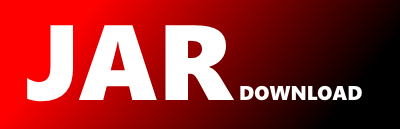
main.app.cash.backfila.protos.service.ConfigureServiceRequest Maven / Gradle / Ivy
// Code generated by Wire protocol buffer compiler, do not edit.
// Source: app.cash.backfila.protos.service.ConfigureServiceRequest in app/cash/backfila/service.proto
package app.cash.backfila.protos.service;
import com.squareup.wire.FieldEncoding;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoAdapter;
import com.squareup.wire.ProtoReader;
import com.squareup.wire.ProtoWriter;
import com.squareup.wire.ReverseProtoWriter;
import com.squareup.wire.Syntax;
import com.squareup.wire.WireField;
import com.squareup.wire.internal.Internal;
import java.io.IOException;
import java.lang.Boolean;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.List;
import okio.ByteString;
public final class ConfigureServiceRequest extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_ConfigureServiceRequest();
private static final long serialVersionUID = 0L;
public static final String DEFAULT_CONNECTOR_TYPE = "";
public static final String DEFAULT_CONNECTOR_EXTRA_DATA = "";
public static final String DEFAULT_SLACK_CHANNEL = "";
public static final String DEFAULT_VARIANT = "";
/**
* List of all available backfills in this service
*/
@WireField(
tag = 1,
adapter = "app.cash.backfila.protos.service.ConfigureServiceRequest$BackfillData#ADAPTER",
label = WireField.Label.REPEATED
)
public final List backfills;
/**
* This isn't encoded as protobuf so connector implementations can be added without modifying
* protos (thus avoiding modifying the core backfila codebase).
*/
@WireField(
tag = 2,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String connector_type;
/**
* Extra data that is passed through to the connector corresponding to connector_type.
* Suggested encoding is JSON. For example ENVOY type could have the cluster type as extra data:
* e.g.: `{"cluster_type": "production-jobs"}`
*/
@WireField(
tag = 3,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String connector_extra_data;
@WireField(
tag = 4,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String slack_channel;
/**
* A service can have multiple variants, each registered independently with Backfila.
* Variants have their own set of runs, use different connector configs, etc but are grouped and still identify as the same service.
*
* As an example use case, a service could register different variants for a production and sandbox deployment.
* Backfila would then be able to run backfills for both deployments independently.
*/
@WireField(
tag = 5,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String variant;
public ConfigureServiceRequest(List backfills, String connector_type,
String connector_extra_data, String slack_channel, String variant) {
this(backfills, connector_type, connector_extra_data, slack_channel, variant, ByteString.EMPTY);
}
public ConfigureServiceRequest(List backfills, String connector_type,
String connector_extra_data, String slack_channel, String variant, ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.backfills = Internal.immutableCopyOf("backfills", backfills);
this.connector_type = connector_type;
this.connector_extra_data = connector_extra_data;
this.slack_channel = slack_channel;
this.variant = variant;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.backfills = Internal.copyOf(backfills);
builder.connector_type = connector_type;
builder.connector_extra_data = connector_extra_data;
builder.slack_channel = slack_channel;
builder.variant = variant;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof ConfigureServiceRequest)) return false;
ConfigureServiceRequest o = (ConfigureServiceRequest) other;
return unknownFields().equals(o.unknownFields())
&& backfills.equals(o.backfills)
&& Internal.equals(connector_type, o.connector_type)
&& Internal.equals(connector_extra_data, o.connector_extra_data)
&& Internal.equals(slack_channel, o.slack_channel)
&& Internal.equals(variant, o.variant);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + backfills.hashCode();
result = result * 37 + (connector_type != null ? connector_type.hashCode() : 0);
result = result * 37 + (connector_extra_data != null ? connector_extra_data.hashCode() : 0);
result = result * 37 + (slack_channel != null ? slack_channel.hashCode() : 0);
result = result * 37 + (variant != null ? variant.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
if (!backfills.isEmpty()) builder.append(", backfills=").append(backfills);
if (connector_type != null) builder.append(", connector_type=").append(Internal.sanitize(connector_type));
if (connector_extra_data != null) builder.append(", connector_extra_data=").append(Internal.sanitize(connector_extra_data));
if (slack_channel != null) builder.append(", slack_channel=").append(Internal.sanitize(slack_channel));
if (variant != null) builder.append(", variant=").append(Internal.sanitize(variant));
return builder.replace(0, 2, "ConfigureServiceRequest{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public List backfills;
public String connector_type;
public String connector_extra_data;
public String slack_channel;
public String variant;
public Builder() {
backfills = Internal.newMutableList();
}
/**
* List of all available backfills in this service
*/
public Builder backfills(List backfills) {
Internal.checkElementsNotNull(backfills);
this.backfills = backfills;
return this;
}
/**
* This isn't encoded as protobuf so connector implementations can be added without modifying
* protos (thus avoiding modifying the core backfila codebase).
*/
public Builder connector_type(String connector_type) {
this.connector_type = connector_type;
return this;
}
/**
* Extra data that is passed through to the connector corresponding to connector_type.
* Suggested encoding is JSON. For example ENVOY type could have the cluster type as extra data:
* e.g.: `{"cluster_type": "production-jobs"}`
*/
public Builder connector_extra_data(String connector_extra_data) {
this.connector_extra_data = connector_extra_data;
return this;
}
public Builder slack_channel(String slack_channel) {
this.slack_channel = slack_channel;
return this;
}
/**
* A service can have multiple variants, each registered independently with Backfila.
* Variants have their own set of runs, use different connector configs, etc but are grouped and still identify as the same service.
*
* As an example use case, a service could register different variants for a production and sandbox deployment.
* Backfila would then be able to run backfills for both deployments independently.
*/
public Builder variant(String variant) {
this.variant = variant;
return this;
}
@Override
public ConfigureServiceRequest build() {
return new ConfigureServiceRequest(backfills, connector_type, connector_extra_data, slack_channel, variant, super.buildUnknownFields());
}
}
public static final class BackfillData extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_BackfillData();
private static final long serialVersionUID = 0L;
public static final String DEFAULT_NAME = "";
public static final String DEFAULT_DESCRIPTION = "";
public static final String DEFAULT_TYPE_PROVIDED = "";
public static final String DEFAULT_TYPE_CONSUMED = "";
public static final Boolean DEFAULT_REQUIRES_APPROVAL = false;
public static final Long DEFAULT_DELETE_BY = 0L;
/**
* Identifies the backfill. Must be unique per service.
*/
@WireField(
tag = 1,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String name;
/**
* Description to show in the UI.
*/
@WireField(
tag = 2,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String description;
/**
* Parameters the backfill will accept. An input is displayed in the UI for each parameter.
*/
@WireField(
tag = 3,
adapter = "app.cash.backfila.protos.service.Parameter#ADAPTER",
label = WireField.Label.REPEATED
)
public final List parameters;
/**
* The class name of pipelined data, only set if backfill supports pipelining.
*/
@WireField(
tag = 4,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String type_provided;
@WireField(
tag = 5,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String type_consumed;
/**
* If true the backfill will need approval from another user before it can be started.
*/
@WireField(
tag = 6,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean requires_approval;
/**
* Notes when this backfill should no longer be useful and the code should be deleted. This will
* be used to power removal reminders. It will guarantee that reminders will not occur until
* after this date. This defaults to as soon as possible if it is missing.
*/
@WireField(
tag = 7,
adapter = "com.squareup.wire.ProtoAdapter#UINT64"
)
public final Long delete_by;
public BackfillData(String name, String description, List parameters,
String type_provided, String type_consumed, Boolean requires_approval, Long delete_by) {
this(name, description, parameters, type_provided, type_consumed, requires_approval, delete_by, ByteString.EMPTY);
}
public BackfillData(String name, String description, List parameters,
String type_provided, String type_consumed, Boolean requires_approval, Long delete_by,
ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.name = name;
this.description = description;
this.parameters = Internal.immutableCopyOf("parameters", parameters);
this.type_provided = type_provided;
this.type_consumed = type_consumed;
this.requires_approval = requires_approval;
this.delete_by = delete_by;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.name = name;
builder.description = description;
builder.parameters = Internal.copyOf(parameters);
builder.type_provided = type_provided;
builder.type_consumed = type_consumed;
builder.requires_approval = requires_approval;
builder.delete_by = delete_by;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof BackfillData)) return false;
BackfillData o = (BackfillData) other;
return unknownFields().equals(o.unknownFields())
&& Internal.equals(name, o.name)
&& Internal.equals(description, o.description)
&& parameters.equals(o.parameters)
&& Internal.equals(type_provided, o.type_provided)
&& Internal.equals(type_consumed, o.type_consumed)
&& Internal.equals(requires_approval, o.requires_approval)
&& Internal.equals(delete_by, o.delete_by);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + (name != null ? name.hashCode() : 0);
result = result * 37 + (description != null ? description.hashCode() : 0);
result = result * 37 + parameters.hashCode();
result = result * 37 + (type_provided != null ? type_provided.hashCode() : 0);
result = result * 37 + (type_consumed != null ? type_consumed.hashCode() : 0);
result = result * 37 + (requires_approval != null ? requires_approval.hashCode() : 0);
result = result * 37 + (delete_by != null ? delete_by.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
if (name != null) builder.append(", name=").append(Internal.sanitize(name));
if (description != null) builder.append(", description=").append(Internal.sanitize(description));
if (!parameters.isEmpty()) builder.append(", parameters=").append(parameters);
if (type_provided != null) builder.append(", type_provided=").append(Internal.sanitize(type_provided));
if (type_consumed != null) builder.append(", type_consumed=").append(Internal.sanitize(type_consumed));
if (requires_approval != null) builder.append(", requires_approval=").append(requires_approval);
if (delete_by != null) builder.append(", delete_by=").append(delete_by);
return builder.replace(0, 2, "BackfillData{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public String name;
public String description;
public List parameters;
public String type_provided;
public String type_consumed;
public Boolean requires_approval;
public Long delete_by;
public Builder() {
parameters = Internal.newMutableList();
}
/**
* Identifies the backfill. Must be unique per service.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* Description to show in the UI.
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* Parameters the backfill will accept. An input is displayed in the UI for each parameter.
*/
public Builder parameters(List parameters) {
Internal.checkElementsNotNull(parameters);
this.parameters = parameters;
return this;
}
/**
* The class name of pipelined data, only set if backfill supports pipelining.
*/
public Builder type_provided(String type_provided) {
this.type_provided = type_provided;
return this;
}
public Builder type_consumed(String type_consumed) {
this.type_consumed = type_consumed;
return this;
}
/**
* If true the backfill will need approval from another user before it can be started.
*/
public Builder requires_approval(Boolean requires_approval) {
this.requires_approval = requires_approval;
return this;
}
/**
* Notes when this backfill should no longer be useful and the code should be deleted. This will
* be used to power removal reminders. It will guarantee that reminders will not occur until
* after this date. This defaults to as soon as possible if it is missing.
*/
public Builder delete_by(Long delete_by) {
this.delete_by = delete_by;
return this;
}
@Override
public BackfillData build() {
return new BackfillData(name, description, parameters, type_provided, type_consumed, requires_approval, delete_by, super.buildUnknownFields());
}
}
private static final class ProtoAdapter_BackfillData extends ProtoAdapter {
public ProtoAdapter_BackfillData() {
super(FieldEncoding.LENGTH_DELIMITED, BackfillData.class, "type.googleapis.com/app.cash.backfila.protos.service.ConfigureServiceRequest.BackfillData", Syntax.PROTO_2, null, "app/cash/backfila/service.proto");
}
@Override
public int encodedSize(BackfillData value) {
int result = 0;
result += ProtoAdapter.STRING.encodedSizeWithTag(1, value.name);
result += ProtoAdapter.STRING.encodedSizeWithTag(2, value.description);
result += Parameter.ADAPTER.asRepeated().encodedSizeWithTag(3, value.parameters);
result += ProtoAdapter.STRING.encodedSizeWithTag(4, value.type_provided);
result += ProtoAdapter.STRING.encodedSizeWithTag(5, value.type_consumed);
result += ProtoAdapter.BOOL.encodedSizeWithTag(6, value.requires_approval);
result += ProtoAdapter.UINT64.encodedSizeWithTag(7, value.delete_by);
result += value.unknownFields().size();
return result;
}
@Override
public void encode(ProtoWriter writer, BackfillData value) throws IOException {
ProtoAdapter.STRING.encodeWithTag(writer, 1, value.name);
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.description);
Parameter.ADAPTER.asRepeated().encodeWithTag(writer, 3, value.parameters);
ProtoAdapter.STRING.encodeWithTag(writer, 4, value.type_provided);
ProtoAdapter.STRING.encodeWithTag(writer, 5, value.type_consumed);
ProtoAdapter.BOOL.encodeWithTag(writer, 6, value.requires_approval);
ProtoAdapter.UINT64.encodeWithTag(writer, 7, value.delete_by);
writer.writeBytes(value.unknownFields());
}
@Override
public void encode(ReverseProtoWriter writer, BackfillData value) throws IOException {
writer.writeBytes(value.unknownFields());
ProtoAdapter.UINT64.encodeWithTag(writer, 7, value.delete_by);
ProtoAdapter.BOOL.encodeWithTag(writer, 6, value.requires_approval);
ProtoAdapter.STRING.encodeWithTag(writer, 5, value.type_consumed);
ProtoAdapter.STRING.encodeWithTag(writer, 4, value.type_provided);
Parameter.ADAPTER.asRepeated().encodeWithTag(writer, 3, value.parameters);
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.description);
ProtoAdapter.STRING.encodeWithTag(writer, 1, value.name);
}
@Override
public BackfillData decode(ProtoReader reader) throws IOException {
Builder builder = new Builder();
long token = reader.beginMessage();
for (int tag; (tag = reader.nextTag()) != -1;) {
switch (tag) {
case 1: builder.name(ProtoAdapter.STRING.decode(reader)); break;
case 2: builder.description(ProtoAdapter.STRING.decode(reader)); break;
case 3: builder.parameters.add(Parameter.ADAPTER.decode(reader)); break;
case 4: builder.type_provided(ProtoAdapter.STRING.decode(reader)); break;
case 5: builder.type_consumed(ProtoAdapter.STRING.decode(reader)); break;
case 6: builder.requires_approval(ProtoAdapter.BOOL.decode(reader)); break;
case 7: builder.delete_by(ProtoAdapter.UINT64.decode(reader)); break;
default: {
reader.readUnknownField(tag);
}
}
}
builder.addUnknownFields(reader.endMessageAndGetUnknownFields(token));
return builder.build();
}
@Override
public BackfillData redact(BackfillData value) {
Builder builder = value.newBuilder();
Internal.redactElements(builder.parameters, Parameter.ADAPTER);
builder.clearUnknownFields();
return builder.build();
}
}
}
private static final class ProtoAdapter_ConfigureServiceRequest extends ProtoAdapter {
public ProtoAdapter_ConfigureServiceRequest() {
super(FieldEncoding.LENGTH_DELIMITED, ConfigureServiceRequest.class, "type.googleapis.com/app.cash.backfila.protos.service.ConfigureServiceRequest", Syntax.PROTO_2, null, "app/cash/backfila/service.proto");
}
@Override
public int encodedSize(ConfigureServiceRequest value) {
int result = 0;
result += BackfillData.ADAPTER.asRepeated().encodedSizeWithTag(1, value.backfills);
result += ProtoAdapter.STRING.encodedSizeWithTag(2, value.connector_type);
result += ProtoAdapter.STRING.encodedSizeWithTag(3, value.connector_extra_data);
result += ProtoAdapter.STRING.encodedSizeWithTag(4, value.slack_channel);
result += ProtoAdapter.STRING.encodedSizeWithTag(5, value.variant);
result += value.unknownFields().size();
return result;
}
@Override
public void encode(ProtoWriter writer, ConfigureServiceRequest value) throws IOException {
BackfillData.ADAPTER.asRepeated().encodeWithTag(writer, 1, value.backfills);
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.connector_type);
ProtoAdapter.STRING.encodeWithTag(writer, 3, value.connector_extra_data);
ProtoAdapter.STRING.encodeWithTag(writer, 4, value.slack_channel);
ProtoAdapter.STRING.encodeWithTag(writer, 5, value.variant);
writer.writeBytes(value.unknownFields());
}
@Override
public void encode(ReverseProtoWriter writer, ConfigureServiceRequest value) throws
IOException {
writer.writeBytes(value.unknownFields());
ProtoAdapter.STRING.encodeWithTag(writer, 5, value.variant);
ProtoAdapter.STRING.encodeWithTag(writer, 4, value.slack_channel);
ProtoAdapter.STRING.encodeWithTag(writer, 3, value.connector_extra_data);
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.connector_type);
BackfillData.ADAPTER.asRepeated().encodeWithTag(writer, 1, value.backfills);
}
@Override
public ConfigureServiceRequest decode(ProtoReader reader) throws IOException {
Builder builder = new Builder();
long token = reader.beginMessage();
for (int tag; (tag = reader.nextTag()) != -1;) {
switch (tag) {
case 1: builder.backfills.add(BackfillData.ADAPTER.decode(reader)); break;
case 2: builder.connector_type(ProtoAdapter.STRING.decode(reader)); break;
case 3: builder.connector_extra_data(ProtoAdapter.STRING.decode(reader)); break;
case 4: builder.slack_channel(ProtoAdapter.STRING.decode(reader)); break;
case 5: builder.variant(ProtoAdapter.STRING.decode(reader)); break;
default: {
reader.readUnknownField(tag);
}
}
}
builder.addUnknownFields(reader.endMessageAndGetUnknownFields(token));
return builder.build();
}
@Override
public ConfigureServiceRequest redact(ConfigureServiceRequest value) {
Builder builder = value.newBuilder();
Internal.redactElements(builder.backfills, BackfillData.ADAPTER);
builder.clearUnknownFields();
return builder.build();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy