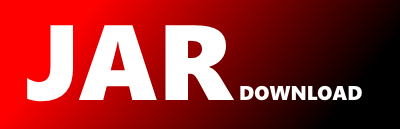
ai.grakn.engine.controller.response.AutoValue_EmbeddedAttribute Maven / Gradle / Ivy
package ai.grakn.engine.controller.response;
import ai.grakn.concept.ConceptId;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EmbeddedAttribute extends EmbeddedAttribute {
private final ConceptId id;
private final Link selfLink;
private final EmbeddedSchemaConcept type;
private final String value;
private final String dataType;
AutoValue_EmbeddedAttribute(
ConceptId id,
Link selfLink,
EmbeddedSchemaConcept type,
String value,
String dataType) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (selfLink == null) {
throw new NullPointerException("Null selfLink");
}
this.selfLink = selfLink;
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (value == null) {
throw new NullPointerException("Null value");
}
this.value = value;
if (dataType == null) {
throw new NullPointerException("Null dataType");
}
this.dataType = dataType;
}
@JsonProperty
@Override
public ConceptId id() {
return id;
}
@JsonProperty(value = "@id")
@Override
public Link selfLink() {
return selfLink;
}
@JsonProperty
@Override
public EmbeddedSchemaConcept type() {
return type;
}
@JsonProperty
@Override
public String value() {
return value;
}
@JsonProperty(value = "data-type")
@Override
public String dataType() {
return dataType;
}
@Override
public String toString() {
return "EmbeddedAttribute{"
+ "id=" + id + ", "
+ "selfLink=" + selfLink + ", "
+ "type=" + type + ", "
+ "value=" + value + ", "
+ "dataType=" + dataType
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EmbeddedAttribute) {
EmbeddedAttribute that = (EmbeddedAttribute) o;
return (this.id.equals(that.id()))
&& (this.selfLink.equals(that.selfLink()))
&& (this.type.equals(that.type()))
&& (this.value.equals(that.value()))
&& (this.dataType.equals(that.dataType()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.selfLink.hashCode();
h *= 1000003;
h ^= this.type.hashCode();
h *= 1000003;
h ^= this.value.hashCode();
h *= 1000003;
h ^= this.dataType.hashCode();
return h;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy