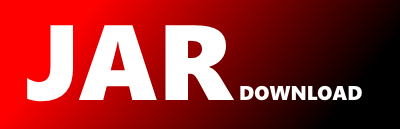
main.app.cash.backfila.protos.clientservice.PrepareBackfillResponse Maven / Gradle / Ivy
// Code generated by Wire protocol buffer compiler, do not edit.
// Source: app.cash.backfila.protos.clientservice.PrepareBackfillResponse in app/cash/backfila/client_service.proto
package app.cash.backfila.protos.clientservice;
import com.squareup.wire.FieldEncoding;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoAdapter;
import com.squareup.wire.ProtoReader;
import com.squareup.wire.ProtoWriter;
import com.squareup.wire.ReverseProtoWriter;
import com.squareup.wire.Syntax;
import com.squareup.wire.WireField;
import com.squareup.wire.internal.Internal;
import java.io.IOException;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.List;
import java.util.Map;
import okio.ByteString;
public final class PrepareBackfillResponse extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_PrepareBackfillResponse();
private static final long serialVersionUID = 0L;
public static final String DEFAULT_ERROR_MESSAGE = "";
@WireField(
tag = 1,
adapter = "app.cash.backfila.protos.clientservice.PrepareBackfillResponse$Partition#ADAPTER",
label = WireField.Label.REPEATED
)
public final List partitions;
/**
* Parameters created by the client to keep for the life of the backfill.
* Every backfill call will pass these parameters back, in addition to user provided parameters.
*/
@WireField(
tag = 2,
keyAdapter = "com.squareup.wire.ProtoAdapter#STRING",
adapter = "com.squareup.wire.ProtoAdapter#BYTES"
)
public final Map parameters;
/**
* Error message that will be surfaced to the user.
* If this is provided this prepare request is assumed to have failed and no backfill will be created.
*/
@WireField(
tag = 3,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String error_message;
public PrepareBackfillResponse(List partitions, Map parameters,
String error_message) {
this(partitions, parameters, error_message, ByteString.EMPTY);
}
public PrepareBackfillResponse(List partitions, Map parameters,
String error_message, ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.partitions = Internal.immutableCopyOf("partitions", partitions);
this.parameters = Internal.immutableCopyOf("parameters", parameters);
this.error_message = error_message;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.partitions = Internal.copyOf(partitions);
builder.parameters = Internal.copyOf(parameters);
builder.error_message = error_message;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof PrepareBackfillResponse)) return false;
PrepareBackfillResponse o = (PrepareBackfillResponse) other;
return unknownFields().equals(o.unknownFields())
&& partitions.equals(o.partitions)
&& parameters.equals(o.parameters)
&& Internal.equals(error_message, o.error_message);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + partitions.hashCode();
result = result * 37 + parameters.hashCode();
result = result * 37 + (error_message != null ? error_message.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
if (!partitions.isEmpty()) builder.append(", partitions=").append(partitions);
if (!parameters.isEmpty()) builder.append(", parameters=").append(parameters);
if (error_message != null) builder.append(", error_message=").append(Internal.sanitize(error_message));
return builder.replace(0, 2, "PrepareBackfillResponse{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public List partitions;
public Map parameters;
public String error_message;
public Builder() {
partitions = Internal.newMutableList();
parameters = Internal.newMutableMap();
}
public Builder partitions(List partitions) {
Internal.checkElementsNotNull(partitions);
this.partitions = partitions;
return this;
}
/**
* Parameters created by the client to keep for the life of the backfill.
* Every backfill call will pass these parameters back, in addition to user provided parameters.
*/
public Builder parameters(Map parameters) {
Internal.checkElementsNotNull(parameters);
this.parameters = parameters;
return this;
}
/**
* Error message that will be surfaced to the user.
* If this is provided this prepare request is assumed to have failed and no backfill will be created.
*/
public Builder error_message(String error_message) {
this.error_message = error_message;
return this;
}
@Override
public PrepareBackfillResponse build() {
return new PrepareBackfillResponse(partitions, parameters, error_message, super.buildUnknownFields());
}
}
public static final class Partition extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_Partition();
private static final long serialVersionUID = 0L;
public static final String DEFAULT_PARTITION_NAME = "";
public static final Long DEFAULT_ESTIMATED_RECORD_COUNT = 0L;
@WireField(
tag = 1,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String partition_name;
@WireField(
tag = 2,
adapter = "app.cash.backfila.protos.clientservice.KeyRange#ADAPTER"
)
public final KeyRange backfill_range;
/**
* If available, an estimate can be provided here to display while the
* actual count is being computed.
*/
@WireField(
tag = 3,
adapter = "com.squareup.wire.ProtoAdapter#UINT64"
)
public final Long estimated_record_count;
public Partition(String partition_name, KeyRange backfill_range, Long estimated_record_count) {
this(partition_name, backfill_range, estimated_record_count, ByteString.EMPTY);
}
public Partition(String partition_name, KeyRange backfill_range, Long estimated_record_count,
ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.partition_name = partition_name;
this.backfill_range = backfill_range;
this.estimated_record_count = estimated_record_count;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.partition_name = partition_name;
builder.backfill_range = backfill_range;
builder.estimated_record_count = estimated_record_count;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof Partition)) return false;
Partition o = (Partition) other;
return unknownFields().equals(o.unknownFields())
&& Internal.equals(partition_name, o.partition_name)
&& Internal.equals(backfill_range, o.backfill_range)
&& Internal.equals(estimated_record_count, o.estimated_record_count);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + (partition_name != null ? partition_name.hashCode() : 0);
result = result * 37 + (backfill_range != null ? backfill_range.hashCode() : 0);
result = result * 37 + (estimated_record_count != null ? estimated_record_count.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
if (partition_name != null) builder.append(", partition_name=").append(Internal.sanitize(partition_name));
if (backfill_range != null) builder.append(", backfill_range=").append(backfill_range);
if (estimated_record_count != null) builder.append(", estimated_record_count=").append(estimated_record_count);
return builder.replace(0, 2, "Partition{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public String partition_name;
public KeyRange backfill_range;
public Long estimated_record_count;
public Builder() {
}
public Builder partition_name(String partition_name) {
this.partition_name = partition_name;
return this;
}
public Builder backfill_range(KeyRange backfill_range) {
this.backfill_range = backfill_range;
return this;
}
/**
* If available, an estimate can be provided here to display while the
* actual count is being computed.
*/
public Builder estimated_record_count(Long estimated_record_count) {
this.estimated_record_count = estimated_record_count;
return this;
}
@Override
public Partition build() {
return new Partition(partition_name, backfill_range, estimated_record_count, super.buildUnknownFields());
}
}
private static final class ProtoAdapter_Partition extends ProtoAdapter {
public ProtoAdapter_Partition() {
super(FieldEncoding.LENGTH_DELIMITED, Partition.class, "type.googleapis.com/app.cash.backfila.protos.clientservice.PrepareBackfillResponse.Partition", Syntax.PROTO_2, null, "app/cash/backfila/client_service.proto");
}
@Override
public int encodedSize(Partition value) {
int result = 0;
result += ProtoAdapter.STRING.encodedSizeWithTag(1, value.partition_name);
result += KeyRange.ADAPTER.encodedSizeWithTag(2, value.backfill_range);
result += ProtoAdapter.UINT64.encodedSizeWithTag(3, value.estimated_record_count);
result += value.unknownFields().size();
return result;
}
@Override
public void encode(ProtoWriter writer, Partition value) throws IOException {
ProtoAdapter.STRING.encodeWithTag(writer, 1, value.partition_name);
KeyRange.ADAPTER.encodeWithTag(writer, 2, value.backfill_range);
ProtoAdapter.UINT64.encodeWithTag(writer, 3, value.estimated_record_count);
writer.writeBytes(value.unknownFields());
}
@Override
public void encode(ReverseProtoWriter writer, Partition value) throws IOException {
writer.writeBytes(value.unknownFields());
ProtoAdapter.UINT64.encodeWithTag(writer, 3, value.estimated_record_count);
KeyRange.ADAPTER.encodeWithTag(writer, 2, value.backfill_range);
ProtoAdapter.STRING.encodeWithTag(writer, 1, value.partition_name);
}
@Override
public Partition decode(ProtoReader reader) throws IOException {
Builder builder = new Builder();
long token = reader.beginMessage();
for (int tag; (tag = reader.nextTag()) != -1;) {
switch (tag) {
case 1: builder.partition_name(ProtoAdapter.STRING.decode(reader)); break;
case 2: builder.backfill_range(KeyRange.ADAPTER.decode(reader)); break;
case 3: builder.estimated_record_count(ProtoAdapter.UINT64.decode(reader)); break;
default: {
reader.readUnknownField(tag);
}
}
}
builder.addUnknownFields(reader.endMessageAndGetUnknownFields(token));
return builder.build();
}
@Override
public Partition redact(Partition value) {
Builder builder = value.newBuilder();
if (builder.backfill_range != null) builder.backfill_range = KeyRange.ADAPTER.redact(builder.backfill_range);
builder.clearUnknownFields();
return builder.build();
}
}
}
private static final class ProtoAdapter_PrepareBackfillResponse extends ProtoAdapter {
private ProtoAdapter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy