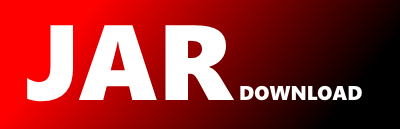
swim.runtime.lane.DemandLaneView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-runtime Show documentation
Show all versions of swim-runtime Show documentation
Uploads all artifacts belonging to configuration ':swim-runtime:archives'
// Copyright 2015-2019 SWIM.AI inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.runtime.lane;
import swim.api.Link;
import swim.api.SwimContext;
import swim.api.agent.AgentContext;
import swim.api.http.function.DecodeRequestHttp;
import swim.api.http.function.DidRequestHttp;
import swim.api.http.function.DidRespondHttp;
import swim.api.http.function.DoRespondHttp;
import swim.api.http.function.WillRequestHttp;
import swim.api.http.function.WillRespondHttp;
import swim.api.lane.DemandLane;
import swim.api.lane.Lane;
import swim.api.lane.function.DidCommand;
import swim.api.lane.function.DidEnter;
import swim.api.lane.function.DidLeave;
import swim.api.lane.function.DidUplink;
import swim.api.lane.function.OnCue;
import swim.api.lane.function.WillCommand;
import swim.api.lane.function.WillEnter;
import swim.api.lane.function.WillLeave;
import swim.api.lane.function.WillUplink;
import swim.api.uplink.Uplink;
import swim.concurrent.Conts;
import swim.structure.Form;
import swim.structure.Value;
public class DemandLaneView extends LaneView implements DemandLane {
protected final AgentContext agentContext;
protected Form valueForm;
protected DemandLaneModel laneBinding;
protected volatile OnCue onCue;
public DemandLaneView(AgentContext agentContext, Form valueForm, Object observers) {
super(observers);
this.agentContext = agentContext;
this.valueForm = valueForm;
this.onCue = onCue;
}
public DemandLaneView(AgentContext agentContext, Form valueForm) {
this(agentContext, valueForm, null);
}
@Override
public AgentContext agentContext() {
return this.agentContext;
}
@Override
public DemandLaneModel getLaneBinding() {
return this.laneBinding;
}
void setLaneBinding(DemandLaneModel laneBinding) {
this.laneBinding = laneBinding;
}
@Override
public DemandLaneModel createLaneBinding() {
return new DemandLaneModel();
}
@Override
public final Form valueForm() {
return this.valueForm;
}
@Override
public DemandLaneView valueForm(Form valueForm) {
return new DemandLaneView(this.agentContext, valueForm, typesafeObservers(this.observers));
}
@Override
public DemandLaneView valueClass(Class valueClass) {
return valueForm(Form.forClass(valueClass));
}
public void setValueForm(Form valueForm) {
this.valueForm = valueForm;
}
protected Object typesafeObservers(Object observers) {
// TODO: filter out OnCue
return observers;
}
@Override
public final boolean isSigned() {
return false; // TODO
}
@Override
public DemandLaneView isSigned(boolean isSigned) {
return this; // TODO
}
@Override
public void close() {
this.laneBinding.closeLaneView(this);
}
@SuppressWarnings("unchecked")
@Override
public DemandLaneView observe(Object observer) {
return (DemandLaneView) super.observe(observer);
}
@SuppressWarnings("unchecked")
@Override
public DemandLaneView unobserve(Object observer) {
return (DemandLaneView) super.unobserve(observer);
}
@Override
public DemandLaneView onCue(OnCue onCue) {
return observe(onCue);
}
@Override
public DemandLaneView willCommand(WillCommand willCommand) {
return observe(willCommand);
}
@Override
public DemandLaneView didCommand(DidCommand didCommand) {
return observe(didCommand);
}
@Override
public DemandLaneView willUplink(WillUplink willUplink) {
return observe(willUplink);
}
@Override
public DemandLaneView didUplink(DidUplink didUplink) {
return observe(didUplink);
}
@Override
public DemandLaneView willEnter(WillEnter willEnter) {
return observe(willEnter);
}
@Override
public DemandLaneView didEnter(DidEnter didEnter) {
return observe(didEnter);
}
@Override
public DemandLaneView willLeave(WillLeave willLeave) {
return observe(willLeave);
}
@Override
public DemandLaneView didLeave(DidLeave didLeave) {
return observe(didLeave);
}
@Override
public DemandLaneView decodeRequest(DecodeRequestHttp
© 2015 - 2025 Weber Informatics LLC | Privacy Policy