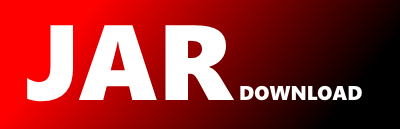
ai.platon.pulsar.common.collect.queue.ConcurrentNonReentrantQueue.kt Maven / Gradle / Ivy
package ai.platon.pulsar.common.collect.queue
import java.util.*
import java.util.concurrent.ConcurrentSkipListSet
import kotlin.collections.HashSet
open class ConcurrentNonReentrantQueue: AbstractQueue() {
private val set = ConcurrentSkipListSet()
private val historyHash = HashSet()
open fun count(e: E) = if (historyHash.contains(e.hashCode())) 1 else 0
override fun add(element: E) = offer(element)
override fun offer(e: E): Boolean {
val hashCode = e.hashCode()
synchronized(this) {
if (!historyHash.contains(hashCode)) {
historyHash.add(hashCode)
return set.add(e)
}
}
return false
}
override fun iterator(): MutableIterator = set.iterator()
override fun peek(): E? = set.firstOrNull()
override fun poll(): E? = set.pollFirst()
override val size: Int get() = set.size
}
typealias ConcurrentUniqueQueue = ConcurrentNonReentrantQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy