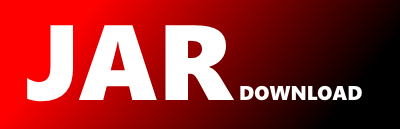
at.willhaben.willtest.proxy.impl.ProxyWrapperImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core classes with as few dependencies as possible
package at.willhaben.willtest.proxy.impl;
import at.willhaben.willtest.proxy.ProxyWrapper;
import net.lightbody.bmp.BrowserMobProxy;
import net.lightbody.bmp.core.har.HarEntry;
import net.lightbody.bmp.core.har.HarPostDataParam;
import net.lightbody.bmp.core.har.HarRequest;
import java.util.List;
import java.util.stream.Collectors;
public class ProxyWrapperImpl implements ProxyWrapper {
private BrowserMobProxy proxy;
public ProxyWrapperImpl(BrowserMobProxy proxy) {
this.proxy = proxy;
}
@Override
public BrowserMobProxy getProxy() {
return proxy;
}
@Override
public List getRequests() {
return getEntries()
.stream()
.map(HarEntry::getRequest)
.collect(Collectors.toList());
}
@Override
public List getRequestsUrls() {
return getRequests().stream()
.map(HarRequest::getUrl)
.collect(Collectors.toList());
}
@Override
public List getRequestsByUrl(String urlPattern) {
return getEntries().stream()
.filter(entry -> entry.getRequest().getUrl().matches(urlPattern))
.collect(Collectors.toList());
}
@Override
public HarEntry getRequestByUrl(String urlPattern) {
List matchedEntries = getRequestsByUrl(urlPattern);
if (matchedEntries.size() == 1) {
return matchedEntries.get(0);
} else if (matchedEntries.size() == 0) {
throw new RuntimeException("No request match the given pattern '" + urlPattern + "'.");
} else {
List matchedUrlList = matchedEntries.stream()
.map(entry -> entry.getRequest().getUrl())
.collect(Collectors.toList());
throw new RuntimeException("Multiple request match the given pattern '" + urlPattern + "'. " + matchedUrlList.toString());
}
}
@Override
public List getRequestFormParamsByUrl(String urlPattern) {
return getRequestByUrl(urlPattern)
.getRequest()
.getPostData()
.getParams();
}
@Override
public void clearRequests() {
proxy.newHar();
}
private List getEntries() {
return proxy.getHar()
.getLog()
.getEntries();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy