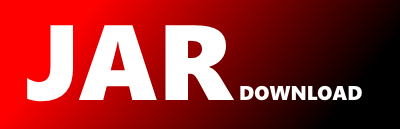
ai.grakn.kb.internal.cache.TxRuleCache Maven / Gradle / Ivy
/*
* GRAKN.AI - THE KNOWLEDGE GRAPH
* Copyright (C) 2018 Grakn Labs Ltd
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package ai.grakn.kb.internal.cache;
import ai.grakn.concept.Rule;
import ai.grakn.concept.SchemaConcept;
import ai.grakn.kb.internal.EmbeddedGraknTx;
import ai.grakn.util.Schema;
import com.google.common.collect.Sets;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Caches rules applicable to schema concepts and their conversion to InferenceRule object (parsing is expensive when large number of rules present).
* NB: non-committed rules are alse cached.
*
* @author Kasper Piskorski
*/
public class TxRuleCache {
private final Map> ruleMap = new HashMap<>();
private final Map ruleConversionMap = new HashMap<>();
private final EmbeddedGraknTx tx;
public TxRuleCache(EmbeddedGraknTx tx){
this.tx = tx;
}
/**
* @return set of inference rules contained in the graph
*/
public Stream getRules() {
Rule metaRule = tx.getMetaRule();
return metaRule.subs().filter(sub -> !sub.equals(metaRule));
}
/**
* @param type to be update
* @param rule to be appended
* @return updated entry vlue
*/
public Set updateRules(SchemaConcept type, Rule rule){
Set match = ruleMap.get(type);
if (match == null){
Set rules = Sets.newHashSet(rule);
getTypes(type, false).stream()
.flatMap(SchemaConcept::thenRules)
.forEach(rules::add);
ruleMap.put(type, rules);
return rules;
}
match.add(rule);
return match;
}
/**
* @param type for which rules containing it in the head are sought
* @return rules containing specified type in the head
*/
public Stream getRulesWithType(SchemaConcept type){
return getRulesWithType(type, false);
}
private Set getTypes(SchemaConcept type, boolean direct) {
Set types = direct ? Sets.newHashSet(type) : type.subs().collect(Collectors.toSet());
return type.isImplicit()?
types.stream().flatMap(t -> Stream.of(t, tx.getSchemaConcept(Schema.ImplicitType.explicitLabel(t.label())))).collect(Collectors.toSet()):
types;
}
/**
* @param type for which rules containing it in the head are sought
* @param direct way of assessing isa edges
* @return rules containing specified type in the head
*/
public Stream getRulesWithType(SchemaConcept type, boolean direct){
if (type == null) return getRules();
Set match = ruleMap.get(type);
if (match != null) return match.stream();
Set rules = new HashSet<>();
ruleMap.put(type, rules);
return getTypes(type, direct).stream()
.flatMap(SchemaConcept::thenRules)
.peek(rules::add);
}
/**
*
* @param rule for which the parsed rule should be retrieved
* @param converter rule converter
* @param type of object converter converts to
* @return parsed rule object
*/
public T getRule(Rule rule, Supplier converter){
T match = (T) ruleConversionMap.get(rule);
if (match != null) return match;
T newMatch = converter.get();
ruleConversionMap.put(rule, newMatch);
return newMatch;
}
/**
* cleans cache contents
*/
public void closeTx(){
ruleMap.clear();
ruleConversionMap.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy