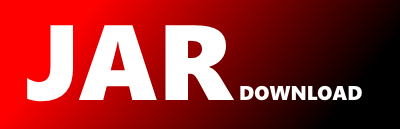
prompto.reader.CSVReader.js Maven / Gradle / Ivy
/* we can't use Papa or other js csv libs, because they either load everything in memory or require a continuation */
/* ideally we would use generators, but support with browsers is poor so far */
/* so we use our own stuff until ES6 becomes widespread */
function csvIterate(text, columns, separator, encloser) {
return new CSVIterator(text, columns, separator, encloser);
}
function csvRead(text, columns, separator, encloser) {
var list = new intrinsic.List();
var iter = new CSVIterator(text, columns, separator, encloser);
while(iter.hasNext())
list.push(iter.next());
return list;
}
function csvReadHeaders(text, separator, encloser) {
var iter = new CSVIterator(text, null, separator, encloser);
var headers = iter.readHeaders();
return new intrinsic.List(false, headers);
}
function CSVIterator(text, columns, separator, encloser) {
this.text = text || null;
this.index = 0;
this.columns = columns;
this.separator = (separator || ',').charCodeAt(0);
this.encloser = (encloser || '"').charCodeAt(0);
this.headers = null;
this.peekedChar = null;
this.nextChar = 0;
return this;
}
var DQ = '"'.charCodeAt(0);
var CR = '\r'.charCodeAt(0);
var LF = '\n'.charCodeAt(0);
var ESC = '\\'.charCodeAt(0);
CSVIterator.prototype.iterator = function() {
return this;
};
CSVIterator.prototype.readHeaders = function() {
if(this.nextChar==0)
this.fetchChar(true);
return this.parseHeaders();
};
CSVIterator.prototype.hasNext = function() {
if(this.nextChar==0)
this.fetchChar(true);
if(this.headers==null)
this.headers = this.parseHeaders();
return this.nextChar>0;
};
CSVIterator.prototype.next = function() {
if(!this.hasNext()) // will parse headers
return null;
var values = this.parseLine();
var doc = new intrinsic.Document();
for(var i=0;i0) {
chars = chars.map(function(c) {
return String.fromCharCode(c);
});
list.push(chars.join(""));
}
return;
}
}
};
CSVIterator.prototype.handleOtherChar = function(chars, _endChar, _list) {
chars.push(this.nextChar);
this.fetchChar();
return false;
};
CSVIterator.prototype.handleEscape = function(chars, _endChar, _list) {
if(this.peekChar()!=-1) {
chars.push(this.peekChar());
this.fetchChar();
}
this.fetchChar();
return false;
};
CSVIterator.prototype.handleEOF = function(_chars, _endChar, _list) {
return true;
}
CSVIterator.prototype.handleEndChar = function(chars, endChar, _list) {
if(endChar==DQ && this.peekChar()==endChar) {
chars.push(this.nextChar);
this.fetchChar();
this.fetchChar();
return false;
} else {
this.fetchChar();
return true;
}
};
CSVIterator.prototype.handleNewLine = function(chars, endChar, _list) {
if(endChar==DQ) {
chars.push(this.nextChar);
this.fetchChar();
return false;
} else {
return true;
}
}
exports.csvIterate = csvIterate;
exports.csvRead = csvRead;
exports.csvReadHeaders = csvReadHeaders;
© 2015 - 2024 Weber Informatics LLC | Privacy Policy