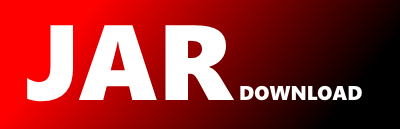
META-INF.resources.js.trip.min.js Maven / Gradle / Ivy
/**
* Trip.js
*
* This is a jQuery plugin that can help you customize your tutorial trip
* with full flexibilities.
*
* Version: 3.0.0
*
* Author: EragonJ
* Blog: http://eragonj.me
*
* @preserve
*/
!function(a){function b(){this._DEFAULT_TRIP_NODES_SELECTOR="[data-trip]",this._DEFAULT_TRIP_POSITION="n",this._DEFAULT_TRIP_ANIMATION="tada"}b.prototype={_getAllTripNodes:function(a){return document.querySelectorAll(a)},_parseTripData:function(a){{var b=a.dataset.tripIndex,c=a.dataset.tripContent,d=a.dataset.tripDelay,e=a.dataset.tripPosition||this._DEFAULT_TRIP_POSITION;a.dataset.tripAnimation||this._DEFAULT_TRIP_ANIMATION}if(a&&"undefined"!=typeof b&&""!==c){b=parseInt(b,10),d=parseInt(d,10);var f={};return f.sel=a,f._index=b,f.position=e,f.content=c,d&&!isNaN(d)&&(f.delay=d),f}return null},_sort:function(a){a.sort(function(a,b){return a._index-b._index})},parse:function(a){if("string"!=typeof a)throw"Please check your selector - "+a+" , and make sure it is String type";a="default"===a?this._DEFAULT_TRIP_NODES_SELECTOR:a;var b=this,c=[],d=this._getAllTripNodes(a);return d&&[].forEach.call(d,function(a){var d=b._parseTripData(a);d&&c.push(d)}),this._sort(c),c}},a.TripParser=new b}(window),function(a,b){function c(a,b){var c,d,e=b;this.pause=function(){window.clearTimeout(c),e-=new Date-d},this.resume=function(){return d=new Date,c=window.setTimeout(a,e),e},this.stop=function(){window.clearTimeout(c)},this.resume()}var d=["flash","bounce","shake","tada","fadeIn","fadeInUp","fadeInDown","fadeInLeft","fadeInRight","fadeInUpBig","fadeInDownBig","fadeInLeftBig","fadeInRightBig","bounceIn","bounceInDown","bounceInUp","bounceInLeft","bounceInRight","rotateIn","rotateInDownLeft","rotateInDownRight","rotateInUpLeft","rotateInUpRight"],e=function(){var a,c;if(0===arguments.length)a=window.TripParser.parse("default"),c={};else if(1===arguments.length)if(this.isArray(arguments[0]))a=arguments[0],c={};else if(this.isObject(arguments[0]))a=window.TripParser.parse("default"),c=arguments[0];else{if(!this.isString(arguments[0]))throw"Please check documents for passing parameters, you may pass wrong parameters into constructor function !";a=window.TripParser.parse(arguments[0]),c={}}else this.isArray(arguments[0])?a=arguments[0]:this.isString(arguments[0])&&(a=window.TripParser.parse(arguments[0])),c=arguments[1];this.settings=b.extend({tripIndex:0,tripTheme:"black",backToTopWhenEnded:!1,overlayHolder:"body",overlayZindex:99999,delay:1e3,enableKeyBinding:!0,enableAnimation:!0,showCloseBox:!1,showHeader:!1,skipUndefinedTrip:!1,showNavigation:!1,canGoNext:!0,canGoPrev:!0,nextLabel:"Next",prevLabel:"Back",finishLabel:"Dismiss",closeBoxLabel:"×",header:"Step {{tripIndex}}",onStart:b.noop,onEnd:b.noop,onTripStart:b.noop,onTripEnd:b.noop,onTripStop:b.noop,onTripPause:b.noop,onTripResume:b.noop,onTripChange:b.noop,onTripClose:b.noop,animation:"tada",tripBlockHTML:['"]},c),this.tripData=a,this.$tripBlock=null,this.$overlay=null,this.$bar=null,this.$root=b("body, html"),this.tripIndex=this.settings.tripIndex,this.tripDirection="next",this.timer=null,this.progressing=!1,this.hasExpose=!1,this.CONSTANTS={LEFT_ARROW:37,UP_ARROW:38,RIGHT_ARROW:39,DOWN_ARROW:40,ESC:27,SPACE:32,TRIP_BLOCK_OFFSET_VERTICAL:10,TRIP_BLOCK_OFFSET_HORIZONTAL:10,RESIZE_TIMEOUT:200},this.console=window.console||{}};e.prototype={preInit:function(){if("undefined"==typeof this.console){var a=this,c=["log","warn","debug","info","error"];b.each(c,function(c,d){a.console[d]=b.noop})}},showExpose:function(){var a,c,d,e=this.getCurrentTripObject();d="string"==typeof e.expose?b(e.expose):e.expose instanceof b?e.expose:b(e.sel),this.hasExpose=!0,void 0!==d.get(0)&&(a={position:d.css("position"),zIndex:d.css("z-Index")},c={position:"relative",zIndex:this.settings.overlayZindex+1},d.data("trip-old-css",a).css(c).addClass("trip-exposed")),this.$overlay.show()},hideExpose:function(){var a=b(".trip-exposed");if(this.hasExpose=!1,void 0!==a.get(0)){var c=a.data("trip-old-css");a.css(c).removeClass("trip-exposed")}this.$overlay.hide()},bindResizeEvents:function(){var a,c=this;b(window).on("resize.Trip",function(){window.clearTimeout(a),a=window.setTimeout(function(){c.run()},c.CONSTANTS.RESIZE_TIMEOUT)})},unbindResizeEvents:function(){b(window).off("resize.Trip")},bindKeyEvents:function(){var a=this;b(document).on({"keydown.Trip":function(b){a.keyEvent.call(a,b)}})},unbindKeyEvents:function(){b(document).off("keydown.Trip")},keyEvent:function(a){switch(a.which){case this.CONSTANTS.ESC:this.stop();break;case this.CONSTANTS.SPACE:a.preventDefault(),this.pause();break;case this.CONSTANTS.LEFT_ARROW:case this.CONSTANTS.UP_ARROW:this.prev();break;case this.CONSTANTS.RIGHT_ARROW:case this.CONSTANTS.DOWN_ARROW:this.next()}},stop:function(){this.timer&&this.timer.stop(),this.hasExpose&&this.hideExpose(),this.hideTripBlock(),this.unbindKeyEvents(),this.unbindResizeEvents();var a=this.getCurrentTripObject(),b=a.onTripStop||this.settings.onTripStop;b(this.tripIndex,a),this.settings.onEnd(this.tripIndex,a),this.tripIndex=this.settings.tripIndex},pauseOrResume:function(){if(this.progressing)this.timer.pause(),this.pauseProgressBar();else{var a=this.timer.resume();this.resumeProgressBar(a)}this.progressing=!this.progressing},pause:function(){this.pauseOrResume();var a=this.getCurrentTripObject(),b=a.onTripPause||this.settings.onTripPause;b(this.tripIndex,a)},resume:function(){this.pauseOrResume();var a=this.getCurrentTripObject(),b=a.onTripResume||this.settings.onTripResume;b(this.tripIndex,a)},next:function(){if(!this.canGoNext())return this.run();this.tripDirection="next";var a=this.getCurrentTripObject(),b=a.onTripEnd||this.settings.onTripEnd;b(this.tripIndex,a),this.isLast()?this.doLastOperation():(this.increaseIndex(),this.run())},prev:function(){this.tripDirection="prev";var a=this.getCurrentTripObject(),b=a.onTripEnd||this.settings.onTripEnd;b(this.tripIndex,a),!this.isFirst()&&this.canGoPrev()&&this.decreaseIndex(),this.run()},showCurrentTrip:function(a){this.settings.enableAnimation&&this.removeAnimation(),this.timer&&this.timer.stop(),this.hasExpose&&this.hideExpose(),this.progressing&&(this.hideProgressBar(),this.progressing=!1),this.setTripBlock(a),this.showTripBlock(a),this.settings.enableAnimation&&this.addAnimation(a),a.expose&&this.showExpose()},doLastOperation:function(){this.timer&&this.timer.stop(),this.settings.enableKeyBinding&&this.unbindKeyEvents(),this.hideTripBlock(),this.unbindResizeEvents(),this.hasExpose&&this.hideExpose(),this.settings.backToTopWhenEnded&&this.$root.animate({scrollTop:0},"slow");var a=this.getCurrentTripObject();return this.settings.onEnd(this.tripIndex,a),this.tripIndex=this.settings.tripIndex,!1},showProgressBar:function(a){var b=this;this.$bar.animate({width:"100%"},a,"linear",function(){b.$bar.width(0)})},hideProgressBar:function(){this.$bar.width(0),this.$bar.stop(!0)},pauseProgressBar:function(){this.$bar.stop(!0)},resumeProgressBar:function(a){this.showProgressBar(a)},run:function(){var a=this,b=this.getCurrentTripObject(),d=b.onTripStart||this.settings.onTripStart,e=b.onTripChange||this.settings.onTripChange,f=b.delay||this.settings.delay;return this.isTripDataValid(b)?(this.showCurrentTrip(b),this.showProgressBar(f),this.progressing=!0,e(this.tripIndex,b),d(this.tripIndex,b),void(f>=0&&(this.timer=new c(function(){a.next()},f)))):this.settings.skipUndefinedTrip===!1?(this.console.error("Your tripData is not valid at index: "+this.tripIndex),this.stop(),!1):this[this.tripDirection]()},isFirst:function(){return 0===this.tripIndex?!0:!1},isLast:function(){return this.tripIndex===this.tripData.length-1?!0:!1},isTripDataValid:function(a){return this.hasSpeicalDirections()?!0:"undefined"==typeof a.content||"undefined"==typeof a.sel||null===a.sel||0===a.sel.length||0===b(a.sel).length?!1:!0},hasSpeicalDirections:function(){var a=this.getCurrentTripObject(),c=a.position,d=["screen-ne","screen-se","screen-sw","screen-nw","screen-center"];return b.inArray(c,d)>=0?!0:!1},canGoPrev:function(){var a=this.tripData[this.tripIndex],b=a.canGoPrev||this.settings.canGoPrev;return"function"==typeof b&&(b=b.call(a)),b},canGoNext:function(){var a=this.tripData[this.tripIndex],b=a.canGoNext||this.settings.canGoNext;return"function"==typeof b&&(b=b.call(a)),b},increaseIndex:function(){this.tripIndex>=this.tripData.length-1||(this.tripIndex+=1)},decreaseIndex:function(){this.tripIndex<=0||(this.tripIndex-=1)},isArray:function(a){return"[object Array]"===Object.prototype.toString.call(a)},isString:function(a){return"string"==typeof a},isObject:function(a){return"[object Object]"===Object.prototype.toString.call(a)},getCurrentTripObject:function(){return this.tripData[this.tripIndex]},getReplacedTripContent:function(a){a=a||"";var b=/\{\{(tripIndex)\}\}/g,c=/\{\{(tripTotal)\}\}/g;return a=a.replace(b,this.tripIndex+1),a=a.replace(c,this.tripData.length)},setTripBlock:function(a){var b=this.$tripBlock,c=a.showCloseBox||this.settings.showCloseBox,d=a.showNavigation||this.settings.showNavigation,e=a.showHeader||this.settings.showHeader,f=a.closeBoxLabel||this.settings.closeBoxLabel,g=a.prevLabel||this.settings.prevLabel,h=a.nextLabel||this.settings.nextLabel,i=a.finishLabel||this.settings.finishLabel,j=a.header||this.settings.header;b.find(".trip-header").html(this.getReplacedTripContent(j)).toggle(e),b.find(".trip-content").html(this.getReplacedTripContent(a.content)),b.find(".trip-prev").html(g).toggle(d&&!this.isFirst()),b.find(".trip-next").html(this.isLast()?i:h).toggle(d),b.find(".trip-close").html(f).toggle(c),b.removeClass("e s w n screen-ne screen-se screen-sw screen-nw screen-center"),b.addClass(a.position),this.setTripBlockPosition(a,"horizontal"),this.setTripBlockPosition(a,"vertical")},setTripBlockPosition:function(a,c){var d,e,f=this.$tripBlock,g=b(a.sel),h=g&&g.outerWidth(),i=g&&g.outerHeight(),j=f.outerWidth(),k=f.outerHeight(),l=10,m=10;switch(a.position){case"screen-center":d="50%",e="50%";break;case"screen-ne":case"screen-se":case"screen-nw":case"screen-sw":d=this.CONSTANTS.TRIP_BLOCK_OFFSET_HORIZONTAL,e=this.CONSTANTS.TRIP_BLOCK_OFFSET_VERTICAL;break;case"e":d=g.offset().left+h+m,e=g.offset().top-(k-i)/2;break;case"s":d=g.offset().left+(h-j)/2,e=g.offset().top+i+l;break;case"w":d=g.offset().left-(m+j),e=g.offset().top-(k-i)/2;break;case"n":default:d=g.offset().left+(h-j)/2,e=g.offset().top-l-k}if("horizontal"===c)switch(f.css({left:"",right:"",marginLeft:""}),a.position){case"screen-center":f.css({left:d,marginLeft:-.5*j});break;case"screen-se":case"screen-ne":f.css({right:d});break;case"screen-sw":case"screen-nw":case"e":case"s":case"w":case"n":default:f.css({left:d})}else if("vertical"===c)switch(f.css({top:"",bottom:"",marginTop:""}),a.position){case"screen-center":f.css({top:e,marginTop:-.5*k});break;case"screen-sw":case"screen-se":f.css({bottom:e});break;case"screen-nw":case"screen-ne":case"e":case"s":case"w":case"n":default:f.css({top:e})}},addAnimation:function(a){var c=a.animation||this.settings.animation;b.inArray(c,d)>=0&&(this.$tripBlock.addClass("animated"),this.$tripBlock.addClass(c))},removeAnimation:function(){this.$tripBlock.removeClass(d.join(" ")),this.$tripBlock.removeClass("animated")},showTripBlock:function(){this.$tripBlock.css({display:"inline-block",zIndex:this.settings.overlayZindex+1});var a=b(window).height(),c=b(window).scrollTop(),d=this.$tripBlock.offset().top,e=this.$tripBlock.height(),f=100;c+a>d+e&&d>=c||this.$root.animate({scrollTop:d-f},"slow")},hideTripBlock:function(){this.$tripBlock.fadeOut("slow")},create:function(){this.createTripBlock(),this.createOverlay()},createTripBlock:function(){if("undefined"==typeof b(".trip-block").get(0)){var a=this,c=this.settings.tripBlockHTML.join(""),d=b(c).addClass(this.settings.tripTheme);b("body").append(d),d.find(".trip-close").on("click",function(b){b.preventDefault();var c=a.getCurrentTripObject(),d=c.onTripClose||a.settings.onTripClose;d(a.tripIndex,c),a.stop()}),d.find(".trip-prev").on("click",function(c){c.preventDefault(),b(this).blur(),a.prev()}),d.find(".trip-next").on("click",function(c){c.preventDefault(),b(this).blur(),a.next()})}},createOverlay:function(){if("undefined"==typeof b(".trip-overlay").get(0)){var a=[' "].join(""),c=b(a);c.height(b(window).height()).css({zIndex:this.settings.overlayZindex}),b(this.settings.overlayHolder).append(c)}},cleanup:function(){b(".trip-overlay, .trip-block").remove()},init:function(){this.preInit(),this.settings.enableKeyBinding&&this.bindKeyEvents(),this.bindResizeEvents(),this.$tripBlock=b(".trip-block"),this.$bar=b(".trip-progress-bar"),this.$overlay=b(".trip-overlay")},start:function(){this.cleanup(),this.settings.onStart(),this.create(),this.init(),this.run()}},a.Trip=e}(window,jQuery);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy