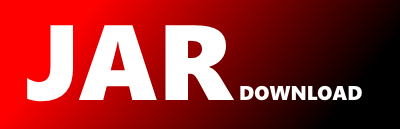
ai.grakn.kb.internal.structure.AbstractElement Maven / Gradle / Ivy
/*
* GRAKN.AI - THE KNOWLEDGE GRAPH
* Copyright (C) 2018 Grakn Labs Ltd
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package ai.grakn.kb.internal.structure;
import ai.grakn.GraknTx;
import ai.grakn.concept.Concept;
import ai.grakn.exception.GraknTxOperationException;
import ai.grakn.exception.PropertyNotUniqueException;
import ai.grakn.kb.internal.EmbeddedGraknTx;
import org.apache.tinkerpop.gremlin.process.traversal.dsl.graph.GraphTraversal;
import org.apache.tinkerpop.gremlin.structure.Element;
import org.apache.tinkerpop.gremlin.structure.Property;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import javax.annotation.Nullable;
import java.util.Objects;
import java.util.function.Function;
import static org.apache.tinkerpop.gremlin.structure.T.id;
/**
*
* {@link GraknTx} AbstractElement
*
*
*
* Base class used to represent a construct in the graph. This includes exposed constructs such as {@link Concept}
* and hidden constructs such as {@link EdgeElement} and {@link Casting}
*
*
* @param The type of the element. Either {@link VertexElement} of {@link EdgeElement}
* @param Enum indicating the allowed properties on each type. Either {@link ai.grakn.util.Schema.VertexProperty} or
* {@link ai.grakn.util.Schema.EdgeProperty}
*
* @author fppt
*
*/
public abstract class AbstractElement {
private final String prefix;
private final E element;
private final EmbeddedGraknTx tx;
AbstractElement(EmbeddedGraknTx tx, E element, String prefix){
this.tx = tx;
this.element = element;
this.prefix = prefix;
}
public E element(){
return element;
}
public ElementId id(){
return ElementId.of(prefix + element().id());
}
/**
* Deletes the element from the graph
*/
public void delete(){
element().remove();
}
/**
*
* @param key The key of the property to mutate
* @param value The value to commit into the property
*/
public void property(P key, Object value){
if(value == null) {
element().property(key.name()).remove();
} else {
Property