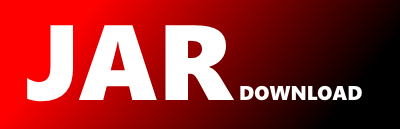
app.cash.quiver.extensions.Option.kt Maven / Gradle / Ivy
@file:Suppress("TYPEALIAS_EXPANSION_DEPRECATION", "DEPRECATION")
package app.cash.quiver.extensions
import arrow.core.Either
import arrow.core.None
import arrow.core.Option
import arrow.core.Some
import arrow.core.ValidatedNel
import arrow.core.getOrElse
import arrow.core.nonEmptyListOf
import kotlin.experimental.ExperimentalTypeInference
import app.cash.quiver.extensions.traverse as quiverTraverse
/**
* Takes a function to run if your Option is None. Returns Unit if your Option is Some.
*/
inline fun Option.ifAbsent(f: () -> Unit): Unit {
onNone { f() }
}
/**
* Runs a side effect if the option is a Some. Same as forEach, just a different name for better symmetry with ifAbsent.
*/
inline fun Option.ifPresent(f: (A) -> Unit) {
forEach { f(it) }
}
/**
* Runs a side effect if the option is a Some
*/
inline fun Option.forEach(f: (A) -> Unit) {
onSome { f(it) }
}
/**
* Turns your Option into a Validated list of T if it's a Some.
* If it's a None, will return a Nel of the error function passed in
*/
inline fun Option.toValidatedNel(error: () -> E): ValidatedNel =
ValidatedNel.fromOption(this) { nonEmptyListOf(error()) }
/**
* Map some to Unit. This restores `.void()` which was deprecated by Arrow.
*/
fun Option.unit() = map { }
/**
* Returns `this` if it's a Some, otherwise returns the `other` instance
*/
infix fun Option.or(other: () -> Option): Option = when (this) {
is Some -> this
is None -> other()
}
/**
* Returns `this` if it's a Some, otherwise returns the `other` instance
*/
@Deprecated(
"Use or(other: () -> Option) instead",
ReplaceWith("or { other }")
)
infix fun Option.or(other: Option): Option = when (this) {
is Some -> this
is None -> other
}
/**
* Will return an empty string if the Optional value supplied is None
*/
fun Option.orEmpty(f: (T) -> String): String = this.map(f).getOrElse { "" }
/**
* Map a function that returns an Either across the Option.
*
* If the option is a None, return a Right(None).
* If the option is a Some, apply f to the value. If f returns a Right, wrap the result in a Some.
*/
@Suppress("EXTENSION_SHADOWED_BY_MEMBER")
inline fun Option.traverse(f: (T) -> Either): Either> =
fold( { Either.Right(None) }, { a -> f(a).map { Some(it) } })
/**
* Synonym for traverse((T)-> Either): Either>
*/
@Suppress("EXTENSION_SHADOWED_BY_MEMBER")
inline fun Option.traverseEither(f: (T) -> Either): Either> =
quiverTraverse(f)
/**
* Map a function that returns an Iterable across the Option.
*
* If the option is a None, return an empty List
* If the option is a Some, apply f to the value and wrap the result in a Some.
*/
@Suppress("EXTENSION_SHADOWED_BY_MEMBER")
@OptIn(ExperimentalTypeInference::class)
@OverloadResolutionByLambdaReturnType
inline fun Option.traverse(f: (A) -> Iterable): List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy