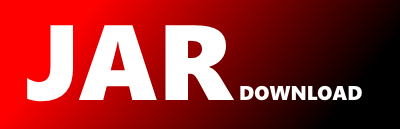
ai.promoted.proto.event.Action Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deliveryclient Show documentation
Show all versions of deliveryclient Show documentation
A Java Client to contact the Promoted.ai Delivery API.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: proto/event/event.proto
package ai.promoted.proto.event;
/**
* Protobuf type {@code event.Action}
*/
public final class Action extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:event.Action)
ActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Action.newBuilder() to construct.
private Action(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Action() {
actionId_ = "";
impressionId_ = "";
insertionId_ = "";
requestId_ = "";
viewId_ = "";
autoViewId_ = "";
sessionId_ = "";
contentId_ = "";
name_ = "";
actionType_ = 0;
customActionType_ = "";
elementId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Action();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Action(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
platformId_ = input.readUInt64();
break;
}
case 18: {
ai.promoted.proto.common.UserInfo.Builder subBuilder = null;
if (userInfo_ != null) {
subBuilder = userInfo_.toBuilder();
}
userInfo_ = input.readMessage(ai.promoted.proto.common.UserInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(userInfo_);
userInfo_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ai.promoted.proto.common.Timing.Builder subBuilder = null;
if (timing_ != null) {
subBuilder = timing_.toBuilder();
}
timing_ = input.readMessage(ai.promoted.proto.common.Timing.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timing_);
timing_ = subBuilder.buildPartial();
}
break;
}
case 34: {
ai.promoted.proto.common.ClientInfo.Builder subBuilder = null;
if (clientInfo_ != null) {
subBuilder = clientInfo_.toBuilder();
}
clientInfo_ = input.readMessage(ai.promoted.proto.common.ClientInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientInfo_);
clientInfo_ = subBuilder.buildPartial();
}
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
actionId_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
impressionId_ = s;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
insertionId_ = s;
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
requestId_ = s;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
sessionId_ = s;
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
viewId_ = s;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 112: {
int rawValue = input.readEnum();
actionType_ = rawValue;
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
customActionType_ = s;
break;
}
case 138: {
java.lang.String s = input.readStringRequireUtf8();
elementId_ = s;
break;
}
case 146: {
ai.promoted.proto.event.NavigateAction.Builder subBuilder = null;
if (actionCase_ == 18) {
subBuilder = ((ai.promoted.proto.event.NavigateAction) action_).toBuilder();
}
action_ =
input.readMessage(ai.promoted.proto.event.NavigateAction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((ai.promoted.proto.event.NavigateAction) action_);
action_ = subBuilder.buildPartial();
}
actionCase_ = 18;
break;
}
case 162: {
ai.promoted.proto.common.Properties.Builder subBuilder = null;
if (properties_ != null) {
subBuilder = properties_.toBuilder();
}
properties_ = input.readMessage(ai.promoted.proto.common.Properties.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(properties_);
properties_ = subBuilder.buildPartial();
}
break;
}
case 170: {
java.lang.String s = input.readStringRequireUtf8();
contentId_ = s;
break;
}
case 176: {
hasSuperimposedViews_ = input.readBool();
break;
}
case 186: {
java.lang.String s = input.readStringRequireUtf8();
autoViewId_ = s;
break;
}
case 194: {
ai.promoted.proto.event.IndexPath.Builder subBuilder = null;
if (clientPosition_ != null) {
subBuilder = clientPosition_.toBuilder();
}
clientPosition_ = input.readMessage(ai.promoted.proto.event.IndexPath.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientPosition_);
clientPosition_ = subBuilder.buildPartial();
}
break;
}
case 202: {
ai.promoted.proto.event.IdentifierProvenances.Builder subBuilder = null;
if (idProvenances_ != null) {
subBuilder = idProvenances_.toBuilder();
}
idProvenances_ = input.readMessage(ai.promoted.proto.event.IdentifierProvenances.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(idProvenances_);
idProvenances_ = subBuilder.buildPartial();
}
break;
}
case 210: {
ai.promoted.proto.common.Device.Builder subBuilder = null;
if (device_ != null) {
subBuilder = device_.toBuilder();
}
device_ = input.readMessage(ai.promoted.proto.common.Device.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(device_);
device_ = subBuilder.buildPartial();
}
break;
}
case 226: {
ai.promoted.proto.event.Cart.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = cart_.toBuilder();
}
cart_ = input.readMessage(ai.promoted.proto.event.Cart.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(cart_);
cart_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.event.Event.internal_static_event_Action_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.event.Event.internal_static_event_Action_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.event.Action.class, ai.promoted.proto.event.Action.Builder.class);
}
private int bitField0_;
private int actionCase_ = 0;
private java.lang.Object action_;
public enum ActionCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
NAVIGATE_ACTION(18),
ACTION_NOT_SET(0);
private final int value;
private ActionCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ActionCase valueOf(int value) {
return forNumber(value);
}
public static ActionCase forNumber(int value) {
switch (value) {
case 18: return NAVIGATE_ACTION;
case 0: return ACTION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ActionCase
getActionCase() {
return ActionCase.forNumber(
actionCase_);
}
public static final int PLATFORM_ID_FIELD_NUMBER = 1;
private long platformId_;
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @return The platformId.
*/
@java.lang.Override
public long getPlatformId() {
return platformId_;
}
public static final int USER_INFO_FIELD_NUMBER = 2;
private ai.promoted.proto.common.UserInfo userInfo_;
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return Whether the userInfo field is set.
*/
@java.lang.Override
public boolean hasUserInfo() {
return userInfo_ != null;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return The userInfo.
*/
@java.lang.Override
public ai.promoted.proto.common.UserInfo getUserInfo() {
return userInfo_ == null ? ai.promoted.proto.common.UserInfo.getDefaultInstance() : userInfo_;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
@java.lang.Override
public ai.promoted.proto.common.UserInfoOrBuilder getUserInfoOrBuilder() {
return getUserInfo();
}
public static final int TIMING_FIELD_NUMBER = 3;
private ai.promoted.proto.common.Timing timing_;
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return Whether the timing field is set.
*/
@java.lang.Override
public boolean hasTiming() {
return timing_ != null;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return The timing.
*/
@java.lang.Override
public ai.promoted.proto.common.Timing getTiming() {
return timing_ == null ? ai.promoted.proto.common.Timing.getDefaultInstance() : timing_;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
@java.lang.Override
public ai.promoted.proto.common.TimingOrBuilder getTimingOrBuilder() {
return getTiming();
}
public static final int CLIENT_INFO_FIELD_NUMBER = 4;
private ai.promoted.proto.common.ClientInfo clientInfo_;
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return Whether the clientInfo field is set.
*/
@java.lang.Override
public boolean hasClientInfo() {
return clientInfo_ != null;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return The clientInfo.
*/
@java.lang.Override
public ai.promoted.proto.common.ClientInfo getClientInfo() {
return clientInfo_ == null ? ai.promoted.proto.common.ClientInfo.getDefaultInstance() : clientInfo_;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
@java.lang.Override
public ai.promoted.proto.common.ClientInfoOrBuilder getClientInfoOrBuilder() {
return getClientInfo();
}
public static final int ACTION_ID_FIELD_NUMBER = 6;
private volatile java.lang.Object actionId_;
/**
* string action_id = 6 [json_name = "actionId"];
* @return The actionId.
*/
@java.lang.Override
public java.lang.String getActionId() {
java.lang.Object ref = actionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
actionId_ = s;
return s;
}
}
/**
* string action_id = 6 [json_name = "actionId"];
* @return The bytes for actionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getActionIdBytes() {
java.lang.Object ref = actionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMPRESSION_ID_FIELD_NUMBER = 7;
private volatile java.lang.Object impressionId_;
/**
* string impression_id = 7 [json_name = "impressionId"];
* @return The impressionId.
*/
@java.lang.Override
public java.lang.String getImpressionId() {
java.lang.Object ref = impressionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
impressionId_ = s;
return s;
}
}
/**
* string impression_id = 7 [json_name = "impressionId"];
* @return The bytes for impressionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getImpressionIdBytes() {
java.lang.Object ref = impressionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
impressionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSERTION_ID_FIELD_NUMBER = 8;
private volatile java.lang.Object insertionId_;
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @return The insertionId.
*/
@java.lang.Override
public java.lang.String getInsertionId() {
java.lang.Object ref = insertionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
insertionId_ = s;
return s;
}
}
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @return The bytes for insertionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInsertionIdBytes() {
java.lang.Object ref = insertionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
insertionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_ID_FIELD_NUMBER = 9;
private volatile java.lang.Object requestId_;
/**
* string request_id = 9 [json_name = "requestId"];
* @return The requestId.
*/
@java.lang.Override
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
}
}
/**
* string request_id = 9 [json_name = "requestId"];
* @return The bytes for requestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VIEW_ID_FIELD_NUMBER = 11;
private volatile java.lang.Object viewId_;
/**
* string view_id = 11 [json_name = "viewId"];
* @return The viewId.
*/
@java.lang.Override
public java.lang.String getViewId() {
java.lang.Object ref = viewId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
viewId_ = s;
return s;
}
}
/**
* string view_id = 11 [json_name = "viewId"];
* @return The bytes for viewId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getViewIdBytes() {
java.lang.Object ref = viewId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
viewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTO_VIEW_ID_FIELD_NUMBER = 23;
private volatile java.lang.Object autoViewId_;
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @return The autoViewId.
*/
@java.lang.Override
public java.lang.String getAutoViewId() {
java.lang.Object ref = autoViewId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
autoViewId_ = s;
return s;
}
}
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @return The bytes for autoViewId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAutoViewIdBytes() {
java.lang.Object ref = autoViewId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
autoViewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_ID_FIELD_NUMBER = 10;
private volatile java.lang.Object sessionId_;
/**
* string session_id = 10 [json_name = "sessionId"];
* @return The sessionId.
*/
@java.lang.Override
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
* string session_id = 10 [json_name = "sessionId"];
* @return The bytes for sessionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTENT_ID_FIELD_NUMBER = 21;
private volatile java.lang.Object contentId_;
/**
* string content_id = 21 [json_name = "contentId"];
* @return The contentId.
*/
@java.lang.Override
public java.lang.String getContentId() {
java.lang.Object ref = contentId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contentId_ = s;
return s;
}
}
/**
* string content_id = 21 [json_name = "contentId"];
* @return The bytes for contentId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContentIdBytes() {
java.lang.Object ref = contentId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contentId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 12;
private volatile java.lang.Object name_;
/**
* string name = 12 [json_name = "name"];
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 12 [json_name = "name"];
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTION_TYPE_FIELD_NUMBER = 14;
private int actionType_;
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @return The enum numeric value on the wire for actionType.
*/
@java.lang.Override public int getActionTypeValue() {
return actionType_;
}
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @return The actionType.
*/
@java.lang.Override public ai.promoted.proto.event.ActionType getActionType() {
@SuppressWarnings("deprecation")
ai.promoted.proto.event.ActionType result = ai.promoted.proto.event.ActionType.valueOf(actionType_);
return result == null ? ai.promoted.proto.event.ActionType.UNRECOGNIZED : result;
}
public static final int CUSTOM_ACTION_TYPE_FIELD_NUMBER = 15;
private volatile java.lang.Object customActionType_;
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @return The customActionType.
*/
@java.lang.Override
public java.lang.String getCustomActionType() {
java.lang.Object ref = customActionType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customActionType_ = s;
return s;
}
}
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @return The bytes for customActionType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomActionTypeBytes() {
java.lang.Object ref = customActionType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customActionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ELEMENT_ID_FIELD_NUMBER = 17;
private volatile java.lang.Object elementId_;
/**
* string element_id = 17 [json_name = "elementId"];
* @return The elementId.
*/
@java.lang.Override
public java.lang.String getElementId() {
java.lang.Object ref = elementId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
elementId_ = s;
return s;
}
}
/**
* string element_id = 17 [json_name = "elementId"];
* @return The bytes for elementId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getElementIdBytes() {
java.lang.Object ref = elementId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
elementId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAVIGATE_ACTION_FIELD_NUMBER = 18;
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
* @return Whether the navigateAction field is set.
*/
@java.lang.Override
public boolean hasNavigateAction() {
return actionCase_ == 18;
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
* @return The navigateAction.
*/
@java.lang.Override
public ai.promoted.proto.event.NavigateAction getNavigateAction() {
if (actionCase_ == 18) {
return (ai.promoted.proto.event.NavigateAction) action_;
}
return ai.promoted.proto.event.NavigateAction.getDefaultInstance();
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
@java.lang.Override
public ai.promoted.proto.event.NavigateActionOrBuilder getNavigateActionOrBuilder() {
if (actionCase_ == 18) {
return (ai.promoted.proto.event.NavigateAction) action_;
}
return ai.promoted.proto.event.NavigateAction.getDefaultInstance();
}
public static final int HAS_SUPERIMPOSED_VIEWS_FIELD_NUMBER = 22;
private boolean hasSuperimposedViews_;
/**
* bool has_superimposed_views = 22 [json_name = "hasSuperimposedViews"];
* @return The hasSuperimposedViews.
*/
@java.lang.Override
public boolean getHasSuperimposedViews() {
return hasSuperimposedViews_;
}
public static final int CLIENT_POSITION_FIELD_NUMBER = 24;
private ai.promoted.proto.event.IndexPath clientPosition_;
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
* @return Whether the clientPosition field is set.
*/
@java.lang.Override
public boolean hasClientPosition() {
return clientPosition_ != null;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
* @return The clientPosition.
*/
@java.lang.Override
public ai.promoted.proto.event.IndexPath getClientPosition() {
return clientPosition_ == null ? ai.promoted.proto.event.IndexPath.getDefaultInstance() : clientPosition_;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
@java.lang.Override
public ai.promoted.proto.event.IndexPathOrBuilder getClientPositionOrBuilder() {
return getClientPosition();
}
public static final int ID_PROVENANCES_FIELD_NUMBER = 25;
private ai.promoted.proto.event.IdentifierProvenances idProvenances_;
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
* @return Whether the idProvenances field is set.
*/
@java.lang.Override
public boolean hasIdProvenances() {
return idProvenances_ != null;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
* @return The idProvenances.
*/
@java.lang.Override
public ai.promoted.proto.event.IdentifierProvenances getIdProvenances() {
return idProvenances_ == null ? ai.promoted.proto.event.IdentifierProvenances.getDefaultInstance() : idProvenances_;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
@java.lang.Override
public ai.promoted.proto.event.IdentifierProvenancesOrBuilder getIdProvenancesOrBuilder() {
return getIdProvenances();
}
public static final int PROPERTIES_FIELD_NUMBER = 20;
private ai.promoted.proto.common.Properties properties_;
/**
* .common.Properties properties = 20 [json_name = "properties"];
* @return Whether the properties field is set.
*/
@java.lang.Override
public boolean hasProperties() {
return properties_ != null;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
* @return The properties.
*/
@java.lang.Override
public ai.promoted.proto.common.Properties getProperties() {
return properties_ == null ? ai.promoted.proto.common.Properties.getDefaultInstance() : properties_;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
@java.lang.Override
public ai.promoted.proto.common.PropertiesOrBuilder getPropertiesOrBuilder() {
return getProperties();
}
public static final int DEVICE_FIELD_NUMBER = 26;
private ai.promoted.proto.common.Device device_;
/**
* .common.Device device = 26 [json_name = "device"];
* @return Whether the device field is set.
*/
@java.lang.Override
public boolean hasDevice() {
return device_ != null;
}
/**
* .common.Device device = 26 [json_name = "device"];
* @return The device.
*/
@java.lang.Override
public ai.promoted.proto.common.Device getDevice() {
return device_ == null ? ai.promoted.proto.common.Device.getDefaultInstance() : device_;
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
@java.lang.Override
public ai.promoted.proto.common.DeviceOrBuilder getDeviceOrBuilder() {
return getDevice();
}
public static final int CART_FIELD_NUMBER = 28;
private ai.promoted.proto.event.Cart cart_;
/**
* .event.Cart cart = 28 [json_name = "cart"];
* @return Whether the cart field is set.
*/
@java.lang.Override
public boolean hasCart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
* @return The cart.
*/
@java.lang.Override
public ai.promoted.proto.event.Cart getCart() {
return cart_ == null ? ai.promoted.proto.event.Cart.getDefaultInstance() : cart_;
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
@java.lang.Override
public ai.promoted.proto.event.CartOrBuilder getCartOrBuilder() {
return cart_ == null ? ai.promoted.proto.event.Cart.getDefaultInstance() : cart_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (platformId_ != 0L) {
output.writeUInt64(1, platformId_);
}
if (userInfo_ != null) {
output.writeMessage(2, getUserInfo());
}
if (timing_ != null) {
output.writeMessage(3, getTiming());
}
if (clientInfo_ != null) {
output.writeMessage(4, getClientInfo());
}
if (!getActionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, actionId_);
}
if (!getImpressionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, impressionId_);
}
if (!getInsertionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, insertionId_);
}
if (!getRequestIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, requestId_);
}
if (!getSessionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, sessionId_);
}
if (!getViewIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, viewId_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, name_);
}
if (actionType_ != ai.promoted.proto.event.ActionType.UNKNOWN_ACTION_TYPE.getNumber()) {
output.writeEnum(14, actionType_);
}
if (!getCustomActionTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, customActionType_);
}
if (!getElementIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, elementId_);
}
if (actionCase_ == 18) {
output.writeMessage(18, (ai.promoted.proto.event.NavigateAction) action_);
}
if (properties_ != null) {
output.writeMessage(20, getProperties());
}
if (!getContentIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, contentId_);
}
if (hasSuperimposedViews_ != false) {
output.writeBool(22, hasSuperimposedViews_);
}
if (!getAutoViewIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 23, autoViewId_);
}
if (clientPosition_ != null) {
output.writeMessage(24, getClientPosition());
}
if (idProvenances_ != null) {
output.writeMessage(25, getIdProvenances());
}
if (device_ != null) {
output.writeMessage(26, getDevice());
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(28, getCart());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (platformId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, platformId_);
}
if (userInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getUserInfo());
}
if (timing_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getTiming());
}
if (clientInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getClientInfo());
}
if (!getActionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, actionId_);
}
if (!getImpressionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, impressionId_);
}
if (!getInsertionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, insertionId_);
}
if (!getRequestIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, requestId_);
}
if (!getSessionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, sessionId_);
}
if (!getViewIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, viewId_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, name_);
}
if (actionType_ != ai.promoted.proto.event.ActionType.UNKNOWN_ACTION_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, actionType_);
}
if (!getCustomActionTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, customActionType_);
}
if (!getElementIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, elementId_);
}
if (actionCase_ == 18) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, (ai.promoted.proto.event.NavigateAction) action_);
}
if (properties_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, getProperties());
}
if (!getContentIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, contentId_);
}
if (hasSuperimposedViews_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(22, hasSuperimposedViews_);
}
if (!getAutoViewIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(23, autoViewId_);
}
if (clientPosition_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, getClientPosition());
}
if (idProvenances_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, getIdProvenances());
}
if (device_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, getDevice());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(28, getCart());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ai.promoted.proto.event.Action)) {
return super.equals(obj);
}
ai.promoted.proto.event.Action other = (ai.promoted.proto.event.Action) obj;
if (getPlatformId()
!= other.getPlatformId()) return false;
if (hasUserInfo() != other.hasUserInfo()) return false;
if (hasUserInfo()) {
if (!getUserInfo()
.equals(other.getUserInfo())) return false;
}
if (hasTiming() != other.hasTiming()) return false;
if (hasTiming()) {
if (!getTiming()
.equals(other.getTiming())) return false;
}
if (hasClientInfo() != other.hasClientInfo()) return false;
if (hasClientInfo()) {
if (!getClientInfo()
.equals(other.getClientInfo())) return false;
}
if (!getActionId()
.equals(other.getActionId())) return false;
if (!getImpressionId()
.equals(other.getImpressionId())) return false;
if (!getInsertionId()
.equals(other.getInsertionId())) return false;
if (!getRequestId()
.equals(other.getRequestId())) return false;
if (!getViewId()
.equals(other.getViewId())) return false;
if (!getAutoViewId()
.equals(other.getAutoViewId())) return false;
if (!getSessionId()
.equals(other.getSessionId())) return false;
if (!getContentId()
.equals(other.getContentId())) return false;
if (!getName()
.equals(other.getName())) return false;
if (actionType_ != other.actionType_) return false;
if (!getCustomActionType()
.equals(other.getCustomActionType())) return false;
if (!getElementId()
.equals(other.getElementId())) return false;
if (getHasSuperimposedViews()
!= other.getHasSuperimposedViews()) return false;
if (hasClientPosition() != other.hasClientPosition()) return false;
if (hasClientPosition()) {
if (!getClientPosition()
.equals(other.getClientPosition())) return false;
}
if (hasIdProvenances() != other.hasIdProvenances()) return false;
if (hasIdProvenances()) {
if (!getIdProvenances()
.equals(other.getIdProvenances())) return false;
}
if (hasProperties() != other.hasProperties()) return false;
if (hasProperties()) {
if (!getProperties()
.equals(other.getProperties())) return false;
}
if (hasDevice() != other.hasDevice()) return false;
if (hasDevice()) {
if (!getDevice()
.equals(other.getDevice())) return false;
}
if (hasCart() != other.hasCart()) return false;
if (hasCart()) {
if (!getCart()
.equals(other.getCart())) return false;
}
if (!getActionCase().equals(other.getActionCase())) return false;
switch (actionCase_) {
case 18:
if (!getNavigateAction()
.equals(other.getNavigateAction())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PLATFORM_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPlatformId());
if (hasUserInfo()) {
hash = (37 * hash) + USER_INFO_FIELD_NUMBER;
hash = (53 * hash) + getUserInfo().hashCode();
}
if (hasTiming()) {
hash = (37 * hash) + TIMING_FIELD_NUMBER;
hash = (53 * hash) + getTiming().hashCode();
}
if (hasClientInfo()) {
hash = (37 * hash) + CLIENT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getClientInfo().hashCode();
}
hash = (37 * hash) + ACTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getActionId().hashCode();
hash = (37 * hash) + IMPRESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getImpressionId().hashCode();
hash = (37 * hash) + INSERTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getInsertionId().hashCode();
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestId().hashCode();
hash = (37 * hash) + VIEW_ID_FIELD_NUMBER;
hash = (53 * hash) + getViewId().hashCode();
hash = (37 * hash) + AUTO_VIEW_ID_FIELD_NUMBER;
hash = (53 * hash) + getAutoViewId().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
hash = (37 * hash) + CONTENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getContentId().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + ACTION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + actionType_;
hash = (37 * hash) + CUSTOM_ACTION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getCustomActionType().hashCode();
hash = (37 * hash) + ELEMENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getElementId().hashCode();
hash = (37 * hash) + HAS_SUPERIMPOSED_VIEWS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasSuperimposedViews());
if (hasClientPosition()) {
hash = (37 * hash) + CLIENT_POSITION_FIELD_NUMBER;
hash = (53 * hash) + getClientPosition().hashCode();
}
if (hasIdProvenances()) {
hash = (37 * hash) + ID_PROVENANCES_FIELD_NUMBER;
hash = (53 * hash) + getIdProvenances().hashCode();
}
if (hasProperties()) {
hash = (37 * hash) + PROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + getProperties().hashCode();
}
if (hasDevice()) {
hash = (37 * hash) + DEVICE_FIELD_NUMBER;
hash = (53 * hash) + getDevice().hashCode();
}
if (hasCart()) {
hash = (37 * hash) + CART_FIELD_NUMBER;
hash = (53 * hash) + getCart().hashCode();
}
switch (actionCase_) {
case 18:
hash = (37 * hash) + NAVIGATE_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getNavigateAction().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ai.promoted.proto.event.Action parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.event.Action parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.event.Action parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.event.Action parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.event.Action parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.event.Action parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.event.Action parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.event.Action parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.event.Action parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ai.promoted.proto.event.Action parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.event.Action parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.event.Action parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ai.promoted.proto.event.Action prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code event.Action}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:event.Action)
ai.promoted.proto.event.ActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.event.Event.internal_static_event_Action_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.event.Event.internal_static_event_Action_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.event.Action.class, ai.promoted.proto.event.Action.Builder.class);
}
// Construct using ai.promoted.proto.event.Action.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCartFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
platformId_ = 0L;
if (userInfoBuilder_ == null) {
userInfo_ = null;
} else {
userInfo_ = null;
userInfoBuilder_ = null;
}
if (timingBuilder_ == null) {
timing_ = null;
} else {
timing_ = null;
timingBuilder_ = null;
}
if (clientInfoBuilder_ == null) {
clientInfo_ = null;
} else {
clientInfo_ = null;
clientInfoBuilder_ = null;
}
actionId_ = "";
impressionId_ = "";
insertionId_ = "";
requestId_ = "";
viewId_ = "";
autoViewId_ = "";
sessionId_ = "";
contentId_ = "";
name_ = "";
actionType_ = 0;
customActionType_ = "";
elementId_ = "";
hasSuperimposedViews_ = false;
if (clientPositionBuilder_ == null) {
clientPosition_ = null;
} else {
clientPosition_ = null;
clientPositionBuilder_ = null;
}
if (idProvenancesBuilder_ == null) {
idProvenances_ = null;
} else {
idProvenances_ = null;
idProvenancesBuilder_ = null;
}
if (propertiesBuilder_ == null) {
properties_ = null;
} else {
properties_ = null;
propertiesBuilder_ = null;
}
if (deviceBuilder_ == null) {
device_ = null;
} else {
device_ = null;
deviceBuilder_ = null;
}
if (cartBuilder_ == null) {
cart_ = null;
} else {
cartBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
actionCase_ = 0;
action_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ai.promoted.proto.event.Event.internal_static_event_Action_descriptor;
}
@java.lang.Override
public ai.promoted.proto.event.Action getDefaultInstanceForType() {
return ai.promoted.proto.event.Action.getDefaultInstance();
}
@java.lang.Override
public ai.promoted.proto.event.Action build() {
ai.promoted.proto.event.Action result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ai.promoted.proto.event.Action buildPartial() {
ai.promoted.proto.event.Action result = new ai.promoted.proto.event.Action(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.platformId_ = platformId_;
if (userInfoBuilder_ == null) {
result.userInfo_ = userInfo_;
} else {
result.userInfo_ = userInfoBuilder_.build();
}
if (timingBuilder_ == null) {
result.timing_ = timing_;
} else {
result.timing_ = timingBuilder_.build();
}
if (clientInfoBuilder_ == null) {
result.clientInfo_ = clientInfo_;
} else {
result.clientInfo_ = clientInfoBuilder_.build();
}
result.actionId_ = actionId_;
result.impressionId_ = impressionId_;
result.insertionId_ = insertionId_;
result.requestId_ = requestId_;
result.viewId_ = viewId_;
result.autoViewId_ = autoViewId_;
result.sessionId_ = sessionId_;
result.contentId_ = contentId_;
result.name_ = name_;
result.actionType_ = actionType_;
result.customActionType_ = customActionType_;
result.elementId_ = elementId_;
if (actionCase_ == 18) {
if (navigateActionBuilder_ == null) {
result.action_ = action_;
} else {
result.action_ = navigateActionBuilder_.build();
}
}
result.hasSuperimposedViews_ = hasSuperimposedViews_;
if (clientPositionBuilder_ == null) {
result.clientPosition_ = clientPosition_;
} else {
result.clientPosition_ = clientPositionBuilder_.build();
}
if (idProvenancesBuilder_ == null) {
result.idProvenances_ = idProvenances_;
} else {
result.idProvenances_ = idProvenancesBuilder_.build();
}
if (propertiesBuilder_ == null) {
result.properties_ = properties_;
} else {
result.properties_ = propertiesBuilder_.build();
}
if (deviceBuilder_ == null) {
result.device_ = device_;
} else {
result.device_ = deviceBuilder_.build();
}
if (((from_bitField0_ & 0x00000001) != 0)) {
if (cartBuilder_ == null) {
result.cart_ = cart_;
} else {
result.cart_ = cartBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
result.bitField0_ = to_bitField0_;
result.actionCase_ = actionCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ai.promoted.proto.event.Action) {
return mergeFrom((ai.promoted.proto.event.Action)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ai.promoted.proto.event.Action other) {
if (other == ai.promoted.proto.event.Action.getDefaultInstance()) return this;
if (other.getPlatformId() != 0L) {
setPlatformId(other.getPlatformId());
}
if (other.hasUserInfo()) {
mergeUserInfo(other.getUserInfo());
}
if (other.hasTiming()) {
mergeTiming(other.getTiming());
}
if (other.hasClientInfo()) {
mergeClientInfo(other.getClientInfo());
}
if (!other.getActionId().isEmpty()) {
actionId_ = other.actionId_;
onChanged();
}
if (!other.getImpressionId().isEmpty()) {
impressionId_ = other.impressionId_;
onChanged();
}
if (!other.getInsertionId().isEmpty()) {
insertionId_ = other.insertionId_;
onChanged();
}
if (!other.getRequestId().isEmpty()) {
requestId_ = other.requestId_;
onChanged();
}
if (!other.getViewId().isEmpty()) {
viewId_ = other.viewId_;
onChanged();
}
if (!other.getAutoViewId().isEmpty()) {
autoViewId_ = other.autoViewId_;
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
onChanged();
}
if (!other.getContentId().isEmpty()) {
contentId_ = other.contentId_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.actionType_ != 0) {
setActionTypeValue(other.getActionTypeValue());
}
if (!other.getCustomActionType().isEmpty()) {
customActionType_ = other.customActionType_;
onChanged();
}
if (!other.getElementId().isEmpty()) {
elementId_ = other.elementId_;
onChanged();
}
if (other.getHasSuperimposedViews() != false) {
setHasSuperimposedViews(other.getHasSuperimposedViews());
}
if (other.hasClientPosition()) {
mergeClientPosition(other.getClientPosition());
}
if (other.hasIdProvenances()) {
mergeIdProvenances(other.getIdProvenances());
}
if (other.hasProperties()) {
mergeProperties(other.getProperties());
}
if (other.hasDevice()) {
mergeDevice(other.getDevice());
}
if (other.hasCart()) {
mergeCart(other.getCart());
}
switch (other.getActionCase()) {
case NAVIGATE_ACTION: {
mergeNavigateAction(other.getNavigateAction());
break;
}
case ACTION_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ai.promoted.proto.event.Action parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ai.promoted.proto.event.Action) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int actionCase_ = 0;
private java.lang.Object action_;
public ActionCase
getActionCase() {
return ActionCase.forNumber(
actionCase_);
}
public Builder clearAction() {
actionCase_ = 0;
action_ = null;
onChanged();
return this;
}
private int bitField0_;
private long platformId_ ;
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @return The platformId.
*/
@java.lang.Override
public long getPlatformId() {
return platformId_;
}
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @param value The platformId to set.
* @return This builder for chaining.
*/
public Builder setPlatformId(long value) {
platformId_ = value;
onChanged();
return this;
}
/**
* uint64 platform_id = 1 [json_name = "platformId"];
* @return This builder for chaining.
*/
public Builder clearPlatformId() {
platformId_ = 0L;
onChanged();
return this;
}
private ai.promoted.proto.common.UserInfo userInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.UserInfo, ai.promoted.proto.common.UserInfo.Builder, ai.promoted.proto.common.UserInfoOrBuilder> userInfoBuilder_;
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return Whether the userInfo field is set.
*/
public boolean hasUserInfo() {
return userInfoBuilder_ != null || userInfo_ != null;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
* @return The userInfo.
*/
public ai.promoted.proto.common.UserInfo getUserInfo() {
if (userInfoBuilder_ == null) {
return userInfo_ == null ? ai.promoted.proto.common.UserInfo.getDefaultInstance() : userInfo_;
} else {
return userInfoBuilder_.getMessage();
}
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder setUserInfo(ai.promoted.proto.common.UserInfo value) {
if (userInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userInfo_ = value;
onChanged();
} else {
userInfoBuilder_.setMessage(value);
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder setUserInfo(
ai.promoted.proto.common.UserInfo.Builder builderForValue) {
if (userInfoBuilder_ == null) {
userInfo_ = builderForValue.build();
onChanged();
} else {
userInfoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder mergeUserInfo(ai.promoted.proto.common.UserInfo value) {
if (userInfoBuilder_ == null) {
if (userInfo_ != null) {
userInfo_ =
ai.promoted.proto.common.UserInfo.newBuilder(userInfo_).mergeFrom(value).buildPartial();
} else {
userInfo_ = value;
}
onChanged();
} else {
userInfoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public Builder clearUserInfo() {
if (userInfoBuilder_ == null) {
userInfo_ = null;
onChanged();
} else {
userInfo_ = null;
userInfoBuilder_ = null;
}
return this;
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public ai.promoted.proto.common.UserInfo.Builder getUserInfoBuilder() {
onChanged();
return getUserInfoFieldBuilder().getBuilder();
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
public ai.promoted.proto.common.UserInfoOrBuilder getUserInfoOrBuilder() {
if (userInfoBuilder_ != null) {
return userInfoBuilder_.getMessageOrBuilder();
} else {
return userInfo_ == null ?
ai.promoted.proto.common.UserInfo.getDefaultInstance() : userInfo_;
}
}
/**
* .common.UserInfo user_info = 2 [json_name = "userInfo"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.UserInfo, ai.promoted.proto.common.UserInfo.Builder, ai.promoted.proto.common.UserInfoOrBuilder>
getUserInfoFieldBuilder() {
if (userInfoBuilder_ == null) {
userInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.UserInfo, ai.promoted.proto.common.UserInfo.Builder, ai.promoted.proto.common.UserInfoOrBuilder>(
getUserInfo(),
getParentForChildren(),
isClean());
userInfo_ = null;
}
return userInfoBuilder_;
}
private ai.promoted.proto.common.Timing timing_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Timing, ai.promoted.proto.common.Timing.Builder, ai.promoted.proto.common.TimingOrBuilder> timingBuilder_;
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return Whether the timing field is set.
*/
public boolean hasTiming() {
return timingBuilder_ != null || timing_ != null;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
* @return The timing.
*/
public ai.promoted.proto.common.Timing getTiming() {
if (timingBuilder_ == null) {
return timing_ == null ? ai.promoted.proto.common.Timing.getDefaultInstance() : timing_;
} else {
return timingBuilder_.getMessage();
}
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder setTiming(ai.promoted.proto.common.Timing value) {
if (timingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timing_ = value;
onChanged();
} else {
timingBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder setTiming(
ai.promoted.proto.common.Timing.Builder builderForValue) {
if (timingBuilder_ == null) {
timing_ = builderForValue.build();
onChanged();
} else {
timingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder mergeTiming(ai.promoted.proto.common.Timing value) {
if (timingBuilder_ == null) {
if (timing_ != null) {
timing_ =
ai.promoted.proto.common.Timing.newBuilder(timing_).mergeFrom(value).buildPartial();
} else {
timing_ = value;
}
onChanged();
} else {
timingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public Builder clearTiming() {
if (timingBuilder_ == null) {
timing_ = null;
onChanged();
} else {
timing_ = null;
timingBuilder_ = null;
}
return this;
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public ai.promoted.proto.common.Timing.Builder getTimingBuilder() {
onChanged();
return getTimingFieldBuilder().getBuilder();
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
public ai.promoted.proto.common.TimingOrBuilder getTimingOrBuilder() {
if (timingBuilder_ != null) {
return timingBuilder_.getMessageOrBuilder();
} else {
return timing_ == null ?
ai.promoted.proto.common.Timing.getDefaultInstance() : timing_;
}
}
/**
* .common.Timing timing = 3 [json_name = "timing"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Timing, ai.promoted.proto.common.Timing.Builder, ai.promoted.proto.common.TimingOrBuilder>
getTimingFieldBuilder() {
if (timingBuilder_ == null) {
timingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Timing, ai.promoted.proto.common.Timing.Builder, ai.promoted.proto.common.TimingOrBuilder>(
getTiming(),
getParentForChildren(),
isClean());
timing_ = null;
}
return timingBuilder_;
}
private ai.promoted.proto.common.ClientInfo clientInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.ClientInfo, ai.promoted.proto.common.ClientInfo.Builder, ai.promoted.proto.common.ClientInfoOrBuilder> clientInfoBuilder_;
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return Whether the clientInfo field is set.
*/
public boolean hasClientInfo() {
return clientInfoBuilder_ != null || clientInfo_ != null;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
* @return The clientInfo.
*/
public ai.promoted.proto.common.ClientInfo getClientInfo() {
if (clientInfoBuilder_ == null) {
return clientInfo_ == null ? ai.promoted.proto.common.ClientInfo.getDefaultInstance() : clientInfo_;
} else {
return clientInfoBuilder_.getMessage();
}
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder setClientInfo(ai.promoted.proto.common.ClientInfo value) {
if (clientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientInfo_ = value;
onChanged();
} else {
clientInfoBuilder_.setMessage(value);
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder setClientInfo(
ai.promoted.proto.common.ClientInfo.Builder builderForValue) {
if (clientInfoBuilder_ == null) {
clientInfo_ = builderForValue.build();
onChanged();
} else {
clientInfoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder mergeClientInfo(ai.promoted.proto.common.ClientInfo value) {
if (clientInfoBuilder_ == null) {
if (clientInfo_ != null) {
clientInfo_ =
ai.promoted.proto.common.ClientInfo.newBuilder(clientInfo_).mergeFrom(value).buildPartial();
} else {
clientInfo_ = value;
}
onChanged();
} else {
clientInfoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public Builder clearClientInfo() {
if (clientInfoBuilder_ == null) {
clientInfo_ = null;
onChanged();
} else {
clientInfo_ = null;
clientInfoBuilder_ = null;
}
return this;
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public ai.promoted.proto.common.ClientInfo.Builder getClientInfoBuilder() {
onChanged();
return getClientInfoFieldBuilder().getBuilder();
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
public ai.promoted.proto.common.ClientInfoOrBuilder getClientInfoOrBuilder() {
if (clientInfoBuilder_ != null) {
return clientInfoBuilder_.getMessageOrBuilder();
} else {
return clientInfo_ == null ?
ai.promoted.proto.common.ClientInfo.getDefaultInstance() : clientInfo_;
}
}
/**
* .common.ClientInfo client_info = 4 [json_name = "clientInfo"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.ClientInfo, ai.promoted.proto.common.ClientInfo.Builder, ai.promoted.proto.common.ClientInfoOrBuilder>
getClientInfoFieldBuilder() {
if (clientInfoBuilder_ == null) {
clientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.ClientInfo, ai.promoted.proto.common.ClientInfo.Builder, ai.promoted.proto.common.ClientInfoOrBuilder>(
getClientInfo(),
getParentForChildren(),
isClean());
clientInfo_ = null;
}
return clientInfoBuilder_;
}
private java.lang.Object actionId_ = "";
/**
* string action_id = 6 [json_name = "actionId"];
* @return The actionId.
*/
public java.lang.String getActionId() {
java.lang.Object ref = actionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
actionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string action_id = 6 [json_name = "actionId"];
* @return The bytes for actionId.
*/
public com.google.protobuf.ByteString
getActionIdBytes() {
java.lang.Object ref = actionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string action_id = 6 [json_name = "actionId"];
* @param value The actionId to set.
* @return This builder for chaining.
*/
public Builder setActionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
actionId_ = value;
onChanged();
return this;
}
/**
* string action_id = 6 [json_name = "actionId"];
* @return This builder for chaining.
*/
public Builder clearActionId() {
actionId_ = getDefaultInstance().getActionId();
onChanged();
return this;
}
/**
* string action_id = 6 [json_name = "actionId"];
* @param value The bytes for actionId to set.
* @return This builder for chaining.
*/
public Builder setActionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
actionId_ = value;
onChanged();
return this;
}
private java.lang.Object impressionId_ = "";
/**
* string impression_id = 7 [json_name = "impressionId"];
* @return The impressionId.
*/
public java.lang.String getImpressionId() {
java.lang.Object ref = impressionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
impressionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string impression_id = 7 [json_name = "impressionId"];
* @return The bytes for impressionId.
*/
public com.google.protobuf.ByteString
getImpressionIdBytes() {
java.lang.Object ref = impressionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
impressionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string impression_id = 7 [json_name = "impressionId"];
* @param value The impressionId to set.
* @return This builder for chaining.
*/
public Builder setImpressionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
impressionId_ = value;
onChanged();
return this;
}
/**
* string impression_id = 7 [json_name = "impressionId"];
* @return This builder for chaining.
*/
public Builder clearImpressionId() {
impressionId_ = getDefaultInstance().getImpressionId();
onChanged();
return this;
}
/**
* string impression_id = 7 [json_name = "impressionId"];
* @param value The bytes for impressionId to set.
* @return This builder for chaining.
*/
public Builder setImpressionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
impressionId_ = value;
onChanged();
return this;
}
private java.lang.Object insertionId_ = "";
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @return The insertionId.
*/
public java.lang.String getInsertionId() {
java.lang.Object ref = insertionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
insertionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @return The bytes for insertionId.
*/
public com.google.protobuf.ByteString
getInsertionIdBytes() {
java.lang.Object ref = insertionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
insertionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @param value The insertionId to set.
* @return This builder for chaining.
*/
public Builder setInsertionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
insertionId_ = value;
onChanged();
return this;
}
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @return This builder for chaining.
*/
public Builder clearInsertionId() {
insertionId_ = getDefaultInstance().getInsertionId();
onChanged();
return this;
}
/**
* string insertion_id = 8 [json_name = "insertionId"];
* @param value The bytes for insertionId to set.
* @return This builder for chaining.
*/
public Builder setInsertionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
insertionId_ = value;
onChanged();
return this;
}
private java.lang.Object requestId_ = "";
/**
* string request_id = 9 [json_name = "requestId"];
* @return The requestId.
*/
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string request_id = 9 [json_name = "requestId"];
* @return The bytes for requestId.
*/
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string request_id = 9 [json_name = "requestId"];
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestId_ = value;
onChanged();
return this;
}
/**
* string request_id = 9 [json_name = "requestId"];
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = getDefaultInstance().getRequestId();
onChanged();
return this;
}
/**
* string request_id = 9 [json_name = "requestId"];
* @param value The bytes for requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestId_ = value;
onChanged();
return this;
}
private java.lang.Object viewId_ = "";
/**
* string view_id = 11 [json_name = "viewId"];
* @return The viewId.
*/
public java.lang.String getViewId() {
java.lang.Object ref = viewId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
viewId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string view_id = 11 [json_name = "viewId"];
* @return The bytes for viewId.
*/
public com.google.protobuf.ByteString
getViewIdBytes() {
java.lang.Object ref = viewId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
viewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string view_id = 11 [json_name = "viewId"];
* @param value The viewId to set.
* @return This builder for chaining.
*/
public Builder setViewId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
viewId_ = value;
onChanged();
return this;
}
/**
* string view_id = 11 [json_name = "viewId"];
* @return This builder for chaining.
*/
public Builder clearViewId() {
viewId_ = getDefaultInstance().getViewId();
onChanged();
return this;
}
/**
* string view_id = 11 [json_name = "viewId"];
* @param value The bytes for viewId to set.
* @return This builder for chaining.
*/
public Builder setViewIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
viewId_ = value;
onChanged();
return this;
}
private java.lang.Object autoViewId_ = "";
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @return The autoViewId.
*/
public java.lang.String getAutoViewId() {
java.lang.Object ref = autoViewId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
autoViewId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @return The bytes for autoViewId.
*/
public com.google.protobuf.ByteString
getAutoViewIdBytes() {
java.lang.Object ref = autoViewId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
autoViewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @param value The autoViewId to set.
* @return This builder for chaining.
*/
public Builder setAutoViewId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
autoViewId_ = value;
onChanged();
return this;
}
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @return This builder for chaining.
*/
public Builder clearAutoViewId() {
autoViewId_ = getDefaultInstance().getAutoViewId();
onChanged();
return this;
}
/**
* string auto_view_id = 23 [json_name = "autoViewId"];
* @param value The bytes for autoViewId to set.
* @return This builder for chaining.
*/
public Builder setAutoViewIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
autoViewId_ = value;
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
* string session_id = 10 [json_name = "sessionId"];
* @return The sessionId.
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string session_id = 10 [json_name = "sessionId"];
* @return The bytes for sessionId.
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string session_id = 10 [json_name = "sessionId"];
* @param value The sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
onChanged();
return this;
}
/**
* string session_id = 10 [json_name = "sessionId"];
* @return This builder for chaining.
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
* string session_id = 10 [json_name = "sessionId"];
* @param value The bytes for sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
onChanged();
return this;
}
private java.lang.Object contentId_ = "";
/**
* string content_id = 21 [json_name = "contentId"];
* @return The contentId.
*/
public java.lang.String getContentId() {
java.lang.Object ref = contentId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contentId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string content_id = 21 [json_name = "contentId"];
* @return The bytes for contentId.
*/
public com.google.protobuf.ByteString
getContentIdBytes() {
java.lang.Object ref = contentId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contentId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string content_id = 21 [json_name = "contentId"];
* @param value The contentId to set.
* @return This builder for chaining.
*/
public Builder setContentId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contentId_ = value;
onChanged();
return this;
}
/**
* string content_id = 21 [json_name = "contentId"];
* @return This builder for chaining.
*/
public Builder clearContentId() {
contentId_ = getDefaultInstance().getContentId();
onChanged();
return this;
}
/**
* string content_id = 21 [json_name = "contentId"];
* @param value The bytes for contentId to set.
* @return This builder for chaining.
*/
public Builder setContentIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contentId_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 12 [json_name = "name"];
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 12 [json_name = "name"];
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 12 [json_name = "name"];
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 12 [json_name = "name"];
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 12 [json_name = "name"];
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private int actionType_ = 0;
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @return The enum numeric value on the wire for actionType.
*/
@java.lang.Override public int getActionTypeValue() {
return actionType_;
}
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @param value The enum numeric value on the wire for actionType to set.
* @return This builder for chaining.
*/
public Builder setActionTypeValue(int value) {
actionType_ = value;
onChanged();
return this;
}
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @return The actionType.
*/
@java.lang.Override
public ai.promoted.proto.event.ActionType getActionType() {
@SuppressWarnings("deprecation")
ai.promoted.proto.event.ActionType result = ai.promoted.proto.event.ActionType.valueOf(actionType_);
return result == null ? ai.promoted.proto.event.ActionType.UNRECOGNIZED : result;
}
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @param value The actionType to set.
* @return This builder for chaining.
*/
public Builder setActionType(ai.promoted.proto.event.ActionType value) {
if (value == null) {
throw new NullPointerException();
}
actionType_ = value.getNumber();
onChanged();
return this;
}
/**
* .event.ActionType action_type = 14 [json_name = "actionType"];
* @return This builder for chaining.
*/
public Builder clearActionType() {
actionType_ = 0;
onChanged();
return this;
}
private java.lang.Object customActionType_ = "";
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @return The customActionType.
*/
public java.lang.String getCustomActionType() {
java.lang.Object ref = customActionType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customActionType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @return The bytes for customActionType.
*/
public com.google.protobuf.ByteString
getCustomActionTypeBytes() {
java.lang.Object ref = customActionType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customActionType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @param value The customActionType to set.
* @return This builder for chaining.
*/
public Builder setCustomActionType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
customActionType_ = value;
onChanged();
return this;
}
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @return This builder for chaining.
*/
public Builder clearCustomActionType() {
customActionType_ = getDefaultInstance().getCustomActionType();
onChanged();
return this;
}
/**
* string custom_action_type = 15 [json_name = "customActionType"];
* @param value The bytes for customActionType to set.
* @return This builder for chaining.
*/
public Builder setCustomActionTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
customActionType_ = value;
onChanged();
return this;
}
private java.lang.Object elementId_ = "";
/**
* string element_id = 17 [json_name = "elementId"];
* @return The elementId.
*/
public java.lang.String getElementId() {
java.lang.Object ref = elementId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
elementId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string element_id = 17 [json_name = "elementId"];
* @return The bytes for elementId.
*/
public com.google.protobuf.ByteString
getElementIdBytes() {
java.lang.Object ref = elementId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
elementId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string element_id = 17 [json_name = "elementId"];
* @param value The elementId to set.
* @return This builder for chaining.
*/
public Builder setElementId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
elementId_ = value;
onChanged();
return this;
}
/**
* string element_id = 17 [json_name = "elementId"];
* @return This builder for chaining.
*/
public Builder clearElementId() {
elementId_ = getDefaultInstance().getElementId();
onChanged();
return this;
}
/**
* string element_id = 17 [json_name = "elementId"];
* @param value The bytes for elementId to set.
* @return This builder for chaining.
*/
public Builder setElementIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
elementId_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.NavigateAction, ai.promoted.proto.event.NavigateAction.Builder, ai.promoted.proto.event.NavigateActionOrBuilder> navigateActionBuilder_;
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
* @return Whether the navigateAction field is set.
*/
@java.lang.Override
public boolean hasNavigateAction() {
return actionCase_ == 18;
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
* @return The navigateAction.
*/
@java.lang.Override
public ai.promoted.proto.event.NavigateAction getNavigateAction() {
if (navigateActionBuilder_ == null) {
if (actionCase_ == 18) {
return (ai.promoted.proto.event.NavigateAction) action_;
}
return ai.promoted.proto.event.NavigateAction.getDefaultInstance();
} else {
if (actionCase_ == 18) {
return navigateActionBuilder_.getMessage();
}
return ai.promoted.proto.event.NavigateAction.getDefaultInstance();
}
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
public Builder setNavigateAction(ai.promoted.proto.event.NavigateAction value) {
if (navigateActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
navigateActionBuilder_.setMessage(value);
}
actionCase_ = 18;
return this;
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
public Builder setNavigateAction(
ai.promoted.proto.event.NavigateAction.Builder builderForValue) {
if (navigateActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
navigateActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 18;
return this;
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
public Builder mergeNavigateAction(ai.promoted.proto.event.NavigateAction value) {
if (navigateActionBuilder_ == null) {
if (actionCase_ == 18 &&
action_ != ai.promoted.proto.event.NavigateAction.getDefaultInstance()) {
action_ = ai.promoted.proto.event.NavigateAction.newBuilder((ai.promoted.proto.event.NavigateAction) action_)
.mergeFrom(value).buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 18) {
navigateActionBuilder_.mergeFrom(value);
}
navigateActionBuilder_.setMessage(value);
}
actionCase_ = 18;
return this;
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
public Builder clearNavigateAction() {
if (navigateActionBuilder_ == null) {
if (actionCase_ == 18) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 18) {
actionCase_ = 0;
action_ = null;
}
navigateActionBuilder_.clear();
}
return this;
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
public ai.promoted.proto.event.NavigateAction.Builder getNavigateActionBuilder() {
return getNavigateActionFieldBuilder().getBuilder();
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
@java.lang.Override
public ai.promoted.proto.event.NavigateActionOrBuilder getNavigateActionOrBuilder() {
if ((actionCase_ == 18) && (navigateActionBuilder_ != null)) {
return navigateActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 18) {
return (ai.promoted.proto.event.NavigateAction) action_;
}
return ai.promoted.proto.event.NavigateAction.getDefaultInstance();
}
}
/**
* .event.NavigateAction navigate_action = 18 [json_name = "navigateAction"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.NavigateAction, ai.promoted.proto.event.NavigateAction.Builder, ai.promoted.proto.event.NavigateActionOrBuilder>
getNavigateActionFieldBuilder() {
if (navigateActionBuilder_ == null) {
if (!(actionCase_ == 18)) {
action_ = ai.promoted.proto.event.NavigateAction.getDefaultInstance();
}
navigateActionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.NavigateAction, ai.promoted.proto.event.NavigateAction.Builder, ai.promoted.proto.event.NavigateActionOrBuilder>(
(ai.promoted.proto.event.NavigateAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 18;
onChanged();;
return navigateActionBuilder_;
}
private boolean hasSuperimposedViews_ ;
/**
* bool has_superimposed_views = 22 [json_name = "hasSuperimposedViews"];
* @return The hasSuperimposedViews.
*/
@java.lang.Override
public boolean getHasSuperimposedViews() {
return hasSuperimposedViews_;
}
/**
* bool has_superimposed_views = 22 [json_name = "hasSuperimposedViews"];
* @param value The hasSuperimposedViews to set.
* @return This builder for chaining.
*/
public Builder setHasSuperimposedViews(boolean value) {
hasSuperimposedViews_ = value;
onChanged();
return this;
}
/**
* bool has_superimposed_views = 22 [json_name = "hasSuperimposedViews"];
* @return This builder for chaining.
*/
public Builder clearHasSuperimposedViews() {
hasSuperimposedViews_ = false;
onChanged();
return this;
}
private ai.promoted.proto.event.IndexPath clientPosition_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.IndexPath, ai.promoted.proto.event.IndexPath.Builder, ai.promoted.proto.event.IndexPathOrBuilder> clientPositionBuilder_;
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
* @return Whether the clientPosition field is set.
*/
public boolean hasClientPosition() {
return clientPositionBuilder_ != null || clientPosition_ != null;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
* @return The clientPosition.
*/
public ai.promoted.proto.event.IndexPath getClientPosition() {
if (clientPositionBuilder_ == null) {
return clientPosition_ == null ? ai.promoted.proto.event.IndexPath.getDefaultInstance() : clientPosition_;
} else {
return clientPositionBuilder_.getMessage();
}
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
public Builder setClientPosition(ai.promoted.proto.event.IndexPath value) {
if (clientPositionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientPosition_ = value;
onChanged();
} else {
clientPositionBuilder_.setMessage(value);
}
return this;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
public Builder setClientPosition(
ai.promoted.proto.event.IndexPath.Builder builderForValue) {
if (clientPositionBuilder_ == null) {
clientPosition_ = builderForValue.build();
onChanged();
} else {
clientPositionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
public Builder mergeClientPosition(ai.promoted.proto.event.IndexPath value) {
if (clientPositionBuilder_ == null) {
if (clientPosition_ != null) {
clientPosition_ =
ai.promoted.proto.event.IndexPath.newBuilder(clientPosition_).mergeFrom(value).buildPartial();
} else {
clientPosition_ = value;
}
onChanged();
} else {
clientPositionBuilder_.mergeFrom(value);
}
return this;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
public Builder clearClientPosition() {
if (clientPositionBuilder_ == null) {
clientPosition_ = null;
onChanged();
} else {
clientPosition_ = null;
clientPositionBuilder_ = null;
}
return this;
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
public ai.promoted.proto.event.IndexPath.Builder getClientPositionBuilder() {
onChanged();
return getClientPositionFieldBuilder().getBuilder();
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
public ai.promoted.proto.event.IndexPathOrBuilder getClientPositionOrBuilder() {
if (clientPositionBuilder_ != null) {
return clientPositionBuilder_.getMessageOrBuilder();
} else {
return clientPosition_ == null ?
ai.promoted.proto.event.IndexPath.getDefaultInstance() : clientPosition_;
}
}
/**
* .event.IndexPath client_position = 24 [json_name = "clientPosition"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.IndexPath, ai.promoted.proto.event.IndexPath.Builder, ai.promoted.proto.event.IndexPathOrBuilder>
getClientPositionFieldBuilder() {
if (clientPositionBuilder_ == null) {
clientPositionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.IndexPath, ai.promoted.proto.event.IndexPath.Builder, ai.promoted.proto.event.IndexPathOrBuilder>(
getClientPosition(),
getParentForChildren(),
isClean());
clientPosition_ = null;
}
return clientPositionBuilder_;
}
private ai.promoted.proto.event.IdentifierProvenances idProvenances_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.IdentifierProvenances, ai.promoted.proto.event.IdentifierProvenances.Builder, ai.promoted.proto.event.IdentifierProvenancesOrBuilder> idProvenancesBuilder_;
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
* @return Whether the idProvenances field is set.
*/
public boolean hasIdProvenances() {
return idProvenancesBuilder_ != null || idProvenances_ != null;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
* @return The idProvenances.
*/
public ai.promoted.proto.event.IdentifierProvenances getIdProvenances() {
if (idProvenancesBuilder_ == null) {
return idProvenances_ == null ? ai.promoted.proto.event.IdentifierProvenances.getDefaultInstance() : idProvenances_;
} else {
return idProvenancesBuilder_.getMessage();
}
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
public Builder setIdProvenances(ai.promoted.proto.event.IdentifierProvenances value) {
if (idProvenancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
idProvenances_ = value;
onChanged();
} else {
idProvenancesBuilder_.setMessage(value);
}
return this;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
public Builder setIdProvenances(
ai.promoted.proto.event.IdentifierProvenances.Builder builderForValue) {
if (idProvenancesBuilder_ == null) {
idProvenances_ = builderForValue.build();
onChanged();
} else {
idProvenancesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
public Builder mergeIdProvenances(ai.promoted.proto.event.IdentifierProvenances value) {
if (idProvenancesBuilder_ == null) {
if (idProvenances_ != null) {
idProvenances_ =
ai.promoted.proto.event.IdentifierProvenances.newBuilder(idProvenances_).mergeFrom(value).buildPartial();
} else {
idProvenances_ = value;
}
onChanged();
} else {
idProvenancesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
public Builder clearIdProvenances() {
if (idProvenancesBuilder_ == null) {
idProvenances_ = null;
onChanged();
} else {
idProvenances_ = null;
idProvenancesBuilder_ = null;
}
return this;
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
public ai.promoted.proto.event.IdentifierProvenances.Builder getIdProvenancesBuilder() {
onChanged();
return getIdProvenancesFieldBuilder().getBuilder();
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
public ai.promoted.proto.event.IdentifierProvenancesOrBuilder getIdProvenancesOrBuilder() {
if (idProvenancesBuilder_ != null) {
return idProvenancesBuilder_.getMessageOrBuilder();
} else {
return idProvenances_ == null ?
ai.promoted.proto.event.IdentifierProvenances.getDefaultInstance() : idProvenances_;
}
}
/**
* .event.IdentifierProvenances id_provenances = 25 [json_name = "idProvenances"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.IdentifierProvenances, ai.promoted.proto.event.IdentifierProvenances.Builder, ai.promoted.proto.event.IdentifierProvenancesOrBuilder>
getIdProvenancesFieldBuilder() {
if (idProvenancesBuilder_ == null) {
idProvenancesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.IdentifierProvenances, ai.promoted.proto.event.IdentifierProvenances.Builder, ai.promoted.proto.event.IdentifierProvenancesOrBuilder>(
getIdProvenances(),
getParentForChildren(),
isClean());
idProvenances_ = null;
}
return idProvenancesBuilder_;
}
private ai.promoted.proto.common.Properties properties_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Properties, ai.promoted.proto.common.Properties.Builder, ai.promoted.proto.common.PropertiesOrBuilder> propertiesBuilder_;
/**
* .common.Properties properties = 20 [json_name = "properties"];
* @return Whether the properties field is set.
*/
public boolean hasProperties() {
return propertiesBuilder_ != null || properties_ != null;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
* @return The properties.
*/
public ai.promoted.proto.common.Properties getProperties() {
if (propertiesBuilder_ == null) {
return properties_ == null ? ai.promoted.proto.common.Properties.getDefaultInstance() : properties_;
} else {
return propertiesBuilder_.getMessage();
}
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
public Builder setProperties(ai.promoted.proto.common.Properties value) {
if (propertiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
properties_ = value;
onChanged();
} else {
propertiesBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
public Builder setProperties(
ai.promoted.proto.common.Properties.Builder builderForValue) {
if (propertiesBuilder_ == null) {
properties_ = builderForValue.build();
onChanged();
} else {
propertiesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
public Builder mergeProperties(ai.promoted.proto.common.Properties value) {
if (propertiesBuilder_ == null) {
if (properties_ != null) {
properties_ =
ai.promoted.proto.common.Properties.newBuilder(properties_).mergeFrom(value).buildPartial();
} else {
properties_ = value;
}
onChanged();
} else {
propertiesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
public Builder clearProperties() {
if (propertiesBuilder_ == null) {
properties_ = null;
onChanged();
} else {
properties_ = null;
propertiesBuilder_ = null;
}
return this;
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
public ai.promoted.proto.common.Properties.Builder getPropertiesBuilder() {
onChanged();
return getPropertiesFieldBuilder().getBuilder();
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
public ai.promoted.proto.common.PropertiesOrBuilder getPropertiesOrBuilder() {
if (propertiesBuilder_ != null) {
return propertiesBuilder_.getMessageOrBuilder();
} else {
return properties_ == null ?
ai.promoted.proto.common.Properties.getDefaultInstance() : properties_;
}
}
/**
* .common.Properties properties = 20 [json_name = "properties"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Properties, ai.promoted.proto.common.Properties.Builder, ai.promoted.proto.common.PropertiesOrBuilder>
getPropertiesFieldBuilder() {
if (propertiesBuilder_ == null) {
propertiesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Properties, ai.promoted.proto.common.Properties.Builder, ai.promoted.proto.common.PropertiesOrBuilder>(
getProperties(),
getParentForChildren(),
isClean());
properties_ = null;
}
return propertiesBuilder_;
}
private ai.promoted.proto.common.Device device_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Device, ai.promoted.proto.common.Device.Builder, ai.promoted.proto.common.DeviceOrBuilder> deviceBuilder_;
/**
* .common.Device device = 26 [json_name = "device"];
* @return Whether the device field is set.
*/
public boolean hasDevice() {
return deviceBuilder_ != null || device_ != null;
}
/**
* .common.Device device = 26 [json_name = "device"];
* @return The device.
*/
public ai.promoted.proto.common.Device getDevice() {
if (deviceBuilder_ == null) {
return device_ == null ? ai.promoted.proto.common.Device.getDefaultInstance() : device_;
} else {
return deviceBuilder_.getMessage();
}
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
public Builder setDevice(ai.promoted.proto.common.Device value) {
if (deviceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
device_ = value;
onChanged();
} else {
deviceBuilder_.setMessage(value);
}
return this;
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
public Builder setDevice(
ai.promoted.proto.common.Device.Builder builderForValue) {
if (deviceBuilder_ == null) {
device_ = builderForValue.build();
onChanged();
} else {
deviceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
public Builder mergeDevice(ai.promoted.proto.common.Device value) {
if (deviceBuilder_ == null) {
if (device_ != null) {
device_ =
ai.promoted.proto.common.Device.newBuilder(device_).mergeFrom(value).buildPartial();
} else {
device_ = value;
}
onChanged();
} else {
deviceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
public Builder clearDevice() {
if (deviceBuilder_ == null) {
device_ = null;
onChanged();
} else {
device_ = null;
deviceBuilder_ = null;
}
return this;
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
public ai.promoted.proto.common.Device.Builder getDeviceBuilder() {
onChanged();
return getDeviceFieldBuilder().getBuilder();
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
public ai.promoted.proto.common.DeviceOrBuilder getDeviceOrBuilder() {
if (deviceBuilder_ != null) {
return deviceBuilder_.getMessageOrBuilder();
} else {
return device_ == null ?
ai.promoted.proto.common.Device.getDefaultInstance() : device_;
}
}
/**
* .common.Device device = 26 [json_name = "device"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Device, ai.promoted.proto.common.Device.Builder, ai.promoted.proto.common.DeviceOrBuilder>
getDeviceFieldBuilder() {
if (deviceBuilder_ == null) {
deviceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.common.Device, ai.promoted.proto.common.Device.Builder, ai.promoted.proto.common.DeviceOrBuilder>(
getDevice(),
getParentForChildren(),
isClean());
device_ = null;
}
return deviceBuilder_;
}
private ai.promoted.proto.event.Cart cart_;
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.Cart, ai.promoted.proto.event.Cart.Builder, ai.promoted.proto.event.CartOrBuilder> cartBuilder_;
/**
* .event.Cart cart = 28 [json_name = "cart"];
* @return Whether the cart field is set.
*/
public boolean hasCart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
* @return The cart.
*/
public ai.promoted.proto.event.Cart getCart() {
if (cartBuilder_ == null) {
return cart_ == null ? ai.promoted.proto.event.Cart.getDefaultInstance() : cart_;
} else {
return cartBuilder_.getMessage();
}
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
public Builder setCart(ai.promoted.proto.event.Cart value) {
if (cartBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cart_ = value;
onChanged();
} else {
cartBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
public Builder setCart(
ai.promoted.proto.event.Cart.Builder builderForValue) {
if (cartBuilder_ == null) {
cart_ = builderForValue.build();
onChanged();
} else {
cartBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
public Builder mergeCart(ai.promoted.proto.event.Cart value) {
if (cartBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
cart_ != null &&
cart_ != ai.promoted.proto.event.Cart.getDefaultInstance()) {
cart_ =
ai.promoted.proto.event.Cart.newBuilder(cart_).mergeFrom(value).buildPartial();
} else {
cart_ = value;
}
onChanged();
} else {
cartBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
public Builder clearCart() {
if (cartBuilder_ == null) {
cart_ = null;
onChanged();
} else {
cartBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
public ai.promoted.proto.event.Cart.Builder getCartBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getCartFieldBuilder().getBuilder();
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
public ai.promoted.proto.event.CartOrBuilder getCartOrBuilder() {
if (cartBuilder_ != null) {
return cartBuilder_.getMessageOrBuilder();
} else {
return cart_ == null ?
ai.promoted.proto.event.Cart.getDefaultInstance() : cart_;
}
}
/**
* .event.Cart cart = 28 [json_name = "cart"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.Cart, ai.promoted.proto.event.Cart.Builder, ai.promoted.proto.event.CartOrBuilder>
getCartFieldBuilder() {
if (cartBuilder_ == null) {
cartBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ai.promoted.proto.event.Cart, ai.promoted.proto.event.Cart.Builder, ai.promoted.proto.event.CartOrBuilder>(
getCart(),
getParentForChildren(),
isClean());
cart_ = null;
}
return cartBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:event.Action)
}
// @@protoc_insertion_point(class_scope:event.Action)
private static final ai.promoted.proto.event.Action DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ai.promoted.proto.event.Action();
}
public static ai.promoted.proto.event.Action getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Action parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Action(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ai.promoted.proto.event.Action getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy