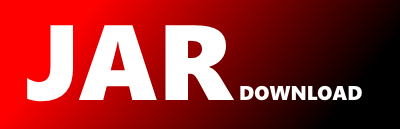
ai.grakn.engine.controller.response.AutoValue_Rule Maven / Gradle / Ivy
package ai.grakn.engine.controller.response;
import ai.grakn.concept.ConceptId;
import ai.grakn.concept.Label;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Rule extends Rule {
private final String baseType;
private final ConceptId id;
private final Link selfLink;
private final Label label;
private final Boolean implicit;
private final EmbeddedSchemaConcept sup;
private final Link subs;
private final String when;
private final String then;
AutoValue_Rule(
String baseType,
ConceptId id,
Link selfLink,
Label label,
Boolean implicit,
@Nullable EmbeddedSchemaConcept sup,
Link subs,
@Nullable String when,
@Nullable String then) {
if (baseType == null) {
throw new NullPointerException("Null baseType");
}
this.baseType = baseType;
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (selfLink == null) {
throw new NullPointerException("Null selfLink");
}
this.selfLink = selfLink;
if (label == null) {
throw new NullPointerException("Null label");
}
this.label = label;
if (implicit == null) {
throw new NullPointerException("Null implicit");
}
this.implicit = implicit;
this.sup = sup;
if (subs == null) {
throw new NullPointerException("Null subs");
}
this.subs = subs;
this.when = when;
this.then = then;
}
@JsonProperty(value = "base-type")
@Override
public String baseType() {
return baseType;
}
@JsonProperty(value = "id")
@Override
public ConceptId id() {
return id;
}
@JsonProperty(value = "@id")
@Override
public Link selfLink() {
return selfLink;
}
@JsonProperty
@Override
public Label label() {
return label;
}
@JsonProperty
@Override
public Boolean implicit() {
return implicit;
}
@Nullable
@JsonProperty(value = "super")
@Override
public EmbeddedSchemaConcept sup() {
return sup;
}
@JsonProperty
@Override
public Link subs() {
return subs;
}
@Nullable
@JsonProperty
@Override
public String when() {
return when;
}
@Nullable
@JsonProperty
@Override
public String then() {
return then;
}
@Override
public String toString() {
return "Rule{"
+ "baseType=" + baseType + ", "
+ "id=" + id + ", "
+ "selfLink=" + selfLink + ", "
+ "label=" + label + ", "
+ "implicit=" + implicit + ", "
+ "sup=" + sup + ", "
+ "subs=" + subs + ", "
+ "when=" + when + ", "
+ "then=" + then
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Rule) {
Rule that = (Rule) o;
return (this.baseType.equals(that.baseType()))
&& (this.id.equals(that.id()))
&& (this.selfLink.equals(that.selfLink()))
&& (this.label.equals(that.label()))
&& (this.implicit.equals(that.implicit()))
&& ((this.sup == null) ? (that.sup() == null) : this.sup.equals(that.sup()))
&& (this.subs.equals(that.subs()))
&& ((this.when == null) ? (that.when() == null) : this.when.equals(that.when()))
&& ((this.then == null) ? (that.then() == null) : this.then.equals(that.then()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.baseType.hashCode();
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.selfLink.hashCode();
h *= 1000003;
h ^= this.label.hashCode();
h *= 1000003;
h ^= this.implicit.hashCode();
h *= 1000003;
h ^= (sup == null) ? 0 : this.sup.hashCode();
h *= 1000003;
h ^= this.subs.hashCode();
h *= 1000003;
h ^= (when == null) ? 0 : this.when.hashCode();
h *= 1000003;
h ^= (then == null) ? 0 : this.then.hashCode();
return h;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy