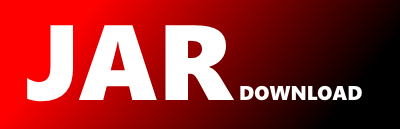
at.newmedialab.ldpath.util.Collections Maven / Gradle / Ivy
package at.newmedialab.ldpath.util;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
/**
* Add file description here!
*
* Author: Sebastian Schaffert
*/
public class Collections {
/**
* Copies all entries of the parsed collections to an new list
* @param lists
* @return
*/
public static List concat(final Collection... lists) {
List result = new ArrayList();
for(Collection list : lists) {
result.addAll(list);
}
return result;
}
/**
* Returns an iterable over all lists without copying any data
* @param lists the array with the lists
* @return the plain iterator over all elements of the lists
*/
public static Iterator iterator(final Collection...lists){
return iterator(0,lists);
}
/**
* Returns an iterable over all lists starting by the parsed offset.
* @param offset the offset of the first entry those elements should be
* included in the returned entries
* @param lists the array with the lists of elements
* @return the plain iterator over all elements of the lists starting from
* index offset
*/
public static Iterator iterator(final int offset,final Collection...lists){
if(offset < 0){
throw new IllegalArgumentException("The parsed Offest MUST NOT be < 0!");
}
if(lists == null){
return null;
} else if( lists.length <= offset){
return java.util.Collections.EMPTY_LIST.iterator();
}
return new Iterator() {
private int listsIndex = offset-1;
private Iterator it;
@Override
public boolean hasNext() {
while(it == null || !it.hasNext()){
listsIndex++;
if(listsIndex < lists.length ){
Collection list = lists[listsIndex];
if(list != null){
it = lists[listsIndex].iterator();
}
} else {
return false;
}
}
return true;
}
@Override
public T next() {
if(it == null){
hasNext();
}
return it.next();
}
@Override
public void remove() {
if(it != null){
it.remove();
}
}};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy