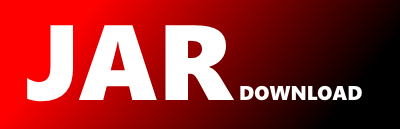
org.wicketstuff.datatables.media.js.ColReorder.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wicketstuff-datatables Show documentation
Show all versions of wicketstuff-datatables Show documentation
DataTables jQuery integration for Wicket
/*
* File: ColReorder.js
* Version: 1.0.0
* CVS: $Id$
* Description: Controls for column visiblity in DataTables
* Author: Allan Jardine (www.sprymedia.co.uk)
* Created: Wed Sep 15 18:23:29 BST 2010
* Modified: $Date$ by $Author$
* Language: Javascript
* License: LGPL
* Project: DataTables
* Contact: www.sprymedia.co.uk/contact
*
* Copyright 2010 Allan Jardine, all rights reserved.
*
*/
(function($, window, document) {
/**
* Switch the key value pairing of an index array to be value key (i.e. the old value is now the
* key). For example consider [ 2, 0, 1 ] this would be returned as [ 1, 2, 0 ].
* @method fnInvertKeyValues
* @param array aIn Array to switch around
* @returns array
*/
function fnInvertKeyValues( aIn )
{
var aRet=[];
for ( var i=0, iLen=aIn.length ; i= iCols )
{
this.oApi._fnLog( oSettings, 1, "ColReorder 'from' index is out of bounds: "+iFrom );
return;
}
if ( iTo < 0 || iTo >= iCols )
{
this.oApi._fnLog( oSettings, 1, "ColReorder 'to' index is out of bounds: "+iTo );
return;
}
/*
* Calculate the new column array index, so we have a mapping between the old and new
*/
var aiMapping = [];
for ( i=0, iLen=iCols ; i this.s.fixed-1 )
{
$(this.s.dt.aoColumns[i].nTh).bind( 'mousedown.ColReorder', function (e) {
that._fnMouseDown.call( that, e );
return false;
} );
}
/* Mark the original column order for later reference */
this.s.dt.aoColumns[i]._ColReorder_iOrigCol = i;
}
/* State saving */
this.s.dt.aoStateSave.push( {
"fn": function (oS, sVal) {
return that._fnStateSave.call( that, sVal );
},
"sName": "ColReorder_State"
} );
/* An initial column order has been specified */
var aiOrder = null;
if ( typeof this.s.init.aiOrder != 'undefined' )
{
aiOrder = this.s.init.aiOrder.slice();
}
/* State loading, overrides the column order given */
if ( this.s.dt.oLoadedState && typeof this.s.dt.oLoadedState.ColReorder != 'undefined' &&
this.s.dt.oLoadedState.ColReorder.length == this.s.dt.aoColumns.length )
{
aiOrder = this.s.dt.oLoadedState.ColReorder;
}
/* If we have an order to apply - do so */
if ( aiOrder )
{
/* We might be called during or after the DataTables initialisation. If before, then we need
* to wait until the draw is done, if after, then do what we need to do right away
*/
if ( !that.s.dt._bInitComplete )
{
var bDone = false;
this.s.dt.aoDrawCallback.push( {
"fn": function () {
if ( !that.s.dt._bInitComplete && !bDone )
{
bDone = true;
var resort = fnInvertKeyValues( aiOrder );
that._fnOrderColumns.call( that, resort );
}
},
"sName": "ColReorder_Pre"
} );
}
else
{
var resort = fnInvertKeyValues( aiOrder );
that._fnOrderColumns.call( that, resort );
}
}
},
/**
* Set the column order from an array
* @method _fnOrderColumns
* @param array a An array of integers which dictate the column order that should be applied
* @returns void
* @private
*/
"_fnOrderColumns": function ( a )
{
if ( a.length != this.s.dt.aoColumns.length )
{
this.s.dt.oInstance.oApi._fnLog( oDTSettings, 1, "ColReorder - array reorder does not "+
"match known number of columns. Skipping." );
return;
}
for ( var i=0, iLen=a.length ; i 0 )
{
this.dom.drag.removeChild( this.dom.drag.getElementsByTagName('tbody')[0] );
}
while ( this.dom.drag.getElementsByTagName('tfoot').length > 0 )
{
this.dom.drag.removeChild( this.dom.drag.getElementsByTagName('tfoot')[0] );
}
$('thead tr:eq(0)', this.dom.drag).each( function () {
$('th:not(:eq('+that.s.mouse.targetIndex+'))', this).remove();
} );
$('tr', this.dom.drag).height( $(that.s.dt.nTHead).height() );
$('thead tr:gt(0)', this.dom.drag).remove();
$('thead th:eq(0)', this.dom.drag).each( function (i) {
this.style.width = $('th:eq('+that.s.mouse.targetIndex+')', that.s.dt.nTHead).width()+"px";
} );
this.dom.drag.style.position = "absolute";
this.dom.drag.style.top = "0px";
this.dom.drag.style.left = "0px";
this.dom.drag.style.width = $('th:eq('+that.s.mouse.targetIndex+')', that.s.dt.nTHead).outerWidth()+"px";
this.dom.pointer = document.createElement( 'div' );
this.dom.pointer.className = "DTCR_pointer";
this.dom.pointer.style.position = "absolute";
if ( this.s.dt.oScroll.sX === "" && this.s.dt.oScroll.sY === "" )
{
this.dom.pointer.style.top = $(this.s.dt.nTable).offset().top+"px";
this.dom.pointer.style.height = $(this.s.dt.nTable).height()+"px";
}
else
{
this.dom.pointer.style.top = $('div.dataTables_scroll', this.s.dt.nTableWrapper).offset().top+"px";
this.dom.pointer.style.height = $('div.dataTables_scroll', this.s.dt.nTableWrapper).height()+"px";
}
document.body.appendChild( this.dom.pointer );
document.body.appendChild( this.dom.drag );
}
};
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
* Static parameters
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
/**
* Array of all ColReorder instances for later reference
* @property ColReorder.aoInstances
* @type array
* @default []
* @static
*/
ColReorder.aoInstances = [];
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
* Static functions
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
/**
* Reset the column ordering for a DataTables instance
* @method ColReorder.fnReset
* @param object oTable DataTables instance to consider
* @returns void
* @static
*/
ColReorder.fnReset = function ( oTable )
{
for ( var i=0, iLen=ColReorder.aoInstances.length ; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy