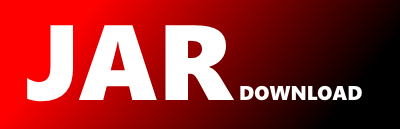
at.newmedialab.ldpath.model.functions.math.MinFunction Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2013 Salzburg Research.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package at.newmedialab.ldpath.model.functions.math;
import at.newmedialab.ldpath.api.backend.RDFBackend;
import at.newmedialab.ldpath.model.Constants;
import at.newmedialab.ldpath.model.transformers.DoubleTransformer;
import java.net.URI;
import java.util.ArrayList;
import java.util.Collection;
public class MinFunction extends MathFunction {
protected final DoubleTransformer doubleTransformer = new DoubleTransformer();
protected final URI doubleType = URI.create(Constants.NS_XSD + "double");
@Override
public Collection apply(RDFBackend backend, Node context, Collection... args)
throws IllegalArgumentException {
ArrayList result = new ArrayList();
for (Collection arg : args) {
Node res = calc(backend, arg);
if (res != null) {
result.add(res);
}
}
return result;
}
protected Node calc(RDFBackend backend, Collection arg) {
/* MIN */
double d = Double.MAX_VALUE;
Node min = null;
for (Node n : arg) {
try {
Double val = doubleTransformer.transform(backend, n);
if (val < d) {
d = val;
min = n;
}
} catch (IllegalArgumentException e) {
// we just ignore non-numeric nodes
}
}
return min;
}
@Override
public String getDescription() {
return "select the min of each argument";
}
@Override
public String getSignature() {
return "fn:min(LiteralList l [, ...]) :: NumberLiteral(s)";
}
@Override
public String getLocalName() {
return "min";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy