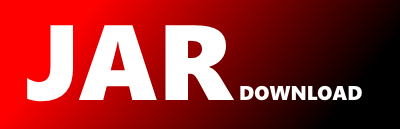
ai.promoted.proto.delivery.Request Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deliveryclient Show documentation
Show all versions of deliveryclient Show documentation
A Java Client to contact the Promoted.ai Delivery API.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: proto/delivery/delivery.proto
package ai.promoted.proto.delivery;
/**
* Protobuf type {@code delivery.Request}
*/
public final class Request extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:delivery.Request)
RequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use Request.newBuilder() to construct.
private Request(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Request() {
requestId_ = "";
viewId_ = "";
autoViewId_ = "";
sessionId_ = "";
clientRequestId_ = "";
useCase_ = 0;
searchQuery_ = "";
insertion_ = java.util.Collections.emptyList();
insertionMatrixHeaders_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Request();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Request(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
platformId_ = input.readUInt64();
break;
}
case 18: {
ai.promoted.proto.common.UserInfo.Builder subBuilder = null;
if (userInfo_ != null) {
subBuilder = userInfo_.toBuilder();
}
userInfo_ = input.readMessage(ai.promoted.proto.common.UserInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(userInfo_);
userInfo_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ai.promoted.proto.common.Timing.Builder subBuilder = null;
if (timing_ != null) {
subBuilder = timing_.toBuilder();
}
timing_ = input.readMessage(ai.promoted.proto.common.Timing.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timing_);
timing_ = subBuilder.buildPartial();
}
break;
}
case 34: {
ai.promoted.proto.common.ClientInfo.Builder subBuilder = null;
if (clientInfo_ != null) {
subBuilder = clientInfo_.toBuilder();
}
clientInfo_ = input.readMessage(ai.promoted.proto.common.ClientInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientInfo_);
clientInfo_ = subBuilder.buildPartial();
}
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
requestId_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
viewId_ = s;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
sessionId_ = s;
break;
}
case 72: {
int rawValue = input.readEnum();
useCase_ = rawValue;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
searchQuery_ = s;
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
insertion_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
insertion_.add(
input.readMessage(ai.promoted.proto.delivery.Insertion.parser(), extensionRegistry));
break;
}
case 106: {
ai.promoted.proto.common.Properties.Builder subBuilder = null;
if (properties_ != null) {
subBuilder = properties_.toBuilder();
}
properties_ = input.readMessage(ai.promoted.proto.common.Properties.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(properties_);
properties_ = subBuilder.buildPartial();
}
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
clientRequestId_ = s;
break;
}
case 138: {
ai.promoted.proto.delivery.Paging.Builder subBuilder = null;
if (paging_ != null) {
subBuilder = paging_.toBuilder();
}
paging_ = input.readMessage(ai.promoted.proto.delivery.Paging.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(paging_);
paging_ = subBuilder.buildPartial();
}
break;
}
case 146: {
ai.promoted.proto.common.Device.Builder subBuilder = null;
if (device_ != null) {
subBuilder = device_.toBuilder();
}
device_ = input.readMessage(ai.promoted.proto.common.Device.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(device_);
device_ = subBuilder.buildPartial();
}
break;
}
case 154: {
java.lang.String s = input.readStringRequireUtf8();
autoViewId_ = s;
break;
}
case 162: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
insertionMatrixHeaders_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
insertionMatrixHeaders_.add(s);
break;
}
case 170: {
com.google.protobuf.ListValue.Builder subBuilder = null;
if (insertionMatrix_ != null) {
subBuilder = insertionMatrix_.toBuilder();
}
insertionMatrix_ = input.readMessage(com.google.protobuf.ListValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(insertionMatrix_);
insertionMatrix_ = subBuilder.buildPartial();
}
break;
}
case 176: {
disablePersonalization_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
insertion_ = java.util.Collections.unmodifiableList(insertion_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
insertionMatrixHeaders_ = insertionMatrixHeaders_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.delivery.Delivery.internal_static_delivery_Request_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.delivery.Delivery.internal_static_delivery_Request_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.delivery.Request.class, ai.promoted.proto.delivery.Request.Builder.class);
}
public static final int PLATFORM_ID_FIELD_NUMBER = 1;
private long platformId_;
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @return The platformId.
*/
@java.lang.Override
public long getPlatformId() {
return platformId_;
}
public static final int USER_INFO_FIELD_NUMBER = 2;
private ai.promoted.proto.common.UserInfo userInfo_;
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return Whether the userInfo field is set.
*/
@java.lang.Override
public boolean hasUserInfo() {
return userInfo_ != null;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return The userInfo.
*/
@java.lang.Override
public ai.promoted.proto.common.UserInfo getUserInfo() {
return userInfo_ == null ? ai.promoted.proto.common.UserInfo.getDefaultInstance() : userInfo_;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
@java.lang.Override
public ai.promoted.proto.common.UserInfoOrBuilder getUserInfoOrBuilder() {
return getUserInfo();
}
public static final int TIMING_FIELD_NUMBER = 3;
private ai.promoted.proto.common.Timing timing_;
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return Whether the timing field is set.
*/
@java.lang.Override
public boolean hasTiming() {
return timing_ != null;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return The timing.
*/
@java.lang.Override
public ai.promoted.proto.common.Timing getTiming() {
return timing_ == null ? ai.promoted.proto.common.Timing.getDefaultInstance() : timing_;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
@java.lang.Override
public ai.promoted.proto.common.TimingOrBuilder getTimingOrBuilder() {
return getTiming();
}
public static final int CLIENT_INFO_FIELD_NUMBER = 4;
private ai.promoted.proto.common.ClientInfo clientInfo_;
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return Whether the clientInfo field is set.
*/
@java.lang.Override
public boolean hasClientInfo() {
return clientInfo_ != null;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return The clientInfo.
*/
@java.lang.Override
public ai.promoted.proto.common.ClientInfo getClientInfo() {
return clientInfo_ == null ? ai.promoted.proto.common.ClientInfo.getDefaultInstance() : clientInfo_;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
@java.lang.Override
public ai.promoted.proto.common.ClientInfoOrBuilder getClientInfoOrBuilder() {
return getClientInfo();
}
public static final int DEVICE_FIELD_NUMBER = 18;
private ai.promoted.proto.common.Device device_;
/**
* .common.Device device = 18 [json_name = "device"];
* @return Whether the device field is set.
*/
@java.lang.Override
public boolean hasDevice() {
return device_ != null;
}
/**
* .common.Device device = 18 [json_name = "device"];
* @return The device.
*/
@java.lang.Override
public ai.promoted.proto.common.Device getDevice() {
return device_ == null ? ai.promoted.proto.common.Device.getDefaultInstance() : device_;
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
@java.lang.Override
public ai.promoted.proto.common.DeviceOrBuilder getDeviceOrBuilder() {
return getDevice();
}
public static final int REQUEST_ID_FIELD_NUMBER = 6;
private volatile java.lang.Object requestId_;
/**
* string request_id = 6 [json_name = "requestId"];
* @return The requestId.
*/
@java.lang.Override
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
}
}
/**
* string request_id = 6 [json_name = "requestId"];
* @return The bytes for requestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VIEW_ID_FIELD_NUMBER = 7;
private volatile java.lang.Object viewId_;
/**
* string view_id = 7 [json_name = "viewId"];
* @return The viewId.
*/
@java.lang.Override
public java.lang.String getViewId() {
java.lang.Object ref = viewId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
viewId_ = s;
return s;
}
}
/**
* string view_id = 7 [json_name = "viewId"];
* @return The bytes for viewId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getViewIdBytes() {
java.lang.Object ref = viewId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
viewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTO_VIEW_ID_FIELD_NUMBER = 19;
private volatile java.lang.Object autoViewId_;
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @return The autoViewId.
*/
@java.lang.Override
public java.lang.String getAutoViewId() {
java.lang.Object ref = autoViewId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
autoViewId_ = s;
return s;
}
}
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @return The bytes for autoViewId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAutoViewIdBytes() {
java.lang.Object ref = autoViewId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
autoViewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_ID_FIELD_NUMBER = 8;
private volatile java.lang.Object sessionId_;
/**
* string session_id = 8 [json_name = "sessionId"];
* @return The sessionId.
*/
@java.lang.Override
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
* string session_id = 8 [json_name = "sessionId"];
* @return The bytes for sessionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_REQUEST_ID_FIELD_NUMBER = 14;
private volatile java.lang.Object clientRequestId_;
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @return The clientRequestId.
*/
@java.lang.Override
public java.lang.String getClientRequestId() {
java.lang.Object ref = clientRequestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientRequestId_ = s;
return s;
}
}
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @return The bytes for clientRequestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClientRequestIdBytes() {
java.lang.Object ref = clientRequestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientRequestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USE_CASE_FIELD_NUMBER = 9;
private int useCase_;
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @return The enum numeric value on the wire for useCase.
*/
@java.lang.Override public int getUseCaseValue() {
return useCase_;
}
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @return The useCase.
*/
@java.lang.Override public ai.promoted.proto.delivery.UseCase getUseCase() {
@SuppressWarnings("deprecation")
ai.promoted.proto.delivery.UseCase result = ai.promoted.proto.delivery.UseCase.valueOf(useCase_);
return result == null ? ai.promoted.proto.delivery.UseCase.UNRECOGNIZED : result;
}
public static final int SEARCH_QUERY_FIELD_NUMBER = 10;
private volatile java.lang.Object searchQuery_;
/**
* string search_query = 10 [json_name = "searchQuery"];
* @return The searchQuery.
*/
@java.lang.Override
public java.lang.String getSearchQuery() {
java.lang.Object ref = searchQuery_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
searchQuery_ = s;
return s;
}
}
/**
* string search_query = 10 [json_name = "searchQuery"];
* @return The bytes for searchQuery.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSearchQueryBytes() {
java.lang.Object ref = searchQuery_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
searchQuery_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGING_FIELD_NUMBER = 17;
private ai.promoted.proto.delivery.Paging paging_;
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
* @return Whether the paging field is set.
*/
@java.lang.Override
public boolean hasPaging() {
return paging_ != null;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
* @return The paging.
*/
@java.lang.Override
public ai.promoted.proto.delivery.Paging getPaging() {
return paging_ == null ? ai.promoted.proto.delivery.Paging.getDefaultInstance() : paging_;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
@java.lang.Override
public ai.promoted.proto.delivery.PagingOrBuilder getPagingOrBuilder() {
return getPaging();
}
public static final int INSERTION_FIELD_NUMBER = 11;
private java.util.List insertion_;
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
@java.lang.Override
public java.util.List getInsertionList() {
return insertion_;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
@java.lang.Override
public java.util.List extends ai.promoted.proto.delivery.InsertionOrBuilder>
getInsertionOrBuilderList() {
return insertion_;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
@java.lang.Override
public int getInsertionCount() {
return insertion_.size();
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
@java.lang.Override
public ai.promoted.proto.delivery.Insertion getInsertion(int index) {
return insertion_.get(index);
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
@java.lang.Override
public ai.promoted.proto.delivery.InsertionOrBuilder getInsertionOrBuilder(
int index) {
return insertion_.get(index);
}
public static final int INSERTION_MATRIX_HEADERS_FIELD_NUMBER = 20;
private com.google.protobuf.LazyStringList insertionMatrixHeaders_;
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @return A list containing the insertionMatrixHeaders.
*/
public com.google.protobuf.ProtocolStringList
getInsertionMatrixHeadersList() {
return insertionMatrixHeaders_;
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @return The count of insertionMatrixHeaders.
*/
public int getInsertionMatrixHeadersCount() {
return insertionMatrixHeaders_.size();
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param index The index of the element to return.
* @return The insertionMatrixHeaders at the given index.
*/
public java.lang.String getInsertionMatrixHeaders(int index) {
return insertionMatrixHeaders_.get(index);
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param index The index of the value to return.
* @return The bytes of the insertionMatrixHeaders at the given index.
*/
public com.google.protobuf.ByteString
getInsertionMatrixHeadersBytes(int index) {
return insertionMatrixHeaders_.getByteString(index);
}
public static final int INSERTION_MATRIX_FIELD_NUMBER = 21;
private com.google.protobuf.ListValue insertionMatrix_;
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
* @return Whether the insertionMatrix field is set.
*/
@java.lang.Override
public boolean hasInsertionMatrix() {
return insertionMatrix_ != null;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
* @return The insertionMatrix.
*/
@java.lang.Override
public com.google.protobuf.ListValue getInsertionMatrix() {
return insertionMatrix_ == null ? com.google.protobuf.ListValue.getDefaultInstance() : insertionMatrix_;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
@java.lang.Override
public com.google.protobuf.ListValueOrBuilder getInsertionMatrixOrBuilder() {
return getInsertionMatrix();
}
public static final int PROPERTIES_FIELD_NUMBER = 13;
private ai.promoted.proto.common.Properties properties_;
/**
* .common.Properties properties = 13 [json_name = "properties"];
* @return Whether the properties field is set.
*/
@java.lang.Override
public boolean hasProperties() {
return properties_ != null;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
* @return The properties.
*/
@java.lang.Override
public ai.promoted.proto.common.Properties getProperties() {
return properties_ == null ? ai.promoted.proto.common.Properties.getDefaultInstance() : properties_;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
@java.lang.Override
public ai.promoted.proto.common.PropertiesOrBuilder getPropertiesOrBuilder() {
return getProperties();
}
public static final int DISABLE_PERSONALIZATION_FIELD_NUMBER = 22;
private boolean disablePersonalization_;
/**
* bool disable_personalization = 22 [json_name = "disablePersonalization"];
* @return The disablePersonalization.
*/
@java.lang.Override
public boolean getDisablePersonalization() {
return disablePersonalization_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (platformId_ != 0L) {
output.writeUInt64(1, platformId_);
}
if (userInfo_ != null) {
output.writeMessage(2, getUserInfo());
}
if (timing_ != null) {
output.writeMessage(3, getTiming());
}
if (clientInfo_ != null) {
output.writeMessage(4, getClientInfo());
}
if (!getRequestIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, requestId_);
}
if (!getViewIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, viewId_);
}
if (!getSessionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, sessionId_);
}
if (useCase_ != ai.promoted.proto.delivery.UseCase.UNKNOWN_USE_CASE.getNumber()) {
output.writeEnum(9, useCase_);
}
if (!getSearchQueryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, searchQuery_);
}
for (int i = 0; i < insertion_.size(); i++) {
output.writeMessage(11, insertion_.get(i));
}
if (properties_ != null) {
output.writeMessage(13, getProperties());
}
if (!getClientRequestIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, clientRequestId_);
}
if (paging_ != null) {
output.writeMessage(17, getPaging());
}
if (device_ != null) {
output.writeMessage(18, getDevice());
}
if (!getAutoViewIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, autoViewId_);
}
for (int i = 0; i < insertionMatrixHeaders_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, insertionMatrixHeaders_.getRaw(i));
}
if (insertionMatrix_ != null) {
output.writeMessage(21, getInsertionMatrix());
}
if (disablePersonalization_ != false) {
output.writeBool(22, disablePersonalization_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (platformId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, platformId_);
}
if (userInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getUserInfo());
}
if (timing_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getTiming());
}
if (clientInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getClientInfo());
}
if (!getRequestIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, requestId_);
}
if (!getViewIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, viewId_);
}
if (!getSessionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, sessionId_);
}
if (useCase_ != ai.promoted.proto.delivery.UseCase.UNKNOWN_USE_CASE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, useCase_);
}
if (!getSearchQueryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, searchQuery_);
}
for (int i = 0; i < insertion_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, insertion_.get(i));
}
if (properties_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getProperties());
}
if (!getClientRequestIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, clientRequestId_);
}
if (paging_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, getPaging());
}
if (device_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getDevice());
}
if (!getAutoViewIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, autoViewId_);
}
{
int dataSize = 0;
for (int i = 0; i < insertionMatrixHeaders_.size(); i++) {
dataSize += computeStringSizeNoTag(insertionMatrixHeaders_.getRaw(i));
}
size += dataSize;
size += 2 * getInsertionMatrixHeadersList().size();
}
if (insertionMatrix_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getInsertionMatrix());
}
if (disablePersonalization_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(22, disablePersonalization_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ai.promoted.proto.delivery.Request)) {
return super.equals(obj);
}
ai.promoted.proto.delivery.Request other = (ai.promoted.proto.delivery.Request) obj;
if (getPlatformId()
!= other.getPlatformId()) return false;
if (hasUserInfo() != other.hasUserInfo()) return false;
if (hasUserInfo()) {
if (!getUserInfo()
.equals(other.getUserInfo())) return false;
}
if (hasTiming() != other.hasTiming()) return false;
if (hasTiming()) {
if (!getTiming()
.equals(other.getTiming())) return false;
}
if (hasClientInfo() != other.hasClientInfo()) return false;
if (hasClientInfo()) {
if (!getClientInfo()
.equals(other.getClientInfo())) return false;
}
if (hasDevice() != other.hasDevice()) return false;
if (hasDevice()) {
if (!getDevice()
.equals(other.getDevice())) return false;
}
if (!getRequestId()
.equals(other.getRequestId())) return false;
if (!getViewId()
.equals(other.getViewId())) return false;
if (!getAutoViewId()
.equals(other.getAutoViewId())) return false;
if (!getSessionId()
.equals(other.getSessionId())) return false;
if (!getClientRequestId()
.equals(other.getClientRequestId())) return false;
if (useCase_ != other.useCase_) return false;
if (!getSearchQuery()
.equals(other.getSearchQuery())) return false;
if (hasPaging() != other.hasPaging()) return false;
if (hasPaging()) {
if (!getPaging()
.equals(other.getPaging())) return false;
}
if (!getInsertionList()
.equals(other.getInsertionList())) return false;
if (!getInsertionMatrixHeadersList()
.equals(other.getInsertionMatrixHeadersList())) return false;
if (hasInsertionMatrix() != other.hasInsertionMatrix()) return false;
if (hasInsertionMatrix()) {
if (!getInsertionMatrix()
.equals(other.getInsertionMatrix())) return false;
}
if (hasProperties() != other.hasProperties()) return false;
if (hasProperties()) {
if (!getProperties()
.equals(other.getProperties())) return false;
}
if (getDisablePersonalization()
!= other.getDisablePersonalization()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PLATFORM_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPlatformId());
if (hasUserInfo()) {
hash = (37 * hash) + USER_INFO_FIELD_NUMBER;
hash = (53 * hash) + getUserInfo().hashCode();
}
if (hasTiming()) {
hash = (37 * hash) + TIMING_FIELD_NUMBER;
hash = (53 * hash) + getTiming().hashCode();
}
if (hasClientInfo()) {
hash = (37 * hash) + CLIENT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getClientInfo().hashCode();
}
if (hasDevice()) {
hash = (37 * hash) + DEVICE_FIELD_NUMBER;
hash = (53 * hash) + getDevice().hashCode();
}
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestId().hashCode();
hash = (37 * hash) + VIEW_ID_FIELD_NUMBER;
hash = (53 * hash) + getViewId().hashCode();
hash = (37 * hash) + AUTO_VIEW_ID_FIELD_NUMBER;
hash = (53 * hash) + getAutoViewId().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
hash = (37 * hash) + CLIENT_REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientRequestId().hashCode();
hash = (37 * hash) + USE_CASE_FIELD_NUMBER;
hash = (53 * hash) + useCase_;
hash = (37 * hash) + SEARCH_QUERY_FIELD_NUMBER;
hash = (53 * hash) + getSearchQuery().hashCode();
if (hasPaging()) {
hash = (37 * hash) + PAGING_FIELD_NUMBER;
hash = (53 * hash) + getPaging().hashCode();
}
if (getInsertionCount() > 0) {
hash = (37 * hash) + INSERTION_FIELD_NUMBER;
hash = (53 * hash) + getInsertionList().hashCode();
}
if (getInsertionMatrixHeadersCount() > 0) {
hash = (37 * hash) + INSERTION_MATRIX_HEADERS_FIELD_NUMBER;
hash = (53 * hash) + getInsertionMatrixHeadersList().hashCode();
}
if (hasInsertionMatrix()) {
hash = (37 * hash) + INSERTION_MATRIX_FIELD_NUMBER;
hash = (53 * hash) + getInsertionMatrix().hashCode();
}
if (hasProperties()) {
hash = (37 * hash) + PROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + getProperties().hashCode();
}
hash = (37 * hash) + DISABLE_PERSONALIZATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDisablePersonalization());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ai.promoted.proto.delivery.Request parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.delivery.Request parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.delivery.Request parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.delivery.Request parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.delivery.Request parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.delivery.Request parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.delivery.Request parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.delivery.Request parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.delivery.Request parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ai.promoted.proto.delivery.Request parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.delivery.Request parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.delivery.Request parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ai.promoted.proto.delivery.Request prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code delivery.Request}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:delivery.Request)
ai.promoted.proto.delivery.RequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.delivery.Delivery.internal_static_delivery_Request_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.delivery.Delivery.internal_static_delivery_Request_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.delivery.Request.class, ai.promoted.proto.delivery.Request.Builder.class);
}
// Construct using ai.promoted.proto.delivery.Request.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getInsertionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
platformId_ = 0L;
if (userInfoBuilder_ == null) {
userInfo_ = null;
} else {
userInfo_ = null;
userInfoBuilder_ = null;
}
if (timingBuilder_ == null) {
timing_ = null;
} else {
timing_ = null;
timingBuilder_ = null;
}
if (clientInfoBuilder_ == null) {
clientInfo_ = null;
} else {
clientInfo_ = null;
clientInfoBuilder_ = null;
}
if (deviceBuilder_ == null) {
device_ = null;
} else {
device_ = null;
deviceBuilder_ = null;
}
requestId_ = "";
viewId_ = "";
autoViewId_ = "";
sessionId_ = "";
clientRequestId_ = "";
useCase_ = 0;
searchQuery_ = "";
if (pagingBuilder_ == null) {
paging_ = null;
} else {
paging_ = null;
pagingBuilder_ = null;
}
if (insertionBuilder_ == null) {
insertion_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
insertionBuilder_.clear();
}
insertionMatrixHeaders_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
if (insertionMatrixBuilder_ == null) {
insertionMatrix_ = null;
} else {
insertionMatrix_ = null;
insertionMatrixBuilder_ = null;
}
if (propertiesBuilder_ == null) {
properties_ = null;
} else {
properties_ = null;
propertiesBuilder_ = null;
}
disablePersonalization_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ai.promoted.proto.delivery.Delivery.internal_static_delivery_Request_descriptor;
}
@java.lang.Override
public ai.promoted.proto.delivery.Request getDefaultInstanceForType() {
return ai.promoted.proto.delivery.Request.getDefaultInstance();
}
@java.lang.Override
public ai.promoted.proto.delivery.Request build() {
ai.promoted.proto.delivery.Request result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ai.promoted.proto.delivery.Request buildPartial() {
ai.promoted.proto.delivery.Request result = new ai.promoted.proto.delivery.Request(this);
int from_bitField0_ = bitField0_;
result.platformId_ = platformId_;
if (userInfoBuilder_ == null) {
result.userInfo_ = userInfo_;
} else {
result.userInfo_ = userInfoBuilder_.build();
}
if (timingBuilder_ == null) {
result.timing_ = timing_;
} else {
result.timing_ = timingBuilder_.build();
}
if (clientInfoBuilder_ == null) {
result.clientInfo_ = clientInfo_;
} else {
result.clientInfo_ = clientInfoBuilder_.build();
}
if (deviceBuilder_ == null) {
result.device_ = device_;
} else {
result.device_ = deviceBuilder_.build();
}
result.requestId_ = requestId_;
result.viewId_ = viewId_;
result.autoViewId_ = autoViewId_;
result.sessionId_ = sessionId_;
result.clientRequestId_ = clientRequestId_;
result.useCase_ = useCase_;
result.searchQuery_ = searchQuery_;
if (pagingBuilder_ == null) {
result.paging_ = paging_;
} else {
result.paging_ = pagingBuilder_.build();
}
if (insertionBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
insertion_ = java.util.Collections.unmodifiableList(insertion_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.insertion_ = insertion_;
} else {
result.insertion_ = insertionBuilder_.build();
}
if (((bitField0_ & 0x00000002) != 0)) {
insertionMatrixHeaders_ = insertionMatrixHeaders_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.insertionMatrixHeaders_ = insertionMatrixHeaders_;
if (insertionMatrixBuilder_ == null) {
result.insertionMatrix_ = insertionMatrix_;
} else {
result.insertionMatrix_ = insertionMatrixBuilder_.build();
}
if (propertiesBuilder_ == null) {
result.properties_ = properties_;
} else {
result.properties_ = propertiesBuilder_.build();
}
result.disablePersonalization_ = disablePersonalization_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ai.promoted.proto.delivery.Request) {
return mergeFrom((ai.promoted.proto.delivery.Request)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ai.promoted.proto.delivery.Request other) {
if (other == ai.promoted.proto.delivery.Request.getDefaultInstance()) return this;
if (other.getPlatformId() != 0L) {
setPlatformId(other.getPlatformId());
}
if (other.hasUserInfo()) {
mergeUserInfo(other.getUserInfo());
}
if (other.hasTiming()) {
mergeTiming(other.getTiming());
}
if (other.hasClientInfo()) {
mergeClientInfo(other.getClientInfo());
}
if (other.hasDevice()) {
mergeDevice(other.getDevice());
}
if (!other.getRequestId().isEmpty()) {
requestId_ = other.requestId_;
onChanged();
}
if (!other.getViewId().isEmpty()) {
viewId_ = other.viewId_;
onChanged();
}
if (!other.getAutoViewId().isEmpty()) {
autoViewId_ = other.autoViewId_;
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
onChanged();
}
if (!other.getClientRequestId().isEmpty()) {
clientRequestId_ = other.clientRequestId_;
onChanged();
}
if (other.useCase_ != 0) {
setUseCaseValue(other.getUseCaseValue());
}
if (!other.getSearchQuery().isEmpty()) {
searchQuery_ = other.searchQuery_;
onChanged();
}
if (other.hasPaging()) {
mergePaging(other.getPaging());
}
if (insertionBuilder_ == null) {
if (!other.insertion_.isEmpty()) {
if (insertion_.isEmpty()) {
insertion_ = other.insertion_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureInsertionIsMutable();
insertion_.addAll(other.insertion_);
}
onChanged();
}
} else {
if (!other.insertion_.isEmpty()) {
if (insertionBuilder_.isEmpty()) {
insertionBuilder_.dispose();
insertionBuilder_ = null;
insertion_ = other.insertion_;
bitField0_ = (bitField0_ & ~0x00000001);
insertionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getInsertionFieldBuilder() : null;
} else {
insertionBuilder_.addAllMessages(other.insertion_);
}
}
}
if (!other.insertionMatrixHeaders_.isEmpty()) {
if (insertionMatrixHeaders_.isEmpty()) {
insertionMatrixHeaders_ = other.insertionMatrixHeaders_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureInsertionMatrixHeadersIsMutable();
insertionMatrixHeaders_.addAll(other.insertionMatrixHeaders_);
}
onChanged();
}
if (other.hasInsertionMatrix()) {
mergeInsertionMatrix(other.getInsertionMatrix());
}
if (other.hasProperties()) {
mergeProperties(other.getProperties());
}
if (other.getDisablePersonalization() != false) {
setDisablePersonalization(other.getDisablePersonalization());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ai.promoted.proto.delivery.Request parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ai.promoted.proto.delivery.Request) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long platformId_ ;
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @return The platformId.
*/
@java.lang.Override
public long getPlatformId() {
return platformId_;
}
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @param value The platformId to set.
* @return This builder for chaining.
*/
public Builder setPlatformId(long value) {
platformId_ = value;
onChanged();
return this;
}
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @return This builder for chaining.
*/
public Builder clearPlatformId() {
platformId_ = 0L;
onChanged();
return this;
}
private ai.promoted.proto.common.UserInfo userInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.UserInfo, ai.promoted.proto.common.UserInfo.Builder, ai.promoted.proto.common.UserInfoOrBuilder> userInfoBuilder_;
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return Whether the userInfo field is set.
*/
public boolean hasUserInfo() {
return userInfoBuilder_ != null || userInfo_ != null;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return The userInfo.
*/
public ai.promoted.proto.common.UserInfo getUserInfo() {
if (userInfoBuilder_ == null) {
return userInfo_ == null ? ai.promoted.proto.common.UserInfo.getDefaultInstance() : userInfo_;
} else {
return userInfoBuilder_.getMessage();
}
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder setUserInfo(ai.promoted.proto.common.UserInfo value) {
if (userInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userInfo_ = value;
onChanged();
} else {
userInfoBuilder_.setMessage(value);
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder setUserInfo(
ai.promoted.proto.common.UserInfo.Builder builderForValue) {
if (userInfoBuilder_ == null) {
userInfo_ = builderForValue.build();
onChanged();
} else {
userInfoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder mergeUserInfo(ai.promoted.proto.common.UserInfo value) {
if (userInfoBuilder_ == null) {
if (userInfo_ != null) {
userInfo_ =
ai.promoted.proto.common.UserInfo.newBuilder(userInfo_).mergeFrom(value).buildPartial();
} else {
userInfo_ = value;
}
onChanged();
} else {
userInfoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder clearUserInfo() {
if (userInfoBuilder_ == null) {
userInfo_ = null;
onChanged();
} else {
userInfo_ = null;
userInfoBuilder_ = null;
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public ai.promoted.proto.common.UserInfo.Builder getUserInfoBuilder() {
onChanged();
return getUserInfoFieldBuilder().getBuilder();
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public ai.promoted.proto.common.UserInfoOrBuilder getUserInfoOrBuilder() {
if (userInfoBuilder_ != null) {
return userInfoBuilder_.getMessageOrBuilder();
} else {
return userInfo_ == null ?
ai.promoted.proto.common.UserInfo.getDefaultInstance() : userInfo_;
}
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.UserInfo, ai.promoted.proto.common.UserInfo.Builder, ai.promoted.proto.common.UserInfoOrBuilder>
getUserInfoFieldBuilder() {
if (userInfoBuilder_ == null) {
userInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.UserInfo, ai.promoted.proto.common.UserInfo.Builder, ai.promoted.proto.common.UserInfoOrBuilder>(
getUserInfo(),
getParentForChildren(),
isClean());
userInfo_ = null;
}
return userInfoBuilder_;
}
private ai.promoted.proto.common.Timing timing_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Timing, ai.promoted.proto.common.Timing.Builder, ai.promoted.proto.common.TimingOrBuilder> timingBuilder_;
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return Whether the timing field is set.
*/
public boolean hasTiming() {
return timingBuilder_ != null || timing_ != null;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return The timing.
*/
public ai.promoted.proto.common.Timing getTiming() {
if (timingBuilder_ == null) {
return timing_ == null ? ai.promoted.proto.common.Timing.getDefaultInstance() : timing_;
} else {
return timingBuilder_.getMessage();
}
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder setTiming(ai.promoted.proto.common.Timing value) {
if (timingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timing_ = value;
onChanged();
} else {
timingBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder setTiming(
ai.promoted.proto.common.Timing.Builder builderForValue) {
if (timingBuilder_ == null) {
timing_ = builderForValue.build();
onChanged();
} else {
timingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder mergeTiming(ai.promoted.proto.common.Timing value) {
if (timingBuilder_ == null) {
if (timing_ != null) {
timing_ =
ai.promoted.proto.common.Timing.newBuilder(timing_).mergeFrom(value).buildPartial();
} else {
timing_ = value;
}
onChanged();
} else {
timingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder clearTiming() {
if (timingBuilder_ == null) {
timing_ = null;
onChanged();
} else {
timing_ = null;
timingBuilder_ = null;
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public ai.promoted.proto.common.Timing.Builder getTimingBuilder() {
onChanged();
return getTimingFieldBuilder().getBuilder();
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public ai.promoted.proto.common.TimingOrBuilder getTimingOrBuilder() {
if (timingBuilder_ != null) {
return timingBuilder_.getMessageOrBuilder();
} else {
return timing_ == null ?
ai.promoted.proto.common.Timing.getDefaultInstance() : timing_;
}
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Timing, ai.promoted.proto.common.Timing.Builder, ai.promoted.proto.common.TimingOrBuilder>
getTimingFieldBuilder() {
if (timingBuilder_ == null) {
timingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Timing, ai.promoted.proto.common.Timing.Builder, ai.promoted.proto.common.TimingOrBuilder>(
getTiming(),
getParentForChildren(),
isClean());
timing_ = null;
}
return timingBuilder_;
}
private ai.promoted.proto.common.ClientInfo clientInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.ClientInfo, ai.promoted.proto.common.ClientInfo.Builder, ai.promoted.proto.common.ClientInfoOrBuilder> clientInfoBuilder_;
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return Whether the clientInfo field is set.
*/
public boolean hasClientInfo() {
return clientInfoBuilder_ != null || clientInfo_ != null;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return The clientInfo.
*/
public ai.promoted.proto.common.ClientInfo getClientInfo() {
if (clientInfoBuilder_ == null) {
return clientInfo_ == null ? ai.promoted.proto.common.ClientInfo.getDefaultInstance() : clientInfo_;
} else {
return clientInfoBuilder_.getMessage();
}
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder setClientInfo(ai.promoted.proto.common.ClientInfo value) {
if (clientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientInfo_ = value;
onChanged();
} else {
clientInfoBuilder_.setMessage(value);
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder setClientInfo(
ai.promoted.proto.common.ClientInfo.Builder builderForValue) {
if (clientInfoBuilder_ == null) {
clientInfo_ = builderForValue.build();
onChanged();
} else {
clientInfoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder mergeClientInfo(ai.promoted.proto.common.ClientInfo value) {
if (clientInfoBuilder_ == null) {
if (clientInfo_ != null) {
clientInfo_ =
ai.promoted.proto.common.ClientInfo.newBuilder(clientInfo_).mergeFrom(value).buildPartial();
} else {
clientInfo_ = value;
}
onChanged();
} else {
clientInfoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder clearClientInfo() {
if (clientInfoBuilder_ == null) {
clientInfo_ = null;
onChanged();
} else {
clientInfo_ = null;
clientInfoBuilder_ = null;
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public ai.promoted.proto.common.ClientInfo.Builder getClientInfoBuilder() {
onChanged();
return getClientInfoFieldBuilder().getBuilder();
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public ai.promoted.proto.common.ClientInfoOrBuilder getClientInfoOrBuilder() {
if (clientInfoBuilder_ != null) {
return clientInfoBuilder_.getMessageOrBuilder();
} else {
return clientInfo_ == null ?
ai.promoted.proto.common.ClientInfo.getDefaultInstance() : clientInfo_;
}
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.ClientInfo, ai.promoted.proto.common.ClientInfo.Builder, ai.promoted.proto.common.ClientInfoOrBuilder>
getClientInfoFieldBuilder() {
if (clientInfoBuilder_ == null) {
clientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.ClientInfo, ai.promoted.proto.common.ClientInfo.Builder, ai.promoted.proto.common.ClientInfoOrBuilder>(
getClientInfo(),
getParentForChildren(),
isClean());
clientInfo_ = null;
}
return clientInfoBuilder_;
}
private ai.promoted.proto.common.Device device_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Device, ai.promoted.proto.common.Device.Builder, ai.promoted.proto.common.DeviceOrBuilder> deviceBuilder_;
/**
* .common.Device device = 18 [json_name = "device"];
* @return Whether the device field is set.
*/
public boolean hasDevice() {
return deviceBuilder_ != null || device_ != null;
}
/**
* .common.Device device = 18 [json_name = "device"];
* @return The device.
*/
public ai.promoted.proto.common.Device getDevice() {
if (deviceBuilder_ == null) {
return device_ == null ? ai.promoted.proto.common.Device.getDefaultInstance() : device_;
} else {
return deviceBuilder_.getMessage();
}
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
public Builder setDevice(ai.promoted.proto.common.Device value) {
if (deviceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
device_ = value;
onChanged();
} else {
deviceBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
public Builder setDevice(
ai.promoted.proto.common.Device.Builder builderForValue) {
if (deviceBuilder_ == null) {
device_ = builderForValue.build();
onChanged();
} else {
deviceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
public Builder mergeDevice(ai.promoted.proto.common.Device value) {
if (deviceBuilder_ == null) {
if (device_ != null) {
device_ =
ai.promoted.proto.common.Device.newBuilder(device_).mergeFrom(value).buildPartial();
} else {
device_ = value;
}
onChanged();
} else {
deviceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
public Builder clearDevice() {
if (deviceBuilder_ == null) {
device_ = null;
onChanged();
} else {
device_ = null;
deviceBuilder_ = null;
}
return this;
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
public ai.promoted.proto.common.Device.Builder getDeviceBuilder() {
onChanged();
return getDeviceFieldBuilder().getBuilder();
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
public ai.promoted.proto.common.DeviceOrBuilder getDeviceOrBuilder() {
if (deviceBuilder_ != null) {
return deviceBuilder_.getMessageOrBuilder();
} else {
return device_ == null ?
ai.promoted.proto.common.Device.getDefaultInstance() : device_;
}
}
/**
* .common.Device device = 18 [json_name = "device"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Device, ai.promoted.proto.common.Device.Builder, ai.promoted.proto.common.DeviceOrBuilder>
getDeviceFieldBuilder() {
if (deviceBuilder_ == null) {
deviceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Device, ai.promoted.proto.common.Device.Builder, ai.promoted.proto.common.DeviceOrBuilder>(
getDevice(),
getParentForChildren(),
isClean());
device_ = null;
}
return deviceBuilder_;
}
private java.lang.Object requestId_ = "";
/**
* string request_id = 6 [json_name = "requestId"];
* @return The requestId.
*/
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string request_id = 6 [json_name = "requestId"];
* @return The bytes for requestId.
*/
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string request_id = 6 [json_name = "requestId"];
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestId_ = value;
onChanged();
return this;
}
/**
* string request_id = 6 [json_name = "requestId"];
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = getDefaultInstance().getRequestId();
onChanged();
return this;
}
/**
* string request_id = 6 [json_name = "requestId"];
* @param value The bytes for requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestId_ = value;
onChanged();
return this;
}
private java.lang.Object viewId_ = "";
/**
* string view_id = 7 [json_name = "viewId"];
* @return The viewId.
*/
public java.lang.String getViewId() {
java.lang.Object ref = viewId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
viewId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string view_id = 7 [json_name = "viewId"];
* @return The bytes for viewId.
*/
public com.google.protobuf.ByteString
getViewIdBytes() {
java.lang.Object ref = viewId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
viewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string view_id = 7 [json_name = "viewId"];
* @param value The viewId to set.
* @return This builder for chaining.
*/
public Builder setViewId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
viewId_ = value;
onChanged();
return this;
}
/**
* string view_id = 7 [json_name = "viewId"];
* @return This builder for chaining.
*/
public Builder clearViewId() {
viewId_ = getDefaultInstance().getViewId();
onChanged();
return this;
}
/**
* string view_id = 7 [json_name = "viewId"];
* @param value The bytes for viewId to set.
* @return This builder for chaining.
*/
public Builder setViewIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
viewId_ = value;
onChanged();
return this;
}
private java.lang.Object autoViewId_ = "";
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @return The autoViewId.
*/
public java.lang.String getAutoViewId() {
java.lang.Object ref = autoViewId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
autoViewId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @return The bytes for autoViewId.
*/
public com.google.protobuf.ByteString
getAutoViewIdBytes() {
java.lang.Object ref = autoViewId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
autoViewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @param value The autoViewId to set.
* @return This builder for chaining.
*/
public Builder setAutoViewId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
autoViewId_ = value;
onChanged();
return this;
}
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @return This builder for chaining.
*/
public Builder clearAutoViewId() {
autoViewId_ = getDefaultInstance().getAutoViewId();
onChanged();
return this;
}
/**
* string auto_view_id = 19 [json_name = "autoViewId"];
* @param value The bytes for autoViewId to set.
* @return This builder for chaining.
*/
public Builder setAutoViewIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
autoViewId_ = value;
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
* string session_id = 8 [json_name = "sessionId"];
* @return The sessionId.
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string session_id = 8 [json_name = "sessionId"];
* @return The bytes for sessionId.
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string session_id = 8 [json_name = "sessionId"];
* @param value The sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
onChanged();
return this;
}
/**
* string session_id = 8 [json_name = "sessionId"];
* @return This builder for chaining.
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
* string session_id = 8 [json_name = "sessionId"];
* @param value The bytes for sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
onChanged();
return this;
}
private java.lang.Object clientRequestId_ = "";
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @return The clientRequestId.
*/
public java.lang.String getClientRequestId() {
java.lang.Object ref = clientRequestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientRequestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @return The bytes for clientRequestId.
*/
public com.google.protobuf.ByteString
getClientRequestIdBytes() {
java.lang.Object ref = clientRequestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientRequestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @param value The clientRequestId to set.
* @return This builder for chaining.
*/
public Builder setClientRequestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientRequestId_ = value;
onChanged();
return this;
}
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @return This builder for chaining.
*/
public Builder clearClientRequestId() {
clientRequestId_ = getDefaultInstance().getClientRequestId();
onChanged();
return this;
}
/**
* string client_request_id = 14 [json_name = "clientRequestId"];
* @param value The bytes for clientRequestId to set.
* @return This builder for chaining.
*/
public Builder setClientRequestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientRequestId_ = value;
onChanged();
return this;
}
private int useCase_ = 0;
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @return The enum numeric value on the wire for useCase.
*/
@java.lang.Override public int getUseCaseValue() {
return useCase_;
}
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @param value The enum numeric value on the wire for useCase to set.
* @return This builder for chaining.
*/
public Builder setUseCaseValue(int value) {
useCase_ = value;
onChanged();
return this;
}
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @return The useCase.
*/
@java.lang.Override
public ai.promoted.proto.delivery.UseCase getUseCase() {
@SuppressWarnings("deprecation")
ai.promoted.proto.delivery.UseCase result = ai.promoted.proto.delivery.UseCase.valueOf(useCase_);
return result == null ? ai.promoted.proto.delivery.UseCase.UNRECOGNIZED : result;
}
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @param value The useCase to set.
* @return This builder for chaining.
*/
public Builder setUseCase(ai.promoted.proto.delivery.UseCase value) {
if (value == null) {
throw new NullPointerException();
}
useCase_ = value.getNumber();
onChanged();
return this;
}
/**
* .delivery.UseCase use_case = 9 [json_name = "useCase"];
* @return This builder for chaining.
*/
public Builder clearUseCase() {
useCase_ = 0;
onChanged();
return this;
}
private java.lang.Object searchQuery_ = "";
/**
* string search_query = 10 [json_name = "searchQuery"];
* @return The searchQuery.
*/
public java.lang.String getSearchQuery() {
java.lang.Object ref = searchQuery_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
searchQuery_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string search_query = 10 [json_name = "searchQuery"];
* @return The bytes for searchQuery.
*/
public com.google.protobuf.ByteString
getSearchQueryBytes() {
java.lang.Object ref = searchQuery_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
searchQuery_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string search_query = 10 [json_name = "searchQuery"];
* @param value The searchQuery to set.
* @return This builder for chaining.
*/
public Builder setSearchQuery(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
searchQuery_ = value;
onChanged();
return this;
}
/**
* string search_query = 10 [json_name = "searchQuery"];
* @return This builder for chaining.
*/
public Builder clearSearchQuery() {
searchQuery_ = getDefaultInstance().getSearchQuery();
onChanged();
return this;
}
/**
* string search_query = 10 [json_name = "searchQuery"];
* @param value The bytes for searchQuery to set.
* @return This builder for chaining.
*/
public Builder setSearchQueryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
searchQuery_ = value;
onChanged();
return this;
}
private ai.promoted.proto.delivery.Paging paging_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.delivery.Paging, ai.promoted.proto.delivery.Paging.Builder, ai.promoted.proto.delivery.PagingOrBuilder> pagingBuilder_;
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
* @return Whether the paging field is set.
*/
public boolean hasPaging() {
return pagingBuilder_ != null || paging_ != null;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
* @return The paging.
*/
public ai.promoted.proto.delivery.Paging getPaging() {
if (pagingBuilder_ == null) {
return paging_ == null ? ai.promoted.proto.delivery.Paging.getDefaultInstance() : paging_;
} else {
return pagingBuilder_.getMessage();
}
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
public Builder setPaging(ai.promoted.proto.delivery.Paging value) {
if (pagingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
paging_ = value;
onChanged();
} else {
pagingBuilder_.setMessage(value);
}
return this;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
public Builder setPaging(
ai.promoted.proto.delivery.Paging.Builder builderForValue) {
if (pagingBuilder_ == null) {
paging_ = builderForValue.build();
onChanged();
} else {
pagingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
public Builder mergePaging(ai.promoted.proto.delivery.Paging value) {
if (pagingBuilder_ == null) {
if (paging_ != null) {
paging_ =
ai.promoted.proto.delivery.Paging.newBuilder(paging_).mergeFrom(value).buildPartial();
} else {
paging_ = value;
}
onChanged();
} else {
pagingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
public Builder clearPaging() {
if (pagingBuilder_ == null) {
paging_ = null;
onChanged();
} else {
paging_ = null;
pagingBuilder_ = null;
}
return this;
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
public ai.promoted.proto.delivery.Paging.Builder getPagingBuilder() {
onChanged();
return getPagingFieldBuilder().getBuilder();
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
public ai.promoted.proto.delivery.PagingOrBuilder getPagingOrBuilder() {
if (pagingBuilder_ != null) {
return pagingBuilder_.getMessageOrBuilder();
} else {
return paging_ == null ?
ai.promoted.proto.delivery.Paging.getDefaultInstance() : paging_;
}
}
/**
* .delivery.Paging paging = 17 [json_name = "paging"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.delivery.Paging, ai.promoted.proto.delivery.Paging.Builder, ai.promoted.proto.delivery.PagingOrBuilder>
getPagingFieldBuilder() {
if (pagingBuilder_ == null) {
pagingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.delivery.Paging, ai.promoted.proto.delivery.Paging.Builder, ai.promoted.proto.delivery.PagingOrBuilder>(
getPaging(),
getParentForChildren(),
isClean());
paging_ = null;
}
return pagingBuilder_;
}
private java.util.List insertion_ =
java.util.Collections.emptyList();
private void ensureInsertionIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
insertion_ = new java.util.ArrayList(insertion_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.delivery.Insertion, ai.promoted.proto.delivery.Insertion.Builder, ai.promoted.proto.delivery.InsertionOrBuilder> insertionBuilder_;
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public java.util.List getInsertionList() {
if (insertionBuilder_ == null) {
return java.util.Collections.unmodifiableList(insertion_);
} else {
return insertionBuilder_.getMessageList();
}
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public int getInsertionCount() {
if (insertionBuilder_ == null) {
return insertion_.size();
} else {
return insertionBuilder_.getCount();
}
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public ai.promoted.proto.delivery.Insertion getInsertion(int index) {
if (insertionBuilder_ == null) {
return insertion_.get(index);
} else {
return insertionBuilder_.getMessage(index);
}
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder setInsertion(
int index, ai.promoted.proto.delivery.Insertion value) {
if (insertionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertionIsMutable();
insertion_.set(index, value);
onChanged();
} else {
insertionBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder setInsertion(
int index, ai.promoted.proto.delivery.Insertion.Builder builderForValue) {
if (insertionBuilder_ == null) {
ensureInsertionIsMutable();
insertion_.set(index, builderForValue.build());
onChanged();
} else {
insertionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder addInsertion(ai.promoted.proto.delivery.Insertion value) {
if (insertionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertionIsMutable();
insertion_.add(value);
onChanged();
} else {
insertionBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder addInsertion(
int index, ai.promoted.proto.delivery.Insertion value) {
if (insertionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertionIsMutable();
insertion_.add(index, value);
onChanged();
} else {
insertionBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder addInsertion(
ai.promoted.proto.delivery.Insertion.Builder builderForValue) {
if (insertionBuilder_ == null) {
ensureInsertionIsMutable();
insertion_.add(builderForValue.build());
onChanged();
} else {
insertionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder addInsertion(
int index, ai.promoted.proto.delivery.Insertion.Builder builderForValue) {
if (insertionBuilder_ == null) {
ensureInsertionIsMutable();
insertion_.add(index, builderForValue.build());
onChanged();
} else {
insertionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder addAllInsertion(
java.lang.Iterable extends ai.promoted.proto.delivery.Insertion> values) {
if (insertionBuilder_ == null) {
ensureInsertionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, insertion_);
onChanged();
} else {
insertionBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder clearInsertion() {
if (insertionBuilder_ == null) {
insertion_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
insertionBuilder_.clear();
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public Builder removeInsertion(int index) {
if (insertionBuilder_ == null) {
ensureInsertionIsMutable();
insertion_.remove(index);
onChanged();
} else {
insertionBuilder_.remove(index);
}
return this;
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public ai.promoted.proto.delivery.Insertion.Builder getInsertionBuilder(
int index) {
return getInsertionFieldBuilder().getBuilder(index);
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public ai.promoted.proto.delivery.InsertionOrBuilder getInsertionOrBuilder(
int index) {
if (insertionBuilder_ == null) {
return insertion_.get(index); } else {
return insertionBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public java.util.List extends ai.promoted.proto.delivery.InsertionOrBuilder>
getInsertionOrBuilderList() {
if (insertionBuilder_ != null) {
return insertionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(insertion_);
}
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public ai.promoted.proto.delivery.Insertion.Builder addInsertionBuilder() {
return getInsertionFieldBuilder().addBuilder(
ai.promoted.proto.delivery.Insertion.getDefaultInstance());
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public ai.promoted.proto.delivery.Insertion.Builder addInsertionBuilder(
int index) {
return getInsertionFieldBuilder().addBuilder(
index, ai.promoted.proto.delivery.Insertion.getDefaultInstance());
}
/**
* repeated .delivery.Insertion insertion = 11 [json_name = "insertion"];
*/
public java.util.List
getInsertionBuilderList() {
return getInsertionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.delivery.Insertion, ai.promoted.proto.delivery.Insertion.Builder, ai.promoted.proto.delivery.InsertionOrBuilder>
getInsertionFieldBuilder() {
if (insertionBuilder_ == null) {
insertionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.delivery.Insertion, ai.promoted.proto.delivery.Insertion.Builder, ai.promoted.proto.delivery.InsertionOrBuilder>(
insertion_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
insertion_ = null;
}
return insertionBuilder_;
}
private com.google.protobuf.LazyStringList insertionMatrixHeaders_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureInsertionMatrixHeadersIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
insertionMatrixHeaders_ = new com.google.protobuf.LazyStringArrayList(insertionMatrixHeaders_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @return A list containing the insertionMatrixHeaders.
*/
public com.google.protobuf.ProtocolStringList
getInsertionMatrixHeadersList() {
return insertionMatrixHeaders_.getUnmodifiableView();
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @return The count of insertionMatrixHeaders.
*/
public int getInsertionMatrixHeadersCount() {
return insertionMatrixHeaders_.size();
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param index The index of the element to return.
* @return The insertionMatrixHeaders at the given index.
*/
public java.lang.String getInsertionMatrixHeaders(int index) {
return insertionMatrixHeaders_.get(index);
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param index The index of the value to return.
* @return The bytes of the insertionMatrixHeaders at the given index.
*/
public com.google.protobuf.ByteString
getInsertionMatrixHeadersBytes(int index) {
return insertionMatrixHeaders_.getByteString(index);
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param index The index to set the value at.
* @param value The insertionMatrixHeaders to set.
* @return This builder for chaining.
*/
public Builder setInsertionMatrixHeaders(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertionMatrixHeadersIsMutable();
insertionMatrixHeaders_.set(index, value);
onChanged();
return this;
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param value The insertionMatrixHeaders to add.
* @return This builder for chaining.
*/
public Builder addInsertionMatrixHeaders(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertionMatrixHeadersIsMutable();
insertionMatrixHeaders_.add(value);
onChanged();
return this;
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param values The insertionMatrixHeaders to add.
* @return This builder for chaining.
*/
public Builder addAllInsertionMatrixHeaders(
java.lang.Iterable values) {
ensureInsertionMatrixHeadersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, insertionMatrixHeaders_);
onChanged();
return this;
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @return This builder for chaining.
*/
public Builder clearInsertionMatrixHeaders() {
insertionMatrixHeaders_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string insertion_matrix_headers = 20 [json_name = "insertionMatrixHeaders"];
* @param value The bytes of the insertionMatrixHeaders to add.
* @return This builder for chaining.
*/
public Builder addInsertionMatrixHeadersBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureInsertionMatrixHeadersIsMutable();
insertionMatrixHeaders_.add(value);
onChanged();
return this;
}
private com.google.protobuf.ListValue insertionMatrix_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.ListValue, com.google.protobuf.ListValue.Builder, com.google.protobuf.ListValueOrBuilder> insertionMatrixBuilder_;
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
* @return Whether the insertionMatrix field is set.
*/
public boolean hasInsertionMatrix() {
return insertionMatrixBuilder_ != null || insertionMatrix_ != null;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
* @return The insertionMatrix.
*/
public com.google.protobuf.ListValue getInsertionMatrix() {
if (insertionMatrixBuilder_ == null) {
return insertionMatrix_ == null ? com.google.protobuf.ListValue.getDefaultInstance() : insertionMatrix_;
} else {
return insertionMatrixBuilder_.getMessage();
}
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
public Builder setInsertionMatrix(com.google.protobuf.ListValue value) {
if (insertionMatrixBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
insertionMatrix_ = value;
onChanged();
} else {
insertionMatrixBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
public Builder setInsertionMatrix(
com.google.protobuf.ListValue.Builder builderForValue) {
if (insertionMatrixBuilder_ == null) {
insertionMatrix_ = builderForValue.build();
onChanged();
} else {
insertionMatrixBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
public Builder mergeInsertionMatrix(com.google.protobuf.ListValue value) {
if (insertionMatrixBuilder_ == null) {
if (insertionMatrix_ != null) {
insertionMatrix_ =
com.google.protobuf.ListValue.newBuilder(insertionMatrix_).mergeFrom(value).buildPartial();
} else {
insertionMatrix_ = value;
}
onChanged();
} else {
insertionMatrixBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
public Builder clearInsertionMatrix() {
if (insertionMatrixBuilder_ == null) {
insertionMatrix_ = null;
onChanged();
} else {
insertionMatrix_ = null;
insertionMatrixBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
public com.google.protobuf.ListValue.Builder getInsertionMatrixBuilder() {
onChanged();
return getInsertionMatrixFieldBuilder().getBuilder();
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
public com.google.protobuf.ListValueOrBuilder getInsertionMatrixOrBuilder() {
if (insertionMatrixBuilder_ != null) {
return insertionMatrixBuilder_.getMessageOrBuilder();
} else {
return insertionMatrix_ == null ?
com.google.protobuf.ListValue.getDefaultInstance() : insertionMatrix_;
}
}
/**
* .google.protobuf.ListValue insertion_matrix = 21 [json_name = "insertionMatrix"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.ListValue, com.google.protobuf.ListValue.Builder, com.google.protobuf.ListValueOrBuilder>
getInsertionMatrixFieldBuilder() {
if (insertionMatrixBuilder_ == null) {
insertionMatrixBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.ListValue, com.google.protobuf.ListValue.Builder, com.google.protobuf.ListValueOrBuilder>(
getInsertionMatrix(),
getParentForChildren(),
isClean());
insertionMatrix_ = null;
}
return insertionMatrixBuilder_;
}
private ai.promoted.proto.common.Properties properties_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Properties, ai.promoted.proto.common.Properties.Builder, ai.promoted.proto.common.PropertiesOrBuilder> propertiesBuilder_;
/**
* .common.Properties properties = 13 [json_name = "properties"];
* @return Whether the properties field is set.
*/
public boolean hasProperties() {
return propertiesBuilder_ != null || properties_ != null;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
* @return The properties.
*/
public ai.promoted.proto.common.Properties getProperties() {
if (propertiesBuilder_ == null) {
return properties_ == null ? ai.promoted.proto.common.Properties.getDefaultInstance() : properties_;
} else {
return propertiesBuilder_.getMessage();
}
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
public Builder setProperties(ai.promoted.proto.common.Properties value) {
if (propertiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
properties_ = value;
onChanged();
} else {
propertiesBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
public Builder setProperties(
ai.promoted.proto.common.Properties.Builder builderForValue) {
if (propertiesBuilder_ == null) {
properties_ = builderForValue.build();
onChanged();
} else {
propertiesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
public Builder mergeProperties(ai.promoted.proto.common.Properties value) {
if (propertiesBuilder_ == null) {
if (properties_ != null) {
properties_ =
ai.promoted.proto.common.Properties.newBuilder(properties_).mergeFrom(value).buildPartial();
} else {
properties_ = value;
}
onChanged();
} else {
propertiesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
public Builder clearProperties() {
if (propertiesBuilder_ == null) {
properties_ = null;
onChanged();
} else {
properties_ = null;
propertiesBuilder_ = null;
}
return this;
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
public ai.promoted.proto.common.Properties.Builder getPropertiesBuilder() {
onChanged();
return getPropertiesFieldBuilder().getBuilder();
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
public ai.promoted.proto.common.PropertiesOrBuilder getPropertiesOrBuilder() {
if (propertiesBuilder_ != null) {
return propertiesBuilder_.getMessageOrBuilder();
} else {
return properties_ == null ?
ai.promoted.proto.common.Properties.getDefaultInstance() : properties_;
}
}
/**
* .common.Properties properties = 13 [json_name = "properties"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Properties, ai.promoted.proto.common.Properties.Builder, ai.promoted.proto.common.PropertiesOrBuilder>
getPropertiesFieldBuilder() {
if (propertiesBuilder_ == null) {
propertiesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Properties, ai.promoted.proto.common.Properties.Builder, ai.promoted.proto.common.PropertiesOrBuilder>(
getProperties(),
getParentForChildren(),
isClean());
properties_ = null;
}
return propertiesBuilder_;
}
private boolean disablePersonalization_ ;
/**
* bool disable_personalization = 22 [json_name = "disablePersonalization"];
* @return The disablePersonalization.
*/
@java.lang.Override
public boolean getDisablePersonalization() {
return disablePersonalization_;
}
/**
* bool disable_personalization = 22 [json_name = "disablePersonalization"];
* @param value The disablePersonalization to set.
* @return This builder for chaining.
*/
public Builder setDisablePersonalization(boolean value) {
disablePersonalization_ = value;
onChanged();
return this;
}
/**
* bool disable_personalization = 22 [json_name = "disablePersonalization"];
* @return This builder for chaining.
*/
public Builder clearDisablePersonalization() {
disablePersonalization_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:delivery.Request)
}
// @@protoc_insertion_point(class_scope:delivery.Request)
private static final ai.promoted.proto.delivery.Request DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ai.promoted.proto.delivery.Request();
}
public static ai.promoted.proto.delivery.Request getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Request parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Request(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ai.promoted.proto.delivery.Request getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy