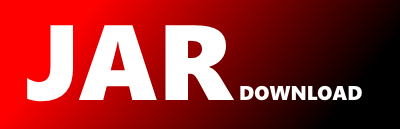
as.leap.vertx.rpc.impl.RPCBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-rpc Show documentation
Show all versions of vertx-rpc Show documentation
Wrap eventBus of vert.x 3 as transport layer for RPC invoking
package as.leap.vertx.rpc.impl;
import as.leap.vertx.rpc.IgnoreWrapCheck;
import as.leap.vertx.rpc.WireProtocol;
import io.protostuff.JsonIOUtil;
import io.protostuff.LinkedBuffer;
import io.protostuff.ProtobufIOUtil;
import io.protostuff.Schema;
import io.protostuff.runtime.RuntimeSchema;
import java.io.IOException;
import java.lang.reflect.Modifier;
import java.util.Collection;
import java.util.Map;
import java.util.Objects;
/**
* Created by stream.
*/
abstract class RPCBase {
protected static final String CALLBACK_TYPE = "callbackType";
private WireProtocol wireProtocol;
public RPCBase(WireProtocol wireProtocol) {
this.wireProtocol = wireProtocol;
}
void checkBusAddress(String address) {
Objects.requireNonNull(address, "service's event bus address can not be null.");
}
boolean isWrapType(Class clazz) {
if (clazz.getAnnotation(IgnoreWrapCheck.class) != null) {
return false;
} else {
return clazz.isPrimitive() || clazz.isArray() || clazz.isEnum()
|| Collection.class.isAssignableFrom(clazz)
|| Map.class.isAssignableFrom(clazz)
|| Modifier.isAbstract(clazz.getModifiers())
|| clazz.isInterface();
}
}
private byte[] toBytes(Schema schema, T object) throws Exception {
LinkedBuffer buffer = LinkedBuffer.allocate();
byte[] bytes = new byte[0];
try {
switch (wireProtocol) {
case PROTOBUF:
bytes = ProtobufIOUtil.toByteArray(object, schema, buffer);
break;
case JSON:
bytes = JsonIOUtil.toByteArray(object, schema, false, buffer);
break;
}
} finally {
buffer.clear();
}
return bytes;
}
byte[] getWrapTypeBytes(Object object, Class clazz) throws Exception {
WrapperType wrapperType = new WrapperType(object, clazz);
Schema schema = RuntimeSchema.getSchema(WrapperType.class);
return toBytes(schema, wrapperType);
}
byte[] asBytes(T object) throws Exception {
return asBytes(object, object.getClass());
}
byte[] asBytes(Object object, Class clazz) throws Exception {
if (isWrapType(clazz)) {
return getWrapTypeBytes(object, clazz);
} else {
Schema
© 2015 - 2025 Weber Informatics LLC | Privacy Policy