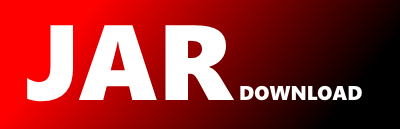
ai.grakn.util.CommonUtil Maven / Gradle / Ivy
/*
* GRAKN.AI - THE KNOWLEDGE GRAPH
* Copyright (C) 2018 Grakn Labs Ltd
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package ai.grakn.util;
import ai.grakn.GraknSystemProperty;
import com.google.common.base.StandardSystemProperty;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMultiset;
import com.google.common.collect.ImmutableSet;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Iterator;
import java.util.Optional;
import java.util.Set;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.function.BiConsumer;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* Common utility methods used within Grakn.
*
* Some of these methods are Grakn-specific, others add important "missing" methods to Java/Guava classes.
*
* @author Grakn Warriors
*/
public class CommonUtil {
private CommonUtil() {}
/**
* @return The project path. If it is not specified as a JVM parameter it will be set equal to
* user.dir folder.
*/
public static Path getProjectPath() {
if (GraknSystemProperty.CURRENT_DIRECTORY.value() == null) {
GraknSystemProperty.CURRENT_DIRECTORY.set(StandardSystemProperty.USER_DIR.value());
}
return Paths.get(GraknSystemProperty.CURRENT_DIRECTORY.value());
}
/**
* @param optional the optional to change into a stream
* @param the type in the optional
* @return a stream of one item if the optional has an element, else an empty stream
*/
public static Stream optionalToStream(Optional optional) {
return optional.map(Stream::of).orElseGet(Stream::empty);
}
public static Stream stream(Iterator iterator) {
return StreamSupport.stream(Spliterators.spliteratorUnknownSize(iterator, Spliterator.ORDERED), false);
}
/**
* Helper which lazily checks if a {@link Stream} contains the number specified
* WARNING: This consumes the stream rendering it unusable afterwards
*
* @param stream the {@link Stream} to check the count against
* @param size the expected number of elements in the stream
* @return true if the expected size is found
*/
public static boolean containsOnly(Stream stream, long size){
long count = 0L;
Iterator it = stream.iterator();
while(it.hasNext()){
it.next();
if(++count > size) return false;
}
return size == count;
}
@CheckReturnValue
public static RuntimeException unreachableStatement(Throwable cause) {
return unreachableStatement(null, cause);
}
@CheckReturnValue
public static RuntimeException unreachableStatement(String message) {
return unreachableStatement(message, null);
}
@CheckReturnValue
public static RuntimeException unreachableStatement(@Nullable String message, Throwable cause) {
return new RuntimeException("Statement expected to be unreachable: " + message, cause);
}
public static Collector> toImmutableSet() {
return new Collector, ImmutableSet>() {
@Override
public Supplier> supplier() {
return ImmutableSet::builder;
}
@Override
public BiConsumer, T> accumulator() {
return ImmutableSet.Builder::add;
}
@Override
public BinaryOperator> combiner() {
return (b1, b2) -> b1.addAll(b2.build());
}
@Override
public Function, ImmutableSet> finisher() {
return ImmutableSet.Builder::build;
}
@Override
public Set characteristics() {
return ImmutableSet.of();
}
};
}
public static Collector> toImmutableList() {
return new Collector, ImmutableList>() {
@Override
public Supplier> supplier() {
return ImmutableList::builder;
}
@Override
public BiConsumer, T> accumulator() {
return ImmutableList.Builder::add;
}
@Override
public BinaryOperator> combiner() {
return (b1, b2) -> b1.addAll(b2.build());
}
@Override
public Function, ImmutableList> finisher() {
return ImmutableList.Builder::build;
}
@Override
public Set characteristics() {
return ImmutableSet.of();
}
};
}
public static Collector> toImmutableMultiset() {
return new Collector, ImmutableMultiset>() {
@Override
public Supplier> supplier() {
return ImmutableMultiset::builder;
}
@Override
public BiConsumer, T> accumulator() {
return ImmutableMultiset.Builder::add;
}
@Override
public BinaryOperator> combiner() {
return (b1, b2) -> b1.addAll(b2.build());
}
@Override
public Function, ImmutableMultiset> finisher() {
return ImmutableMultiset.Builder::build;
}
@Override
public Set characteristics() {
return ImmutableSet.of();
}
};
}
public static StringBuilder simplifyExceptionMessage(Throwable e) {
StringBuilder message = new StringBuilder(e.getMessage());
while(e.getCause() != null) {
e = e.getCause();
message.append("\n").append(e.getMessage());
}
return message;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy