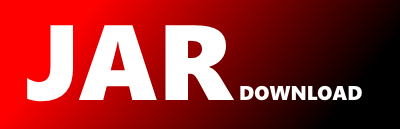
ai.timefold.solver.benchmark.config.ProblemBenchmarksConfig Maven / Gradle / Ivy
package ai.timefold.solver.benchmark.config;
import java.io.File;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.function.Consumer;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import ai.timefold.solver.benchmark.config.statistic.ProblemStatisticType;
import ai.timefold.solver.benchmark.config.statistic.SingleStatisticType;
import ai.timefold.solver.core.config.AbstractConfig;
import ai.timefold.solver.core.config.util.ConfigUtils;
import ai.timefold.solver.persistence.common.api.domain.solution.SolutionFileIO;
@XmlType(propOrder = {
"solutionFileIOClass",
"writeOutputSolutionEnabled",
"inputSolutionFileList",
"problemStatisticEnabled",
"problemStatisticTypeList",
"singleStatisticTypeList"
})
public class ProblemBenchmarksConfig extends AbstractConfig {
private Class extends SolutionFileIO>> solutionFileIOClass = null;
private Boolean writeOutputSolutionEnabled = null;
@XmlElement(name = "inputSolutionFile")
private List inputSolutionFileList = null;
private Boolean problemStatisticEnabled = null;
@XmlElement(name = "problemStatisticType")
private List problemStatisticTypeList = null;
@XmlElement(name = "singleStatisticType")
private List singleStatisticTypeList = null;
// ************************************************************************
// Constructors and simple getters/setters
// ************************************************************************
public Class extends SolutionFileIO>> getSolutionFileIOClass() {
return solutionFileIOClass;
}
public void setSolutionFileIOClass(Class extends SolutionFileIO>> solutionFileIOClass) {
this.solutionFileIOClass = solutionFileIOClass;
}
public Boolean getWriteOutputSolutionEnabled() {
return writeOutputSolutionEnabled;
}
public void setWriteOutputSolutionEnabled(Boolean writeOutputSolutionEnabled) {
this.writeOutputSolutionEnabled = writeOutputSolutionEnabled;
}
public List getInputSolutionFileList() {
return inputSolutionFileList;
}
public void setInputSolutionFileList(List inputSolutionFileList) {
this.inputSolutionFileList = inputSolutionFileList;
}
public Boolean getProblemStatisticEnabled() {
return problemStatisticEnabled;
}
public void setProblemStatisticEnabled(Boolean problemStatisticEnabled) {
this.problemStatisticEnabled = problemStatisticEnabled;
}
public List getProblemStatisticTypeList() {
return problemStatisticTypeList;
}
public void setProblemStatisticTypeList(List problemStatisticTypeList) {
this.problemStatisticTypeList = problemStatisticTypeList;
}
public List getSingleStatisticTypeList() {
return singleStatisticTypeList;
}
public void setSingleStatisticTypeList(List singleStatisticTypeList) {
this.singleStatisticTypeList = singleStatisticTypeList;
}
// ************************************************************************
// With methods
// ************************************************************************
public ProblemBenchmarksConfig withSolutionFileIOClass(Class extends SolutionFileIO>> solutionFileIOClass) {
this.setSolutionFileIOClass(solutionFileIOClass);
return this;
}
public ProblemBenchmarksConfig withWriteOutputSolutionEnabled(Boolean writeOutputSolutionEnabled) {
this.setWriteOutputSolutionEnabled(writeOutputSolutionEnabled);
return this;
}
public ProblemBenchmarksConfig withInputSolutionFileList(List inputSolutionFileList) {
this.setInputSolutionFileList(inputSolutionFileList);
return this;
}
public ProblemBenchmarksConfig withInputSolutionFiles(File... inputSolutionFiles) {
this.setInputSolutionFileList(List.of(inputSolutionFiles));
return this;
}
public ProblemBenchmarksConfig withProblemStatisticsEnabled(Boolean problemStatisticEnabled) {
this.setProblemStatisticEnabled(problemStatisticEnabled);
return this;
}
public ProblemBenchmarksConfig withProblemStatisticTypeList(List problemStatisticTypeList) {
this.setProblemStatisticTypeList(problemStatisticTypeList);
return this;
}
public ProblemBenchmarksConfig withProblemStatisticTypes(ProblemStatisticType... problemStatisticTypes) {
this.setProblemStatisticTypeList(List.of(problemStatisticTypes));
return this;
}
public ProblemBenchmarksConfig withSingleStatisticTypeList(List singleStatisticTypeList) {
this.setSingleStatisticTypeList(singleStatisticTypeList);
return this;
}
public ProblemBenchmarksConfig withSingleStatisticTypes(SingleStatisticType... singleStatisticTypes) {
this.setSingleStatisticTypeList(List.of(singleStatisticTypes));
return this;
}
// ************************************************************************
// Complex methods
// ************************************************************************
/**
* Return the problem statistic type list, or a list containing default metrics if problemStatisticEnabled
* is not false. If problemStatisticEnabled is false, an empty list is returned.
*
* @return never null
*/
public List determineProblemStatisticTypeList() {
if (problemStatisticEnabled != null && !problemStatisticEnabled) {
return Collections.emptyList();
}
if (problemStatisticTypeList == null || problemStatisticTypeList.isEmpty()) {
return ProblemStatisticType.defaultList();
}
return problemStatisticTypeList;
}
/**
* Return the single statistic type list, or an empty list if it is null
*
* @return never null
*/
public List determineSingleStatisticTypeList() {
return Objects.requireNonNullElse(singleStatisticTypeList, Collections.emptyList());
}
@Override
public ProblemBenchmarksConfig inherit(ProblemBenchmarksConfig inheritedConfig) {
solutionFileIOClass = ConfigUtils.inheritOverwritableProperty(solutionFileIOClass,
inheritedConfig.getSolutionFileIOClass());
writeOutputSolutionEnabled = ConfigUtils.inheritOverwritableProperty(writeOutputSolutionEnabled,
inheritedConfig.getWriteOutputSolutionEnabled());
inputSolutionFileList = ConfigUtils.inheritMergeableListProperty(inputSolutionFileList,
inheritedConfig.getInputSolutionFileList());
problemStatisticEnabled = ConfigUtils.inheritOverwritableProperty(problemStatisticEnabled,
inheritedConfig.getProblemStatisticEnabled());
problemStatisticTypeList = ConfigUtils.inheritUniqueMergeableListProperty(problemStatisticTypeList,
inheritedConfig.getProblemStatisticTypeList());
singleStatisticTypeList = ConfigUtils.inheritUniqueMergeableListProperty(singleStatisticTypeList,
inheritedConfig.getSingleStatisticTypeList());
return this;
}
@Override
public ProblemBenchmarksConfig copyConfig() {
return new ProblemBenchmarksConfig().inherit(this);
}
@Override
public void visitReferencedClasses(Consumer> classVisitor) {
classVisitor.accept(solutionFileIOClass);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy