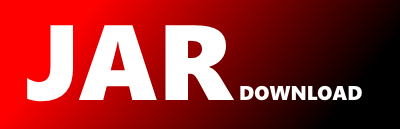
swim.concurrent.Conts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-concurrent Show documentation
Show all versions of swim-concurrent Show documentation
Uploads all artifacts belonging to configuration ':swim-concurrent:archives'
// Copyright 2015-2019 SWIM.AI inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.concurrent;
/**
* Factory functions for {@link Cont}inuation combinators.
*/
public final class Conts {
private Conts() {
}
private static Cont
© 2015 - 2025 Weber Informatics LLC | Privacy Policy