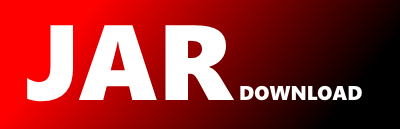
prompto.intrinsic.Utils.js Maven / Gradle / Ivy
The newest version!
function isABoolean(o) {
return typeof(o) === "boolean";
}
function isAnInteger(o) {
return typeof(o) === "number" && o === Math.floor(o);
}
function isADecimal(o) {
return typeof(o) === "number" && o !== Math.floor(o);
}
function isANumber(o) {
return typeof(o) === "number";
}
function isAText(o) {
return typeof(o) === 'string' || o instanceof String;
}
function isAMethod(o, params, result) {
if(typeof o !== 'function')
return false;
function countParams(o) {
var str = o.toString();
str = str.replace(/\/\*[\s\S]*?\*\//g, '')
.replace(/\/\/(.)*/g, '')
.replace(/{[\s\S]*}/, '')
.replace(/=>/g, '')
.trim();
var lpar = str.indexOf("(");
var rpar = str.indexOf(")", lpar + 1);
str = str.substring( lpar + 1, rpar);
var args = str.split(",");
args = args.filter(function(s) { return s.trim().length > 0; });
return args.length;
}
return params.length === countParams(o);
}
function isAnEnum(o) {
if(typeof o !== 'object')
return false;
var proto = Object.getPrototypeOf(o);
return proto && proto.constructor && proto.constructor.symbolOf && proto.constructor.symbols;
}
function isInstanceOf(obj, type) {
return obj instanceof type || (obj instanceof $Root && obj.$categories.indexOf(type)>=0);
}
function StringOrNull(o) {
return o && o.toString ? o.toString() : null;
}
function ArrayOrNull(o) {
return o && o.toArray ? o.toArray() : null;
}
if(!Object.setPrototypeOf)
Object.setPrototypeOf = function(obj, proto) { obj.__proto__ = proto; };
if(!Object.getPrototypeOf)
Object.getPrototypeOf = function(obj) { return obj.__proto__; };
function equalObjects(o1, o2) {
if(Object.is(o1, o2))
return true;
else
return typeof(o1)==='object' && o1.equals && o1.equals(o2);
}
function equalArrays(o1, o2) {
o1 = o1 || null;
o2 = o2 || null;
if(o1===o2) {
return true;
}
if(o1===null || o2===null) {
return false;
}
if(o1.length !== o2.length) {
return false;
}
for(var i=0;i=0) {
// fix IEEE issue
i = s.indexOf('000000', i);
if( i < 0)
return s;
else
return s.substr(0, i);
} else
return s + ".0";
};
Number.prototype.getText = Number.prototype.toString;
Number.prototype.toJson = function() { return JSON.stringify(this); };
Number.prototype.equals = function(value) {
return this == value;
};
String.prototype.hasAll = function(items) {
if(StrictSet && items instanceof StrictSet)
items = Array.from(items.set.values());
for(var i=0;i this.length)
throw new RangeError();
start = start - 1;
} else
start = 0;
if(!last)
return this.substring(start);
if(last >= 0) {
if(last<1 || last>this.length)
throw new RangeError();
return this.substring(start, last);
} else {
if(last<-this.length)
throw new RangeError();
return this.substring(start, this.length + 1 + last)
}
};
String.prototype.getText = String.prototype.toString;
String.prototype.toJson = function() { return JSON.stringify(this); };
String.prototype.indexOf1Based = function(value, fromIndex) {
return 1 + this.indexOf(value, fromIndex - 1);
};
String.prototype.equals = function(value) {
// use == because === fails in Nashorn
return this == value;
};
String.prototype.iterator = function() {
var s = this;
return {
idx: 0,
hasNext: function() { return this.idx < s.length; },
next: function() { return s[this.idx++]; }
};
};
var intrinsic = {};
if(typeof(window)==="object" && window.document) {
window.document.setMember = function(name, value) {
window.document[name] = value;
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy