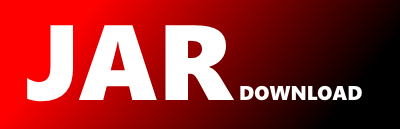
swim.uri.UriSchemeMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-uri Show documentation
Show all versions of swim-uri Show documentation
Uploads all artifacts belonging to configuration ':swim-uri:archives'
// Copyright 2015-2019 SWIM.AI inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.uri;
import java.util.Iterator;
import java.util.Map;
import swim.collections.HashTrieMap;
import swim.util.Murmur3;
final class UriSchemeMapping extends UriSchemeMapper {
final HashTrieMap> table;
UriSchemeMapping(HashTrieMap> table) {
this.table = table;
}
@Override
public boolean isEmpty() {
return this.table.isEmpty();
}
@Override
public int size() {
int size = 0;
final Iterator> routes = this.table.valueIterator();
while (routes.hasNext()) {
size += routes.next().size();
}
return size;
}
@Override
public boolean containsValue(Object value) {
final Iterator> routes = this.table.valueIterator();
while (routes.hasNext()) {
if (routes.next().containsValue(value)) {
return true;
}
}
return false;
}
@Override
T get(UriScheme scheme, UriAuthority authority, UriPath path, UriQuery query, UriFragment fragment) {
final UriAuthorityMapper mapping = this.table.get(scheme.name());
if (mapping != null) {
return mapping.get(authority, path, query, fragment);
} else {
return null;
}
}
UriSchemeMapping merged(UriSchemeMapping that) {
HashTrieMap> table = this.table;
final Iterator>> routes = that.table.iterator();
while (routes.hasNext()) {
final Map.Entry> route = routes.next();
final String schemeName = route.getKey();
UriAuthorityMapper mapping = this.table.get(schemeName);
if (mapping != null) {
mapping = mapping.merged(route.getValue());
} else {
mapping = route.getValue();
}
table = table.updated(schemeName, mapping);
}
return new UriSchemeMapping(table);
}
@Override
UriSchemeMapper merged(UriSchemeMapper that) {
if (that instanceof UriSchemeMapping>) {
return merged((UriSchemeMapping) that);
} else {
return that;
}
}
@SuppressWarnings("unchecked")
@Override
UriSchemeMapper removed(UriScheme scheme, UriAuthority authority, UriPath path, UriQuery query, UriFragment fragment) {
final String schemeName = scheme.name();
final UriAuthorityMapper oldMapping = this.table.get(schemeName);
if (oldMapping != null) {
final UriAuthorityMapper newMapping = oldMapping.removed(authority, path, query, fragment);
if (oldMapping != newMapping) {
if (!oldMapping.isEmpty()) {
return new UriSchemeMapping(this.table.updated(schemeName, newMapping));
} else {
return (UriSchemeMapper) empty();
}
}
}
return this;
}
@SuppressWarnings("unchecked")
UriSchemeMapper unmerged(UriSchemeMapping that) {
HashTrieMap> table = this.table;
final Iterator>> routes = that.table.iterator();
while (routes.hasNext()) {
final Map.Entry> route = routes.next();
final String schemeName = route.getKey();
UriAuthorityMapper mapping = table.get(schemeName);
if (mapping != null) {
mapping = mapping.unmerged(route.getValue());
if (!mapping.isEmpty()) {
table = table.updated(schemeName, mapping);
} else {
table = table.removed(schemeName);
}
}
}
if (!table.isEmpty()) {
return new UriSchemeMapping(table);
} else {
return (UriSchemeMapper) empty();
}
}
@Override
UriSchemeMapper unmerged(UriSchemeMapper that) {
if (that instanceof UriSchemeMapping>) {
return unmerged((UriSchemeMapping) that);
} else {
return this;
}
}
@Override
public Iterator> iterator() {
return new UriSchemeMappingIterator(this.table.valueIterator());
}
@Override
public Iterator keyIterator() {
return new UriSchemeMappingKeyIterator(this.table.valueIterator());
}
@Override
public Iterator valueIterator() {
return new UriSchemeMappingValueIterator(this.table.valueIterator());
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
} else if (other instanceof UriSchemeMapping>) {
final UriSchemeMapping> that = (UriSchemeMapping>) other;
return this.table.equals(that.table);
}
return false;
}
@Override
public int hashCode() {
if (hashSeed == 0) {
hashSeed = Murmur3.seed(UriSchemeMapping.class);
}
return Murmur3.mash(Murmur3.mix(hashSeed, this.table.hashCode()));
}
private static int hashSeed;
}
final class UriSchemeMappingIterator extends FlatteningIterator, Map.Entry> {
UriSchemeMappingIterator(Iterator> outer) {
super(outer);
}
@Override
protected Iterator> childIterator(UriAuthorityMapper parent) {
return parent.iterator();
}
}
final class UriSchemeMappingKeyIterator extends FlatteningIterator, Uri> {
UriSchemeMappingKeyIterator(Iterator> outer) {
super(outer);
}
@Override
protected Iterator childIterator(UriAuthorityMapper parent) {
return parent.keyIterator();
}
}
final class UriSchemeMappingValueIterator extends FlatteningIterator, T> {
UriSchemeMappingValueIterator(Iterator> outer) {
super(outer);
}
@Override
protected Iterator childIterator(UriAuthorityMapper parent) {
return parent.valueIterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy