.fare && this.itinData.fare.fare.regular) {
var decimalPlaces = this.itinData.fare.fare.regular.currency.defaultFractionDigits;
var fare_info = {
'currency': this.itinData.fare.fare.regular.currency.symbol,
'price': (this.itinData.fare.fare.regular.cents/Math.pow(10,decimalPlaces)).toFixed(decimalPlaces),
}
//TRANSLATORS: Fare Currency Fare price
return _tr('%(currency)s %(price)s', fare_info);
}
return "N/A";
},
differentServiceDayFrom : function(itin, offsetHrs) {
offsetHrs = offsetHrs || 3; // default to 3 hrs; i.e. use 3am as breakpoint between days
var time1 = moment(this.itinData.startTime).add("hours", otp.config.timeOffset-offsetHrs).format('D');
var time2 = moment(itin.itinData.startTime).add("hours", otp.config.timeOffset-offsetHrs).format('D');
return time1 !== time2;
},
differentServiceDayFromQuery : function(queryTime, offsetHrs) {
offsetHrs = offsetHrs || 3; // default to 3 hrs; i.e. use 3am as breakpoint between days
var time1 = moment(this.itinData.startTime).add("hours", otp.config.timeOffset-offsetHrs).format('D');
var time2 = moment(queryTime).add("hours", otp.config.timeOffset-offsetHrs).format('D');
return time1 !== time2;
},
/*getTransitSegments : function() {
var segments = [];
for(var l=0; l ';
html += '';
//TRANSLATORS: Start: location at [time date] (Used in print itinerary
//when do you start your trip)
html += '' + _tr('Start: %(location)s at %(time_date)s', { 'location': this.getStartLocationStr(), 'time_date': this.getStartTimeStr()}) + '
';
for(var l=0; l'+(l+1)+'. '+otp.util.Itin.modeString(leg.mode).toUpperCase();//
if(otp.util.Itin.isTransit(leg.mode)) {
html += ': ';
if(leg.route !== leg.routeLongName) html += "("+leg.route+") ";
html += leg.routeLongName;
if(leg.headsign) html += pgettext("bus_direction", " to ") + leg.headsign;
}
else { // walk / bike / car
//TRANSLATORS: [distance] to [name of destination]
html += " "+otp.util.Itin.distanceString(leg.distance)+ pgettext("direction", " to ")+otp.util.Itin.getName(leg.to);
}
html += ''
// main content
if(otp.util.Itin.isTransit(leg.mode)) { // transit
var fromStopIdParts = leg.from.stopId.split(':');
var fromAgencyId = fromStopIdParts.shift();
var fromStopId = fromStopIdParts.join(':');
var toStopIdParts = leg.to.stopId.split(':');
var toAgencyId = toStopIdParts.shift();
var toStopId = toStopIdParts.join(':');
html += '';
//TRANSLATORS: Board Public transit route name (agency name
//Stop ID ) start time
html += '- ' + _tr('Board') + ': ' + leg.from.name + ' ' + _tr("(%(agency_id)s Stop ID #%(stop_id)s),", {'agency_id': fromAgencyId, 'stop_id': fromStopId }) + ' ' + otp.util.Time.formatItinTime(leg.startTime, otp.config.locale.time.time_format) + '
';
html += '- ' + _tr('Time in transit') +': '+otp.util.Time.secsToHrMin(leg.duration)+'
';
//TRANSLATORS: Alight Public transit route name (agency name
//Stop ID ) end time
html += '- ' + _tr('Alight') + ': ' + leg.to.name + ' ' + _tr("(%(agency_id)s Stop ID #%(stop_id)s),", {'agency_id': toAgencyId, 'stop_id': toStopId }) + ' ' + otp.util.Time.formatItinTime(leg.endTime, otp.config.locale.time.time_format) + '
';
html += '
';
}
else if (leg.steps) { // walk / bike / car
for(var i=0; i';
html += '' +
// '' +
// distArr[0]+'
'+distArr[1]+'';
html += ''+text+'
'+dist+'';
html += ' ';
var dist = otp.util.Itin.distanceString(step.distance);
//html += ' ';
}
}
}
//TRANSLATORS: End: location at [time date] (Used in print itinerary
//when do you come at a destination)
html += '' + _tr('End: %(location)s at %(time_date)s', { 'location': this.getEndLocationStr(), 'time_date': this.getEndTimeStr()} )+'
';
// trip summary
html += '';
html += '' + _tr('Trip Summary') +'';
html += '' + _tr('Travel') + ''+this.getStartTimeStr()+'';
html += '' + _tr('Time') + ''+this.getDurationStr()+'';
if(this.hasTransit) {
html += '' + _tr('Transfers') + ''+this.itinData.transfers+'';
if(this.itinData.walkDistance > 0) {
html += '' + _tr('Total Walk') + '' +
otp.util.Itin.distanceString(this.itinData.walkDistance) + '';
}
html += '' + _tr('Fare') + ''+this.getFareStr()+'';
}
html += '';
html += '