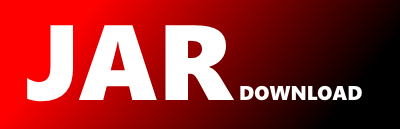
at.gridgears.held.internal.parser.LocationParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of held-api Show documentation
Show all versions of held-api Show documentation
API for Http Enabled Location Discovery (HELD)
package at.gridgears.held.internal.parser;
import at.gridgears.held.AmlData;
import at.gridgears.held.Location;
import at.gridgears.schemas.held.*;
import org.apache.commons.lang3.mutable.MutableObject;
import javax.annotation.Nullable;
import javax.xml.datatype.XMLGregorianCalendar;
import java.time.Instant;
import java.util.LinkedList;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import static at.gridgears.held.internal.parser.ParseUtils.toInstant;
final class LocationParser {
private final AmlDataParser amlDataParser = new AmlDataParser();
List parse(LocationResponseType locationResponseType) {
MutableObject timestamp = new MutableObject<>();
List result = new LinkedList<>();
ParseUtils.getValue(locationResponseType.getAny(), Presence.class)
.map(Presence::getTuple)
.map(ParseUtils::first)
.map(storeTimestamp(timestamp))
.map(Tuple::getStatus)
.map(Status::getAny)
.map(ParseUtils::first)
.map(LocationParser::getGeopriv)
.ifPresent(geopriv -> result.addAll(parseLocation(geopriv, timestamp.getValue())));
return result;
}
private List parseLocation(Geopriv geopriv, Instant timestamp) {
List result = new LinkedList<>();
MutableObject amlData = new MutableObject<>();
ParseUtils.getValue(geopriv.getAny(), AmlType.class).ifPresent(amlType -> amlData.setValue(amlDataParser.parseAmlData(amlType)));
LocInfoType locationInfo = geopriv.getLocationInfo();
if (locationInfo != null) {
result.addAll(parseLocations(locationInfo.getAny(), timestamp, amlData.getValue()));
}
return result;
}
private List parseLocations(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy