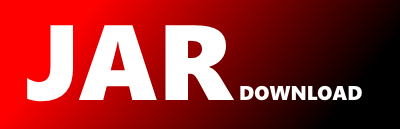
academy.alex.CustomMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of custommatcher Show documentation
Show all versions of custommatcher Show documentation
This is Custom Matcher to validate Credit Card
The newest version!
package academy.alex;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeDiagnosingMatcher;
public abstract class CustomMatcher extends Util{
public static class Type extends TypeSafeDiagnosingMatcher {
public void describeTo(Description description) {description.appendText("Credit Card type ").appendText(" must be valid");}
protected boolean matchesSafely(String cc_type_number, Description description) {
String type = cc_type_number.split(":")[0];
String number = cc_type_number.split(":")[1].replaceAll("\\s", "").replaceAll("-", "");
if (number.startsWith("4") && (number.length() == 13 || number.length() == 16) && type.equals("VISA")) {return true;}
else if ((number.startsWith("51") || number.startsWith("52") || number.startsWith("53") || number.startsWith("54") || number.startsWith("55")) && number.length() == 16 && type.equals("MasterCard")) {return true;}
else if ((number.startsWith("6011") || number.startsWith("62") || number.startsWith("64") || number.startsWith("65")) && number.length() == 16 && type.equals("Discover")) {return true;}
else if ((number.startsWith("34") || number.startsWith("37")) && number.length() == 15 && type.equals("American Express")) {return true;}
else {return false;}
}
}
public static class Luhn extends TypeSafeDiagnosingMatcher {
public void describeTo(Description description) {description.appendText("Credit Card number ").appendText(" must be valid");}
protected boolean matchesSafely(String cc_number, Description description) {
String number = new String(cc_number.replaceAll("\\s", "").replaceAll("-", ""));
int sum = 0; boolean swap = false;
for (int i = number.length() - 1; i >= 0; i--) {
int digit = Integer.parseInt(number.substring(i, i + 1));
if (swap) {digit *= 2;if (digit > 9) {digit -= 9;}}
sum += digit;swap = !swap;};
description.appendValue(cc_number).appendText(" is not valid");
return (sum % 10 == 0);
}
}
public static class Expiration extends TypeSafeDiagnosingMatcher {
public void describeTo(Description description) {description.appendText("Credit Card expiration ").appendText(" must be valid");}
protected boolean matchesSafely(String cc_exp, Description description) {
int exp = Integer.parseInt(cc_exp.substring(3, 5) + cc_exp.substring(0, 2)); // 02/18 => 1802
description.appendValue(cc_exp).appendText(" is already expired");
return today() <= exp;
}
}
public static class CVV extends TypeSafeDiagnosingMatcher {
public void describeTo(Description description) {description.appendText("Credit Card CVV ").appendText(" must be valid");}
protected boolean matchesSafely(String cc_type_cvv, Description description) {
String type = cc_type_cvv.split(":")[0];
String cvv = cc_type_cvv.split(":")[1];
if (type.equals("American Express")) {return cvv.matches("^(\\d{4})$");}
else {return cvv.matches("^(\\d{3})$");}
}
}
public static Matcher validType() {return new Type();}
public static Matcher validNumber() {return new Luhn();}
public static Matcher validExpiration() {return new Expiration();}
public static Matcher validCVV() {return new CVV();}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy