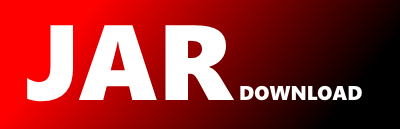
hex.GridSearchSchema Maven / Gradle / Ivy
package hex;
import com.google.gson.Gson;
import water.H2O;
import water.api.API;
import water.api.JobV3;
import water.api.ModelParametersSchema;
import water.api.Schema;
import water.util.IcedHashMap;
import water.util.ReflectionUtils;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
/**
* This is a common grid search schema composed of two parameters: default parameters for a builder and
* hyper parameters which are given as a mapping from parameter name to list of possible values.
*
* @param a specific implementation type for GridSearch holding results of grid search (model list)
* @param self type
* @param actual model parameters type
* @param a specific model builder parameters schema, since we cannot derive it from P
*/
public /* FIXME: abstract */ class GridSearchSchema,
S extends GridSearchSchema,
MP extends Model.Parameters,
P extends ModelParametersSchema> extends Schema {
// Hack from ModelBuilderSchema
public GridSearchSchema() {
this.parameters = createParametersSchema();
}
//
// Inputs
//
@API(help="Basic model builder parameters.")
public P parameters;
@API(help="Grid search parameters.")
public IcedHashMap grid_parameters;
//
// Outputs
//
@API(help="Number of all models generated by grid search.", direction = API.Direction.OUTPUT)
public int total_models;
@API(help = "Job Key.", direction = API.Direction.OUTPUT)
public JobV3 job;
@Override
public S fillFromParms(Properties parms) {
// FIXME: do this in generic way
if (parms.containsKey("grid_parameters")) {
String parameters = parms.getProperty("grid_parameters");
grid_parameters = parseJsonMap(parameters, new IcedHashMap());
parms.remove("grid_parameters");
}
this.parameters.fillFromParms(parms);
return (S) this;
}
@Override
public S fillFromImpl(G impl) {
S s = super.fillFromImpl(impl);
s.parameters = createParametersSchema();
s.parameters.fillFromImpl((MP) parameters.createImpl());
return s;
}
/** Factory method to create the model-specific parameters schema.
* FIXME: shared this code with ModelBuilderScheme
* */
final P createParametersSchema() {
P impl = null;
// special case, because GridSearchSchema is the top of the tree and is parameterized differently
if (GridSearchSchema.class == this.getClass()) {
return (P)new ModelParametersSchema();
}
try {
Class extends ModelParametersSchema> parameters_class = (Class extends ModelParametersSchema>) ReflectionUtils.findActualClassParameter(this.getClass(), 3);
impl = (P)parameters_class.newInstance();
}
catch (Exception e) {
throw H2O.fail("Caught exception trying to instantiate a builder instance for ModelBuilderSchema: " + this + ": " + e, e);
}
return impl;
}
// this method right now using gson
// It parse given json, and
public static > T parseJsonMap(String json, T map) {
Gson gson = new Gson();
Map m = new HashMap();
m = gson.fromJson(json, map.getClass());
for (Map.Entry e : m.entrySet()) {
if (e.getValue() instanceof List) {
map.put(e.getKey(), ((List) e.getValue()).toArray());
} else {
map.put(e.getKey(), new Object[] { e.getValue()});
}
}
return map;
}
}