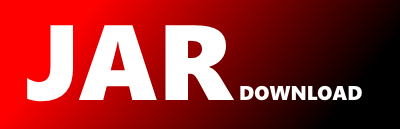
ai.grakn.GraknConfigKey Maven / Gradle / Ivy
/*
* GRAKN.AI - THE KNOWLEDGE GRAPH
* Copyright (C) 2018 Grakn Labs Ltd
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package ai.grakn;
import ai.grakn.util.ErrorMessage;
import com.google.auto.value.AutoValue;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Class for keys of properties in the file {@code grakn.properties}.
*
* @param the type of the values of the key
* @author Grakn Warriors
*/
@AutoValue
public abstract class GraknConfigKey {
/**
* Parser for a {@link GraknConfigKey}.
* Describes how to {@link #read(String)} and {@link #write(Object)} properties.
*
* @param The type of the property value
*/
public interface KeyParser {
T read(String string);
default String write(T value) {
return value.toString();
}
}
// These are helpful parser to describe how to parse parameters of certain types.
public static final KeyParser STRING = string -> string;
public static final KeyParser INT = Integer::parseInt;
public static final KeyParser BOOL = Boolean::parseBoolean;
public static final KeyParser LONG = Long::parseLong;
public static final KeyParser PATH = Paths::get;
public static final KeyParser> CSV = new KeyParser>() {
@Override
public List read(String string) {
Stream split = Arrays.stream(string.split(","));
return split.map(String::trim).filter(t -> !t.isEmpty()).collect(Collectors.toList());
}
@Override
public String write(List value) {
return value.stream().collect(Collectors.joining(","));
}
};
public static final GraknConfigKey WEBSERVER_THREADS = key("webserver.threads", INT);
public static final GraknConfigKey NUM_BACKGROUND_THREADS = key("background-tasks.threads", INT);
public static final GraknConfigKey SERVER_HOST_NAME = key("server.host");
public static final GraknConfigKey SERVER_PORT = key("server.port", INT);
public static final GraknConfigKey GRPC_PORT = key("grpc.port", INT);
public static final GraknConfigKey STORAGE_HOSTNAME = key("storage.hostname", STRING);
public static final GraknConfigKey STORAGE_BATCH_LOADING = key("storage.batch-loading", STRING);
public static final GraknConfigKey STORAGE_KEYSPACE = key("storage.cassandra.keyspace", STRING);
public static final GraknConfigKey STORAGE_REPLICATION_FACTOR = key("storage.cassandra.replication-factor", INT);
public static final GraknConfigKey> REDIS_HOST = key("queue.host", CSV);
public static final GraknConfigKey> REDIS_SENTINEL_HOST = key("queue.sentinel.host", CSV);
public static final GraknConfigKey REDIS_BIND = key("bind");
public static final GraknConfigKey REDIS_SENTINEL_MASTER = key("queue.sentinel.master");
public static final GraknConfigKey REDIS_POOL_SIZE = key("queue.pool-size", INT);
public static final GraknConfigKey POST_PROCESSOR_POOL_SIZE = key("post-processor.pool-size", INT);
public static final GraknConfigKey POST_PROCESSOR_DELAY = key("post-processor.delay", INT);
public static final GraknConfigKey STATIC_FILES_PATH = key("server.static-file-dir", PATH);
public static final GraknConfigKey SESSION_CACHE_TIMEOUT_MS = key("knowledge-base.schema-cache-timeout-ms", INT);
public static final GraknConfigKey TASKS_RETRY_DELAY = key("tasks.retry.delay", INT);
public static final GraknConfigKey SHARDING_THRESHOLD = key("knowledge-base.sharding-threshold", LONG);
public static final GraknConfigKey KB_MODE = key("knowledge-base.mode");
public static final GraknConfigKey KB_ANALYTICS = key("knowledge-base.analytics");
public static final GraknConfigKey DATA_DIR = key("data-dir");
public static final GraknConfigKey LOG_DIR = key("log.dirs");
public static final GraknConfigKey TEST_START_EMBEDDED_COMPONENTS =
key("test.start.embedded.components", BOOL);
/**
* The name of the key, how it looks in the properties file
*/
public abstract String name();
/**
* The parser used to read and write the property.
*/
abstract KeyParser parser();
/**
* Parse the value of a property.
*
* This function should return an empty optional if the key was not present and there is no default value.
*
* @param value the value of the property. Empty if the property isn't in the property file.
* @param configFilePath path to the config file
* @return the parsed value
*
* @throws RuntimeException if the value is not present and there is no default value
*/
public final T parse(String value, Path configFilePath) {
if (value == null) {
throw new RuntimeException(ErrorMessage.UNAVAILABLE_PROPERTY.getMessage(name(), configFilePath));
}
return parser().read(value);
}
/**
* Convert the value of the property into a string to store in a properties file
*/
public final String valueToString(T value) {
return parser().write(value);
}
/**
* Create a key for a string property
*/
public static GraknConfigKey key(String value) {
return key(value, STRING);
}
/**
* Create a key with the given parser
*/
public static GraknConfigKey key(String value, KeyParser parser) {
return new AutoValue_GraknConfigKey<>(value, parser);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy