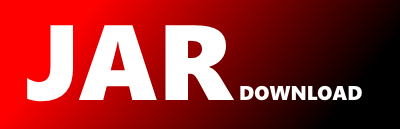
au.com.dius.pact.model.v3.messaging.Message.groovy Maven / Gradle / Ivy
Go to download
Pact model
==========
The model project is responsible for providing:
* a model to represent pacts
* serialization and deserialization
* comparison between two parts of the pact model
* conversion between the pact model and whatever third party libraries used by the pact-consumer and pact-provider requires
You should never need to include this project directly
package au.com.dius.pact.model.v3.messaging
import au.com.dius.pact.model.BasePact
import au.com.dius.pact.model.HttpPart
import au.com.dius.pact.model.Interaction
import au.com.dius.pact.model.OptionalBody
import au.com.dius.pact.model.Response
import groovy.json.JsonOutput
import groovy.json.JsonSlurper
import groovy.transform.Canonical
/**
* Message in a Message Pact
*/
@Canonical
class Message implements Interaction {
private static final String JSON = 'application/json'
String description
String providerState
OptionalBody contents = OptionalBody.missing()
Map> matchingRules = [:]
Map metaData = [:]
byte[] contentsAsBytes() {
if (contents.present) {
contents.value.toString().bytes
} else {
[]
}
}
String getContentType() {
metaData.contentType ?: JSON
}
Map toMap() {
def map = BasePact.convertToMap(this)
if (contents.present) {
if (metaData.contentType == JSON) {
map.contents = new JsonSlurper().parseText(contents.value.toString())
} else {
map.contents = contentsAsBytes().encodeBase64().toString()
}
}
map
}
Message fromMap(Map map) {
description = map.description ?: ''
providerState = map.providerState
if (map.containsKey('contents')) {
if (map.contents == null) {
contents = OptionalBody.nullBody()
} else if (map.contents instanceof String && map.contents.empty) {
contents = OptionalBody.empty()
} else {
contents = OptionalBody.body(JsonOutput.toJson(map.contents))
}
}
matchingRules = map.matchingRules ?: [:]
metaData = map.metaData ?: [:]
this
}
HttpPart asPactRequest() {
new Response(200, ['Content-Type': contentType], contents, matchingRules)
}
@Override
boolean conflictsWith(Interaction other) {
false
}
}