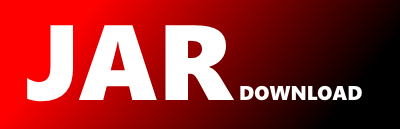
ai.promoted.proto.event.AncestorIdHistory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deliveryclient Show documentation
Show all versions of deliveryclient Show documentation
A Java Client to contact the Promoted.ai Delivery API.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: proto/event/event.proto
package ai.promoted.proto.event;
/**
* Protobuf type {@code event.AncestorIdHistory}
*/
public final class AncestorIdHistory extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:event.AncestorIdHistory)
AncestorIdHistoryOrBuilder {
private static final long serialVersionUID = 0L;
// Use AncestorIdHistory.newBuilder() to construct.
private AncestorIdHistory(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AncestorIdHistory() {
logUserIdHistory_ = java.util.Collections.emptyList();
sessionIdHistory_ = java.util.Collections.emptyList();
viewIdHistory_ = java.util.Collections.emptyList();
autoViewIdHistory_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AncestorIdHistory();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AncestorIdHistory(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
logUserIdHistory_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
logUserIdHistory_.add(
input.readMessage(ai.promoted.proto.event.AncestorIdHistoryItem.parser(), extensionRegistry));
break;
}
case 16: {
totalLogUserIdsLogged_ = input.readInt32();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
sessionIdHistory_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
sessionIdHistory_.add(
input.readMessage(ai.promoted.proto.event.AncestorIdHistoryItem.parser(), extensionRegistry));
break;
}
case 32: {
totalSessionIdsLogged_ = input.readInt32();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
viewIdHistory_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
viewIdHistory_.add(
input.readMessage(ai.promoted.proto.event.AncestorIdHistoryItem.parser(), extensionRegistry));
break;
}
case 48: {
totalViewIdsLogged_ = input.readInt32();
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
autoViewIdHistory_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
autoViewIdHistory_.add(
input.readMessage(ai.promoted.proto.event.AncestorIdHistoryItem.parser(), extensionRegistry));
break;
}
case 64: {
totalAutoViewIdsLogged_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
logUserIdHistory_ = java.util.Collections.unmodifiableList(logUserIdHistory_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
sessionIdHistory_ = java.util.Collections.unmodifiableList(sessionIdHistory_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
viewIdHistory_ = java.util.Collections.unmodifiableList(viewIdHistory_);
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
autoViewIdHistory_ = java.util.Collections.unmodifiableList(autoViewIdHistory_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.event.Event.internal_static_event_AncestorIdHistory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.event.Event.internal_static_event_AncestorIdHistory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.event.AncestorIdHistory.class, ai.promoted.proto.event.AncestorIdHistory.Builder.class);
}
public static final int LOG_USER_ID_HISTORY_FIELD_NUMBER = 1;
private java.util.List logUserIdHistory_;
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
@java.lang.Override
public java.util.List getLogUserIdHistoryList() {
return logUserIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
@java.lang.Override
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getLogUserIdHistoryOrBuilderList() {
return logUserIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
@java.lang.Override
public int getLogUserIdHistoryCount() {
return logUserIdHistory_.size();
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItem getLogUserIdHistory(int index) {
return logUserIdHistory_.get(index);
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getLogUserIdHistoryOrBuilder(
int index) {
return logUserIdHistory_.get(index);
}
public static final int TOTAL_LOG_USER_IDS_LOGGED_FIELD_NUMBER = 2;
private int totalLogUserIdsLogged_;
/**
* int32 total_log_user_ids_logged = 2 [json_name = "totalLogUserIdsLogged"];
* @return The totalLogUserIdsLogged.
*/
@java.lang.Override
public int getTotalLogUserIdsLogged() {
return totalLogUserIdsLogged_;
}
public static final int SESSION_ID_HISTORY_FIELD_NUMBER = 3;
private java.util.List sessionIdHistory_;
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
@java.lang.Override
public java.util.List getSessionIdHistoryList() {
return sessionIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
@java.lang.Override
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getSessionIdHistoryOrBuilderList() {
return sessionIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
@java.lang.Override
public int getSessionIdHistoryCount() {
return sessionIdHistory_.size();
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItem getSessionIdHistory(int index) {
return sessionIdHistory_.get(index);
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getSessionIdHistoryOrBuilder(
int index) {
return sessionIdHistory_.get(index);
}
public static final int TOTAL_SESSION_IDS_LOGGED_FIELD_NUMBER = 4;
private int totalSessionIdsLogged_;
/**
* int32 total_session_ids_logged = 4 [json_name = "totalSessionIdsLogged"];
* @return The totalSessionIdsLogged.
*/
@java.lang.Override
public int getTotalSessionIdsLogged() {
return totalSessionIdsLogged_;
}
public static final int VIEW_ID_HISTORY_FIELD_NUMBER = 5;
private java.util.List viewIdHistory_;
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
@java.lang.Override
public java.util.List getViewIdHistoryList() {
return viewIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
@java.lang.Override
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getViewIdHistoryOrBuilderList() {
return viewIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
@java.lang.Override
public int getViewIdHistoryCount() {
return viewIdHistory_.size();
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItem getViewIdHistory(int index) {
return viewIdHistory_.get(index);
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getViewIdHistoryOrBuilder(
int index) {
return viewIdHistory_.get(index);
}
public static final int TOTAL_VIEW_IDS_LOGGED_FIELD_NUMBER = 6;
private int totalViewIdsLogged_;
/**
* int32 total_view_ids_logged = 6 [json_name = "totalViewIdsLogged"];
* @return The totalViewIdsLogged.
*/
@java.lang.Override
public int getTotalViewIdsLogged() {
return totalViewIdsLogged_;
}
public static final int AUTO_VIEW_ID_HISTORY_FIELD_NUMBER = 7;
private java.util.List autoViewIdHistory_;
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
@java.lang.Override
public java.util.List getAutoViewIdHistoryList() {
return autoViewIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
@java.lang.Override
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getAutoViewIdHistoryOrBuilderList() {
return autoViewIdHistory_;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
@java.lang.Override
public int getAutoViewIdHistoryCount() {
return autoViewIdHistory_.size();
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItem getAutoViewIdHistory(int index) {
return autoViewIdHistory_.get(index);
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getAutoViewIdHistoryOrBuilder(
int index) {
return autoViewIdHistory_.get(index);
}
public static final int TOTAL_AUTO_VIEW_IDS_LOGGED_FIELD_NUMBER = 8;
private int totalAutoViewIdsLogged_;
/**
* int32 total_auto_view_ids_logged = 8 [json_name = "totalAutoViewIdsLogged"];
* @return The totalAutoViewIdsLogged.
*/
@java.lang.Override
public int getTotalAutoViewIdsLogged() {
return totalAutoViewIdsLogged_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < logUserIdHistory_.size(); i++) {
output.writeMessage(1, logUserIdHistory_.get(i));
}
if (totalLogUserIdsLogged_ != 0) {
output.writeInt32(2, totalLogUserIdsLogged_);
}
for (int i = 0; i < sessionIdHistory_.size(); i++) {
output.writeMessage(3, sessionIdHistory_.get(i));
}
if (totalSessionIdsLogged_ != 0) {
output.writeInt32(4, totalSessionIdsLogged_);
}
for (int i = 0; i < viewIdHistory_.size(); i++) {
output.writeMessage(5, viewIdHistory_.get(i));
}
if (totalViewIdsLogged_ != 0) {
output.writeInt32(6, totalViewIdsLogged_);
}
for (int i = 0; i < autoViewIdHistory_.size(); i++) {
output.writeMessage(7, autoViewIdHistory_.get(i));
}
if (totalAutoViewIdsLogged_ != 0) {
output.writeInt32(8, totalAutoViewIdsLogged_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < logUserIdHistory_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, logUserIdHistory_.get(i));
}
if (totalLogUserIdsLogged_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, totalLogUserIdsLogged_);
}
for (int i = 0; i < sessionIdHistory_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, sessionIdHistory_.get(i));
}
if (totalSessionIdsLogged_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, totalSessionIdsLogged_);
}
for (int i = 0; i < viewIdHistory_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, viewIdHistory_.get(i));
}
if (totalViewIdsLogged_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, totalViewIdsLogged_);
}
for (int i = 0; i < autoViewIdHistory_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, autoViewIdHistory_.get(i));
}
if (totalAutoViewIdsLogged_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, totalAutoViewIdsLogged_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ai.promoted.proto.event.AncestorIdHistory)) {
return super.equals(obj);
}
ai.promoted.proto.event.AncestorIdHistory other = (ai.promoted.proto.event.AncestorIdHistory) obj;
if (!getLogUserIdHistoryList()
.equals(other.getLogUserIdHistoryList())) return false;
if (getTotalLogUserIdsLogged()
!= other.getTotalLogUserIdsLogged()) return false;
if (!getSessionIdHistoryList()
.equals(other.getSessionIdHistoryList())) return false;
if (getTotalSessionIdsLogged()
!= other.getTotalSessionIdsLogged()) return false;
if (!getViewIdHistoryList()
.equals(other.getViewIdHistoryList())) return false;
if (getTotalViewIdsLogged()
!= other.getTotalViewIdsLogged()) return false;
if (!getAutoViewIdHistoryList()
.equals(other.getAutoViewIdHistoryList())) return false;
if (getTotalAutoViewIdsLogged()
!= other.getTotalAutoViewIdsLogged()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getLogUserIdHistoryCount() > 0) {
hash = (37 * hash) + LOG_USER_ID_HISTORY_FIELD_NUMBER;
hash = (53 * hash) + getLogUserIdHistoryList().hashCode();
}
hash = (37 * hash) + TOTAL_LOG_USER_IDS_LOGGED_FIELD_NUMBER;
hash = (53 * hash) + getTotalLogUserIdsLogged();
if (getSessionIdHistoryCount() > 0) {
hash = (37 * hash) + SESSION_ID_HISTORY_FIELD_NUMBER;
hash = (53 * hash) + getSessionIdHistoryList().hashCode();
}
hash = (37 * hash) + TOTAL_SESSION_IDS_LOGGED_FIELD_NUMBER;
hash = (53 * hash) + getTotalSessionIdsLogged();
if (getViewIdHistoryCount() > 0) {
hash = (37 * hash) + VIEW_ID_HISTORY_FIELD_NUMBER;
hash = (53 * hash) + getViewIdHistoryList().hashCode();
}
hash = (37 * hash) + TOTAL_VIEW_IDS_LOGGED_FIELD_NUMBER;
hash = (53 * hash) + getTotalViewIdsLogged();
if (getAutoViewIdHistoryCount() > 0) {
hash = (37 * hash) + AUTO_VIEW_ID_HISTORY_FIELD_NUMBER;
hash = (53 * hash) + getAutoViewIdHistoryList().hashCode();
}
hash = (37 * hash) + TOTAL_AUTO_VIEW_IDS_LOGGED_FIELD_NUMBER;
hash = (53 * hash) + getTotalAutoViewIdsLogged();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.event.AncestorIdHistory parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ai.promoted.proto.event.AncestorIdHistory parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ai.promoted.proto.event.AncestorIdHistory parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ai.promoted.proto.event.AncestorIdHistory prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code event.AncestorIdHistory}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:event.AncestorIdHistory)
ai.promoted.proto.event.AncestorIdHistoryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ai.promoted.proto.event.Event.internal_static_event_AncestorIdHistory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ai.promoted.proto.event.Event.internal_static_event_AncestorIdHistory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ai.promoted.proto.event.AncestorIdHistory.class, ai.promoted.proto.event.AncestorIdHistory.Builder.class);
}
// Construct using ai.promoted.proto.event.AncestorIdHistory.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLogUserIdHistoryFieldBuilder();
getSessionIdHistoryFieldBuilder();
getViewIdHistoryFieldBuilder();
getAutoViewIdHistoryFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (logUserIdHistoryBuilder_ == null) {
logUserIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
logUserIdHistoryBuilder_.clear();
}
totalLogUserIdsLogged_ = 0;
if (sessionIdHistoryBuilder_ == null) {
sessionIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
sessionIdHistoryBuilder_.clear();
}
totalSessionIdsLogged_ = 0;
if (viewIdHistoryBuilder_ == null) {
viewIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
viewIdHistoryBuilder_.clear();
}
totalViewIdsLogged_ = 0;
if (autoViewIdHistoryBuilder_ == null) {
autoViewIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
autoViewIdHistoryBuilder_.clear();
}
totalAutoViewIdsLogged_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ai.promoted.proto.event.Event.internal_static_event_AncestorIdHistory_descriptor;
}
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistory getDefaultInstanceForType() {
return ai.promoted.proto.event.AncestorIdHistory.getDefaultInstance();
}
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistory build() {
ai.promoted.proto.event.AncestorIdHistory result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistory buildPartial() {
ai.promoted.proto.event.AncestorIdHistory result = new ai.promoted.proto.event.AncestorIdHistory(this);
int from_bitField0_ = bitField0_;
if (logUserIdHistoryBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
logUserIdHistory_ = java.util.Collections.unmodifiableList(logUserIdHistory_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logUserIdHistory_ = logUserIdHistory_;
} else {
result.logUserIdHistory_ = logUserIdHistoryBuilder_.build();
}
result.totalLogUserIdsLogged_ = totalLogUserIdsLogged_;
if (sessionIdHistoryBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
sessionIdHistory_ = java.util.Collections.unmodifiableList(sessionIdHistory_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.sessionIdHistory_ = sessionIdHistory_;
} else {
result.sessionIdHistory_ = sessionIdHistoryBuilder_.build();
}
result.totalSessionIdsLogged_ = totalSessionIdsLogged_;
if (viewIdHistoryBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
viewIdHistory_ = java.util.Collections.unmodifiableList(viewIdHistory_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.viewIdHistory_ = viewIdHistory_;
} else {
result.viewIdHistory_ = viewIdHistoryBuilder_.build();
}
result.totalViewIdsLogged_ = totalViewIdsLogged_;
if (autoViewIdHistoryBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
autoViewIdHistory_ = java.util.Collections.unmodifiableList(autoViewIdHistory_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.autoViewIdHistory_ = autoViewIdHistory_;
} else {
result.autoViewIdHistory_ = autoViewIdHistoryBuilder_.build();
}
result.totalAutoViewIdsLogged_ = totalAutoViewIdsLogged_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ai.promoted.proto.event.AncestorIdHistory) {
return mergeFrom((ai.promoted.proto.event.AncestorIdHistory)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ai.promoted.proto.event.AncestorIdHistory other) {
if (other == ai.promoted.proto.event.AncestorIdHistory.getDefaultInstance()) return this;
if (logUserIdHistoryBuilder_ == null) {
if (!other.logUserIdHistory_.isEmpty()) {
if (logUserIdHistory_.isEmpty()) {
logUserIdHistory_ = other.logUserIdHistory_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.addAll(other.logUserIdHistory_);
}
onChanged();
}
} else {
if (!other.logUserIdHistory_.isEmpty()) {
if (logUserIdHistoryBuilder_.isEmpty()) {
logUserIdHistoryBuilder_.dispose();
logUserIdHistoryBuilder_ = null;
logUserIdHistory_ = other.logUserIdHistory_;
bitField0_ = (bitField0_ & ~0x00000001);
logUserIdHistoryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogUserIdHistoryFieldBuilder() : null;
} else {
logUserIdHistoryBuilder_.addAllMessages(other.logUserIdHistory_);
}
}
}
if (other.getTotalLogUserIdsLogged() != 0) {
setTotalLogUserIdsLogged(other.getTotalLogUserIdsLogged());
}
if (sessionIdHistoryBuilder_ == null) {
if (!other.sessionIdHistory_.isEmpty()) {
if (sessionIdHistory_.isEmpty()) {
sessionIdHistory_ = other.sessionIdHistory_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.addAll(other.sessionIdHistory_);
}
onChanged();
}
} else {
if (!other.sessionIdHistory_.isEmpty()) {
if (sessionIdHistoryBuilder_.isEmpty()) {
sessionIdHistoryBuilder_.dispose();
sessionIdHistoryBuilder_ = null;
sessionIdHistory_ = other.sessionIdHistory_;
bitField0_ = (bitField0_ & ~0x00000002);
sessionIdHistoryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSessionIdHistoryFieldBuilder() : null;
} else {
sessionIdHistoryBuilder_.addAllMessages(other.sessionIdHistory_);
}
}
}
if (other.getTotalSessionIdsLogged() != 0) {
setTotalSessionIdsLogged(other.getTotalSessionIdsLogged());
}
if (viewIdHistoryBuilder_ == null) {
if (!other.viewIdHistory_.isEmpty()) {
if (viewIdHistory_.isEmpty()) {
viewIdHistory_ = other.viewIdHistory_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureViewIdHistoryIsMutable();
viewIdHistory_.addAll(other.viewIdHistory_);
}
onChanged();
}
} else {
if (!other.viewIdHistory_.isEmpty()) {
if (viewIdHistoryBuilder_.isEmpty()) {
viewIdHistoryBuilder_.dispose();
viewIdHistoryBuilder_ = null;
viewIdHistory_ = other.viewIdHistory_;
bitField0_ = (bitField0_ & ~0x00000004);
viewIdHistoryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getViewIdHistoryFieldBuilder() : null;
} else {
viewIdHistoryBuilder_.addAllMessages(other.viewIdHistory_);
}
}
}
if (other.getTotalViewIdsLogged() != 0) {
setTotalViewIdsLogged(other.getTotalViewIdsLogged());
}
if (autoViewIdHistoryBuilder_ == null) {
if (!other.autoViewIdHistory_.isEmpty()) {
if (autoViewIdHistory_.isEmpty()) {
autoViewIdHistory_ = other.autoViewIdHistory_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.addAll(other.autoViewIdHistory_);
}
onChanged();
}
} else {
if (!other.autoViewIdHistory_.isEmpty()) {
if (autoViewIdHistoryBuilder_.isEmpty()) {
autoViewIdHistoryBuilder_.dispose();
autoViewIdHistoryBuilder_ = null;
autoViewIdHistory_ = other.autoViewIdHistory_;
bitField0_ = (bitField0_ & ~0x00000008);
autoViewIdHistoryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAutoViewIdHistoryFieldBuilder() : null;
} else {
autoViewIdHistoryBuilder_.addAllMessages(other.autoViewIdHistory_);
}
}
}
if (other.getTotalAutoViewIdsLogged() != 0) {
setTotalAutoViewIdsLogged(other.getTotalAutoViewIdsLogged());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ai.promoted.proto.event.AncestorIdHistory parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ai.promoted.proto.event.AncestorIdHistory) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List logUserIdHistory_ =
java.util.Collections.emptyList();
private void ensureLogUserIdHistoryIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
logUserIdHistory_ = new java.util.ArrayList(logUserIdHistory_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder> logUserIdHistoryBuilder_;
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public java.util.List getLogUserIdHistoryList() {
if (logUserIdHistoryBuilder_ == null) {
return java.util.Collections.unmodifiableList(logUserIdHistory_);
} else {
return logUserIdHistoryBuilder_.getMessageList();
}
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public int getLogUserIdHistoryCount() {
if (logUserIdHistoryBuilder_ == null) {
return logUserIdHistory_.size();
} else {
return logUserIdHistoryBuilder_.getCount();
}
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem getLogUserIdHistory(int index) {
if (logUserIdHistoryBuilder_ == null) {
return logUserIdHistory_.get(index);
} else {
return logUserIdHistoryBuilder_.getMessage(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder setLogUserIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (logUserIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.set(index, value);
onChanged();
} else {
logUserIdHistoryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder setLogUserIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (logUserIdHistoryBuilder_ == null) {
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.set(index, builderForValue.build());
onChanged();
} else {
logUserIdHistoryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder addLogUserIdHistory(ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (logUserIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.add(value);
onChanged();
} else {
logUserIdHistoryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder addLogUserIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (logUserIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.add(index, value);
onChanged();
} else {
logUserIdHistoryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder addLogUserIdHistory(
ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (logUserIdHistoryBuilder_ == null) {
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.add(builderForValue.build());
onChanged();
} else {
logUserIdHistoryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder addLogUserIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (logUserIdHistoryBuilder_ == null) {
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.add(index, builderForValue.build());
onChanged();
} else {
logUserIdHistoryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder addAllLogUserIdHistory(
java.lang.Iterable extends ai.promoted.proto.event.AncestorIdHistoryItem> values) {
if (logUserIdHistoryBuilder_ == null) {
ensureLogUserIdHistoryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logUserIdHistory_);
onChanged();
} else {
logUserIdHistoryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder clearLogUserIdHistory() {
if (logUserIdHistoryBuilder_ == null) {
logUserIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
logUserIdHistoryBuilder_.clear();
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public Builder removeLogUserIdHistory(int index) {
if (logUserIdHistoryBuilder_ == null) {
ensureLogUserIdHistoryIsMutable();
logUserIdHistory_.remove(index);
onChanged();
} else {
logUserIdHistoryBuilder_.remove(index);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder getLogUserIdHistoryBuilder(
int index) {
return getLogUserIdHistoryFieldBuilder().getBuilder(index);
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getLogUserIdHistoryOrBuilder(
int index) {
if (logUserIdHistoryBuilder_ == null) {
return logUserIdHistory_.get(index); } else {
return logUserIdHistoryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getLogUserIdHistoryOrBuilderList() {
if (logUserIdHistoryBuilder_ != null) {
return logUserIdHistoryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logUserIdHistory_);
}
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addLogUserIdHistoryBuilder() {
return getLogUserIdHistoryFieldBuilder().addBuilder(
ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addLogUserIdHistoryBuilder(
int index) {
return getLogUserIdHistoryFieldBuilder().addBuilder(
index, ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem log_user_id_history = 1 [json_name = "logUserIdHistory"];
*/
public java.util.List
getLogUserIdHistoryBuilderList() {
return getLogUserIdHistoryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getLogUserIdHistoryFieldBuilder() {
if (logUserIdHistoryBuilder_ == null) {
logUserIdHistoryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>(
logUserIdHistory_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
logUserIdHistory_ = null;
}
return logUserIdHistoryBuilder_;
}
private int totalLogUserIdsLogged_ ;
/**
* int32 total_log_user_ids_logged = 2 [json_name = "totalLogUserIdsLogged"];
* @return The totalLogUserIdsLogged.
*/
@java.lang.Override
public int getTotalLogUserIdsLogged() {
return totalLogUserIdsLogged_;
}
/**
* int32 total_log_user_ids_logged = 2 [json_name = "totalLogUserIdsLogged"];
* @param value The totalLogUserIdsLogged to set.
* @return This builder for chaining.
*/
public Builder setTotalLogUserIdsLogged(int value) {
totalLogUserIdsLogged_ = value;
onChanged();
return this;
}
/**
* int32 total_log_user_ids_logged = 2 [json_name = "totalLogUserIdsLogged"];
* @return This builder for chaining.
*/
public Builder clearTotalLogUserIdsLogged() {
totalLogUserIdsLogged_ = 0;
onChanged();
return this;
}
private java.util.List sessionIdHistory_ =
java.util.Collections.emptyList();
private void ensureSessionIdHistoryIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
sessionIdHistory_ = new java.util.ArrayList(sessionIdHistory_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder> sessionIdHistoryBuilder_;
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public java.util.List getSessionIdHistoryList() {
if (sessionIdHistoryBuilder_ == null) {
return java.util.Collections.unmodifiableList(sessionIdHistory_);
} else {
return sessionIdHistoryBuilder_.getMessageList();
}
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public int getSessionIdHistoryCount() {
if (sessionIdHistoryBuilder_ == null) {
return sessionIdHistory_.size();
} else {
return sessionIdHistoryBuilder_.getCount();
}
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem getSessionIdHistory(int index) {
if (sessionIdHistoryBuilder_ == null) {
return sessionIdHistory_.get(index);
} else {
return sessionIdHistoryBuilder_.getMessage(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder setSessionIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (sessionIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.set(index, value);
onChanged();
} else {
sessionIdHistoryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder setSessionIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (sessionIdHistoryBuilder_ == null) {
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.set(index, builderForValue.build());
onChanged();
} else {
sessionIdHistoryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder addSessionIdHistory(ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (sessionIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.add(value);
onChanged();
} else {
sessionIdHistoryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder addSessionIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (sessionIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.add(index, value);
onChanged();
} else {
sessionIdHistoryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder addSessionIdHistory(
ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (sessionIdHistoryBuilder_ == null) {
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.add(builderForValue.build());
onChanged();
} else {
sessionIdHistoryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder addSessionIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (sessionIdHistoryBuilder_ == null) {
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.add(index, builderForValue.build());
onChanged();
} else {
sessionIdHistoryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder addAllSessionIdHistory(
java.lang.Iterable extends ai.promoted.proto.event.AncestorIdHistoryItem> values) {
if (sessionIdHistoryBuilder_ == null) {
ensureSessionIdHistoryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sessionIdHistory_);
onChanged();
} else {
sessionIdHistoryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder clearSessionIdHistory() {
if (sessionIdHistoryBuilder_ == null) {
sessionIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
sessionIdHistoryBuilder_.clear();
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public Builder removeSessionIdHistory(int index) {
if (sessionIdHistoryBuilder_ == null) {
ensureSessionIdHistoryIsMutable();
sessionIdHistory_.remove(index);
onChanged();
} else {
sessionIdHistoryBuilder_.remove(index);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder getSessionIdHistoryBuilder(
int index) {
return getSessionIdHistoryFieldBuilder().getBuilder(index);
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getSessionIdHistoryOrBuilder(
int index) {
if (sessionIdHistoryBuilder_ == null) {
return sessionIdHistory_.get(index); } else {
return sessionIdHistoryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getSessionIdHistoryOrBuilderList() {
if (sessionIdHistoryBuilder_ != null) {
return sessionIdHistoryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sessionIdHistory_);
}
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addSessionIdHistoryBuilder() {
return getSessionIdHistoryFieldBuilder().addBuilder(
ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addSessionIdHistoryBuilder(
int index) {
return getSessionIdHistoryFieldBuilder().addBuilder(
index, ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem session_id_history = 3 [json_name = "sessionIdHistory"];
*/
public java.util.List
getSessionIdHistoryBuilderList() {
return getSessionIdHistoryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getSessionIdHistoryFieldBuilder() {
if (sessionIdHistoryBuilder_ == null) {
sessionIdHistoryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>(
sessionIdHistory_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
sessionIdHistory_ = null;
}
return sessionIdHistoryBuilder_;
}
private int totalSessionIdsLogged_ ;
/**
* int32 total_session_ids_logged = 4 [json_name = "totalSessionIdsLogged"];
* @return The totalSessionIdsLogged.
*/
@java.lang.Override
public int getTotalSessionIdsLogged() {
return totalSessionIdsLogged_;
}
/**
* int32 total_session_ids_logged = 4 [json_name = "totalSessionIdsLogged"];
* @param value The totalSessionIdsLogged to set.
* @return This builder for chaining.
*/
public Builder setTotalSessionIdsLogged(int value) {
totalSessionIdsLogged_ = value;
onChanged();
return this;
}
/**
* int32 total_session_ids_logged = 4 [json_name = "totalSessionIdsLogged"];
* @return This builder for chaining.
*/
public Builder clearTotalSessionIdsLogged() {
totalSessionIdsLogged_ = 0;
onChanged();
return this;
}
private java.util.List viewIdHistory_ =
java.util.Collections.emptyList();
private void ensureViewIdHistoryIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
viewIdHistory_ = new java.util.ArrayList(viewIdHistory_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder> viewIdHistoryBuilder_;
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public java.util.List getViewIdHistoryList() {
if (viewIdHistoryBuilder_ == null) {
return java.util.Collections.unmodifiableList(viewIdHistory_);
} else {
return viewIdHistoryBuilder_.getMessageList();
}
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public int getViewIdHistoryCount() {
if (viewIdHistoryBuilder_ == null) {
return viewIdHistory_.size();
} else {
return viewIdHistoryBuilder_.getCount();
}
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem getViewIdHistory(int index) {
if (viewIdHistoryBuilder_ == null) {
return viewIdHistory_.get(index);
} else {
return viewIdHistoryBuilder_.getMessage(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder setViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (viewIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureViewIdHistoryIsMutable();
viewIdHistory_.set(index, value);
onChanged();
} else {
viewIdHistoryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder setViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (viewIdHistoryBuilder_ == null) {
ensureViewIdHistoryIsMutable();
viewIdHistory_.set(index, builderForValue.build());
onChanged();
} else {
viewIdHistoryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder addViewIdHistory(ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (viewIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureViewIdHistoryIsMutable();
viewIdHistory_.add(value);
onChanged();
} else {
viewIdHistoryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder addViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (viewIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureViewIdHistoryIsMutable();
viewIdHistory_.add(index, value);
onChanged();
} else {
viewIdHistoryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder addViewIdHistory(
ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (viewIdHistoryBuilder_ == null) {
ensureViewIdHistoryIsMutable();
viewIdHistory_.add(builderForValue.build());
onChanged();
} else {
viewIdHistoryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder addViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (viewIdHistoryBuilder_ == null) {
ensureViewIdHistoryIsMutable();
viewIdHistory_.add(index, builderForValue.build());
onChanged();
} else {
viewIdHistoryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder addAllViewIdHistory(
java.lang.Iterable extends ai.promoted.proto.event.AncestorIdHistoryItem> values) {
if (viewIdHistoryBuilder_ == null) {
ensureViewIdHistoryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, viewIdHistory_);
onChanged();
} else {
viewIdHistoryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder clearViewIdHistory() {
if (viewIdHistoryBuilder_ == null) {
viewIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
viewIdHistoryBuilder_.clear();
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public Builder removeViewIdHistory(int index) {
if (viewIdHistoryBuilder_ == null) {
ensureViewIdHistoryIsMutable();
viewIdHistory_.remove(index);
onChanged();
} else {
viewIdHistoryBuilder_.remove(index);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder getViewIdHistoryBuilder(
int index) {
return getViewIdHistoryFieldBuilder().getBuilder(index);
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getViewIdHistoryOrBuilder(
int index) {
if (viewIdHistoryBuilder_ == null) {
return viewIdHistory_.get(index); } else {
return viewIdHistoryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getViewIdHistoryOrBuilderList() {
if (viewIdHistoryBuilder_ != null) {
return viewIdHistoryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(viewIdHistory_);
}
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addViewIdHistoryBuilder() {
return getViewIdHistoryFieldBuilder().addBuilder(
ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addViewIdHistoryBuilder(
int index) {
return getViewIdHistoryFieldBuilder().addBuilder(
index, ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem view_id_history = 5 [json_name = "viewIdHistory"];
*/
public java.util.List
getViewIdHistoryBuilderList() {
return getViewIdHistoryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getViewIdHistoryFieldBuilder() {
if (viewIdHistoryBuilder_ == null) {
viewIdHistoryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>(
viewIdHistory_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
viewIdHistory_ = null;
}
return viewIdHistoryBuilder_;
}
private int totalViewIdsLogged_ ;
/**
* int32 total_view_ids_logged = 6 [json_name = "totalViewIdsLogged"];
* @return The totalViewIdsLogged.
*/
@java.lang.Override
public int getTotalViewIdsLogged() {
return totalViewIdsLogged_;
}
/**
* int32 total_view_ids_logged = 6 [json_name = "totalViewIdsLogged"];
* @param value The totalViewIdsLogged to set.
* @return This builder for chaining.
*/
public Builder setTotalViewIdsLogged(int value) {
totalViewIdsLogged_ = value;
onChanged();
return this;
}
/**
* int32 total_view_ids_logged = 6 [json_name = "totalViewIdsLogged"];
* @return This builder for chaining.
*/
public Builder clearTotalViewIdsLogged() {
totalViewIdsLogged_ = 0;
onChanged();
return this;
}
private java.util.List autoViewIdHistory_ =
java.util.Collections.emptyList();
private void ensureAutoViewIdHistoryIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
autoViewIdHistory_ = new java.util.ArrayList(autoViewIdHistory_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder> autoViewIdHistoryBuilder_;
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public java.util.List getAutoViewIdHistoryList() {
if (autoViewIdHistoryBuilder_ == null) {
return java.util.Collections.unmodifiableList(autoViewIdHistory_);
} else {
return autoViewIdHistoryBuilder_.getMessageList();
}
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public int getAutoViewIdHistoryCount() {
if (autoViewIdHistoryBuilder_ == null) {
return autoViewIdHistory_.size();
} else {
return autoViewIdHistoryBuilder_.getCount();
}
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem getAutoViewIdHistory(int index) {
if (autoViewIdHistoryBuilder_ == null) {
return autoViewIdHistory_.get(index);
} else {
return autoViewIdHistoryBuilder_.getMessage(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder setAutoViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (autoViewIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.set(index, value);
onChanged();
} else {
autoViewIdHistoryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder setAutoViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (autoViewIdHistoryBuilder_ == null) {
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.set(index, builderForValue.build());
onChanged();
} else {
autoViewIdHistoryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder addAutoViewIdHistory(ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (autoViewIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.add(value);
onChanged();
} else {
autoViewIdHistoryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder addAutoViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem value) {
if (autoViewIdHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.add(index, value);
onChanged();
} else {
autoViewIdHistoryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder addAutoViewIdHistory(
ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (autoViewIdHistoryBuilder_ == null) {
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.add(builderForValue.build());
onChanged();
} else {
autoViewIdHistoryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder addAutoViewIdHistory(
int index, ai.promoted.proto.event.AncestorIdHistoryItem.Builder builderForValue) {
if (autoViewIdHistoryBuilder_ == null) {
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.add(index, builderForValue.build());
onChanged();
} else {
autoViewIdHistoryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder addAllAutoViewIdHistory(
java.lang.Iterable extends ai.promoted.proto.event.AncestorIdHistoryItem> values) {
if (autoViewIdHistoryBuilder_ == null) {
ensureAutoViewIdHistoryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, autoViewIdHistory_);
onChanged();
} else {
autoViewIdHistoryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder clearAutoViewIdHistory() {
if (autoViewIdHistoryBuilder_ == null) {
autoViewIdHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
autoViewIdHistoryBuilder_.clear();
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public Builder removeAutoViewIdHistory(int index) {
if (autoViewIdHistoryBuilder_ == null) {
ensureAutoViewIdHistoryIsMutable();
autoViewIdHistory_.remove(index);
onChanged();
} else {
autoViewIdHistoryBuilder_.remove(index);
}
return this;
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder getAutoViewIdHistoryBuilder(
int index) {
return getAutoViewIdHistoryFieldBuilder().getBuilder(index);
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder getAutoViewIdHistoryOrBuilder(
int index) {
if (autoViewIdHistoryBuilder_ == null) {
return autoViewIdHistory_.get(index); } else {
return autoViewIdHistoryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public java.util.List extends ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getAutoViewIdHistoryOrBuilderList() {
if (autoViewIdHistoryBuilder_ != null) {
return autoViewIdHistoryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(autoViewIdHistory_);
}
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addAutoViewIdHistoryBuilder() {
return getAutoViewIdHistoryFieldBuilder().addBuilder(
ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public ai.promoted.proto.event.AncestorIdHistoryItem.Builder addAutoViewIdHistoryBuilder(
int index) {
return getAutoViewIdHistoryFieldBuilder().addBuilder(
index, ai.promoted.proto.event.AncestorIdHistoryItem.getDefaultInstance());
}
/**
* repeated .event.AncestorIdHistoryItem auto_view_id_history = 7 [json_name = "autoViewIdHistory"];
*/
public java.util.List
getAutoViewIdHistoryBuilderList() {
return getAutoViewIdHistoryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>
getAutoViewIdHistoryFieldBuilder() {
if (autoViewIdHistoryBuilder_ == null) {
autoViewIdHistoryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ai.promoted.proto.event.AncestorIdHistoryItem, ai.promoted.proto.event.AncestorIdHistoryItem.Builder, ai.promoted.proto.event.AncestorIdHistoryItemOrBuilder>(
autoViewIdHistory_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
autoViewIdHistory_ = null;
}
return autoViewIdHistoryBuilder_;
}
private int totalAutoViewIdsLogged_ ;
/**
* int32 total_auto_view_ids_logged = 8 [json_name = "totalAutoViewIdsLogged"];
* @return The totalAutoViewIdsLogged.
*/
@java.lang.Override
public int getTotalAutoViewIdsLogged() {
return totalAutoViewIdsLogged_;
}
/**
* int32 total_auto_view_ids_logged = 8 [json_name = "totalAutoViewIdsLogged"];
* @param value The totalAutoViewIdsLogged to set.
* @return This builder for chaining.
*/
public Builder setTotalAutoViewIdsLogged(int value) {
totalAutoViewIdsLogged_ = value;
onChanged();
return this;
}
/**
* int32 total_auto_view_ids_logged = 8 [json_name = "totalAutoViewIdsLogged"];
* @return This builder for chaining.
*/
public Builder clearTotalAutoViewIdsLogged() {
totalAutoViewIdsLogged_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:event.AncestorIdHistory)
}
// @@protoc_insertion_point(class_scope:event.AncestorIdHistory)
private static final ai.promoted.proto.event.AncestorIdHistory DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ai.promoted.proto.event.AncestorIdHistory();
}
public static ai.promoted.proto.event.AncestorIdHistory getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AncestorIdHistory parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AncestorIdHistory(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ai.promoted.proto.event.AncestorIdHistory getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy