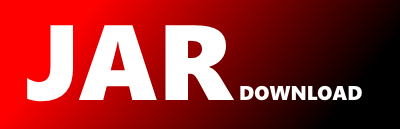
ai.h2o.sparkling.ml.params.H2OAutoMLStoppingCriteriaParams.scala Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ai.h2o.sparkling.ml.params
import ai.h2o.automl.AutoMLBuildSpec.AutoMLStoppingCriteria
import ai.h2o.sparkling.H2OFrame
import hex.ScoreKeeper.StoppingMetric
trait H2OAutoMLStoppingCriteriaParams
extends H2OAlgoParamsBase {
//
// Parameter definitions
//
protected val seed = longParam(
name = "seed",
doc = """Seed for random number generator; set to a value other than -1 for reproducibility.""")
protected val maxModels = intParam(
name = "maxModels",
doc = """Maximum number of models to build (optional). Always set this parameter to ensure AutoML reproducibility: all models are then trained until convergence and none is constrained by a time budget.""")
protected val maxRuntimeSecs = doubleParam(
name = "maxRuntimeSecs",
doc = """This argument specifies the maximum time that the AutoML process will run for. If both max_runtime_secs and max_models are specified, then the AutoML run will stop as soon as it hits either of these limits. If neither max_runtime_secs nor max_models are specified, then max_runtime_secs defaults to 3600 seconds (1 hour).""")
protected val maxRuntimeSecsPerModel = doubleParam(
name = "maxRuntimeSecsPerModel",
doc = """Maximum time to spend on each individual model (optional). Note that models constrained by a time budget are not guaranteed reproducible.""")
protected val stoppingRounds = intParam(
name = "stoppingRounds",
doc = """Early stopping based on convergence of stopping_metric. Stop if simple moving average of length k of the stopping_metric does not improve for k:=stopping_rounds scoring events (0 to disable).""")
protected val stoppingMetric = stringParam(
name = "stoppingMetric",
doc = """Metric to use for early stopping (AUTO: logloss for classification, deviance for regression). Possible values are ``"AUTO"``, ``"deviance"``, ``"logloss"``, ``"MSE"``, ``"RMSE"``, ``"MAE"``, ``"RMSLE"``, ``"AUC"``, ``"AUCPR"``, ``"lift_top_group"``, ``"misclassification"``, ``"mean_per_class_error"``, ``"anomaly_score"``, ``"AUUC"``, ``"ATE"``, ``"ATT"``, ``"ATC"``, ``"qini"``, ``"custom"``, ``"custom_increasing"``.""")
protected val stoppingTolerance = doubleParam(
name = "stoppingTolerance",
doc = """Relative tolerance for metric-based stopping criterion (stop if relative improvement is not at least this much).""")
//
// Default values
//
setDefault(
seed -> -1L,
maxModels -> 0,
maxRuntimeSecs -> 0.0,
maxRuntimeSecsPerModel -> 0.0,
stoppingRounds -> 3,
stoppingMetric -> StoppingMetric.AUTO.name(),
stoppingTolerance -> -1.0)
//
// Getters
//
def getSeed(): Long = $(seed)
def getMaxModels(): Int = $(maxModels)
def getMaxRuntimeSecs(): Double = $(maxRuntimeSecs)
def getMaxRuntimeSecsPerModel(): Double = $(maxRuntimeSecsPerModel)
def getStoppingRounds(): Int = $(stoppingRounds)
def getStoppingMetric(): String = $(stoppingMetric)
def getStoppingTolerance(): Double = $(stoppingTolerance)
//
// Setters
//
def setSeed(value: Long): this.type = {
set(seed, value)
}
def setMaxModels(value: Int): this.type = {
set(maxModels, value)
}
def setMaxRuntimeSecs(value: Double): this.type = {
set(maxRuntimeSecs, value)
}
def setMaxRuntimeSecsPerModel(value: Double): this.type = {
set(maxRuntimeSecsPerModel, value)
}
def setStoppingRounds(value: Int): this.type = {
set(stoppingRounds, value)
}
def setStoppingMetric(value: String): this.type = {
val validated = EnumParamValidator.getValidatedEnumValue[StoppingMetric](value)
set(stoppingMetric, validated)
}
def setStoppingTolerance(value: Double): this.type = {
set(stoppingTolerance, value)
}
override private[sparkling] def getH2OAlgorithmParams(trainingFrame: H2OFrame): Map[String, Any] = {
super.getH2OAlgorithmParams(trainingFrame) ++ getH2OAutoMLStoppingCriteriaParams(trainingFrame)
}
private[sparkling] def getH2OAutoMLStoppingCriteriaParams(trainingFrame: H2OFrame): Map[String, Any] = {
Map(
"seed" -> getSeed(),
"max_models" -> getMaxModels(),
"max_runtime_secs" -> getMaxRuntimeSecs(),
"max_runtime_secs_per_model" -> getMaxRuntimeSecsPerModel(),
"stopping_rounds" -> getStoppingRounds(),
"stopping_metric" -> getStoppingMetric(),
"stopping_tolerance" -> getStoppingTolerance())
}
override private[sparkling] def getSWtoH2OParamNameMap(): Map[String, String] = {
super.getSWtoH2OParamNameMap() ++
Map(
"seed" -> "seed",
"maxModels" -> "max_models",
"maxRuntimeSecs" -> "max_runtime_secs",
"maxRuntimeSecsPerModel" -> "max_runtime_secs_per_model",
"stoppingRounds" -> "stopping_rounds",
"stoppingMetric" -> "stopping_metric",
"stoppingTolerance" -> "stopping_tolerance")
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy