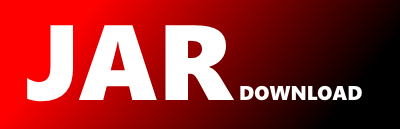
structr.js.widgets.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structr-ui Show documentation
Show all versions of structr-ui Show documentation
Structr is an open source framework based on the popular Neo4j graph database.
The newest version!
/*
* Copyright (C) 2010-2016 Structr GmbH
*
* This file is part of Structr .
*
* Structr is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* Structr is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with Structr. If not, see .
*/
var widgets, remoteWidgets, widgetsUrl = 'https://widgets.structr.org/structr/rest/widgets';
var win = $(window);
var widgetData = [], remoteWidgetData = [], remoteWidgetFilter;
$(document).ready(function() {
Structr.registerModule('widgets', _Widgets);
Structr.classes.push('widget');
});
var _Widgets = {
icon: 'icon/layout.png',
group_icon: 'icon/folder.png',
add_widget_icon: 'icon/layout_add.png',
delete_widget_icon: 'icon/layout_delete.png',
init: function() {
_Pager.initPager('widgets-widgets', 'Widget', 1, 25);
},
onload: function() {
_Widgets.init();
$('#main-help a').attr('href', 'http://docs.structr.org/frontend-user-guide#Widgets');
_Logger.log(_LogType.WIDGETS, 'onload');
main.append('Local Widgets
Remote Widgets
');
widgets = $('#widgets-content');
remoteWidgets = $('#remoteWidgets-content');
$('#remoteWidgetsFilter').keyup(function (event) {
var e = event || window.event();
if (e.keyCode === 27) {
$(this).val("");
}
_Widgets.repaintRemoteWidgets($(this).val());
});
_Widgets.refreshWidgets();
_Widgets.refreshRemoteWidgets();
Structr.resize();
win.off('resize');
win.resize(function() {
Structr.resize();
});
Structr.unblockMenu(100);
},
unload: function() {
$(main.children('table')).remove();
},
refreshWidgets: function() {
widgets.empty();
widgets.append('');
$('.add_widgets_icon', main).on('click', function(e) {
e.stopPropagation();
Command.create({'type': 'Widget'});
});
var wPager = _Pager.addPager('widgets-widgets', widgets, true, 'Widget', 'public');
wPager.cleanupFunction = function() {
$('.node', wPager.el).remove();
};
Structr.resize();
},
refreshRemoteWidgets: function() {
remoteWidgetFilter = undefined;
if (widgetsUrl.startsWith(document.location.hostname)) {
return;
}
_Widgets.getRemoteWidgets(widgetsUrl, function(entity) {
var obj = StructrModel.create(entity, null, false);
obj.srcUrl = widgetsUrl + '/' + entity.id;
remoteWidgetData.push(obj);
}, function () {
_Widgets.repaintRemoteWidgets('');
});
// remoteWidgets.append('');
// $('#connect_button', main).on('click', function(e) {
// e.stopPropagation();
// });
},
repaintRemoteWidgets: function (search) {
if (search !== remoteWidgetFilter) {
remoteWidgetFilter = search;
if (search && search.length > 0) {
search = search.toLowerCase();
remoteWidgets.empty();
remoteWidgetData.forEach(function (obj) {
if (obj.name.toLowerCase().indexOf(search) !== -1) {
_Widgets.appendWidgetElement(obj, true, remoteWidgets);
}
});
} else {
remoteWidgets.empty();
remoteWidgetData.forEach(function (obj) {
_Widgets.appendWidgetElement(obj, true, remoteWidgets);
});
}
}
},
getRemoteWidgets: function(baseUrl, callback, finishCallback) {
$.ajax({
//url: $('#widgetServerUrl').val(),
url: baseUrl + '?sort=treePath',
type: 'GET',
dataType: 'json',
contentType: 'application/json; charset=utf-8',
//async: false,
statusCode: {
200: function(data) {
if (callback) {
$.each(data.result, function(i, entity) {
callback(entity);
});
if (finishCallback) {
finishCallback();
}
}
},
400: function(data, status, xhr) {
console.log(data, status, xhr);
},
401: function(data, status, xhr) {
console.log(data, status, xhr);
},
403: function(data, status, xhr) {
console.log(data, status, xhr);
},
404: function(data, status, xhr) {
console.log(data, status, xhr);
},
422: function(data, status, xhr) {
console.log(data, status, xhr);
},
500: function(data, status, xhr) {
console.log(data, status, xhr);
}
}
});
},
getIcon: function() {
var icon = _Widgets.icon; // default
return icon;
},
getTreeParent: function(element, treePath, suffix) {
var parent = element;
if (treePath) {
var parts = treePath.split('/');
var num = parts.length;
var i = 0;
for (i = 0; i < num; i++) {
var part = parts[i];
if (part) {
var lowerPart = part.toLowerCase().replace(/ /g, '');
var idString = lowerPart + suffix;
var newParent = $('#' + idString);
if (newParent.size() === 0) {
_Widgets.appendFolderElement(parent, idString, _Widgets.group_icon, part);
// parent.append(' ');
newParent = $('#' + idString);
}
parent = newParent;
}
}
} else {
var idString = 'other' + suffix;
var newParent = $('#' + idString);
if (newParent.size() === 0) {
_Widgets.appendFolderElement(parent, idString, _Widgets.group_icon, 'Uncategorized');
//parent.append(' ');
newParent = $('#' + idString);
}
parent = newParent;
}
return parent;
},
appendFolderElement: function(parent, id, icon, name) {
var expanded = isExpanded(id);
parent.append(' ');
var div = $('#' + id + '_folder');
_Widgets.appendVisualExpandIcon(div, id, name, true, false);
},
appendWidgetElement: function(widget, remote, el) {
_Logger.log(_LogType.WIDGETS, 'Widgets.appendWidgetElement', widget, remote);
var icon = _Widgets.getIcon(widget);
var parent = _Widgets.getTreeParent(el ? el : (remote ? remoteWidgets : widgets), widget.treePath, remote ? '_remote' : '_local');
var delIcon, newDelIcon;
var div = Structr.node(widget.id);
if (div && div.length) {
var formerParent = div.parent();
if (!Structr.containsNodes(formerParent)) {
_Entities.removeExpandIcon(formerParent);
enable($('.delete_icon', formerParent)[0]);
}
} else {
parent.append(' ');
div = Structr.node(widget.id);
var typeIcon = $('.typeIcon', div);
typeIcon.on('click', function() {
Structr.dialog('Source code of ' + widget.name, function() {}, function() {});
var text = widget.source || '';
var div = dialogText.append('');
_Logger.log(_LogType.WIDGETS, div);
var contentBox = $('.editor', dialogText);
editor = CodeMirror(contentBox.get(0), {
value: unescapeTags(text),
mode: 'text/html',
lineNumbers: true,
indentUnit: 4,
tabSize:4,
indentWithTabs: true
});
editor.focus();
Structr.resize();
});
}
if (!div)
return;
if (!remote) {
_Entities.appendAccessControlIcon(div, widget);
delIcon = div.children('.delete_icon');
newDelIcon = '
';
div.append(newDelIcon);
delIcon = div.children('.delete_icon');
div.children('.delete_icon').on('click', function(e) {
e.stopPropagation();
_Entities.deleteNode(this, widget);
});
}
div.draggable({
iframeFix: true,
revert: 'invalid',
containment: 'body',
helper: 'clone',
appendTo: '#main',
stack: '.node',
zIndex: 99,
stop: function(e, ui) {
//$('#pages_').droppable('enable').removeClass('nodeHover');
}
});
if (!remote) {
div.append('
');
$('.edit_icon', div).on('click', function(e) {
e.stopPropagation();
Structr.dialog('Edit widget "' + widget.name + '"', function() {
_Logger.log(_LogType.WIDGETS, 'Widget source saved');
}, function() {
_Logger.log(_LogType.WIDGETS, 'cancelled');
});
if (!widget.id) {
return false;
}
Command.get(widget.id, function(entity) {
_Widgets.editWidget(this, entity, dialogText);
});
});
_Entities.appendEditPropertiesIcon(div, widget);
}
_Entities.setMouseOver(div, false);
if (remote) {
div.children('b.name_').off('click').css({cursor: 'move'});
}
// div.append('');
// //$('.preview', div).contents().find('body').html('' + widget.name + ' ' + widget.source + '');
// widget.pictures.forEach(function(pic) {
// $('.preview', div).append('
');
// });
return div;
},
editWidget: function(button, entity, element) {
if (isDisabled(button))
return;
var text = entity.source || '';
var div = element.append('');
_Logger.log(_LogType.WIDGETS, div);
var contentBox = $('.editor', element);
editor = CodeMirror(contentBox.get(0), {
value: unescapeTags(text),
mode: 'text/html',
lineNumbers: true,
indentUnit: 4,
tabSize:4,
indentWithTabs: true
});
editor.focus();
Structr.resize();
dialogBtn.append('');
dialogBtn.append('');
dialogSaveButton = $('#editorSave', dialogBtn);
var saveAndClose = $('#saveAndClose', dialogBtn);
text1 = text;
editor.on('change', function(cm, change) {
text2 = editor.getValue();
if (text1 === text2) {
dialogSaveButton.prop("disabled", true).addClass('disabled');
saveAndClose.prop("disabled", true).addClass('disabled');
} else {
dialogSaveButton.prop("disabled", false).removeClass('disabled');
saveAndClose.prop("disabled", false).removeClass('disabled');
}
});
saveAndClose.on('click', function(e) {
e.stopPropagation();
dialogSaveButton.click();
setTimeout(function() {
dialogSaveButton.remove();
saveAndClose.remove();
dialogCancelButton.click();
}, 500);
});
dialogSaveButton.on('click', function() {
var newText = editor.getValue();
if (text1 === newText) {
return;
}
if (entity.srcUrl) {
var data = JSON.stringify({'source': newText});
_Logger.log(_LogType.WIDGETS, 'update remote widget', entity.srcUrl, data);
$.ajax({
//url: $('#widgetServerUrl').val(),
url: entity.srcUrl,
type: 'PUT',
dataType: 'json',
data: data,
contentType: 'application/json; charset=utf-8',
//async: false,
statusCode: {
200: function(data) {
dialogMsg.html('Widget source saved.');
$('.infoBox', dialogMsg).delay(2000).fadeOut(200);
text1 = newText;
dialogSaveButton.prop("disabled", true).addClass('disabled');
saveAndClose.prop("disabled", true).addClass('disabled');
},
400: function(data, status, xhr) {
console.log(data, status, xhr);
},
401: function(data, status, xhr) {
console.log(data, status, xhr);
},
403: function(data, status, xhr) {
console.log(data, status, xhr);
},
404: function(data, status, xhr) {
console.log(data, status, xhr);
},
422: function(data, status, xhr) {
console.log(data, status, xhr);
},
500: function(data, status, xhr) {
console.log(data, status, xhr);
}
}
});
} else {
Command.setProperty(entity.id, 'source', newText, false, function() {
dialogMsg.html('Widget saved.');
$('.infoBox', dialogMsg).delay(2000).fadeOut(200);
text1 = newText;
dialogSaveButton.prop("disabled", true).addClass('disabled');
saveAndClose.prop("disabled", true).addClass('disabled');
});
}
});
editor.id = entity.id;
},
appendVisualExpandIcon: function(el, id, name, hasChildren, expand) {
if (hasChildren) {
_Logger.log(_LogType.WIDGETS, 'appendExpandIcon hasChildren?', hasChildren, 'expand?', expand);
var typeIcon = $(el.children('.typeIcon').first());
var icon = $(el).children('.node').hasClass('hidden') ? Structr.expand_icon : Structr.expanded_icon;
typeIcon.css({
paddingRight: 0 + 'px'
}).after('
');
var expandIcon = el.children('.expand_icon').first();
$(el).on('click', function(e) {
e.stopPropagation();
var body = $('#' + id);
body.toggleClass('hidden');
var expanded = body.hasClass('hidden');
if (expanded) {
addExpandedNode(id);
expandIcon.prop('src', 'icon/tree_arrow_right.png');
} else {
removeExpandedNode(id);
expandIcon.prop('src', 'icon/tree_arrow_down.png');
}
});
button = $(el.children('.expand_icon').first());
if (button) {
button.on('click', function(e) {
e.stopPropagation();
var body = $('#' + id);
body.toggleClass('hidden');
var expanded = body.hasClass('hidden');
if (expanded) {
addExpandedNode(id);
expandIcon.prop('src', 'icon/tree_arrow_right.png');
} else {
removeExpandedNode(id);
expandIcon.prop('src', 'icon/tree_arrow_down.png');
}
});
// Prevent expand icon from being draggable
button.on('mousedown', function(e) {
e.stopPropagation();
});
if (expand) {
}
}
} else {
el.children('.typeIcon').css({
paddingRight: 11 + 'px'
});
}
}
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy