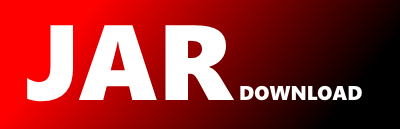
bluecrystal.service.validator.CrlValidatorImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bluecrystal.deps.service Show documentation
Show all versions of bluecrystal.deps.service Show documentation
API that should be used by applications
/*
Blue Crystal: Document Digital Signature Tool
Copyright (C) 2007-2015 Sergio Leal
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU Affero General Public License as
published by the Free Software Foundation, either version 3 of the
License, or (at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Affero General Public License for more details.
You should have received a copy of the GNU Affero General Public License
along with this program. If not, see .
*/
package bluecrystal.service.validator;
import java.io.IOException;
import java.security.cert.CRLException;
import java.security.cert.CertificateException;
import java.security.cert.X509CRL;
import java.security.cert.X509CRLEntry;
import java.security.cert.X509Certificate;
import java.util.Date;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import bluecrystal.domain.OperationStatus;
import bluecrystal.domain.StatusConst;
import bluecrystal.service.exception.RevokedException;
import bluecrystal.service.exception.UndefStateException;
import bluecrystal.service.loader.LCRLoader;
public class CrlValidatorImpl implements CrlValidator {
static final Logger logger = LoggerFactory.getLogger(CrlValidatorImpl.class);
private LCRLoader lcrLoader;
public CrlValidatorImpl(LCRLoader lcrLoader) {
super();
this.lcrLoader = lcrLoader;
}
public OperationStatus verifyLCR(X509Certificate nextCert,
Date date, List distPoints)
throws IOException, CertificateException, CRLException,
UndefStateException, RevokedException {
// List distPoints = getCrlDistributionPoints(nextCert);
X509CRL lcr = null;
if (distPoints.size() != 0) {
lcr = lcrLoader.get(distPoints, date);
if (lcr != null) {
X509CRLEntry entry = lcr.getRevokedCertificate(nextCert
.getSerialNumber());
if (entry != null) {
if (entry.getRevocationDate() != null
&& entry.getRevocationDate().before(date)) {
throw new RevokedException();
}
}
if (lcr.getThisUpdate().before(date)) {
logger.debug("LCR ? anterior a data da assinatura, n?o tenho como ter certeza do status: UNKOWN");
Date upd = lcr.getNextUpdate();
return new OperationStatus(StatusConst.UNKNOWN, upd);
}
Date upd = lcr.getNextUpdate();
return new OperationStatus(StatusConst.GOOD, upd);
}
else {
logger.error("LCR is NULL");
return new OperationStatus(StatusConst.UNTRUSTED, null);
}
} else {
logger.error("CRL DP not found");
return new OperationStatus(StatusConst.UNKNOWN, null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy