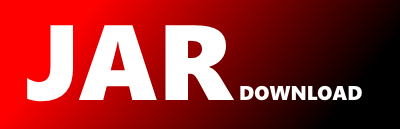
ai.promoted.proto.common.Device Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deliveryclient Show documentation
Show all versions of deliveryclient Show documentation
A Java Client to contact the Promoted.ai Delivery API.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: proto/common/common.proto
package ai.promoted.proto.common;
/**
* Protobuf type {@code common.Device}
*/
public final class Device extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:common.Device)
DeviceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Device.newBuilder() to construct.
private Device(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Device() {
deviceType_ = 0;
brand_ = "";
manufacturer_ = "";
identifier_ = "";
osVersion_ = "";
ipAddress_ = "";
platformAppVersion_ = "";
promotedMobileSdkVersion_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Device();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Device(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
deviceType_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
brand_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
manufacturer_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
identifier_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
osVersion_ = s;
break;
}
case 50: {
ai.promoted.proto.common.Locale.Builder subBuilder = null;
if (locale_ != null) {
subBuilder = locale_.toBuilder();
}
locale_ = input.readMessage(ai.promoted.proto.common.Locale.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(locale_);
locale_ = subBuilder.buildPartial();
}
break;
}
case 58: {
ai.promoted.proto.common.Screen.Builder subBuilder = null;
if (screen_ != null) {
subBuilder = screen_.toBuilder();
}
screen_ = input.readMessage(ai.promoted.proto.common.Screen.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(screen_);
screen_ = subBuilder.buildPartial();
}
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
ipAddress_ = s;
break;
}
case 74: {
ai.promoted.proto.common.Location.Builder subBuilder = null;
if (location_ != null) {
subBuilder = location_.toBuilder();
}
location_ = input.readMessage(ai.promoted.proto.common.Location.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(location_);
location_ = subBuilder.buildPartial();
}
break;
}
case 82: {
ai.promoted.proto.common.Browser.Builder subBuilder = null;
if (browser_ != null) {
subBuilder = browser_.toBuilder();
}
browser_ = input.readMessage(ai.promoted.proto.common.Browser.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(browser_);
browser_ = subBuilder.buildPartial();
}
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
platformAppVersion_ = s;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
promotedMobileSdkVersion_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.common.CommonProto.internal_static_common_Device_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.common.CommonProto.internal_static_common_Device_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.common.Device.class, ai.promoted.proto.common.Device.Builder.class);
}
public static final int DEVICE_TYPE_FIELD_NUMBER = 1;
private int deviceType_;
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @return The enum numeric value on the wire for deviceType.
*/
@java.lang.Override public int getDeviceTypeValue() {
return deviceType_;
}
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @return The deviceType.
*/
@java.lang.Override public ai.promoted.proto.common.DeviceType getDeviceType() {
@SuppressWarnings("deprecation")
ai.promoted.proto.common.DeviceType result = ai.promoted.proto.common.DeviceType.valueOf(deviceType_);
return result == null ? ai.promoted.proto.common.DeviceType.UNRECOGNIZED : result;
}
public static final int BRAND_FIELD_NUMBER = 2;
private volatile java.lang.Object brand_;
/**
* string brand = 2 [json_name = "brand"];
* @return The brand.
*/
@java.lang.Override
public java.lang.String getBrand() {
java.lang.Object ref = brand_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
brand_ = s;
return s;
}
}
/**
* string brand = 2 [json_name = "brand"];
* @return The bytes for brand.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBrandBytes() {
java.lang.Object ref = brand_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
brand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MANUFACTURER_FIELD_NUMBER = 3;
private volatile java.lang.Object manufacturer_;
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @return The manufacturer.
*/
@java.lang.Override
public java.lang.String getManufacturer() {
java.lang.Object ref = manufacturer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
manufacturer_ = s;
return s;
}
}
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @return The bytes for manufacturer.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getManufacturerBytes() {
java.lang.Object ref = manufacturer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
manufacturer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IDENTIFIER_FIELD_NUMBER = 4;
private volatile java.lang.Object identifier_;
/**
* string identifier = 4 [json_name = "identifier"];
* @return The identifier.
*/
@java.lang.Override
public java.lang.String getIdentifier() {
java.lang.Object ref = identifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identifier_ = s;
return s;
}
}
/**
* string identifier = 4 [json_name = "identifier"];
* @return The bytes for identifier.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdentifierBytes() {
java.lang.Object ref = identifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OS_VERSION_FIELD_NUMBER = 5;
private volatile java.lang.Object osVersion_;
/**
* string os_version = 5 [json_name = "osVersion"];
* @return The osVersion.
*/
@java.lang.Override
public java.lang.String getOsVersion() {
java.lang.Object ref = osVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
osVersion_ = s;
return s;
}
}
/**
* string os_version = 5 [json_name = "osVersion"];
* @return The bytes for osVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOsVersionBytes() {
java.lang.Object ref = osVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCALE_FIELD_NUMBER = 6;
private ai.promoted.proto.common.Locale locale_;
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
* @return Whether the locale field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasLocale() {
return locale_ != null;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
* @return The locale.
*/
@java.lang.Override
@java.lang.Deprecated public ai.promoted.proto.common.Locale getLocale() {
return locale_ == null ? ai.promoted.proto.common.Locale.getDefaultInstance() : locale_;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public ai.promoted.proto.common.LocaleOrBuilder getLocaleOrBuilder() {
return getLocale();
}
public static final int SCREEN_FIELD_NUMBER = 7;
private ai.promoted.proto.common.Screen screen_;
/**
* .common.Screen screen = 7 [json_name = "screen"];
* @return Whether the screen field is set.
*/
@java.lang.Override
public boolean hasScreen() {
return screen_ != null;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
* @return The screen.
*/
@java.lang.Override
public ai.promoted.proto.common.Screen getScreen() {
return screen_ == null ? ai.promoted.proto.common.Screen.getDefaultInstance() : screen_;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
@java.lang.Override
public ai.promoted.proto.common.ScreenOrBuilder getScreenOrBuilder() {
return getScreen();
}
public static final int IP_ADDRESS_FIELD_NUMBER = 8;
private volatile java.lang.Object ipAddress_;
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @return The ipAddress.
*/
@java.lang.Override
public java.lang.String getIpAddress() {
java.lang.Object ref = ipAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipAddress_ = s;
return s;
}
}
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @return The bytes for ipAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIpAddressBytes() {
java.lang.Object ref = ipAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ipAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCATION_FIELD_NUMBER = 9;
private ai.promoted.proto.common.Location location_;
/**
* .common.Location location = 9 [json_name = "location"];
* @return Whether the location field is set.
*/
@java.lang.Override
public boolean hasLocation() {
return location_ != null;
}
/**
* .common.Location location = 9 [json_name = "location"];
* @return The location.
*/
@java.lang.Override
public ai.promoted.proto.common.Location getLocation() {
return location_ == null ? ai.promoted.proto.common.Location.getDefaultInstance() : location_;
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
@java.lang.Override
public ai.promoted.proto.common.LocationOrBuilder getLocationOrBuilder() {
return getLocation();
}
public static final int BROWSER_FIELD_NUMBER = 10;
private ai.promoted.proto.common.Browser browser_;
/**
* .common.Browser browser = 10 [json_name = "browser"];
* @return Whether the browser field is set.
*/
@java.lang.Override
public boolean hasBrowser() {
return browser_ != null;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
* @return The browser.
*/
@java.lang.Override
public ai.promoted.proto.common.Browser getBrowser() {
return browser_ == null ? ai.promoted.proto.common.Browser.getDefaultInstance() : browser_;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
@java.lang.Override
public ai.promoted.proto.common.BrowserOrBuilder getBrowserOrBuilder() {
return getBrowser();
}
public static final int PLATFORM_APP_VERSION_FIELD_NUMBER = 11;
private volatile java.lang.Object platformAppVersion_;
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @return The platformAppVersion.
*/
@java.lang.Override
public java.lang.String getPlatformAppVersion() {
java.lang.Object ref = platformAppVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
platformAppVersion_ = s;
return s;
}
}
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @return The bytes for platformAppVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPlatformAppVersionBytes() {
java.lang.Object ref = platformAppVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platformAppVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROMOTED_MOBILE_SDK_VERSION_FIELD_NUMBER = 12;
private volatile java.lang.Object promotedMobileSdkVersion_;
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @return The promotedMobileSdkVersion.
*/
@java.lang.Override
public java.lang.String getPromotedMobileSdkVersion() {
java.lang.Object ref = promotedMobileSdkVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
promotedMobileSdkVersion_ = s;
return s;
}
}
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @return The bytes for promotedMobileSdkVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPromotedMobileSdkVersionBytes() {
java.lang.Object ref = promotedMobileSdkVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
promotedMobileSdkVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (deviceType_ != ai.promoted.proto.common.DeviceType.UNKNOWN_DEVICE_TYPE.getNumber()) {
output.writeEnum(1, deviceType_);
}
if (!getBrandBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, brand_);
}
if (!getManufacturerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, manufacturer_);
}
if (!getIdentifierBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, identifier_);
}
if (!getOsVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, osVersion_);
}
if (locale_ != null) {
output.writeMessage(6, getLocale());
}
if (screen_ != null) {
output.writeMessage(7, getScreen());
}
if (!getIpAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, ipAddress_);
}
if (location_ != null) {
output.writeMessage(9, getLocation());
}
if (browser_ != null) {
output.writeMessage(10, getBrowser());
}
if (!getPlatformAppVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, platformAppVersion_);
}
if (!getPromotedMobileSdkVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, promotedMobileSdkVersion_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (deviceType_ != ai.promoted.proto.common.DeviceType.UNKNOWN_DEVICE_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, deviceType_);
}
if (!getBrandBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, brand_);
}
if (!getManufacturerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, manufacturer_);
}
if (!getIdentifierBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, identifier_);
}
if (!getOsVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, osVersion_);
}
if (locale_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getLocale());
}
if (screen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getScreen());
}
if (!getIpAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, ipAddress_);
}
if (location_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getLocation());
}
if (browser_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getBrowser());
}
if (!getPlatformAppVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, platformAppVersion_);
}
if (!getPromotedMobileSdkVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, promotedMobileSdkVersion_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ai.promoted.proto.common.Device)) {
return super.equals(obj);
}
ai.promoted.proto.common.Device other = (ai.promoted.proto.common.Device) obj;
if (deviceType_ != other.deviceType_) return false;
if (!getBrand()
.equals(other.getBrand())) return false;
if (!getManufacturer()
.equals(other.getManufacturer())) return false;
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
if (!getOsVersion()
.equals(other.getOsVersion())) return false;
if (hasLocale() != other.hasLocale()) return false;
if (hasLocale()) {
if (!getLocale()
.equals(other.getLocale())) return false;
}
if (hasScreen() != other.hasScreen()) return false;
if (hasScreen()) {
if (!getScreen()
.equals(other.getScreen())) return false;
}
if (!getIpAddress()
.equals(other.getIpAddress())) return false;
if (hasLocation() != other.hasLocation()) return false;
if (hasLocation()) {
if (!getLocation()
.equals(other.getLocation())) return false;
}
if (hasBrowser() != other.hasBrowser()) return false;
if (hasBrowser()) {
if (!getBrowser()
.equals(other.getBrowser())) return false;
}
if (!getPlatformAppVersion()
.equals(other.getPlatformAppVersion())) return false;
if (!getPromotedMobileSdkVersion()
.equals(other.getPromotedMobileSdkVersion())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DEVICE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + deviceType_;
hash = (37 * hash) + BRAND_FIELD_NUMBER;
hash = (53 * hash) + getBrand().hashCode();
hash = (37 * hash) + MANUFACTURER_FIELD_NUMBER;
hash = (53 * hash) + getManufacturer().hashCode();
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
hash = (37 * hash) + OS_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getOsVersion().hashCode();
if (hasLocale()) {
hash = (37 * hash) + LOCALE_FIELD_NUMBER;
hash = (53 * hash) + getLocale().hashCode();
}
if (hasScreen()) {
hash = (37 * hash) + SCREEN_FIELD_NUMBER;
hash = (53 * hash) + getScreen().hashCode();
}
hash = (37 * hash) + IP_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getIpAddress().hashCode();
if (hasLocation()) {
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
}
if (hasBrowser()) {
hash = (37 * hash) + BROWSER_FIELD_NUMBER;
hash = (53 * hash) + getBrowser().hashCode();
}
hash = (37 * hash) + PLATFORM_APP_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getPlatformAppVersion().hashCode();
hash = (37 * hash) + PROMOTED_MOBILE_SDK_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getPromotedMobileSdkVersion().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ai.promoted.proto.common.Device parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.common.Device parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.common.Device parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.common.Device parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.common.Device parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.common.Device parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.common.Device parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.common.Device parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.common.Device parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ai.promoted.proto.common.Device parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.common.Device parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.common.Device parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ai.promoted.proto.common.Device prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code common.Device}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:common.Device)
ai.promoted.proto.common.DeviceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.common.CommonProto.internal_static_common_Device_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.common.CommonProto.internal_static_common_Device_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.common.Device.class, ai.promoted.proto.common.Device.Builder.class);
}
// Construct using ai.promoted.proto.common.Device.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
deviceType_ = 0;
brand_ = "";
manufacturer_ = "";
identifier_ = "";
osVersion_ = "";
if (localeBuilder_ == null) {
locale_ = null;
} else {
locale_ = null;
localeBuilder_ = null;
}
if (screenBuilder_ == null) {
screen_ = null;
} else {
screen_ = null;
screenBuilder_ = null;
}
ipAddress_ = "";
if (locationBuilder_ == null) {
location_ = null;
} else {
location_ = null;
locationBuilder_ = null;
}
if (browserBuilder_ == null) {
browser_ = null;
} else {
browser_ = null;
browserBuilder_ = null;
}
platformAppVersion_ = "";
promotedMobileSdkVersion_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ai.promoted.proto.common.CommonProto.internal_static_common_Device_descriptor;
}
@java.lang.Override
public ai.promoted.proto.common.Device getDefaultInstanceForType() {
return ai.promoted.proto.common.Device.getDefaultInstance();
}
@java.lang.Override
public ai.promoted.proto.common.Device build() {
ai.promoted.proto.common.Device result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ai.promoted.proto.common.Device buildPartial() {
ai.promoted.proto.common.Device result = new ai.promoted.proto.common.Device(this);
result.deviceType_ = deviceType_;
result.brand_ = brand_;
result.manufacturer_ = manufacturer_;
result.identifier_ = identifier_;
result.osVersion_ = osVersion_;
if (localeBuilder_ == null) {
result.locale_ = locale_;
} else {
result.locale_ = localeBuilder_.build();
}
if (screenBuilder_ == null) {
result.screen_ = screen_;
} else {
result.screen_ = screenBuilder_.build();
}
result.ipAddress_ = ipAddress_;
if (locationBuilder_ == null) {
result.location_ = location_;
} else {
result.location_ = locationBuilder_.build();
}
if (browserBuilder_ == null) {
result.browser_ = browser_;
} else {
result.browser_ = browserBuilder_.build();
}
result.platformAppVersion_ = platformAppVersion_;
result.promotedMobileSdkVersion_ = promotedMobileSdkVersion_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ai.promoted.proto.common.Device) {
return mergeFrom((ai.promoted.proto.common.Device)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ai.promoted.proto.common.Device other) {
if (other == ai.promoted.proto.common.Device.getDefaultInstance()) return this;
if (other.deviceType_ != 0) {
setDeviceTypeValue(other.getDeviceTypeValue());
}
if (!other.getBrand().isEmpty()) {
brand_ = other.brand_;
onChanged();
}
if (!other.getManufacturer().isEmpty()) {
manufacturer_ = other.manufacturer_;
onChanged();
}
if (!other.getIdentifier().isEmpty()) {
identifier_ = other.identifier_;
onChanged();
}
if (!other.getOsVersion().isEmpty()) {
osVersion_ = other.osVersion_;
onChanged();
}
if (other.hasLocale()) {
mergeLocale(other.getLocale());
}
if (other.hasScreen()) {
mergeScreen(other.getScreen());
}
if (!other.getIpAddress().isEmpty()) {
ipAddress_ = other.ipAddress_;
onChanged();
}
if (other.hasLocation()) {
mergeLocation(other.getLocation());
}
if (other.hasBrowser()) {
mergeBrowser(other.getBrowser());
}
if (!other.getPlatformAppVersion().isEmpty()) {
platformAppVersion_ = other.platformAppVersion_;
onChanged();
}
if (!other.getPromotedMobileSdkVersion().isEmpty()) {
promotedMobileSdkVersion_ = other.promotedMobileSdkVersion_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ai.promoted.proto.common.Device parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ai.promoted.proto.common.Device) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int deviceType_ = 0;
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @return The enum numeric value on the wire for deviceType.
*/
@java.lang.Override public int getDeviceTypeValue() {
return deviceType_;
}
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @param value The enum numeric value on the wire for deviceType to set.
* @return This builder for chaining.
*/
public Builder setDeviceTypeValue(int value) {
deviceType_ = value;
onChanged();
return this;
}
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @return The deviceType.
*/
@java.lang.Override
public ai.promoted.proto.common.DeviceType getDeviceType() {
@SuppressWarnings("deprecation")
ai.promoted.proto.common.DeviceType result = ai.promoted.proto.common.DeviceType.valueOf(deviceType_);
return result == null ? ai.promoted.proto.common.DeviceType.UNRECOGNIZED : result;
}
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @param value The deviceType to set.
* @return This builder for chaining.
*/
public Builder setDeviceType(ai.promoted.proto.common.DeviceType value) {
if (value == null) {
throw new NullPointerException();
}
deviceType_ = value.getNumber();
onChanged();
return this;
}
/**
* .common.DeviceType device_type = 1 [json_name = "deviceType"];
* @return This builder for chaining.
*/
public Builder clearDeviceType() {
deviceType_ = 0;
onChanged();
return this;
}
private java.lang.Object brand_ = "";
/**
* string brand = 2 [json_name = "brand"];
* @return The brand.
*/
public java.lang.String getBrand() {
java.lang.Object ref = brand_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
brand_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string brand = 2 [json_name = "brand"];
* @return The bytes for brand.
*/
public com.google.protobuf.ByteString
getBrandBytes() {
java.lang.Object ref = brand_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
brand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string brand = 2 [json_name = "brand"];
* @param value The brand to set.
* @return This builder for chaining.
*/
public Builder setBrand(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
brand_ = value;
onChanged();
return this;
}
/**
* string brand = 2 [json_name = "brand"];
* @return This builder for chaining.
*/
public Builder clearBrand() {
brand_ = getDefaultInstance().getBrand();
onChanged();
return this;
}
/**
* string brand = 2 [json_name = "brand"];
* @param value The bytes for brand to set.
* @return This builder for chaining.
*/
public Builder setBrandBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
brand_ = value;
onChanged();
return this;
}
private java.lang.Object manufacturer_ = "";
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @return The manufacturer.
*/
public java.lang.String getManufacturer() {
java.lang.Object ref = manufacturer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
manufacturer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @return The bytes for manufacturer.
*/
public com.google.protobuf.ByteString
getManufacturerBytes() {
java.lang.Object ref = manufacturer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
manufacturer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @param value The manufacturer to set.
* @return This builder for chaining.
*/
public Builder setManufacturer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
manufacturer_ = value;
onChanged();
return this;
}
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @return This builder for chaining.
*/
public Builder clearManufacturer() {
manufacturer_ = getDefaultInstance().getManufacturer();
onChanged();
return this;
}
/**
* string manufacturer = 3 [json_name = "manufacturer"];
* @param value The bytes for manufacturer to set.
* @return This builder for chaining.
*/
public Builder setManufacturerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
manufacturer_ = value;
onChanged();
return this;
}
private java.lang.Object identifier_ = "";
/**
* string identifier = 4 [json_name = "identifier"];
* @return The identifier.
*/
public java.lang.String getIdentifier() {
java.lang.Object ref = identifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string identifier = 4 [json_name = "identifier"];
* @return The bytes for identifier.
*/
public com.google.protobuf.ByteString
getIdentifierBytes() {
java.lang.Object ref = identifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string identifier = 4 [json_name = "identifier"];
* @param value The identifier to set.
* @return This builder for chaining.
*/
public Builder setIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
return this;
}
/**
* string identifier = 4 [json_name = "identifier"];
* @return This builder for chaining.
*/
public Builder clearIdentifier() {
identifier_ = getDefaultInstance().getIdentifier();
onChanged();
return this;
}
/**
* string identifier = 4 [json_name = "identifier"];
* @param value The bytes for identifier to set.
* @return This builder for chaining.
*/
public Builder setIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
identifier_ = value;
onChanged();
return this;
}
private java.lang.Object osVersion_ = "";
/**
* string os_version = 5 [json_name = "osVersion"];
* @return The osVersion.
*/
public java.lang.String getOsVersion() {
java.lang.Object ref = osVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
osVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string os_version = 5 [json_name = "osVersion"];
* @return The bytes for osVersion.
*/
public com.google.protobuf.ByteString
getOsVersionBytes() {
java.lang.Object ref = osVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string os_version = 5 [json_name = "osVersion"];
* @param value The osVersion to set.
* @return This builder for chaining.
*/
public Builder setOsVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
osVersion_ = value;
onChanged();
return this;
}
/**
* string os_version = 5 [json_name = "osVersion"];
* @return This builder for chaining.
*/
public Builder clearOsVersion() {
osVersion_ = getDefaultInstance().getOsVersion();
onChanged();
return this;
}
/**
* string os_version = 5 [json_name = "osVersion"];
* @param value The bytes for osVersion to set.
* @return This builder for chaining.
*/
public Builder setOsVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
osVersion_ = value;
onChanged();
return this;
}
private ai.promoted.proto.common.Locale locale_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Locale, ai.promoted.proto.common.Locale.Builder, ai.promoted.proto.common.LocaleOrBuilder> localeBuilder_;
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
* @return Whether the locale field is set.
*/
@java.lang.Deprecated public boolean hasLocale() {
return localeBuilder_ != null || locale_ != null;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
* @return The locale.
*/
@java.lang.Deprecated public ai.promoted.proto.common.Locale getLocale() {
if (localeBuilder_ == null) {
return locale_ == null ? ai.promoted.proto.common.Locale.getDefaultInstance() : locale_;
} else {
return localeBuilder_.getMessage();
}
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Deprecated public Builder setLocale(ai.promoted.proto.common.Locale value) {
if (localeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
locale_ = value;
onChanged();
} else {
localeBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Deprecated public Builder setLocale(
ai.promoted.proto.common.Locale.Builder builderForValue) {
if (localeBuilder_ == null) {
locale_ = builderForValue.build();
onChanged();
} else {
localeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Deprecated public Builder mergeLocale(ai.promoted.proto.common.Locale value) {
if (localeBuilder_ == null) {
if (locale_ != null) {
locale_ =
ai.promoted.proto.common.Locale.newBuilder(locale_).mergeFrom(value).buildPartial();
} else {
locale_ = value;
}
onChanged();
} else {
localeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Deprecated public Builder clearLocale() {
if (localeBuilder_ == null) {
locale_ = null;
onChanged();
} else {
locale_ = null;
localeBuilder_ = null;
}
return this;
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Deprecated public ai.promoted.proto.common.Locale.Builder getLocaleBuilder() {
onChanged();
return getLocaleFieldBuilder().getBuilder();
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
@java.lang.Deprecated public ai.promoted.proto.common.LocaleOrBuilder getLocaleOrBuilder() {
if (localeBuilder_ != null) {
return localeBuilder_.getMessageOrBuilder();
} else {
return locale_ == null ?
ai.promoted.proto.common.Locale.getDefaultInstance() : locale_;
}
}
/**
* .common.Locale locale = 6 [json_name = "locale", deprecated = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Locale, ai.promoted.proto.common.Locale.Builder, ai.promoted.proto.common.LocaleOrBuilder>
getLocaleFieldBuilder() {
if (localeBuilder_ == null) {
localeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Locale, ai.promoted.proto.common.Locale.Builder, ai.promoted.proto.common.LocaleOrBuilder>(
getLocale(),
getParentForChildren(),
isClean());
locale_ = null;
}
return localeBuilder_;
}
private ai.promoted.proto.common.Screen screen_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Screen, ai.promoted.proto.common.Screen.Builder, ai.promoted.proto.common.ScreenOrBuilder> screenBuilder_;
/**
* .common.Screen screen = 7 [json_name = "screen"];
* @return Whether the screen field is set.
*/
public boolean hasScreen() {
return screenBuilder_ != null || screen_ != null;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
* @return The screen.
*/
public ai.promoted.proto.common.Screen getScreen() {
if (screenBuilder_ == null) {
return screen_ == null ? ai.promoted.proto.common.Screen.getDefaultInstance() : screen_;
} else {
return screenBuilder_.getMessage();
}
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
public Builder setScreen(ai.promoted.proto.common.Screen value) {
if (screenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
screen_ = value;
onChanged();
} else {
screenBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
public Builder setScreen(
ai.promoted.proto.common.Screen.Builder builderForValue) {
if (screenBuilder_ == null) {
screen_ = builderForValue.build();
onChanged();
} else {
screenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
public Builder mergeScreen(ai.promoted.proto.common.Screen value) {
if (screenBuilder_ == null) {
if (screen_ != null) {
screen_ =
ai.promoted.proto.common.Screen.newBuilder(screen_).mergeFrom(value).buildPartial();
} else {
screen_ = value;
}
onChanged();
} else {
screenBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
public Builder clearScreen() {
if (screenBuilder_ == null) {
screen_ = null;
onChanged();
} else {
screen_ = null;
screenBuilder_ = null;
}
return this;
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
public ai.promoted.proto.common.Screen.Builder getScreenBuilder() {
onChanged();
return getScreenFieldBuilder().getBuilder();
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
public ai.promoted.proto.common.ScreenOrBuilder getScreenOrBuilder() {
if (screenBuilder_ != null) {
return screenBuilder_.getMessageOrBuilder();
} else {
return screen_ == null ?
ai.promoted.proto.common.Screen.getDefaultInstance() : screen_;
}
}
/**
* .common.Screen screen = 7 [json_name = "screen"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Screen, ai.promoted.proto.common.Screen.Builder, ai.promoted.proto.common.ScreenOrBuilder>
getScreenFieldBuilder() {
if (screenBuilder_ == null) {
screenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Screen, ai.promoted.proto.common.Screen.Builder, ai.promoted.proto.common.ScreenOrBuilder>(
getScreen(),
getParentForChildren(),
isClean());
screen_ = null;
}
return screenBuilder_;
}
private java.lang.Object ipAddress_ = "";
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @return The ipAddress.
*/
public java.lang.String getIpAddress() {
java.lang.Object ref = ipAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @return The bytes for ipAddress.
*/
public com.google.protobuf.ByteString
getIpAddressBytes() {
java.lang.Object ref = ipAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ipAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @param value The ipAddress to set.
* @return This builder for chaining.
*/
public Builder setIpAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ipAddress_ = value;
onChanged();
return this;
}
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @return This builder for chaining.
*/
public Builder clearIpAddress() {
ipAddress_ = getDefaultInstance().getIpAddress();
onChanged();
return this;
}
/**
* string ip_address = 8 [json_name = "ipAddress"];
* @param value The bytes for ipAddress to set.
* @return This builder for chaining.
*/
public Builder setIpAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ipAddress_ = value;
onChanged();
return this;
}
private ai.promoted.proto.common.Location location_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Location, ai.promoted.proto.common.Location.Builder, ai.promoted.proto.common.LocationOrBuilder> locationBuilder_;
/**
* .common.Location location = 9 [json_name = "location"];
* @return Whether the location field is set.
*/
public boolean hasLocation() {
return locationBuilder_ != null || location_ != null;
}
/**
* .common.Location location = 9 [json_name = "location"];
* @return The location.
*/
public ai.promoted.proto.common.Location getLocation() {
if (locationBuilder_ == null) {
return location_ == null ? ai.promoted.proto.common.Location.getDefaultInstance() : location_;
} else {
return locationBuilder_.getMessage();
}
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
public Builder setLocation(ai.promoted.proto.common.Location value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
location_ = value;
onChanged();
} else {
locationBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
public Builder setLocation(
ai.promoted.proto.common.Location.Builder builderForValue) {
if (locationBuilder_ == null) {
location_ = builderForValue.build();
onChanged();
} else {
locationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
public Builder mergeLocation(ai.promoted.proto.common.Location value) {
if (locationBuilder_ == null) {
if (location_ != null) {
location_ =
ai.promoted.proto.common.Location.newBuilder(location_).mergeFrom(value).buildPartial();
} else {
location_ = value;
}
onChanged();
} else {
locationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
public Builder clearLocation() {
if (locationBuilder_ == null) {
location_ = null;
onChanged();
} else {
location_ = null;
locationBuilder_ = null;
}
return this;
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
public ai.promoted.proto.common.Location.Builder getLocationBuilder() {
onChanged();
return getLocationFieldBuilder().getBuilder();
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
public ai.promoted.proto.common.LocationOrBuilder getLocationOrBuilder() {
if (locationBuilder_ != null) {
return locationBuilder_.getMessageOrBuilder();
} else {
return location_ == null ?
ai.promoted.proto.common.Location.getDefaultInstance() : location_;
}
}
/**
* .common.Location location = 9 [json_name = "location"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Location, ai.promoted.proto.common.Location.Builder, ai.promoted.proto.common.LocationOrBuilder>
getLocationFieldBuilder() {
if (locationBuilder_ == null) {
locationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Location, ai.promoted.proto.common.Location.Builder, ai.promoted.proto.common.LocationOrBuilder>(
getLocation(),
getParentForChildren(),
isClean());
location_ = null;
}
return locationBuilder_;
}
private ai.promoted.proto.common.Browser browser_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Browser, ai.promoted.proto.common.Browser.Builder, ai.promoted.proto.common.BrowserOrBuilder> browserBuilder_;
/**
* .common.Browser browser = 10 [json_name = "browser"];
* @return Whether the browser field is set.
*/
public boolean hasBrowser() {
return browserBuilder_ != null || browser_ != null;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
* @return The browser.
*/
public ai.promoted.proto.common.Browser getBrowser() {
if (browserBuilder_ == null) {
return browser_ == null ? ai.promoted.proto.common.Browser.getDefaultInstance() : browser_;
} else {
return browserBuilder_.getMessage();
}
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
public Builder setBrowser(ai.promoted.proto.common.Browser value) {
if (browserBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
browser_ = value;
onChanged();
} else {
browserBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
public Builder setBrowser(
ai.promoted.proto.common.Browser.Builder builderForValue) {
if (browserBuilder_ == null) {
browser_ = builderForValue.build();
onChanged();
} else {
browserBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
public Builder mergeBrowser(ai.promoted.proto.common.Browser value) {
if (browserBuilder_ == null) {
if (browser_ != null) {
browser_ =
ai.promoted.proto.common.Browser.newBuilder(browser_).mergeFrom(value).buildPartial();
} else {
browser_ = value;
}
onChanged();
} else {
browserBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
public Builder clearBrowser() {
if (browserBuilder_ == null) {
browser_ = null;
onChanged();
} else {
browser_ = null;
browserBuilder_ = null;
}
return this;
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
public ai.promoted.proto.common.Browser.Builder getBrowserBuilder() {
onChanged();
return getBrowserFieldBuilder().getBuilder();
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
public ai.promoted.proto.common.BrowserOrBuilder getBrowserOrBuilder() {
if (browserBuilder_ != null) {
return browserBuilder_.getMessageOrBuilder();
} else {
return browser_ == null ?
ai.promoted.proto.common.Browser.getDefaultInstance() : browser_;
}
}
/**
* .common.Browser browser = 10 [json_name = "browser"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Browser, ai.promoted.proto.common.Browser.Builder, ai.promoted.proto.common.BrowserOrBuilder>
getBrowserFieldBuilder() {
if (browserBuilder_ == null) {
browserBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Browser, ai.promoted.proto.common.Browser.Builder, ai.promoted.proto.common.BrowserOrBuilder>(
getBrowser(),
getParentForChildren(),
isClean());
browser_ = null;
}
return browserBuilder_;
}
private java.lang.Object platformAppVersion_ = "";
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @return The platformAppVersion.
*/
public java.lang.String getPlatformAppVersion() {
java.lang.Object ref = platformAppVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
platformAppVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @return The bytes for platformAppVersion.
*/
public com.google.protobuf.ByteString
getPlatformAppVersionBytes() {
java.lang.Object ref = platformAppVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platformAppVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @param value The platformAppVersion to set.
* @return This builder for chaining.
*/
public Builder setPlatformAppVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
platformAppVersion_ = value;
onChanged();
return this;
}
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @return This builder for chaining.
*/
public Builder clearPlatformAppVersion() {
platformAppVersion_ = getDefaultInstance().getPlatformAppVersion();
onChanged();
return this;
}
/**
* string platform_app_version = 11 [json_name = "platformAppVersion"];
* @param value The bytes for platformAppVersion to set.
* @return This builder for chaining.
*/
public Builder setPlatformAppVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
platformAppVersion_ = value;
onChanged();
return this;
}
private java.lang.Object promotedMobileSdkVersion_ = "";
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @return The promotedMobileSdkVersion.
*/
public java.lang.String getPromotedMobileSdkVersion() {
java.lang.Object ref = promotedMobileSdkVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
promotedMobileSdkVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @return The bytes for promotedMobileSdkVersion.
*/
public com.google.protobuf.ByteString
getPromotedMobileSdkVersionBytes() {
java.lang.Object ref = promotedMobileSdkVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
promotedMobileSdkVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @param value The promotedMobileSdkVersion to set.
* @return This builder for chaining.
*/
public Builder setPromotedMobileSdkVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
promotedMobileSdkVersion_ = value;
onChanged();
return this;
}
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @return This builder for chaining.
*/
public Builder clearPromotedMobileSdkVersion() {
promotedMobileSdkVersion_ = getDefaultInstance().getPromotedMobileSdkVersion();
onChanged();
return this;
}
/**
* string promoted_mobile_sdk_version = 12 [json_name = "promotedMobileSdkVersion"];
* @param value The bytes for promotedMobileSdkVersion to set.
* @return This builder for chaining.
*/
public Builder setPromotedMobileSdkVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
promotedMobileSdkVersion_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:common.Device)
}
// @@protoc_insertion_point(class_scope:common.Device)
private static final ai.promoted.proto.common.Device DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ai.promoted.proto.common.Device();
}
public static ai.promoted.proto.common.Device getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Device parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Device(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ai.promoted.proto.common.Device getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy