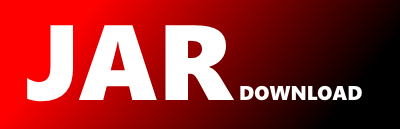
le.conventional-release-gradle-plugin.0.2.0.source-code.se.bjurr.gradle.conventional-release.gradle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of conventional-release-gradle-plugin Show documentation
Show all versions of conventional-release-gradle-plugin Show documentation
Release Java or Gradle Plugin projects with conventional versioning.
def getGivenConfigConventionalelease() {
def givenConfig = [
// ---- default config ----
// repoType: JAR # JAR, GRADLE, COMMAND
repoType: project.getProperties().getOrDefault("repoType", "JAR"),
// relocate: org:org,com:com # Empty by default will.
relocate: project.getProperties().getOrDefault("relocate", ""),
website: project.getProperties().getOrDefault("website", "https://github.com/tomasbjerre/" + project.name),
vcsUrl: project.getProperties().getOrDefault("vcsUrl", "https://github.com/tomasbjerre/" + project.name),
licenseName: project.getProperties().getOrDefault("licenseName", "The Apache Software License, Version 2.0"),
licenseUrl: project.getProperties().getOrDefault("licenseUrl", "http://www.apache.org/licenses/LICENSE-2.0.txt"),
developerId: project.getProperties().getOrDefault("developerId", "tomasbjerre"),
developerName: project.getProperties().getOrDefault("developerName", "Tomas Bjerre"),
developerEmail: project.getProperties().getOrDefault("developerEmail", "[email protected]"),
mavenRepositoryName: project.getProperties().getOrDefault("mavenRepositoryName", "nexus"),
mavenRepositoryUrl: project.getProperties().getOrDefault("mavenRepositoryUrl", "https://oss.sonatype.org/service/local/"),
nexusUsernameEnvOrProp: project.getProperties().getOrDefault("nexusUsernameEnvOrProp", "nexusUsername"),
nexusPasswordEnvOrProp: project.getProperties().getOrDefault("nexusPasswordEnvOrProp", "nexusPassword"),
signingKeyEnvOrProp: project.getProperties().getOrDefault("signingKeyEnvOrProp", "signing.keyId"),
signingPasswordEnvOrProp: project.getProperties().getOrDefault("signingPasswordEnvOrProp", "signing.password"),
// tags: a,b,c # Empty by default
tags: project.getProperties().getOrDefault("tags", ""),
implementationClass: project.getProperties().getOrDefault("implementationClass", ""),
stripGradlePluginSuffix: project.getProperties().getOrDefault("stripGradlePluginSuffix", "true") == "true",
// If it should be published to Plugin Portal or Central
publishGradlePluginToGradlePortal: project.getProperties().getOrDefault("publishGradlePluginToGradlePortal", "true") == "true",
// ---- default config ----
]
return givenConfig
}
def getEffectiveConfigConventionalelease() {
def givenConfig = getGivenConfigConventionalelease();
return givenConfig + [
useShadowJar: !givenConfig.relocate.isEmpty(),
tags: givenConfig.tags.tokenize(','),
relocate: givenConfig.relocate.tokenize(',')]
}
logger.info("Conventional Release: Given config: ${getGivenConfigConventionalelease()}")
logger.info("Conventional Release: Effective config: ${getEffectiveConfigConventionalelease()}")
if (getEffectiveConfigConventionalelease().useShadowJar) {
project.plugins.apply "com.gradleup.shadow"
}
project.plugins.apply 'java-library'
project.plugins.apply 'maven-publish'
project.plugins.apply 'signing'
project.plugins.apply "io.github.gradle-nexus.publish-plugin"
if (getEffectiveConfigConventionalelease().publishGradlePluginToGradlePortal) {
project.plugins.apply "com.gradle.plugin-publish"
}
project.plugins.apply "se.bjurr.gitchangelog.git-changelog-gradle-plugin"
/**
* NPM
*/
task publishNpm() {
doLast {
runCommand(['npm', 'publish'])
}
}
task setupNpmJavaRunnerFiles() {
doLast {
shouldGitIgnore('index.js')
copyIfFound("npm-java-runner-index.js", "index.js")
shouldGitIgnore('package.json')
shouldGitIgnore('package-lock.json')
shouldGitIgnore('node_modules')
copyIfFound("npm-java-runner-package.json", "package.json")
ant.replace(file: new File("package.json"), token: "PKG_NAME", value: project.name)
ant.replace(file: new File("package.json"), token: "PKG_VERSION", value: version)
ant.replace(file: new File("package.json"), token: "PKG_WEBSITE", value: getEffectiveConfigConventionalelease().website)
ant.replace(file: new File("package.json"), token: "PKG_GIT", value: getEffectiveConfigConventionalelease().vcsUrl)
ant.replace(file: new File("package.json"), token: "PKG_AUTHOR", value: getEffectiveConfigConventionalelease().developerName)
ant.replace(file: new File("package.json"), token: "PKG_DESCRIPTION", value: project.description.replaceAll("[\"']",""))
}
}
gitChangelogSemanticVersion {
suffixSnapshotIfNotTagged = false
}
/**
* Relocating
*/
if (getEffectiveConfigConventionalelease().useShadowJar) {
getEffectiveConfigConventionalelease().relocate.each { it ->
if (it.isEmpty()) {
return
}
def relocateFrom = ""
def relocateTo = ""
if (it.contains(":")) {
relocateFrom = it.split(":")[0]
relocateTo = it.split(":")[1]
} else {
relocateFrom = it
relocateTo = (project.group + '.' + project.name + '.' + relocateFrom)
.replaceAll('[^a-zA-Z\\.]','')
}
logger.lifecycle("Relocating ${relocateFrom} to ${relocateTo}")
shadowJar {
relocate relocateFrom, relocateTo
}
}
shadowJar {
archiveBaseName.set(project.name)
archiveClassifier.set('')
archiveVersion.set(project.version)
}
build.dependsOn shadowJar
} else {
logger.lifecycle("Not including shadow jar.")
}
/**
* GRADLE
*/
if (getEffectiveConfigConventionalelease().publishGradlePluginToGradlePortal) {
def pluginId = (project.group + '.' + project.name)
.replaceAll("[\"']","")
if (getEffectiveConfigConventionalelease().stripGradlePluginSuffix) {
pluginId = pluginId.replaceAll(/-gradle-plugin$/,"")
}
def displayNameValue = (name.substring(0, 1).toUpperCase() + name.substring(1))
.replaceAll('-',' ')
.replaceAll("[\"']","")
logger.lifecycle("pluginId ${pluginId} displayName ${displayNameValue}")
gradlePlugin {
website = getEffectiveConfigConventionalelease().website
vcsUrl = getEffectiveConfigConventionalelease().vcsUrl
description = project.description.replaceAll("[\"']","")
plugins {
matching { it.name.startsWith(project.group) }.configureEach {
tags.set(getEffectiveConfigConventionalelease().tags)
description = project.description.replaceAll("[\"']","")
id = pluginId
displayName = displayNameValue
implementationClass = getEffectiveConfigConventionalelease().implementationClass
}
}
}
}
/**
* Maven publishing
*/
if (getEffectiveConfigConventionalelease().repoType != "GRADLE") {
publishing {
publications {
mavenJava(MavenPublication) { publication ->
if (getEffectiveConfigConventionalelease().useShadowJar) {
logger.lifecycle("shadow jar in maven publication")
project.shadow.component(publication)
artifact javadocJar
artifact sourcesJar
} else {
logger.lifecycle("Regular jar in maven publication")
from project.components.java
}
logger.lifecycle("group: ${project.group} name: ${project.name}")
groupId project.group.replaceAll("[\"']","")
artifactId project.name.replaceAll("[\"']","")
version version
versionMapping {
allVariants {
fromResolutionResult()
}
}
}
}
}
repositories {
maven {
name = getEffectiveConfigConventionalelease().mavenRepositoryName
credentials(PasswordCredentials)
url = getEffectiveConfigConventionalelease().mavenRepositoryUrl+"staging/deploy/maven2/"
}
}
}
afterEvaluate {
tasks.withType(GenerateMavenPom) { task ->
doFirst {
task.pom.description = project.description.replaceAll("[\"']","")
task.pom.name = project.name
task.pom.withXml {
def root = asNode()
root.appendNode('url', getEffectiveConfigConventionalelease().website)
root.appendNode('inceptionYear', new Date().getYear() + 1900)
root.children().last() + {
scm {
url getEffectiveConfigConventionalelease().website
connection getEffectiveConfigConventionalelease().vcsUrl
developerConnection getEffectiveConfigConventionalelease().vcsUrl
}
licenses {
license {
name getEffectiveConfigConventionalelease().licenseName
url getEffectiveConfigConventionalelease().licenseUrl
distribution 'repo'
}
}
developers {
developer {
id getEffectiveConfigConventionalelease().developerId
name getEffectiveConfigConventionalelease().developerName
email getEffectiveConfigConventionalelease().developerEmail
}
}
}
}
}
}
}
java {
withSourcesJar()
withJavadocJar()
}
nexusPublishing {
repositories {
sonatype {
nexusUrl = uri(getEffectiveConfigConventionalelease().mavenRepositoryUrl)
username = findEnvOrPropValue(getEffectiveConfigConventionalelease().nexusUsernameEnvOrProp)
password = findEnvOrPropValue(getEffectiveConfigConventionalelease().nexusPasswordEnvOrProp)
}
}
}
signing {
def signingKey = findEnvOrPropValue(getEffectiveConfigConventionalelease().signingKeyEnvOrProp)
def signingPassword = findEnvOrPropValue(getEffectiveConfigConventionalelease().signingPasswordEnvOrProp)
def hasSigningCredentials = signingKey != null && signingPassword != null
// I use "signing.keyId" prop when building locally, remote probably different and it probably has in memory
def assumeThereIsAnInMemoryPgpKeys = getEffectiveConfigConventionalelease().signingKeyEnvOrProp != "signing.keyId"
required {
hasSigningCredentials && gradle.taskGraph.hasTask("closeAndReleaseStagingRepositories")
}
if (assumeThereIsAnInMemoryPgpKeys && hasSigningCredentials) {
logger.lifecycle("Configuring signing key and password")
useInMemoryPgpKeys(signingKey, signingPassword)
} else {
logger.lifecycle("Not configuring signing key and password")
}
sign(publishing.publications)
}
tasks.withType(AbstractPublishToMaven) { publishTask ->
tasks.withType(Sign) { signTask ->
logger.info("${publishTask} must run after ${signTask}")
publishTask.mustRunAfter(signTask)
tasks.withType(Jar) { jarTask ->
logger.info("${signTask} must run after ${jarTask}")
signTask.mustRunAfter(jarTask)
}
}
}
/**
* Release process
*/
task release(type: GradleBuild) {
tasks = [
'gitChangelogSemanticVersion',
'commitNewVersionTask',
'clean',
'build',
'publishToMavenLocal',
]
if (getEffectiveConfigConventionalelease().publishGradlePluginToGradlePortal) {
tasks += ["publishPlugins"]
} else {
tasks += [
"publish",
"closeAndReleaseStagingRepositories",
]
}
tasks += [
'gitChangelog',
'commitChangelogTask'
]
if (getEffectiveConfigConventionalelease().repoType == 'COMMAND') {
tasks += [
"setupNpmJavaRunnerFiles",
"publishNpm"
]
}
}
def shouldGitIgnore(filename) {
if (!file('.gitignore').text.contains(filename)) {
throw new RuntimeException("The .gitignore should include '${filename}'")
}
logger.lifecycle("${filename} is ignored")
}
def findEnvOrPropValue(variable) {
def envValue = providers.environmentVariable(variable).orElse("").get()
def propValue = project.findProperty(variable)
if (!envValue.isEmpty()) {
return envValue
}
return propValue;
}
def runCommand(args, int attempts = 5) {
logger.lifecycle("Executing ${args.join(' ')}")
def stdout = new ByteArrayOutputStream()
try {
exec {
commandLine args
standardOutput = stdout
}
return stdout.toString().trim()
} catch (e) {
def err = stdout.toString().trim() + "\n" + "Command: ${args.join(' ')}" + "\n" + "Trying ${attempts} more times"
logger.lifecycle(err)
if (attempts == 0) {
throw new RuntimeException(err, e)
}
sleep(5 * 1000)
return runCommand(args, attempts - 1)
}
}
task commitNewVersionTask() {
doLast {
try {
runCommand([
'git',
'commit',
'-a',
'-m',
"chore(release): ${version} [GRADLE SCRIPT]"
])
runCommand(['git', 'push'])
runCommand(['git', 'tag', "${version}"])
runCommand([
'git',
'push',
'origin',
"${version}"
])
} catch (e) {
logger.error("Unable commit/push new version, skipping that.",e.getMessage())
}
}
}
task commitChangelogTask() {
doLast {
try {
runCommand([
'git',
'commit',
'-a',
'-m',
"chore(release): Updating changelog with ${version} [GRADLE SCRIPT]"
])
runCommand(['git', 'push'])
} catch (e) {
logger.error("Unable commit/push changelog, skipping that.",e.getMessage())
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy