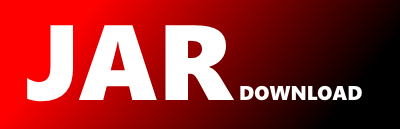
at.chrl.callbacks.EnhancedObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chrl-callbacks Show documentation
Show all versions of chrl-callbacks Show documentation
Callback Framework to hook up on method calls with annotations
/**
* This file is part of aion-lightning .
*
* aion-lightning is free software: you can redistribute it and/or modify it
* under the terms of the GNU General Public License as published by the Free
* Software Foundation, either version 3 of the License, or (at your option) any
* later version.
*
* aion-lightning is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* aion-lightning. If not, see .
*/
package at.chrl.callbacks;
import java.util.List;
import java.util.Map;
import java.util.concurrent.locks.ReentrantReadWriteLock;
/**
* Generic interface for all enhanced object.
* NEVER IMPLEMENT THIS CLASS MANUALLY!!!
*
* Thread safety, concurrency, deadlocks:
* It's allowed to remove/add listeners from listeners.
* Listeners are stored in the {@link java.util.concurrent.CopyOnWriteArrayList}
* , so their behavior is similar.
* Briefly speaking, if you will try to remove/add a listener from another
* listener - the current invocation won't be affected, current implementation
* allocates all listeners that are going to be invoked before execution.
*
* {@link Callback#beforeCall(Object, Object[])} and
* {@link Callback#afterCall(Object, Object[], Object)} are treated as separate
* invocations, so adding/removing listener in beforeCall will affect afterCall.
*
* @author SoulKeeper
*/
@SuppressWarnings("rawtypes")
public interface EnhancedObject {
/**
* Adds callback to this object.
* {@link at.chrl.callbacks.EnhancedObject concurrency description}
*
* @param callback
* instance of callback to add
* @see at.chrl.callbacks.util.ObjectCallbackHelper#addCallback(Callback,
* EnhancedObject)
*/
public void addCallback(Callback callback);
/**
* Removes callback from this object.
* {@link at.chrl.callbacks.EnhancedObject concurrency description}
*
* @param callback
* instance of callback to remove
* @see at.chrl.callbacks.util.ObjectCallbackHelper#removeCallback(Callback,
* EnhancedObject)
*/
public void removeCallback(Callback callback);
/**
* Returns all callbacks associated with this.
*
* Iteration over this map is not thread-safe, please
* make sure that {@link #getCallbackLock()} is locked in read mode to read.
*
* Same for writing. If you are going to write something here - please make
* sure that {@link #getCallbackLock()} is in write mode
*
* @return map with callbacks associated with this object or null if there
* is no callbacks
*/
public Map, List> getCallbacks();
/**
* Associates callback map with this object.
*
* Please make sure that {@link #getCallbackLock()} is
* in write-mode lock when calling this method
*
* @param callbacks
* callbackMap or null
*/
public void setCallbacks(Map, List> callbacks);
/**
* Returns lock that is used to ensure thread safety
*
* @return lock that is used to ensure thread safety
*/
public ReentrantReadWriteLock getCallbackLock();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy