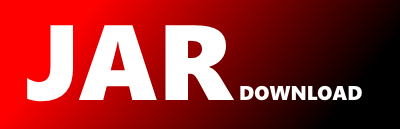
au.com.integradev.delphi.antlr.DelphiParser Maven / Gradle / Ivy
The newest version!
// $ANTLR 3.5.3 au/com/integradev/delphi/antlr/Delphi.g 2024-12-06 07:24:56
/*
* Sonar Delphi Plugin
* Copyright (C) 2010 SonarSource
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package au.com.integradev.delphi.antlr;
import au.com.integradev.delphi.antlr.ast.node.*;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import org.antlr.runtime.tree.*;
@SuppressWarnings({"cast","rawtypes","unchecked"})
@javax.annotation.processing.Generated("ANTLR")
public class DelphiParser extends Parser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "A", "ABSOLUTE", "ABSTRACT", "ADDRESS",
"ALIGN", "AMPERSAND__deprecated", "AND", "ARRAY", "AS", "ASM", "ASSEMBLER",
"ASSEMBLY", "ASSIGN", "AT", "AUTOMATED", "Alpha", "B", "BEGIN", "BinaryDigit",
"BinaryDigitSeq", "C", "CASE", "CDECL", "CLASS", "COLON", "COMMA", "COMMENT",
"CONST", "CONSTRUCTOR", "CONTAINS", "D", "DEFAULT", "DELAYED", "DEPRECATED",
"DEREFERENCE", "DESTRUCTOR", "DISPID", "DISPINTERFACE", "DIV", "DIVIDE",
"DO", "DOT", "DOT_DOT", "DOWNTO", "DYNAMIC", "Digit", "DigitSeq", "E",
"ELSE", "END", "EQUAL", "EXCEPT", "EXPERIMENTAL", "EXPORT", "EXPORTS",
"EXTERNAL", "F", "FAR", "FILE", "FINAL", "FINALIZATION", "FINALLY", "FOR",
"FORWARD", "FUNCTION", "FullWidthNumeral", "G", "GOTO", "GREATER_THAN",
"GREATER_THAN_EQUAL", "H", "HELPER", "HexDigit", "HexDigitSeq", "I", "IF",
"IMPLEMENTATION", "IMPLEMENTS", "IN", "INDEX", "INHERITED", "INITIALIZATION",
"INLINE", "INTERFACE", "IS", "J", "K", "L", "LABEL", "LESS_THAN", "LESS_THAN_EQUAL",
"LIBRARY", "LOCAL", "M", "MESSAGE", "MINUS", "MOD", "MULTIPLY", "N", "NAME",
"NEAR", "NIL", "NODEFAULT", "NOT", "NOT_EQUAL", "O", "OBJECT", "OF", "ON",
"OPERATOR", "OR", "OUT", "OVERLOAD", "OVERRIDE", "P", "PACKAGE", "PACKED",
"PAREN_BRACKET_LEFT", "PAREN_BRACKET_RIGHT", "PAREN_LEFT", "PAREN_RIGHT",
"PASCAL", "PLATFORM", "PLUS", "PRIVATE", "PROCEDURE", "PROGRAM", "PROPERTY",
"PROTECTED", "PUBLIC", "PUBLISHED", "Q", "R", "RAISE", "READ", "READONLY",
"RECORD", "REFERENCE", "REGISTER", "REINTRODUCE", "REPEAT", "REQUIRES",
"RESIDENT", "RESOURCESTRING", "S", "SAFECALL", "SEALED", "SEMICOLON",
"SET", "SHL", "SHR", "SQUARE_BRACKET_LEFT", "SQUARE_BRACKET_RIGHT", "STATIC",
"STDCALL", "STORED", "STRICT", "STRING", "ScaleFactor", "T", "THEN", "THREADVAR",
"TO", "TRY", "TYPE", "TkAnonymousMethod", "TkAnonymousMethodHeading",
"TkAnyChar", "TkArgument", "TkArrayAccessorNode", "TkArrayConstructor",
"TkArrayIndices", "TkAsmDoubleQuotedString", "TkAsmHexNum", "TkAsmId",
"TkAttribute", "TkAttributeGroup", "TkAttributeList", "TkBinaryNumber",
"TkCaseItem", "TkCharacterEscapeCode", "TkClassParents", "TkCompilerDirective",
"TkConstDeclaration", "TkEnumElement", "TkEscapedCharacter", "TkExpressionStatement",
"TkFieldDeclaration", "TkFieldSection", "TkForLoopVar", "TkFormalParameter",
"TkFormalParameterList", "TkGenericArguments", "TkGenericDefinition",
"TkGuid", "TkHexNumber", "TkIdentifier", "TkIntNumber", "TkLabelStatement",
"TkLocalDeclarations", "TkMethodResolveClause", "TkMultilineString", "TkNameDeclaration",
"TkNameDeclarationList", "TkNameReference", "TkNestedExpression", "TkPrimaryExpression",
"TkProcedureType", "TkQuotedString", "TkRealNumber", "TkRecordExpressionItem",
"TkRecordVariantItem", "TkRecordVariantTag", "TkRootNode", "TkRoutineBody",
"TkRoutineDeclaration", "TkRoutineHeading", "TkRoutineImplementation",
"TkRoutineName", "TkRoutineParameters", "TkRoutineReturn", "TkStatementList",
"TkTextLiteral", "TkTypeDeclaration", "TkTypeParameter", "TkTypeReference",
"TkUnitImport", "TkVarDeclaration", "TkVisibility", "TkVisibilitySection",
"TkWeakAlias", "U", "UNIT", "UNSAFE", "UNTIL", "USES", "V", "VAR", "VARARGS",
"VIRTUAL", "W", "WHILE", "WHITESPACE", "WINAPI", "WITH", "WRITE", "WRITEONLY",
"X", "XOR", "Y", "Z"
};
public static final int EOF=-1;
public static final int A=4;
public static final int ABSOLUTE=5;
public static final int ABSTRACT=6;
public static final int ADDRESS=7;
public static final int ALIGN=8;
public static final int AMPERSAND__deprecated=9;
public static final int AND=10;
public static final int ARRAY=11;
public static final int AS=12;
public static final int ASM=13;
public static final int ASSEMBLER=14;
public static final int ASSEMBLY=15;
public static final int ASSIGN=16;
public static final int AT=17;
public static final int AUTOMATED=18;
public static final int Alpha=19;
public static final int B=20;
public static final int BEGIN=21;
public static final int BinaryDigit=22;
public static final int BinaryDigitSeq=23;
public static final int C=24;
public static final int CASE=25;
public static final int CDECL=26;
public static final int CLASS=27;
public static final int COLON=28;
public static final int COMMA=29;
public static final int COMMENT=30;
public static final int CONST=31;
public static final int CONSTRUCTOR=32;
public static final int CONTAINS=33;
public static final int D=34;
public static final int DEFAULT=35;
public static final int DELAYED=36;
public static final int DEPRECATED=37;
public static final int DEREFERENCE=38;
public static final int DESTRUCTOR=39;
public static final int DISPID=40;
public static final int DISPINTERFACE=41;
public static final int DIV=42;
public static final int DIVIDE=43;
public static final int DO=44;
public static final int DOT=45;
public static final int DOT_DOT=46;
public static final int DOWNTO=47;
public static final int DYNAMIC=48;
public static final int Digit=49;
public static final int DigitSeq=50;
public static final int E=51;
public static final int ELSE=52;
public static final int END=53;
public static final int EQUAL=54;
public static final int EXCEPT=55;
public static final int EXPERIMENTAL=56;
public static final int EXPORT=57;
public static final int EXPORTS=58;
public static final int EXTERNAL=59;
public static final int F=60;
public static final int FAR=61;
public static final int FILE=62;
public static final int FINAL=63;
public static final int FINALIZATION=64;
public static final int FINALLY=65;
public static final int FOR=66;
public static final int FORWARD=67;
public static final int FUNCTION=68;
public static final int FullWidthNumeral=69;
public static final int G=70;
public static final int GOTO=71;
public static final int GREATER_THAN=72;
public static final int GREATER_THAN_EQUAL=73;
public static final int H=74;
public static final int HELPER=75;
public static final int HexDigit=76;
public static final int HexDigitSeq=77;
public static final int I=78;
public static final int IF=79;
public static final int IMPLEMENTATION=80;
public static final int IMPLEMENTS=81;
public static final int IN=82;
public static final int INDEX=83;
public static final int INHERITED=84;
public static final int INITIALIZATION=85;
public static final int INLINE=86;
public static final int INTERFACE=87;
public static final int IS=88;
public static final int J=89;
public static final int K=90;
public static final int L=91;
public static final int LABEL=92;
public static final int LESS_THAN=93;
public static final int LESS_THAN_EQUAL=94;
public static final int LIBRARY=95;
public static final int LOCAL=96;
public static final int M=97;
public static final int MESSAGE=98;
public static final int MINUS=99;
public static final int MOD=100;
public static final int MULTIPLY=101;
public static final int N=102;
public static final int NAME=103;
public static final int NEAR=104;
public static final int NIL=105;
public static final int NODEFAULT=106;
public static final int NOT=107;
public static final int NOT_EQUAL=108;
public static final int O=109;
public static final int OBJECT=110;
public static final int OF=111;
public static final int ON=112;
public static final int OPERATOR=113;
public static final int OR=114;
public static final int OUT=115;
public static final int OVERLOAD=116;
public static final int OVERRIDE=117;
public static final int P=118;
public static final int PACKAGE=119;
public static final int PACKED=120;
public static final int PAREN_BRACKET_LEFT=121;
public static final int PAREN_BRACKET_RIGHT=122;
public static final int PAREN_LEFT=123;
public static final int PAREN_RIGHT=124;
public static final int PASCAL=125;
public static final int PLATFORM=126;
public static final int PLUS=127;
public static final int PRIVATE=128;
public static final int PROCEDURE=129;
public static final int PROGRAM=130;
public static final int PROPERTY=131;
public static final int PROTECTED=132;
public static final int PUBLIC=133;
public static final int PUBLISHED=134;
public static final int Q=135;
public static final int R=136;
public static final int RAISE=137;
public static final int READ=138;
public static final int READONLY=139;
public static final int RECORD=140;
public static final int REFERENCE=141;
public static final int REGISTER=142;
public static final int REINTRODUCE=143;
public static final int REPEAT=144;
public static final int REQUIRES=145;
public static final int RESIDENT=146;
public static final int RESOURCESTRING=147;
public static final int S=148;
public static final int SAFECALL=149;
public static final int SEALED=150;
public static final int SEMICOLON=151;
public static final int SET=152;
public static final int SHL=153;
public static final int SHR=154;
public static final int SQUARE_BRACKET_LEFT=155;
public static final int SQUARE_BRACKET_RIGHT=156;
public static final int STATIC=157;
public static final int STDCALL=158;
public static final int STORED=159;
public static final int STRICT=160;
public static final int STRING=161;
public static final int ScaleFactor=162;
public static final int T=163;
public static final int THEN=164;
public static final int THREADVAR=165;
public static final int TO=166;
public static final int TRY=167;
public static final int TYPE=168;
public static final int TkAnonymousMethod=169;
public static final int TkAnonymousMethodHeading=170;
public static final int TkAnyChar=171;
public static final int TkArgument=172;
public static final int TkArrayAccessorNode=173;
public static final int TkArrayConstructor=174;
public static final int TkArrayIndices=175;
public static final int TkAsmDoubleQuotedString=176;
public static final int TkAsmHexNum=177;
public static final int TkAsmId=178;
public static final int TkAttribute=179;
public static final int TkAttributeGroup=180;
public static final int TkAttributeList=181;
public static final int TkBinaryNumber=182;
public static final int TkCaseItem=183;
public static final int TkCharacterEscapeCode=184;
public static final int TkClassParents=185;
public static final int TkCompilerDirective=186;
public static final int TkConstDeclaration=187;
public static final int TkEnumElement=188;
public static final int TkEscapedCharacter=189;
public static final int TkExpressionStatement=190;
public static final int TkFieldDeclaration=191;
public static final int TkFieldSection=192;
public static final int TkForLoopVar=193;
public static final int TkFormalParameter=194;
public static final int TkFormalParameterList=195;
public static final int TkGenericArguments=196;
public static final int TkGenericDefinition=197;
public static final int TkGuid=198;
public static final int TkHexNumber=199;
public static final int TkIdentifier=200;
public static final int TkIntNumber=201;
public static final int TkLabelStatement=202;
public static final int TkLocalDeclarations=203;
public static final int TkMethodResolveClause=204;
public static final int TkMultilineString=205;
public static final int TkNameDeclaration=206;
public static final int TkNameDeclarationList=207;
public static final int TkNameReference=208;
public static final int TkNestedExpression=209;
public static final int TkPrimaryExpression=210;
public static final int TkProcedureType=211;
public static final int TkQuotedString=212;
public static final int TkRealNumber=213;
public static final int TkRecordExpressionItem=214;
public static final int TkRecordVariantItem=215;
public static final int TkRecordVariantTag=216;
public static final int TkRootNode=217;
public static final int TkRoutineBody=218;
public static final int TkRoutineDeclaration=219;
public static final int TkRoutineHeading=220;
public static final int TkRoutineImplementation=221;
public static final int TkRoutineName=222;
public static final int TkRoutineParameters=223;
public static final int TkRoutineReturn=224;
public static final int TkStatementList=225;
public static final int TkTextLiteral=226;
public static final int TkTypeDeclaration=227;
public static final int TkTypeParameter=228;
public static final int TkTypeReference=229;
public static final int TkUnitImport=230;
public static final int TkVarDeclaration=231;
public static final int TkVisibility=232;
public static final int TkVisibilitySection=233;
public static final int TkWeakAlias=234;
public static final int U=235;
public static final int UNIT=236;
public static final int UNSAFE=237;
public static final int UNTIL=238;
public static final int USES=239;
public static final int V=240;
public static final int VAR=241;
public static final int VARARGS=242;
public static final int VIRTUAL=243;
public static final int W=244;
public static final int WHILE=245;
public static final int WHITESPACE=246;
public static final int WINAPI=247;
public static final int WITH=248;
public static final int WRITE=249;
public static final int WRITEONLY=250;
public static final int X=251;
public static final int XOR=252;
public static final int Y=253;
public static final int Z=254;
// delegates
public Parser[] getDelegates() {
return new Parser[] {};
}
// delegators
public DelphiParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public DelphiParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
this.state.ruleMemo = new HashMap[872+1];
}
protected TreeAdaptor adaptor = new CommonTreeAdaptor();
public void setTreeAdaptor(TreeAdaptor adaptor) {
this.adaptor = adaptor;
}
public TreeAdaptor getTreeAdaptor() {
return adaptor;
}
@Override public String[] getTokenNames() { return DelphiParser.tokenNames; }
@Override public String getGrammarFileName() { return "au/com/integradev/delphi/antlr/Delphi.g"; }
private Token changeTokenType(int type) {
return changeTokenType(type, -1);
}
private Token changeTokenType(int type, int offset) {
CommonToken t = new CommonToken(input.LT(offset));
t.setType(type);
return t;
}
private Token combineLastNTokens(int count) {
CommonToken firstToken = (CommonToken) input.LT(-count);
CommonToken lastToken = (CommonToken) input.LT(-1);
lastToken.setStartIndex(firstToken.getStartIndex());
return lastToken;
}
@Override
public void reportError(RecognitionException e) {
String hdr = this.getErrorHeader(e);
String msg = this.getErrorMessage(e, this.getTokenNames());
throw new ParserException(hdr + " " + msg, e);
}
@Override
public String getErrorHeader(RecognitionException e) {
return "line " + e.line + ":" + e.charPositionInLine;
}
private void resetBinaryExpressionTokens(Object rootExpression) {
if (!(rootExpression instanceof BinaryExpressionNodeImpl)) {
return;
}
var binaryExpression = (BinaryExpressionNodeImpl) rootExpression;
binaryExpression.setFirstToken(null);
binaryExpression.setLastToken(null);
resetBinaryExpressionTokens(binaryExpression.getLeft());
resetBinaryExpressionTokens(binaryExpression.getRight());
}
public static class ParserException extends RuntimeException {
public ParserException(String message, Throwable cause) {
super(message, cause);
}
}
public static class file_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "file"
// au/com/integradev/delphi/antlr/Delphi.g:374:1: file : ( program | library | unit | package_ );
public final DelphiParser.file_return file() throws RecognitionException {
DelphiParser.file_return retval = new DelphiParser.file_return();
retval.start = input.LT(1);
int file_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope program1 =null;
ParserRuleReturnScope library2 =null;
ParserRuleReturnScope unit3 =null;
ParserRuleReturnScope package_4 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 1) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:374:30: ( program | library | unit | package_ )
int alt1=4;
switch ( input.LA(1) ) {
case BEGIN:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case END:
case EXPORTS:
case FUNCTION:
case LABEL:
case OPERATOR:
case PAREN_BRACKET_LEFT:
case PROCEDURE:
case PROGRAM:
case RESOURCESTRING:
case SQUARE_BRACKET_LEFT:
case THREADVAR:
case TYPE:
case USES:
case VAR:
{
alt1=1;
}
break;
case LIBRARY:
{
alt1=2;
}
break;
case UNIT:
{
alt1=3;
}
break;
case PACKAGE:
{
alt1=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 1, 0, input);
throw nvae;
}
switch (alt1) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:374:32: program
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_program_in_file405);
program1=program();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, program1.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:374:42: library
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_library_in_file409);
library2=library();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, library2.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:374:52: unit
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unit_in_file413);
unit3=unit();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unit3.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:374:59: package_
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_package__in_file417);
package_4=package_();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, package_4.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 1, file_StartIndex); }
}
return retval;
}
// $ANTLR end "file"
public static class fileWithoutImplementation_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fileWithoutImplementation"
// au/com/integradev/delphi/antlr/Delphi.g:376:1: fileWithoutImplementation : ( program | library | unitWithoutImplementation | package_ );
public final DelphiParser.fileWithoutImplementation_return fileWithoutImplementation() throws RecognitionException {
DelphiParser.fileWithoutImplementation_return retval = new DelphiParser.fileWithoutImplementation_return();
retval.start = input.LT(1);
int fileWithoutImplementation_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope program5 =null;
ParserRuleReturnScope library6 =null;
ParserRuleReturnScope unitWithoutImplementation7 =null;
ParserRuleReturnScope package_8 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 2) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:376:30: ( program | library | unitWithoutImplementation | package_ )
int alt2=4;
switch ( input.LA(1) ) {
case BEGIN:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case END:
case EXPORTS:
case FUNCTION:
case LABEL:
case OPERATOR:
case PAREN_BRACKET_LEFT:
case PROCEDURE:
case PROGRAM:
case RESOURCESTRING:
case SQUARE_BRACKET_LEFT:
case THREADVAR:
case TYPE:
case USES:
case VAR:
{
alt2=1;
}
break;
case LIBRARY:
{
alt2=2;
}
break;
case UNIT:
{
alt2=3;
}
break;
case PACKAGE:
{
alt2=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:376:32: program
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_program_in_fileWithoutImplementation457);
program5=program();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, program5.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:376:42: library
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_library_in_fileWithoutImplementation461);
library6=library();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, library6.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:376:52: unitWithoutImplementation
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unitWithoutImplementation_in_fileWithoutImplementation465);
unitWithoutImplementation7=unitWithoutImplementation();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitWithoutImplementation7.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:376:80: package_
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_package__in_fileWithoutImplementation469);
package_8=package_();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, package_8.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 2, fileWithoutImplementation_StartIndex); }
}
return retval;
}
// $ANTLR end "fileWithoutImplementation"
public static class program_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "program"
// au/com/integradev/delphi/antlr/Delphi.g:383:1: program : ( programHead )? ( usesFileClause )? programBody '.' ;
public final DelphiParser.program_return program() throws RecognitionException {
DelphiParser.program_return retval = new DelphiParser.program_return();
retval.start = input.LT(1);
int program_StartIndex = input.index();
Object root_0 = null;
Token char_literal12=null;
ParserRuleReturnScope programHead9 =null;
ParserRuleReturnScope usesFileClause10 =null;
ParserRuleReturnScope programBody11 =null;
Object char_literal12_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 3) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:383:30: ( ( programHead )? ( usesFileClause )? programBody '.' )
// au/com/integradev/delphi/antlr/Delphi.g:383:32: ( programHead )? ( usesFileClause )? programBody '.'
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:383:32: ( programHead )?
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==PROGRAM) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:383:32: programHead
{
pushFollow(FOLLOW_programHead_in_program532);
programHead9=programHead();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, programHead9.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:383:45: ( usesFileClause )?
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0==USES) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:383:45: usesFileClause
{
pushFollow(FOLLOW_usesFileClause_in_program535);
usesFileClause10=usesFileClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, usesFileClause10.getTree());
}
break;
}
pushFollow(FOLLOW_programBody_in_program538);
programBody11=programBody();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, programBody11.getTree());
char_literal12=(Token)match(input,DOT,FOLLOW_DOT_in_program540); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal12_tree = (Object)adaptor.create(char_literal12);
adaptor.addChild(root_0, char_literal12_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 3, program_StartIndex); }
}
return retval;
}
// $ANTLR end "program"
public static class programHead_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "programHead"
// au/com/integradev/delphi/antlr/Delphi.g:385:1: programHead : PROGRAM ^ qualifiedNameDeclaration ( programParameters )? ';' !;
public final DelphiParser.programHead_return programHead() throws RecognitionException {
DelphiParser.programHead_return retval = new DelphiParser.programHead_return();
retval.start = input.LT(1);
int programHead_StartIndex = input.index();
Object root_0 = null;
Token PROGRAM13=null;
Token char_literal16=null;
ParserRuleReturnScope qualifiedNameDeclaration14 =null;
ParserRuleReturnScope programParameters15 =null;
Object PROGRAM13_tree=null;
Object char_literal16_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 4) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:385:30: ( PROGRAM ^ qualifiedNameDeclaration ( programParameters )? ';' !)
// au/com/integradev/delphi/antlr/Delphi.g:385:32: PROGRAM ^ qualifiedNameDeclaration ( programParameters )? ';' !
{
root_0 = (Object)adaptor.nil();
PROGRAM13=(Token)match(input,PROGRAM,FOLLOW_PROGRAM_in_programHead594); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PROGRAM13_tree = new ProgramDeclarationNodeImpl(PROGRAM13) ;
root_0 = (Object)adaptor.becomeRoot(PROGRAM13_tree, root_0);
}
pushFollow(FOLLOW_qualifiedNameDeclaration_in_programHead600);
qualifiedNameDeclaration14=qualifiedNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, qualifiedNameDeclaration14.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:385:94: ( programParameters )?
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0==PAREN_LEFT) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:385:94: programParameters
{
pushFollow(FOLLOW_programParameters_in_programHead602);
programParameters15=programParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, programParameters15.getTree());
}
break;
}
char_literal16=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_programHead605); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 4, programHead_StartIndex); }
}
return retval;
}
// $ANTLR end "programHead"
public static class programParameters_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "programParameters"
// au/com/integradev/delphi/antlr/Delphi.g:387:1: programParameters : '(' ( ident ( ',' ident )* )? ')' ;
public final DelphiParser.programParameters_return programParameters() throws RecognitionException {
DelphiParser.programParameters_return retval = new DelphiParser.programParameters_return();
retval.start = input.LT(1);
int programParameters_StartIndex = input.index();
Object root_0 = null;
Token char_literal17=null;
Token char_literal19=null;
Token char_literal21=null;
ParserRuleReturnScope ident18 =null;
ParserRuleReturnScope ident20 =null;
Object char_literal17_tree=null;
Object char_literal19_tree=null;
Object char_literal21_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 5) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:387:30: ( '(' ( ident ( ',' ident )* )? ')' )
// au/com/integradev/delphi/antlr/Delphi.g:387:32: '(' ( ident ( ',' ident )* )? ')'
{
root_0 = (Object)adaptor.nil();
char_literal17=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_programParameters654); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal17_tree = (Object)adaptor.create(char_literal17);
adaptor.addChild(root_0, char_literal17_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:387:36: ( ident ( ',' ident )* )?
int alt7=2;
int LA7_0 = input.LA(1);
if ( ((LA7_0 >= ABSOLUTE && LA7_0 <= ABSTRACT)||LA7_0==ALIGN||(LA7_0 >= ASSEMBLER && LA7_0 <= ASSEMBLY)||(LA7_0 >= AT && LA7_0 <= AUTOMATED)||LA7_0==CDECL||LA7_0==CONTAINS||(LA7_0 >= DEFAULT && LA7_0 <= DEPRECATED)||LA7_0==DISPID||LA7_0==DYNAMIC||(LA7_0 >= EXPERIMENTAL && LA7_0 <= EXPORT)||LA7_0==EXTERNAL||LA7_0==FAR||LA7_0==FINAL||LA7_0==FORWARD||LA7_0==HELPER||LA7_0==IMPLEMENTS||LA7_0==INDEX||LA7_0==LABEL||LA7_0==LOCAL||LA7_0==MESSAGE||(LA7_0 >= NAME && LA7_0 <= NEAR)||LA7_0==NODEFAULT||(LA7_0 >= ON && LA7_0 <= OPERATOR)||(LA7_0 >= OUT && LA7_0 <= OVERRIDE)||LA7_0==PACKAGE||(LA7_0 >= PASCAL && LA7_0 <= PLATFORM)||LA7_0==PRIVATE||(LA7_0 >= PROTECTED && LA7_0 <= PUBLISHED)||(LA7_0 >= READ && LA7_0 <= READONLY)||(LA7_0 >= REFERENCE && LA7_0 <= REINTRODUCE)||(LA7_0 >= REQUIRES && LA7_0 <= RESIDENT)||(LA7_0 >= SAFECALL && LA7_0 <= SEALED)||(LA7_0 >= STATIC && LA7_0 <= STRICT)||LA7_0==TkIdentifier||LA7_0==UNSAFE||(LA7_0 >= VARARGS && LA7_0 <= VIRTUAL)||LA7_0==WINAPI||(LA7_0 >= WRITE && LA7_0 <= WRITEONLY)) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:387:37: ident ( ',' ident )*
{
pushFollow(FOLLOW_ident_in_programParameters657);
ident18=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident18.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:387:43: ( ',' ident )*
loop6:
while (true) {
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==COMMA) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:387:44: ',' ident
{
char_literal19=(Token)match(input,COMMA,FOLLOW_COMMA_in_programParameters660); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal19_tree = (Object)adaptor.create(char_literal19);
adaptor.addChild(root_0, char_literal19_tree);
}
pushFollow(FOLLOW_ident_in_programParameters662);
ident20=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident20.getTree());
}
break;
default :
break loop6;
}
}
}
break;
}
char_literal21=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_programParameters669); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal21_tree = (Object)adaptor.create(char_literal21);
adaptor.addChild(root_0, char_literal21_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 5, programParameters_StartIndex); }
}
return retval;
}
// $ANTLR end "programParameters"
public static class programBody_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "programBody"
// au/com/integradev/delphi/antlr/Delphi.g:389:1: programBody : ( localDeclSection )? ( compoundStatement | END ) ;
public final DelphiParser.programBody_return programBody() throws RecognitionException {
DelphiParser.programBody_return retval = new DelphiParser.programBody_return();
retval.start = input.LT(1);
int programBody_StartIndex = input.index();
Object root_0 = null;
Token END24=null;
ParserRuleReturnScope localDeclSection22 =null;
ParserRuleReturnScope compoundStatement23 =null;
Object END24_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 6) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:389:30: ( ( localDeclSection )? ( compoundStatement | END ) )
// au/com/integradev/delphi/antlr/Delphi.g:389:32: ( localDeclSection )? ( compoundStatement | END )
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:389:32: ( localDeclSection )?
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==CLASS||(LA8_0 >= CONST && LA8_0 <= CONSTRUCTOR)||LA8_0==DESTRUCTOR||LA8_0==EXPORTS||LA8_0==FUNCTION||LA8_0==LABEL||LA8_0==OPERATOR||LA8_0==PAREN_BRACKET_LEFT||LA8_0==PROCEDURE||LA8_0==RESOURCESTRING||LA8_0==SQUARE_BRACKET_LEFT||LA8_0==THREADVAR||LA8_0==TYPE||LA8_0==VAR) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:389:32: localDeclSection
{
pushFollow(FOLLOW_localDeclSection_in_programBody724);
localDeclSection22=localDeclSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, localDeclSection22.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:389:50: ( compoundStatement | END )
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0==BEGIN) ) {
alt9=1;
}
else if ( (LA9_0==END) ) {
alt9=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:389:51: compoundStatement
{
pushFollow(FOLLOW_compoundStatement_in_programBody728);
compoundStatement23=compoundStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, compoundStatement23.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:389:71: END
{
END24=(Token)match(input,END,FOLLOW_END_in_programBody732); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END24_tree = (Object)adaptor.create(END24);
adaptor.addChild(root_0, END24_tree);
}
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 6, programBody_StartIndex); }
}
return retval;
}
// $ANTLR end "programBody"
public static class library_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "library"
// au/com/integradev/delphi/antlr/Delphi.g:391:1: library : libraryHead ( usesFileClause )? programBody '.' ;
public final DelphiParser.library_return library() throws RecognitionException {
DelphiParser.library_return retval = new DelphiParser.library_return();
retval.start = input.LT(1);
int library_StartIndex = input.index();
Object root_0 = null;
Token char_literal28=null;
ParserRuleReturnScope libraryHead25 =null;
ParserRuleReturnScope usesFileClause26 =null;
ParserRuleReturnScope programBody27 =null;
Object char_literal28_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 7) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:391:30: ( libraryHead ( usesFileClause )? programBody '.' )
// au/com/integradev/delphi/antlr/Delphi.g:391:32: libraryHead ( usesFileClause )? programBody '.'
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_libraryHead_in_library791);
libraryHead25=libraryHead();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, libraryHead25.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:391:44: ( usesFileClause )?
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==USES) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:391:44: usesFileClause
{
pushFollow(FOLLOW_usesFileClause_in_library793);
usesFileClause26=usesFileClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, usesFileClause26.getTree());
}
break;
}
pushFollow(FOLLOW_programBody_in_library796);
programBody27=programBody();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, programBody27.getTree());
char_literal28=(Token)match(input,DOT,FOLLOW_DOT_in_library798); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal28_tree = (Object)adaptor.create(char_literal28);
adaptor.addChild(root_0, char_literal28_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 7, library_StartIndex); }
}
return retval;
}
// $ANTLR end "library"
public static class libraryHead_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "libraryHead"
// au/com/integradev/delphi/antlr/Delphi.g:393:1: libraryHead : LIBRARY ^ qualifiedNameDeclaration ( portabilityDirective !)* ';' !;
public final DelphiParser.libraryHead_return libraryHead() throws RecognitionException {
DelphiParser.libraryHead_return retval = new DelphiParser.libraryHead_return();
retval.start = input.LT(1);
int libraryHead_StartIndex = input.index();
Object root_0 = null;
Token LIBRARY29=null;
Token char_literal32=null;
ParserRuleReturnScope qualifiedNameDeclaration30 =null;
ParserRuleReturnScope portabilityDirective31 =null;
Object LIBRARY29_tree=null;
Object char_literal32_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 8) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:393:30: ( LIBRARY ^ qualifiedNameDeclaration ( portabilityDirective !)* ';' !)
// au/com/integradev/delphi/antlr/Delphi.g:393:32: LIBRARY ^ qualifiedNameDeclaration ( portabilityDirective !)* ';' !
{
root_0 = (Object)adaptor.nil();
LIBRARY29=(Token)match(input,LIBRARY,FOLLOW_LIBRARY_in_libraryHead852); if (state.failed) return retval;
if ( state.backtracking==0 ) {
LIBRARY29_tree = new LibraryDeclarationNodeImpl(LIBRARY29) ;
root_0 = (Object)adaptor.becomeRoot(LIBRARY29_tree, root_0);
}
pushFollow(FOLLOW_qualifiedNameDeclaration_in_libraryHead858);
qualifiedNameDeclaration30=qualifiedNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, qualifiedNameDeclaration30.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:393:94: ( portabilityDirective !)*
loop11:
while (true) {
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0==DEPRECATED||LA11_0==EXPERIMENTAL||LA11_0==LIBRARY||LA11_0==PLATFORM) ) {
alt11=1;
}
switch (alt11) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:393:95: portabilityDirective !
{
pushFollow(FOLLOW_portabilityDirective_in_libraryHead861);
portabilityDirective31=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
}
break;
default :
break loop11;
}
}
char_literal32=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_libraryHead866); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 8, libraryHead_StartIndex); }
}
return retval;
}
// $ANTLR end "libraryHead"
public static class package__return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "package_"
// au/com/integradev/delphi/antlr/Delphi.g:395:1: package_ : packageHead ( requiresClause )? ( containsClause )? ( attributeList )* END '.' ;
public final DelphiParser.package__return package_() throws RecognitionException {
DelphiParser.package__return retval = new DelphiParser.package__return();
retval.start = input.LT(1);
int package__StartIndex = input.index();
Object root_0 = null;
Token END37=null;
Token char_literal38=null;
ParserRuleReturnScope packageHead33 =null;
ParserRuleReturnScope requiresClause34 =null;
ParserRuleReturnScope containsClause35 =null;
ParserRuleReturnScope attributeList36 =null;
Object END37_tree=null;
Object char_literal38_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 9) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:395:30: ( packageHead ( requiresClause )? ( containsClause )? ( attributeList )* END '.' )
// au/com/integradev/delphi/antlr/Delphi.g:395:32: packageHead ( requiresClause )? ( containsClause )? ( attributeList )* END '.'
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_packageHead_in_package_924);
packageHead33=packageHead();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, packageHead33.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:395:44: ( requiresClause )?
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==REQUIRES) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:395:44: requiresClause
{
pushFollow(FOLLOW_requiresClause_in_package_926);
requiresClause34=requiresClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, requiresClause34.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:395:60: ( containsClause )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==CONTAINS) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:395:60: containsClause
{
pushFollow(FOLLOW_containsClause_in_package_929);
containsClause35=containsClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, containsClause35.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:395:76: ( attributeList )*
loop14:
while (true) {
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0==PAREN_BRACKET_LEFT||LA14_0==SQUARE_BRACKET_LEFT) ) {
alt14=1;
}
switch (alt14) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:395:76: attributeList
{
pushFollow(FOLLOW_attributeList_in_package_932);
attributeList36=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, attributeList36.getTree());
}
break;
default :
break loop14;
}
}
END37=(Token)match(input,END,FOLLOW_END_in_package_935); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END37_tree = (Object)adaptor.create(END37);
adaptor.addChild(root_0, END37_tree);
}
char_literal38=(Token)match(input,DOT,FOLLOW_DOT_in_package_937); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal38_tree = (Object)adaptor.create(char_literal38);
adaptor.addChild(root_0, char_literal38_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 9, package__StartIndex); }
}
return retval;
}
// $ANTLR end "package_"
public static class packageHead_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "packageHead"
// au/com/integradev/delphi/antlr/Delphi.g:397:1: packageHead : PACKAGE ^ qualifiedNameDeclaration ';' !;
public final DelphiParser.packageHead_return packageHead() throws RecognitionException {
DelphiParser.packageHead_return retval = new DelphiParser.packageHead_return();
retval.start = input.LT(1);
int packageHead_StartIndex = input.index();
Object root_0 = null;
Token PACKAGE39=null;
Token char_literal41=null;
ParserRuleReturnScope qualifiedNameDeclaration40 =null;
Object PACKAGE39_tree=null;
Object char_literal41_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 10) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:397:30: ( PACKAGE ^ qualifiedNameDeclaration ';' !)
// au/com/integradev/delphi/antlr/Delphi.g:397:32: PACKAGE ^ qualifiedNameDeclaration ';' !
{
root_0 = (Object)adaptor.nil();
PACKAGE39=(Token)match(input,PACKAGE,FOLLOW_PACKAGE_in_packageHead991); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PACKAGE39_tree = new PackageDeclarationNodeImpl(PACKAGE39) ;
root_0 = (Object)adaptor.becomeRoot(PACKAGE39_tree, root_0);
}
pushFollow(FOLLOW_qualifiedNameDeclaration_in_packageHead997);
qualifiedNameDeclaration40=qualifiedNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, qualifiedNameDeclaration40.getTree());
char_literal41=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_packageHead999); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 10, packageHead_StartIndex); }
}
return retval;
}
// $ANTLR end "packageHead"
public static class unit_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unit"
// au/com/integradev/delphi/antlr/Delphi.g:399:1: unit : unitHead unitInterface unitImplementation unitBlock '.' ;
public final DelphiParser.unit_return unit() throws RecognitionException {
DelphiParser.unit_return retval = new DelphiParser.unit_return();
retval.start = input.LT(1);
int unit_StartIndex = input.index();
Object root_0 = null;
Token char_literal46=null;
ParserRuleReturnScope unitHead42 =null;
ParserRuleReturnScope unitInterface43 =null;
ParserRuleReturnScope unitImplementation44 =null;
ParserRuleReturnScope unitBlock45 =null;
Object char_literal46_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 11) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:399:30: ( unitHead unitInterface unitImplementation unitBlock '.' )
// au/com/integradev/delphi/antlr/Delphi.g:399:32: unitHead unitInterface unitImplementation unitBlock '.'
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unitHead_in_unit1061);
unitHead42=unitHead();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitHead42.getTree());
pushFollow(FOLLOW_unitInterface_in_unit1063);
unitInterface43=unitInterface();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitInterface43.getTree());
pushFollow(FOLLOW_unitImplementation_in_unit1065);
unitImplementation44=unitImplementation();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitImplementation44.getTree());
pushFollow(FOLLOW_unitBlock_in_unit1067);
unitBlock45=unitBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitBlock45.getTree());
char_literal46=(Token)match(input,DOT,FOLLOW_DOT_in_unit1069); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal46_tree = (Object)adaptor.create(char_literal46);
adaptor.addChild(root_0, char_literal46_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 11, unit_StartIndex); }
}
return retval;
}
// $ANTLR end "unit"
public static class unitWithoutImplementation_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitWithoutImplementation"
// au/com/integradev/delphi/antlr/Delphi.g:401:1: unitWithoutImplementation : unitHead unitInterface ;
public final DelphiParser.unitWithoutImplementation_return unitWithoutImplementation() throws RecognitionException {
DelphiParser.unitWithoutImplementation_return retval = new DelphiParser.unitWithoutImplementation_return();
retval.start = input.LT(1);
int unitWithoutImplementation_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope unitHead47 =null;
ParserRuleReturnScope unitInterface48 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 12) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:401:30: ( unitHead unitInterface )
// au/com/integradev/delphi/antlr/Delphi.g:401:32: unitHead unitInterface
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unitHead_in_unitWithoutImplementation1109);
unitHead47=unitHead();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitHead47.getTree());
pushFollow(FOLLOW_unitInterface_in_unitWithoutImplementation1111);
unitInterface48=unitInterface();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitInterface48.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 12, unitWithoutImplementation_StartIndex); }
}
return retval;
}
// $ANTLR end "unitWithoutImplementation"
public static class unitHead_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitHead"
// au/com/integradev/delphi/antlr/Delphi.g:403:1: unitHead : UNIT ^ qualifiedNameDeclaration ( portabilityDirective )* ';' !;
public final DelphiParser.unitHead_return unitHead() throws RecognitionException {
DelphiParser.unitHead_return retval = new DelphiParser.unitHead_return();
retval.start = input.LT(1);
int unitHead_StartIndex = input.index();
Object root_0 = null;
Token UNIT49=null;
Token char_literal52=null;
ParserRuleReturnScope qualifiedNameDeclaration50 =null;
ParserRuleReturnScope portabilityDirective51 =null;
Object UNIT49_tree=null;
Object char_literal52_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 13) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:403:30: ( UNIT ^ qualifiedNameDeclaration ( portabilityDirective )* ';' !)
// au/com/integradev/delphi/antlr/Delphi.g:403:32: UNIT ^ qualifiedNameDeclaration ( portabilityDirective )* ';' !
{
root_0 = (Object)adaptor.nil();
UNIT49=(Token)match(input,UNIT,FOLLOW_UNIT_in_unitHead1168); if (state.failed) return retval;
if ( state.backtracking==0 ) {
UNIT49_tree = new UnitDeclarationNodeImpl(UNIT49) ;
root_0 = (Object)adaptor.becomeRoot(UNIT49_tree, root_0);
}
pushFollow(FOLLOW_qualifiedNameDeclaration_in_unitHead1174);
qualifiedNameDeclaration50=qualifiedNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, qualifiedNameDeclaration50.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:403:88: ( portabilityDirective )*
loop15:
while (true) {
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0==DEPRECATED||LA15_0==EXPERIMENTAL||LA15_0==LIBRARY||LA15_0==PLATFORM) ) {
alt15=1;
}
switch (alt15) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:403:88: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_unitHead1176);
portabilityDirective51=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, portabilityDirective51.getTree());
}
break;
default :
break loop15;
}
}
char_literal52=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_unitHead1179); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 13, unitHead_StartIndex); }
}
return retval;
}
// $ANTLR end "unitHead"
public static class unitInterface_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitInterface"
// au/com/integradev/delphi/antlr/Delphi.g:405:1: unitInterface : INTERFACE ^ ( usesClause )? ( interfaceDecl )* ;
public final DelphiParser.unitInterface_return unitInterface() throws RecognitionException {
DelphiParser.unitInterface_return retval = new DelphiParser.unitInterface_return();
retval.start = input.LT(1);
int unitInterface_StartIndex = input.index();
Object root_0 = null;
Token INTERFACE53=null;
ParserRuleReturnScope usesClause54 =null;
ParserRuleReturnScope interfaceDecl55 =null;
Object INTERFACE53_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 14) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:405:30: ( INTERFACE ^ ( usesClause )? ( interfaceDecl )* )
// au/com/integradev/delphi/antlr/Delphi.g:405:32: INTERFACE ^ ( usesClause )? ( interfaceDecl )*
{
root_0 = (Object)adaptor.nil();
INTERFACE53=(Token)match(input,INTERFACE,FOLLOW_INTERFACE_in_unitInterface1232); if (state.failed) return retval;
if ( state.backtracking==0 ) {
INTERFACE53_tree = new InterfaceSectionNodeImpl(INTERFACE53) ;
root_0 = (Object)adaptor.becomeRoot(INTERFACE53_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:405:69: ( usesClause )?
int alt16=2;
int LA16_0 = input.LA(1);
if ( (LA16_0==USES) ) {
alt16=1;
}
switch (alt16) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:405:69: usesClause
{
pushFollow(FOLLOW_usesClause_in_unitInterface1238);
usesClause54=usesClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, usesClause54.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:405:81: ( interfaceDecl )*
loop17:
while (true) {
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0==CLASS||(LA17_0 >= CONST && LA17_0 <= CONSTRUCTOR)||LA17_0==DESTRUCTOR||LA17_0==EXPORTS||LA17_0==FUNCTION||LA17_0==OPERATOR||LA17_0==PAREN_BRACKET_LEFT||LA17_0==PROCEDURE||LA17_0==RESOURCESTRING||LA17_0==SQUARE_BRACKET_LEFT||LA17_0==THREADVAR||LA17_0==TYPE||LA17_0==VAR) ) {
alt17=1;
}
switch (alt17) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:405:81: interfaceDecl
{
pushFollow(FOLLOW_interfaceDecl_in_unitInterface1241);
interfaceDecl55=interfaceDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceDecl55.getTree());
}
break;
default :
break loop17;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 14, unitInterface_StartIndex); }
}
return retval;
}
// $ANTLR end "unitInterface"
public static class unitImplementation_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitImplementation"
// au/com/integradev/delphi/antlr/Delphi.g:407:1: unitImplementation : IMPLEMENTATION ^ ( usesClause )? ( declSection )* ;
public final DelphiParser.unitImplementation_return unitImplementation() throws RecognitionException {
DelphiParser.unitImplementation_return retval = new DelphiParser.unitImplementation_return();
retval.start = input.LT(1);
int unitImplementation_StartIndex = input.index();
Object root_0 = null;
Token IMPLEMENTATION56=null;
ParserRuleReturnScope usesClause57 =null;
ParserRuleReturnScope declSection58 =null;
Object IMPLEMENTATION56_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 15) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:407:30: ( IMPLEMENTATION ^ ( usesClause )? ( declSection )* )
// au/com/integradev/delphi/antlr/Delphi.g:407:32: IMPLEMENTATION ^ ( usesClause )? ( declSection )*
{
root_0 = (Object)adaptor.nil();
IMPLEMENTATION56=(Token)match(input,IMPLEMENTATION,FOLLOW_IMPLEMENTATION_in_unitImplementation1289); if (state.failed) return retval;
if ( state.backtracking==0 ) {
IMPLEMENTATION56_tree = new ImplementationSectionNodeImpl(IMPLEMENTATION56) ;
root_0 = (Object)adaptor.becomeRoot(IMPLEMENTATION56_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:407:79: ( usesClause )?
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0==USES) ) {
alt18=1;
}
switch (alt18) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:407:79: usesClause
{
pushFollow(FOLLOW_usesClause_in_unitImplementation1295);
usesClause57=usesClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, usesClause57.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:407:91: ( declSection )*
loop19:
while (true) {
int alt19=2;
int LA19_0 = input.LA(1);
if ( (LA19_0==CLASS||(LA19_0 >= CONST && LA19_0 <= CONSTRUCTOR)||LA19_0==DESTRUCTOR||LA19_0==EXPORTS||LA19_0==FUNCTION||LA19_0==LABEL||LA19_0==OPERATOR||LA19_0==PAREN_BRACKET_LEFT||LA19_0==PROCEDURE||LA19_0==RESOURCESTRING||LA19_0==SQUARE_BRACKET_LEFT||LA19_0==THREADVAR||LA19_0==TYPE||LA19_0==VAR) ) {
alt19=1;
}
switch (alt19) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:407:91: declSection
{
pushFollow(FOLLOW_declSection_in_unitImplementation1298);
declSection58=declSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, declSection58.getTree());
}
break;
default :
break loop19;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 15, unitImplementation_StartIndex); }
}
return retval;
}
// $ANTLR end "unitImplementation"
public static class unitBlock_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitBlock"
// au/com/integradev/delphi/antlr/Delphi.g:409:1: unitBlock : ( ( initializationFinalization )? END | compoundStatement );
public final DelphiParser.unitBlock_return unitBlock() throws RecognitionException {
DelphiParser.unitBlock_return retval = new DelphiParser.unitBlock_return();
retval.start = input.LT(1);
int unitBlock_StartIndex = input.index();
Object root_0 = null;
Token END60=null;
ParserRuleReturnScope initializationFinalization59 =null;
ParserRuleReturnScope compoundStatement61 =null;
Object END60_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 16) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:409:30: ( ( initializationFinalization )? END | compoundStatement )
int alt21=2;
int LA21_0 = input.LA(1);
if ( (LA21_0==END||LA21_0==INITIALIZATION) ) {
alt21=1;
}
else if ( (LA21_0==BEGIN) ) {
alt21=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 21, 0, input);
throw nvae;
}
switch (alt21) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:409:32: ( initializationFinalization )? END
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:409:32: ( initializationFinalization )?
int alt20=2;
int LA20_0 = input.LA(1);
if ( (LA20_0==INITIALIZATION) ) {
alt20=1;
}
switch (alt20) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:409:32: initializationFinalization
{
pushFollow(FOLLOW_initializationFinalization_in_unitBlock1355);
initializationFinalization59=initializationFinalization();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, initializationFinalization59.getTree());
}
break;
}
END60=(Token)match(input,END,FOLLOW_END_in_unitBlock1358); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END60_tree = (Object)adaptor.create(END60);
adaptor.addChild(root_0, END60_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:410:32: compoundStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_compoundStatement_in_unitBlock1391);
compoundStatement61=compoundStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, compoundStatement61.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 16, unitBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "unitBlock"
public static class initializationFinalization_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "initializationFinalization"
// au/com/integradev/delphi/antlr/Delphi.g:412:1: initializationFinalization : initializationSection ( finalizationSection )? ;
public final DelphiParser.initializationFinalization_return initializationFinalization() throws RecognitionException {
DelphiParser.initializationFinalization_return retval = new DelphiParser.initializationFinalization_return();
retval.start = input.LT(1);
int initializationFinalization_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope initializationSection62 =null;
ParserRuleReturnScope finalizationSection63 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 17) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:412:30: ( initializationSection ( finalizationSection )? )
// au/com/integradev/delphi/antlr/Delphi.g:412:32: initializationSection ( finalizationSection )?
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_initializationSection_in_initializationFinalization1430);
initializationSection62=initializationSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, initializationSection62.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:412:54: ( finalizationSection )?
int alt22=2;
int LA22_0 = input.LA(1);
if ( (LA22_0==FINALIZATION) ) {
alt22=1;
}
switch (alt22) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:412:54: finalizationSection
{
pushFollow(FOLLOW_finalizationSection_in_initializationFinalization1432);
finalizationSection63=finalizationSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, finalizationSection63.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 17, initializationFinalization_StartIndex); }
}
return retval;
}
// $ANTLR end "initializationFinalization"
public static class initializationSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "initializationSection"
// au/com/integradev/delphi/antlr/Delphi.g:414:1: initializationSection : INITIALIZATION ^ statementList ;
public final DelphiParser.initializationSection_return initializationSection() throws RecognitionException {
DelphiParser.initializationSection_return retval = new DelphiParser.initializationSection_return();
retval.start = input.LT(1);
int initializationSection_StartIndex = input.index();
Object root_0 = null;
Token INITIALIZATION64=null;
ParserRuleReturnScope statementList65 =null;
Object INITIALIZATION64_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 18) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:414:30: ( INITIALIZATION ^ statementList )
// au/com/integradev/delphi/antlr/Delphi.g:414:32: INITIALIZATION ^ statementList
{
root_0 = (Object)adaptor.nil();
INITIALIZATION64=(Token)match(input,INITIALIZATION,FOLLOW_INITIALIZATION_in_initializationSection1477); if (state.failed) return retval;
if ( state.backtracking==0 ) {
INITIALIZATION64_tree = new InitializationSectionNodeImpl(INITIALIZATION64) ;
root_0 = (Object)adaptor.becomeRoot(INITIALIZATION64_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_initializationSection1483);
statementList65=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList65.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 18, initializationSection_StartIndex); }
}
return retval;
}
// $ANTLR end "initializationSection"
public static class finalizationSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "finalizationSection"
// au/com/integradev/delphi/antlr/Delphi.g:416:1: finalizationSection : FINALIZATION ^ statementList ;
public final DelphiParser.finalizationSection_return finalizationSection() throws RecognitionException {
DelphiParser.finalizationSection_return retval = new DelphiParser.finalizationSection_return();
retval.start = input.LT(1);
int finalizationSection_StartIndex = input.index();
Object root_0 = null;
Token FINALIZATION66=null;
ParserRuleReturnScope statementList67 =null;
Object FINALIZATION66_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 19) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:416:30: ( FINALIZATION ^ statementList )
// au/com/integradev/delphi/antlr/Delphi.g:416:32: FINALIZATION ^ statementList
{
root_0 = (Object)adaptor.nil();
FINALIZATION66=(Token)match(input,FINALIZATION,FOLLOW_FINALIZATION_in_finalizationSection1529); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FINALIZATION66_tree = new FinalizationSectionNodeImpl(FINALIZATION66) ;
root_0 = (Object)adaptor.becomeRoot(FINALIZATION66_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_finalizationSection1535);
statementList67=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList67.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 19, finalizationSection_StartIndex); }
}
return retval;
}
// $ANTLR end "finalizationSection"
public static class containsClause_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "containsClause"
// au/com/integradev/delphi/antlr/Delphi.g:422:1: containsClause : CONTAINS ^ unitInFileImportList ;
public final DelphiParser.containsClause_return containsClause() throws RecognitionException {
DelphiParser.containsClause_return retval = new DelphiParser.containsClause_return();
retval.start = input.LT(1);
int containsClause_StartIndex = input.index();
Object root_0 = null;
Token CONTAINS68=null;
ParserRuleReturnScope unitInFileImportList69 =null;
Object CONTAINS68_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 20) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:422:30: ( CONTAINS ^ unitInFileImportList )
// au/com/integradev/delphi/antlr/Delphi.g:422:32: CONTAINS ^ unitInFileImportList
{
root_0 = (Object)adaptor.nil();
CONTAINS68=(Token)match(input,CONTAINS,FOLLOW_CONTAINS_in_containsClause1590); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CONTAINS68_tree = new ContainsClauseNodeImpl(CONTAINS68) ;
root_0 = (Object)adaptor.becomeRoot(CONTAINS68_tree, root_0);
}
pushFollow(FOLLOW_unitInFileImportList_in_containsClause1596);
unitInFileImportList69=unitInFileImportList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitInFileImportList69.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 20, containsClause_StartIndex); }
}
return retval;
}
// $ANTLR end "containsClause"
public static class requiresClause_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "requiresClause"
// au/com/integradev/delphi/antlr/Delphi.g:424:1: requiresClause : REQUIRES ^ unitImportList ;
public final DelphiParser.requiresClause_return requiresClause() throws RecognitionException {
DelphiParser.requiresClause_return retval = new DelphiParser.requiresClause_return();
retval.start = input.LT(1);
int requiresClause_StartIndex = input.index();
Object root_0 = null;
Token REQUIRES70=null;
ParserRuleReturnScope unitImportList71 =null;
Object REQUIRES70_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 21) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:424:30: ( REQUIRES ^ unitImportList )
// au/com/integradev/delphi/antlr/Delphi.g:424:32: REQUIRES ^ unitImportList
{
root_0 = (Object)adaptor.nil();
REQUIRES70=(Token)match(input,REQUIRES,FOLLOW_REQUIRES_in_requiresClause1647); if (state.failed) return retval;
if ( state.backtracking==0 ) {
REQUIRES70_tree = new RequiresClauseNodeImpl(REQUIRES70) ;
root_0 = (Object)adaptor.becomeRoot(REQUIRES70_tree, root_0);
}
pushFollow(FOLLOW_unitImportList_in_requiresClause1653);
unitImportList71=unitImportList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitImportList71.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 21, requiresClause_StartIndex); }
}
return retval;
}
// $ANTLR end "requiresClause"
public static class usesClause_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "usesClause"
// au/com/integradev/delphi/antlr/Delphi.g:426:1: usesClause : USES ^ unitImportList ;
public final DelphiParser.usesClause_return usesClause() throws RecognitionException {
DelphiParser.usesClause_return retval = new DelphiParser.usesClause_return();
retval.start = input.LT(1);
int usesClause_StartIndex = input.index();
Object root_0 = null;
Token USES72=null;
ParserRuleReturnScope unitImportList73 =null;
Object USES72_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 22) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:426:30: ( USES ^ unitImportList )
// au/com/integradev/delphi/antlr/Delphi.g:426:32: USES ^ unitImportList
{
root_0 = (Object)adaptor.nil();
USES72=(Token)match(input,USES,FOLLOW_USES_in_usesClause1708); if (state.failed) return retval;
if ( state.backtracking==0 ) {
USES72_tree = new UsesClauseNodeImpl(USES72) ;
root_0 = (Object)adaptor.becomeRoot(USES72_tree, root_0);
}
pushFollow(FOLLOW_unitImportList_in_usesClause1714);
unitImportList73=unitImportList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitImportList73.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 22, usesClause_StartIndex); }
}
return retval;
}
// $ANTLR end "usesClause"
public static class usesFileClause_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "usesFileClause"
// au/com/integradev/delphi/antlr/Delphi.g:428:1: usesFileClause : USES ^ unitInFileImportList ;
public final DelphiParser.usesFileClause_return usesFileClause() throws RecognitionException {
DelphiParser.usesFileClause_return retval = new DelphiParser.usesFileClause_return();
retval.start = input.LT(1);
int usesFileClause_StartIndex = input.index();
Object root_0 = null;
Token USES74=null;
ParserRuleReturnScope unitInFileImportList75 =null;
Object USES74_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 23) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:428:30: ( USES ^ unitInFileImportList )
// au/com/integradev/delphi/antlr/Delphi.g:428:32: USES ^ unitInFileImportList
{
root_0 = (Object)adaptor.nil();
USES74=(Token)match(input,USES,FOLLOW_USES_in_usesFileClause1765); if (state.failed) return retval;
if ( state.backtracking==0 ) {
USES74_tree = new UsesClauseNodeImpl(USES74) ;
root_0 = (Object)adaptor.becomeRoot(USES74_tree, root_0);
}
pushFollow(FOLLOW_unitInFileImportList_in_usesFileClause1771);
unitInFileImportList75=unitInFileImportList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitInFileImportList75.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 23, usesFileClause_StartIndex); }
}
return retval;
}
// $ANTLR end "usesFileClause"
public static class unitInFileImportList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitInFileImportList"
// au/com/integradev/delphi/antlr/Delphi.g:430:1: unitInFileImportList : unitInFileImport ( ',' unitInFileImport )* ';' ;
public final DelphiParser.unitInFileImportList_return unitInFileImportList() throws RecognitionException {
DelphiParser.unitInFileImportList_return retval = new DelphiParser.unitInFileImportList_return();
retval.start = input.LT(1);
int unitInFileImportList_StartIndex = input.index();
Object root_0 = null;
Token char_literal77=null;
Token char_literal79=null;
ParserRuleReturnScope unitInFileImport76 =null;
ParserRuleReturnScope unitInFileImport78 =null;
Object char_literal77_tree=null;
Object char_literal79_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 24) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:430:30: ( unitInFileImport ( ',' unitInFileImport )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:430:32: unitInFileImport ( ',' unitInFileImport )* ';'
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unitInFileImport_in_unitInFileImportList1816);
unitInFileImport76=unitInFileImport();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitInFileImport76.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:430:49: ( ',' unitInFileImport )*
loop23:
while (true) {
int alt23=2;
int LA23_0 = input.LA(1);
if ( (LA23_0==COMMA) ) {
alt23=1;
}
switch (alt23) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:430:50: ',' unitInFileImport
{
char_literal77=(Token)match(input,COMMA,FOLLOW_COMMA_in_unitInFileImportList1819); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal77_tree = (Object)adaptor.create(char_literal77);
adaptor.addChild(root_0, char_literal77_tree);
}
pushFollow(FOLLOW_unitInFileImport_in_unitInFileImportList1821);
unitInFileImport78=unitInFileImport();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitInFileImport78.getTree());
}
break;
default :
break loop23;
}
}
char_literal79=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_unitInFileImportList1825); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal79_tree = (Object)adaptor.create(char_literal79);
adaptor.addChild(root_0, char_literal79_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 24, unitInFileImportList_StartIndex); }
}
return retval;
}
// $ANTLR end "unitInFileImportList"
public static class unitImportList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitImportList"
// au/com/integradev/delphi/antlr/Delphi.g:432:1: unitImportList : unitImport ( ',' unitImport )* ';' ;
public final DelphiParser.unitImportList_return unitImportList() throws RecognitionException {
DelphiParser.unitImportList_return retval = new DelphiParser.unitImportList_return();
retval.start = input.LT(1);
int unitImportList_StartIndex = input.index();
Object root_0 = null;
Token char_literal81=null;
Token char_literal83=null;
ParserRuleReturnScope unitImport80 =null;
ParserRuleReturnScope unitImport82 =null;
Object char_literal81_tree=null;
Object char_literal83_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 25) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:432:30: ( unitImport ( ',' unitImport )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:432:32: unitImport ( ',' unitImport )* ';'
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unitImport_in_unitImportList1876);
unitImport80=unitImport();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitImport80.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:432:43: ( ',' unitImport )*
loop24:
while (true) {
int alt24=2;
int LA24_0 = input.LA(1);
if ( (LA24_0==COMMA) ) {
alt24=1;
}
switch (alt24) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:432:44: ',' unitImport
{
char_literal81=(Token)match(input,COMMA,FOLLOW_COMMA_in_unitImportList1879); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal81_tree = (Object)adaptor.create(char_literal81);
adaptor.addChild(root_0, char_literal81_tree);
}
pushFollow(FOLLOW_unitImport_in_unitImportList1881);
unitImport82=unitImport();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unitImport82.getTree());
}
break;
default :
break loop24;
}
}
char_literal83=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_unitImportList1885); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal83_tree = (Object)adaptor.create(char_literal83);
adaptor.addChild(root_0, char_literal83_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 25, unitImportList_StartIndex); }
}
return retval;
}
// $ANTLR end "unitImportList"
public static class unitImport_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitImport"
// au/com/integradev/delphi/antlr/Delphi.g:434:1: unitImport : qualifiedNameDeclaration -> ^( TkUnitImport qualifiedNameDeclaration ) ;
public final DelphiParser.unitImport_return unitImport() throws RecognitionException {
DelphiParser.unitImport_return retval = new DelphiParser.unitImport_return();
retval.start = input.LT(1);
int unitImport_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope qualifiedNameDeclaration84 =null;
RewriteRuleSubtreeStream stream_qualifiedNameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule qualifiedNameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 26) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:434:30: ( qualifiedNameDeclaration -> ^( TkUnitImport qualifiedNameDeclaration ) )
// au/com/integradev/delphi/antlr/Delphi.g:434:32: qualifiedNameDeclaration
{
pushFollow(FOLLOW_qualifiedNameDeclaration_in_unitImport1940);
qualifiedNameDeclaration84=qualifiedNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_qualifiedNameDeclaration.add(qualifiedNameDeclaration84.getTree());
// AST REWRITE
// elements: qualifiedNameDeclaration
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 435:30: -> ^( TkUnitImport qualifiedNameDeclaration )
{
// au/com/integradev/delphi/antlr/Delphi.g:435:33: ^( TkUnitImport qualifiedNameDeclaration )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new UnitImportNodeImpl(TkUnitImport), root_1);
adaptor.addChild(root_1, stream_qualifiedNameDeclaration.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 26, unitImport_StartIndex); }
}
return retval;
}
// $ANTLR end "unitImport"
public static class unitInFileImport_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unitInFileImport"
// au/com/integradev/delphi/antlr/Delphi.g:437:1: unitInFileImport : qualifiedNameDeclaration ( IN textLiteral )? -> ^( TkUnitImport qualifiedNameDeclaration ( IN textLiteral )? ) ;
public final DelphiParser.unitInFileImport_return unitInFileImport() throws RecognitionException {
DelphiParser.unitInFileImport_return retval = new DelphiParser.unitInFileImport_return();
retval.start = input.LT(1);
int unitInFileImport_StartIndex = input.index();
Object root_0 = null;
Token IN86=null;
ParserRuleReturnScope qualifiedNameDeclaration85 =null;
ParserRuleReturnScope textLiteral87 =null;
Object IN86_tree=null;
RewriteRuleTokenStream stream_IN=new RewriteRuleTokenStream(adaptor,"token IN");
RewriteRuleSubtreeStream stream_textLiteral=new RewriteRuleSubtreeStream(adaptor,"rule textLiteral");
RewriteRuleSubtreeStream stream_qualifiedNameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule qualifiedNameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 27) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:437:30: ( qualifiedNameDeclaration ( IN textLiteral )? -> ^( TkUnitImport qualifiedNameDeclaration ( IN textLiteral )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:437:32: qualifiedNameDeclaration ( IN textLiteral )?
{
pushFollow(FOLLOW_qualifiedNameDeclaration_in_unitInFileImport2029);
qualifiedNameDeclaration85=qualifiedNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_qualifiedNameDeclaration.add(qualifiedNameDeclaration85.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:437:57: ( IN textLiteral )?
int alt25=2;
int LA25_0 = input.LA(1);
if ( (LA25_0==IN) ) {
alt25=1;
}
switch (alt25) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:437:58: IN textLiteral
{
IN86=(Token)match(input,IN,FOLLOW_IN_in_unitInFileImport2032); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_IN.add(IN86);
pushFollow(FOLLOW_textLiteral_in_unitInFileImport2034);
textLiteral87=textLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_textLiteral.add(textLiteral87.getTree());
}
break;
}
// AST REWRITE
// elements: qualifiedNameDeclaration, IN, textLiteral
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 438:30: -> ^( TkUnitImport qualifiedNameDeclaration ( IN textLiteral )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:438:33: ^( TkUnitImport qualifiedNameDeclaration ( IN textLiteral )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new UnitImportNodeImpl(TkUnitImport), root_1);
adaptor.addChild(root_1, stream_qualifiedNameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:438:93: ( IN textLiteral )?
if ( stream_IN.hasNext()||stream_textLiteral.hasNext() ) {
adaptor.addChild(root_1, stream_IN.nextNode());
adaptor.addChild(root_1, stream_textLiteral.nextTree());
}
stream_IN.reset();
stream_textLiteral.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 27, unitInFileImport_StartIndex); }
}
return retval;
}
// $ANTLR end "unitInFileImport"
public static class block_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "block"
// au/com/integradev/delphi/antlr/Delphi.g:444:1: block : ( localDeclSection )? blockBody ;
public final DelphiParser.block_return block() throws RecognitionException {
DelphiParser.block_return retval = new DelphiParser.block_return();
retval.start = input.LT(1);
int block_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope localDeclSection88 =null;
ParserRuleReturnScope blockBody89 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 28) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:444:30: ( ( localDeclSection )? blockBody )
// au/com/integradev/delphi/antlr/Delphi.g:444:32: ( localDeclSection )? blockBody
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:444:32: ( localDeclSection )?
int alt26=2;
int LA26_0 = input.LA(1);
if ( (LA26_0==CLASS||(LA26_0 >= CONST && LA26_0 <= CONSTRUCTOR)||LA26_0==DESTRUCTOR||LA26_0==EXPORTS||LA26_0==FUNCTION||LA26_0==LABEL||LA26_0==OPERATOR||LA26_0==PAREN_BRACKET_LEFT||LA26_0==PROCEDURE||LA26_0==RESOURCESTRING||LA26_0==SQUARE_BRACKET_LEFT||LA26_0==THREADVAR||LA26_0==TYPE||LA26_0==VAR) ) {
alt26=1;
}
switch (alt26) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:444:32: localDeclSection
{
pushFollow(FOLLOW_localDeclSection_in_block2147);
localDeclSection88=localDeclSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, localDeclSection88.getTree());
}
break;
}
pushFollow(FOLLOW_blockBody_in_block2150);
blockBody89=blockBody();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, blockBody89.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 28, block_StartIndex); }
}
return retval;
}
// $ANTLR end "block"
public static class localDeclSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "localDeclSection"
// au/com/integradev/delphi/antlr/Delphi.g:446:1: localDeclSection : ( declSection )+ -> ^( TkLocalDeclarations ( declSection )+ ) ;
public final DelphiParser.localDeclSection_return localDeclSection() throws RecognitionException {
DelphiParser.localDeclSection_return retval = new DelphiParser.localDeclSection_return();
retval.start = input.LT(1);
int localDeclSection_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope declSection90 =null;
RewriteRuleSubtreeStream stream_declSection=new RewriteRuleSubtreeStream(adaptor,"rule declSection");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 29) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:446:30: ( ( declSection )+ -> ^( TkLocalDeclarations ( declSection )+ ) )
// au/com/integradev/delphi/antlr/Delphi.g:446:32: ( declSection )+
{
// au/com/integradev/delphi/antlr/Delphi.g:446:32: ( declSection )+
int cnt27=0;
loop27:
while (true) {
int alt27=2;
int LA27_0 = input.LA(1);
if ( (LA27_0==CLASS||(LA27_0 >= CONST && LA27_0 <= CONSTRUCTOR)||LA27_0==DESTRUCTOR||LA27_0==EXPORTS||LA27_0==FUNCTION||LA27_0==LABEL||LA27_0==OPERATOR||LA27_0==PAREN_BRACKET_LEFT||LA27_0==PROCEDURE||LA27_0==RESOURCESTRING||LA27_0==SQUARE_BRACKET_LEFT||LA27_0==THREADVAR||LA27_0==TYPE||LA27_0==VAR) ) {
alt27=1;
}
switch (alt27) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:446:32: declSection
{
pushFollow(FOLLOW_declSection_in_localDeclSection2199);
declSection90=declSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_declSection.add(declSection90.getTree());
}
break;
default :
if ( cnt27 >= 1 ) break loop27;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(27, input);
throw eee;
}
cnt27++;
}
// AST REWRITE
// elements: declSection
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 446:45: -> ^( TkLocalDeclarations ( declSection )+ )
{
// au/com/integradev/delphi/antlr/Delphi.g:446:48: ^( TkLocalDeclarations ( declSection )+ )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new LocalDeclarationSectionNodeImpl(TkLocalDeclarations), root_1);
if ( !(stream_declSection.hasNext()) ) {
throw new RewriteEarlyExitException();
}
while ( stream_declSection.hasNext() ) {
adaptor.addChild(root_1, stream_declSection.nextTree());
}
stream_declSection.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 29, localDeclSection_StartIndex); }
}
return retval;
}
// $ANTLR end "localDeclSection"
public static class blockBody_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "blockBody"
// au/com/integradev/delphi/antlr/Delphi.g:448:1: blockBody : ( compoundStatement | assemblerStatement );
public final DelphiParser.blockBody_return blockBody() throws RecognitionException {
DelphiParser.blockBody_return retval = new DelphiParser.blockBody_return();
retval.start = input.LT(1);
int blockBody_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope compoundStatement91 =null;
ParserRuleReturnScope assemblerStatement92 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 30) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:448:30: ( compoundStatement | assemblerStatement )
int alt28=2;
int LA28_0 = input.LA(1);
if ( (LA28_0==BEGIN) ) {
alt28=1;
}
else if ( (LA28_0==ASM) ) {
alt28=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 28, 0, input);
throw nvae;
}
switch (alt28) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:448:32: compoundStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_compoundStatement_in_blockBody2268);
compoundStatement91=compoundStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, compoundStatement91.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:449:32: assemblerStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_assemblerStatement_in_blockBody2301);
assemblerStatement92=assemblerStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, assemblerStatement92.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 30, blockBody_StartIndex); }
}
return retval;
}
// $ANTLR end "blockBody"
public static class declSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "declSection"
// au/com/integradev/delphi/antlr/Delphi.g:451:1: declSection : ( labelDeclSection | constSection | typeSection | varSection | routineImplementation | exportsSection | attributeList );
public final DelphiParser.declSection_return declSection() throws RecognitionException {
DelphiParser.declSection_return retval = new DelphiParser.declSection_return();
retval.start = input.LT(1);
int declSection_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope labelDeclSection93 =null;
ParserRuleReturnScope constSection94 =null;
ParserRuleReturnScope typeSection95 =null;
ParserRuleReturnScope varSection96 =null;
ParserRuleReturnScope routineImplementation97 =null;
ParserRuleReturnScope exportsSection98 =null;
ParserRuleReturnScope attributeList99 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 31) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:451:30: ( labelDeclSection | constSection | typeSection | varSection | routineImplementation | exportsSection | attributeList )
int alt29=7;
switch ( input.LA(1) ) {
case LABEL:
{
alt29=1;
}
break;
case CONST:
case RESOURCESTRING:
{
alt29=2;
}
break;
case TYPE:
{
alt29=3;
}
break;
case THREADVAR:
case VAR:
{
alt29=4;
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA29_7 = input.LA(2);
if ( (synpred37_Delphi()) ) {
alt29=5;
}
else if ( (true) ) {
alt29=7;
}
}
break;
case CLASS:
case CONSTRUCTOR:
case DESTRUCTOR:
case FUNCTION:
case OPERATOR:
case PROCEDURE:
{
alt29=5;
}
break;
case EXPORTS:
{
alt29=6;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 29, 0, input);
throw nvae;
}
switch (alt29) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:451:32: labelDeclSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_labelDeclSection_in_declSection2355);
labelDeclSection93=labelDeclSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, labelDeclSection93.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:452:32: constSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_constSection_in_declSection2388);
constSection94=constSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constSection94.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:453:32: typeSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeSection_in_declSection2421);
typeSection95=typeSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeSection95.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:454:32: varSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_varSection_in_declSection2454);
varSection96=varSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varSection96.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:455:32: routineImplementation
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_routineImplementation_in_declSection2487);
routineImplementation97=routineImplementation();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineImplementation97.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:456:32: exportsSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_exportsSection_in_declSection2520);
exportsSection98=exportsSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, exportsSection98.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:457:32: attributeList
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_attributeList_in_declSection2553);
attributeList99=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, attributeList99.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 31, declSection_StartIndex); }
}
return retval;
}
// $ANTLR end "declSection"
public static class interfaceDecl_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceDecl"
// au/com/integradev/delphi/antlr/Delphi.g:459:1: interfaceDecl : ( constSection | typeSection | varSection | exportsSection | routineInterface | attributeList );
public final DelphiParser.interfaceDecl_return interfaceDecl() throws RecognitionException {
DelphiParser.interfaceDecl_return retval = new DelphiParser.interfaceDecl_return();
retval.start = input.LT(1);
int interfaceDecl_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope constSection100 =null;
ParserRuleReturnScope typeSection101 =null;
ParserRuleReturnScope varSection102 =null;
ParserRuleReturnScope exportsSection103 =null;
ParserRuleReturnScope routineInterface104 =null;
ParserRuleReturnScope attributeList105 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 32) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:459:30: ( constSection | typeSection | varSection | exportsSection | routineInterface | attributeList )
int alt30=6;
switch ( input.LA(1) ) {
case CONST:
case RESOURCESTRING:
{
alt30=1;
}
break;
case TYPE:
{
alt30=2;
}
break;
case THREADVAR:
case VAR:
{
alt30=3;
}
break;
case EXPORTS:
{
alt30=4;
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA30_7 = input.LA(2);
if ( (synpred43_Delphi()) ) {
alt30=5;
}
else if ( (true) ) {
alt30=6;
}
}
break;
case CLASS:
case CONSTRUCTOR:
case DESTRUCTOR:
case FUNCTION:
case OPERATOR:
case PROCEDURE:
{
alt30=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 30, 0, input);
throw nvae;
}
switch (alt30) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:459:32: constSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_constSection_in_interfaceDecl2605);
constSection100=constSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constSection100.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:460:32: typeSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeSection_in_interfaceDecl2638);
typeSection101=typeSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeSection101.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:461:32: varSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_varSection_in_interfaceDecl2671);
varSection102=varSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varSection102.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:462:32: exportsSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_exportsSection_in_interfaceDecl2704);
exportsSection103=exportsSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, exportsSection103.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:463:32: routineInterface
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_routineInterface_in_interfaceDecl2737);
routineInterface104=routineInterface();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineInterface104.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:464:32: attributeList
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_attributeList_in_interfaceDecl2770);
attributeList105=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, attributeList105.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 32, interfaceDecl_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceDecl"
public static class labelDeclSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "labelDeclSection"
// au/com/integradev/delphi/antlr/Delphi.g:466:1: labelDeclSection : LABEL ^ labelNameDeclaration ( ',' labelNameDeclaration )* ';' ;
public final DelphiParser.labelDeclSection_return labelDeclSection() throws RecognitionException {
DelphiParser.labelDeclSection_return retval = new DelphiParser.labelDeclSection_return();
retval.start = input.LT(1);
int labelDeclSection_StartIndex = input.index();
Object root_0 = null;
Token LABEL106=null;
Token char_literal108=null;
Token char_literal110=null;
ParserRuleReturnScope labelNameDeclaration107 =null;
ParserRuleReturnScope labelNameDeclaration109 =null;
Object LABEL106_tree=null;
Object char_literal108_tree=null;
Object char_literal110_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 33) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:466:30: ( LABEL ^ labelNameDeclaration ( ',' labelNameDeclaration )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:466:32: LABEL ^ labelNameDeclaration ( ',' labelNameDeclaration )* ';'
{
root_0 = (Object)adaptor.nil();
LABEL106=(Token)match(input,LABEL,FOLLOW_LABEL_in_labelDeclSection2819); if (state.failed) return retval;
if ( state.backtracking==0 ) {
LABEL106_tree = new LabelDeclarationNodeImpl(LABEL106) ;
root_0 = (Object)adaptor.becomeRoot(LABEL106_tree, root_0);
}
pushFollow(FOLLOW_labelNameDeclaration_in_labelDeclSection2825);
labelNameDeclaration107=labelNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, labelNameDeclaration107.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:466:86: ( ',' labelNameDeclaration )*
loop31:
while (true) {
int alt31=2;
int LA31_0 = input.LA(1);
if ( (LA31_0==COMMA) ) {
alt31=1;
}
switch (alt31) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:466:87: ',' labelNameDeclaration
{
char_literal108=(Token)match(input,COMMA,FOLLOW_COMMA_in_labelDeclSection2828); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal108_tree = (Object)adaptor.create(char_literal108);
adaptor.addChild(root_0, char_literal108_tree);
}
pushFollow(FOLLOW_labelNameDeclaration_in_labelDeclSection2830);
labelNameDeclaration109=labelNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, labelNameDeclaration109.getTree());
}
break;
default :
break loop31;
}
}
char_literal110=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_labelDeclSection2834); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal110_tree = (Object)adaptor.create(char_literal110);
adaptor.addChild(root_0, char_literal110_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 33, labelDeclSection_StartIndex); }
}
return retval;
}
// $ANTLR end "labelDeclSection"
public static class constSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "constSection"
// au/com/integradev/delphi/antlr/Delphi.g:468:1: constSection : ( CONST ^| RESOURCESTRING ^) ( constDeclaration )* ;
public final DelphiParser.constSection_return constSection() throws RecognitionException {
DelphiParser.constSection_return retval = new DelphiParser.constSection_return();
retval.start = input.LT(1);
int constSection_StartIndex = input.index();
Object root_0 = null;
Token CONST111=null;
Token RESOURCESTRING112=null;
ParserRuleReturnScope constDeclaration113 =null;
Object CONST111_tree=null;
Object RESOURCESTRING112_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 34) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:468:30: ( ( CONST ^| RESOURCESTRING ^) ( constDeclaration )* )
// au/com/integradev/delphi/antlr/Delphi.g:468:32: ( CONST ^| RESOURCESTRING ^) ( constDeclaration )*
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:468:32: ( CONST ^| RESOURCESTRING ^)
int alt32=2;
int LA32_0 = input.LA(1);
if ( (LA32_0==CONST) ) {
alt32=1;
}
else if ( (LA32_0==RESOURCESTRING) ) {
alt32=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 32, 0, input);
throw nvae;
}
switch (alt32) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:468:33: CONST ^
{
CONST111=(Token)match(input,CONST,FOLLOW_CONST_in_constSection2888); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CONST111_tree = new ConstSectionNodeImpl(CONST111) ;
root_0 = (Object)adaptor.becomeRoot(CONST111_tree, root_0);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:468:64: RESOURCESTRING ^
{
RESOURCESTRING112=(Token)match(input,RESOURCESTRING,FOLLOW_RESOURCESTRING_in_constSection2896); if (state.failed) return retval;
if ( state.backtracking==0 ) {
RESOURCESTRING112_tree = new ConstSectionNodeImpl(RESOURCESTRING112) ;
root_0 = (Object)adaptor.becomeRoot(RESOURCESTRING112_tree, root_0);
}
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:468:103: ( constDeclaration )*
loop33:
while (true) {
int alt33=2;
switch ( input.LA(1) ) {
case LABEL:
{
int LA33_4 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA33_10 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case OPERATOR:
{
int LA33_12 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case STRICT:
{
int LA33_17 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case PROTECTED:
{
int LA33_18 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case PRIVATE:
{
int LA33_19 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case PUBLIC:
{
int LA33_20 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case PUBLISHED:
{
int LA33_21 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case AUTOMATED:
{
int LA33_22 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case TkIdentifier:
{
int LA33_23 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA33_25 = input.LA(2);
if ( (synpred46_Delphi()) ) {
alt33=1;
}
}
break;
}
switch (alt33) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:468:103: constDeclaration
{
pushFollow(FOLLOW_constDeclaration_in_constSection2903);
constDeclaration113=constDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constDeclaration113.getTree());
}
break;
default :
break loop33;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 34, constSection_StartIndex); }
}
return retval;
}
// $ANTLR end "constSection"
public static class constDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "constDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:473:1: constDeclaration : ( ( attributeList )? nameDeclaration ':' fixedArrayType '=' arrayExpression ( portabilityDirective )* ';' -> ^( TkConstDeclaration nameDeclaration arrayExpression fixedArrayType ( attributeList )? ( portabilityDirective )* ) | ( attributeList )? nameDeclaration ( ':' varType )? '=' constExpression ( portabilityDirective )* ';' -> ^( TkConstDeclaration nameDeclaration constExpression ( varType )? ( attributeList )? ( portabilityDirective )* ) );
public final DelphiParser.constDeclaration_return constDeclaration() throws RecognitionException {
DelphiParser.constDeclaration_return retval = new DelphiParser.constDeclaration_return();
retval.start = input.LT(1);
int constDeclaration_StartIndex = input.index();
Object root_0 = null;
Token char_literal116=null;
Token char_literal118=null;
Token char_literal121=null;
Token char_literal124=null;
Token char_literal126=null;
Token char_literal129=null;
ParserRuleReturnScope attributeList114 =null;
ParserRuleReturnScope nameDeclaration115 =null;
ParserRuleReturnScope fixedArrayType117 =null;
ParserRuleReturnScope arrayExpression119 =null;
ParserRuleReturnScope portabilityDirective120 =null;
ParserRuleReturnScope attributeList122 =null;
ParserRuleReturnScope nameDeclaration123 =null;
ParserRuleReturnScope varType125 =null;
ParserRuleReturnScope constExpression127 =null;
ParserRuleReturnScope portabilityDirective128 =null;
Object char_literal116_tree=null;
Object char_literal118_tree=null;
Object char_literal121_tree=null;
Object char_literal124_tree=null;
Object char_literal126_tree=null;
Object char_literal129_tree=null;
RewriteRuleTokenStream stream_EQUAL=new RewriteRuleTokenStream(adaptor,"token EQUAL");
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_fixedArrayType=new RewriteRuleSubtreeStream(adaptor,"rule fixedArrayType");
RewriteRuleSubtreeStream stream_portabilityDirective=new RewriteRuleSubtreeStream(adaptor,"rule portabilityDirective");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_arrayExpression=new RewriteRuleSubtreeStream(adaptor,"rule arrayExpression");
RewriteRuleSubtreeStream stream_constExpression=new RewriteRuleSubtreeStream(adaptor,"rule constExpression");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 35) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:473:30: ( ( attributeList )? nameDeclaration ':' fixedArrayType '=' arrayExpression ( portabilityDirective )* ';' -> ^( TkConstDeclaration nameDeclaration arrayExpression fixedArrayType ( attributeList )? ( portabilityDirective )* ) | ( attributeList )? nameDeclaration ( ':' varType )? '=' constExpression ( portabilityDirective )* ';' -> ^( TkConstDeclaration nameDeclaration constExpression ( varType )? ( attributeList )? ( portabilityDirective )* ) )
int alt39=2;
switch ( input.LA(1) ) {
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA39_1 = input.LA(2);
if ( (synpred49_Delphi()) ) {
alt39=1;
}
else if ( (true) ) {
alt39=2;
}
}
break;
case TkIdentifier:
{
int LA39_2 = input.LA(2);
if ( (synpred49_Delphi()) ) {
alt39=1;
}
else if ( (true) ) {
alt39=2;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA39_3 = input.LA(2);
if ( (synpred49_Delphi()) ) {
alt39=1;
}
else if ( (true) ) {
alt39=2;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 39, 0, input);
throw nvae;
}
switch (alt39) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:473:32: ( attributeList )? nameDeclaration ':' fixedArrayType '=' arrayExpression ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:473:32: ( attributeList )?
int alt34=2;
int LA34_0 = input.LA(1);
if ( (LA34_0==PAREN_BRACKET_LEFT||LA34_0==SQUARE_BRACKET_LEFT) ) {
alt34=1;
}
switch (alt34) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:473:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_constDeclaration3043);
attributeList114=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList114.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclaration_in_constDeclaration3046);
nameDeclaration115=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration115.getTree());
char_literal116=(Token)match(input,COLON,FOLLOW_COLON_in_constDeclaration3048); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal116);
pushFollow(FOLLOW_fixedArrayType_in_constDeclaration3050);
fixedArrayType117=fixedArrayType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_fixedArrayType.add(fixedArrayType117.getTree());
char_literal118=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_constDeclaration3052); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal118);
pushFollow(FOLLOW_arrayExpression_in_constDeclaration3054);
arrayExpression119=arrayExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arrayExpression.add(arrayExpression119.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:473:102: ( portabilityDirective )*
loop35:
while (true) {
int alt35=2;
int LA35_0 = input.LA(1);
if ( (LA35_0==DEPRECATED||LA35_0==EXPERIMENTAL||LA35_0==LIBRARY||LA35_0==PLATFORM) ) {
alt35=1;
}
switch (alt35) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:473:102: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_constDeclaration3056);
portabilityDirective120=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective120.getTree());
}
break;
default :
break loop35;
}
}
char_literal121=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_constDeclaration3059); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal121);
// AST REWRITE
// elements: arrayExpression, attributeList, fixedArrayType, portabilityDirective, nameDeclaration
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 474:30: -> ^( TkConstDeclaration nameDeclaration arrayExpression fixedArrayType ( attributeList )? ( portabilityDirective )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:474:33: ^( TkConstDeclaration nameDeclaration arrayExpression fixedArrayType ( attributeList )? ( portabilityDirective )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ConstDeclarationNodeImpl(TkConstDeclaration), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
adaptor.addChild(root_1, stream_arrayExpression.nextTree());
adaptor.addChild(root_1, stream_fixedArrayType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:474:127: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:474:142: ( portabilityDirective )*
while ( stream_portabilityDirective.hasNext() ) {
adaptor.addChild(root_1, stream_portabilityDirective.nextTree());
}
stream_portabilityDirective.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:475:32: ( attributeList )? nameDeclaration ( ':' varType )? '=' constExpression ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:475:32: ( attributeList )?
int alt36=2;
int LA36_0 = input.LA(1);
if ( (LA36_0==PAREN_BRACKET_LEFT||LA36_0==SQUARE_BRACKET_LEFT) ) {
alt36=1;
}
switch (alt36) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:475:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_constDeclaration3142);
attributeList122=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList122.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclaration_in_constDeclaration3145);
nameDeclaration123=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration123.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:475:63: ( ':' varType )?
int alt37=2;
int LA37_0 = input.LA(1);
if ( (LA37_0==COLON) ) {
alt37=1;
}
switch (alt37) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:475:64: ':' varType
{
char_literal124=(Token)match(input,COLON,FOLLOW_COLON_in_constDeclaration3148); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal124);
pushFollow(FOLLOW_varType_in_constDeclaration3150);
varType125=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType125.getTree());
}
break;
}
char_literal126=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_constDeclaration3154); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal126);
pushFollow(FOLLOW_constExpression_in_constDeclaration3156);
constExpression127=constExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_constExpression.add(constExpression127.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:475:98: ( portabilityDirective )*
loop38:
while (true) {
int alt38=2;
int LA38_0 = input.LA(1);
if ( (LA38_0==DEPRECATED||LA38_0==EXPERIMENTAL||LA38_0==LIBRARY||LA38_0==PLATFORM) ) {
alt38=1;
}
switch (alt38) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:475:98: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_constDeclaration3158);
portabilityDirective128=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective128.getTree());
}
break;
default :
break loop38;
}
}
char_literal129=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_constDeclaration3161); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal129);
// AST REWRITE
// elements: varType, nameDeclaration, constExpression, portabilityDirective, attributeList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 476:30: -> ^( TkConstDeclaration nameDeclaration constExpression ( varType )? ( attributeList )? ( portabilityDirective )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:476:33: ^( TkConstDeclaration nameDeclaration constExpression ( varType )? ( attributeList )? ( portabilityDirective )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ConstDeclarationNodeImpl(TkConstDeclaration), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
adaptor.addChild(root_1, stream_constExpression.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:476:112: ( varType )?
if ( stream_varType.hasNext() ) {
adaptor.addChild(root_1, stream_varType.nextTree());
}
stream_varType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:476:121: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:476:136: ( portabilityDirective )*
while ( stream_portabilityDirective.hasNext() ) {
adaptor.addChild(root_1, stream_portabilityDirective.nextTree());
}
stream_portabilityDirective.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 35, constDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "constDeclaration"
public static class typeSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeSection"
// au/com/integradev/delphi/antlr/Delphi.g:478:1: typeSection : TYPE ^ ( typeDeclaration )+ ;
public final DelphiParser.typeSection_return typeSection() throws RecognitionException {
DelphiParser.typeSection_return retval = new DelphiParser.typeSection_return();
retval.start = input.LT(1);
int typeSection_StartIndex = input.index();
Object root_0 = null;
Token TYPE130=null;
ParserRuleReturnScope typeDeclaration131 =null;
Object TYPE130_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 36) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:478:30: ( TYPE ^ ( typeDeclaration )+ )
// au/com/integradev/delphi/antlr/Delphi.g:478:32: TYPE ^ ( typeDeclaration )+
{
root_0 = (Object)adaptor.nil();
TYPE130=(Token)match(input,TYPE,FOLLOW_TYPE_in_typeSection3266); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TYPE130_tree = new TypeSectionNodeImpl(TYPE130) ;
root_0 = (Object)adaptor.becomeRoot(TYPE130_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:478:59: ( typeDeclaration )+
int cnt40=0;
loop40:
while (true) {
int alt40=2;
switch ( input.LA(1) ) {
case LABEL:
{
int LA40_4 = input.LA(2);
if ( (synpred53_Delphi()) ) {
alt40=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA40_10 = input.LA(2);
if ( (synpred53_Delphi()) ) {
alt40=1;
}
}
break;
case OPERATOR:
{
int LA40_12 = input.LA(2);
if ( (synpred53_Delphi()) ) {
alt40=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt40=1;
}
break;
}
switch (alt40) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:478:59: typeDeclaration
{
pushFollow(FOLLOW_typeDeclaration_in_typeSection3272);
typeDeclaration131=typeDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeDeclaration131.getTree());
}
break;
default :
if ( cnt40 >= 1 ) break loop40;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(40, input);
throw eee;
}
cnt40++;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 36, typeSection_StartIndex); }
}
return retval;
}
// $ANTLR end "typeSection"
public static class innerTypeSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "innerTypeSection"
// au/com/integradev/delphi/antlr/Delphi.g:480:1: innerTypeSection : TYPE ^ ( typeDeclaration )* ;
public final DelphiParser.innerTypeSection_return innerTypeSection() throws RecognitionException {
DelphiParser.innerTypeSection_return retval = new DelphiParser.innerTypeSection_return();
retval.start = input.LT(1);
int innerTypeSection_StartIndex = input.index();
Object root_0 = null;
Token TYPE132=null;
ParserRuleReturnScope typeDeclaration133 =null;
Object TYPE132_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 37) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:480:30: ( TYPE ^ ( typeDeclaration )* )
// au/com/integradev/delphi/antlr/Delphi.g:480:32: TYPE ^ ( typeDeclaration )*
{
root_0 = (Object)adaptor.nil();
TYPE132=(Token)match(input,TYPE,FOLLOW_TYPE_in_innerTypeSection3322); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TYPE132_tree = new TypeSectionNodeImpl(TYPE132) ;
root_0 = (Object)adaptor.becomeRoot(TYPE132_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:480:59: ( typeDeclaration )*
loop41:
while (true) {
int alt41=2;
switch ( input.LA(1) ) {
case STRICT:
{
int LA41_2 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case PROTECTED:
{
int LA41_3 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case PRIVATE:
{
int LA41_4 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case PUBLIC:
{
int LA41_5 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case PUBLISHED:
{
int LA41_6 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case AUTOMATED:
{
int LA41_7 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA41_10 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case TkIdentifier:
{
int LA41_11 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case OPERATOR:
{
int LA41_12 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA41_13 = input.LA(2);
if ( (synpred54_Delphi()) ) {
alt41=1;
}
}
break;
}
switch (alt41) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:480:59: typeDeclaration
{
pushFollow(FOLLOW_typeDeclaration_in_innerTypeSection3328);
typeDeclaration133=typeDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeDeclaration133.getTree());
}
break;
default :
break loop41;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 37, innerTypeSection_StartIndex); }
}
return retval;
}
// $ANTLR end "innerTypeSection"
public static class typeDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:482:1: typeDeclaration : ( attributeList )? genericNameDeclaration '=' typeDecl ( portabilityDirective )* ';' -> ^( TkTypeDeclaration genericNameDeclaration typeDecl ( attributeList )? ( portabilityDirective )* ) ;
public final DelphiParser.typeDeclaration_return typeDeclaration() throws RecognitionException {
DelphiParser.typeDeclaration_return retval = new DelphiParser.typeDeclaration_return();
retval.start = input.LT(1);
int typeDeclaration_StartIndex = input.index();
Object root_0 = null;
Token char_literal136=null;
Token char_literal139=null;
ParserRuleReturnScope attributeList134 =null;
ParserRuleReturnScope genericNameDeclaration135 =null;
ParserRuleReturnScope typeDecl137 =null;
ParserRuleReturnScope portabilityDirective138 =null;
Object char_literal136_tree=null;
Object char_literal139_tree=null;
RewriteRuleTokenStream stream_EQUAL=new RewriteRuleTokenStream(adaptor,"token EQUAL");
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleSubtreeStream stream_portabilityDirective=new RewriteRuleSubtreeStream(adaptor,"rule portabilityDirective");
RewriteRuleSubtreeStream stream_genericNameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule genericNameDeclaration");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_typeDecl=new RewriteRuleSubtreeStream(adaptor,"rule typeDecl");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 38) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:482:30: ( ( attributeList )? genericNameDeclaration '=' typeDecl ( portabilityDirective )* ';' -> ^( TkTypeDeclaration genericNameDeclaration typeDecl ( attributeList )? ( portabilityDirective )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:482:32: ( attributeList )? genericNameDeclaration '=' typeDecl ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:482:32: ( attributeList )?
int alt42=2;
int LA42_0 = input.LA(1);
if ( (LA42_0==PAREN_BRACKET_LEFT||LA42_0==SQUARE_BRACKET_LEFT) ) {
alt42=1;
}
switch (alt42) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:482:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_typeDeclaration3379);
attributeList134=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList134.getTree());
}
break;
}
pushFollow(FOLLOW_genericNameDeclaration_in_typeDeclaration3382);
genericNameDeclaration135=genericNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericNameDeclaration.add(genericNameDeclaration135.getTree());
char_literal136=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_typeDeclaration3384); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal136);
pushFollow(FOLLOW_typeDecl_in_typeDeclaration3386);
typeDecl137=typeDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeDecl.add(typeDecl137.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:482:83: ( portabilityDirective )*
loop43:
while (true) {
int alt43=2;
int LA43_0 = input.LA(1);
if ( (LA43_0==DEPRECATED||LA43_0==EXPERIMENTAL||LA43_0==LIBRARY||LA43_0==PLATFORM) ) {
alt43=1;
}
switch (alt43) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:482:83: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_typeDeclaration3388);
portabilityDirective138=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective138.getTree());
}
break;
default :
break loop43;
}
}
char_literal139=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_typeDeclaration3391); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal139);
// AST REWRITE
// elements: typeDecl, attributeList, genericNameDeclaration, portabilityDirective
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 483:30: -> ^( TkTypeDeclaration genericNameDeclaration typeDecl ( attributeList )? ( portabilityDirective )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:483:33: ^( TkTypeDeclaration genericNameDeclaration typeDecl ( attributeList )? ( portabilityDirective )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new TypeDeclarationNodeImpl(TkTypeDeclaration), root_1);
adaptor.addChild(root_1, stream_genericNameDeclaration.nextTree());
adaptor.addChild(root_1, stream_typeDecl.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:483:110: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:483:125: ( portabilityDirective )*
while ( stream_portabilityDirective.hasNext() ) {
adaptor.addChild(root_1, stream_portabilityDirective.nextTree());
}
stream_portabilityDirective.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 38, typeDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "typeDeclaration"
public static class varSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "varSection"
// au/com/integradev/delphi/antlr/Delphi.g:485:1: varSection : ( VAR ^| THREADVAR ^) varDeclaration ( varDeclaration )* ;
public final DelphiParser.varSection_return varSection() throws RecognitionException {
DelphiParser.varSection_return retval = new DelphiParser.varSection_return();
retval.start = input.LT(1);
int varSection_StartIndex = input.index();
Object root_0 = null;
Token VAR140=null;
Token THREADVAR141=null;
ParserRuleReturnScope varDeclaration142 =null;
ParserRuleReturnScope varDeclaration143 =null;
Object VAR140_tree=null;
Object THREADVAR141_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 39) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:485:30: ( ( VAR ^| THREADVAR ^) varDeclaration ( varDeclaration )* )
// au/com/integradev/delphi/antlr/Delphi.g:485:32: ( VAR ^| THREADVAR ^) varDeclaration ( varDeclaration )*
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:485:32: ( VAR ^| THREADVAR ^)
int alt44=2;
int LA44_0 = input.LA(1);
if ( (LA44_0==VAR) ) {
alt44=1;
}
else if ( (LA44_0==THREADVAR) ) {
alt44=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 44, 0, input);
throw nvae;
}
switch (alt44) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:485:33: VAR ^
{
VAR140=(Token)match(input,VAR,FOLLOW_VAR_in_varSection3495); if (state.failed) return retval;
if ( state.backtracking==0 ) {
VAR140_tree = new VarSectionNodeImpl(VAR140) ;
root_0 = (Object)adaptor.becomeRoot(VAR140_tree, root_0);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:485:60: THREADVAR ^
{
THREADVAR141=(Token)match(input,THREADVAR,FOLLOW_THREADVAR_in_varSection3503); if (state.failed) return retval;
if ( state.backtracking==0 ) {
THREADVAR141_tree = new VarSectionNodeImpl(THREADVAR141) ;
root_0 = (Object)adaptor.becomeRoot(THREADVAR141_tree, root_0);
}
}
break;
}
pushFollow(FOLLOW_varDeclaration_in_varSection3510);
varDeclaration142=varDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varDeclaration142.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:485:107: ( varDeclaration )*
loop45:
while (true) {
int alt45=2;
switch ( input.LA(1) ) {
case LABEL:
{
int LA45_4 = input.LA(2);
if ( (synpred58_Delphi()) ) {
alt45=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA45_10 = input.LA(2);
if ( (synpred58_Delphi()) ) {
alt45=1;
}
}
break;
case OPERATOR:
{
int LA45_12 = input.LA(2);
if ( (synpred58_Delphi()) ) {
alt45=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt45=1;
}
break;
}
switch (alt45) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:485:107: varDeclaration
{
pushFollow(FOLLOW_varDeclaration_in_varSection3512);
varDeclaration143=varDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varDeclaration143.getTree());
}
break;
default :
break loop45;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 39, varSection_StartIndex); }
}
return retval;
}
// $ANTLR end "varSection"
public static class varDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "varDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:487:1: varDeclaration : ( ( attributeList )? nameDeclarationList ':' fixedArrayType ( portabilityDirective )* ( arrayVarValueSpec )? ( portabilityDirective )* ';' -> ^( TkVarDeclaration nameDeclarationList fixedArrayType ( arrayVarValueSpec )? ( attributeList )? ) | ( attributeList )? nameDeclarationList ':' varType ( portabilityDirective )* ( varValueSpec )? ( portabilityDirective )* ';' -> ^( TkVarDeclaration nameDeclarationList varType ( varValueSpec )? ( attributeList )? ) );
public final DelphiParser.varDeclaration_return varDeclaration() throws RecognitionException {
DelphiParser.varDeclaration_return retval = new DelphiParser.varDeclaration_return();
retval.start = input.LT(1);
int varDeclaration_StartIndex = input.index();
Object root_0 = null;
Token char_literal146=null;
Token char_literal151=null;
Token char_literal154=null;
Token char_literal159=null;
ParserRuleReturnScope attributeList144 =null;
ParserRuleReturnScope nameDeclarationList145 =null;
ParserRuleReturnScope fixedArrayType147 =null;
ParserRuleReturnScope portabilityDirective148 =null;
ParserRuleReturnScope arrayVarValueSpec149 =null;
ParserRuleReturnScope portabilityDirective150 =null;
ParserRuleReturnScope attributeList152 =null;
ParserRuleReturnScope nameDeclarationList153 =null;
ParserRuleReturnScope varType155 =null;
ParserRuleReturnScope portabilityDirective156 =null;
ParserRuleReturnScope varValueSpec157 =null;
ParserRuleReturnScope portabilityDirective158 =null;
Object char_literal146_tree=null;
Object char_literal151_tree=null;
Object char_literal154_tree=null;
Object char_literal159_tree=null;
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_fixedArrayType=new RewriteRuleSubtreeStream(adaptor,"rule fixedArrayType");
RewriteRuleSubtreeStream stream_portabilityDirective=new RewriteRuleSubtreeStream(adaptor,"rule portabilityDirective");
RewriteRuleSubtreeStream stream_nameDeclarationList=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclarationList");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_arrayVarValueSpec=new RewriteRuleSubtreeStream(adaptor,"rule arrayVarValueSpec");
RewriteRuleSubtreeStream stream_varValueSpec=new RewriteRuleSubtreeStream(adaptor,"rule varValueSpec");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 40) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:487:30: ( ( attributeList )? nameDeclarationList ':' fixedArrayType ( portabilityDirective )* ( arrayVarValueSpec )? ( portabilityDirective )* ';' -> ^( TkVarDeclaration nameDeclarationList fixedArrayType ( arrayVarValueSpec )? ( attributeList )? ) | ( attributeList )? nameDeclarationList ':' varType ( portabilityDirective )* ( varValueSpec )? ( portabilityDirective )* ';' -> ^( TkVarDeclaration nameDeclarationList varType ( varValueSpec )? ( attributeList )? ) )
int alt54=2;
switch ( input.LA(1) ) {
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA54_1 = input.LA(2);
if ( (synpred63_Delphi()) ) {
alt54=1;
}
else if ( (true) ) {
alt54=2;
}
}
break;
case TkIdentifier:
{
int LA54_2 = input.LA(2);
if ( (synpred63_Delphi()) ) {
alt54=1;
}
else if ( (true) ) {
alt54=2;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA54_3 = input.LA(2);
if ( (synpred63_Delphi()) ) {
alt54=1;
}
else if ( (true) ) {
alt54=2;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 54, 0, input);
throw nvae;
}
switch (alt54) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:32: ( attributeList )? nameDeclarationList ':' fixedArrayType ( portabilityDirective )* ( arrayVarValueSpec )? ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:487:32: ( attributeList )?
int alt46=2;
int LA46_0 = input.LA(1);
if ( (LA46_0==PAREN_BRACKET_LEFT||LA46_0==SQUARE_BRACKET_LEFT) ) {
alt46=1;
}
switch (alt46) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_varDeclaration3564);
attributeList144=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList144.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclarationList_in_varDeclaration3567);
nameDeclarationList145=nameDeclarationList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclarationList.add(nameDeclarationList145.getTree());
char_literal146=(Token)match(input,COLON,FOLLOW_COLON_in_varDeclaration3569); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal146);
pushFollow(FOLLOW_fixedArrayType_in_varDeclaration3571);
fixedArrayType147=fixedArrayType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_fixedArrayType.add(fixedArrayType147.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:487:86: ( portabilityDirective )*
loop47:
while (true) {
int alt47=2;
switch ( input.LA(1) ) {
case DEPRECATED:
{
int LA47_2 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt47=1;
}
}
break;
case EXPERIMENTAL:
{
int LA47_3 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt47=1;
}
}
break;
case PLATFORM:
{
int LA47_4 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt47=1;
}
}
break;
case LIBRARY:
{
int LA47_5 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt47=1;
}
}
break;
}
switch (alt47) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:86: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_varDeclaration3573);
portabilityDirective148=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective148.getTree());
}
break;
default :
break loop47;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:487:108: ( arrayVarValueSpec )?
int alt48=2;
int LA48_0 = input.LA(1);
if ( (LA48_0==ABSOLUTE||LA48_0==EQUAL) ) {
alt48=1;
}
switch (alt48) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:108: arrayVarValueSpec
{
pushFollow(FOLLOW_arrayVarValueSpec_in_varDeclaration3576);
arrayVarValueSpec149=arrayVarValueSpec();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arrayVarValueSpec.add(arrayVarValueSpec149.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:487:127: ( portabilityDirective )*
loop49:
while (true) {
int alt49=2;
int LA49_0 = input.LA(1);
if ( (LA49_0==DEPRECATED||LA49_0==EXPERIMENTAL||LA49_0==LIBRARY||LA49_0==PLATFORM) ) {
alt49=1;
}
switch (alt49) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:127: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_varDeclaration3579);
portabilityDirective150=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective150.getTree());
}
break;
default :
break loop49;
}
}
char_literal151=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_varDeclaration3582); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal151);
// AST REWRITE
// elements: attributeList, nameDeclarationList, fixedArrayType, arrayVarValueSpec
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 488:30: -> ^( TkVarDeclaration nameDeclarationList fixedArrayType ( arrayVarValueSpec )? ( attributeList )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:488:33: ^( TkVarDeclaration nameDeclarationList fixedArrayType ( arrayVarValueSpec )? ( attributeList )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new VarDeclarationNodeImpl(TkVarDeclaration), root_1);
adaptor.addChild(root_1, stream_nameDeclarationList.nextTree());
adaptor.addChild(root_1, stream_fixedArrayType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:488:111: ( arrayVarValueSpec )?
if ( stream_arrayVarValueSpec.hasNext() ) {
adaptor.addChild(root_1, stream_arrayVarValueSpec.nextTree());
}
stream_arrayVarValueSpec.reset();
// au/com/integradev/delphi/antlr/Delphi.g:488:130: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:489:32: ( attributeList )? nameDeclarationList ':' varType ( portabilityDirective )* ( varValueSpec )? ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:489:32: ( attributeList )?
int alt50=2;
int LA50_0 = input.LA(1);
if ( (LA50_0==PAREN_BRACKET_LEFT||LA50_0==SQUARE_BRACKET_LEFT) ) {
alt50=1;
}
switch (alt50) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:489:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_varDeclaration3663);
attributeList152=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList152.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclarationList_in_varDeclaration3666);
nameDeclarationList153=nameDeclarationList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclarationList.add(nameDeclarationList153.getTree());
char_literal154=(Token)match(input,COLON,FOLLOW_COLON_in_varDeclaration3668); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal154);
pushFollow(FOLLOW_varType_in_varDeclaration3670);
varType155=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType155.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:489:79: ( portabilityDirective )*
loop51:
while (true) {
int alt51=2;
switch ( input.LA(1) ) {
case DEPRECATED:
{
int LA51_2 = input.LA(2);
if ( (synpred65_Delphi()) ) {
alt51=1;
}
}
break;
case EXPERIMENTAL:
{
int LA51_3 = input.LA(2);
if ( (synpred65_Delphi()) ) {
alt51=1;
}
}
break;
case PLATFORM:
{
int LA51_4 = input.LA(2);
if ( (synpred65_Delphi()) ) {
alt51=1;
}
}
break;
case LIBRARY:
{
int LA51_5 = input.LA(2);
if ( (synpred65_Delphi()) ) {
alt51=1;
}
}
break;
}
switch (alt51) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:489:79: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_varDeclaration3672);
portabilityDirective156=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective156.getTree());
}
break;
default :
break loop51;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:489:101: ( varValueSpec )?
int alt52=2;
int LA52_0 = input.LA(1);
if ( (LA52_0==ABSOLUTE||LA52_0==EQUAL) ) {
alt52=1;
}
switch (alt52) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:489:101: varValueSpec
{
pushFollow(FOLLOW_varValueSpec_in_varDeclaration3675);
varValueSpec157=varValueSpec();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varValueSpec.add(varValueSpec157.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:489:115: ( portabilityDirective )*
loop53:
while (true) {
int alt53=2;
int LA53_0 = input.LA(1);
if ( (LA53_0==DEPRECATED||LA53_0==EXPERIMENTAL||LA53_0==LIBRARY||LA53_0==PLATFORM) ) {
alt53=1;
}
switch (alt53) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:489:115: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_varDeclaration3678);
portabilityDirective158=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective158.getTree());
}
break;
default :
break loop53;
}
}
char_literal159=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_varDeclaration3681); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal159);
// AST REWRITE
// elements: varType, nameDeclarationList, attributeList, varValueSpec
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 490:30: -> ^( TkVarDeclaration nameDeclarationList varType ( varValueSpec )? ( attributeList )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:490:33: ^( TkVarDeclaration nameDeclarationList varType ( varValueSpec )? ( attributeList )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new VarDeclarationNodeImpl(TkVarDeclaration), root_1);
adaptor.addChild(root_1, stream_nameDeclarationList.nextTree());
adaptor.addChild(root_1, stream_varType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:490:104: ( varValueSpec )?
if ( stream_varValueSpec.hasNext() ) {
adaptor.addChild(root_1, stream_varValueSpec.nextTree());
}
stream_varValueSpec.reset();
// au/com/integradev/delphi/antlr/Delphi.g:490:118: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 40, varDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "varDeclaration"
public static class arrayVarValueSpec_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayVarValueSpec"
// au/com/integradev/delphi/antlr/Delphi.g:492:1: arrayVarValueSpec : ( ABSOLUTE expression | '=' arrayExpression );
public final DelphiParser.arrayVarValueSpec_return arrayVarValueSpec() throws RecognitionException {
DelphiParser.arrayVarValueSpec_return retval = new DelphiParser.arrayVarValueSpec_return();
retval.start = input.LT(1);
int arrayVarValueSpec_StartIndex = input.index();
Object root_0 = null;
Token ABSOLUTE160=null;
Token char_literal162=null;
ParserRuleReturnScope expression161 =null;
ParserRuleReturnScope arrayExpression163 =null;
Object ABSOLUTE160_tree=null;
Object char_literal162_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 41) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:492:30: ( ABSOLUTE expression | '=' arrayExpression )
int alt55=2;
int LA55_0 = input.LA(1);
if ( (LA55_0==ABSOLUTE) ) {
alt55=1;
}
else if ( (LA55_0==EQUAL) ) {
alt55=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 55, 0, input);
throw nvae;
}
switch (alt55) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:492:32: ABSOLUTE expression
{
root_0 = (Object)adaptor.nil();
ABSOLUTE160=(Token)match(input,ABSOLUTE,FOLLOW_ABSOLUTE_in_arrayVarValueSpec3777); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ABSOLUTE160_tree = (Object)adaptor.create(ABSOLUTE160);
adaptor.addChild(root_0, ABSOLUTE160_tree);
}
pushFollow(FOLLOW_expression_in_arrayVarValueSpec3779);
expression161=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression161.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:493:32: '=' arrayExpression
{
root_0 = (Object)adaptor.nil();
char_literal162=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_arrayVarValueSpec3812); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal162_tree = (Object)adaptor.create(char_literal162);
adaptor.addChild(root_0, char_literal162_tree);
}
pushFollow(FOLLOW_arrayExpression_in_arrayVarValueSpec3814);
arrayExpression163=arrayExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayExpression163.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 41, arrayVarValueSpec_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayVarValueSpec"
public static class varValueSpec_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "varValueSpec"
// au/com/integradev/delphi/antlr/Delphi.g:495:1: varValueSpec : ( ABSOLUTE expression | '=' constExpression );
public final DelphiParser.varValueSpec_return varValueSpec() throws RecognitionException {
DelphiParser.varValueSpec_return retval = new DelphiParser.varValueSpec_return();
retval.start = input.LT(1);
int varValueSpec_StartIndex = input.index();
Object root_0 = null;
Token ABSOLUTE164=null;
Token char_literal166=null;
ParserRuleReturnScope expression165 =null;
ParserRuleReturnScope constExpression167 =null;
Object ABSOLUTE164_tree=null;
Object char_literal166_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 42) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:495:30: ( ABSOLUTE expression | '=' constExpression )
int alt56=2;
int LA56_0 = input.LA(1);
if ( (LA56_0==ABSOLUTE) ) {
alt56=1;
}
else if ( (LA56_0==EQUAL) ) {
alt56=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 56, 0, input);
throw nvae;
}
switch (alt56) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:495:32: ABSOLUTE expression
{
root_0 = (Object)adaptor.nil();
ABSOLUTE164=(Token)match(input,ABSOLUTE,FOLLOW_ABSOLUTE_in_varValueSpec3867); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ABSOLUTE164_tree = (Object)adaptor.create(ABSOLUTE164);
adaptor.addChild(root_0, ABSOLUTE164_tree);
}
pushFollow(FOLLOW_expression_in_varValueSpec3869);
expression165=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression165.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:496:32: '=' constExpression
{
root_0 = (Object)adaptor.nil();
char_literal166=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_varValueSpec3902); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal166_tree = (Object)adaptor.create(char_literal166);
adaptor.addChild(root_0, char_literal166_tree);
}
pushFollow(FOLLOW_constExpression_in_varValueSpec3904);
constExpression167=constExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constExpression167.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 42, varValueSpec_StartIndex); }
}
return retval;
}
// $ANTLR end "varValueSpec"
public static class exportsSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "exportsSection"
// au/com/integradev/delphi/antlr/Delphi.g:498:1: exportsSection : EXPORTS ident exportItem ( ',' ident exportItem )* ';' ;
public final DelphiParser.exportsSection_return exportsSection() throws RecognitionException {
DelphiParser.exportsSection_return retval = new DelphiParser.exportsSection_return();
retval.start = input.LT(1);
int exportsSection_StartIndex = input.index();
Object root_0 = null;
Token EXPORTS168=null;
Token char_literal171=null;
Token char_literal174=null;
ParserRuleReturnScope ident169 =null;
ParserRuleReturnScope exportItem170 =null;
ParserRuleReturnScope ident172 =null;
ParserRuleReturnScope exportItem173 =null;
Object EXPORTS168_tree=null;
Object char_literal171_tree=null;
Object char_literal174_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 43) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:498:30: ( EXPORTS ident exportItem ( ',' ident exportItem )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:498:32: EXPORTS ident exportItem ( ',' ident exportItem )* ';'
{
root_0 = (Object)adaptor.nil();
EXPORTS168=(Token)match(input,EXPORTS,FOLLOW_EXPORTS_in_exportsSection3955); if (state.failed) return retval;
if ( state.backtracking==0 ) {
EXPORTS168_tree = (Object)adaptor.create(EXPORTS168);
adaptor.addChild(root_0, EXPORTS168_tree);
}
pushFollow(FOLLOW_ident_in_exportsSection3957);
ident169=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident169.getTree());
pushFollow(FOLLOW_exportItem_in_exportsSection3959);
exportItem170=exportItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, exportItem170.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:498:57: ( ',' ident exportItem )*
loop57:
while (true) {
int alt57=2;
int LA57_0 = input.LA(1);
if ( (LA57_0==COMMA) ) {
alt57=1;
}
switch (alt57) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:498:58: ',' ident exportItem
{
char_literal171=(Token)match(input,COMMA,FOLLOW_COMMA_in_exportsSection3962); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal171_tree = (Object)adaptor.create(char_literal171);
adaptor.addChild(root_0, char_literal171_tree);
}
pushFollow(FOLLOW_ident_in_exportsSection3964);
ident172=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident172.getTree());
pushFollow(FOLLOW_exportItem_in_exportsSection3966);
exportItem173=exportItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, exportItem173.getTree());
}
break;
default :
break loop57;
}
}
char_literal174=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_exportsSection3970); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal174_tree = (Object)adaptor.create(char_literal174);
adaptor.addChild(root_0, char_literal174_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 43, exportsSection_StartIndex); }
}
return retval;
}
// $ANTLR end "exportsSection"
public static class exportItem_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "exportItem"
// au/com/integradev/delphi/antlr/Delphi.g:500:1: exportItem : ( '(' formalParameterList ')' )? ( INDEX expression )? ( NAME expression )? ( RESIDENT )? ;
public final DelphiParser.exportItem_return exportItem() throws RecognitionException {
DelphiParser.exportItem_return retval = new DelphiParser.exportItem_return();
retval.start = input.LT(1);
int exportItem_StartIndex = input.index();
Object root_0 = null;
Token char_literal175=null;
Token char_literal177=null;
Token INDEX178=null;
Token NAME180=null;
Token RESIDENT182=null;
ParserRuleReturnScope formalParameterList176 =null;
ParserRuleReturnScope expression179 =null;
ParserRuleReturnScope expression181 =null;
Object char_literal175_tree=null;
Object char_literal177_tree=null;
Object INDEX178_tree=null;
Object NAME180_tree=null;
Object RESIDENT182_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 44) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:500:30: ( ( '(' formalParameterList ')' )? ( INDEX expression )? ( NAME expression )? ( RESIDENT )? )
// au/com/integradev/delphi/antlr/Delphi.g:500:32: ( '(' formalParameterList ')' )? ( INDEX expression )? ( NAME expression )? ( RESIDENT )?
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:500:32: ( '(' formalParameterList ')' )?
int alt58=2;
int LA58_0 = input.LA(1);
if ( (LA58_0==PAREN_LEFT) ) {
alt58=1;
}
switch (alt58) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:500:33: '(' formalParameterList ')'
{
char_literal175=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_exportItem4026); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal175_tree = (Object)adaptor.create(char_literal175);
adaptor.addChild(root_0, char_literal175_tree);
}
pushFollow(FOLLOW_formalParameterList_in_exportItem4028);
formalParameterList176=formalParameterList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, formalParameterList176.getTree());
char_literal177=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_exportItem4030); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal177_tree = (Object)adaptor.create(char_literal177);
adaptor.addChild(root_0, char_literal177_tree);
}
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:500:63: ( INDEX expression )?
int alt59=2;
int LA59_0 = input.LA(1);
if ( (LA59_0==INDEX) ) {
alt59=1;
}
switch (alt59) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:500:64: INDEX expression
{
INDEX178=(Token)match(input,INDEX,FOLLOW_INDEX_in_exportItem4035); if (state.failed) return retval;
if ( state.backtracking==0 ) {
INDEX178_tree = (Object)adaptor.create(INDEX178);
adaptor.addChild(root_0, INDEX178_tree);
}
pushFollow(FOLLOW_expression_in_exportItem4037);
expression179=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression179.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:500:83: ( NAME expression )?
int alt60=2;
int LA60_0 = input.LA(1);
if ( (LA60_0==NAME) ) {
alt60=1;
}
switch (alt60) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:500:84: NAME expression
{
NAME180=(Token)match(input,NAME,FOLLOW_NAME_in_exportItem4042); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NAME180_tree = (Object)adaptor.create(NAME180);
adaptor.addChild(root_0, NAME180_tree);
}
pushFollow(FOLLOW_expression_in_exportItem4044);
expression181=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression181.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:500:102: ( RESIDENT )?
int alt61=2;
int LA61_0 = input.LA(1);
if ( (LA61_0==RESIDENT) ) {
alt61=1;
}
switch (alt61) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:500:103: RESIDENT
{
RESIDENT182=(Token)match(input,RESIDENT,FOLLOW_RESIDENT_in_exportItem4049); if (state.failed) return retval;
if ( state.backtracking==0 ) {
RESIDENT182_tree = (Object)adaptor.create(RESIDENT182);
adaptor.addChild(root_0, RESIDENT182_tree);
}
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 44, exportItem_StartIndex); }
}
return retval;
}
// $ANTLR end "exportItem"
public static class typeDecl_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeDecl"
// au/com/integradev/delphi/antlr/Delphi.g:506:1: typeDecl : ( arrayType | setType | fullFileType | classHelperType | classReferenceType | classType | interfaceType | objectType | recordType | recordHelperType | pointerType | fullStringType | procedureType | subRangeType | typeOfType | strongAliasType | weakAliasType | enumType | PACKED typeDecl ^);
public final DelphiParser.typeDecl_return typeDecl() throws RecognitionException {
DelphiParser.typeDecl_return retval = new DelphiParser.typeDecl_return();
retval.start = input.LT(1);
int typeDecl_StartIndex = input.index();
Object root_0 = null;
Token PACKED201=null;
ParserRuleReturnScope arrayType183 =null;
ParserRuleReturnScope setType184 =null;
ParserRuleReturnScope fullFileType185 =null;
ParserRuleReturnScope classHelperType186 =null;
ParserRuleReturnScope classReferenceType187 =null;
ParserRuleReturnScope classType188 =null;
ParserRuleReturnScope interfaceType189 =null;
ParserRuleReturnScope objectType190 =null;
ParserRuleReturnScope recordType191 =null;
ParserRuleReturnScope recordHelperType192 =null;
ParserRuleReturnScope pointerType193 =null;
ParserRuleReturnScope fullStringType194 =null;
ParserRuleReturnScope procedureType195 =null;
ParserRuleReturnScope subRangeType196 =null;
ParserRuleReturnScope typeOfType197 =null;
ParserRuleReturnScope strongAliasType198 =null;
ParserRuleReturnScope weakAliasType199 =null;
ParserRuleReturnScope enumType200 =null;
ParserRuleReturnScope typeDecl202 =null;
Object PACKED201_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 45) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:506:30: ( arrayType | setType | fullFileType | classHelperType | classReferenceType | classType | interfaceType | objectType | recordType | recordHelperType | pointerType | fullStringType | procedureType | subRangeType | typeOfType | strongAliasType | weakAliasType | enumType | PACKED typeDecl ^)
int alt62=19;
switch ( input.LA(1) ) {
case ARRAY:
{
alt62=1;
}
break;
case SET:
{
alt62=2;
}
break;
case FILE:
{
int LA62_3 = input.LA(2);
if ( (synpred77_Delphi()) ) {
alt62=3;
}
else if ( (synpred88_Delphi()) ) {
alt62=14;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case CLASS:
{
int LA62_4 = input.LA(2);
if ( (synpred78_Delphi()) ) {
alt62=4;
}
else if ( (synpred79_Delphi()) ) {
alt62=5;
}
else if ( (synpred80_Delphi()) ) {
alt62=6;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case DISPINTERFACE:
case INTERFACE:
{
alt62=7;
}
break;
case OBJECT:
{
alt62=8;
}
break;
case RECORD:
{
int LA62_8 = input.LA(2);
if ( (synpred83_Delphi()) ) {
alt62=9;
}
else if ( (synpred84_Delphi()) ) {
alt62=10;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 8, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case DEREFERENCE:
{
int LA62_9 = input.LA(2);
if ( (synpred85_Delphi()) ) {
alt62=11;
}
else if ( (synpred88_Delphi()) ) {
alt62=14;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 9, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case STRING:
{
int LA62_10 = input.LA(2);
if ( (synpred86_Delphi()) ) {
alt62=12;
}
else if ( (synpred88_Delphi()) ) {
alt62=14;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 10, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FUNCTION:
{
int LA62_11 = input.LA(2);
if ( (synpred87_Delphi()) ) {
alt62=13;
}
else if ( (synpred88_Delphi()) ) {
alt62=14;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 11, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PROCEDURE:
{
int LA62_12 = input.LA(2);
if ( (synpred87_Delphi()) ) {
alt62=13;
}
else if ( (synpred88_Delphi()) ) {
alt62=14;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 12, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case REFERENCE:
{
int LA62_13 = input.LA(2);
if ( (synpred87_Delphi()) ) {
alt62=13;
}
else if ( (synpred88_Delphi()) ) {
alt62=14;
}
else if ( (synpred91_Delphi()) ) {
alt62=17;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 13, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ADDRESS:
case INHERITED:
case MINUS:
case NIL:
case NOT:
case PAREN_BRACKET_LEFT:
case PLUS:
case SQUARE_BRACKET_LEFT:
case TkBinaryNumber:
case TkCharacterEscapeCode:
case TkHexNumber:
case TkIntNumber:
case TkMultilineString:
case TkQuotedString:
case TkRealNumber:
{
alt62=14;
}
break;
case TkIdentifier:
{
int LA62_26 = input.LA(2);
if ( (synpred88_Delphi()) ) {
alt62=14;
}
else if ( (synpred91_Delphi()) ) {
alt62=17;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 26, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA62_27 = input.LA(2);
if ( (synpred88_Delphi()) ) {
alt62=14;
}
else if ( (synpred91_Delphi()) ) {
alt62=17;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 27, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PAREN_LEFT:
{
int LA62_29 = input.LA(2);
if ( (synpred88_Delphi()) ) {
alt62=14;
}
else if ( (synpred92_Delphi()) ) {
alt62=18;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 29, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TYPE:
{
int LA62_31 = input.LA(2);
if ( (synpred89_Delphi()) ) {
alt62=15;
}
else if ( (synpred90_Delphi()) ) {
alt62=16;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 62, 31, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PACKED:
{
alt62=19;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 62, 0, input);
throw nvae;
}
switch (alt62) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:506:32: arrayType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_arrayType_in_typeDecl4112);
arrayType183=arrayType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayType183.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:507:32: setType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_setType_in_typeDecl4145);
setType184=setType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, setType184.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:508:32: fullFileType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fullFileType_in_typeDecl4178);
fullFileType185=fullFileType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fullFileType185.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:509:32: classHelperType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_classHelperType_in_typeDecl4211);
classHelperType186=classHelperType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, classHelperType186.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:510:32: classReferenceType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_classReferenceType_in_typeDecl4244);
classReferenceType187=classReferenceType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, classReferenceType187.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:511:32: classType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_classType_in_typeDecl4277);
classType188=classType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, classType188.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:512:32: interfaceType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_interfaceType_in_typeDecl4310);
interfaceType189=interfaceType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceType189.getTree());
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:513:32: objectType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_objectType_in_typeDecl4343);
objectType190=objectType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, objectType190.getTree());
}
break;
case 9 :
// au/com/integradev/delphi/antlr/Delphi.g:514:32: recordType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_recordType_in_typeDecl4376);
recordType191=recordType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordType191.getTree());
}
break;
case 10 :
// au/com/integradev/delphi/antlr/Delphi.g:515:32: recordHelperType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_recordHelperType_in_typeDecl4409);
recordHelperType192=recordHelperType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordHelperType192.getTree());
}
break;
case 11 :
// au/com/integradev/delphi/antlr/Delphi.g:516:32: pointerType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_pointerType_in_typeDecl4442);
pointerType193=pointerType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, pointerType193.getTree());
}
break;
case 12 :
// au/com/integradev/delphi/antlr/Delphi.g:517:32: fullStringType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fullStringType_in_typeDecl4475);
fullStringType194=fullStringType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fullStringType194.getTree());
}
break;
case 13 :
// au/com/integradev/delphi/antlr/Delphi.g:518:32: procedureType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_procedureType_in_typeDecl4508);
procedureType195=procedureType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, procedureType195.getTree());
}
break;
case 14 :
// au/com/integradev/delphi/antlr/Delphi.g:519:32: subRangeType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_subRangeType_in_typeDecl4541);
subRangeType196=subRangeType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, subRangeType196.getTree());
}
break;
case 15 :
// au/com/integradev/delphi/antlr/Delphi.g:520:32: typeOfType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeOfType_in_typeDecl4574);
typeOfType197=typeOfType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeOfType197.getTree());
}
break;
case 16 :
// au/com/integradev/delphi/antlr/Delphi.g:521:32: strongAliasType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_strongAliasType_in_typeDecl4607);
strongAliasType198=strongAliasType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, strongAliasType198.getTree());
}
break;
case 17 :
// au/com/integradev/delphi/antlr/Delphi.g:522:32: weakAliasType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_weakAliasType_in_typeDecl4640);
weakAliasType199=weakAliasType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, weakAliasType199.getTree());
}
break;
case 18 :
// au/com/integradev/delphi/antlr/Delphi.g:523:32: enumType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_enumType_in_typeDecl4673);
enumType200=enumType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, enumType200.getTree());
}
break;
case 19 :
// au/com/integradev/delphi/antlr/Delphi.g:524:32: PACKED typeDecl ^
{
root_0 = (Object)adaptor.nil();
PACKED201=(Token)match(input,PACKED,FOLLOW_PACKED_in_typeDecl4706); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PACKED201_tree = (Object)adaptor.create(PACKED201);
adaptor.addChild(root_0, PACKED201_tree);
}
pushFollow(FOLLOW_typeDecl_in_typeDecl4708);
typeDecl202=typeDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(typeDecl202.getTree(), root_0);
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 45, typeDecl_StartIndex); }
}
return retval;
}
// $ANTLR end "typeDecl"
public static class varType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "varType"
// au/com/integradev/delphi/antlr/Delphi.g:526:1: varType : ( arrayType | setType | fullStringType | fullFileType | recordType | pointerType | procedureType | subRangeType | typeReference | enumType | PACKED varType ^);
public final DelphiParser.varType_return varType() throws RecognitionException {
DelphiParser.varType_return retval = new DelphiParser.varType_return();
retval.start = input.LT(1);
int varType_StartIndex = input.index();
Object root_0 = null;
Token PACKED213=null;
ParserRuleReturnScope arrayType203 =null;
ParserRuleReturnScope setType204 =null;
ParserRuleReturnScope fullStringType205 =null;
ParserRuleReturnScope fullFileType206 =null;
ParserRuleReturnScope recordType207 =null;
ParserRuleReturnScope pointerType208 =null;
ParserRuleReturnScope procedureType209 =null;
ParserRuleReturnScope subRangeType210 =null;
ParserRuleReturnScope typeReference211 =null;
ParserRuleReturnScope enumType212 =null;
ParserRuleReturnScope varType214 =null;
Object PACKED213_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 46) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:526:30: ( arrayType | setType | fullStringType | fullFileType | recordType | pointerType | procedureType | subRangeType | typeReference | enumType | PACKED varType ^)
int alt63=11;
switch ( input.LA(1) ) {
case ARRAY:
{
alt63=1;
}
break;
case SET:
{
alt63=2;
}
break;
case STRING:
{
int LA63_3 = input.LA(2);
if ( (synpred95_Delphi()) ) {
alt63=3;
}
else if ( (synpred100_Delphi()) ) {
alt63=8;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FILE:
{
int LA63_4 = input.LA(2);
if ( (synpred96_Delphi()) ) {
alt63=4;
}
else if ( (synpred100_Delphi()) ) {
alt63=8;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case RECORD:
{
alt63=5;
}
break;
case DEREFERENCE:
{
int LA63_6 = input.LA(2);
if ( (synpred98_Delphi()) ) {
alt63=6;
}
else if ( (synpred100_Delphi()) ) {
alt63=8;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 6, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FUNCTION:
{
int LA63_7 = input.LA(2);
if ( (synpred99_Delphi()) ) {
alt63=7;
}
else if ( (synpred100_Delphi()) ) {
alt63=8;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 7, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PROCEDURE:
{
int LA63_8 = input.LA(2);
if ( (synpred99_Delphi()) ) {
alt63=7;
}
else if ( (synpred100_Delphi()) ) {
alt63=8;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 8, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case REFERENCE:
{
int LA63_9 = input.LA(2);
if ( (synpred99_Delphi()) ) {
alt63=7;
}
else if ( (synpred100_Delphi()) ) {
alt63=8;
}
else if ( (synpred101_Delphi()) ) {
alt63=9;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 9, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ADDRESS:
case INHERITED:
case MINUS:
case NIL:
case NOT:
case PAREN_BRACKET_LEFT:
case PLUS:
case SQUARE_BRACKET_LEFT:
case TkBinaryNumber:
case TkCharacterEscapeCode:
case TkHexNumber:
case TkIntNumber:
case TkMultilineString:
case TkQuotedString:
case TkRealNumber:
{
alt63=8;
}
break;
case TkIdentifier:
{
int LA63_22 = input.LA(2);
if ( (synpred100_Delphi()) ) {
alt63=8;
}
else if ( (synpred101_Delphi()) ) {
alt63=9;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 22, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA63_23 = input.LA(2);
if ( (synpred100_Delphi()) ) {
alt63=8;
}
else if ( (synpred101_Delphi()) ) {
alt63=9;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 23, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PAREN_LEFT:
{
int LA63_25 = input.LA(2);
if ( (synpred100_Delphi()) ) {
alt63=8;
}
else if ( (synpred102_Delphi()) ) {
alt63=10;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 63, 25, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PACKED:
{
alt63=11;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 63, 0, input);
throw nvae;
}
switch (alt63) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:526:32: arrayType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_arrayType_in_varType4767);
arrayType203=arrayType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayType203.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:527:32: setType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_setType_in_varType4800);
setType204=setType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, setType204.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:528:32: fullStringType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fullStringType_in_varType4833);
fullStringType205=fullStringType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fullStringType205.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:529:32: fullFileType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fullFileType_in_varType4866);
fullFileType206=fullFileType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fullFileType206.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:530:32: recordType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_recordType_in_varType4899);
recordType207=recordType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordType207.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:531:32: pointerType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_pointerType_in_varType4932);
pointerType208=pointerType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, pointerType208.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:532:32: procedureType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_procedureType_in_varType4965);
procedureType209=procedureType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, procedureType209.getTree());
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:533:32: subRangeType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_subRangeType_in_varType4998);
subRangeType210=subRangeType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, subRangeType210.getTree());
}
break;
case 9 :
// au/com/integradev/delphi/antlr/Delphi.g:534:32: typeReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeReference_in_varType5031);
typeReference211=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference211.getTree());
}
break;
case 10 :
// au/com/integradev/delphi/antlr/Delphi.g:535:32: enumType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_enumType_in_varType5064);
enumType212=enumType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, enumType212.getTree());
}
break;
case 11 :
// au/com/integradev/delphi/antlr/Delphi.g:536:32: PACKED varType ^
{
root_0 = (Object)adaptor.nil();
PACKED213=(Token)match(input,PACKED,FOLLOW_PACKED_in_varType5097); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PACKED213_tree = (Object)adaptor.create(PACKED213);
adaptor.addChild(root_0, PACKED213_tree);
}
pushFollow(FOLLOW_varType_in_varType5099);
varType214=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(varType214.getTree(), root_0);
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 46, varType_StartIndex); }
}
return retval;
}
// $ANTLR end "varType"
public static class parameterType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "parameterType"
// au/com/integradev/delphi/antlr/Delphi.g:538:1: parameterType : ( stringType | fileType | arrayType | typeReference | PACKED parameterType ^);
public final DelphiParser.parameterType_return parameterType() throws RecognitionException {
DelphiParser.parameterType_return retval = new DelphiParser.parameterType_return();
retval.start = input.LT(1);
int parameterType_StartIndex = input.index();
Object root_0 = null;
Token PACKED219=null;
ParserRuleReturnScope stringType215 =null;
ParserRuleReturnScope fileType216 =null;
ParserRuleReturnScope arrayType217 =null;
ParserRuleReturnScope typeReference218 =null;
ParserRuleReturnScope parameterType220 =null;
Object PACKED219_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 47) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:538:30: ( stringType | fileType | arrayType | typeReference | PACKED parameterType ^)
int alt64=5;
switch ( input.LA(1) ) {
case STRING:
{
alt64=1;
}
break;
case FILE:
{
alt64=2;
}
break;
case ARRAY:
{
alt64=3;
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt64=4;
}
break;
case PACKED:
{
alt64=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 64, 0, input);
throw nvae;
}
switch (alt64) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:538:32: stringType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_stringType_in_parameterType5152);
stringType215=stringType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, stringType215.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:539:32: fileType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fileType_in_parameterType5185);
fileType216=fileType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fileType216.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:540:32: arrayType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_arrayType_in_parameterType5218);
arrayType217=arrayType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayType217.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:541:32: typeReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeReference_in_parameterType5251);
typeReference218=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference218.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:542:32: PACKED parameterType ^
{
root_0 = (Object)adaptor.nil();
PACKED219=(Token)match(input,PACKED,FOLLOW_PACKED_in_parameterType5284); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PACKED219_tree = (Object)adaptor.create(PACKED219);
adaptor.addChild(root_0, PACKED219_tree);
}
pushFollow(FOLLOW_parameterType_in_parameterType5286);
parameterType220=parameterType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(parameterType220.getTree(), root_0);
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 47, parameterType_StartIndex); }
}
return retval;
}
// $ANTLR end "parameterType"
public static class fixedArrayType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fixedArrayType"
// au/com/integradev/delphi/antlr/Delphi.g:544:1: fixedArrayType : ARRAY arrayIndices OF arrayElementType -> ^( ARRAY OF arrayElementType arrayIndices ) ;
public final DelphiParser.fixedArrayType_return fixedArrayType() throws RecognitionException {
DelphiParser.fixedArrayType_return retval = new DelphiParser.fixedArrayType_return();
retval.start = input.LT(1);
int fixedArrayType_StartIndex = input.index();
Object root_0 = null;
Token ARRAY221=null;
Token OF223=null;
ParserRuleReturnScope arrayIndices222 =null;
ParserRuleReturnScope arrayElementType224 =null;
Object ARRAY221_tree=null;
Object OF223_tree=null;
RewriteRuleTokenStream stream_ARRAY=new RewriteRuleTokenStream(adaptor,"token ARRAY");
RewriteRuleTokenStream stream_OF=new RewriteRuleTokenStream(adaptor,"token OF");
RewriteRuleSubtreeStream stream_arrayElementType=new RewriteRuleSubtreeStream(adaptor,"rule arrayElementType");
RewriteRuleSubtreeStream stream_arrayIndices=new RewriteRuleSubtreeStream(adaptor,"rule arrayIndices");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 48) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:544:30: ( ARRAY arrayIndices OF arrayElementType -> ^( ARRAY OF arrayElementType arrayIndices ) )
// au/com/integradev/delphi/antlr/Delphi.g:544:32: ARRAY arrayIndices OF arrayElementType
{
ARRAY221=(Token)match(input,ARRAY,FOLLOW_ARRAY_in_fixedArrayType5338); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ARRAY.add(ARRAY221);
pushFollow(FOLLOW_arrayIndices_in_fixedArrayType5340);
arrayIndices222=arrayIndices();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arrayIndices.add(arrayIndices222.getTree());
OF223=(Token)match(input,OF,FOLLOW_OF_in_fixedArrayType5342); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_OF.add(OF223);
pushFollow(FOLLOW_arrayElementType_in_fixedArrayType5344);
arrayElementType224=arrayElementType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arrayElementType.add(arrayElementType224.getTree());
// AST REWRITE
// elements: arrayElementType, OF, ARRAY, arrayIndices
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 545:30: -> ^( ARRAY OF arrayElementType arrayIndices )
{
// au/com/integradev/delphi/antlr/Delphi.g:545:33: ^( ARRAY OF arrayElementType arrayIndices )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ArrayTypeNodeImpl(stream_ARRAY.nextToken()), root_1);
adaptor.addChild(root_1, stream_OF.nextNode());
adaptor.addChild(root_1, stream_arrayElementType.nextTree());
adaptor.addChild(root_1, stream_arrayIndices.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 48, fixedArrayType_StartIndex); }
}
return retval;
}
// $ANTLR end "fixedArrayType"
public static class arrayType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayType"
// au/com/integradev/delphi/antlr/Delphi.g:547:1: arrayType : ARRAY ( arrayIndices )? OF arrayElementType -> ^( ARRAY OF arrayElementType ( arrayIndices )? ) ;
public final DelphiParser.arrayType_return arrayType() throws RecognitionException {
DelphiParser.arrayType_return retval = new DelphiParser.arrayType_return();
retval.start = input.LT(1);
int arrayType_StartIndex = input.index();
Object root_0 = null;
Token ARRAY225=null;
Token OF227=null;
ParserRuleReturnScope arrayIndices226 =null;
ParserRuleReturnScope arrayElementType228 =null;
Object ARRAY225_tree=null;
Object OF227_tree=null;
RewriteRuleTokenStream stream_ARRAY=new RewriteRuleTokenStream(adaptor,"token ARRAY");
RewriteRuleTokenStream stream_OF=new RewriteRuleTokenStream(adaptor,"token OF");
RewriteRuleSubtreeStream stream_arrayElementType=new RewriteRuleSubtreeStream(adaptor,"rule arrayElementType");
RewriteRuleSubtreeStream stream_arrayIndices=new RewriteRuleSubtreeStream(adaptor,"rule arrayIndices");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 49) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:547:30: ( ARRAY ( arrayIndices )? OF arrayElementType -> ^( ARRAY OF arrayElementType ( arrayIndices )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:547:33: ARRAY ( arrayIndices )? OF arrayElementType
{
ARRAY225=(Token)match(input,ARRAY,FOLLOW_ARRAY_in_arrayType5446); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ARRAY.add(ARRAY225);
// au/com/integradev/delphi/antlr/Delphi.g:547:39: ( arrayIndices )?
int alt65=2;
int LA65_0 = input.LA(1);
if ( (LA65_0==PAREN_BRACKET_LEFT||LA65_0==SQUARE_BRACKET_LEFT) ) {
alt65=1;
}
switch (alt65) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:547:39: arrayIndices
{
pushFollow(FOLLOW_arrayIndices_in_arrayType5448);
arrayIndices226=arrayIndices();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arrayIndices.add(arrayIndices226.getTree());
}
break;
}
OF227=(Token)match(input,OF,FOLLOW_OF_in_arrayType5451); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_OF.add(OF227);
pushFollow(FOLLOW_arrayElementType_in_arrayType5453);
arrayElementType228=arrayElementType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arrayElementType.add(arrayElementType228.getTree());
// AST REWRITE
// elements: arrayIndices, ARRAY, arrayElementType, OF
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 548:30: -> ^( ARRAY OF arrayElementType ( arrayIndices )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:548:33: ^( ARRAY OF arrayElementType ( arrayIndices )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ArrayTypeNodeImpl(stream_ARRAY.nextToken()), root_1);
adaptor.addChild(root_1, stream_OF.nextNode());
adaptor.addChild(root_1, stream_arrayElementType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:548:80: ( arrayIndices )?
if ( stream_arrayIndices.hasNext() ) {
adaptor.addChild(root_1, stream_arrayIndices.nextTree());
}
stream_arrayIndices.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 49, arrayType_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayType"
public static class lbrack_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "lbrack"
// au/com/integradev/delphi/antlr/Delphi.g:550:1: lbrack : ( '[' | '(.' );
public final DelphiParser.lbrack_return lbrack() throws RecognitionException {
DelphiParser.lbrack_return retval = new DelphiParser.lbrack_return();
retval.start = input.LT(1);
int lbrack_StartIndex = input.index();
Object root_0 = null;
Token set229=null;
Object set229_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 50) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:550:30: ( '[' | '(.' )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set229=input.LT(1);
if ( input.LA(1)==PAREN_BRACKET_LEFT||input.LA(1)==SQUARE_BRACKET_LEFT ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set229));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 50, lbrack_StartIndex); }
}
return retval;
}
// $ANTLR end "lbrack"
public static class rbrack_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "rbrack"
// au/com/integradev/delphi/antlr/Delphi.g:553:1: rbrack : ( ']' | '.)' );
public final DelphiParser.rbrack_return rbrack() throws RecognitionException {
DelphiParser.rbrack_return retval = new DelphiParser.rbrack_return();
retval.start = input.LT(1);
int rbrack_StartIndex = input.index();
Object root_0 = null;
Token set230=null;
Object set230_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 51) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:553:30: ( ']' | '.)' )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set230=input.LT(1);
if ( input.LA(1)==PAREN_BRACKET_RIGHT||input.LA(1)==SQUARE_BRACKET_RIGHT ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set230));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 51, rbrack_StartIndex); }
}
return retval;
}
// $ANTLR end "rbrack"
public static class arrayIndices_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayIndices"
// au/com/integradev/delphi/antlr/Delphi.g:556:1: arrayIndices : lbrack ( varType ( ',' )? )+ rbrack -> ^( TkArrayIndices lbrack ( varType ( ',' )? )+ rbrack ) ;
public final DelphiParser.arrayIndices_return arrayIndices() throws RecognitionException {
DelphiParser.arrayIndices_return retval = new DelphiParser.arrayIndices_return();
retval.start = input.LT(1);
int arrayIndices_StartIndex = input.index();
Object root_0 = null;
Token char_literal233=null;
ParserRuleReturnScope lbrack231 =null;
ParserRuleReturnScope varType232 =null;
ParserRuleReturnScope rbrack234 =null;
Object char_literal233_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_rbrack=new RewriteRuleSubtreeStream(adaptor,"rule rbrack");
RewriteRuleSubtreeStream stream_lbrack=new RewriteRuleSubtreeStream(adaptor,"rule lbrack");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 52) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:556:30: ( lbrack ( varType ( ',' )? )+ rbrack -> ^( TkArrayIndices lbrack ( varType ( ',' )? )+ rbrack ) )
// au/com/integradev/delphi/antlr/Delphi.g:556:32: lbrack ( varType ( ',' )? )+ rbrack
{
pushFollow(FOLLOW_lbrack_in_arrayIndices5736);
lbrack231=lbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_lbrack.add(lbrack231.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:556:39: ( varType ( ',' )? )+
int cnt67=0;
loop67:
while (true) {
int alt67=2;
int LA67_0 = input.LA(1);
if ( ((LA67_0 >= ABSOLUTE && LA67_0 <= ALIGN)||LA67_0==ARRAY||(LA67_0 >= ASSEMBLER && LA67_0 <= ASSEMBLY)||(LA67_0 >= AT && LA67_0 <= AUTOMATED)||LA67_0==CDECL||LA67_0==CONTAINS||(LA67_0 >= DEFAULT && LA67_0 <= DEREFERENCE)||LA67_0==DISPID||LA67_0==DYNAMIC||(LA67_0 >= EXPERIMENTAL && LA67_0 <= EXPORT)||LA67_0==EXTERNAL||(LA67_0 >= FAR && LA67_0 <= FINAL)||(LA67_0 >= FORWARD && LA67_0 <= FUNCTION)||LA67_0==HELPER||LA67_0==IMPLEMENTS||(LA67_0 >= INDEX && LA67_0 <= INHERITED)||LA67_0==LABEL||LA67_0==LOCAL||(LA67_0 >= MESSAGE && LA67_0 <= MINUS)||(LA67_0 >= NAME && LA67_0 <= NOT)||(LA67_0 >= ON && LA67_0 <= OPERATOR)||(LA67_0 >= OUT && LA67_0 <= OVERRIDE)||(LA67_0 >= PACKAGE && LA67_0 <= PAREN_BRACKET_LEFT)||LA67_0==PAREN_LEFT||(LA67_0 >= PASCAL && LA67_0 <= PROCEDURE)||(LA67_0 >= PROTECTED && LA67_0 <= PUBLISHED)||(LA67_0 >= READ && LA67_0 <= REINTRODUCE)||(LA67_0 >= REQUIRES && LA67_0 <= RESIDENT)||(LA67_0 >= SAFECALL && LA67_0 <= SEALED)||LA67_0==SET||LA67_0==SQUARE_BRACKET_LEFT||(LA67_0 >= STATIC && LA67_0 <= STRING)||LA67_0==TkBinaryNumber||LA67_0==TkCharacterEscapeCode||(LA67_0 >= TkHexNumber && LA67_0 <= TkIntNumber)||LA67_0==TkMultilineString||(LA67_0 >= TkQuotedString && LA67_0 <= TkRealNumber)||LA67_0==UNSAFE||(LA67_0 >= VARARGS && LA67_0 <= VIRTUAL)||LA67_0==WINAPI||(LA67_0 >= WRITE && LA67_0 <= WRITEONLY)) ) {
alt67=1;
}
switch (alt67) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:556:40: varType ( ',' )?
{
pushFollow(FOLLOW_varType_in_arrayIndices5739);
varType232=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType232.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:556:48: ( ',' )?
int alt66=2;
int LA66_0 = input.LA(1);
if ( (LA66_0==COMMA) ) {
alt66=1;
}
switch (alt66) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:556:48: ','
{
char_literal233=(Token)match(input,COMMA,FOLLOW_COMMA_in_arrayIndices5741); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal233);
}
break;
}
}
break;
default :
if ( cnt67 >= 1 ) break loop67;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(67, input);
throw eee;
}
cnt67++;
}
pushFollow(FOLLOW_rbrack_in_arrayIndices5746);
rbrack234=rbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_rbrack.add(rbrack234.getTree());
// AST REWRITE
// elements: lbrack, varType, COMMA, rbrack
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 557:30: -> ^( TkArrayIndices lbrack ( varType ( ',' )? )+ rbrack )
{
// au/com/integradev/delphi/antlr/Delphi.g:557:33: ^( TkArrayIndices lbrack ( varType ( ',' )? )+ rbrack )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ArrayIndicesNodeImpl(TkArrayIndices), root_1);
adaptor.addChild(root_1, stream_lbrack.nextTree());
if ( !(stream_varType.hasNext()) ) {
throw new RewriteEarlyExitException();
}
while ( stream_varType.hasNext() ) {
adaptor.addChild(root_1, stream_varType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:557:88: ( ',' )?
if ( stream_COMMA.hasNext() ) {
adaptor.addChild(root_1, stream_COMMA.nextNode());
}
stream_COMMA.reset();
}
stream_varType.reset();
adaptor.addChild(root_1, stream_rbrack.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 52, arrayIndices_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayIndices"
public static class arrayElementType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayElementType"
// au/com/integradev/delphi/antlr/Delphi.g:559:1: arrayElementType : ( CONST | varType );
public final DelphiParser.arrayElementType_return arrayElementType() throws RecognitionException {
DelphiParser.arrayElementType_return retval = new DelphiParser.arrayElementType_return();
retval.start = input.LT(1);
int arrayElementType_StartIndex = input.index();
Object root_0 = null;
Token CONST235=null;
ParserRuleReturnScope varType236 =null;
Object CONST235_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 53) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:559:30: ( CONST | varType )
int alt68=2;
int LA68_0 = input.LA(1);
if ( (LA68_0==CONST) ) {
alt68=1;
}
else if ( ((LA68_0 >= ABSOLUTE && LA68_0 <= ALIGN)||LA68_0==ARRAY||(LA68_0 >= ASSEMBLER && LA68_0 <= ASSEMBLY)||(LA68_0 >= AT && LA68_0 <= AUTOMATED)||LA68_0==CDECL||LA68_0==CONTAINS||(LA68_0 >= DEFAULT && LA68_0 <= DEREFERENCE)||LA68_0==DISPID||LA68_0==DYNAMIC||(LA68_0 >= EXPERIMENTAL && LA68_0 <= EXPORT)||LA68_0==EXTERNAL||(LA68_0 >= FAR && LA68_0 <= FINAL)||(LA68_0 >= FORWARD && LA68_0 <= FUNCTION)||LA68_0==HELPER||LA68_0==IMPLEMENTS||(LA68_0 >= INDEX && LA68_0 <= INHERITED)||LA68_0==LABEL||LA68_0==LOCAL||(LA68_0 >= MESSAGE && LA68_0 <= MINUS)||(LA68_0 >= NAME && LA68_0 <= NOT)||(LA68_0 >= ON && LA68_0 <= OPERATOR)||(LA68_0 >= OUT && LA68_0 <= OVERRIDE)||(LA68_0 >= PACKAGE && LA68_0 <= PAREN_BRACKET_LEFT)||LA68_0==PAREN_LEFT||(LA68_0 >= PASCAL && LA68_0 <= PROCEDURE)||(LA68_0 >= PROTECTED && LA68_0 <= PUBLISHED)||(LA68_0 >= READ && LA68_0 <= REINTRODUCE)||(LA68_0 >= REQUIRES && LA68_0 <= RESIDENT)||(LA68_0 >= SAFECALL && LA68_0 <= SEALED)||LA68_0==SET||LA68_0==SQUARE_BRACKET_LEFT||(LA68_0 >= STATIC && LA68_0 <= STRING)||LA68_0==TkBinaryNumber||LA68_0==TkCharacterEscapeCode||(LA68_0 >= TkHexNumber && LA68_0 <= TkIntNumber)||LA68_0==TkMultilineString||(LA68_0 >= TkQuotedString && LA68_0 <= TkRealNumber)||LA68_0==UNSAFE||(LA68_0 >= VARARGS && LA68_0 <= VIRTUAL)||LA68_0==WINAPI||(LA68_0 >= WRITE && LA68_0 <= WRITEONLY)) ) {
alt68=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 68, 0, input);
throw nvae;
}
switch (alt68) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:559:32: CONST
{
root_0 = (Object)adaptor.nil();
CONST235=(Token)match(input,CONST,FOLLOW_CONST_in_arrayElementType5845); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CONST235_tree = new ConstArrayElementTypeNodeImpl(CONST235) ;
adaptor.addChild(root_0, CONST235_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:560:32: varType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_varType_in_arrayElementType5881);
varType236=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varType236.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 53, arrayElementType_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayElementType"
public static class fileType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fileType"
// au/com/integradev/delphi/antlr/Delphi.g:562:1: fileType : FILE ^;
public final DelphiParser.fileType_return fileType() throws RecognitionException {
DelphiParser.fileType_return retval = new DelphiParser.fileType_return();
retval.start = input.LT(1);
int fileType_StartIndex = input.index();
Object root_0 = null;
Token FILE237=null;
Object FILE237_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 54) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:562:30: ( FILE ^)
// au/com/integradev/delphi/antlr/Delphi.g:562:32: FILE ^
{
root_0 = (Object)adaptor.nil();
FILE237=(Token)match(input,FILE,FOLLOW_FILE_in_fileType5938); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FILE237_tree = new FileTypeNodeImpl(FILE237) ;
root_0 = (Object)adaptor.becomeRoot(FILE237_tree, root_0);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 54, fileType_StartIndex); }
}
return retval;
}
// $ANTLR end "fileType"
public static class fullFileType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fullFileType"
// au/com/integradev/delphi/antlr/Delphi.g:564:1: fullFileType : FILE ^ ( OF varType )? ;
public final DelphiParser.fullFileType_return fullFileType() throws RecognitionException {
DelphiParser.fullFileType_return retval = new DelphiParser.fullFileType_return();
retval.start = input.LT(1);
int fullFileType_StartIndex = input.index();
Object root_0 = null;
Token FILE238=null;
Token OF239=null;
ParserRuleReturnScope varType240 =null;
Object FILE238_tree=null;
Object OF239_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 55) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:564:30: ( FILE ^ ( OF varType )? )
// au/com/integradev/delphi/antlr/Delphi.g:564:32: FILE ^ ( OF varType )?
{
root_0 = (Object)adaptor.nil();
FILE238=(Token)match(input,FILE,FOLLOW_FILE_in_fullFileType5995); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FILE238_tree = new FileTypeNodeImpl(FILE238) ;
root_0 = (Object)adaptor.becomeRoot(FILE238_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:564:56: ( OF varType )?
int alt69=2;
int LA69_0 = input.LA(1);
if ( (LA69_0==OF) ) {
alt69=1;
}
switch (alt69) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:564:57: OF varType
{
OF239=(Token)match(input,OF,FOLLOW_OF_in_fullFileType6002); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF239_tree = (Object)adaptor.create(OF239);
adaptor.addChild(root_0, OF239_tree);
}
pushFollow(FOLLOW_varType_in_fullFileType6004);
varType240=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varType240.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 55, fullFileType_StartIndex); }
}
return retval;
}
// $ANTLR end "fullFileType"
public static class setType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "setType"
// au/com/integradev/delphi/antlr/Delphi.g:566:1: setType : SET ^ OF varType ;
public final DelphiParser.setType_return setType() throws RecognitionException {
DelphiParser.setType_return retval = new DelphiParser.setType_return();
retval.start = input.LT(1);
int setType_StartIndex = input.index();
Object root_0 = null;
Token SET241=null;
Token OF242=null;
ParserRuleReturnScope varType243 =null;
Object SET241_tree=null;
Object OF242_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 56) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:566:30: ( SET ^ OF varType )
// au/com/integradev/delphi/antlr/Delphi.g:566:32: SET ^ OF varType
{
root_0 = (Object)adaptor.nil();
SET241=(Token)match(input,SET,FOLLOW_SET_in_setType6064); if (state.failed) return retval;
if ( state.backtracking==0 ) {
SET241_tree = new SetTypeNodeImpl(SET241) ;
root_0 = (Object)adaptor.becomeRoot(SET241_tree, root_0);
}
OF242=(Token)match(input,OF,FOLLOW_OF_in_setType6070); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF242_tree = (Object)adaptor.create(OF242);
adaptor.addChild(root_0, OF242_tree);
}
pushFollow(FOLLOW_varType_in_setType6072);
varType243=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varType243.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 56, setType_StartIndex); }
}
return retval;
}
// $ANTLR end "setType"
public static class pointerType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "pointerType"
// au/com/integradev/delphi/antlr/Delphi.g:568:1: pointerType : '^' ^ varType ;
public final DelphiParser.pointerType_return pointerType() throws RecognitionException {
DelphiParser.pointerType_return retval = new DelphiParser.pointerType_return();
retval.start = input.LT(1);
int pointerType_StartIndex = input.index();
Object root_0 = null;
Token char_literal244=null;
ParserRuleReturnScope varType245 =null;
Object char_literal244_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 57) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:568:30: ( '^' ^ varType )
// au/com/integradev/delphi/antlr/Delphi.g:568:32: '^' ^ varType
{
root_0 = (Object)adaptor.nil();
char_literal244=(Token)match(input,DEREFERENCE,FOLLOW_DEREFERENCE_in_pointerType6126); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal244_tree = new PointerTypeNodeImpl(char_literal244) ;
root_0 = (Object)adaptor.becomeRoot(char_literal244_tree, root_0);
}
pushFollow(FOLLOW_varType_in_pointerType6132);
varType245=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varType245.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 57, pointerType_StartIndex); }
}
return retval;
}
// $ANTLR end "pointerType"
public static class stringType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "stringType"
// au/com/integradev/delphi/antlr/Delphi.g:570:1: stringType : STRING ^;
public final DelphiParser.stringType_return stringType() throws RecognitionException {
DelphiParser.stringType_return retval = new DelphiParser.stringType_return();
retval.start = input.LT(1);
int stringType_StartIndex = input.index();
Object root_0 = null;
Token STRING246=null;
Object STRING246_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 58) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:570:30: ( STRING ^)
// au/com/integradev/delphi/antlr/Delphi.g:570:32: STRING ^
{
root_0 = (Object)adaptor.nil();
STRING246=(Token)match(input,STRING,FOLLOW_STRING_in_stringType6187); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STRING246_tree = new StringTypeNodeImpl(STRING246) ;
root_0 = (Object)adaptor.becomeRoot(STRING246_tree, root_0);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 58, stringType_StartIndex); }
}
return retval;
}
// $ANTLR end "stringType"
public static class fullStringType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fullStringType"
// au/com/integradev/delphi/antlr/Delphi.g:572:1: fullStringType : STRING ^ ( lbrack expression rbrack )? ;
public final DelphiParser.fullStringType_return fullStringType() throws RecognitionException {
DelphiParser.fullStringType_return retval = new DelphiParser.fullStringType_return();
retval.start = input.LT(1);
int fullStringType_StartIndex = input.index();
Object root_0 = null;
Token STRING247=null;
ParserRuleReturnScope lbrack248 =null;
ParserRuleReturnScope expression249 =null;
ParserRuleReturnScope rbrack250 =null;
Object STRING247_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 59) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:572:30: ( STRING ^ ( lbrack expression rbrack )? )
// au/com/integradev/delphi/antlr/Delphi.g:572:32: STRING ^ ( lbrack expression rbrack )?
{
root_0 = (Object)adaptor.nil();
STRING247=(Token)match(input,STRING,FOLLOW_STRING_in_fullStringType6242); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STRING247_tree = new StringTypeNodeImpl(STRING247) ;
root_0 = (Object)adaptor.becomeRoot(STRING247_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:572:60: ( lbrack expression rbrack )?
int alt70=2;
alt70 = dfa70.predict(input);
switch (alt70) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:572:61: lbrack expression rbrack
{
pushFollow(FOLLOW_lbrack_in_fullStringType6249);
lbrack248=lbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, lbrack248.getTree());
pushFollow(FOLLOW_expression_in_fullStringType6251);
expression249=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression249.getTree());
pushFollow(FOLLOW_rbrack_in_fullStringType6253);
rbrack250=rbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, rbrack250.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 59, fullStringType_StartIndex); }
}
return retval;
}
// $ANTLR end "fullStringType"
public static class procedureType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "procedureType"
// au/com/integradev/delphi/antlr/Delphi.g:574:1: procedureType : ( procedureOfObject | procedureReference | simpleProcedureType );
public final DelphiParser.procedureType_return procedureType() throws RecognitionException {
DelphiParser.procedureType_return retval = new DelphiParser.procedureType_return();
retval.start = input.LT(1);
int procedureType_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope procedureOfObject251 =null;
ParserRuleReturnScope procedureReference252 =null;
ParserRuleReturnScope simpleProcedureType253 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 60) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:574:30: ( procedureOfObject | procedureReference | simpleProcedureType )
int alt71=3;
switch ( input.LA(1) ) {
case FUNCTION:
{
int LA71_1 = input.LA(2);
if ( (synpred115_Delphi()) ) {
alt71=1;
}
else if ( (true) ) {
alt71=3;
}
}
break;
case PROCEDURE:
{
int LA71_2 = input.LA(2);
if ( (synpred115_Delphi()) ) {
alt71=1;
}
else if ( (true) ) {
alt71=3;
}
}
break;
case REFERENCE:
{
alt71=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 71, 0, input);
throw nvae;
}
switch (alt71) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:574:32: procedureOfObject
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_procedureOfObject_in_procedureType6307);
procedureOfObject251=procedureOfObject();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, procedureOfObject251.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:575:32: procedureReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_procedureReference_in_procedureType6340);
procedureReference252=procedureReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, procedureReference252.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:576:32: simpleProcedureType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_simpleProcedureType_in_procedureType6373);
simpleProcedureType253=simpleProcedureType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, simpleProcedureType253.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 60, procedureType_StartIndex); }
}
return retval;
}
// $ANTLR end "procedureType"
public static class procedureOfObject_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "procedureOfObject"
// au/com/integradev/delphi/antlr/Delphi.g:578:1: procedureOfObject : procedureTypeHeading OF OBJECT ^ ( ( ';' )? interfaceDirective )* ;
public final DelphiParser.procedureOfObject_return procedureOfObject() throws RecognitionException {
DelphiParser.procedureOfObject_return retval = new DelphiParser.procedureOfObject_return();
retval.start = input.LT(1);
int procedureOfObject_StartIndex = input.index();
Object root_0 = null;
Token OF255=null;
Token OBJECT256=null;
Token char_literal257=null;
ParserRuleReturnScope procedureTypeHeading254 =null;
ParserRuleReturnScope interfaceDirective258 =null;
Object OF255_tree=null;
Object OBJECT256_tree=null;
Object char_literal257_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 61) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:578:30: ( procedureTypeHeading OF OBJECT ^ ( ( ';' )? interfaceDirective )* )
// au/com/integradev/delphi/antlr/Delphi.g:578:32: procedureTypeHeading OF OBJECT ^ ( ( ';' )? interfaceDirective )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_procedureTypeHeading_in_procedureOfObject6421);
procedureTypeHeading254=procedureTypeHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, procedureTypeHeading254.getTree());
OF255=(Token)match(input,OF,FOLLOW_OF_in_procedureOfObject6423); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF255_tree = (Object)adaptor.create(OF255);
adaptor.addChild(root_0, OF255_tree);
}
OBJECT256=(Token)match(input,OBJECT,FOLLOW_OBJECT_in_procedureOfObject6425); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OBJECT256_tree = new ProcedureOfObjectTypeNodeImpl(OBJECT256) ;
root_0 = (Object)adaptor.becomeRoot(OBJECT256_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:578:95: ( ( ';' )? interfaceDirective )*
loop73:
while (true) {
int alt73=2;
alt73 = dfa73.predict(input);
switch (alt73) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:578:96: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:578:96: ( ';' )?
int alt72=2;
int LA72_0 = input.LA(1);
if ( (LA72_0==SEMICOLON) ) {
alt72=1;
}
switch (alt72) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:578:97: ';'
{
char_literal257=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_procedureOfObject6433); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal257_tree = (Object)adaptor.create(char_literal257);
adaptor.addChild(root_0, char_literal257_tree);
}
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_procedureOfObject6437);
interfaceDirective258=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceDirective258.getTree());
}
break;
default :
break loop73;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 61, procedureOfObject_StartIndex); }
}
return retval;
}
// $ANTLR end "procedureOfObject"
public static class procedureReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "procedureReference"
// au/com/integradev/delphi/antlr/Delphi.g:580:1: procedureReference : REFERENCE ^ TO ! procedureTypeHeading ;
public final DelphiParser.procedureReference_return procedureReference() throws RecognitionException {
DelphiParser.procedureReference_return retval = new DelphiParser.procedureReference_return();
retval.start = input.LT(1);
int procedureReference_StartIndex = input.index();
Object root_0 = null;
Token REFERENCE259=null;
Token TO260=null;
ParserRuleReturnScope procedureTypeHeading261 =null;
Object REFERENCE259_tree=null;
Object TO260_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 62) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:580:30: ( REFERENCE ^ TO ! procedureTypeHeading )
// au/com/integradev/delphi/antlr/Delphi.g:580:32: REFERENCE ^ TO ! procedureTypeHeading
{
root_0 = (Object)adaptor.nil();
REFERENCE259=(Token)match(input,REFERENCE,FOLLOW_REFERENCE_in_procedureReference6486); if (state.failed) return retval;
if ( state.backtracking==0 ) {
REFERENCE259_tree = new ProcedureReferenceTypeNodeImpl(REFERENCE259) ;
root_0 = (Object)adaptor.becomeRoot(REFERENCE259_tree, root_0);
}
TO260=(Token)match(input,TO,FOLLOW_TO_in_procedureReference6492); if (state.failed) return retval;
pushFollow(FOLLOW_procedureTypeHeading_in_procedureReference6495);
procedureTypeHeading261=procedureTypeHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, procedureTypeHeading261.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 62, procedureReference_StartIndex); }
}
return retval;
}
// $ANTLR end "procedureReference"
public static class simpleProcedureType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "simpleProcedureType"
// au/com/integradev/delphi/antlr/Delphi.g:582:1: simpleProcedureType : procedureTypeHeading -> ^( TkProcedureType procedureTypeHeading ) ;
public final DelphiParser.simpleProcedureType_return simpleProcedureType() throws RecognitionException {
DelphiParser.simpleProcedureType_return retval = new DelphiParser.simpleProcedureType_return();
retval.start = input.LT(1);
int simpleProcedureType_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope procedureTypeHeading262 =null;
RewriteRuleSubtreeStream stream_procedureTypeHeading=new RewriteRuleSubtreeStream(adaptor,"rule procedureTypeHeading");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 63) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:582:30: ( procedureTypeHeading -> ^( TkProcedureType procedureTypeHeading ) )
// au/com/integradev/delphi/antlr/Delphi.g:582:32: procedureTypeHeading
{
pushFollow(FOLLOW_procedureTypeHeading_in_simpleProcedureType6541);
procedureTypeHeading262=procedureTypeHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_procedureTypeHeading.add(procedureTypeHeading262.getTree());
// AST REWRITE
// elements: procedureTypeHeading
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 582:53: -> ^( TkProcedureType procedureTypeHeading )
{
// au/com/integradev/delphi/antlr/Delphi.g:582:56: ^( TkProcedureType procedureTypeHeading )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ProcedureTypeNodeImpl(TkProcedureType), root_1);
adaptor.addChild(root_1, stream_procedureTypeHeading.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 63, simpleProcedureType_StartIndex); }
}
return retval;
}
// $ANTLR end "simpleProcedureType"
public static class procedureTypeHeading_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "procedureTypeHeading"
// au/com/integradev/delphi/antlr/Delphi.g:584:1: procedureTypeHeading : ( FUNCTION ^ ( routineParameters )? ( routineReturnType )? ( ( ';' )? interfaceDirective )* | PROCEDURE ^ ( routineParameters )? ( ( ';' )? interfaceDirective )* );
public final DelphiParser.procedureTypeHeading_return procedureTypeHeading() throws RecognitionException {
DelphiParser.procedureTypeHeading_return retval = new DelphiParser.procedureTypeHeading_return();
retval.start = input.LT(1);
int procedureTypeHeading_StartIndex = input.index();
Object root_0 = null;
Token FUNCTION263=null;
Token char_literal266=null;
Token PROCEDURE268=null;
Token char_literal270=null;
ParserRuleReturnScope routineParameters264 =null;
ParserRuleReturnScope routineReturnType265 =null;
ParserRuleReturnScope interfaceDirective267 =null;
ParserRuleReturnScope routineParameters269 =null;
ParserRuleReturnScope interfaceDirective271 =null;
Object FUNCTION263_tree=null;
Object char_literal266_tree=null;
Object PROCEDURE268_tree=null;
Object char_literal270_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 64) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:584:30: ( FUNCTION ^ ( routineParameters )? ( routineReturnType )? ( ( ';' )? interfaceDirective )* | PROCEDURE ^ ( routineParameters )? ( ( ';' )? interfaceDirective )* )
int alt81=2;
int LA81_0 = input.LA(1);
if ( (LA81_0==FUNCTION) ) {
alt81=1;
}
else if ( (LA81_0==PROCEDURE) ) {
alt81=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 81, 0, input);
throw nvae;
}
switch (alt81) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:584:32: FUNCTION ^ ( routineParameters )? ( routineReturnType )? ( ( ';' )? interfaceDirective )*
{
root_0 = (Object)adaptor.nil();
FUNCTION263=(Token)match(input,FUNCTION,FOLLOW_FUNCTION_in_procedureTypeHeading6597); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FUNCTION263_tree = new ProcedureTypeHeadingNodeImpl(FUNCTION263) ;
root_0 = (Object)adaptor.becomeRoot(FUNCTION263_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:584:72: ( routineParameters )?
int alt74=2;
alt74 = dfa74.predict(input);
switch (alt74) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:584:72: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_procedureTypeHeading6603);
routineParameters264=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineParameters264.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:584:91: ( routineReturnType )?
int alt75=2;
int LA75_0 = input.LA(1);
if ( (LA75_0==COLON) ) {
alt75=1;
}
switch (alt75) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:584:91: routineReturnType
{
pushFollow(FOLLOW_routineReturnType_in_procedureTypeHeading6606);
routineReturnType265=routineReturnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineReturnType265.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:584:110: ( ( ';' )? interfaceDirective )*
loop77:
while (true) {
int alt77=2;
alt77 = dfa77.predict(input);
switch (alt77) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:584:111: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:584:111: ( ';' )?
int alt76=2;
int LA76_0 = input.LA(1);
if ( (LA76_0==SEMICOLON) ) {
alt76=1;
}
switch (alt76) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:584:112: ';'
{
char_literal266=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_procedureTypeHeading6611); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal266_tree = (Object)adaptor.create(char_literal266);
adaptor.addChild(root_0, char_literal266_tree);
}
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_procedureTypeHeading6615);
interfaceDirective267=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceDirective267.getTree());
}
break;
default :
break loop77;
}
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:585:32: PROCEDURE ^ ( routineParameters )? ( ( ';' )? interfaceDirective )*
{
root_0 = (Object)adaptor.nil();
PROCEDURE268=(Token)match(input,PROCEDURE,FOLLOW_PROCEDURE_in_procedureTypeHeading6650); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PROCEDURE268_tree = new ProcedureTypeHeadingNodeImpl(PROCEDURE268) ;
root_0 = (Object)adaptor.becomeRoot(PROCEDURE268_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:585:73: ( routineParameters )?
int alt78=2;
alt78 = dfa78.predict(input);
switch (alt78) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:585:73: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_procedureTypeHeading6656);
routineParameters269=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineParameters269.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:585:92: ( ( ';' )? interfaceDirective )*
loop80:
while (true) {
int alt80=2;
alt80 = dfa80.predict(input);
switch (alt80) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:585:93: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:585:93: ( ';' )?
int alt79=2;
int LA79_0 = input.LA(1);
if ( (LA79_0==SEMICOLON) ) {
alt79=1;
}
switch (alt79) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:585:94: ';'
{
char_literal270=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_procedureTypeHeading6661); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal270_tree = (Object)adaptor.create(char_literal270);
adaptor.addChild(root_0, char_literal270_tree);
}
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_procedureTypeHeading6665);
interfaceDirective271=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceDirective271.getTree());
}
break;
default :
break loop80;
}
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 64, procedureTypeHeading_StartIndex); }
}
return retval;
}
// $ANTLR end "procedureTypeHeading"
public static class typeOfType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeOfType"
// au/com/integradev/delphi/antlr/Delphi.g:587:1: typeOfType : TYPE ^ OF typeDecl ;
public final DelphiParser.typeOfType_return typeOfType() throws RecognitionException {
DelphiParser.typeOfType_return retval = new DelphiParser.typeOfType_return();
retval.start = input.LT(1);
int typeOfType_StartIndex = input.index();
Object root_0 = null;
Token TYPE272=null;
Token OF273=null;
ParserRuleReturnScope typeDecl274 =null;
Object TYPE272_tree=null;
Object OF273_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 65) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:587:30: ( TYPE ^ OF typeDecl )
// au/com/integradev/delphi/antlr/Delphi.g:587:32: TYPE ^ OF typeDecl
{
root_0 = (Object)adaptor.nil();
TYPE272=(Token)match(input,TYPE,FOLLOW_TYPE_in_typeOfType6722); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TYPE272_tree = new TypeOfTypeNodeImpl(TYPE272) ;
root_0 = (Object)adaptor.becomeRoot(TYPE272_tree, root_0);
}
OF273=(Token)match(input,OF,FOLLOW_OF_in_typeOfType6728); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF273_tree = (Object)adaptor.create(OF273);
adaptor.addChild(root_0, OF273_tree);
}
pushFollow(FOLLOW_typeDecl_in_typeOfType6730);
typeDecl274=typeDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeDecl274.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 65, typeOfType_StartIndex); }
}
return retval;
}
// $ANTLR end "typeOfType"
public static class strongAliasType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "strongAliasType"
// au/com/integradev/delphi/antlr/Delphi.g:589:1: strongAliasType : TYPE ^ typeReferenceOrStringOrFile ( codePageExpression )? ;
public final DelphiParser.strongAliasType_return strongAliasType() throws RecognitionException {
DelphiParser.strongAliasType_return retval = new DelphiParser.strongAliasType_return();
retval.start = input.LT(1);
int strongAliasType_StartIndex = input.index();
Object root_0 = null;
Token TYPE275=null;
ParserRuleReturnScope typeReferenceOrStringOrFile276 =null;
ParserRuleReturnScope codePageExpression277 =null;
Object TYPE275_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 66) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:589:30: ( TYPE ^ typeReferenceOrStringOrFile ( codePageExpression )? )
// au/com/integradev/delphi/antlr/Delphi.g:589:32: TYPE ^ typeReferenceOrStringOrFile ( codePageExpression )?
{
root_0 = (Object)adaptor.nil();
TYPE275=(Token)match(input,TYPE,FOLLOW_TYPE_in_strongAliasType6780); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TYPE275_tree = new StrongAliasTypeNodeImpl(TYPE275) ;
root_0 = (Object)adaptor.becomeRoot(TYPE275_tree, root_0);
}
pushFollow(FOLLOW_typeReferenceOrStringOrFile_in_strongAliasType6786);
typeReferenceOrStringOrFile276=typeReferenceOrStringOrFile();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReferenceOrStringOrFile276.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:589:91: ( codePageExpression )?
int alt82=2;
int LA82_0 = input.LA(1);
if ( (LA82_0==PAREN_LEFT) ) {
alt82=1;
}
switch (alt82) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:589:91: codePageExpression
{
pushFollow(FOLLOW_codePageExpression_in_strongAliasType6788);
codePageExpression277=codePageExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, codePageExpression277.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 66, strongAliasType_StartIndex); }
}
return retval;
}
// $ANTLR end "strongAliasType"
public static class codePageExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "codePageExpression"
// au/com/integradev/delphi/antlr/Delphi.g:591:1: codePageExpression : '(' ! expression ')' !;
public final DelphiParser.codePageExpression_return codePageExpression() throws RecognitionException {
DelphiParser.codePageExpression_return retval = new DelphiParser.codePageExpression_return();
retval.start = input.LT(1);
int codePageExpression_StartIndex = input.index();
Object root_0 = null;
Token char_literal278=null;
Token char_literal280=null;
ParserRuleReturnScope expression279 =null;
Object char_literal278_tree=null;
Object char_literal280_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 67) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:591:30: ( '(' ! expression ')' !)
// au/com/integradev/delphi/antlr/Delphi.g:591:32: '(' ! expression ')' !
{
root_0 = (Object)adaptor.nil();
char_literal278=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_codePageExpression6836); if (state.failed) return retval;
pushFollow(FOLLOW_expression_in_codePageExpression6839);
expression279=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression279.getTree());
char_literal280=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_codePageExpression6841); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 67, codePageExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "codePageExpression"
public static class weakAliasType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "weakAliasType"
// au/com/integradev/delphi/antlr/Delphi.g:593:1: weakAliasType : typeReference -> ^( TkWeakAlias typeReference ) ;
public final DelphiParser.weakAliasType_return weakAliasType() throws RecognitionException {
DelphiParser.weakAliasType_return retval = new DelphiParser.weakAliasType_return();
retval.start = input.LT(1);
int weakAliasType_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope typeReference281 =null;
RewriteRuleSubtreeStream stream_typeReference=new RewriteRuleSubtreeStream(adaptor,"rule typeReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 68) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:593:30: ( typeReference -> ^( TkWeakAlias typeReference ) )
// au/com/integradev/delphi/antlr/Delphi.g:593:32: typeReference
{
pushFollow(FOLLOW_typeReference_in_weakAliasType6894);
typeReference281=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReference.add(typeReference281.getTree());
// AST REWRITE
// elements: typeReference
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 593:46: -> ^( TkWeakAlias typeReference )
{
// au/com/integradev/delphi/antlr/Delphi.g:593:49: ^( TkWeakAlias typeReference )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new WeakAliasTypeNodeImpl(TkWeakAlias), root_1);
adaptor.addChild(root_1, stream_typeReference.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 68, weakAliasType_StartIndex); }
}
return retval;
}
// $ANTLR end "weakAliasType"
public static class subRangeType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "subRangeType"
// au/com/integradev/delphi/antlr/Delphi.g:595:1: subRangeType : expression '..' ^ expression ;
public final DelphiParser.subRangeType_return subRangeType() throws RecognitionException {
DelphiParser.subRangeType_return retval = new DelphiParser.subRangeType_return();
retval.start = input.LT(1);
int subRangeType_StartIndex = input.index();
Object root_0 = null;
Token string_literal283=null;
ParserRuleReturnScope expression282 =null;
ParserRuleReturnScope expression284 =null;
Object string_literal283_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 69) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:595:30: ( expression '..' ^ expression )
// au/com/integradev/delphi/antlr/Delphi.g:595:32: expression '..' ^ expression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_expression_in_subRangeType6958);
expression282=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression282.getTree());
string_literal283=(Token)match(input,DOT_DOT,FOLLOW_DOT_DOT_in_subRangeType6960); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal283_tree = new SubRangeTypeNodeImpl(string_literal283) ;
root_0 = (Object)adaptor.becomeRoot(string_literal283_tree, root_0);
}
pushFollow(FOLLOW_expression_in_subRangeType6966);
expression284=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression284.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 69, subRangeType_StartIndex); }
}
return retval;
}
// $ANTLR end "subRangeType"
public static class enumType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "enumType"
// au/com/integradev/delphi/antlr/Delphi.g:597:1: enumType : '(' ^ ( enumTypeElement ( ',' )? )* ')' !;
public final DelphiParser.enumType_return enumType() throws RecognitionException {
DelphiParser.enumType_return retval = new DelphiParser.enumType_return();
retval.start = input.LT(1);
int enumType_StartIndex = input.index();
Object root_0 = null;
Token char_literal285=null;
Token char_literal287=null;
Token char_literal288=null;
ParserRuleReturnScope enumTypeElement286 =null;
Object char_literal285_tree=null;
Object char_literal287_tree=null;
Object char_literal288_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 70) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:597:30: ( '(' ^ ( enumTypeElement ( ',' )? )* ')' !)
// au/com/integradev/delphi/antlr/Delphi.g:597:32: '(' ^ ( enumTypeElement ( ',' )? )* ')' !
{
root_0 = (Object)adaptor.nil();
char_literal285=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_enumType7023); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal285_tree = new EnumTypeNodeImpl(char_literal285) ;
root_0 = (Object)adaptor.becomeRoot(char_literal285_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:597:55: ( enumTypeElement ( ',' )? )*
loop84:
while (true) {
int alt84=2;
int LA84_0 = input.LA(1);
if ( ((LA84_0 >= ABSOLUTE && LA84_0 <= ABSTRACT)||LA84_0==ALIGN||(LA84_0 >= ASSEMBLER && LA84_0 <= ASSEMBLY)||(LA84_0 >= AT && LA84_0 <= AUTOMATED)||LA84_0==CDECL||LA84_0==CONTAINS||(LA84_0 >= DEFAULT && LA84_0 <= DEPRECATED)||LA84_0==DISPID||LA84_0==DYNAMIC||(LA84_0 >= EXPERIMENTAL && LA84_0 <= EXPORT)||LA84_0==EXTERNAL||LA84_0==FAR||LA84_0==FINAL||LA84_0==FORWARD||LA84_0==HELPER||LA84_0==IMPLEMENTS||LA84_0==INDEX||LA84_0==LABEL||LA84_0==LOCAL||LA84_0==MESSAGE||(LA84_0 >= NAME && LA84_0 <= NEAR)||LA84_0==NODEFAULT||(LA84_0 >= ON && LA84_0 <= OPERATOR)||(LA84_0 >= OUT && LA84_0 <= OVERRIDE)||LA84_0==PACKAGE||(LA84_0 >= PASCAL && LA84_0 <= PLATFORM)||LA84_0==PRIVATE||(LA84_0 >= PROTECTED && LA84_0 <= PUBLISHED)||(LA84_0 >= READ && LA84_0 <= READONLY)||(LA84_0 >= REFERENCE && LA84_0 <= REINTRODUCE)||(LA84_0 >= REQUIRES && LA84_0 <= RESIDENT)||(LA84_0 >= SAFECALL && LA84_0 <= SEALED)||(LA84_0 >= STATIC && LA84_0 <= STRICT)||LA84_0==TkIdentifier||LA84_0==UNSAFE||(LA84_0 >= VARARGS && LA84_0 <= VIRTUAL)||LA84_0==WINAPI||(LA84_0 >= WRITE && LA84_0 <= WRITEONLY)) ) {
alt84=1;
}
switch (alt84) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:597:56: enumTypeElement ( ',' )?
{
pushFollow(FOLLOW_enumTypeElement_in_enumType7030);
enumTypeElement286=enumTypeElement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, enumTypeElement286.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:597:72: ( ',' )?
int alt83=2;
int LA83_0 = input.LA(1);
if ( (LA83_0==COMMA) ) {
alt83=1;
}
switch (alt83) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:597:73: ','
{
char_literal287=(Token)match(input,COMMA,FOLLOW_COMMA_in_enumType7033); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal287_tree = (Object)adaptor.create(char_literal287);
adaptor.addChild(root_0, char_literal287_tree);
}
}
break;
}
}
break;
default :
break loop84;
}
}
char_literal288=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_enumType7039); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 70, enumType_StartIndex); }
}
return retval;
}
// $ANTLR end "enumType"
public static class enumTypeElement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "enumTypeElement"
// au/com/integradev/delphi/antlr/Delphi.g:599:1: enumTypeElement : nameDeclaration ( '=' expression )? -> ^( TkEnumElement nameDeclaration ( expression )? ) ;
public final DelphiParser.enumTypeElement_return enumTypeElement() throws RecognitionException {
DelphiParser.enumTypeElement_return retval = new DelphiParser.enumTypeElement_return();
retval.start = input.LT(1);
int enumTypeElement_StartIndex = input.index();
Object root_0 = null;
Token char_literal290=null;
ParserRuleReturnScope nameDeclaration289 =null;
ParserRuleReturnScope expression291 =null;
Object char_literal290_tree=null;
RewriteRuleTokenStream stream_EQUAL=new RewriteRuleTokenStream(adaptor,"token EQUAL");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 71) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:599:30: ( nameDeclaration ( '=' expression )? -> ^( TkEnumElement nameDeclaration ( expression )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:599:32: nameDeclaration ( '=' expression )?
{
pushFollow(FOLLOW_nameDeclaration_in_enumTypeElement7090);
nameDeclaration289=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration289.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:599:48: ( '=' expression )?
int alt85=2;
int LA85_0 = input.LA(1);
if ( (LA85_0==EQUAL) ) {
alt85=1;
}
switch (alt85) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:599:49: '=' expression
{
char_literal290=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_enumTypeElement7093); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal290);
pushFollow(FOLLOW_expression_in_enumTypeElement7095);
expression291=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression291.getTree());
}
break;
}
// AST REWRITE
// elements: expression, nameDeclaration
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 599:66: -> ^( TkEnumElement nameDeclaration ( expression )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:599:69: ^( TkEnumElement nameDeclaration ( expression )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new EnumElementNodeImpl(TkEnumElement), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:599:122: ( expression )?
if ( stream_expression.hasNext() ) {
adaptor.addChild(root_1, stream_expression.nextTree());
}
stream_expression.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 71, enumTypeElement_StartIndex); }
}
return retval;
}
// $ANTLR end "enumTypeElement"
public static class typeReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeReference"
// au/com/integradev/delphi/antlr/Delphi.g:601:1: typeReference : nameReference -> ^( TkTypeReference nameReference ) ;
public final DelphiParser.typeReference_return typeReference() throws RecognitionException {
DelphiParser.typeReference_return retval = new DelphiParser.typeReference_return();
retval.start = input.LT(1);
int typeReference_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope nameReference292 =null;
RewriteRuleSubtreeStream stream_nameReference=new RewriteRuleSubtreeStream(adaptor,"rule nameReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 72) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:601:30: ( nameReference -> ^( TkTypeReference nameReference ) )
// au/com/integradev/delphi/antlr/Delphi.g:601:32: nameReference
{
pushFollow(FOLLOW_nameReference_in_typeReference7163);
nameReference292=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameReference.add(nameReference292.getTree());
// AST REWRITE
// elements: nameReference
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 601:46: -> ^( TkTypeReference nameReference )
{
// au/com/integradev/delphi/antlr/Delphi.g:601:49: ^( TkTypeReference nameReference )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new TypeReferenceNodeImpl(TkTypeReference), root_1);
adaptor.addChild(root_1, stream_nameReference.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 72, typeReference_StartIndex); }
}
return retval;
}
// $ANTLR end "typeReference"
public static class typeReferenceOrString_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeReferenceOrString"
// au/com/integradev/delphi/antlr/Delphi.g:603:1: typeReferenceOrString : ( stringType | typeReference );
public final DelphiParser.typeReferenceOrString_return typeReferenceOrString() throws RecognitionException {
DelphiParser.typeReferenceOrString_return retval = new DelphiParser.typeReferenceOrString_return();
retval.start = input.LT(1);
int typeReferenceOrString_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope stringType293 =null;
ParserRuleReturnScope typeReference294 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 73) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:603:30: ( stringType | typeReference )
int alt86=2;
int LA86_0 = input.LA(1);
if ( (LA86_0==STRING) ) {
alt86=1;
}
else if ( ((LA86_0 >= ABSOLUTE && LA86_0 <= ABSTRACT)||LA86_0==ALIGN||(LA86_0 >= ASSEMBLER && LA86_0 <= ASSEMBLY)||(LA86_0 >= AT && LA86_0 <= AUTOMATED)||LA86_0==CDECL||LA86_0==CONTAINS||(LA86_0 >= DEFAULT && LA86_0 <= DEPRECATED)||LA86_0==DISPID||LA86_0==DYNAMIC||(LA86_0 >= EXPERIMENTAL && LA86_0 <= EXPORT)||LA86_0==EXTERNAL||LA86_0==FAR||LA86_0==FINAL||LA86_0==FORWARD||LA86_0==HELPER||LA86_0==IMPLEMENTS||LA86_0==INDEX||LA86_0==LABEL||LA86_0==LOCAL||LA86_0==MESSAGE||(LA86_0 >= NAME && LA86_0 <= NEAR)||LA86_0==NODEFAULT||(LA86_0 >= ON && LA86_0 <= OPERATOR)||(LA86_0 >= OUT && LA86_0 <= OVERRIDE)||LA86_0==PACKAGE||(LA86_0 >= PASCAL && LA86_0 <= PLATFORM)||LA86_0==PRIVATE||(LA86_0 >= PROTECTED && LA86_0 <= PUBLISHED)||(LA86_0 >= READ && LA86_0 <= READONLY)||(LA86_0 >= REFERENCE && LA86_0 <= REINTRODUCE)||(LA86_0 >= REQUIRES && LA86_0 <= RESIDENT)||(LA86_0 >= SAFECALL && LA86_0 <= SEALED)||(LA86_0 >= STATIC && LA86_0 <= STRICT)||LA86_0==TkIdentifier||LA86_0==UNSAFE||(LA86_0 >= VARARGS && LA86_0 <= VIRTUAL)||LA86_0==WINAPI||(LA86_0 >= WRITE && LA86_0 <= WRITEONLY)) ) {
alt86=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 86, 0, input);
throw nvae;
}
switch (alt86) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:603:32: stringType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_stringType_in_typeReferenceOrString7218);
stringType293=stringType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, stringType293.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:604:32: typeReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeReference_in_typeReferenceOrString7251);
typeReference294=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference294.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 73, typeReferenceOrString_StartIndex); }
}
return retval;
}
// $ANTLR end "typeReferenceOrString"
public static class typeReferenceOrStringOrFile_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeReferenceOrStringOrFile"
// au/com/integradev/delphi/antlr/Delphi.g:606:1: typeReferenceOrStringOrFile : ( stringType | fileType | typeReference );
public final DelphiParser.typeReferenceOrStringOrFile_return typeReferenceOrStringOrFile() throws RecognitionException {
DelphiParser.typeReferenceOrStringOrFile_return retval = new DelphiParser.typeReferenceOrStringOrFile_return();
retval.start = input.LT(1);
int typeReferenceOrStringOrFile_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope stringType295 =null;
ParserRuleReturnScope fileType296 =null;
ParserRuleReturnScope typeReference297 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 74) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:606:30: ( stringType | fileType | typeReference )
int alt87=3;
switch ( input.LA(1) ) {
case STRING:
{
alt87=1;
}
break;
case FILE:
{
alt87=2;
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt87=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 87, 0, input);
throw nvae;
}
switch (alt87) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:606:32: stringType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_stringType_in_typeReferenceOrStringOrFile7289);
stringType295=stringType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, stringType295.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:607:32: fileType
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fileType_in_typeReferenceOrStringOrFile7322);
fileType296=fileType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fileType296.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:608:32: typeReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeReference_in_typeReferenceOrStringOrFile7355);
typeReference297=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference297.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 74, typeReferenceOrStringOrFile_StartIndex); }
}
return retval;
}
// $ANTLR end "typeReferenceOrStringOrFile"
public static class classReferenceType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "classReferenceType"
// au/com/integradev/delphi/antlr/Delphi.g:614:1: classReferenceType : CLASS ^ OF typeReference ;
public final DelphiParser.classReferenceType_return classReferenceType() throws RecognitionException {
DelphiParser.classReferenceType_return retval = new DelphiParser.classReferenceType_return();
retval.start = input.LT(1);
int classReferenceType_StartIndex = input.index();
Object root_0 = null;
Token CLASS298=null;
Token OF299=null;
ParserRuleReturnScope typeReference300 =null;
Object CLASS298_tree=null;
Object OF299_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 75) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:614:30: ( CLASS ^ OF typeReference )
// au/com/integradev/delphi/antlr/Delphi.g:614:32: CLASS ^ OF typeReference
{
root_0 = (Object)adaptor.nil();
CLASS298=(Token)match(input,CLASS,FOLLOW_CLASS_in_classReferenceType7406); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CLASS298_tree = new ClassReferenceTypeNodeImpl(CLASS298) ;
root_0 = (Object)adaptor.becomeRoot(CLASS298_tree, root_0);
}
OF299=(Token)match(input,OF,FOLLOW_OF_in_classReferenceType7412); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF299_tree = (Object)adaptor.create(OF299);
adaptor.addChild(root_0, OF299_tree);
}
pushFollow(FOLLOW_typeReference_in_classReferenceType7414);
typeReference300=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference300.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 75, classReferenceType_StartIndex); }
}
return retval;
}
// $ANTLR end "classReferenceType"
public static class classType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "classType"
// au/com/integradev/delphi/antlr/Delphi.g:616:1: classType : CLASS ( classState )? ( classParent )? ( ( visibilitySection )* END )? -> ^( CLASS ( classParent )? ( classState )? ( ( visibilitySection )* END )? ) ;
public final DelphiParser.classType_return classType() throws RecognitionException {
DelphiParser.classType_return retval = new DelphiParser.classType_return();
retval.start = input.LT(1);
int classType_StartIndex = input.index();
Object root_0 = null;
Token CLASS301=null;
Token END305=null;
ParserRuleReturnScope classState302 =null;
ParserRuleReturnScope classParent303 =null;
ParserRuleReturnScope visibilitySection304 =null;
Object CLASS301_tree=null;
Object END305_tree=null;
RewriteRuleTokenStream stream_END=new RewriteRuleTokenStream(adaptor,"token END");
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_classState=new RewriteRuleSubtreeStream(adaptor,"rule classState");
RewriteRuleSubtreeStream stream_visibilitySection=new RewriteRuleSubtreeStream(adaptor,"rule visibilitySection");
RewriteRuleSubtreeStream stream_classParent=new RewriteRuleSubtreeStream(adaptor,"rule classParent");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 76) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:616:30: ( CLASS ( classState )? ( classParent )? ( ( visibilitySection )* END )? -> ^( CLASS ( classParent )? ( classState )? ( ( visibilitySection )* END )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:616:32: CLASS ( classState )? ( classParent )? ( ( visibilitySection )* END )?
{
CLASS301=(Token)match(input,CLASS,FOLLOW_CLASS_in_classType7470); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS301);
// au/com/integradev/delphi/antlr/Delphi.g:616:38: ( classState )?
int alt88=2;
int LA88_0 = input.LA(1);
if ( (LA88_0==ABSTRACT||LA88_0==SEALED) ) {
int LA88_1 = input.LA(2);
if ( (LA88_1==EOF||(LA88_1 >= ABSOLUTE && LA88_1 <= ABSTRACT)||LA88_1==ALIGN||(LA88_1 >= ASSEMBLER && LA88_1 <= ASSEMBLY)||(LA88_1 >= AT && LA88_1 <= AUTOMATED)||(LA88_1 >= CDECL && LA88_1 <= CLASS)||(LA88_1 >= CONST && LA88_1 <= CONTAINS)||(LA88_1 >= DEFAULT && LA88_1 <= DEPRECATED)||(LA88_1 >= DESTRUCTOR && LA88_1 <= DISPID)||LA88_1==DYNAMIC||LA88_1==END||(LA88_1 >= EXPERIMENTAL && LA88_1 <= EXPORT)||LA88_1==EXTERNAL||LA88_1==FAR||LA88_1==FINAL||(LA88_1 >= FORWARD && LA88_1 <= FUNCTION)||LA88_1==HELPER||LA88_1==IMPLEMENTS||LA88_1==INDEX||LA88_1==LABEL||(LA88_1 >= LIBRARY && LA88_1 <= LOCAL)||LA88_1==MESSAGE||(LA88_1 >= NAME && LA88_1 <= NEAR)||LA88_1==NODEFAULT||(LA88_1 >= ON && LA88_1 <= OPERATOR)||(LA88_1 >= OUT && LA88_1 <= OVERRIDE)||LA88_1==PACKAGE||LA88_1==PAREN_BRACKET_LEFT||LA88_1==PAREN_LEFT||(LA88_1 >= PASCAL && LA88_1 <= PLATFORM)||(LA88_1 >= PRIVATE && LA88_1 <= PROCEDURE)||(LA88_1 >= PROPERTY && LA88_1 <= PUBLISHED)||(LA88_1 >= READ && LA88_1 <= READONLY)||(LA88_1 >= REFERENCE && LA88_1 <= REINTRODUCE)||(LA88_1 >= REQUIRES && LA88_1 <= RESOURCESTRING)||(LA88_1 >= SAFECALL && LA88_1 <= SEMICOLON)||LA88_1==SQUARE_BRACKET_LEFT||(LA88_1 >= STATIC && LA88_1 <= STRICT)||LA88_1==THREADVAR||LA88_1==TYPE||LA88_1==TkIdentifier||LA88_1==UNSAFE||(LA88_1 >= VAR && LA88_1 <= VIRTUAL)||LA88_1==WINAPI||(LA88_1 >= WRITE && LA88_1 <= WRITEONLY)) ) {
alt88=1;
}
}
switch (alt88) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:616:38: classState
{
pushFollow(FOLLOW_classState_in_classType7472);
classState302=classState();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_classState.add(classState302.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:616:50: ( classParent )?
int alt89=2;
int LA89_0 = input.LA(1);
if ( (LA89_0==PAREN_LEFT) ) {
alt89=1;
}
switch (alt89) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:616:50: classParent
{
pushFollow(FOLLOW_classParent_in_classType7475);
classParent303=classParent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_classParent.add(classParent303.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:616:63: ( ( visibilitySection )* END )?
int alt91=2;
switch ( input.LA(1) ) {
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CLASS:
case CONST:
case CONSTRUCTOR:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DESTRUCTOR:
case DISPID:
case DYNAMIC:
case END:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case FUNCTION:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PAREN_BRACKET_LEFT:
case PASCAL:
case PRIVATE:
case PROCEDURE:
case PROPERTY:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case RESOURCESTRING:
case SAFECALL:
case SEALED:
case SQUARE_BRACKET_LEFT:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case THREADVAR:
case TYPE:
case TkIdentifier:
case UNSAFE:
case VAR:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt91=1;
}
break;
case DEPRECATED:
{
int LA91_2 = input.LA(2);
if ( ((LA91_2 >= COLON && LA91_2 <= COMMA)) ) {
alt91=1;
}
}
break;
case EXPERIMENTAL:
{
int LA91_3 = input.LA(2);
if ( ((LA91_3 >= COLON && LA91_3 <= COMMA)) ) {
alt91=1;
}
}
break;
case PLATFORM:
{
int LA91_4 = input.LA(2);
if ( ((LA91_4 >= COLON && LA91_4 <= COMMA)) ) {
alt91=1;
}
}
break;
}
switch (alt91) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:616:64: ( visibilitySection )* END
{
// au/com/integradev/delphi/antlr/Delphi.g:616:64: ( visibilitySection )*
loop90:
while (true) {
int alt90=2;
int LA90_0 = input.LA(1);
if ( ((LA90_0 >= ABSOLUTE && LA90_0 <= ABSTRACT)||LA90_0==ALIGN||(LA90_0 >= ASSEMBLER && LA90_0 <= ASSEMBLY)||(LA90_0 >= AT && LA90_0 <= AUTOMATED)||(LA90_0 >= CDECL && LA90_0 <= CLASS)||(LA90_0 >= CONST && LA90_0 <= CONTAINS)||(LA90_0 >= DEFAULT && LA90_0 <= DEPRECATED)||(LA90_0 >= DESTRUCTOR && LA90_0 <= DISPID)||LA90_0==DYNAMIC||(LA90_0 >= EXPERIMENTAL && LA90_0 <= EXPORT)||LA90_0==EXTERNAL||LA90_0==FAR||LA90_0==FINAL||(LA90_0 >= FORWARD && LA90_0 <= FUNCTION)||LA90_0==HELPER||LA90_0==IMPLEMENTS||LA90_0==INDEX||LA90_0==LABEL||LA90_0==LOCAL||LA90_0==MESSAGE||(LA90_0 >= NAME && LA90_0 <= NEAR)||LA90_0==NODEFAULT||(LA90_0 >= ON && LA90_0 <= OPERATOR)||(LA90_0 >= OUT && LA90_0 <= OVERRIDE)||LA90_0==PACKAGE||LA90_0==PAREN_BRACKET_LEFT||(LA90_0 >= PASCAL && LA90_0 <= PLATFORM)||(LA90_0 >= PRIVATE && LA90_0 <= PROCEDURE)||(LA90_0 >= PROPERTY && LA90_0 <= PUBLISHED)||(LA90_0 >= READ && LA90_0 <= READONLY)||(LA90_0 >= REFERENCE && LA90_0 <= REINTRODUCE)||(LA90_0 >= REQUIRES && LA90_0 <= RESOURCESTRING)||(LA90_0 >= SAFECALL && LA90_0 <= SEALED)||LA90_0==SQUARE_BRACKET_LEFT||(LA90_0 >= STATIC && LA90_0 <= STRICT)||LA90_0==THREADVAR||LA90_0==TYPE||LA90_0==TkIdentifier||LA90_0==UNSAFE||(LA90_0 >= VAR && LA90_0 <= VIRTUAL)||LA90_0==WINAPI||(LA90_0 >= WRITE && LA90_0 <= WRITEONLY)) ) {
alt90=1;
}
switch (alt90) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:616:64: visibilitySection
{
pushFollow(FOLLOW_visibilitySection_in_classType7479);
visibilitySection304=visibilitySection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_visibilitySection.add(visibilitySection304.getTree());
}
break;
default :
break loop90;
}
}
END305=(Token)match(input,END,FOLLOW_END_in_classType7482); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_END.add(END305);
}
break;
}
// AST REWRITE
// elements: classParent, visibilitySection, END, CLASS, classState
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 617:30: -> ^( CLASS ( classParent )? ( classState )? ( ( visibilitySection )* END )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:617:33: ^( CLASS ( classParent )? ( classState )? ( ( visibilitySection )* END )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ClassTypeNodeImpl(stream_CLASS.nextToken()), root_1);
// au/com/integradev/delphi/antlr/Delphi.g:617:60: ( classParent )?
if ( stream_classParent.hasNext() ) {
adaptor.addChild(root_1, stream_classParent.nextTree());
}
stream_classParent.reset();
// au/com/integradev/delphi/antlr/Delphi.g:617:73: ( classState )?
if ( stream_classState.hasNext() ) {
adaptor.addChild(root_1, stream_classState.nextTree());
}
stream_classState.reset();
// au/com/integradev/delphi/antlr/Delphi.g:617:85: ( ( visibilitySection )* END )?
if ( stream_visibilitySection.hasNext()||stream_END.hasNext() ) {
// au/com/integradev/delphi/antlr/Delphi.g:617:86: ( visibilitySection )*
while ( stream_visibilitySection.hasNext() ) {
adaptor.addChild(root_1, stream_visibilitySection.nextTree());
}
stream_visibilitySection.reset();
adaptor.addChild(root_1, stream_END.nextNode());
}
stream_visibilitySection.reset();
stream_END.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 76, classType_StartIndex); }
}
return retval;
}
// $ANTLR end "classType"
public static class classState_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "classState"
// au/com/integradev/delphi/antlr/Delphi.g:619:1: classState : ( SEALED | ABSTRACT );
public final DelphiParser.classState_return classState() throws RecognitionException {
DelphiParser.classState_return retval = new DelphiParser.classState_return();
retval.start = input.LT(1);
int classState_StartIndex = input.index();
Object root_0 = null;
Token set306=null;
Object set306_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 77) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:619:30: ( SEALED | ABSTRACT )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set306=input.LT(1);
if ( input.LA(1)==ABSTRACT||input.LA(1)==SEALED ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set306));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 77, classState_StartIndex); }
}
return retval;
}
// $ANTLR end "classState"
public static class classParent_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "classParent"
// au/com/integradev/delphi/antlr/Delphi.g:622:1: classParent : '(' typeReference ( ',' typeReference )* ')' -> ^( TkClassParents typeReference ( typeReference )* ) ;
public final DelphiParser.classParent_return classParent() throws RecognitionException {
DelphiParser.classParent_return retval = new DelphiParser.classParent_return();
retval.start = input.LT(1);
int classParent_StartIndex = input.index();
Object root_0 = null;
Token char_literal307=null;
Token char_literal309=null;
Token char_literal311=null;
ParserRuleReturnScope typeReference308 =null;
ParserRuleReturnScope typeReference310 =null;
Object char_literal307_tree=null;
Object char_literal309_tree=null;
Object char_literal311_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleTokenStream stream_PAREN_RIGHT=new RewriteRuleTokenStream(adaptor,"token PAREN_RIGHT");
RewriteRuleTokenStream stream_PAREN_LEFT=new RewriteRuleTokenStream(adaptor,"token PAREN_LEFT");
RewriteRuleSubtreeStream stream_typeReference=new RewriteRuleSubtreeStream(adaptor,"rule typeReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 78) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:622:30: ( '(' typeReference ( ',' typeReference )* ')' -> ^( TkClassParents typeReference ( typeReference )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:622:32: '(' typeReference ( ',' typeReference )* ')'
{
char_literal307=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_classParent7678); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_LEFT.add(char_literal307);
pushFollow(FOLLOW_typeReference_in_classParent7680);
typeReference308=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReference.add(typeReference308.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:622:50: ( ',' typeReference )*
loop92:
while (true) {
int alt92=2;
int LA92_0 = input.LA(1);
if ( (LA92_0==COMMA) ) {
alt92=1;
}
switch (alt92) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:622:51: ',' typeReference
{
char_literal309=(Token)match(input,COMMA,FOLLOW_COMMA_in_classParent7683); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal309);
pushFollow(FOLLOW_typeReference_in_classParent7685);
typeReference310=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReference.add(typeReference310.getTree());
}
break;
default :
break loop92;
}
}
char_literal311=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_classParent7689); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_RIGHT.add(char_literal311);
// AST REWRITE
// elements: typeReference, typeReference
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 623:30: -> ^( TkClassParents typeReference ( typeReference )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:623:33: ^( TkClassParents typeReference ( typeReference )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AncestorListNodeImpl(TkClassParents), root_1);
adaptor.addChild(root_1, stream_typeReference.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:623:86: ( typeReference )*
while ( stream_typeReference.hasNext() ) {
adaptor.addChild(root_1, stream_typeReference.nextTree());
}
stream_typeReference.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 78, classParent_StartIndex); }
}
return retval;
}
// $ANTLR end "classParent"
public static class visibilitySection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "visibilitySection"
// au/com/integradev/delphi/antlr/Delphi.g:625:1: visibilitySection : visibilitySection_ -> ^( TkVisibilitySection visibilitySection_ ) ;
public final DelphiParser.visibilitySection_return visibilitySection() throws RecognitionException {
DelphiParser.visibilitySection_return retval = new DelphiParser.visibilitySection_return();
retval.start = input.LT(1);
int visibilitySection_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope visibilitySection_312 =null;
RewriteRuleSubtreeStream stream_visibilitySection_=new RewriteRuleSubtreeStream(adaptor,"rule visibilitySection_");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 79) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:625:30: ( visibilitySection_ -> ^( TkVisibilitySection visibilitySection_ ) )
// au/com/integradev/delphi/antlr/Delphi.g:625:32: visibilitySection_
{
pushFollow(FOLLOW_visibilitySection__in_visibilitySection7780);
visibilitySection_312=visibilitySection_();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_visibilitySection_.add(visibilitySection_312.getTree());
// AST REWRITE
// elements: visibilitySection_
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 625:51: -> ^( TkVisibilitySection visibilitySection_ )
{
// au/com/integradev/delphi/antlr/Delphi.g:625:54: ^( TkVisibilitySection visibilitySection_ )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new VisibilitySectionNodeImpl(TkVisibilitySection), root_1);
adaptor.addChild(root_1, stream_visibilitySection_.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 79, visibilitySection_StartIndex); }
}
return retval;
}
// $ANTLR end "visibilitySection"
public static class visibilitySection__return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "visibilitySection_"
// au/com/integradev/delphi/antlr/Delphi.g:627:1: visibilitySection_ : ( visibility ( visibilitySectionItem )* | ( visibilitySectionItem )+ );
public final DelphiParser.visibilitySection__return visibilitySection_() throws RecognitionException {
DelphiParser.visibilitySection__return retval = new DelphiParser.visibilitySection__return();
retval.start = input.LT(1);
int visibilitySection__StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope visibility313 =null;
ParserRuleReturnScope visibilitySectionItem314 =null;
ParserRuleReturnScope visibilitySectionItem315 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 80) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:627:30: ( visibility ( visibilitySectionItem )* | ( visibilitySectionItem )+ )
int alt95=2;
switch ( input.LA(1) ) {
case STRICT:
{
int LA95_1 = input.LA(2);
if ( (LA95_1==PRIVATE||LA95_1==PROTECTED) ) {
alt95=1;
}
else if ( ((LA95_1 >= COLON && LA95_1 <= COMMA)) ) {
alt95=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 95, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PROTECTED:
{
int LA95_2 = input.LA(2);
if ( (LA95_2==EOF||(LA95_2 >= ABSOLUTE && LA95_2 <= ABSTRACT)||LA95_2==ALIGN||(LA95_2 >= ASSEMBLER && LA95_2 <= ASSEMBLY)||(LA95_2 >= AT && LA95_2 <= AUTOMATED)||(LA95_2 >= CASE && LA95_2 <= CLASS)||(LA95_2 >= CONST && LA95_2 <= CONTAINS)||(LA95_2 >= DEFAULT && LA95_2 <= DEPRECATED)||(LA95_2 >= DESTRUCTOR && LA95_2 <= DISPID)||LA95_2==DYNAMIC||LA95_2==END||(LA95_2 >= EXPERIMENTAL && LA95_2 <= EXPORT)||LA95_2==EXTERNAL||LA95_2==FAR||LA95_2==FINAL||(LA95_2 >= FORWARD && LA95_2 <= FUNCTION)||LA95_2==HELPER||LA95_2==IMPLEMENTS||LA95_2==INDEX||LA95_2==LABEL||LA95_2==LOCAL||LA95_2==MESSAGE||(LA95_2 >= NAME && LA95_2 <= NEAR)||LA95_2==NODEFAULT||(LA95_2 >= ON && LA95_2 <= OPERATOR)||(LA95_2 >= OUT && LA95_2 <= OVERRIDE)||LA95_2==PACKAGE||LA95_2==PAREN_BRACKET_LEFT||(LA95_2 >= PASCAL && LA95_2 <= PLATFORM)||(LA95_2 >= PRIVATE && LA95_2 <= PROCEDURE)||(LA95_2 >= PROPERTY && LA95_2 <= PUBLISHED)||(LA95_2 >= READ && LA95_2 <= READONLY)||(LA95_2 >= REFERENCE && LA95_2 <= REINTRODUCE)||(LA95_2 >= REQUIRES && LA95_2 <= RESOURCESTRING)||(LA95_2 >= SAFECALL && LA95_2 <= SEALED)||LA95_2==SQUARE_BRACKET_LEFT||(LA95_2 >= STATIC && LA95_2 <= STRICT)||LA95_2==THREADVAR||LA95_2==TYPE||LA95_2==TkIdentifier||LA95_2==UNSAFE||(LA95_2 >= VAR && LA95_2 <= VIRTUAL)||LA95_2==WINAPI||(LA95_2 >= WRITE && LA95_2 <= WRITEONLY)) ) {
alt95=1;
}
else if ( ((LA95_2 >= COLON && LA95_2 <= COMMA)) ) {
alt95=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 95, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PRIVATE:
{
int LA95_3 = input.LA(2);
if ( (LA95_3==EOF||(LA95_3 >= ABSOLUTE && LA95_3 <= ABSTRACT)||LA95_3==ALIGN||(LA95_3 >= ASSEMBLER && LA95_3 <= ASSEMBLY)||(LA95_3 >= AT && LA95_3 <= AUTOMATED)||(LA95_3 >= CASE && LA95_3 <= CLASS)||(LA95_3 >= CONST && LA95_3 <= CONTAINS)||(LA95_3 >= DEFAULT && LA95_3 <= DEPRECATED)||(LA95_3 >= DESTRUCTOR && LA95_3 <= DISPID)||LA95_3==DYNAMIC||LA95_3==END||(LA95_3 >= EXPERIMENTAL && LA95_3 <= EXPORT)||LA95_3==EXTERNAL||LA95_3==FAR||LA95_3==FINAL||(LA95_3 >= FORWARD && LA95_3 <= FUNCTION)||LA95_3==HELPER||LA95_3==IMPLEMENTS||LA95_3==INDEX||LA95_3==LABEL||LA95_3==LOCAL||LA95_3==MESSAGE||(LA95_3 >= NAME && LA95_3 <= NEAR)||LA95_3==NODEFAULT||(LA95_3 >= ON && LA95_3 <= OPERATOR)||(LA95_3 >= OUT && LA95_3 <= OVERRIDE)||LA95_3==PACKAGE||LA95_3==PAREN_BRACKET_LEFT||(LA95_3 >= PASCAL && LA95_3 <= PLATFORM)||(LA95_3 >= PRIVATE && LA95_3 <= PROCEDURE)||(LA95_3 >= PROPERTY && LA95_3 <= PUBLISHED)||(LA95_3 >= READ && LA95_3 <= READONLY)||(LA95_3 >= REFERENCE && LA95_3 <= REINTRODUCE)||(LA95_3 >= REQUIRES && LA95_3 <= RESOURCESTRING)||(LA95_3 >= SAFECALL && LA95_3 <= SEALED)||LA95_3==SQUARE_BRACKET_LEFT||(LA95_3 >= STATIC && LA95_3 <= STRICT)||LA95_3==THREADVAR||LA95_3==TYPE||LA95_3==TkIdentifier||LA95_3==UNSAFE||(LA95_3 >= VAR && LA95_3 <= VIRTUAL)||LA95_3==WINAPI||(LA95_3 >= WRITE && LA95_3 <= WRITEONLY)) ) {
alt95=1;
}
else if ( ((LA95_3 >= COLON && LA95_3 <= COMMA)) ) {
alt95=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 95, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PUBLIC:
{
int LA95_4 = input.LA(2);
if ( (LA95_4==EOF||(LA95_4 >= ABSOLUTE && LA95_4 <= ABSTRACT)||LA95_4==ALIGN||(LA95_4 >= ASSEMBLER && LA95_4 <= ASSEMBLY)||(LA95_4 >= AT && LA95_4 <= AUTOMATED)||(LA95_4 >= CASE && LA95_4 <= CLASS)||(LA95_4 >= CONST && LA95_4 <= CONTAINS)||(LA95_4 >= DEFAULT && LA95_4 <= DEPRECATED)||(LA95_4 >= DESTRUCTOR && LA95_4 <= DISPID)||LA95_4==DYNAMIC||LA95_4==END||(LA95_4 >= EXPERIMENTAL && LA95_4 <= EXPORT)||LA95_4==EXTERNAL||LA95_4==FAR||LA95_4==FINAL||(LA95_4 >= FORWARD && LA95_4 <= FUNCTION)||LA95_4==HELPER||LA95_4==IMPLEMENTS||LA95_4==INDEX||LA95_4==LABEL||LA95_4==LOCAL||LA95_4==MESSAGE||(LA95_4 >= NAME && LA95_4 <= NEAR)||LA95_4==NODEFAULT||(LA95_4 >= ON && LA95_4 <= OPERATOR)||(LA95_4 >= OUT && LA95_4 <= OVERRIDE)||LA95_4==PACKAGE||LA95_4==PAREN_BRACKET_LEFT||(LA95_4 >= PASCAL && LA95_4 <= PLATFORM)||(LA95_4 >= PRIVATE && LA95_4 <= PROCEDURE)||(LA95_4 >= PROPERTY && LA95_4 <= PUBLISHED)||(LA95_4 >= READ && LA95_4 <= READONLY)||(LA95_4 >= REFERENCE && LA95_4 <= REINTRODUCE)||(LA95_4 >= REQUIRES && LA95_4 <= RESOURCESTRING)||(LA95_4 >= SAFECALL && LA95_4 <= SEALED)||LA95_4==SQUARE_BRACKET_LEFT||(LA95_4 >= STATIC && LA95_4 <= STRICT)||LA95_4==THREADVAR||LA95_4==TYPE||LA95_4==TkIdentifier||LA95_4==UNSAFE||(LA95_4 >= VAR && LA95_4 <= VIRTUAL)||LA95_4==WINAPI||(LA95_4 >= WRITE && LA95_4 <= WRITEONLY)) ) {
alt95=1;
}
else if ( ((LA95_4 >= COLON && LA95_4 <= COMMA)) ) {
alt95=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 95, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PUBLISHED:
{
int LA95_5 = input.LA(2);
if ( (LA95_5==EOF||(LA95_5 >= ABSOLUTE && LA95_5 <= ABSTRACT)||LA95_5==ALIGN||(LA95_5 >= ASSEMBLER && LA95_5 <= ASSEMBLY)||(LA95_5 >= AT && LA95_5 <= AUTOMATED)||(LA95_5 >= CASE && LA95_5 <= CLASS)||(LA95_5 >= CONST && LA95_5 <= CONTAINS)||(LA95_5 >= DEFAULT && LA95_5 <= DEPRECATED)||(LA95_5 >= DESTRUCTOR && LA95_5 <= DISPID)||LA95_5==DYNAMIC||LA95_5==END||(LA95_5 >= EXPERIMENTAL && LA95_5 <= EXPORT)||LA95_5==EXTERNAL||LA95_5==FAR||LA95_5==FINAL||(LA95_5 >= FORWARD && LA95_5 <= FUNCTION)||LA95_5==HELPER||LA95_5==IMPLEMENTS||LA95_5==INDEX||LA95_5==LABEL||LA95_5==LOCAL||LA95_5==MESSAGE||(LA95_5 >= NAME && LA95_5 <= NEAR)||LA95_5==NODEFAULT||(LA95_5 >= ON && LA95_5 <= OPERATOR)||(LA95_5 >= OUT && LA95_5 <= OVERRIDE)||LA95_5==PACKAGE||LA95_5==PAREN_BRACKET_LEFT||(LA95_5 >= PASCAL && LA95_5 <= PLATFORM)||(LA95_5 >= PRIVATE && LA95_5 <= PROCEDURE)||(LA95_5 >= PROPERTY && LA95_5 <= PUBLISHED)||(LA95_5 >= READ && LA95_5 <= READONLY)||(LA95_5 >= REFERENCE && LA95_5 <= REINTRODUCE)||(LA95_5 >= REQUIRES && LA95_5 <= RESOURCESTRING)||(LA95_5 >= SAFECALL && LA95_5 <= SEALED)||LA95_5==SQUARE_BRACKET_LEFT||(LA95_5 >= STATIC && LA95_5 <= STRICT)||LA95_5==THREADVAR||LA95_5==TYPE||LA95_5==TkIdentifier||LA95_5==UNSAFE||(LA95_5 >= VAR && LA95_5 <= VIRTUAL)||LA95_5==WINAPI||(LA95_5 >= WRITE && LA95_5 <= WRITEONLY)) ) {
alt95=1;
}
else if ( ((LA95_5 >= COLON && LA95_5 <= COMMA)) ) {
alt95=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 95, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case AUTOMATED:
{
int LA95_6 = input.LA(2);
if ( (LA95_6==EOF||(LA95_6 >= ABSOLUTE && LA95_6 <= ABSTRACT)||LA95_6==ALIGN||(LA95_6 >= ASSEMBLER && LA95_6 <= ASSEMBLY)||(LA95_6 >= AT && LA95_6 <= AUTOMATED)||(LA95_6 >= CASE && LA95_6 <= CLASS)||(LA95_6 >= CONST && LA95_6 <= CONTAINS)||(LA95_6 >= DEFAULT && LA95_6 <= DEPRECATED)||(LA95_6 >= DESTRUCTOR && LA95_6 <= DISPID)||LA95_6==DYNAMIC||LA95_6==END||(LA95_6 >= EXPERIMENTAL && LA95_6 <= EXPORT)||LA95_6==EXTERNAL||LA95_6==FAR||LA95_6==FINAL||(LA95_6 >= FORWARD && LA95_6 <= FUNCTION)||LA95_6==HELPER||LA95_6==IMPLEMENTS||LA95_6==INDEX||LA95_6==LABEL||LA95_6==LOCAL||LA95_6==MESSAGE||(LA95_6 >= NAME && LA95_6 <= NEAR)||LA95_6==NODEFAULT||(LA95_6 >= ON && LA95_6 <= OPERATOR)||(LA95_6 >= OUT && LA95_6 <= OVERRIDE)||LA95_6==PACKAGE||LA95_6==PAREN_BRACKET_LEFT||(LA95_6 >= PASCAL && LA95_6 <= PLATFORM)||(LA95_6 >= PRIVATE && LA95_6 <= PROCEDURE)||(LA95_6 >= PROPERTY && LA95_6 <= PUBLISHED)||(LA95_6 >= READ && LA95_6 <= READONLY)||(LA95_6 >= REFERENCE && LA95_6 <= REINTRODUCE)||(LA95_6 >= REQUIRES && LA95_6 <= RESOURCESTRING)||(LA95_6 >= SAFECALL && LA95_6 <= SEALED)||LA95_6==SQUARE_BRACKET_LEFT||(LA95_6 >= STATIC && LA95_6 <= STRICT)||LA95_6==THREADVAR||LA95_6==TYPE||LA95_6==TkIdentifier||LA95_6==UNSAFE||(LA95_6 >= VAR && LA95_6 <= VIRTUAL)||LA95_6==WINAPI||(LA95_6 >= WRITE && LA95_6 <= WRITEONLY)) ) {
alt95=1;
}
else if ( ((LA95_6 >= COLON && LA95_6 <= COMMA)) ) {
alt95=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 95, 6, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CLASS:
case CONST:
case CONSTRUCTOR:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DESTRUCTOR:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case FUNCTION:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PAREN_BRACKET_LEFT:
case PASCAL:
case PLATFORM:
case PROCEDURE:
case PROPERTY:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case RESOURCESTRING:
case SAFECALL:
case SEALED:
case SQUARE_BRACKET_LEFT:
case STATIC:
case STDCALL:
case STORED:
case THREADVAR:
case TYPE:
case TkIdentifier:
case UNSAFE:
case VAR:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt95=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 95, 0, input);
throw nvae;
}
switch (alt95) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:627:32: visibility ( visibilitySectionItem )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_visibility_in_visibilitySection_7838);
visibility313=visibility();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibility313.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:627:43: ( visibilitySectionItem )*
loop93:
while (true) {
int alt93=2;
switch ( input.LA(1) ) {
case STRICT:
{
int LA93_2 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PROTECTED:
{
int LA93_3 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PRIVATE:
{
int LA93_4 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PUBLIC:
{
int LA93_5 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PUBLISHED:
{
int LA93_6 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case AUTOMATED:
{
int LA93_7 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case CLASS:
{
int LA93_8 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case THREADVAR:
case VAR:
{
int LA93_9 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA93_10 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case TkIdentifier:
{
int LA93_11 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case OPERATOR:
{
int LA93_12 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA93_13 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case FUNCTION:
{
int LA93_14 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PROCEDURE:
{
int LA93_15 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case CONSTRUCTOR:
case DESTRUCTOR:
{
int LA93_16 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case PROPERTY:
{
int LA93_17 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case CONST:
{
int LA93_18 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case RESOURCESTRING:
{
int LA93_19 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
case TYPE:
{
int LA93_20 = input.LA(2);
if ( (synpred140_Delphi()) ) {
alt93=1;
}
}
break;
}
switch (alt93) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:627:43: visibilitySectionItem
{
pushFollow(FOLLOW_visibilitySectionItem_in_visibilitySection_7840);
visibilitySectionItem314=visibilitySectionItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibilitySectionItem314.getTree());
}
break;
default :
break loop93;
}
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:628:32: ( visibilitySectionItem )+
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:628:32: ( visibilitySectionItem )+
int cnt94=0;
loop94:
while (true) {
int alt94=2;
switch ( input.LA(1) ) {
case STRICT:
{
int LA94_2 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PROTECTED:
{
int LA94_3 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PRIVATE:
{
int LA94_4 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PUBLIC:
{
int LA94_5 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PUBLISHED:
{
int LA94_6 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case AUTOMATED:
{
int LA94_7 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case CLASS:
{
int LA94_8 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case THREADVAR:
case VAR:
{
int LA94_9 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA94_10 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case TkIdentifier:
{
int LA94_11 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case OPERATOR:
{
int LA94_12 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA94_13 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case FUNCTION:
{
int LA94_14 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PROCEDURE:
{
int LA94_15 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case CONSTRUCTOR:
case DESTRUCTOR:
{
int LA94_16 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case PROPERTY:
{
int LA94_17 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case CONST:
{
int LA94_18 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case RESOURCESTRING:
{
int LA94_19 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
case TYPE:
{
int LA94_20 = input.LA(2);
if ( (synpred142_Delphi()) ) {
alt94=1;
}
}
break;
}
switch (alt94) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:628:32: visibilitySectionItem
{
pushFollow(FOLLOW_visibilitySectionItem_in_visibilitySection_7874);
visibilitySectionItem315=visibilitySectionItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibilitySectionItem315.getTree());
}
break;
default :
if ( cnt94 >= 1 ) break loop94;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(94, input);
throw eee;
}
cnt94++;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 80, visibilitySection__StartIndex); }
}
return retval;
}
// $ANTLR end "visibilitySection_"
public static class visibilitySectionItem_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "visibilitySectionItem"
// au/com/integradev/delphi/antlr/Delphi.g:630:1: visibilitySectionItem : ( fieldSection | routineInterface | methodResolutionClause | property | constSection | innerTypeSection );
public final DelphiParser.visibilitySectionItem_return visibilitySectionItem() throws RecognitionException {
DelphiParser.visibilitySectionItem_return retval = new DelphiParser.visibilitySectionItem_return();
retval.start = input.LT(1);
int visibilitySectionItem_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope fieldSection316 =null;
ParserRuleReturnScope routineInterface317 =null;
ParserRuleReturnScope methodResolutionClause318 =null;
ParserRuleReturnScope property319 =null;
ParserRuleReturnScope constSection320 =null;
ParserRuleReturnScope innerTypeSection321 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 81) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:630:30: ( fieldSection | routineInterface | methodResolutionClause | property | constSection | innerTypeSection )
int alt96=6;
switch ( input.LA(1) ) {
case CLASS:
{
int LA96_1 = input.LA(2);
if ( (synpred143_Delphi()) ) {
alt96=1;
}
else if ( (synpred144_Delphi()) ) {
alt96=2;
}
else if ( (synpred146_Delphi()) ) {
alt96=4;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 96, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case THREADVAR:
case TkIdentifier:
case UNSAFE:
case VAR:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt96=1;
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA96_3 = input.LA(2);
if ( (synpred143_Delphi()) ) {
alt96=1;
}
else if ( (synpred144_Delphi()) ) {
alt96=2;
}
else if ( (synpred146_Delphi()) ) {
alt96=4;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 96, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case OPERATOR:
{
int LA96_5 = input.LA(2);
if ( (synpred143_Delphi()) ) {
alt96=1;
}
else if ( (synpred144_Delphi()) ) {
alt96=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 96, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FUNCTION:
{
int LA96_7 = input.LA(2);
if ( (synpred144_Delphi()) ) {
alt96=2;
}
else if ( (synpred145_Delphi()) ) {
alt96=3;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 96, 7, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PROCEDURE:
{
int LA96_8 = input.LA(2);
if ( (synpred144_Delphi()) ) {
alt96=2;
}
else if ( (synpred145_Delphi()) ) {
alt96=3;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 96, 8, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case CONSTRUCTOR:
case DESTRUCTOR:
{
alt96=2;
}
break;
case PROPERTY:
{
alt96=4;
}
break;
case CONST:
case RESOURCESTRING:
{
alt96=5;
}
break;
case TYPE:
{
alt96=6;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 96, 0, input);
throw nvae;
}
switch (alt96) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:630:32: fieldSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fieldSection_in_visibilitySectionItem7919);
fieldSection316=fieldSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fieldSection316.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:631:32: routineInterface
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_routineInterface_in_visibilitySectionItem7952);
routineInterface317=routineInterface();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineInterface317.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:632:32: methodResolutionClause
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_methodResolutionClause_in_visibilitySectionItem7985);
methodResolutionClause318=methodResolutionClause();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, methodResolutionClause318.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:633:32: property
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_property_in_visibilitySectionItem8018);
property319=property();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, property319.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:634:32: constSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_constSection_in_visibilitySectionItem8051);
constSection320=constSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constSection320.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:635:32: innerTypeSection
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_innerTypeSection_in_visibilitySectionItem8084);
innerTypeSection321=innerTypeSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, innerTypeSection321.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 81, visibilitySectionItem_StartIndex); }
}
return retval;
}
// $ANTLR end "visibilitySectionItem"
public static class fieldSectionKey_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fieldSectionKey"
// au/com/integradev/delphi/antlr/Delphi.g:637:1: fieldSectionKey : ( VAR | THREADVAR );
public final DelphiParser.fieldSectionKey_return fieldSectionKey() throws RecognitionException {
DelphiParser.fieldSectionKey_return retval = new DelphiParser.fieldSectionKey_return();
retval.start = input.LT(1);
int fieldSectionKey_StartIndex = input.index();
Object root_0 = null;
Token set322=null;
Object set322_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 82) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:637:30: ( VAR | THREADVAR )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set322=input.LT(1);
if ( input.LA(1)==THREADVAR||input.LA(1)==VAR ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set322));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 82, fieldSectionKey_StartIndex); }
}
return retval;
}
// $ANTLR end "fieldSectionKey"
public static class fieldSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fieldSection"
// au/com/integradev/delphi/antlr/Delphi.g:640:1: fieldSection : ( ( CLASS )? fieldSectionKey ( fieldDecl )* -> ^( TkFieldSection ( CLASS )? fieldSectionKey ( fieldDecl )* ) | ( fieldDecl )+ -> ^( TkFieldSection ( fieldDecl )+ ) );
public final DelphiParser.fieldSection_return fieldSection() throws RecognitionException {
DelphiParser.fieldSection_return retval = new DelphiParser.fieldSection_return();
retval.start = input.LT(1);
int fieldSection_StartIndex = input.index();
Object root_0 = null;
Token CLASS323=null;
ParserRuleReturnScope fieldSectionKey324 =null;
ParserRuleReturnScope fieldDecl325 =null;
ParserRuleReturnScope fieldDecl326 =null;
Object CLASS323_tree=null;
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_fieldSectionKey=new RewriteRuleSubtreeStream(adaptor,"rule fieldSectionKey");
RewriteRuleSubtreeStream stream_fieldDecl=new RewriteRuleSubtreeStream(adaptor,"rule fieldDecl");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 83) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:640:30: ( ( CLASS )? fieldSectionKey ( fieldDecl )* -> ^( TkFieldSection ( CLASS )? fieldSectionKey ( fieldDecl )* ) | ( fieldDecl )+ -> ^( TkFieldSection ( fieldDecl )+ ) )
int alt100=2;
int LA100_0 = input.LA(1);
if ( (LA100_0==CLASS||LA100_0==THREADVAR||LA100_0==VAR) ) {
alt100=1;
}
else if ( ((LA100_0 >= ABSOLUTE && LA100_0 <= ABSTRACT)||LA100_0==ALIGN||(LA100_0 >= ASSEMBLER && LA100_0 <= ASSEMBLY)||(LA100_0 >= AT && LA100_0 <= AUTOMATED)||LA100_0==CDECL||LA100_0==CONTAINS||(LA100_0 >= DEFAULT && LA100_0 <= DEPRECATED)||LA100_0==DISPID||LA100_0==DYNAMIC||(LA100_0 >= EXPERIMENTAL && LA100_0 <= EXPORT)||LA100_0==EXTERNAL||LA100_0==FAR||LA100_0==FINAL||LA100_0==FORWARD||LA100_0==HELPER||LA100_0==IMPLEMENTS||LA100_0==INDEX||LA100_0==LABEL||LA100_0==LOCAL||LA100_0==MESSAGE||(LA100_0 >= NAME && LA100_0 <= NEAR)||LA100_0==NODEFAULT||(LA100_0 >= ON && LA100_0 <= OPERATOR)||(LA100_0 >= OUT && LA100_0 <= OVERRIDE)||LA100_0==PACKAGE||LA100_0==PAREN_BRACKET_LEFT||(LA100_0 >= PASCAL && LA100_0 <= PLATFORM)||LA100_0==PRIVATE||(LA100_0 >= PROTECTED && LA100_0 <= PUBLISHED)||(LA100_0 >= READ && LA100_0 <= READONLY)||(LA100_0 >= REFERENCE && LA100_0 <= REINTRODUCE)||(LA100_0 >= REQUIRES && LA100_0 <= RESIDENT)||(LA100_0 >= SAFECALL && LA100_0 <= SEALED)||LA100_0==SQUARE_BRACKET_LEFT||(LA100_0 >= STATIC && LA100_0 <= STRICT)||LA100_0==TkIdentifier||LA100_0==UNSAFE||(LA100_0 >= VARARGS && LA100_0 <= VIRTUAL)||LA100_0==WINAPI||(LA100_0 >= WRITE && LA100_0 <= WRITEONLY)) ) {
alt100=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 100, 0, input);
throw nvae;
}
switch (alt100) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:640:32: ( CLASS )? fieldSectionKey ( fieldDecl )*
{
// au/com/integradev/delphi/antlr/Delphi.g:640:32: ( CLASS )?
int alt97=2;
int LA97_0 = input.LA(1);
if ( (LA97_0==CLASS) ) {
alt97=1;
}
switch (alt97) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:640:32: CLASS
{
CLASS323=(Token)match(input,CLASS,FOLLOW_CLASS_in_fieldSection8220); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS323);
}
break;
}
pushFollow(FOLLOW_fieldSectionKey_in_fieldSection8223);
fieldSectionKey324=fieldSectionKey();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_fieldSectionKey.add(fieldSectionKey324.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:640:55: ( fieldDecl )*
loop98:
while (true) {
int alt98=2;
switch ( input.LA(1) ) {
case STRICT:
{
int LA98_2 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case PROTECTED:
{
int LA98_3 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case PRIVATE:
{
int LA98_4 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case PUBLIC:
{
int LA98_5 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case PUBLISHED:
{
int LA98_6 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case AUTOMATED:
{
int LA98_7 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA98_10 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case TkIdentifier:
{
int LA98_11 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case OPERATOR:
{
int LA98_12 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA98_13 = input.LA(2);
if ( (synpred150_Delphi()) ) {
alt98=1;
}
}
break;
}
switch (alt98) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:640:55: fieldDecl
{
pushFollow(FOLLOW_fieldDecl_in_fieldSection8225);
fieldDecl325=fieldDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_fieldDecl.add(fieldDecl325.getTree());
}
break;
default :
break loop98;
}
}
// AST REWRITE
// elements: fieldSectionKey, CLASS, fieldDecl
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 640:66: -> ^( TkFieldSection ( CLASS )? fieldSectionKey ( fieldDecl )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:640:69: ^( TkFieldSection ( CLASS )? fieldSectionKey ( fieldDecl )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new FieldSectionNodeImpl(TkFieldSection), root_1);
// au/com/integradev/delphi/antlr/Delphi.g:640:108: ( CLASS )?
if ( stream_CLASS.hasNext() ) {
adaptor.addChild(root_1, stream_CLASS.nextNode());
}
stream_CLASS.reset();
adaptor.addChild(root_1, stream_fieldSectionKey.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:640:131: ( fieldDecl )*
while ( stream_fieldDecl.hasNext() ) {
adaptor.addChild(root_1, stream_fieldDecl.nextTree());
}
stream_fieldDecl.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:641:32: ( fieldDecl )+
{
// au/com/integradev/delphi/antlr/Delphi.g:641:32: ( fieldDecl )+
int cnt99=0;
loop99:
while (true) {
int alt99=2;
switch ( input.LA(1) ) {
case STRICT:
{
int LA99_2 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case PROTECTED:
{
int LA99_3 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case PRIVATE:
{
int LA99_4 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case PUBLIC:
{
int LA99_5 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case PUBLISHED:
{
int LA99_6 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case AUTOMATED:
{
int LA99_7 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA99_8 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case TkIdentifier:
{
int LA99_9 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case OPERATOR:
{
int LA99_10 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA99_11 = input.LA(2);
if ( (synpred152_Delphi()) ) {
alt99=1;
}
}
break;
}
switch (alt99) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:641:32: fieldDecl
{
pushFollow(FOLLOW_fieldDecl_in_fieldSection8276);
fieldDecl326=fieldDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_fieldDecl.add(fieldDecl326.getTree());
}
break;
default :
if ( cnt99 >= 1 ) break loop99;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(99, input);
throw eee;
}
cnt99++;
}
// AST REWRITE
// elements: fieldDecl
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 641:43: -> ^( TkFieldSection ( fieldDecl )+ )
{
// au/com/integradev/delphi/antlr/Delphi.g:641:46: ^( TkFieldSection ( fieldDecl )+ )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new FieldSectionNodeImpl(TkFieldSection), root_1);
if ( !(stream_fieldDecl.hasNext()) ) {
throw new RewriteEarlyExitException();
}
while ( stream_fieldDecl.hasNext() ) {
adaptor.addChild(root_1, stream_fieldDecl.nextTree());
}
stream_fieldDecl.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 83, fieldSection_StartIndex); }
}
return retval;
}
// $ANTLR end "fieldSection"
public static class fieldDecl_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fieldDecl"
// au/com/integradev/delphi/antlr/Delphi.g:643:1: fieldDecl : ( attributeList )? nameDeclarationList ':' varType ( portabilityDirective )* ( ';' )? -> ^( TkFieldDeclaration nameDeclarationList varType ( portabilityDirective )* ( attributeList )? ( ';' )? ) ;
public final DelphiParser.fieldDecl_return fieldDecl() throws RecognitionException {
DelphiParser.fieldDecl_return retval = new DelphiParser.fieldDecl_return();
retval.start = input.LT(1);
int fieldDecl_StartIndex = input.index();
Object root_0 = null;
Token char_literal329=null;
Token char_literal332=null;
ParserRuleReturnScope attributeList327 =null;
ParserRuleReturnScope nameDeclarationList328 =null;
ParserRuleReturnScope varType330 =null;
ParserRuleReturnScope portabilityDirective331 =null;
Object char_literal329_tree=null;
Object char_literal332_tree=null;
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_portabilityDirective=new RewriteRuleSubtreeStream(adaptor,"rule portabilityDirective");
RewriteRuleSubtreeStream stream_nameDeclarationList=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclarationList");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 84) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:643:30: ( ( attributeList )? nameDeclarationList ':' varType ( portabilityDirective )* ( ';' )? -> ^( TkFieldDeclaration nameDeclarationList varType ( portabilityDirective )* ( attributeList )? ( ';' )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:643:32: ( attributeList )? nameDeclarationList ':' varType ( portabilityDirective )* ( ';' )?
{
// au/com/integradev/delphi/antlr/Delphi.g:643:32: ( attributeList )?
int alt101=2;
int LA101_0 = input.LA(1);
if ( (LA101_0==PAREN_BRACKET_LEFT||LA101_0==SQUARE_BRACKET_LEFT) ) {
alt101=1;
}
switch (alt101) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:643:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_fieldDecl8345);
attributeList327=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList327.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclarationList_in_fieldDecl8348);
nameDeclarationList328=nameDeclarationList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclarationList.add(nameDeclarationList328.getTree());
char_literal329=(Token)match(input,COLON,FOLLOW_COLON_in_fieldDecl8350); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal329);
pushFollow(FOLLOW_varType_in_fieldDecl8352);
varType330=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType330.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:643:79: ( portabilityDirective )*
loop102:
while (true) {
int alt102=2;
switch ( input.LA(1) ) {
case DEPRECATED:
{
int LA102_2 = input.LA(2);
if ( (LA102_2==EOF||(LA102_2 >= ABSOLUTE && LA102_2 <= ABSTRACT)||LA102_2==ALIGN||(LA102_2 >= ASSEMBLER && LA102_2 <= ASSEMBLY)||(LA102_2 >= AT && LA102_2 <= AUTOMATED)||(LA102_2 >= CASE && LA102_2 <= CLASS)||(LA102_2 >= CONST && LA102_2 <= CONTAINS)||(LA102_2 >= DEFAULT && LA102_2 <= DISPID)||LA102_2==DYNAMIC||LA102_2==END||(LA102_2 >= EXPERIMENTAL && LA102_2 <= EXPORT)||LA102_2==EXTERNAL||LA102_2==FAR||LA102_2==FINAL||(LA102_2 >= FORWARD && LA102_2 <= FUNCTION)||LA102_2==HELPER||LA102_2==IMPLEMENTS||LA102_2==INDEX||LA102_2==LABEL||(LA102_2 >= LIBRARY && LA102_2 <= LOCAL)||LA102_2==MESSAGE||(LA102_2 >= NAME && LA102_2 <= NEAR)||LA102_2==NODEFAULT||(LA102_2 >= ON && LA102_2 <= OPERATOR)||(LA102_2 >= OUT && LA102_2 <= OVERRIDE)||LA102_2==PACKAGE||LA102_2==PAREN_BRACKET_LEFT||(LA102_2 >= PAREN_RIGHT && LA102_2 <= PLATFORM)||(LA102_2 >= PRIVATE && LA102_2 <= PROCEDURE)||(LA102_2 >= PROPERTY && LA102_2 <= PUBLISHED)||(LA102_2 >= READ && LA102_2 <= READONLY)||(LA102_2 >= REFERENCE && LA102_2 <= REINTRODUCE)||(LA102_2 >= REQUIRES && LA102_2 <= RESOURCESTRING)||(LA102_2 >= SAFECALL && LA102_2 <= SEMICOLON)||LA102_2==SQUARE_BRACKET_LEFT||(LA102_2 >= STATIC && LA102_2 <= STRICT)||LA102_2==THREADVAR||LA102_2==TYPE||LA102_2==TkCharacterEscapeCode||LA102_2==TkIdentifier||LA102_2==TkMultilineString||LA102_2==TkQuotedString||LA102_2==UNSAFE||(LA102_2 >= VAR && LA102_2 <= VIRTUAL)||LA102_2==WINAPI||(LA102_2 >= WRITE && LA102_2 <= WRITEONLY)) ) {
alt102=1;
}
}
break;
case EXPERIMENTAL:
{
int LA102_3 = input.LA(2);
if ( (LA102_3==EOF||(LA102_3 >= ABSOLUTE && LA102_3 <= ABSTRACT)||LA102_3==ALIGN||(LA102_3 >= ASSEMBLER && LA102_3 <= ASSEMBLY)||(LA102_3 >= AT && LA102_3 <= AUTOMATED)||(LA102_3 >= CASE && LA102_3 <= CLASS)||(LA102_3 >= CONST && LA102_3 <= CONTAINS)||(LA102_3 >= DEFAULT && LA102_3 <= DEPRECATED)||(LA102_3 >= DESTRUCTOR && LA102_3 <= DISPID)||LA102_3==DYNAMIC||LA102_3==END||(LA102_3 >= EXPERIMENTAL && LA102_3 <= EXPORT)||LA102_3==EXTERNAL||LA102_3==FAR||LA102_3==FINAL||(LA102_3 >= FORWARD && LA102_3 <= FUNCTION)||LA102_3==HELPER||LA102_3==IMPLEMENTS||LA102_3==INDEX||LA102_3==LABEL||(LA102_3 >= LIBRARY && LA102_3 <= LOCAL)||LA102_3==MESSAGE||(LA102_3 >= NAME && LA102_3 <= NEAR)||LA102_3==NODEFAULT||(LA102_3 >= ON && LA102_3 <= OPERATOR)||(LA102_3 >= OUT && LA102_3 <= OVERRIDE)||LA102_3==PACKAGE||LA102_3==PAREN_BRACKET_LEFT||(LA102_3 >= PAREN_RIGHT && LA102_3 <= PLATFORM)||(LA102_3 >= PRIVATE && LA102_3 <= PROCEDURE)||(LA102_3 >= PROPERTY && LA102_3 <= PUBLISHED)||(LA102_3 >= READ && LA102_3 <= READONLY)||(LA102_3 >= REFERENCE && LA102_3 <= REINTRODUCE)||(LA102_3 >= REQUIRES && LA102_3 <= RESOURCESTRING)||(LA102_3 >= SAFECALL && LA102_3 <= SEMICOLON)||LA102_3==SQUARE_BRACKET_LEFT||(LA102_3 >= STATIC && LA102_3 <= STRICT)||LA102_3==THREADVAR||LA102_3==TYPE||LA102_3==TkIdentifier||LA102_3==UNSAFE||(LA102_3 >= VAR && LA102_3 <= VIRTUAL)||LA102_3==WINAPI||(LA102_3 >= WRITE && LA102_3 <= WRITEONLY)) ) {
alt102=1;
}
}
break;
case PLATFORM:
{
int LA102_4 = input.LA(2);
if ( (LA102_4==EOF||(LA102_4 >= ABSOLUTE && LA102_4 <= ABSTRACT)||LA102_4==ALIGN||(LA102_4 >= ASSEMBLER && LA102_4 <= ASSEMBLY)||(LA102_4 >= AT && LA102_4 <= AUTOMATED)||(LA102_4 >= CASE && LA102_4 <= CLASS)||(LA102_4 >= CONST && LA102_4 <= CONTAINS)||(LA102_4 >= DEFAULT && LA102_4 <= DEPRECATED)||(LA102_4 >= DESTRUCTOR && LA102_4 <= DISPID)||LA102_4==DYNAMIC||LA102_4==END||(LA102_4 >= EXPERIMENTAL && LA102_4 <= EXPORT)||LA102_4==EXTERNAL||LA102_4==FAR||LA102_4==FINAL||(LA102_4 >= FORWARD && LA102_4 <= FUNCTION)||LA102_4==HELPER||LA102_4==IMPLEMENTS||LA102_4==INDEX||LA102_4==LABEL||(LA102_4 >= LIBRARY && LA102_4 <= LOCAL)||LA102_4==MESSAGE||(LA102_4 >= NAME && LA102_4 <= NEAR)||LA102_4==NODEFAULT||(LA102_4 >= ON && LA102_4 <= OPERATOR)||(LA102_4 >= OUT && LA102_4 <= OVERRIDE)||LA102_4==PACKAGE||LA102_4==PAREN_BRACKET_LEFT||(LA102_4 >= PAREN_RIGHT && LA102_4 <= PLATFORM)||(LA102_4 >= PRIVATE && LA102_4 <= PROCEDURE)||(LA102_4 >= PROPERTY && LA102_4 <= PUBLISHED)||(LA102_4 >= READ && LA102_4 <= READONLY)||(LA102_4 >= REFERENCE && LA102_4 <= REINTRODUCE)||(LA102_4 >= REQUIRES && LA102_4 <= RESOURCESTRING)||(LA102_4 >= SAFECALL && LA102_4 <= SEMICOLON)||LA102_4==SQUARE_BRACKET_LEFT||(LA102_4 >= STATIC && LA102_4 <= STRICT)||LA102_4==THREADVAR||LA102_4==TYPE||LA102_4==TkIdentifier||LA102_4==UNSAFE||(LA102_4 >= VAR && LA102_4 <= VIRTUAL)||LA102_4==WINAPI||(LA102_4 >= WRITE && LA102_4 <= WRITEONLY)) ) {
alt102=1;
}
}
break;
case LIBRARY:
{
alt102=1;
}
break;
}
switch (alt102) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:643:79: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_fieldDecl8354);
portabilityDirective331=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_portabilityDirective.add(portabilityDirective331.getTree());
}
break;
default :
break loop102;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:643:101: ( ';' )?
int alt103=2;
int LA103_0 = input.LA(1);
if ( (LA103_0==SEMICOLON) ) {
alt103=1;
}
switch (alt103) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:643:101: ';'
{
char_literal332=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_fieldDecl8357); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal332);
}
break;
}
// AST REWRITE
// elements: attributeList, SEMICOLON, varType, nameDeclarationList, portabilityDirective
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 644:30: -> ^( TkFieldDeclaration nameDeclarationList varType ( portabilityDirective )* ( attributeList )? ( ';' )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:644:33: ^( TkFieldDeclaration nameDeclarationList varType ( portabilityDirective )* ( attributeList )? ( ';' )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new FieldDeclarationNodeImpl(TkFieldDeclaration), root_1);
adaptor.addChild(root_1, stream_nameDeclarationList.nextTree());
adaptor.addChild(root_1, stream_varType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:644:108: ( portabilityDirective )*
while ( stream_portabilityDirective.hasNext() ) {
adaptor.addChild(root_1, stream_portabilityDirective.nextTree());
}
stream_portabilityDirective.reset();
// au/com/integradev/delphi/antlr/Delphi.g:644:130: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:644:145: ( ';' )?
if ( stream_SEMICOLON.hasNext() ) {
adaptor.addChild(root_1, stream_SEMICOLON.nextNode());
}
stream_SEMICOLON.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 84, fieldDecl_StartIndex); }
}
return retval;
}
// $ANTLR end "fieldDecl"
public static class classHelperType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "classHelperType"
// au/com/integradev/delphi/antlr/Delphi.g:646:1: classHelperType : CLASS ^ HELPER ( classParent )? FOR typeReference ( visibilitySection )* END ;
public final DelphiParser.classHelperType_return classHelperType() throws RecognitionException {
DelphiParser.classHelperType_return retval = new DelphiParser.classHelperType_return();
retval.start = input.LT(1);
int classHelperType_StartIndex = input.index();
Object root_0 = null;
Token CLASS333=null;
Token HELPER334=null;
Token FOR336=null;
Token END339=null;
ParserRuleReturnScope classParent335 =null;
ParserRuleReturnScope typeReference337 =null;
ParserRuleReturnScope visibilitySection338 =null;
Object CLASS333_tree=null;
Object HELPER334_tree=null;
Object FOR336_tree=null;
Object END339_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 85) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:646:30: ( CLASS ^ HELPER ( classParent )? FOR typeReference ( visibilitySection )* END )
// au/com/integradev/delphi/antlr/Delphi.g:646:32: CLASS ^ HELPER ( classParent )? FOR typeReference ( visibilitySection )* END
{
root_0 = (Object)adaptor.nil();
CLASS333=(Token)match(input,CLASS,FOLLOW_CLASS_in_classHelperType8459); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CLASS333_tree = new ClassHelperTypeNodeImpl(CLASS333) ;
root_0 = (Object)adaptor.becomeRoot(CLASS333_tree, root_0);
}
HELPER334=(Token)match(input,HELPER,FOLLOW_HELPER_in_classHelperType8465); if (state.failed) return retval;
if ( state.backtracking==0 ) {
HELPER334_tree = (Object)adaptor.create(HELPER334);
adaptor.addChild(root_0, HELPER334_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:646:71: ( classParent )?
int alt104=2;
int LA104_0 = input.LA(1);
if ( (LA104_0==PAREN_LEFT) ) {
alt104=1;
}
switch (alt104) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:646:71: classParent
{
pushFollow(FOLLOW_classParent_in_classHelperType8467);
classParent335=classParent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, classParent335.getTree());
}
break;
}
FOR336=(Token)match(input,FOR,FOLLOW_FOR_in_classHelperType8470); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FOR336_tree = (Object)adaptor.create(FOR336);
adaptor.addChild(root_0, FOR336_tree);
}
pushFollow(FOLLOW_typeReference_in_classHelperType8472);
typeReference337=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference337.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:646:102: ( visibilitySection )*
loop105:
while (true) {
int alt105=2;
int LA105_0 = input.LA(1);
if ( ((LA105_0 >= ABSOLUTE && LA105_0 <= ABSTRACT)||LA105_0==ALIGN||(LA105_0 >= ASSEMBLER && LA105_0 <= ASSEMBLY)||(LA105_0 >= AT && LA105_0 <= AUTOMATED)||(LA105_0 >= CDECL && LA105_0 <= CLASS)||(LA105_0 >= CONST && LA105_0 <= CONTAINS)||(LA105_0 >= DEFAULT && LA105_0 <= DEPRECATED)||(LA105_0 >= DESTRUCTOR && LA105_0 <= DISPID)||LA105_0==DYNAMIC||(LA105_0 >= EXPERIMENTAL && LA105_0 <= EXPORT)||LA105_0==EXTERNAL||LA105_0==FAR||LA105_0==FINAL||(LA105_0 >= FORWARD && LA105_0 <= FUNCTION)||LA105_0==HELPER||LA105_0==IMPLEMENTS||LA105_0==INDEX||LA105_0==LABEL||LA105_0==LOCAL||LA105_0==MESSAGE||(LA105_0 >= NAME && LA105_0 <= NEAR)||LA105_0==NODEFAULT||(LA105_0 >= ON && LA105_0 <= OPERATOR)||(LA105_0 >= OUT && LA105_0 <= OVERRIDE)||LA105_0==PACKAGE||LA105_0==PAREN_BRACKET_LEFT||(LA105_0 >= PASCAL && LA105_0 <= PLATFORM)||(LA105_0 >= PRIVATE && LA105_0 <= PROCEDURE)||(LA105_0 >= PROPERTY && LA105_0 <= PUBLISHED)||(LA105_0 >= READ && LA105_0 <= READONLY)||(LA105_0 >= REFERENCE && LA105_0 <= REINTRODUCE)||(LA105_0 >= REQUIRES && LA105_0 <= RESOURCESTRING)||(LA105_0 >= SAFECALL && LA105_0 <= SEALED)||LA105_0==SQUARE_BRACKET_LEFT||(LA105_0 >= STATIC && LA105_0 <= STRICT)||LA105_0==THREADVAR||LA105_0==TYPE||LA105_0==TkIdentifier||LA105_0==UNSAFE||(LA105_0 >= VAR && LA105_0 <= VIRTUAL)||LA105_0==WINAPI||(LA105_0 >= WRITE && LA105_0 <= WRITEONLY)) ) {
alt105=1;
}
switch (alt105) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:646:102: visibilitySection
{
pushFollow(FOLLOW_visibilitySection_in_classHelperType8474);
visibilitySection338=visibilitySection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibilitySection338.getTree());
}
break;
default :
break loop105;
}
}
END339=(Token)match(input,END,FOLLOW_END_in_classHelperType8477); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END339_tree = (Object)adaptor.create(END339);
adaptor.addChild(root_0, END339_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 85, classHelperType_StartIndex); }
}
return retval;
}
// $ANTLR end "classHelperType"
public static class interfaceType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceType"
// au/com/integradev/delphi/antlr/Delphi.g:648:1: interfaceType : ( INTERFACE ^| DISPINTERFACE ^) ( classParent )? ( ( interfaceGuid )? ( interfaceItems )? END )? ;
public final DelphiParser.interfaceType_return interfaceType() throws RecognitionException {
DelphiParser.interfaceType_return retval = new DelphiParser.interfaceType_return();
retval.start = input.LT(1);
int interfaceType_StartIndex = input.index();
Object root_0 = null;
Token INTERFACE340=null;
Token DISPINTERFACE341=null;
Token END345=null;
ParserRuleReturnScope classParent342 =null;
ParserRuleReturnScope interfaceGuid343 =null;
ParserRuleReturnScope interfaceItems344 =null;
Object INTERFACE340_tree=null;
Object DISPINTERFACE341_tree=null;
Object END345_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 86) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:648:30: ( ( INTERFACE ^| DISPINTERFACE ^) ( classParent )? ( ( interfaceGuid )? ( interfaceItems )? END )? )
// au/com/integradev/delphi/antlr/Delphi.g:648:32: ( INTERFACE ^| DISPINTERFACE ^) ( classParent )? ( ( interfaceGuid )? ( interfaceItems )? END )?
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:648:32: ( INTERFACE ^| DISPINTERFACE ^)
int alt106=2;
int LA106_0 = input.LA(1);
if ( (LA106_0==INTERFACE) ) {
alt106=1;
}
else if ( (LA106_0==DISPINTERFACE) ) {
alt106=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 106, 0, input);
throw nvae;
}
switch (alt106) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:648:33: INTERFACE ^
{
INTERFACE340=(Token)match(input,INTERFACE,FOLLOW_INTERFACE_in_interfaceType8530); if (state.failed) return retval;
if ( state.backtracking==0 ) {
INTERFACE340_tree = new InterfaceTypeNodeImpl(INTERFACE340) ;
root_0 = (Object)adaptor.becomeRoot(INTERFACE340_tree, root_0);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:648:69: DISPINTERFACE ^
{
DISPINTERFACE341=(Token)match(input,DISPINTERFACE,FOLLOW_DISPINTERFACE_in_interfaceType8538); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DISPINTERFACE341_tree = new InterfaceTypeNodeImpl(DISPINTERFACE341) ;
root_0 = (Object)adaptor.becomeRoot(DISPINTERFACE341_tree, root_0);
}
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:648:108: ( classParent )?
int alt107=2;
int LA107_0 = input.LA(1);
if ( (LA107_0==PAREN_LEFT) ) {
alt107=1;
}
switch (alt107) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:648:108: classParent
{
pushFollow(FOLLOW_classParent_in_interfaceType8545);
classParent342=classParent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, classParent342.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:648:121: ( ( interfaceGuid )? ( interfaceItems )? END )?
int alt110=2;
int LA110_0 = input.LA(1);
if ( (LA110_0==CLASS||LA110_0==CONSTRUCTOR||LA110_0==DESTRUCTOR||LA110_0==END||LA110_0==FUNCTION||LA110_0==OPERATOR||LA110_0==PAREN_BRACKET_LEFT||LA110_0==PROCEDURE||LA110_0==PROPERTY||LA110_0==SQUARE_BRACKET_LEFT) ) {
alt110=1;
}
switch (alt110) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:648:122: ( interfaceGuid )? ( interfaceItems )? END
{
// au/com/integradev/delphi/antlr/Delphi.g:648:122: ( interfaceGuid )?
int alt108=2;
int LA108_0 = input.LA(1);
if ( (LA108_0==PAREN_BRACKET_LEFT||LA108_0==SQUARE_BRACKET_LEFT) ) {
int LA108_1 = input.LA(2);
if ( (synpred160_Delphi()) ) {
alt108=1;
}
}
switch (alt108) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:648:122: interfaceGuid
{
pushFollow(FOLLOW_interfaceGuid_in_interfaceType8549);
interfaceGuid343=interfaceGuid();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceGuid343.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:648:137: ( interfaceItems )?
int alt109=2;
int LA109_0 = input.LA(1);
if ( (LA109_0==CLASS||LA109_0==CONSTRUCTOR||LA109_0==DESTRUCTOR||LA109_0==FUNCTION||LA109_0==OPERATOR||LA109_0==PAREN_BRACKET_LEFT||LA109_0==PROCEDURE||LA109_0==PROPERTY||LA109_0==SQUARE_BRACKET_LEFT) ) {
alt109=1;
}
switch (alt109) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:648:137: interfaceItems
{
pushFollow(FOLLOW_interfaceItems_in_interfaceType8552);
interfaceItems344=interfaceItems();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceItems344.getTree());
}
break;
}
END345=(Token)match(input,END,FOLLOW_END_in_interfaceType8555); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END345_tree = (Object)adaptor.create(END345);
adaptor.addChild(root_0, END345_tree);
}
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 86, interfaceType_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceType"
public static class interfaceGuid_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceGuid"
// au/com/integradev/delphi/antlr/Delphi.g:650:1: interfaceGuid : lbrack expression rbrack -> ^( TkGuid expression ) ;
public final DelphiParser.interfaceGuid_return interfaceGuid() throws RecognitionException {
DelphiParser.interfaceGuid_return retval = new DelphiParser.interfaceGuid_return();
retval.start = input.LT(1);
int interfaceGuid_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope lbrack346 =null;
ParserRuleReturnScope expression347 =null;
ParserRuleReturnScope rbrack348 =null;
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
RewriteRuleSubtreeStream stream_rbrack=new RewriteRuleSubtreeStream(adaptor,"rule rbrack");
RewriteRuleSubtreeStream stream_lbrack=new RewriteRuleSubtreeStream(adaptor,"rule lbrack");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 87) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:650:30: ( lbrack expression rbrack -> ^( TkGuid expression ) )
// au/com/integradev/delphi/antlr/Delphi.g:650:32: lbrack expression rbrack
{
pushFollow(FOLLOW_lbrack_in_interfaceGuid8609);
lbrack346=lbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_lbrack.add(lbrack346.getTree());
pushFollow(FOLLOW_expression_in_interfaceGuid8611);
expression347=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression347.getTree());
pushFollow(FOLLOW_rbrack_in_interfaceGuid8613);
rbrack348=rbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_rbrack.add(rbrack348.getTree());
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 650:57: -> ^( TkGuid expression )
{
// au/com/integradev/delphi/antlr/Delphi.g:650:60: ^( TkGuid expression )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new InterfaceGuidNodeImpl(TkGuid), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 87, interfaceGuid_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceGuid"
public static class interfaceItems_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceItems"
// au/com/integradev/delphi/antlr/Delphi.g:652:1: interfaceItems : ( interfaceItem )+ -> ^( TkVisibilitySection ( interfaceItem )+ ) ;
public final DelphiParser.interfaceItems_return interfaceItems() throws RecognitionException {
DelphiParser.interfaceItems_return retval = new DelphiParser.interfaceItems_return();
retval.start = input.LT(1);
int interfaceItems_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope interfaceItem349 =null;
RewriteRuleSubtreeStream stream_interfaceItem=new RewriteRuleSubtreeStream(adaptor,"rule interfaceItem");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 88) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:652:30: ( ( interfaceItem )+ -> ^( TkVisibilitySection ( interfaceItem )+ ) )
// au/com/integradev/delphi/antlr/Delphi.g:652:32: ( interfaceItem )+
{
// au/com/integradev/delphi/antlr/Delphi.g:652:32: ( interfaceItem )+
int cnt111=0;
loop111:
while (true) {
int alt111=2;
int LA111_0 = input.LA(1);
if ( (LA111_0==CLASS||LA111_0==CONSTRUCTOR||LA111_0==DESTRUCTOR||LA111_0==FUNCTION||LA111_0==OPERATOR||LA111_0==PAREN_BRACKET_LEFT||LA111_0==PROCEDURE||LA111_0==PROPERTY||LA111_0==SQUARE_BRACKET_LEFT) ) {
alt111=1;
}
switch (alt111) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:652:32: interfaceItem
{
pushFollow(FOLLOW_interfaceItem_in_interfaceItems8675);
interfaceItem349=interfaceItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_interfaceItem.add(interfaceItem349.getTree());
}
break;
default :
if ( cnt111 >= 1 ) break loop111;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(111, input);
throw eee;
}
cnt111++;
}
// AST REWRITE
// elements: interfaceItem
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 652:47: -> ^( TkVisibilitySection ( interfaceItem )+ )
{
// au/com/integradev/delphi/antlr/Delphi.g:652:50: ^( TkVisibilitySection ( interfaceItem )+ )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new VisibilitySectionNodeImpl(TkVisibilitySection), root_1);
if ( !(stream_interfaceItem.hasNext()) ) {
throw new RewriteEarlyExitException();
}
while ( stream_interfaceItem.hasNext() ) {
adaptor.addChild(root_1, stream_interfaceItem.nextTree());
}
stream_interfaceItem.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 88, interfaceItems_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceItems"
public static class interfaceItem_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceItem"
// au/com/integradev/delphi/antlr/Delphi.g:654:1: interfaceItem : ( routineInterface | property );
public final DelphiParser.interfaceItem_return interfaceItem() throws RecognitionException {
DelphiParser.interfaceItem_return retval = new DelphiParser.interfaceItem_return();
retval.start = input.LT(1);
int interfaceItem_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope routineInterface350 =null;
ParserRuleReturnScope property351 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 89) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:654:30: ( routineInterface | property )
int alt112=2;
switch ( input.LA(1) ) {
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA112_1 = input.LA(2);
if ( (synpred164_Delphi()) ) {
alt112=1;
}
else if ( (true) ) {
alt112=2;
}
}
break;
case CLASS:
{
int LA112_2 = input.LA(2);
if ( (synpred164_Delphi()) ) {
alt112=1;
}
else if ( (true) ) {
alt112=2;
}
}
break;
case CONSTRUCTOR:
case DESTRUCTOR:
case FUNCTION:
case OPERATOR:
case PROCEDURE:
{
alt112=1;
}
break;
case PROPERTY:
{
alt112=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 112, 0, input);
throw nvae;
}
switch (alt112) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:654:32: routineInterface
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_routineInterface_in_interfaceItem8740);
routineInterface350=routineInterface();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, routineInterface350.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:655:32: property
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_property_in_interfaceItem8773);
property351=property();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, property351.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 89, interfaceItem_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceItem"
public static class objectType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "objectType"
// au/com/integradev/delphi/antlr/Delphi.g:657:1: objectType : OBJECT ^ ( classParent )? ( visibilitySection )* END ;
public final DelphiParser.objectType_return objectType() throws RecognitionException {
DelphiParser.objectType_return retval = new DelphiParser.objectType_return();
retval.start = input.LT(1);
int objectType_StartIndex = input.index();
Object root_0 = null;
Token OBJECT352=null;
Token END355=null;
ParserRuleReturnScope classParent353 =null;
ParserRuleReturnScope visibilitySection354 =null;
Object OBJECT352_tree=null;
Object END355_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 90) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:657:30: ( OBJECT ^ ( classParent )? ( visibilitySection )* END )
// au/com/integradev/delphi/antlr/Delphi.g:657:32: OBJECT ^ ( classParent )? ( visibilitySection )* END
{
root_0 = (Object)adaptor.nil();
OBJECT352=(Token)match(input,OBJECT,FOLLOW_OBJECT_in_objectType8828); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OBJECT352_tree = new ObjectTypeNodeImpl(OBJECT352) ;
root_0 = (Object)adaptor.becomeRoot(OBJECT352_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:657:60: ( classParent )?
int alt113=2;
int LA113_0 = input.LA(1);
if ( (LA113_0==PAREN_LEFT) ) {
alt113=1;
}
switch (alt113) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:657:60: classParent
{
pushFollow(FOLLOW_classParent_in_objectType8834);
classParent353=classParent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, classParent353.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:657:73: ( visibilitySection )*
loop114:
while (true) {
int alt114=2;
int LA114_0 = input.LA(1);
if ( ((LA114_0 >= ABSOLUTE && LA114_0 <= ABSTRACT)||LA114_0==ALIGN||(LA114_0 >= ASSEMBLER && LA114_0 <= ASSEMBLY)||(LA114_0 >= AT && LA114_0 <= AUTOMATED)||(LA114_0 >= CDECL && LA114_0 <= CLASS)||(LA114_0 >= CONST && LA114_0 <= CONTAINS)||(LA114_0 >= DEFAULT && LA114_0 <= DEPRECATED)||(LA114_0 >= DESTRUCTOR && LA114_0 <= DISPID)||LA114_0==DYNAMIC||(LA114_0 >= EXPERIMENTAL && LA114_0 <= EXPORT)||LA114_0==EXTERNAL||LA114_0==FAR||LA114_0==FINAL||(LA114_0 >= FORWARD && LA114_0 <= FUNCTION)||LA114_0==HELPER||LA114_0==IMPLEMENTS||LA114_0==INDEX||LA114_0==LABEL||LA114_0==LOCAL||LA114_0==MESSAGE||(LA114_0 >= NAME && LA114_0 <= NEAR)||LA114_0==NODEFAULT||(LA114_0 >= ON && LA114_0 <= OPERATOR)||(LA114_0 >= OUT && LA114_0 <= OVERRIDE)||LA114_0==PACKAGE||LA114_0==PAREN_BRACKET_LEFT||(LA114_0 >= PASCAL && LA114_0 <= PLATFORM)||(LA114_0 >= PRIVATE && LA114_0 <= PROCEDURE)||(LA114_0 >= PROPERTY && LA114_0 <= PUBLISHED)||(LA114_0 >= READ && LA114_0 <= READONLY)||(LA114_0 >= REFERENCE && LA114_0 <= REINTRODUCE)||(LA114_0 >= REQUIRES && LA114_0 <= RESOURCESTRING)||(LA114_0 >= SAFECALL && LA114_0 <= SEALED)||LA114_0==SQUARE_BRACKET_LEFT||(LA114_0 >= STATIC && LA114_0 <= STRICT)||LA114_0==THREADVAR||LA114_0==TYPE||LA114_0==TkIdentifier||LA114_0==UNSAFE||(LA114_0 >= VAR && LA114_0 <= VIRTUAL)||LA114_0==WINAPI||(LA114_0 >= WRITE && LA114_0 <= WRITEONLY)) ) {
alt114=1;
}
switch (alt114) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:657:73: visibilitySection
{
pushFollow(FOLLOW_visibilitySection_in_objectType8837);
visibilitySection354=visibilitySection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibilitySection354.getTree());
}
break;
default :
break loop114;
}
}
END355=(Token)match(input,END,FOLLOW_END_in_objectType8840); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END355_tree = (Object)adaptor.create(END355);
adaptor.addChild(root_0, END355_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 90, objectType_StartIndex); }
}
return retval;
}
// $ANTLR end "objectType"
public static class recordType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordType"
// au/com/integradev/delphi/antlr/Delphi.g:659:1: recordType : RECORD ^ ( visibilitySection )* ( recordVariantSection )? END ( ALIGN expression )? ;
public final DelphiParser.recordType_return recordType() throws RecognitionException {
DelphiParser.recordType_return retval = new DelphiParser.recordType_return();
retval.start = input.LT(1);
int recordType_StartIndex = input.index();
Object root_0 = null;
Token RECORD356=null;
Token END359=null;
Token ALIGN360=null;
ParserRuleReturnScope visibilitySection357 =null;
ParserRuleReturnScope recordVariantSection358 =null;
ParserRuleReturnScope expression361 =null;
Object RECORD356_tree=null;
Object END359_tree=null;
Object ALIGN360_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 91) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:659:30: ( RECORD ^ ( visibilitySection )* ( recordVariantSection )? END ( ALIGN expression )? )
// au/com/integradev/delphi/antlr/Delphi.g:659:32: RECORD ^ ( visibilitySection )* ( recordVariantSection )? END ( ALIGN expression )?
{
root_0 = (Object)adaptor.nil();
RECORD356=(Token)match(input,RECORD,FOLLOW_RECORD_in_recordType8961); if (state.failed) return retval;
if ( state.backtracking==0 ) {
RECORD356_tree = new RecordTypeNodeImpl(RECORD356) ;
root_0 = (Object)adaptor.becomeRoot(RECORD356_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:659:60: ( visibilitySection )*
loop115:
while (true) {
int alt115=2;
int LA115_0 = input.LA(1);
if ( ((LA115_0 >= ABSOLUTE && LA115_0 <= ABSTRACT)||LA115_0==ALIGN||(LA115_0 >= ASSEMBLER && LA115_0 <= ASSEMBLY)||(LA115_0 >= AT && LA115_0 <= AUTOMATED)||(LA115_0 >= CDECL && LA115_0 <= CLASS)||(LA115_0 >= CONST && LA115_0 <= CONTAINS)||(LA115_0 >= DEFAULT && LA115_0 <= DEPRECATED)||(LA115_0 >= DESTRUCTOR && LA115_0 <= DISPID)||LA115_0==DYNAMIC||(LA115_0 >= EXPERIMENTAL && LA115_0 <= EXPORT)||LA115_0==EXTERNAL||LA115_0==FAR||LA115_0==FINAL||(LA115_0 >= FORWARD && LA115_0 <= FUNCTION)||LA115_0==HELPER||LA115_0==IMPLEMENTS||LA115_0==INDEX||LA115_0==LABEL||LA115_0==LOCAL||LA115_0==MESSAGE||(LA115_0 >= NAME && LA115_0 <= NEAR)||LA115_0==NODEFAULT||(LA115_0 >= ON && LA115_0 <= OPERATOR)||(LA115_0 >= OUT && LA115_0 <= OVERRIDE)||LA115_0==PACKAGE||LA115_0==PAREN_BRACKET_LEFT||(LA115_0 >= PASCAL && LA115_0 <= PLATFORM)||(LA115_0 >= PRIVATE && LA115_0 <= PROCEDURE)||(LA115_0 >= PROPERTY && LA115_0 <= PUBLISHED)||(LA115_0 >= READ && LA115_0 <= READONLY)||(LA115_0 >= REFERENCE && LA115_0 <= REINTRODUCE)||(LA115_0 >= REQUIRES && LA115_0 <= RESOURCESTRING)||(LA115_0 >= SAFECALL && LA115_0 <= SEALED)||LA115_0==SQUARE_BRACKET_LEFT||(LA115_0 >= STATIC && LA115_0 <= STRICT)||LA115_0==THREADVAR||LA115_0==TYPE||LA115_0==TkIdentifier||LA115_0==UNSAFE||(LA115_0 >= VAR && LA115_0 <= VIRTUAL)||LA115_0==WINAPI||(LA115_0 >= WRITE && LA115_0 <= WRITEONLY)) ) {
alt115=1;
}
switch (alt115) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:659:60: visibilitySection
{
pushFollow(FOLLOW_visibilitySection_in_recordType8967);
visibilitySection357=visibilitySection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibilitySection357.getTree());
}
break;
default :
break loop115;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:659:79: ( recordVariantSection )?
int alt116=2;
int LA116_0 = input.LA(1);
if ( (LA116_0==CASE) ) {
alt116=1;
}
switch (alt116) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:659:79: recordVariantSection
{
pushFollow(FOLLOW_recordVariantSection_in_recordType8970);
recordVariantSection358=recordVariantSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordVariantSection358.getTree());
}
break;
}
END359=(Token)match(input,END,FOLLOW_END_in_recordType8973); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END359_tree = (Object)adaptor.create(END359);
adaptor.addChild(root_0, END359_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:659:105: ( ALIGN expression )?
int alt117=2;
alt117 = dfa117.predict(input);
switch (alt117) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:659:106: ALIGN expression
{
ALIGN360=(Token)match(input,ALIGN,FOLLOW_ALIGN_in_recordType8976); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ALIGN360_tree = (Object)adaptor.create(ALIGN360);
adaptor.addChild(root_0, ALIGN360_tree);
}
pushFollow(FOLLOW_expression_in_recordType8978);
expression361=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression361.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 91, recordType_StartIndex); }
}
return retval;
}
// $ANTLR end "recordType"
public static class recordVariantSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordVariantSection"
// au/com/integradev/delphi/antlr/Delphi.g:661:1: recordVariantSection : CASE ^ recordVariantTag OF ( recordVariant )+ ;
public final DelphiParser.recordVariantSection_return recordVariantSection() throws RecognitionException {
DelphiParser.recordVariantSection_return retval = new DelphiParser.recordVariantSection_return();
retval.start = input.LT(1);
int recordVariantSection_StartIndex = input.index();
Object root_0 = null;
Token CASE362=null;
Token OF364=null;
ParserRuleReturnScope recordVariantTag363 =null;
ParserRuleReturnScope recordVariant365 =null;
Object CASE362_tree=null;
Object OF364_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 92) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:661:30: ( CASE ^ recordVariantTag OF ( recordVariant )+ )
// au/com/integradev/delphi/antlr/Delphi.g:661:32: CASE ^ recordVariantTag OF ( recordVariant )+
{
root_0 = (Object)adaptor.nil();
CASE362=(Token)match(input,CASE,FOLLOW_CASE_in_recordVariantSection9025); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CASE362_tree = new RecordVariantSectionNodeImpl(CASE362) ;
root_0 = (Object)adaptor.becomeRoot(CASE362_tree, root_0);
}
pushFollow(FOLLOW_recordVariantTag_in_recordVariantSection9031);
recordVariantTag363=recordVariantTag();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordVariantTag363.getTree());
OF364=(Token)match(input,OF,FOLLOW_OF_in_recordVariantSection9033); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF364_tree = (Object)adaptor.create(OF364);
adaptor.addChild(root_0, OF364_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:661:88: ( recordVariant )+
int cnt118=0;
loop118:
while (true) {
int alt118=2;
int LA118_0 = input.LA(1);
if ( ((LA118_0 >= ABSOLUTE && LA118_0 <= ALIGN)||(LA118_0 >= ASSEMBLER && LA118_0 <= ASSEMBLY)||(LA118_0 >= AT && LA118_0 <= AUTOMATED)||LA118_0==CDECL||LA118_0==CONTAINS||(LA118_0 >= DEFAULT && LA118_0 <= DEREFERENCE)||LA118_0==DISPID||LA118_0==DYNAMIC||(LA118_0 >= EXPERIMENTAL && LA118_0 <= EXPORT)||LA118_0==EXTERNAL||(LA118_0 >= FAR && LA118_0 <= FINAL)||(LA118_0 >= FORWARD && LA118_0 <= FUNCTION)||LA118_0==HELPER||LA118_0==IMPLEMENTS||(LA118_0 >= INDEX && LA118_0 <= INHERITED)||LA118_0==LABEL||LA118_0==LOCAL||(LA118_0 >= MESSAGE && LA118_0 <= MINUS)||(LA118_0 >= NAME && LA118_0 <= NOT)||(LA118_0 >= ON && LA118_0 <= OPERATOR)||(LA118_0 >= OUT && LA118_0 <= OVERRIDE)||LA118_0==PACKAGE||LA118_0==PAREN_BRACKET_LEFT||LA118_0==PAREN_LEFT||(LA118_0 >= PASCAL && LA118_0 <= PROCEDURE)||(LA118_0 >= PROTECTED && LA118_0 <= PUBLISHED)||(LA118_0 >= READ && LA118_0 <= READONLY)||(LA118_0 >= REFERENCE && LA118_0 <= REINTRODUCE)||(LA118_0 >= REQUIRES && LA118_0 <= RESIDENT)||(LA118_0 >= SAFECALL && LA118_0 <= SEALED)||LA118_0==SQUARE_BRACKET_LEFT||(LA118_0 >= STATIC && LA118_0 <= STRING)||LA118_0==TkBinaryNumber||LA118_0==TkCharacterEscapeCode||(LA118_0 >= TkHexNumber && LA118_0 <= TkIntNumber)||LA118_0==TkMultilineString||(LA118_0 >= TkQuotedString && LA118_0 <= TkRealNumber)||LA118_0==UNSAFE||(LA118_0 >= VARARGS && LA118_0 <= VIRTUAL)||LA118_0==WINAPI||(LA118_0 >= WRITE && LA118_0 <= WRITEONLY)) ) {
alt118=1;
}
switch (alt118) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:661:88: recordVariant
{
pushFollow(FOLLOW_recordVariant_in_recordVariantSection9035);
recordVariant365=recordVariant();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordVariant365.getTree());
}
break;
default :
if ( cnt118 >= 1 ) break loop118;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(118, input);
throw eee;
}
cnt118++;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 92, recordVariantSection_StartIndex); }
}
return retval;
}
// $ANTLR end "recordVariantSection"
public static class recordVariantTag_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordVariantTag"
// au/com/integradev/delphi/antlr/Delphi.g:663:1: recordVariantTag : ( nameDeclaration ':' )? typeReference -> ^( TkRecordVariantTag ( nameDeclaration )? typeReference ) ;
public final DelphiParser.recordVariantTag_return recordVariantTag() throws RecognitionException {
DelphiParser.recordVariantTag_return retval = new DelphiParser.recordVariantTag_return();
retval.start = input.LT(1);
int recordVariantTag_StartIndex = input.index();
Object root_0 = null;
Token char_literal367=null;
ParserRuleReturnScope nameDeclaration366 =null;
ParserRuleReturnScope typeReference368 =null;
Object char_literal367_tree=null;
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_typeReference=new RewriteRuleSubtreeStream(adaptor,"rule typeReference");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 93) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:663:30: ( ( nameDeclaration ':' )? typeReference -> ^( TkRecordVariantTag ( nameDeclaration )? typeReference ) )
// au/com/integradev/delphi/antlr/Delphi.g:663:32: ( nameDeclaration ':' )? typeReference
{
// au/com/integradev/delphi/antlr/Delphi.g:663:32: ( nameDeclaration ':' )?
int alt119=2;
int LA119_0 = input.LA(1);
if ( (LA119_0==TkIdentifier) ) {
int LA119_1 = input.LA(2);
if ( (LA119_1==COLON) ) {
alt119=1;
}
}
else if ( ((LA119_0 >= ABSOLUTE && LA119_0 <= ABSTRACT)||LA119_0==ALIGN||(LA119_0 >= ASSEMBLER && LA119_0 <= ASSEMBLY)||(LA119_0 >= AT && LA119_0 <= AUTOMATED)||LA119_0==CDECL||LA119_0==CONTAINS||(LA119_0 >= DEFAULT && LA119_0 <= DEPRECATED)||LA119_0==DISPID||LA119_0==DYNAMIC||(LA119_0 >= EXPERIMENTAL && LA119_0 <= EXPORT)||LA119_0==EXTERNAL||LA119_0==FAR||LA119_0==FINAL||LA119_0==FORWARD||LA119_0==HELPER||LA119_0==IMPLEMENTS||LA119_0==INDEX||LA119_0==LABEL||LA119_0==LOCAL||LA119_0==MESSAGE||(LA119_0 >= NAME && LA119_0 <= NEAR)||LA119_0==NODEFAULT||(LA119_0 >= ON && LA119_0 <= OPERATOR)||(LA119_0 >= OUT && LA119_0 <= OVERRIDE)||LA119_0==PACKAGE||(LA119_0 >= PASCAL && LA119_0 <= PLATFORM)||LA119_0==PRIVATE||(LA119_0 >= PROTECTED && LA119_0 <= PUBLISHED)||(LA119_0 >= READ && LA119_0 <= READONLY)||(LA119_0 >= REFERENCE && LA119_0 <= REINTRODUCE)||(LA119_0 >= REQUIRES && LA119_0 <= RESIDENT)||(LA119_0 >= SAFECALL && LA119_0 <= SEALED)||(LA119_0 >= STATIC && LA119_0 <= STRICT)||LA119_0==UNSAFE||(LA119_0 >= VARARGS && LA119_0 <= VIRTUAL)||LA119_0==WINAPI||(LA119_0 >= WRITE && LA119_0 <= WRITEONLY)) ) {
int LA119_2 = input.LA(2);
if ( (LA119_2==COLON) ) {
alt119=1;
}
}
switch (alt119) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:663:33: nameDeclaration ':'
{
pushFollow(FOLLOW_nameDeclaration_in_recordVariantTag9086);
nameDeclaration366=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration366.getTree());
char_literal367=(Token)match(input,COLON,FOLLOW_COLON_in_recordVariantTag9088); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal367);
}
break;
}
pushFollow(FOLLOW_typeReference_in_recordVariantTag9092);
typeReference368=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReference.add(typeReference368.getTree());
// AST REWRITE
// elements: typeReference, nameDeclaration
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 664:30: -> ^( TkRecordVariantTag ( nameDeclaration )? typeReference )
{
// au/com/integradev/delphi/antlr/Delphi.g:664:33: ^( TkRecordVariantTag ( nameDeclaration )? typeReference )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RecordVariantTagNodeImpl(TkRecordVariantTag), root_1);
// au/com/integradev/delphi/antlr/Delphi.g:664:80: ( nameDeclaration )?
if ( stream_nameDeclaration.hasNext() ) {
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
}
stream_nameDeclaration.reset();
adaptor.addChild(root_1, stream_typeReference.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 93, recordVariantTag_StartIndex); }
}
return retval;
}
// $ANTLR end "recordVariantTag"
public static class recordVariant_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordVariant"
// au/com/integradev/delphi/antlr/Delphi.g:666:1: recordVariant : expressionList ':' '(' ( fieldDecl )* ( recordVariantSection )? ')' ( ';' )? -> ^( TkRecordVariantItem expressionList ( fieldDecl )* ( recordVariantSection )? ( ';' )? ) ;
public final DelphiParser.recordVariant_return recordVariant() throws RecognitionException {
DelphiParser.recordVariant_return retval = new DelphiParser.recordVariant_return();
retval.start = input.LT(1);
int recordVariant_StartIndex = input.index();
Object root_0 = null;
Token char_literal370=null;
Token char_literal371=null;
Token char_literal374=null;
Token char_literal375=null;
ParserRuleReturnScope expressionList369 =null;
ParserRuleReturnScope fieldDecl372 =null;
ParserRuleReturnScope recordVariantSection373 =null;
Object char_literal370_tree=null;
Object char_literal371_tree=null;
Object char_literal374_tree=null;
Object char_literal375_tree=null;
RewriteRuleTokenStream stream_PAREN_RIGHT=new RewriteRuleTokenStream(adaptor,"token PAREN_RIGHT");
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_PAREN_LEFT=new RewriteRuleTokenStream(adaptor,"token PAREN_LEFT");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_expressionList=new RewriteRuleSubtreeStream(adaptor,"rule expressionList");
RewriteRuleSubtreeStream stream_recordVariantSection=new RewriteRuleSubtreeStream(adaptor,"rule recordVariantSection");
RewriteRuleSubtreeStream stream_fieldDecl=new RewriteRuleSubtreeStream(adaptor,"rule fieldDecl");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 94) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:666:30: ( expressionList ':' '(' ( fieldDecl )* ( recordVariantSection )? ')' ( ';' )? -> ^( TkRecordVariantItem expressionList ( fieldDecl )* ( recordVariantSection )? ( ';' )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:666:32: expressionList ':' '(' ( fieldDecl )* ( recordVariantSection )? ')' ( ';' )?
{
pushFollow(FOLLOW_expressionList_in_recordVariant9187);
expressionList369=expressionList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expressionList.add(expressionList369.getTree());
char_literal370=(Token)match(input,COLON,FOLLOW_COLON_in_recordVariant9189); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal370);
char_literal371=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_recordVariant9191); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_LEFT.add(char_literal371);
// au/com/integradev/delphi/antlr/Delphi.g:666:55: ( fieldDecl )*
loop120:
while (true) {
int alt120=2;
int LA120_0 = input.LA(1);
if ( ((LA120_0 >= ABSOLUTE && LA120_0 <= ABSTRACT)||LA120_0==ALIGN||(LA120_0 >= ASSEMBLER && LA120_0 <= ASSEMBLY)||(LA120_0 >= AT && LA120_0 <= AUTOMATED)||LA120_0==CDECL||LA120_0==CONTAINS||(LA120_0 >= DEFAULT && LA120_0 <= DEPRECATED)||LA120_0==DISPID||LA120_0==DYNAMIC||(LA120_0 >= EXPERIMENTAL && LA120_0 <= EXPORT)||LA120_0==EXTERNAL||LA120_0==FAR||LA120_0==FINAL||LA120_0==FORWARD||LA120_0==HELPER||LA120_0==IMPLEMENTS||LA120_0==INDEX||LA120_0==LABEL||LA120_0==LOCAL||LA120_0==MESSAGE||(LA120_0 >= NAME && LA120_0 <= NEAR)||LA120_0==NODEFAULT||(LA120_0 >= ON && LA120_0 <= OPERATOR)||(LA120_0 >= OUT && LA120_0 <= OVERRIDE)||LA120_0==PACKAGE||LA120_0==PAREN_BRACKET_LEFT||(LA120_0 >= PASCAL && LA120_0 <= PLATFORM)||LA120_0==PRIVATE||(LA120_0 >= PROTECTED && LA120_0 <= PUBLISHED)||(LA120_0 >= READ && LA120_0 <= READONLY)||(LA120_0 >= REFERENCE && LA120_0 <= REINTRODUCE)||(LA120_0 >= REQUIRES && LA120_0 <= RESIDENT)||(LA120_0 >= SAFECALL && LA120_0 <= SEALED)||LA120_0==SQUARE_BRACKET_LEFT||(LA120_0 >= STATIC && LA120_0 <= STRICT)||LA120_0==TkIdentifier||LA120_0==UNSAFE||(LA120_0 >= VARARGS && LA120_0 <= VIRTUAL)||LA120_0==WINAPI||(LA120_0 >= WRITE && LA120_0 <= WRITEONLY)) ) {
alt120=1;
}
switch (alt120) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:666:55: fieldDecl
{
pushFollow(FOLLOW_fieldDecl_in_recordVariant9193);
fieldDecl372=fieldDecl();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_fieldDecl.add(fieldDecl372.getTree());
}
break;
default :
break loop120;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:666:66: ( recordVariantSection )?
int alt121=2;
int LA121_0 = input.LA(1);
if ( (LA121_0==CASE) ) {
alt121=1;
}
switch (alt121) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:666:66: recordVariantSection
{
pushFollow(FOLLOW_recordVariantSection_in_recordVariant9196);
recordVariantSection373=recordVariantSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_recordVariantSection.add(recordVariantSection373.getTree());
}
break;
}
char_literal374=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_recordVariant9199); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_RIGHT.add(char_literal374);
// au/com/integradev/delphi/antlr/Delphi.g:666:92: ( ';' )?
int alt122=2;
int LA122_0 = input.LA(1);
if ( (LA122_0==SEMICOLON) ) {
alt122=1;
}
switch (alt122) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:666:92: ';'
{
char_literal375=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_recordVariant9201); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal375);
}
break;
}
// AST REWRITE
// elements: recordVariantSection, SEMICOLON, fieldDecl, expressionList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 667:30: -> ^( TkRecordVariantItem expressionList ( fieldDecl )* ( recordVariantSection )? ( ';' )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:667:33: ^( TkRecordVariantItem expressionList ( fieldDecl )* ( recordVariantSection )? ( ';' )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RecordVariantItemNodeImpl(TkRecordVariantItem), root_1);
adaptor.addChild(root_1, stream_expressionList.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:667:97: ( fieldDecl )*
while ( stream_fieldDecl.hasNext() ) {
adaptor.addChild(root_1, stream_fieldDecl.nextTree());
}
stream_fieldDecl.reset();
// au/com/integradev/delphi/antlr/Delphi.g:667:108: ( recordVariantSection )?
if ( stream_recordVariantSection.hasNext() ) {
adaptor.addChild(root_1, stream_recordVariantSection.nextTree());
}
stream_recordVariantSection.reset();
// au/com/integradev/delphi/antlr/Delphi.g:667:130: ( ';' )?
if ( stream_SEMICOLON.hasNext() ) {
adaptor.addChild(root_1, stream_SEMICOLON.nextNode());
}
stream_SEMICOLON.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 94, recordVariant_StartIndex); }
}
return retval;
}
// $ANTLR end "recordVariant"
public static class recordHelperType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordHelperType"
// au/com/integradev/delphi/antlr/Delphi.g:669:1: recordHelperType : RECORD ^ HELPER FOR typeReferenceOrStringOrFile ( visibilitySection )* END ;
public final DelphiParser.recordHelperType_return recordHelperType() throws RecognitionException {
DelphiParser.recordHelperType_return retval = new DelphiParser.recordHelperType_return();
retval.start = input.LT(1);
int recordHelperType_StartIndex = input.index();
Object root_0 = null;
Token RECORD376=null;
Token HELPER377=null;
Token FOR378=null;
Token END381=null;
ParserRuleReturnScope typeReferenceOrStringOrFile379 =null;
ParserRuleReturnScope visibilitySection380 =null;
Object RECORD376_tree=null;
Object HELPER377_tree=null;
Object FOR378_tree=null;
Object END381_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 95) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:669:30: ( RECORD ^ HELPER FOR typeReferenceOrStringOrFile ( visibilitySection )* END )
// au/com/integradev/delphi/antlr/Delphi.g:669:32: RECORD ^ HELPER FOR typeReferenceOrStringOrFile ( visibilitySection )* END
{
root_0 = (Object)adaptor.nil();
RECORD376=(Token)match(input,RECORD,FOLLOW_RECORD_in_recordHelperType9300); if (state.failed) return retval;
if ( state.backtracking==0 ) {
RECORD376_tree = new RecordHelperTypeNodeImpl(RECORD376) ;
root_0 = (Object)adaptor.becomeRoot(RECORD376_tree, root_0);
}
HELPER377=(Token)match(input,HELPER,FOLLOW_HELPER_in_recordHelperType9306); if (state.failed) return retval;
if ( state.backtracking==0 ) {
HELPER377_tree = (Object)adaptor.create(HELPER377);
adaptor.addChild(root_0, HELPER377_tree);
}
FOR378=(Token)match(input,FOR,FOLLOW_FOR_in_recordHelperType9308); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FOR378_tree = (Object)adaptor.create(FOR378);
adaptor.addChild(root_0, FOR378_tree);
}
pushFollow(FOLLOW_typeReferenceOrStringOrFile_in_recordHelperType9310);
typeReferenceOrStringOrFile379=typeReferenceOrStringOrFile();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReferenceOrStringOrFile379.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:669:105: ( visibilitySection )*
loop123:
while (true) {
int alt123=2;
int LA123_0 = input.LA(1);
if ( ((LA123_0 >= ABSOLUTE && LA123_0 <= ABSTRACT)||LA123_0==ALIGN||(LA123_0 >= ASSEMBLER && LA123_0 <= ASSEMBLY)||(LA123_0 >= AT && LA123_0 <= AUTOMATED)||(LA123_0 >= CDECL && LA123_0 <= CLASS)||(LA123_0 >= CONST && LA123_0 <= CONTAINS)||(LA123_0 >= DEFAULT && LA123_0 <= DEPRECATED)||(LA123_0 >= DESTRUCTOR && LA123_0 <= DISPID)||LA123_0==DYNAMIC||(LA123_0 >= EXPERIMENTAL && LA123_0 <= EXPORT)||LA123_0==EXTERNAL||LA123_0==FAR||LA123_0==FINAL||(LA123_0 >= FORWARD && LA123_0 <= FUNCTION)||LA123_0==HELPER||LA123_0==IMPLEMENTS||LA123_0==INDEX||LA123_0==LABEL||LA123_0==LOCAL||LA123_0==MESSAGE||(LA123_0 >= NAME && LA123_0 <= NEAR)||LA123_0==NODEFAULT||(LA123_0 >= ON && LA123_0 <= OPERATOR)||(LA123_0 >= OUT && LA123_0 <= OVERRIDE)||LA123_0==PACKAGE||LA123_0==PAREN_BRACKET_LEFT||(LA123_0 >= PASCAL && LA123_0 <= PLATFORM)||(LA123_0 >= PRIVATE && LA123_0 <= PROCEDURE)||(LA123_0 >= PROPERTY && LA123_0 <= PUBLISHED)||(LA123_0 >= READ && LA123_0 <= READONLY)||(LA123_0 >= REFERENCE && LA123_0 <= REINTRODUCE)||(LA123_0 >= REQUIRES && LA123_0 <= RESOURCESTRING)||(LA123_0 >= SAFECALL && LA123_0 <= SEALED)||LA123_0==SQUARE_BRACKET_LEFT||(LA123_0 >= STATIC && LA123_0 <= STRICT)||LA123_0==THREADVAR||LA123_0==TYPE||LA123_0==TkIdentifier||LA123_0==UNSAFE||(LA123_0 >= VAR && LA123_0 <= VIRTUAL)||LA123_0==WINAPI||(LA123_0 >= WRITE && LA123_0 <= WRITEONLY)) ) {
alt123=1;
}
switch (alt123) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:669:105: visibilitySection
{
pushFollow(FOLLOW_visibilitySection_in_recordHelperType9312);
visibilitySection380=visibilitySection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, visibilitySection380.getTree());
}
break;
default :
break loop123;
}
}
END381=(Token)match(input,END,FOLLOW_END_in_recordHelperType9315); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END381_tree = (Object)adaptor.create(END381);
adaptor.addChild(root_0, END381_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 95, recordHelperType_StartIndex); }
}
return retval;
}
// $ANTLR end "recordHelperType"
public static class property_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "property"
// au/com/integradev/delphi/antlr/Delphi.g:671:1: property : ( attributeList )? ( CLASS )? PROPERTY nameDeclaration ( propertyArray )? ( ':' varType )? ( propertyDirective )* ';' -> ^( PROPERTY nameDeclaration ( propertyArray )? ( varType )? ( CLASS )? ( attributeList )? ( propertyDirective )* ) ;
public final DelphiParser.property_return property() throws RecognitionException {
DelphiParser.property_return retval = new DelphiParser.property_return();
retval.start = input.LT(1);
int property_StartIndex = input.index();
Object root_0 = null;
Token CLASS383=null;
Token PROPERTY384=null;
Token char_literal387=null;
Token char_literal390=null;
ParserRuleReturnScope attributeList382 =null;
ParserRuleReturnScope nameDeclaration385 =null;
ParserRuleReturnScope propertyArray386 =null;
ParserRuleReturnScope varType388 =null;
ParserRuleReturnScope propertyDirective389 =null;
Object CLASS383_tree=null;
Object PROPERTY384_tree=null;
Object char_literal387_tree=null;
Object char_literal390_tree=null;
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_PROPERTY=new RewriteRuleTokenStream(adaptor,"token PROPERTY");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_propertyArray=new RewriteRuleSubtreeStream(adaptor,"rule propertyArray");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_propertyDirective=new RewriteRuleSubtreeStream(adaptor,"rule propertyDirective");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 96) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:671:30: ( ( attributeList )? ( CLASS )? PROPERTY nameDeclaration ( propertyArray )? ( ':' varType )? ( propertyDirective )* ';' -> ^( PROPERTY nameDeclaration ( propertyArray )? ( varType )? ( CLASS )? ( attributeList )? ( propertyDirective )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:671:32: ( attributeList )? ( CLASS )? PROPERTY nameDeclaration ( propertyArray )? ( ':' varType )? ( propertyDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:671:32: ( attributeList )?
int alt124=2;
int LA124_0 = input.LA(1);
if ( (LA124_0==PAREN_BRACKET_LEFT||LA124_0==SQUARE_BRACKET_LEFT) ) {
alt124=1;
}
switch (alt124) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:671:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_property9372);
attributeList382=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList382.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:671:47: ( CLASS )?
int alt125=2;
int LA125_0 = input.LA(1);
if ( (LA125_0==CLASS) ) {
alt125=1;
}
switch (alt125) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:671:47: CLASS
{
CLASS383=(Token)match(input,CLASS,FOLLOW_CLASS_in_property9375); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS383);
}
break;
}
PROPERTY384=(Token)match(input,PROPERTY,FOLLOW_PROPERTY_in_property9378); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PROPERTY.add(PROPERTY384);
pushFollow(FOLLOW_nameDeclaration_in_property9380);
nameDeclaration385=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration385.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:671:79: ( propertyArray )?
int alt126=2;
int LA126_0 = input.LA(1);
if ( (LA126_0==PAREN_BRACKET_LEFT||LA126_0==SQUARE_BRACKET_LEFT) ) {
alt126=1;
}
switch (alt126) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:671:79: propertyArray
{
pushFollow(FOLLOW_propertyArray_in_property9382);
propertyArray386=propertyArray();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_propertyArray.add(propertyArray386.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:671:94: ( ':' varType )?
int alt127=2;
int LA127_0 = input.LA(1);
if ( (LA127_0==COLON) ) {
alt127=1;
}
switch (alt127) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:671:95: ':' varType
{
char_literal387=(Token)match(input,COLON,FOLLOW_COLON_in_property9386); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal387);
pushFollow(FOLLOW_varType_in_property9388);
varType388=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType388.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:671:109: ( propertyDirective )*
loop128:
while (true) {
int alt128=2;
int LA128_0 = input.LA(1);
if ( (LA128_0==SEMICOLON) ) {
int LA128_1 = input.LA(2);
if ( (LA128_1==DEFAULT) ) {
int LA128_4 = input.LA(3);
if ( (LA128_4==DEFAULT||LA128_4==DISPID||LA128_4==IMPLEMENTS||LA128_4==INDEX||LA128_4==NODEFAULT||(LA128_4 >= READ && LA128_4 <= READONLY)||LA128_4==SEMICOLON||LA128_4==STORED||(LA128_4 >= WRITE && LA128_4 <= WRITEONLY)) ) {
alt128=1;
}
}
}
else if ( (LA128_0==DEFAULT||LA128_0==DISPID||LA128_0==IMPLEMENTS||LA128_0==INDEX||LA128_0==NODEFAULT||(LA128_0 >= READ && LA128_0 <= READONLY)||LA128_0==STORED||(LA128_0 >= WRITE && LA128_0 <= WRITEONLY)) ) {
alt128=1;
}
switch (alt128) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:671:110: propertyDirective
{
pushFollow(FOLLOW_propertyDirective_in_property9393);
propertyDirective389=propertyDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_propertyDirective.add(propertyDirective389.getTree());
}
break;
default :
break loop128;
}
}
char_literal390=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_property9397); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal390);
// AST REWRITE
// elements: nameDeclaration, propertyArray, attributeList, PROPERTY, varType, CLASS, propertyDirective
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 672:30: -> ^( PROPERTY nameDeclaration ( propertyArray )? ( varType )? ( CLASS )? ( attributeList )? ( propertyDirective )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:672:33: ^( PROPERTY nameDeclaration ( propertyArray )? ( varType )? ( CLASS )? ( attributeList )? ( propertyDirective )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new PropertyNodeImpl(stream_PROPERTY.nextToken()), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:672:78: ( propertyArray )?
if ( stream_propertyArray.hasNext() ) {
adaptor.addChild(root_1, stream_propertyArray.nextTree());
}
stream_propertyArray.reset();
// au/com/integradev/delphi/antlr/Delphi.g:672:93: ( varType )?
if ( stream_varType.hasNext() ) {
adaptor.addChild(root_1, stream_varType.nextTree());
}
stream_varType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:672:102: ( CLASS )?
if ( stream_CLASS.hasNext() ) {
adaptor.addChild(root_1, stream_CLASS.nextNode());
}
stream_CLASS.reset();
// au/com/integradev/delphi/antlr/Delphi.g:672:109: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:672:124: ( propertyDirective )*
while ( stream_propertyDirective.hasNext() ) {
adaptor.addChild(root_1, stream_propertyDirective.nextTree());
}
stream_propertyDirective.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 96, property_StartIndex); }
}
return retval;
}
// $ANTLR end "property"
public static class propertyArray_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyArray"
// au/com/integradev/delphi/antlr/Delphi.g:674:1: propertyArray : lbrack ! formalParameterList rbrack !;
public final DelphiParser.propertyArray_return propertyArray() throws RecognitionException {
DelphiParser.propertyArray_return retval = new DelphiParser.propertyArray_return();
retval.start = input.LT(1);
int propertyArray_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope lbrack391 =null;
ParserRuleReturnScope formalParameterList392 =null;
ParserRuleReturnScope rbrack393 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 97) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:674:30: ( lbrack ! formalParameterList rbrack !)
// au/com/integradev/delphi/antlr/Delphi.g:674:32: lbrack ! formalParameterList rbrack !
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_lbrack_in_propertyArray9504);
lbrack391=lbrack();
state._fsp--;
if (state.failed) return retval;
pushFollow(FOLLOW_formalParameterList_in_propertyArray9507);
formalParameterList392=formalParameterList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, formalParameterList392.getTree());
pushFollow(FOLLOW_rbrack_in_propertyArray9509);
rbrack393=rbrack();
state._fsp--;
if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 97, propertyArray_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyArray"
public static class propertyDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyDirective"
// au/com/integradev/delphi/antlr/Delphi.g:676:1: propertyDirective : ( ';' propertyDefaultNoExpression | propertyDefault | propertyReadWrite | propertyDispInterface | propertyImplements | propertyIndex | propertyStored | NODEFAULT );
public final DelphiParser.propertyDirective_return propertyDirective() throws RecognitionException {
DelphiParser.propertyDirective_return retval = new DelphiParser.propertyDirective_return();
retval.start = input.LT(1);
int propertyDirective_StartIndex = input.index();
Object root_0 = null;
Token char_literal394=null;
Token NODEFAULT402=null;
ParserRuleReturnScope propertyDefaultNoExpression395 =null;
ParserRuleReturnScope propertyDefault396 =null;
ParserRuleReturnScope propertyReadWrite397 =null;
ParserRuleReturnScope propertyDispInterface398 =null;
ParserRuleReturnScope propertyImplements399 =null;
ParserRuleReturnScope propertyIndex400 =null;
ParserRuleReturnScope propertyStored401 =null;
Object char_literal394_tree=null;
Object NODEFAULT402_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 98) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:676:30: ( ';' propertyDefaultNoExpression | propertyDefault | propertyReadWrite | propertyDispInterface | propertyImplements | propertyIndex | propertyStored | NODEFAULT )
int alt129=8;
switch ( input.LA(1) ) {
case SEMICOLON:
{
alt129=1;
}
break;
case DEFAULT:
{
alt129=2;
}
break;
case READ:
case WRITE:
{
alt129=3;
}
break;
case DISPID:
case READONLY:
case WRITEONLY:
{
alt129=4;
}
break;
case IMPLEMENTS:
{
alt129=5;
}
break;
case INDEX:
{
alt129=6;
}
break;
case STORED:
{
alt129=7;
}
break;
case NODEFAULT:
{
alt129=8;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 129, 0, input);
throw nvae;
}
switch (alt129) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:676:32: ';' propertyDefaultNoExpression
{
root_0 = (Object)adaptor.nil();
char_literal394=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_propertyDirective9558); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal394_tree = (Object)adaptor.create(char_literal394);
adaptor.addChild(root_0, char_literal394_tree);
}
pushFollow(FOLLOW_propertyDefaultNoExpression_in_propertyDirective9560);
propertyDefaultNoExpression395=propertyDefaultNoExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyDefaultNoExpression395.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:677:32: propertyDefault
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_propertyDefault_in_propertyDirective9593);
propertyDefault396=propertyDefault();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyDefault396.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:678:32: propertyReadWrite
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_propertyReadWrite_in_propertyDirective9626);
propertyReadWrite397=propertyReadWrite();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyReadWrite397.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:679:32: propertyDispInterface
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_propertyDispInterface_in_propertyDirective9659);
propertyDispInterface398=propertyDispInterface();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyDispInterface398.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:680:32: propertyImplements
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_propertyImplements_in_propertyDirective9692);
propertyImplements399=propertyImplements();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyImplements399.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:681:32: propertyIndex
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_propertyIndex_in_propertyDirective9725);
propertyIndex400=propertyIndex();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyIndex400.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:682:32: propertyStored
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_propertyStored_in_propertyDirective9758);
propertyStored401=propertyStored();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, propertyStored401.getTree());
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:683:32: NODEFAULT
{
root_0 = (Object)adaptor.nil();
NODEFAULT402=(Token)match(input,NODEFAULT,FOLLOW_NODEFAULT_in_propertyDirective9791); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NODEFAULT402_tree = (Object)adaptor.create(NODEFAULT402);
adaptor.addChild(root_0, NODEFAULT402_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 98, propertyDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyDirective"
public static class propertyDefaultNoExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyDefaultNoExpression"
// au/com/integradev/delphi/antlr/Delphi.g:685:1: propertyDefaultNoExpression : DEFAULT ^;
public final DelphiParser.propertyDefaultNoExpression_return propertyDefaultNoExpression() throws RecognitionException {
DelphiParser.propertyDefaultNoExpression_return retval = new DelphiParser.propertyDefaultNoExpression_return();
retval.start = input.LT(1);
int propertyDefaultNoExpression_StartIndex = input.index();
Object root_0 = null;
Token DEFAULT403=null;
Object DEFAULT403_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 99) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:685:30: ( DEFAULT ^)
// au/com/integradev/delphi/antlr/Delphi.g:685:32: DEFAULT ^
{
root_0 = (Object)adaptor.nil();
DEFAULT403=(Token)match(input,DEFAULT,FOLLOW_DEFAULT_in_propertyDefaultNoExpression9829); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DEFAULT403_tree = new PropertyDefaultSpecifierNodeImpl(DEFAULT403) ;
root_0 = (Object)adaptor.becomeRoot(DEFAULT403_tree, root_0);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 99, propertyDefaultNoExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyDefaultNoExpression"
public static class propertyDefault_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyDefault"
// au/com/integradev/delphi/antlr/Delphi.g:687:1: propertyDefault : DEFAULT ^ expression ;
public final DelphiParser.propertyDefault_return propertyDefault() throws RecognitionException {
DelphiParser.propertyDefault_return retval = new DelphiParser.propertyDefault_return();
retval.start = input.LT(1);
int propertyDefault_StartIndex = input.index();
Object root_0 = null;
Token DEFAULT404=null;
ParserRuleReturnScope expression405 =null;
Object DEFAULT404_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 100) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:687:30: ( DEFAULT ^ expression )
// au/com/integradev/delphi/antlr/Delphi.g:687:32: DEFAULT ^ expression
{
root_0 = (Object)adaptor.nil();
DEFAULT404=(Token)match(input,DEFAULT,FOLLOW_DEFAULT_in_propertyDefault9883); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DEFAULT404_tree = new PropertyDefaultSpecifierNodeImpl(DEFAULT404) ;
root_0 = (Object)adaptor.becomeRoot(DEFAULT404_tree, root_0);
}
pushFollow(FOLLOW_expression_in_propertyDefault9889);
expression405=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression405.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 100, propertyDefault_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyDefault"
public static class propertyReadWrite_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyReadWrite"
// au/com/integradev/delphi/antlr/Delphi.g:689:1: propertyReadWrite : ( READ ^| WRITE ^) primaryExpression ;
public final DelphiParser.propertyReadWrite_return propertyReadWrite() throws RecognitionException {
DelphiParser.propertyReadWrite_return retval = new DelphiParser.propertyReadWrite_return();
retval.start = input.LT(1);
int propertyReadWrite_StartIndex = input.index();
Object root_0 = null;
Token READ406=null;
Token WRITE407=null;
ParserRuleReturnScope primaryExpression408 =null;
Object READ406_tree=null;
Object WRITE407_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 101) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:689:30: ( ( READ ^| WRITE ^) primaryExpression )
// au/com/integradev/delphi/antlr/Delphi.g:689:32: ( READ ^| WRITE ^) primaryExpression
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:689:32: ( READ ^| WRITE ^)
int alt130=2;
int LA130_0 = input.LA(1);
if ( (LA130_0==READ) ) {
alt130=1;
}
else if ( (LA130_0==WRITE) ) {
alt130=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 130, 0, input);
throw nvae;
}
switch (alt130) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:689:33: READ ^
{
READ406=(Token)match(input,READ,FOLLOW_READ_in_propertyReadWrite9938); if (state.failed) return retval;
if ( state.backtracking==0 ) {
READ406_tree = new PropertyReadSpecifierNodeImpl(READ406) ;
root_0 = (Object)adaptor.becomeRoot(READ406_tree, root_0);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:689:72: WRITE ^
{
WRITE407=(Token)match(input,WRITE,FOLLOW_WRITE_in_propertyReadWrite9946); if (state.failed) return retval;
if ( state.backtracking==0 ) {
WRITE407_tree = new PropertyWriteSpecifierNodeImpl(WRITE407) ;
root_0 = (Object)adaptor.becomeRoot(WRITE407_tree, root_0);
}
}
break;
}
pushFollow(FOLLOW_primaryExpression_in_propertyReadWrite9953);
primaryExpression408=primaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, primaryExpression408.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 101, propertyReadWrite_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyReadWrite"
public static class propertyImplements_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyImplements"
// au/com/integradev/delphi/antlr/Delphi.g:691:1: propertyImplements : IMPLEMENTS ^ typeReference ( ',' typeReference )* ;
public final DelphiParser.propertyImplements_return propertyImplements() throws RecognitionException {
DelphiParser.propertyImplements_return retval = new DelphiParser.propertyImplements_return();
retval.start = input.LT(1);
int propertyImplements_StartIndex = input.index();
Object root_0 = null;
Token IMPLEMENTS409=null;
Token char_literal411=null;
ParserRuleReturnScope typeReference410 =null;
ParserRuleReturnScope typeReference412 =null;
Object IMPLEMENTS409_tree=null;
Object char_literal411_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 102) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:691:30: ( IMPLEMENTS ^ typeReference ( ',' typeReference )* )
// au/com/integradev/delphi/antlr/Delphi.g:691:32: IMPLEMENTS ^ typeReference ( ',' typeReference )*
{
root_0 = (Object)adaptor.nil();
IMPLEMENTS409=(Token)match(input,IMPLEMENTS,FOLLOW_IMPLEMENTS_in_propertyImplements10000); if (state.failed) return retval;
if ( state.backtracking==0 ) {
IMPLEMENTS409_tree = new PropertyImplementsSpecifierNodeImpl(IMPLEMENTS409) ;
root_0 = (Object)adaptor.becomeRoot(IMPLEMENTS409_tree, root_0);
}
pushFollow(FOLLOW_typeReference_in_propertyImplements10006);
typeReference410=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference410.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:691:95: ( ',' typeReference )*
loop131:
while (true) {
int alt131=2;
int LA131_0 = input.LA(1);
if ( (LA131_0==COMMA) ) {
alt131=1;
}
switch (alt131) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:691:96: ',' typeReference
{
char_literal411=(Token)match(input,COMMA,FOLLOW_COMMA_in_propertyImplements10009); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal411_tree = (Object)adaptor.create(char_literal411);
adaptor.addChild(root_0, char_literal411_tree);
}
pushFollow(FOLLOW_typeReference_in_propertyImplements10011);
typeReference412=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference412.getTree());
}
break;
default :
break loop131;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 102, propertyImplements_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyImplements"
public static class propertyIndex_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyIndex"
// au/com/integradev/delphi/antlr/Delphi.g:693:1: propertyIndex : INDEX ^ expression ;
public final DelphiParser.propertyIndex_return propertyIndex() throws RecognitionException {
DelphiParser.propertyIndex_return retval = new DelphiParser.propertyIndex_return();
retval.start = input.LT(1);
int propertyIndex_StartIndex = input.index();
Object root_0 = null;
Token INDEX413=null;
ParserRuleReturnScope expression414 =null;
Object INDEX413_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 103) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:693:30: ( INDEX ^ expression )
// au/com/integradev/delphi/antlr/Delphi.g:693:32: INDEX ^ expression
{
root_0 = (Object)adaptor.nil();
INDEX413=(Token)match(input,INDEX,FOLLOW_INDEX_in_propertyIndex10065); if (state.failed) return retval;
if ( state.backtracking==0 ) {
INDEX413_tree = new PropertyIndexSpecifierNodeImpl(INDEX413) ;
root_0 = (Object)adaptor.becomeRoot(INDEX413_tree, root_0);
}
pushFollow(FOLLOW_expression_in_propertyIndex10071);
expression414=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression414.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 103, propertyIndex_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyIndex"
public static class propertyStored_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyStored"
// au/com/integradev/delphi/antlr/Delphi.g:695:1: propertyStored : STORED ^ expression ;
public final DelphiParser.propertyStored_return propertyStored() throws RecognitionException {
DelphiParser.propertyStored_return retval = new DelphiParser.propertyStored_return();
retval.start = input.LT(1);
int propertyStored_StartIndex = input.index();
Object root_0 = null;
Token STORED415=null;
ParserRuleReturnScope expression416 =null;
Object STORED415_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 104) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:695:30: ( STORED ^ expression )
// au/com/integradev/delphi/antlr/Delphi.g:695:32: STORED ^ expression
{
root_0 = (Object)adaptor.nil();
STORED415=(Token)match(input,STORED,FOLLOW_STORED_in_propertyStored10122); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STORED415_tree = new PropertyStoredSpecifierNodeImpl(STORED415) ;
root_0 = (Object)adaptor.becomeRoot(STORED415_tree, root_0);
}
pushFollow(FOLLOW_expression_in_propertyStored10128);
expression416=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression416.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 104, propertyStored_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyStored"
public static class propertyDispInterface_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "propertyDispInterface"
// au/com/integradev/delphi/antlr/Delphi.g:697:1: propertyDispInterface : ( READONLY | WRITEONLY | dispIDDirective );
public final DelphiParser.propertyDispInterface_return propertyDispInterface() throws RecognitionException {
DelphiParser.propertyDispInterface_return retval = new DelphiParser.propertyDispInterface_return();
retval.start = input.LT(1);
int propertyDispInterface_StartIndex = input.index();
Object root_0 = null;
Token READONLY417=null;
Token WRITEONLY418=null;
ParserRuleReturnScope dispIDDirective419 =null;
Object READONLY417_tree=null;
Object WRITEONLY418_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 105) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:697:30: ( READONLY | WRITEONLY | dispIDDirective )
int alt132=3;
switch ( input.LA(1) ) {
case READONLY:
{
alt132=1;
}
break;
case WRITEONLY:
{
alt132=2;
}
break;
case DISPID:
{
alt132=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 132, 0, input);
throw nvae;
}
switch (alt132) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:697:32: READONLY
{
root_0 = (Object)adaptor.nil();
READONLY417=(Token)match(input,READONLY,FOLLOW_READONLY_in_propertyDispInterface10172); if (state.failed) return retval;
if ( state.backtracking==0 ) {
READONLY417_tree = (Object)adaptor.create(READONLY417);
adaptor.addChild(root_0, READONLY417_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:698:32: WRITEONLY
{
root_0 = (Object)adaptor.nil();
WRITEONLY418=(Token)match(input,WRITEONLY,FOLLOW_WRITEONLY_in_propertyDispInterface10205); if (state.failed) return retval;
if ( state.backtracking==0 ) {
WRITEONLY418_tree = (Object)adaptor.create(WRITEONLY418);
adaptor.addChild(root_0, WRITEONLY418_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:699:32: dispIDDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_dispIDDirective_in_propertyDispInterface10238);
dispIDDirective419=dispIDDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, dispIDDirective419.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 105, propertyDispInterface_StartIndex); }
}
return retval;
}
// $ANTLR end "propertyDispInterface"
public static class visibility_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "visibility"
// au/com/integradev/delphi/antlr/Delphi.g:701:1: visibility : ( ( STRICT )? PROTECTED ^| ( STRICT )? PRIVATE ^| PUBLIC | PUBLISHED | AUTOMATED );
public final DelphiParser.visibility_return visibility() throws RecognitionException {
DelphiParser.visibility_return retval = new DelphiParser.visibility_return();
retval.start = input.LT(1);
int visibility_StartIndex = input.index();
Object root_0 = null;
Token STRICT420=null;
Token PROTECTED421=null;
Token STRICT422=null;
Token PRIVATE423=null;
Token PUBLIC424=null;
Token PUBLISHED425=null;
Token AUTOMATED426=null;
Object STRICT420_tree=null;
Object PROTECTED421_tree=null;
Object STRICT422_tree=null;
Object PRIVATE423_tree=null;
Object PUBLIC424_tree=null;
Object PUBLISHED425_tree=null;
Object AUTOMATED426_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 106) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:701:30: ( ( STRICT )? PROTECTED ^| ( STRICT )? PRIVATE ^| PUBLIC | PUBLISHED | AUTOMATED )
int alt135=5;
switch ( input.LA(1) ) {
case STRICT:
{
int LA135_1 = input.LA(2);
if ( (LA135_1==PROTECTED) ) {
alt135=1;
}
else if ( (LA135_1==PRIVATE) ) {
alt135=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 135, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PROTECTED:
{
alt135=1;
}
break;
case PRIVATE:
{
alt135=2;
}
break;
case PUBLIC:
{
alt135=3;
}
break;
case PUBLISHED:
{
alt135=4;
}
break;
case AUTOMATED:
{
alt135=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 135, 0, input);
throw nvae;
}
switch (alt135) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:701:32: ( STRICT )? PROTECTED ^
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:701:32: ( STRICT )?
int alt133=2;
int LA133_0 = input.LA(1);
if ( (LA133_0==STRICT) ) {
alt133=1;
}
switch (alt133) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:701:32: STRICT
{
STRICT420=(Token)match(input,STRICT,FOLLOW_STRICT_in_visibility10293); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STRICT420_tree = (Object)adaptor.create(STRICT420);
adaptor.addChild(root_0, STRICT420_tree);
}
}
break;
}
PROTECTED421=(Token)match(input,PROTECTED,FOLLOW_PROTECTED_in_visibility10296); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PROTECTED421_tree = new VisibilityNodeImpl(PROTECTED421) ;
root_0 = (Object)adaptor.becomeRoot(PROTECTED421_tree, root_0);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:702:32: ( STRICT )? PRIVATE ^
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:702:32: ( STRICT )?
int alt134=2;
int LA134_0 = input.LA(1);
if ( (LA134_0==STRICT) ) {
alt134=1;
}
switch (alt134) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:702:32: STRICT
{
STRICT422=(Token)match(input,STRICT,FOLLOW_STRICT_in_visibility10333); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STRICT422_tree = (Object)adaptor.create(STRICT422);
adaptor.addChild(root_0, STRICT422_tree);
}
}
break;
}
PRIVATE423=(Token)match(input,PRIVATE,FOLLOW_PRIVATE_in_visibility10336); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PRIVATE423_tree = new VisibilityNodeImpl(PRIVATE423) ;
root_0 = (Object)adaptor.becomeRoot(PRIVATE423_tree, root_0);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:703:32: PUBLIC
{
root_0 = (Object)adaptor.nil();
PUBLIC424=(Token)match(input,PUBLIC,FOLLOW_PUBLIC_in_visibility10373); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PUBLIC424_tree = new VisibilityNodeImpl(PUBLIC424) ;
adaptor.addChild(root_0, PUBLIC424_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:704:32: PUBLISHED
{
root_0 = (Object)adaptor.nil();
PUBLISHED425=(Token)match(input,PUBLISHED,FOLLOW_PUBLISHED_in_visibility10409); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PUBLISHED425_tree = new VisibilityNodeImpl(PUBLISHED425) ;
adaptor.addChild(root_0, PUBLISHED425_tree);
}
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:705:32: AUTOMATED
{
root_0 = (Object)adaptor.nil();
AUTOMATED426=(Token)match(input,AUTOMATED,FOLLOW_AUTOMATED_in_visibility10445); if (state.failed) return retval;
if ( state.backtracking==0 ) {
AUTOMATED426_tree = new VisibilityNodeImpl(AUTOMATED426) ;
adaptor.addChild(root_0, AUTOMATED426_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 106, visibility_StartIndex); }
}
return retval;
}
// $ANTLR end "visibility"
public static class genericDefinition_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "genericDefinition"
// au/com/integradev/delphi/antlr/Delphi.g:711:1: genericDefinition : '<' typeParameterList '>' -> ^( TkGenericDefinition typeParameterList ) ;
public final DelphiParser.genericDefinition_return genericDefinition() throws RecognitionException {
DelphiParser.genericDefinition_return retval = new DelphiParser.genericDefinition_return();
retval.start = input.LT(1);
int genericDefinition_StartIndex = input.index();
Object root_0 = null;
Token char_literal427=null;
Token char_literal429=null;
ParserRuleReturnScope typeParameterList428 =null;
Object char_literal427_tree=null;
Object char_literal429_tree=null;
RewriteRuleTokenStream stream_GREATER_THAN=new RewriteRuleTokenStream(adaptor,"token GREATER_THAN");
RewriteRuleTokenStream stream_LESS_THAN=new RewriteRuleTokenStream(adaptor,"token LESS_THAN");
RewriteRuleSubtreeStream stream_typeParameterList=new RewriteRuleSubtreeStream(adaptor,"rule typeParameterList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 107) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:711:30: ( '<' typeParameterList '>' -> ^( TkGenericDefinition typeParameterList ) )
// au/com/integradev/delphi/antlr/Delphi.g:711:32: '<' typeParameterList '>'
{
char_literal427=(Token)match(input,LESS_THAN,FOLLOW_LESS_THAN_in_genericDefinition10532); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LESS_THAN.add(char_literal427);
pushFollow(FOLLOW_typeParameterList_in_genericDefinition10534);
typeParameterList428=typeParameterList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeParameterList.add(typeParameterList428.getTree());
char_literal429=(Token)match(input,GREATER_THAN,FOLLOW_GREATER_THAN_in_genericDefinition10536); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_GREATER_THAN.add(char_literal429);
// AST REWRITE
// elements: typeParameterList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 712:30: -> ^( TkGenericDefinition typeParameterList )
{
// au/com/integradev/delphi/antlr/Delphi.g:712:33: ^( TkGenericDefinition typeParameterList )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new GenericDefinitionNodeImpl(TkGenericDefinition), root_1);
adaptor.addChild(root_1, stream_typeParameterList.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 107, genericDefinition_StartIndex); }
}
return retval;
}
// $ANTLR end "genericDefinition"
public static class typeParameterList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeParameterList"
// au/com/integradev/delphi/antlr/Delphi.g:714:1: typeParameterList : typeParameter ( ';' ! typeParameter )* ;
public final DelphiParser.typeParameterList_return typeParameterList() throws RecognitionException {
DelphiParser.typeParameterList_return retval = new DelphiParser.typeParameterList_return();
retval.start = input.LT(1);
int typeParameterList_StartIndex = input.index();
Object root_0 = null;
Token char_literal431=null;
ParserRuleReturnScope typeParameter430 =null;
ParserRuleReturnScope typeParameter432 =null;
Object char_literal431_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 108) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:714:30: ( typeParameter ( ';' ! typeParameter )* )
// au/com/integradev/delphi/antlr/Delphi.g:714:32: typeParameter ( ';' ! typeParameter )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeParameter_in_typeParameterList10624);
typeParameter430=typeParameter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeParameter430.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:714:46: ( ';' ! typeParameter )*
loop136:
while (true) {
int alt136=2;
int LA136_0 = input.LA(1);
if ( (LA136_0==SEMICOLON) ) {
alt136=1;
}
switch (alt136) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:714:47: ';' ! typeParameter
{
char_literal431=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_typeParameterList10627); if (state.failed) return retval;
pushFollow(FOLLOW_typeParameter_in_typeParameterList10630);
typeParameter432=typeParameter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeParameter432.getTree());
}
break;
default :
break loop136;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 108, typeParameterList_StartIndex); }
}
return retval;
}
// $ANTLR end "typeParameterList"
public static class typeParameter_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "typeParameter"
// au/com/integradev/delphi/antlr/Delphi.g:716:1: typeParameter : nameDeclaration ( ',' nameDeclaration )* ( ':' genericConstraint ( ',' genericConstraint )* )? -> ^( TkTypeParameter nameDeclaration ( nameDeclaration )* ( genericConstraint ( genericConstraint )* )? ) ;
public final DelphiParser.typeParameter_return typeParameter() throws RecognitionException {
DelphiParser.typeParameter_return retval = new DelphiParser.typeParameter_return();
retval.start = input.LT(1);
int typeParameter_StartIndex = input.index();
Object root_0 = null;
Token char_literal434=null;
Token char_literal436=null;
Token char_literal438=null;
ParserRuleReturnScope nameDeclaration433 =null;
ParserRuleReturnScope nameDeclaration435 =null;
ParserRuleReturnScope genericConstraint437 =null;
ParserRuleReturnScope genericConstraint439 =null;
Object char_literal434_tree=null;
Object char_literal436_tree=null;
Object char_literal438_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_genericConstraint=new RewriteRuleSubtreeStream(adaptor,"rule genericConstraint");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 109) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:716:30: ( nameDeclaration ( ',' nameDeclaration )* ( ':' genericConstraint ( ',' genericConstraint )* )? -> ^( TkTypeParameter nameDeclaration ( nameDeclaration )* ( genericConstraint ( genericConstraint )* )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:716:33: nameDeclaration ( ',' nameDeclaration )* ( ':' genericConstraint ( ',' genericConstraint )* )?
{
pushFollow(FOLLOW_nameDeclaration_in_typeParameter10685);
nameDeclaration433=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration433.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:716:49: ( ',' nameDeclaration )*
loop137:
while (true) {
int alt137=2;
int LA137_0 = input.LA(1);
if ( (LA137_0==COMMA) ) {
alt137=1;
}
switch (alt137) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:716:50: ',' nameDeclaration
{
char_literal434=(Token)match(input,COMMA,FOLLOW_COMMA_in_typeParameter10688); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal434);
pushFollow(FOLLOW_nameDeclaration_in_typeParameter10690);
nameDeclaration435=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration435.getTree());
}
break;
default :
break loop137;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:716:72: ( ':' genericConstraint ( ',' genericConstraint )* )?
int alt139=2;
int LA139_0 = input.LA(1);
if ( (LA139_0==COLON) ) {
alt139=1;
}
switch (alt139) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:716:73: ':' genericConstraint ( ',' genericConstraint )*
{
char_literal436=(Token)match(input,COLON,FOLLOW_COLON_in_typeParameter10695); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal436);
pushFollow(FOLLOW_genericConstraint_in_typeParameter10697);
genericConstraint437=genericConstraint();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericConstraint.add(genericConstraint437.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:716:95: ( ',' genericConstraint )*
loop138:
while (true) {
int alt138=2;
int LA138_0 = input.LA(1);
if ( (LA138_0==COMMA) ) {
alt138=1;
}
switch (alt138) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:716:96: ',' genericConstraint
{
char_literal438=(Token)match(input,COMMA,FOLLOW_COMMA_in_typeParameter10700); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal438);
pushFollow(FOLLOW_genericConstraint_in_typeParameter10702);
genericConstraint439=genericConstraint();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericConstraint.add(genericConstraint439.getTree());
}
break;
default :
break loop138;
}
}
}
break;
}
// AST REWRITE
// elements: nameDeclaration, genericConstraint, nameDeclaration, genericConstraint
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 717:30: -> ^( TkTypeParameter nameDeclaration ( nameDeclaration )* ( genericConstraint ( genericConstraint )* )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:717:33: ^( TkTypeParameter nameDeclaration ( nameDeclaration )* ( genericConstraint ( genericConstraint )* )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new TypeParameterNodeImpl(TkTypeParameter), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:718:53: ( nameDeclaration )*
while ( stream_nameDeclaration.hasNext() ) {
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
}
stream_nameDeclaration.reset();
// au/com/integradev/delphi/antlr/Delphi.g:718:72: ( genericConstraint ( genericConstraint )* )?
if ( stream_genericConstraint.hasNext()||stream_genericConstraint.hasNext() ) {
adaptor.addChild(root_1, stream_genericConstraint.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:718:91: ( genericConstraint )*
while ( stream_genericConstraint.hasNext() ) {
adaptor.addChild(root_1, stream_genericConstraint.nextTree());
}
stream_genericConstraint.reset();
}
stream_genericConstraint.reset();
stream_genericConstraint.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 109, typeParameter_StartIndex); }
}
return retval;
}
// $ANTLR end "typeParameter"
public static class genericConstraint_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "genericConstraint"
// au/com/integradev/delphi/antlr/Delphi.g:721:1: genericConstraint : ( typeReference | RECORD | CLASS | CONSTRUCTOR );
public final DelphiParser.genericConstraint_return genericConstraint() throws RecognitionException {
DelphiParser.genericConstraint_return retval = new DelphiParser.genericConstraint_return();
retval.start = input.LT(1);
int genericConstraint_StartIndex = input.index();
Object root_0 = null;
Token RECORD441=null;
Token CLASS442=null;
Token CONSTRUCTOR443=null;
ParserRuleReturnScope typeReference440 =null;
Object RECORD441_tree=null;
Object CLASS442_tree=null;
Object CONSTRUCTOR443_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 110) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:721:30: ( typeReference | RECORD | CLASS | CONSTRUCTOR )
int alt140=4;
switch ( input.LA(1) ) {
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt140=1;
}
break;
case RECORD:
{
alt140=2;
}
break;
case CLASS:
{
alt140=3;
}
break;
case CONSTRUCTOR:
{
alt140=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 140, 0, input);
throw nvae;
}
switch (alt140) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:721:32: typeReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeReference_in_genericConstraint10877);
typeReference440=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference440.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:722:32: RECORD
{
root_0 = (Object)adaptor.nil();
RECORD441=(Token)match(input,RECORD,FOLLOW_RECORD_in_genericConstraint10910); if (state.failed) return retval;
if ( state.backtracking==0 ) {
RECORD441_tree = (Object)adaptor.create(RECORD441);
adaptor.addChild(root_0, RECORD441_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:723:32: CLASS
{
root_0 = (Object)adaptor.nil();
CLASS442=(Token)match(input,CLASS,FOLLOW_CLASS_in_genericConstraint10943); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CLASS442_tree = (Object)adaptor.create(CLASS442);
adaptor.addChild(root_0, CLASS442_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:724:32: CONSTRUCTOR
{
root_0 = (Object)adaptor.nil();
CONSTRUCTOR443=(Token)match(input,CONSTRUCTOR,FOLLOW_CONSTRUCTOR_in_genericConstraint10976); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CONSTRUCTOR443_tree = (Object)adaptor.create(CONSTRUCTOR443);
adaptor.addChild(root_0, CONSTRUCTOR443_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 110, genericConstraint_StartIndex); }
}
return retval;
}
// $ANTLR end "genericConstraint"
public static class genericArguments_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "genericArguments"
// au/com/integradev/delphi/antlr/Delphi.g:726:1: genericArguments : '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>' -> ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>' ) ;
public final DelphiParser.genericArguments_return genericArguments() throws RecognitionException {
DelphiParser.genericArguments_return retval = new DelphiParser.genericArguments_return();
retval.start = input.LT(1);
int genericArguments_StartIndex = input.index();
Object root_0 = null;
Token char_literal444=null;
Token char_literal446=null;
Token char_literal448=null;
ParserRuleReturnScope typeReferenceOrStringOrFile445 =null;
ParserRuleReturnScope typeReferenceOrStringOrFile447 =null;
Object char_literal444_tree=null;
Object char_literal446_tree=null;
Object char_literal448_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleTokenStream stream_GREATER_THAN=new RewriteRuleTokenStream(adaptor,"token GREATER_THAN");
RewriteRuleTokenStream stream_LESS_THAN=new RewriteRuleTokenStream(adaptor,"token LESS_THAN");
RewriteRuleSubtreeStream stream_typeReferenceOrStringOrFile=new RewriteRuleSubtreeStream(adaptor,"rule typeReferenceOrStringOrFile");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 111) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:726:30: ( '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>' -> ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>' ) )
// au/com/integradev/delphi/antlr/Delphi.g:726:32: '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>'
{
char_literal444=(Token)match(input,LESS_THAN,FOLLOW_LESS_THAN_in_genericArguments11025); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LESS_THAN.add(char_literal444);
pushFollow(FOLLOW_typeReferenceOrStringOrFile_in_genericArguments11027);
typeReferenceOrStringOrFile445=typeReferenceOrStringOrFile();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReferenceOrStringOrFile.add(typeReferenceOrStringOrFile445.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:726:64: ( ',' typeReferenceOrStringOrFile )*
loop141:
while (true) {
int alt141=2;
int LA141_0 = input.LA(1);
if ( (LA141_0==COMMA) ) {
alt141=1;
}
switch (alt141) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:726:65: ',' typeReferenceOrStringOrFile
{
char_literal446=(Token)match(input,COMMA,FOLLOW_COMMA_in_genericArguments11030); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal446);
pushFollow(FOLLOW_typeReferenceOrStringOrFile_in_genericArguments11032);
typeReferenceOrStringOrFile447=typeReferenceOrStringOrFile();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReferenceOrStringOrFile.add(typeReferenceOrStringOrFile447.getTree());
}
break;
default :
break loop141;
}
}
char_literal448=(Token)match(input,GREATER_THAN,FOLLOW_GREATER_THAN_in_genericArguments11036); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_GREATER_THAN.add(char_literal448);
// AST REWRITE
// elements: typeReferenceOrStringOrFile, COMMA, LESS_THAN, GREATER_THAN, typeReferenceOrStringOrFile
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 727:30: -> ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>' )
{
// au/com/integradev/delphi/antlr/Delphi.g:727:33: ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( ',' typeReferenceOrStringOrFile )* '>' )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new GenericArgumentsNodeImpl(TkGenericArguments), root_1);
adaptor.addChild(root_1, stream_LESS_THAN.nextNode());
adaptor.addChild(root_1, stream_typeReferenceOrStringOrFile.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:727:112: ( ',' typeReferenceOrStringOrFile )*
while ( stream_typeReferenceOrStringOrFile.hasNext()||stream_COMMA.hasNext() ) {
adaptor.addChild(root_1, stream_COMMA.nextNode());
adaptor.addChild(root_1, stream_typeReferenceOrStringOrFile.nextTree());
}
stream_typeReferenceOrStringOrFile.reset();
stream_COMMA.reset();
adaptor.addChild(root_1, stream_GREATER_THAN.nextNode());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 111, genericArguments_StartIndex); }
}
return retval;
}
// $ANTLR end "genericArguments"
public static class routineNameGenericArguments_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineNameGenericArguments"
// au/com/integradev/delphi/antlr/Delphi.g:729:1: routineNameGenericArguments : '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>' -> ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>' ) ;
public final DelphiParser.routineNameGenericArguments_return routineNameGenericArguments() throws RecognitionException {
DelphiParser.routineNameGenericArguments_return retval = new DelphiParser.routineNameGenericArguments_return();
retval.start = input.LT(1);
int routineNameGenericArguments_StartIndex = input.index();
Object root_0 = null;
Token char_literal449=null;
Token char_literal453=null;
ParserRuleReturnScope typeReferenceOrStringOrFile450 =null;
ParserRuleReturnScope commaOrSemicolon451 =null;
ParserRuleReturnScope typeReferenceOrStringOrFile452 =null;
Object char_literal449_tree=null;
Object char_literal453_tree=null;
RewriteRuleTokenStream stream_GREATER_THAN=new RewriteRuleTokenStream(adaptor,"token GREATER_THAN");
RewriteRuleTokenStream stream_LESS_THAN=new RewriteRuleTokenStream(adaptor,"token LESS_THAN");
RewriteRuleSubtreeStream stream_commaOrSemicolon=new RewriteRuleSubtreeStream(adaptor,"rule commaOrSemicolon");
RewriteRuleSubtreeStream stream_typeReferenceOrStringOrFile=new RewriteRuleSubtreeStream(adaptor,"rule typeReferenceOrStringOrFile");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 112) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:729:30: ( '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>' -> ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>' ) )
// au/com/integradev/delphi/antlr/Delphi.g:729:32: '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>'
{
char_literal449=(Token)match(input,LESS_THAN,FOLLOW_LESS_THAN_in_routineNameGenericArguments11125); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LESS_THAN.add(char_literal449);
pushFollow(FOLLOW_typeReferenceOrStringOrFile_in_routineNameGenericArguments11127);
typeReferenceOrStringOrFile450=typeReferenceOrStringOrFile();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReferenceOrStringOrFile.add(typeReferenceOrStringOrFile450.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:729:64: ( commaOrSemicolon typeReferenceOrStringOrFile )*
loop142:
while (true) {
int alt142=2;
int LA142_0 = input.LA(1);
if ( (LA142_0==COMMA||LA142_0==SEMICOLON) ) {
alt142=1;
}
switch (alt142) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:729:65: commaOrSemicolon typeReferenceOrStringOrFile
{
pushFollow(FOLLOW_commaOrSemicolon_in_routineNameGenericArguments11130);
commaOrSemicolon451=commaOrSemicolon();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_commaOrSemicolon.add(commaOrSemicolon451.getTree());
pushFollow(FOLLOW_typeReferenceOrStringOrFile_in_routineNameGenericArguments11132);
typeReferenceOrStringOrFile452=typeReferenceOrStringOrFile();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_typeReferenceOrStringOrFile.add(typeReferenceOrStringOrFile452.getTree());
}
break;
default :
break loop142;
}
}
char_literal453=(Token)match(input,GREATER_THAN,FOLLOW_GREATER_THAN_in_routineNameGenericArguments11136); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_GREATER_THAN.add(char_literal453);
// AST REWRITE
// elements: typeReferenceOrStringOrFile, GREATER_THAN, LESS_THAN, typeReferenceOrStringOrFile, commaOrSemicolon
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 730:30: -> ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>' )
{
// au/com/integradev/delphi/antlr/Delphi.g:730:33: ^( TkGenericArguments '<' typeReferenceOrStringOrFile ( commaOrSemicolon typeReferenceOrStringOrFile )* '>' )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new GenericArgumentsNodeImpl(TkGenericArguments), root_1);
adaptor.addChild(root_1, stream_LESS_THAN.nextNode());
adaptor.addChild(root_1, stream_typeReferenceOrStringOrFile.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:730:112: ( commaOrSemicolon typeReferenceOrStringOrFile )*
while ( stream_typeReferenceOrStringOrFile.hasNext()||stream_commaOrSemicolon.hasNext() ) {
adaptor.addChild(root_1, stream_commaOrSemicolon.nextTree());
adaptor.addChild(root_1, stream_typeReferenceOrStringOrFile.nextTree());
}
stream_typeReferenceOrStringOrFile.reset();
stream_commaOrSemicolon.reset();
adaptor.addChild(root_1, stream_GREATER_THAN.nextNode());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 112, routineNameGenericArguments_StartIndex); }
}
return retval;
}
// $ANTLR end "routineNameGenericArguments"
public static class commaOrSemicolon_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "commaOrSemicolon"
// au/com/integradev/delphi/antlr/Delphi.g:732:1: commaOrSemicolon : ( ',' | ';' );
public final DelphiParser.commaOrSemicolon_return commaOrSemicolon() throws RecognitionException {
DelphiParser.commaOrSemicolon_return retval = new DelphiParser.commaOrSemicolon_return();
retval.start = input.LT(1);
int commaOrSemicolon_StartIndex = input.index();
Object root_0 = null;
Token set454=null;
Object set454_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 113) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:732:30: ( ',' | ';' )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set454=input.LT(1);
if ( input.LA(1)==COMMA||input.LA(1)==SEMICOLON ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set454));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 113, commaOrSemicolon_StartIndex); }
}
return retval;
}
// $ANTLR end "commaOrSemicolon"
public static class methodResolutionClause_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "methodResolutionClause"
// au/com/integradev/delphi/antlr/Delphi.g:738:1: methodResolutionClause : key= ( FUNCTION | PROCEDURE ) interfaceMethod= nameReference '=' implemented= nameReference ';' -> ^( TkMethodResolveClause $key $interfaceMethod $implemented) ;
public final DelphiParser.methodResolutionClause_return methodResolutionClause() throws RecognitionException {
DelphiParser.methodResolutionClause_return retval = new DelphiParser.methodResolutionClause_return();
retval.start = input.LT(1);
int methodResolutionClause_StartIndex = input.index();
Object root_0 = null;
Token key=null;
Token char_literal455=null;
Token char_literal456=null;
ParserRuleReturnScope interfaceMethod =null;
ParserRuleReturnScope implemented =null;
Object key_tree=null;
Object char_literal455_tree=null;
Object char_literal456_tree=null;
RewriteRuleTokenStream stream_PROCEDURE=new RewriteRuleTokenStream(adaptor,"token PROCEDURE");
RewriteRuleTokenStream stream_EQUAL=new RewriteRuleTokenStream(adaptor,"token EQUAL");
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_FUNCTION=new RewriteRuleTokenStream(adaptor,"token FUNCTION");
RewriteRuleSubtreeStream stream_nameReference=new RewriteRuleSubtreeStream(adaptor,"rule nameReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 114) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:738:30: (key= ( FUNCTION | PROCEDURE ) interfaceMethod= nameReference '=' implemented= nameReference ';' -> ^( TkMethodResolveClause $key $interfaceMethod $implemented) )
// au/com/integradev/delphi/antlr/Delphi.g:738:32: key= ( FUNCTION | PROCEDURE ) interfaceMethod= nameReference '=' implemented= nameReference ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:738:36: ( FUNCTION | PROCEDURE )
int alt143=2;
int LA143_0 = input.LA(1);
if ( (LA143_0==FUNCTION) ) {
alt143=1;
}
else if ( (LA143_0==PROCEDURE) ) {
alt143=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 143, 0, input);
throw nvae;
}
switch (alt143) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:738:37: FUNCTION
{
key=(Token)match(input,FUNCTION,FOLLOW_FUNCTION_in_methodResolutionClause11290); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_FUNCTION.add(key);
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:738:48: PROCEDURE
{
key=(Token)match(input,PROCEDURE,FOLLOW_PROCEDURE_in_methodResolutionClause11294); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PROCEDURE.add(key);
}
break;
}
pushFollow(FOLLOW_nameReference_in_methodResolutionClause11299);
interfaceMethod=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameReference.add(interfaceMethod.getTree());
char_literal455=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_methodResolutionClause11301); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal455);
pushFollow(FOLLOW_nameReference_in_methodResolutionClause11305);
implemented=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameReference.add(implemented.getTree());
char_literal456=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_methodResolutionClause11307); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal456);
// AST REWRITE
// elements: implemented, key, interfaceMethod
// token labels: key
// rule labels: interfaceMethod, implemented, retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleTokenStream stream_key=new RewriteRuleTokenStream(adaptor,"token key",key);
RewriteRuleSubtreeStream stream_interfaceMethod=new RewriteRuleSubtreeStream(adaptor,"rule interfaceMethod",interfaceMethod!=null?interfaceMethod.getTree():null);
RewriteRuleSubtreeStream stream_implemented=new RewriteRuleSubtreeStream(adaptor,"rule implemented",implemented!=null?implemented.getTree():null);
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 739:30: -> ^( TkMethodResolveClause $key $interfaceMethod $implemented)
{
// au/com/integradev/delphi/antlr/Delphi.g:739:33: ^( TkMethodResolveClause $key $interfaceMethod $implemented)
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new MethodResolutionClauseNodeImpl(TkMethodResolveClause), root_1);
adaptor.addChild(root_1, stream_key.nextNode());
adaptor.addChild(root_1, stream_interfaceMethod.nextTree());
adaptor.addChild(root_1, stream_implemented.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 114, methodResolutionClause_StartIndex); }
}
return retval;
}
// $ANTLR end "methodResolutionClause"
public static class routineInterface_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineInterface"
// au/com/integradev/delphi/antlr/Delphi.g:743:1: routineInterface : routineInterfaceHeading -> ^( TkRoutineDeclaration routineInterfaceHeading ) ;
public final DelphiParser.routineInterface_return routineInterface() throws RecognitionException {
DelphiParser.routineInterface_return retval = new DelphiParser.routineInterface_return();
retval.start = input.LT(1);
int routineInterface_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope routineInterfaceHeading457 =null;
RewriteRuleSubtreeStream stream_routineInterfaceHeading=new RewriteRuleSubtreeStream(adaptor,"rule routineInterfaceHeading");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 115) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:743:30: ( routineInterfaceHeading -> ^( TkRoutineDeclaration routineInterfaceHeading ) )
// au/com/integradev/delphi/antlr/Delphi.g:743:32: routineInterfaceHeading
{
pushFollow(FOLLOW_routineInterfaceHeading_in_routineInterface11473);
routineInterfaceHeading457=routineInterfaceHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineInterfaceHeading.add(routineInterfaceHeading457.getTree());
// AST REWRITE
// elements: routineInterfaceHeading
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 744:30: -> ^( TkRoutineDeclaration routineInterfaceHeading )
{
// au/com/integradev/delphi/antlr/Delphi.g:744:33: ^( TkRoutineDeclaration routineInterfaceHeading )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineDeclarationNodeImpl(TkRoutineDeclaration), root_1);
adaptor.addChild(root_1, stream_routineInterfaceHeading.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 115, routineInterface_StartIndex); }
}
return retval;
}
// $ANTLR end "routineInterface"
public static class routineImplementation_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineImplementation"
// au/com/integradev/delphi/antlr/Delphi.g:748:1: routineImplementation : ( fullRoutineImplementation | externalRoutine | forwardRoutine );
public final DelphiParser.routineImplementation_return routineImplementation() throws RecognitionException {
DelphiParser.routineImplementation_return retval = new DelphiParser.routineImplementation_return();
retval.start = input.LT(1);
int routineImplementation_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope fullRoutineImplementation458 =null;
ParserRuleReturnScope externalRoutine459 =null;
ParserRuleReturnScope forwardRoutine460 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 116) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:748:30: ( fullRoutineImplementation | externalRoutine | forwardRoutine )
int alt144=3;
switch ( input.LA(1) ) {
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA144_1 = input.LA(2);
if ( (synpred209_Delphi()) ) {
alt144=1;
}
else if ( (synpred210_Delphi()) ) {
alt144=2;
}
else if ( (true) ) {
alt144=3;
}
}
break;
case CLASS:
{
int LA144_2 = input.LA(2);
if ( (synpred209_Delphi()) ) {
alt144=1;
}
else if ( (synpred210_Delphi()) ) {
alt144=2;
}
else if ( (true) ) {
alt144=3;
}
}
break;
case CONSTRUCTOR:
case DESTRUCTOR:
case FUNCTION:
case OPERATOR:
case PROCEDURE:
{
int LA144_3 = input.LA(2);
if ( (synpred209_Delphi()) ) {
alt144=1;
}
else if ( (synpred210_Delphi()) ) {
alt144=2;
}
else if ( (true) ) {
alt144=3;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 144, 0, input);
throw nvae;
}
switch (alt144) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:748:32: fullRoutineImplementation
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_fullRoutineImplementation_in_routineImplementation11627);
fullRoutineImplementation458=fullRoutineImplementation();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, fullRoutineImplementation458.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:749:32: externalRoutine
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_externalRoutine_in_routineImplementation11660);
externalRoutine459=externalRoutine();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, externalRoutine459.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:750:32: forwardRoutine
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_forwardRoutine_in_routineImplementation11693);
forwardRoutine460=forwardRoutine();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, forwardRoutine460.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 116, routineImplementation_StartIndex); }
}
return retval;
}
// $ANTLR end "routineImplementation"
public static class fullRoutineImplementation_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "fullRoutineImplementation"
// au/com/integradev/delphi/antlr/Delphi.g:752:1: fullRoutineImplementation : routineImplementationHeading routineBody -> ^( TkRoutineImplementation routineImplementationHeading routineBody ) ;
public final DelphiParser.fullRoutineImplementation_return fullRoutineImplementation() throws RecognitionException {
DelphiParser.fullRoutineImplementation_return retval = new DelphiParser.fullRoutineImplementation_return();
retval.start = input.LT(1);
int fullRoutineImplementation_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope routineImplementationHeading461 =null;
ParserRuleReturnScope routineBody462 =null;
RewriteRuleSubtreeStream stream_routineImplementationHeading=new RewriteRuleSubtreeStream(adaptor,"rule routineImplementationHeading");
RewriteRuleSubtreeStream stream_routineBody=new RewriteRuleSubtreeStream(adaptor,"rule routineBody");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 117) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:752:30: ( routineImplementationHeading routineBody -> ^( TkRoutineImplementation routineImplementationHeading routineBody ) )
// au/com/integradev/delphi/antlr/Delphi.g:752:32: routineImplementationHeading routineBody
{
pushFollow(FOLLOW_routineImplementationHeading_in_fullRoutineImplementation11733);
routineImplementationHeading461=routineImplementationHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineImplementationHeading.add(routineImplementationHeading461.getTree());
pushFollow(FOLLOW_routineBody_in_fullRoutineImplementation11735);
routineBody462=routineBody();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineBody.add(routineBody462.getTree());
// AST REWRITE
// elements: routineImplementationHeading, routineBody
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 753:30: -> ^( TkRoutineImplementation routineImplementationHeading routineBody )
{
// au/com/integradev/delphi/antlr/Delphi.g:753:33: ^( TkRoutineImplementation routineImplementationHeading routineBody )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineImplementationNodeImpl(TkRoutineImplementation), root_1);
adaptor.addChild(root_1, stream_routineImplementationHeading.nextTree());
adaptor.addChild(root_1, stream_routineBody.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 117, fullRoutineImplementation_StartIndex); }
}
return retval;
}
// $ANTLR end "fullRoutineImplementation"
public static class externalRoutine_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "externalRoutine"
// au/com/integradev/delphi/antlr/Delphi.g:758:1: externalRoutine : externalRoutineHeading -> ^( TkRoutineImplementation externalRoutineHeading ) ;
public final DelphiParser.externalRoutine_return externalRoutine() throws RecognitionException {
DelphiParser.externalRoutine_return retval = new DelphiParser.externalRoutine_return();
retval.start = input.LT(1);
int externalRoutine_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope externalRoutineHeading463 =null;
RewriteRuleSubtreeStream stream_externalRoutineHeading=new RewriteRuleSubtreeStream(adaptor,"rule externalRoutineHeading");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 118) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:758:30: ( externalRoutineHeading -> ^( TkRoutineImplementation externalRoutineHeading ) )
// au/com/integradev/delphi/antlr/Delphi.g:758:32: externalRoutineHeading
{
pushFollow(FOLLOW_externalRoutineHeading_in_externalRoutine11933);
externalRoutineHeading463=externalRoutineHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_externalRoutineHeading.add(externalRoutineHeading463.getTree());
// AST REWRITE
// elements: externalRoutineHeading
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 759:30: -> ^( TkRoutineImplementation externalRoutineHeading )
{
// au/com/integradev/delphi/antlr/Delphi.g:759:33: ^( TkRoutineImplementation externalRoutineHeading )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineImplementationNodeImpl(TkRoutineImplementation), root_1);
adaptor.addChild(root_1, stream_externalRoutineHeading.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 118, externalRoutine_StartIndex); }
}
return retval;
}
// $ANTLR end "externalRoutine"
public static class forwardRoutine_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "forwardRoutine"
// au/com/integradev/delphi/antlr/Delphi.g:763:1: forwardRoutine : forwardRoutineHeading -> ^( TkRoutineDeclaration forwardRoutineHeading ) ;
public final DelphiParser.forwardRoutine_return forwardRoutine() throws RecognitionException {
DelphiParser.forwardRoutine_return retval = new DelphiParser.forwardRoutine_return();
retval.start = input.LT(1);
int forwardRoutine_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope forwardRoutineHeading464 =null;
RewriteRuleSubtreeStream stream_forwardRoutineHeading=new RewriteRuleSubtreeStream(adaptor,"rule forwardRoutineHeading");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 119) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:763:30: ( forwardRoutineHeading -> ^( TkRoutineDeclaration forwardRoutineHeading ) )
// au/com/integradev/delphi/antlr/Delphi.g:763:32: forwardRoutineHeading
{
pushFollow(FOLLOW_forwardRoutineHeading_in_forwardRoutine12094);
forwardRoutineHeading464=forwardRoutineHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_forwardRoutineHeading.add(forwardRoutineHeading464.getTree());
// AST REWRITE
// elements: forwardRoutineHeading
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 764:30: -> ^( TkRoutineDeclaration forwardRoutineHeading )
{
// au/com/integradev/delphi/antlr/Delphi.g:764:33: ^( TkRoutineDeclaration forwardRoutineHeading )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineDeclarationNodeImpl(TkRoutineDeclaration), root_1);
adaptor.addChild(root_1, stream_forwardRoutineHeading.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 119, forwardRoutine_StartIndex); }
}
return retval;
}
// $ANTLR end "forwardRoutine"
public static class routineInterfaceHeading_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineInterfaceHeading"
// au/com/integradev/delphi/antlr/Delphi.g:768:1: routineInterfaceHeading : ( attributeList )? ( CLASS )? routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? interfaceDirectiveSection -> ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? interfaceDirectiveSection ) ;
public final DelphiParser.routineInterfaceHeading_return routineInterfaceHeading() throws RecognitionException {
DelphiParser.routineInterfaceHeading_return retval = new DelphiParser.routineInterfaceHeading_return();
retval.start = input.LT(1);
int routineInterfaceHeading_StartIndex = input.index();
Object root_0 = null;
Token CLASS466=null;
ParserRuleReturnScope attributeList465 =null;
ParserRuleReturnScope routineKey467 =null;
ParserRuleReturnScope routineDeclarationName468 =null;
ParserRuleReturnScope routineParameters469 =null;
ParserRuleReturnScope routineReturnType470 =null;
ParserRuleReturnScope interfaceDirectiveSection471 =null;
Object CLASS466_tree=null;
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_routineReturnType=new RewriteRuleSubtreeStream(adaptor,"rule routineReturnType");
RewriteRuleSubtreeStream stream_interfaceDirectiveSection=new RewriteRuleSubtreeStream(adaptor,"rule interfaceDirectiveSection");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_routineKey=new RewriteRuleSubtreeStream(adaptor,"rule routineKey");
RewriteRuleSubtreeStream stream_routineDeclarationName=new RewriteRuleSubtreeStream(adaptor,"rule routineDeclarationName");
RewriteRuleSubtreeStream stream_routineParameters=new RewriteRuleSubtreeStream(adaptor,"rule routineParameters");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 120) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:768:30: ( ( attributeList )? ( CLASS )? routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? interfaceDirectiveSection -> ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? interfaceDirectiveSection ) )
// au/com/integradev/delphi/antlr/Delphi.g:768:32: ( attributeList )? ( CLASS )? routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? interfaceDirectiveSection
{
// au/com/integradev/delphi/antlr/Delphi.g:768:32: ( attributeList )?
int alt145=2;
int LA145_0 = input.LA(1);
if ( (LA145_0==PAREN_BRACKET_LEFT||LA145_0==SQUARE_BRACKET_LEFT) ) {
alt145=1;
}
switch (alt145) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:768:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_routineInterfaceHeading12246);
attributeList465=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList465.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:768:47: ( CLASS )?
int alt146=2;
int LA146_0 = input.LA(1);
if ( (LA146_0==CLASS) ) {
alt146=1;
}
switch (alt146) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:768:47: CLASS
{
CLASS466=(Token)match(input,CLASS,FOLLOW_CLASS_in_routineInterfaceHeading12249); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS466);
}
break;
}
pushFollow(FOLLOW_routineKey_in_routineInterfaceHeading12252);
routineKey467=routineKey();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineKey.add(routineKey467.getTree());
pushFollow(FOLLOW_routineDeclarationName_in_routineInterfaceHeading12254);
routineDeclarationName468=routineDeclarationName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineDeclarationName.add(routineDeclarationName468.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:768:88: ( routineParameters )?
int alt147=2;
int LA147_0 = input.LA(1);
if ( (LA147_0==PAREN_LEFT) ) {
alt147=1;
}
switch (alt147) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:768:88: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_routineInterfaceHeading12256);
routineParameters469=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineParameters.add(routineParameters469.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:768:107: ( routineReturnType )?
int alt148=2;
int LA148_0 = input.LA(1);
if ( (LA148_0==COLON) ) {
alt148=1;
}
switch (alt148) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:768:107: routineReturnType
{
pushFollow(FOLLOW_routineReturnType_in_routineInterfaceHeading12259);
routineReturnType470=routineReturnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineReturnType.add(routineReturnType470.getTree());
}
break;
}
pushFollow(FOLLOW_interfaceDirectiveSection_in_routineInterfaceHeading12262);
interfaceDirectiveSection471=interfaceDirectiveSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_interfaceDirectiveSection.add(interfaceDirectiveSection471.getTree());
// AST REWRITE
// elements: CLASS, routineKey, routineReturnType, routineParameters, attributeList, interfaceDirectiveSection, routineDeclarationName
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 769:30: -> ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? interfaceDirectiveSection )
{
// au/com/integradev/delphi/antlr/Delphi.g:769:33: ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? interfaceDirectiveSection )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineHeadingNodeImpl(TkRoutineHeading), root_1);
adaptor.addChild(root_1, stream_routineKey.nextTree());
adaptor.addChild(root_1, stream_routineDeclarationName.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:772:37: ( routineParameters )?
if ( stream_routineParameters.hasNext() ) {
adaptor.addChild(root_1, stream_routineParameters.nextTree());
}
stream_routineParameters.reset();
// au/com/integradev/delphi/antlr/Delphi.g:773:37: ( routineReturnType )?
if ( stream_routineReturnType.hasNext() ) {
adaptor.addChild(root_1, stream_routineReturnType.nextTree());
}
stream_routineReturnType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:774:37: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:775:37: ( CLASS )?
if ( stream_CLASS.hasNext() ) {
adaptor.addChild(root_1, stream_CLASS.nextNode());
}
stream_CLASS.reset();
adaptor.addChild(root_1, stream_interfaceDirectiveSection.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 120, routineInterfaceHeading_StartIndex); }
}
return retval;
}
// $ANTLR end "routineInterfaceHeading"
public static class routineImplementationHeading_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineImplementationHeading"
// au/com/integradev/delphi/antlr/Delphi.g:779:1: routineImplementationHeading : ( attributeList )? ( CLASS )? routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? implDirectiveSection -> ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? implDirectiveSection ) ;
public final DelphiParser.routineImplementationHeading_return routineImplementationHeading() throws RecognitionException {
DelphiParser.routineImplementationHeading_return retval = new DelphiParser.routineImplementationHeading_return();
retval.start = input.LT(1);
int routineImplementationHeading_StartIndex = input.index();
Object root_0 = null;
Token CLASS473=null;
ParserRuleReturnScope attributeList472 =null;
ParserRuleReturnScope routineKey474 =null;
ParserRuleReturnScope routineImplementationName475 =null;
ParserRuleReturnScope routineParameters476 =null;
ParserRuleReturnScope routineReturnType477 =null;
ParserRuleReturnScope implDirectiveSection478 =null;
Object CLASS473_tree=null;
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_routineReturnType=new RewriteRuleSubtreeStream(adaptor,"rule routineReturnType");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_routineKey=new RewriteRuleSubtreeStream(adaptor,"rule routineKey");
RewriteRuleSubtreeStream stream_routineImplementationName=new RewriteRuleSubtreeStream(adaptor,"rule routineImplementationName");
RewriteRuleSubtreeStream stream_routineParameters=new RewriteRuleSubtreeStream(adaptor,"rule routineParameters");
RewriteRuleSubtreeStream stream_implDirectiveSection=new RewriteRuleSubtreeStream(adaptor,"rule implDirectiveSection");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 121) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:779:30: ( ( attributeList )? ( CLASS )? routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? implDirectiveSection -> ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? implDirectiveSection ) )
// au/com/integradev/delphi/antlr/Delphi.g:779:32: ( attributeList )? ( CLASS )? routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? implDirectiveSection
{
// au/com/integradev/delphi/antlr/Delphi.g:779:32: ( attributeList )?
int alt149=2;
int LA149_0 = input.LA(1);
if ( (LA149_0==PAREN_BRACKET_LEFT||LA149_0==SQUARE_BRACKET_LEFT) ) {
alt149=1;
}
switch (alt149) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:779:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_routineImplementationHeading12641);
attributeList472=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList472.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:779:47: ( CLASS )?
int alt150=2;
int LA150_0 = input.LA(1);
if ( (LA150_0==CLASS) ) {
alt150=1;
}
switch (alt150) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:779:47: CLASS
{
CLASS473=(Token)match(input,CLASS,FOLLOW_CLASS_in_routineImplementationHeading12644); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS473);
}
break;
}
pushFollow(FOLLOW_routineKey_in_routineImplementationHeading12647);
routineKey474=routineKey();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineKey.add(routineKey474.getTree());
pushFollow(FOLLOW_routineImplementationName_in_routineImplementationHeading12649);
routineImplementationName475=routineImplementationName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineImplementationName.add(routineImplementationName475.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:779:91: ( routineParameters )?
int alt151=2;
int LA151_0 = input.LA(1);
if ( (LA151_0==PAREN_LEFT) ) {
alt151=1;
}
switch (alt151) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:779:91: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_routineImplementationHeading12651);
routineParameters476=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineParameters.add(routineParameters476.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:779:110: ( routineReturnType )?
int alt152=2;
int LA152_0 = input.LA(1);
if ( (LA152_0==COLON) ) {
alt152=1;
}
switch (alt152) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:779:110: routineReturnType
{
pushFollow(FOLLOW_routineReturnType_in_routineImplementationHeading12654);
routineReturnType477=routineReturnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineReturnType.add(routineReturnType477.getTree());
}
break;
}
pushFollow(FOLLOW_implDirectiveSection_in_routineImplementationHeading12657);
implDirectiveSection478=implDirectiveSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_implDirectiveSection.add(implDirectiveSection478.getTree());
// AST REWRITE
// elements: implDirectiveSection, attributeList, routineKey, routineImplementationName, routineParameters, routineReturnType, CLASS
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 780:30: -> ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? implDirectiveSection )
{
// au/com/integradev/delphi/antlr/Delphi.g:780:33: ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? implDirectiveSection )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineHeadingNodeImpl(TkRoutineHeading), root_1);
adaptor.addChild(root_1, stream_routineKey.nextTree());
adaptor.addChild(root_1, stream_routineImplementationName.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:783:37: ( routineParameters )?
if ( stream_routineParameters.hasNext() ) {
adaptor.addChild(root_1, stream_routineParameters.nextTree());
}
stream_routineParameters.reset();
// au/com/integradev/delphi/antlr/Delphi.g:784:37: ( routineReturnType )?
if ( stream_routineReturnType.hasNext() ) {
adaptor.addChild(root_1, stream_routineReturnType.nextTree());
}
stream_routineReturnType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:785:37: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:786:37: ( CLASS )?
if ( stream_CLASS.hasNext() ) {
adaptor.addChild(root_1, stream_CLASS.nextNode());
}
stream_CLASS.reset();
adaptor.addChild(root_1, stream_implDirectiveSection.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 121, routineImplementationHeading_StartIndex); }
}
return retval;
}
// $ANTLR end "routineImplementationHeading"
public static class externalRoutineHeading_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "externalRoutineHeading"
// au/com/integradev/delphi/antlr/Delphi.g:790:1: externalRoutineHeading : ( attributeList )? ( CLASS )? routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? externalDirectiveSection -> ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? externalDirectiveSection ) ;
public final DelphiParser.externalRoutineHeading_return externalRoutineHeading() throws RecognitionException {
DelphiParser.externalRoutineHeading_return retval = new DelphiParser.externalRoutineHeading_return();
retval.start = input.LT(1);
int externalRoutineHeading_StartIndex = input.index();
Object root_0 = null;
Token CLASS480=null;
ParserRuleReturnScope attributeList479 =null;
ParserRuleReturnScope routineKey481 =null;
ParserRuleReturnScope routineImplementationName482 =null;
ParserRuleReturnScope routineParameters483 =null;
ParserRuleReturnScope routineReturnType484 =null;
ParserRuleReturnScope externalDirectiveSection485 =null;
Object CLASS480_tree=null;
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_routineReturnType=new RewriteRuleSubtreeStream(adaptor,"rule routineReturnType");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_externalDirectiveSection=new RewriteRuleSubtreeStream(adaptor,"rule externalDirectiveSection");
RewriteRuleSubtreeStream stream_routineKey=new RewriteRuleSubtreeStream(adaptor,"rule routineKey");
RewriteRuleSubtreeStream stream_routineImplementationName=new RewriteRuleSubtreeStream(adaptor,"rule routineImplementationName");
RewriteRuleSubtreeStream stream_routineParameters=new RewriteRuleSubtreeStream(adaptor,"rule routineParameters");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 122) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:790:30: ( ( attributeList )? ( CLASS )? routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? externalDirectiveSection -> ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? externalDirectiveSection ) )
// au/com/integradev/delphi/antlr/Delphi.g:790:32: ( attributeList )? ( CLASS )? routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? externalDirectiveSection
{
// au/com/integradev/delphi/antlr/Delphi.g:790:32: ( attributeList )?
int alt153=2;
int LA153_0 = input.LA(1);
if ( (LA153_0==PAREN_BRACKET_LEFT||LA153_0==SQUARE_BRACKET_LEFT) ) {
alt153=1;
}
switch (alt153) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:790:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_externalRoutineHeading13042);
attributeList479=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList479.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:790:47: ( CLASS )?
int alt154=2;
int LA154_0 = input.LA(1);
if ( (LA154_0==CLASS) ) {
alt154=1;
}
switch (alt154) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:790:47: CLASS
{
CLASS480=(Token)match(input,CLASS,FOLLOW_CLASS_in_externalRoutineHeading13045); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS480);
}
break;
}
pushFollow(FOLLOW_routineKey_in_externalRoutineHeading13048);
routineKey481=routineKey();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineKey.add(routineKey481.getTree());
pushFollow(FOLLOW_routineImplementationName_in_externalRoutineHeading13050);
routineImplementationName482=routineImplementationName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineImplementationName.add(routineImplementationName482.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:790:91: ( routineParameters )?
int alt155=2;
int LA155_0 = input.LA(1);
if ( (LA155_0==PAREN_LEFT) ) {
alt155=1;
}
switch (alt155) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:790:91: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_externalRoutineHeading13052);
routineParameters483=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineParameters.add(routineParameters483.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:790:110: ( routineReturnType )?
int alt156=2;
int LA156_0 = input.LA(1);
if ( (LA156_0==COLON) ) {
alt156=1;
}
switch (alt156) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:790:110: routineReturnType
{
pushFollow(FOLLOW_routineReturnType_in_externalRoutineHeading13055);
routineReturnType484=routineReturnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineReturnType.add(routineReturnType484.getTree());
}
break;
}
pushFollow(FOLLOW_externalDirectiveSection_in_externalRoutineHeading13058);
externalDirectiveSection485=externalDirectiveSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_externalDirectiveSection.add(externalDirectiveSection485.getTree());
// AST REWRITE
// elements: attributeList, externalDirectiveSection, routineParameters, CLASS, routineImplementationName, routineReturnType, routineKey
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 791:30: -> ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? externalDirectiveSection )
{
// au/com/integradev/delphi/antlr/Delphi.g:791:33: ^( TkRoutineHeading routineKey routineImplementationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? externalDirectiveSection )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineHeadingNodeImpl(TkRoutineHeading), root_1);
adaptor.addChild(root_1, stream_routineKey.nextTree());
adaptor.addChild(root_1, stream_routineImplementationName.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:794:37: ( routineParameters )?
if ( stream_routineParameters.hasNext() ) {
adaptor.addChild(root_1, stream_routineParameters.nextTree());
}
stream_routineParameters.reset();
// au/com/integradev/delphi/antlr/Delphi.g:795:37: ( routineReturnType )?
if ( stream_routineReturnType.hasNext() ) {
adaptor.addChild(root_1, stream_routineReturnType.nextTree());
}
stream_routineReturnType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:796:37: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:797:37: ( CLASS )?
if ( stream_CLASS.hasNext() ) {
adaptor.addChild(root_1, stream_CLASS.nextNode());
}
stream_CLASS.reset();
adaptor.addChild(root_1, stream_externalDirectiveSection.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 122, externalRoutineHeading_StartIndex); }
}
return retval;
}
// $ANTLR end "externalRoutineHeading"
public static class forwardRoutineHeading_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "forwardRoutineHeading"
// au/com/integradev/delphi/antlr/Delphi.g:801:1: forwardRoutineHeading : ( attributeList )? ( CLASS )? routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? forwardDirectiveSection -> ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? forwardDirectiveSection ) ;
public final DelphiParser.forwardRoutineHeading_return forwardRoutineHeading() throws RecognitionException {
DelphiParser.forwardRoutineHeading_return retval = new DelphiParser.forwardRoutineHeading_return();
retval.start = input.LT(1);
int forwardRoutineHeading_StartIndex = input.index();
Object root_0 = null;
Token CLASS487=null;
ParserRuleReturnScope attributeList486 =null;
ParserRuleReturnScope routineKey488 =null;
ParserRuleReturnScope routineDeclarationName489 =null;
ParserRuleReturnScope routineParameters490 =null;
ParserRuleReturnScope routineReturnType491 =null;
ParserRuleReturnScope forwardDirectiveSection492 =null;
Object CLASS487_tree=null;
RewriteRuleTokenStream stream_CLASS=new RewriteRuleTokenStream(adaptor,"token CLASS");
RewriteRuleSubtreeStream stream_routineReturnType=new RewriteRuleSubtreeStream(adaptor,"rule routineReturnType");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_forwardDirectiveSection=new RewriteRuleSubtreeStream(adaptor,"rule forwardDirectiveSection");
RewriteRuleSubtreeStream stream_routineKey=new RewriteRuleSubtreeStream(adaptor,"rule routineKey");
RewriteRuleSubtreeStream stream_routineDeclarationName=new RewriteRuleSubtreeStream(adaptor,"rule routineDeclarationName");
RewriteRuleSubtreeStream stream_routineParameters=new RewriteRuleSubtreeStream(adaptor,"rule routineParameters");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 123) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:801:30: ( ( attributeList )? ( CLASS )? routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? forwardDirectiveSection -> ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? forwardDirectiveSection ) )
// au/com/integradev/delphi/antlr/Delphi.g:801:32: ( attributeList )? ( CLASS )? routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? forwardDirectiveSection
{
// au/com/integradev/delphi/antlr/Delphi.g:801:32: ( attributeList )?
int alt157=2;
int LA157_0 = input.LA(1);
if ( (LA157_0==PAREN_BRACKET_LEFT||LA157_0==SQUARE_BRACKET_LEFT) ) {
alt157=1;
}
switch (alt157) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:801:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_forwardRoutineHeading13444);
attributeList486=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList486.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:801:47: ( CLASS )?
int alt158=2;
int LA158_0 = input.LA(1);
if ( (LA158_0==CLASS) ) {
alt158=1;
}
switch (alt158) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:801:47: CLASS
{
CLASS487=(Token)match(input,CLASS,FOLLOW_CLASS_in_forwardRoutineHeading13447); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CLASS.add(CLASS487);
}
break;
}
pushFollow(FOLLOW_routineKey_in_forwardRoutineHeading13450);
routineKey488=routineKey();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineKey.add(routineKey488.getTree());
pushFollow(FOLLOW_routineDeclarationName_in_forwardRoutineHeading13452);
routineDeclarationName489=routineDeclarationName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineDeclarationName.add(routineDeclarationName489.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:801:88: ( routineParameters )?
int alt159=2;
int LA159_0 = input.LA(1);
if ( (LA159_0==PAREN_LEFT) ) {
alt159=1;
}
switch (alt159) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:801:88: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_forwardRoutineHeading13454);
routineParameters490=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineParameters.add(routineParameters490.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:801:107: ( routineReturnType )?
int alt160=2;
int LA160_0 = input.LA(1);
if ( (LA160_0==COLON) ) {
alt160=1;
}
switch (alt160) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:801:107: routineReturnType
{
pushFollow(FOLLOW_routineReturnType_in_forwardRoutineHeading13457);
routineReturnType491=routineReturnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineReturnType.add(routineReturnType491.getTree());
}
break;
}
pushFollow(FOLLOW_forwardDirectiveSection_in_forwardRoutineHeading13460);
forwardDirectiveSection492=forwardDirectiveSection();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_forwardDirectiveSection.add(forwardDirectiveSection492.getTree());
// AST REWRITE
// elements: CLASS, forwardDirectiveSection, routineKey, routineReturnType, routineDeclarationName, attributeList, routineParameters
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 802:30: -> ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? forwardDirectiveSection )
{
// au/com/integradev/delphi/antlr/Delphi.g:802:33: ^( TkRoutineHeading routineKey routineDeclarationName ( routineParameters )? ( routineReturnType )? ( attributeList )? ( CLASS )? forwardDirectiveSection )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineHeadingNodeImpl(TkRoutineHeading), root_1);
adaptor.addChild(root_1, stream_routineKey.nextTree());
adaptor.addChild(root_1, stream_routineDeclarationName.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:805:37: ( routineParameters )?
if ( stream_routineParameters.hasNext() ) {
adaptor.addChild(root_1, stream_routineParameters.nextTree());
}
stream_routineParameters.reset();
// au/com/integradev/delphi/antlr/Delphi.g:806:37: ( routineReturnType )?
if ( stream_routineReturnType.hasNext() ) {
adaptor.addChild(root_1, stream_routineReturnType.nextTree());
}
stream_routineReturnType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:807:37: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:808:37: ( CLASS )?
if ( stream_CLASS.hasNext() ) {
adaptor.addChild(root_1, stream_CLASS.nextNode());
}
stream_CLASS.reset();
adaptor.addChild(root_1, stream_forwardDirectiveSection.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 123, forwardRoutineHeading_StartIndex); }
}
return retval;
}
// $ANTLR end "forwardRoutineHeading"
public static class routineDeclarationName_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineDeclarationName"
// au/com/integradev/delphi/antlr/Delphi.g:812:1: routineDeclarationName : (decl= genericNameDeclaration |decl= specialOpNameDeclaration ) -> ^( TkRoutineName $decl) ;
public final DelphiParser.routineDeclarationName_return routineDeclarationName() throws RecognitionException {
DelphiParser.routineDeclarationName_return retval = new DelphiParser.routineDeclarationName_return();
retval.start = input.LT(1);
int routineDeclarationName_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope decl =null;
RewriteRuleSubtreeStream stream_genericNameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule genericNameDeclaration");
RewriteRuleSubtreeStream stream_specialOpNameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule specialOpNameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 124) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:812:30: ( (decl= genericNameDeclaration |decl= specialOpNameDeclaration ) -> ^( TkRoutineName $decl) )
// au/com/integradev/delphi/antlr/Delphi.g:812:32: (decl= genericNameDeclaration |decl= specialOpNameDeclaration )
{
// au/com/integradev/delphi/antlr/Delphi.g:812:32: (decl= genericNameDeclaration |decl= specialOpNameDeclaration )
int alt161=2;
int LA161_0 = input.LA(1);
if ( ((LA161_0 >= ABSOLUTE && LA161_0 <= ABSTRACT)||LA161_0==ALIGN||(LA161_0 >= ASSEMBLER && LA161_0 <= ASSEMBLY)||(LA161_0 >= AT && LA161_0 <= AUTOMATED)||LA161_0==CDECL||LA161_0==CONTAINS||(LA161_0 >= DEFAULT && LA161_0 <= DEPRECATED)||LA161_0==DISPID||LA161_0==DYNAMIC||(LA161_0 >= EXPERIMENTAL && LA161_0 <= EXPORT)||LA161_0==EXTERNAL||LA161_0==FAR||LA161_0==FINAL||LA161_0==FORWARD||LA161_0==HELPER||LA161_0==IMPLEMENTS||LA161_0==INDEX||LA161_0==LABEL||LA161_0==LOCAL||LA161_0==MESSAGE||(LA161_0 >= NAME && LA161_0 <= NEAR)||LA161_0==NODEFAULT||(LA161_0 >= ON && LA161_0 <= OPERATOR)||(LA161_0 >= OUT && LA161_0 <= OVERRIDE)||LA161_0==PACKAGE||(LA161_0 >= PASCAL && LA161_0 <= PLATFORM)||LA161_0==PRIVATE||(LA161_0 >= PROTECTED && LA161_0 <= PUBLISHED)||(LA161_0 >= READ && LA161_0 <= READONLY)||(LA161_0 >= REFERENCE && LA161_0 <= REINTRODUCE)||(LA161_0 >= REQUIRES && LA161_0 <= RESIDENT)||(LA161_0 >= SAFECALL && LA161_0 <= SEALED)||(LA161_0 >= STATIC && LA161_0 <= STRICT)||LA161_0==TkIdentifier||LA161_0==UNSAFE||(LA161_0 >= VARARGS && LA161_0 <= VIRTUAL)||LA161_0==WINAPI||(LA161_0 >= WRITE && LA161_0 <= WRITEONLY)) ) {
alt161=1;
}
else if ( (LA161_0==IN) ) {
alt161=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 161, 0, input);
throw nvae;
}
switch (alt161) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:813:34: decl= genericNameDeclaration
{
pushFollow(FOLLOW_genericNameDeclaration_in_routineDeclarationName13882);
decl=genericNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericNameDeclaration.add(decl.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:814:34: decl= specialOpNameDeclaration
{
pushFollow(FOLLOW_specialOpNameDeclaration_in_routineDeclarationName13919);
decl=specialOpNameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_specialOpNameDeclaration.add(decl.getTree());
}
break;
}
// AST REWRITE
// elements: decl
// token labels:
// rule labels: decl, retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_decl=new RewriteRuleSubtreeStream(adaptor,"rule decl",decl!=null?decl.getTree():null);
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 816:30: -> ^( TkRoutineName $decl)
{
// au/com/integradev/delphi/antlr/Delphi.g:816:33: ^( TkRoutineName $decl)
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineNameNodeImpl(TkRoutineName), root_1);
adaptor.addChild(root_1, stream_decl.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 124, routineDeclarationName_StartIndex); }
}
return retval;
}
// $ANTLR end "routineDeclarationName"
public static class routineImplementationName_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineImplementationName"
// au/com/integradev/delphi/antlr/Delphi.g:818:1: routineImplementationName : routineNameReference -> ^( TkRoutineName routineNameReference ) ;
public final DelphiParser.routineImplementationName_return routineImplementationName() throws RecognitionException {
DelphiParser.routineImplementationName_return retval = new DelphiParser.routineImplementationName_return();
retval.start = input.LT(1);
int routineImplementationName_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope routineNameReference493 =null;
RewriteRuleSubtreeStream stream_routineNameReference=new RewriteRuleSubtreeStream(adaptor,"rule routineNameReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 125) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:818:30: ( routineNameReference -> ^( TkRoutineName routineNameReference ) )
// au/com/integradev/delphi/antlr/Delphi.g:818:32: routineNameReference
{
pushFollow(FOLLOW_routineNameReference_in_routineImplementationName14031);
routineNameReference493=routineNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineNameReference.add(routineNameReference493.getTree());
// AST REWRITE
// elements: routineNameReference
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 818:53: -> ^( TkRoutineName routineNameReference )
{
// au/com/integradev/delphi/antlr/Delphi.g:818:56: ^( TkRoutineName routineNameReference )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineNameNodeImpl(TkRoutineName), root_1);
adaptor.addChild(root_1, stream_routineNameReference.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 125, routineImplementationName_StartIndex); }
}
return retval;
}
// $ANTLR end "routineImplementationName"
public static class routineKey_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineKey"
// au/com/integradev/delphi/antlr/Delphi.g:820:1: routineKey : ( PROCEDURE | CONSTRUCTOR | DESTRUCTOR | FUNCTION | OPERATOR );
public final DelphiParser.routineKey_return routineKey() throws RecognitionException {
DelphiParser.routineKey_return retval = new DelphiParser.routineKey_return();
retval.start = input.LT(1);
int routineKey_StartIndex = input.index();
Object root_0 = null;
Token set494=null;
Object set494_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 126) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:820:30: ( PROCEDURE | CONSTRUCTOR | DESTRUCTOR | FUNCTION | OPERATOR )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set494=input.LT(1);
if ( input.LA(1)==CONSTRUCTOR||input.LA(1)==DESTRUCTOR||input.LA(1)==FUNCTION||input.LA(1)==OPERATOR||input.LA(1)==PROCEDURE ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set494));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 126, routineKey_StartIndex); }
}
return retval;
}
// $ANTLR end "routineKey"
public static class routineReturnType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineReturnType"
// au/com/integradev/delphi/antlr/Delphi.g:826:1: routineReturnType : ':' ( attributeList )? returnType -> ^( TkRoutineReturn returnType ( attributeList )? ) ;
public final DelphiParser.routineReturnType_return routineReturnType() throws RecognitionException {
DelphiParser.routineReturnType_return retval = new DelphiParser.routineReturnType_return();
retval.start = input.LT(1);
int routineReturnType_StartIndex = input.index();
Object root_0 = null;
Token char_literal495=null;
ParserRuleReturnScope attributeList496 =null;
ParserRuleReturnScope returnType497 =null;
Object char_literal495_tree=null;
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_returnType=new RewriteRuleSubtreeStream(adaptor,"rule returnType");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 127) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:826:30: ( ':' ( attributeList )? returnType -> ^( TkRoutineReturn returnType ( attributeList )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:826:32: ':' ( attributeList )? returnType
{
char_literal495=(Token)match(input,COLON,FOLLOW_COLON_in_routineReturnType14277); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal495);
// au/com/integradev/delphi/antlr/Delphi.g:826:36: ( attributeList )?
int alt162=2;
int LA162_0 = input.LA(1);
if ( (LA162_0==PAREN_BRACKET_LEFT||LA162_0==SQUARE_BRACKET_LEFT) ) {
alt162=1;
}
switch (alt162) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:826:36: attributeList
{
pushFollow(FOLLOW_attributeList_in_routineReturnType14279);
attributeList496=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList496.getTree());
}
break;
}
pushFollow(FOLLOW_returnType_in_routineReturnType14282);
returnType497=returnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_returnType.add(returnType497.getTree());
// AST REWRITE
// elements: attributeList, returnType
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 826:62: -> ^( TkRoutineReturn returnType ( attributeList )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:826:65: ^( TkRoutineReturn returnType ( attributeList )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineReturnTypeNodeImpl(TkRoutineReturn), root_1);
adaptor.addChild(root_1, stream_returnType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:826:121: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 127, routineReturnType_StartIndex); }
}
return retval;
}
// $ANTLR end "routineReturnType"
public static class returnType_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "returnType"
// au/com/integradev/delphi/antlr/Delphi.g:828:1: returnType : typeReferenceOrString ;
public final DelphiParser.returnType_return returnType() throws RecognitionException {
DelphiParser.returnType_return retval = new DelphiParser.returnType_return();
retval.start = input.LT(1);
int returnType_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope typeReferenceOrString498 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 128) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:828:30: ( typeReferenceOrString )
// au/com/integradev/delphi/antlr/Delphi.g:828:32: typeReferenceOrString
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_typeReferenceOrString_in_returnType14351);
typeReferenceOrString498=typeReferenceOrString();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReferenceOrString498.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 128, returnType_StartIndex); }
}
return retval;
}
// $ANTLR end "returnType"
public static class routineParameters_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineParameters"
// au/com/integradev/delphi/antlr/Delphi.g:830:1: routineParameters : '(' ( formalParameterList )? ')' -> ^( TkRoutineParameters '(' ( formalParameterList )? ')' ) ;
public final DelphiParser.routineParameters_return routineParameters() throws RecognitionException {
DelphiParser.routineParameters_return retval = new DelphiParser.routineParameters_return();
retval.start = input.LT(1);
int routineParameters_StartIndex = input.index();
Object root_0 = null;
Token char_literal499=null;
Token char_literal501=null;
ParserRuleReturnScope formalParameterList500 =null;
Object char_literal499_tree=null;
Object char_literal501_tree=null;
RewriteRuleTokenStream stream_PAREN_RIGHT=new RewriteRuleTokenStream(adaptor,"token PAREN_RIGHT");
RewriteRuleTokenStream stream_PAREN_LEFT=new RewriteRuleTokenStream(adaptor,"token PAREN_LEFT");
RewriteRuleSubtreeStream stream_formalParameterList=new RewriteRuleSubtreeStream(adaptor,"rule formalParameterList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 129) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:830:30: ( '(' ( formalParameterList )? ')' -> ^( TkRoutineParameters '(' ( formalParameterList )? ')' ) )
// au/com/integradev/delphi/antlr/Delphi.g:830:32: '(' ( formalParameterList )? ')'
{
char_literal499=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_routineParameters14399); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_LEFT.add(char_literal499);
// au/com/integradev/delphi/antlr/Delphi.g:830:36: ( formalParameterList )?
int alt163=2;
int LA163_0 = input.LA(1);
if ( ((LA163_0 >= ABSOLUTE && LA163_0 <= ABSTRACT)||LA163_0==ALIGN||(LA163_0 >= ASSEMBLER && LA163_0 <= ASSEMBLY)||(LA163_0 >= AT && LA163_0 <= AUTOMATED)||LA163_0==CDECL||LA163_0==CONST||LA163_0==CONTAINS||(LA163_0 >= DEFAULT && LA163_0 <= DEPRECATED)||LA163_0==DISPID||LA163_0==DYNAMIC||(LA163_0 >= EXPERIMENTAL && LA163_0 <= EXPORT)||LA163_0==EXTERNAL||LA163_0==FAR||LA163_0==FINAL||LA163_0==FORWARD||LA163_0==HELPER||LA163_0==IMPLEMENTS||LA163_0==INDEX||LA163_0==LABEL||LA163_0==LOCAL||LA163_0==MESSAGE||(LA163_0 >= NAME && LA163_0 <= NEAR)||LA163_0==NODEFAULT||(LA163_0 >= ON && LA163_0 <= OPERATOR)||(LA163_0 >= OUT && LA163_0 <= OVERRIDE)||LA163_0==PACKAGE||LA163_0==PAREN_BRACKET_LEFT||(LA163_0 >= PASCAL && LA163_0 <= PLATFORM)||LA163_0==PRIVATE||(LA163_0 >= PROTECTED && LA163_0 <= PUBLISHED)||(LA163_0 >= READ && LA163_0 <= READONLY)||(LA163_0 >= REFERENCE && LA163_0 <= REINTRODUCE)||(LA163_0 >= REQUIRES && LA163_0 <= RESIDENT)||(LA163_0 >= SAFECALL && LA163_0 <= SEALED)||LA163_0==SQUARE_BRACKET_LEFT||(LA163_0 >= STATIC && LA163_0 <= STRICT)||LA163_0==TkIdentifier||LA163_0==UNSAFE||(LA163_0 >= VAR && LA163_0 <= VIRTUAL)||LA163_0==WINAPI||(LA163_0 >= WRITE && LA163_0 <= WRITEONLY)) ) {
alt163=1;
}
switch (alt163) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:830:36: formalParameterList
{
pushFollow(FOLLOW_formalParameterList_in_routineParameters14401);
formalParameterList500=formalParameterList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_formalParameterList.add(formalParameterList500.getTree());
}
break;
}
char_literal501=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_routineParameters14404); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_RIGHT.add(char_literal501);
// AST REWRITE
// elements: PAREN_RIGHT, formalParameterList, PAREN_LEFT
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 830:61: -> ^( TkRoutineParameters '(' ( formalParameterList )? ')' )
{
// au/com/integradev/delphi/antlr/Delphi.g:830:64: ^( TkRoutineParameters '(' ( formalParameterList )? ')' )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineParametersNodeImpl(TkRoutineParameters), root_1);
adaptor.addChild(root_1, stream_PAREN_LEFT.nextNode());
// au/com/integradev/delphi/antlr/Delphi.g:830:117: ( formalParameterList )?
if ( stream_formalParameterList.hasNext() ) {
adaptor.addChild(root_1, stream_formalParameterList.nextTree());
}
stream_formalParameterList.reset();
adaptor.addChild(root_1, stream_PAREN_RIGHT.nextNode());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 129, routineParameters_StartIndex); }
}
return retval;
}
// $ANTLR end "routineParameters"
public static class formalParameterList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "formalParameterList"
// au/com/integradev/delphi/antlr/Delphi.g:832:1: formalParameterList : formalParameter ( ';' formalParameter )* -> ^( TkFormalParameterList formalParameter ( formalParameter )* ) ;
public final DelphiParser.formalParameterList_return formalParameterList() throws RecognitionException {
DelphiParser.formalParameterList_return retval = new DelphiParser.formalParameterList_return();
retval.start = input.LT(1);
int formalParameterList_StartIndex = input.index();
Object root_0 = null;
Token char_literal503=null;
ParserRuleReturnScope formalParameter502 =null;
ParserRuleReturnScope formalParameter504 =null;
Object char_literal503_tree=null;
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleSubtreeStream stream_formalParameter=new RewriteRuleSubtreeStream(adaptor,"rule formalParameter");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 130) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:832:30: ( formalParameter ( ';' formalParameter )* -> ^( TkFormalParameterList formalParameter ( formalParameter )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:832:32: formalParameter ( ';' formalParameter )*
{
pushFollow(FOLLOW_formalParameter_in_formalParameterList14466);
formalParameter502=formalParameter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_formalParameter.add(formalParameter502.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:832:48: ( ';' formalParameter )*
loop164:
while (true) {
int alt164=2;
int LA164_0 = input.LA(1);
if ( (LA164_0==SEMICOLON) ) {
alt164=1;
}
switch (alt164) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:832:49: ';' formalParameter
{
char_literal503=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_formalParameterList14469); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal503);
pushFollow(FOLLOW_formalParameter_in_formalParameterList14471);
formalParameter504=formalParameter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_formalParameter.add(formalParameter504.getTree());
}
break;
default :
break loop164;
}
}
// AST REWRITE
// elements: formalParameter, formalParameter
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 832:71: -> ^( TkFormalParameterList formalParameter ( formalParameter )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:832:74: ^( TkFormalParameterList formalParameter ( formalParameter )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new FormalParameterListNodeImpl(TkFormalParameterList), root_1);
adaptor.addChild(root_1, stream_formalParameter.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:832:143: ( formalParameter )*
while ( stream_formalParameter.hasNext() ) {
adaptor.addChild(root_1, stream_formalParameter.nextTree());
}
stream_formalParameter.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 130, formalParameterList_StartIndex); }
}
return retval;
}
// $ANTLR end "formalParameterList"
public static class formalParameter_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "formalParameter"
// au/com/integradev/delphi/antlr/Delphi.g:834:1: formalParameter : (a1= attributeList )? ( paramSpecifier (a2= attributeList )? )? nameDeclarationList ( ':' parameterType )? ( '=' expression )? -> ^( TkFormalParameter nameDeclarationList ( parameterType )? ( paramSpecifier )? ( expression )? ( $a1)? ( $a2)? ) ;
public final DelphiParser.formalParameter_return formalParameter() throws RecognitionException {
DelphiParser.formalParameter_return retval = new DelphiParser.formalParameter_return();
retval.start = input.LT(1);
int formalParameter_StartIndex = input.index();
Object root_0 = null;
Token char_literal507=null;
Token char_literal509=null;
ParserRuleReturnScope a1 =null;
ParserRuleReturnScope a2 =null;
ParserRuleReturnScope paramSpecifier505 =null;
ParserRuleReturnScope nameDeclarationList506 =null;
ParserRuleReturnScope parameterType508 =null;
ParserRuleReturnScope expression510 =null;
Object char_literal507_tree=null;
Object char_literal509_tree=null;
RewriteRuleTokenStream stream_EQUAL=new RewriteRuleTokenStream(adaptor,"token EQUAL");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_parameterType=new RewriteRuleSubtreeStream(adaptor,"rule parameterType");
RewriteRuleSubtreeStream stream_nameDeclarationList=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclarationList");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_paramSpecifier=new RewriteRuleSubtreeStream(adaptor,"rule paramSpecifier");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 131) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:834:30: ( (a1= attributeList )? ( paramSpecifier (a2= attributeList )? )? nameDeclarationList ( ':' parameterType )? ( '=' expression )? -> ^( TkFormalParameter nameDeclarationList ( parameterType )? ( paramSpecifier )? ( expression )? ( $a1)? ( $a2)? ) )
// au/com/integradev/delphi/antlr/Delphi.g:834:32: (a1= attributeList )? ( paramSpecifier (a2= attributeList )? )? nameDeclarationList ( ':' parameterType )? ( '=' expression )?
{
// au/com/integradev/delphi/antlr/Delphi.g:834:34: (a1= attributeList )?
int alt165=2;
int LA165_0 = input.LA(1);
if ( (LA165_0==PAREN_BRACKET_LEFT||LA165_0==SQUARE_BRACKET_LEFT) ) {
alt165=1;
}
switch (alt165) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:834:34: a1= attributeList
{
pushFollow(FOLLOW_attributeList_in_formalParameter14539);
a1=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(a1.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:834:50: ( paramSpecifier (a2= attributeList )? )?
int alt167=2;
int LA167_0 = input.LA(1);
if ( (LA167_0==OUT) ) {
int LA167_1 = input.LA(2);
if ( ((LA167_1 >= ABSOLUTE && LA167_1 <= ABSTRACT)||LA167_1==ALIGN||(LA167_1 >= ASSEMBLER && LA167_1 <= ASSEMBLY)||(LA167_1 >= AT && LA167_1 <= AUTOMATED)||LA167_1==CDECL||LA167_1==CONTAINS||(LA167_1 >= DEFAULT && LA167_1 <= DEPRECATED)||LA167_1==DISPID||LA167_1==DYNAMIC||(LA167_1 >= EXPERIMENTAL && LA167_1 <= EXPORT)||LA167_1==EXTERNAL||LA167_1==FAR||LA167_1==FINAL||LA167_1==FORWARD||LA167_1==HELPER||LA167_1==IMPLEMENTS||LA167_1==INDEX||LA167_1==LABEL||LA167_1==LOCAL||LA167_1==MESSAGE||(LA167_1 >= NAME && LA167_1 <= NEAR)||LA167_1==NODEFAULT||(LA167_1 >= ON && LA167_1 <= OPERATOR)||(LA167_1 >= OUT && LA167_1 <= OVERRIDE)||LA167_1==PACKAGE||LA167_1==PAREN_BRACKET_LEFT||(LA167_1 >= PASCAL && LA167_1 <= PLATFORM)||LA167_1==PRIVATE||(LA167_1 >= PROTECTED && LA167_1 <= PUBLISHED)||(LA167_1 >= READ && LA167_1 <= READONLY)||(LA167_1 >= REFERENCE && LA167_1 <= REINTRODUCE)||(LA167_1 >= REQUIRES && LA167_1 <= RESIDENT)||(LA167_1 >= SAFECALL && LA167_1 <= SEALED)||LA167_1==SQUARE_BRACKET_LEFT||(LA167_1 >= STATIC && LA167_1 <= STRICT)||LA167_1==TkIdentifier||LA167_1==UNSAFE||(LA167_1 >= VARARGS && LA167_1 <= VIRTUAL)||LA167_1==WINAPI||(LA167_1 >= WRITE && LA167_1 <= WRITEONLY)) ) {
alt167=1;
}
}
else if ( (LA167_0==CONST||LA167_0==VAR) ) {
alt167=1;
}
switch (alt167) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:834:51: paramSpecifier (a2= attributeList )?
{
pushFollow(FOLLOW_paramSpecifier_in_formalParameter14543);
paramSpecifier505=paramSpecifier();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_paramSpecifier.add(paramSpecifier505.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:834:68: (a2= attributeList )?
int alt166=2;
int LA166_0 = input.LA(1);
if ( (LA166_0==PAREN_BRACKET_LEFT||LA166_0==SQUARE_BRACKET_LEFT) ) {
alt166=1;
}
switch (alt166) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:834:68: a2= attributeList
{
pushFollow(FOLLOW_attributeList_in_formalParameter14547);
a2=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(a2.getTree());
}
break;
}
}
break;
}
pushFollow(FOLLOW_nameDeclarationList_in_formalParameter14552);
nameDeclarationList506=nameDeclarationList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclarationList.add(nameDeclarationList506.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:834:106: ( ':' parameterType )?
int alt168=2;
int LA168_0 = input.LA(1);
if ( (LA168_0==COLON) ) {
alt168=1;
}
switch (alt168) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:834:107: ':' parameterType
{
char_literal507=(Token)match(input,COLON,FOLLOW_COLON_in_formalParameter14555); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal507);
pushFollow(FOLLOW_parameterType_in_formalParameter14557);
parameterType508=parameterType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_parameterType.add(parameterType508.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:834:127: ( '=' expression )?
int alt169=2;
int LA169_0 = input.LA(1);
if ( (LA169_0==EQUAL) ) {
alt169=1;
}
switch (alt169) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:834:128: '=' expression
{
char_literal509=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_formalParameter14562); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal509);
pushFollow(FOLLOW_expression_in_formalParameter14564);
expression510=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression510.getTree());
}
break;
}
// AST REWRITE
// elements: paramSpecifier, a1, nameDeclarationList, parameterType, a2, expression
// token labels:
// rule labels: a1, a2, retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_a1=new RewriteRuleSubtreeStream(adaptor,"rule a1",a1!=null?a1.getTree():null);
RewriteRuleSubtreeStream stream_a2=new RewriteRuleSubtreeStream(adaptor,"rule a2",a2!=null?a2.getTree():null);
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 835:30: -> ^( TkFormalParameter nameDeclarationList ( parameterType )? ( paramSpecifier )? ( expression )? ( $a1)? ( $a2)? )
{
// au/com/integradev/delphi/antlr/Delphi.g:835:33: ^( TkFormalParameter nameDeclarationList ( parameterType )? ( paramSpecifier )? ( expression )? ( $a1)? ( $a2)? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new FormalParameterNodeImpl(TkFormalParameter), root_1);
adaptor.addChild(root_1, stream_nameDeclarationList.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:835:98: ( parameterType )?
if ( stream_parameterType.hasNext() ) {
adaptor.addChild(root_1, stream_parameterType.nextTree());
}
stream_parameterType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:835:113: ( paramSpecifier )?
if ( stream_paramSpecifier.hasNext() ) {
adaptor.addChild(root_1, stream_paramSpecifier.nextTree());
}
stream_paramSpecifier.reset();
// au/com/integradev/delphi/antlr/Delphi.g:835:129: ( expression )?
if ( stream_expression.hasNext() ) {
adaptor.addChild(root_1, stream_expression.nextTree());
}
stream_expression.reset();
// au/com/integradev/delphi/antlr/Delphi.g:835:142: ( $a1)?
if ( stream_a1.hasNext() ) {
adaptor.addChild(root_1, stream_a1.nextTree());
}
stream_a1.reset();
// au/com/integradev/delphi/antlr/Delphi.g:835:147: ( $a2)?
if ( stream_a2.hasNext() ) {
adaptor.addChild(root_1, stream_a2.nextTree());
}
stream_a2.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 131, formalParameter_StartIndex); }
}
return retval;
}
// $ANTLR end "formalParameter"
public static class paramSpecifier_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "paramSpecifier"
// au/com/integradev/delphi/antlr/Delphi.g:837:1: paramSpecifier : ( CONST | VAR | OUT );
public final DelphiParser.paramSpecifier_return paramSpecifier() throws RecognitionException {
DelphiParser.paramSpecifier_return retval = new DelphiParser.paramSpecifier_return();
retval.start = input.LT(1);
int paramSpecifier_StartIndex = input.index();
Object root_0 = null;
Token set511=null;
Object set511_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 132) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:837:30: ( CONST | VAR | OUT )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set511=input.LT(1);
if ( input.LA(1)==CONST||input.LA(1)==OUT||input.LA(1)==VAR ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set511));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 132, paramSpecifier_StartIndex); }
}
return retval;
}
// $ANTLR end "paramSpecifier"
public static class routineBody_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineBody"
// au/com/integradev/delphi/antlr/Delphi.g:841:1: routineBody : block ';' -> ^( TkRoutineBody block ) ;
public final DelphiParser.routineBody_return routineBody() throws RecognitionException {
DelphiParser.routineBody_return retval = new DelphiParser.routineBody_return();
retval.start = input.LT(1);
int routineBody_StartIndex = input.index();
Object root_0 = null;
Token char_literal513=null;
ParserRuleReturnScope block512 =null;
Object char_literal513_tree=null;
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleSubtreeStream stream_block=new RewriteRuleSubtreeStream(adaptor,"rule block");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 133) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:841:30: ( block ';' -> ^( TkRoutineBody block ) )
// au/com/integradev/delphi/antlr/Delphi.g:841:32: block ';'
{
pushFollow(FOLLOW_block_in_routineBody14794);
block512=block();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_block.add(block512.getTree());
char_literal513=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_routineBody14796); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal513);
// AST REWRITE
// elements: block
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 841:42: -> ^( TkRoutineBody block )
{
// au/com/integradev/delphi/antlr/Delphi.g:841:45: ^( TkRoutineBody block )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RoutineBodyNodeImpl(TkRoutineBody), root_1);
adaptor.addChild(root_1, stream_block.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 133, routineBody_StartIndex); }
}
return retval;
}
// $ANTLR end "routineBody"
public static class attributeList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "attributeList"
// au/com/integradev/delphi/antlr/Delphi.g:847:1: attributeList : ( attributeGroup )+ -> ^( TkAttributeList ( attributeGroup )+ ) ;
public final DelphiParser.attributeList_return attributeList() throws RecognitionException {
DelphiParser.attributeList_return retval = new DelphiParser.attributeList_return();
retval.start = input.LT(1);
int attributeList_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope attributeGroup514 =null;
RewriteRuleSubtreeStream stream_attributeGroup=new RewriteRuleSubtreeStream(adaptor,"rule attributeGroup");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 134) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:847:30: ( ( attributeGroup )+ -> ^( TkAttributeList ( attributeGroup )+ ) )
// au/com/integradev/delphi/antlr/Delphi.g:847:32: ( attributeGroup )+
{
// au/com/integradev/delphi/antlr/Delphi.g:847:32: ( attributeGroup )+
int cnt170=0;
loop170:
while (true) {
int alt170=2;
int LA170_0 = input.LA(1);
if ( (LA170_0==PAREN_BRACKET_LEFT||LA170_0==SQUARE_BRACKET_LEFT) ) {
int LA170_2 = input.LA(2);
if ( (synpred242_Delphi()) ) {
alt170=1;
}
}
switch (alt170) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:847:32: attributeGroup
{
pushFollow(FOLLOW_attributeGroup_in_attributeList14863);
attributeGroup514=attributeGroup();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeGroup.add(attributeGroup514.getTree());
}
break;
default :
if ( cnt170 >= 1 ) break loop170;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(170, input);
throw eee;
}
cnt170++;
}
// AST REWRITE
// elements: attributeGroup
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 848:30: -> ^( TkAttributeList ( attributeGroup )+ )
{
// au/com/integradev/delphi/antlr/Delphi.g:848:33: ^( TkAttributeList ( attributeGroup )+ )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AttributeListNodeImpl(TkAttributeList), root_1);
if ( !(stream_attributeGroup.hasNext()) ) {
throw new RewriteEarlyExitException();
}
while ( stream_attributeGroup.hasNext() ) {
adaptor.addChild(root_1, stream_attributeGroup.nextTree());
}
stream_attributeGroup.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 134, attributeList_StartIndex); }
}
return retval;
}
// $ANTLR end "attributeList"
public static class attributeGroup_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "attributeGroup"
// au/com/integradev/delphi/antlr/Delphi.g:850:1: attributeGroup : lbrack ( attribute ( ',' )? )+ rbrack -> ^( TkAttributeGroup ( attribute )+ ) ;
public final DelphiParser.attributeGroup_return attributeGroup() throws RecognitionException {
DelphiParser.attributeGroup_return retval = new DelphiParser.attributeGroup_return();
retval.start = input.LT(1);
int attributeGroup_StartIndex = input.index();
Object root_0 = null;
Token char_literal517=null;
ParserRuleReturnScope lbrack515 =null;
ParserRuleReturnScope attribute516 =null;
ParserRuleReturnScope rbrack518 =null;
Object char_literal517_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleSubtreeStream stream_rbrack=new RewriteRuleSubtreeStream(adaptor,"rule rbrack");
RewriteRuleSubtreeStream stream_lbrack=new RewriteRuleSubtreeStream(adaptor,"rule lbrack");
RewriteRuleSubtreeStream stream_attribute=new RewriteRuleSubtreeStream(adaptor,"rule attribute");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 135) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:850:30: ( lbrack ( attribute ( ',' )? )+ rbrack -> ^( TkAttributeGroup ( attribute )+ ) )
// au/com/integradev/delphi/antlr/Delphi.g:850:32: lbrack ( attribute ( ',' )? )+ rbrack
{
pushFollow(FOLLOW_lbrack_in_attributeGroup14956);
lbrack515=lbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_lbrack.add(lbrack515.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:850:39: ( attribute ( ',' )? )+
int cnt172=0;
loop172:
while (true) {
int alt172=2;
int LA172_0 = input.LA(1);
if ( ((LA172_0 >= ABSOLUTE && LA172_0 <= ABSTRACT)||LA172_0==ALIGN||(LA172_0 >= ASSEMBLER && LA172_0 <= ASSEMBLY)||(LA172_0 >= AT && LA172_0 <= AUTOMATED)||LA172_0==CDECL||LA172_0==CONTAINS||(LA172_0 >= DEFAULT && LA172_0 <= DEPRECATED)||LA172_0==DISPID||LA172_0==DYNAMIC||(LA172_0 >= EXPERIMENTAL && LA172_0 <= EXPORT)||LA172_0==EXTERNAL||LA172_0==FAR||LA172_0==FINAL||LA172_0==FORWARD||LA172_0==HELPER||LA172_0==IMPLEMENTS||LA172_0==INDEX||LA172_0==LABEL||LA172_0==LOCAL||LA172_0==MESSAGE||(LA172_0 >= NAME && LA172_0 <= NEAR)||LA172_0==NODEFAULT||(LA172_0 >= ON && LA172_0 <= OPERATOR)||(LA172_0 >= OUT && LA172_0 <= OVERRIDE)||LA172_0==PACKAGE||(LA172_0 >= PASCAL && LA172_0 <= PLATFORM)||LA172_0==PRIVATE||(LA172_0 >= PROTECTED && LA172_0 <= PUBLISHED)||(LA172_0 >= READ && LA172_0 <= READONLY)||(LA172_0 >= REFERENCE && LA172_0 <= REINTRODUCE)||(LA172_0 >= REQUIRES && LA172_0 <= RESIDENT)||(LA172_0 >= SAFECALL && LA172_0 <= SEALED)||(LA172_0 >= STATIC && LA172_0 <= STRICT)||LA172_0==TkIdentifier||LA172_0==UNSAFE||(LA172_0 >= VARARGS && LA172_0 <= VIRTUAL)||LA172_0==WINAPI||(LA172_0 >= WRITE && LA172_0 <= WRITEONLY)) ) {
alt172=1;
}
switch (alt172) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:850:40: attribute ( ',' )?
{
pushFollow(FOLLOW_attribute_in_attributeGroup14959);
attribute516=attribute();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attribute.add(attribute516.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:850:50: ( ',' )?
int alt171=2;
int LA171_0 = input.LA(1);
if ( (LA171_0==COMMA) ) {
alt171=1;
}
switch (alt171) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:850:50: ','
{
char_literal517=(Token)match(input,COMMA,FOLLOW_COMMA_in_attributeGroup14961); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal517);
}
break;
}
}
break;
default :
if ( cnt172 >= 1 ) break loop172;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(172, input);
throw eee;
}
cnt172++;
}
pushFollow(FOLLOW_rbrack_in_attributeGroup14966);
rbrack518=rbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_rbrack.add(rbrack518.getTree());
// AST REWRITE
// elements: attribute
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 851:30: -> ^( TkAttributeGroup ( attribute )+ )
{
// au/com/integradev/delphi/antlr/Delphi.g:851:33: ^( TkAttributeGroup ( attribute )+ )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AttributeGroupNodeImpl(TkAttributeGroup), root_1);
if ( !(stream_attribute.hasNext()) ) {
throw new RewriteEarlyExitException();
}
while ( stream_attribute.hasNext() ) {
adaptor.addChild(root_1, stream_attribute.nextTree());
}
stream_attribute.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 135, attributeGroup_StartIndex); }
}
return retval;
}
// $ANTLR end "attributeGroup"
public static class attribute_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "attribute"
// au/com/integradev/delphi/antlr/Delphi.g:853:1: attribute : ( ASSEMBLY ':' )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )* -> ^( TkAttribute ( ASSEMBLY )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )* ) ;
public final DelphiParser.attribute_return attribute() throws RecognitionException {
DelphiParser.attribute_return retval = new DelphiParser.attribute_return();
retval.start = input.LT(1);
int attribute_StartIndex = input.index();
Object root_0 = null;
Token ASSEMBLY519=null;
Token char_literal520=null;
Token char_literal523=null;
ParserRuleReturnScope nameReference521 =null;
ParserRuleReturnScope argumentList522 =null;
ParserRuleReturnScope nameReference524 =null;
ParserRuleReturnScope argumentList525 =null;
Object ASSEMBLY519_tree=null;
Object char_literal520_tree=null;
Object char_literal523_tree=null;
RewriteRuleTokenStream stream_ASSEMBLY=new RewriteRuleTokenStream(adaptor,"token ASSEMBLY");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_argumentList=new RewriteRuleSubtreeStream(adaptor,"rule argumentList");
RewriteRuleSubtreeStream stream_nameReference=new RewriteRuleSubtreeStream(adaptor,"rule nameReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 136) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:853:30: ( ( ASSEMBLY ':' )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )* -> ^( TkAttribute ( ASSEMBLY )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:853:32: ( ASSEMBLY ':' )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )*
{
// au/com/integradev/delphi/antlr/Delphi.g:853:32: ( ASSEMBLY ':' )?
int alt173=2;
int LA173_0 = input.LA(1);
if ( (LA173_0==ASSEMBLY) ) {
int LA173_1 = input.LA(2);
if ( (LA173_1==COLON) ) {
int LA173_3 = input.LA(3);
if ( (LA173_3==TkIdentifier) ) {
int LA173_4 = input.LA(4);
if ( (synpred245_Delphi()) ) {
alt173=1;
}
}
else if ( ((LA173_3 >= ABSOLUTE && LA173_3 <= ABSTRACT)||LA173_3==ALIGN||(LA173_3 >= ASSEMBLER && LA173_3 <= ASSEMBLY)||(LA173_3 >= AT && LA173_3 <= AUTOMATED)||LA173_3==CDECL||LA173_3==CONTAINS||(LA173_3 >= DEFAULT && LA173_3 <= DEPRECATED)||LA173_3==DISPID||LA173_3==DYNAMIC||(LA173_3 >= EXPERIMENTAL && LA173_3 <= EXPORT)||LA173_3==EXTERNAL||LA173_3==FAR||LA173_3==FINAL||LA173_3==FORWARD||LA173_3==HELPER||LA173_3==IMPLEMENTS||LA173_3==INDEX||LA173_3==LABEL||LA173_3==LOCAL||LA173_3==MESSAGE||(LA173_3 >= NAME && LA173_3 <= NEAR)||LA173_3==NODEFAULT||(LA173_3 >= ON && LA173_3 <= OPERATOR)||(LA173_3 >= OUT && LA173_3 <= OVERRIDE)||LA173_3==PACKAGE||(LA173_3 >= PASCAL && LA173_3 <= PLATFORM)||LA173_3==PRIVATE||(LA173_3 >= PROTECTED && LA173_3 <= PUBLISHED)||(LA173_3 >= READ && LA173_3 <= READONLY)||(LA173_3 >= REFERENCE && LA173_3 <= REINTRODUCE)||(LA173_3 >= REQUIRES && LA173_3 <= RESIDENT)||(LA173_3 >= SAFECALL && LA173_3 <= SEALED)||(LA173_3 >= STATIC && LA173_3 <= STRICT)||LA173_3==UNSAFE||(LA173_3 >= VARARGS && LA173_3 <= VIRTUAL)||LA173_3==WINAPI||(LA173_3 >= WRITE && LA173_3 <= WRITEONLY)) ) {
int LA173_5 = input.LA(4);
if ( (synpred245_Delphi()) ) {
alt173=1;
}
}
}
}
switch (alt173) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:853:33: ASSEMBLY ':'
{
ASSEMBLY519=(Token)match(input,ASSEMBLY,FOLLOW_ASSEMBLY_in_attribute15064); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ASSEMBLY.add(ASSEMBLY519);
char_literal520=(Token)match(input,COLON,FOLLOW_COLON_in_attribute15066); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal520);
}
break;
}
pushFollow(FOLLOW_nameReference_in_attribute15070);
nameReference521=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameReference.add(nameReference521.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:853:62: ( argumentList )?
int alt174=2;
int LA174_0 = input.LA(1);
if ( (LA174_0==PAREN_LEFT) ) {
alt174=1;
}
switch (alt174) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:853:62: argumentList
{
pushFollow(FOLLOW_argumentList_in_attribute15072);
argumentList522=argumentList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_argumentList.add(argumentList522.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:853:76: ( ':' nameReference ( argumentList )? )*
loop176:
while (true) {
int alt176=2;
int LA176_0 = input.LA(1);
if ( (LA176_0==COLON) ) {
alt176=1;
}
switch (alt176) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:853:77: ':' nameReference ( argumentList )?
{
char_literal523=(Token)match(input,COLON,FOLLOW_COLON_in_attribute15076); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal523);
pushFollow(FOLLOW_nameReference_in_attribute15078);
nameReference524=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameReference.add(nameReference524.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:853:95: ( argumentList )?
int alt175=2;
int LA175_0 = input.LA(1);
if ( (LA175_0==PAREN_LEFT) ) {
alt175=1;
}
switch (alt175) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:853:95: argumentList
{
pushFollow(FOLLOW_argumentList_in_attribute15080);
argumentList525=argumentList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_argumentList.add(argumentList525.getTree());
}
break;
}
}
break;
default :
break loop176;
}
}
// AST REWRITE
// elements: argumentList, COLON, nameReference, ASSEMBLY, nameReference, argumentList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 854:30: -> ^( TkAttribute ( ASSEMBLY )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:854:33: ^( TkAttribute ( ASSEMBLY )? nameReference ( argumentList )? ( ':' nameReference ( argumentList )? )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AttributeNodeImpl(TkAttribute), root_1);
// au/com/integradev/delphi/antlr/Delphi.g:854:66: ( ASSEMBLY )?
if ( stream_ASSEMBLY.hasNext() ) {
adaptor.addChild(root_1, stream_ASSEMBLY.nextNode());
}
stream_ASSEMBLY.reset();
adaptor.addChild(root_1, stream_nameReference.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:854:90: ( argumentList )?
if ( stream_argumentList.hasNext() ) {
adaptor.addChild(root_1, stream_argumentList.nextTree());
}
stream_argumentList.reset();
// au/com/integradev/delphi/antlr/Delphi.g:854:104: ( ':' nameReference ( argumentList )? )*
while ( stream_COLON.hasNext()||stream_nameReference.hasNext() ) {
adaptor.addChild(root_1, stream_COLON.nextNode());
adaptor.addChild(root_1, stream_nameReference.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:854:123: ( argumentList )?
if ( stream_argumentList.hasNext() ) {
adaptor.addChild(root_1, stream_argumentList.nextTree());
}
stream_argumentList.reset();
}
stream_COLON.reset();
stream_nameReference.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 136, attribute_StartIndex); }
}
return retval;
}
// $ANTLR end "attribute"
public static class expression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "expression"
// au/com/integradev/delphi/antlr/Delphi.g:860:1: expression : ( relationalExpression | anonymousMethod );
public final DelphiParser.expression_return expression() throws RecognitionException {
DelphiParser.expression_return retval = new DelphiParser.expression_return();
retval.start = input.LT(1);
int expression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope relationalExpression526 =null;
ParserRuleReturnScope anonymousMethod527 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 137) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:860:30: ( relationalExpression | anonymousMethod )
int alt177=2;
int LA177_0 = input.LA(1);
if ( ((LA177_0 >= ABSOLUTE && LA177_0 <= ALIGN)||(LA177_0 >= ASSEMBLER && LA177_0 <= ASSEMBLY)||(LA177_0 >= AT && LA177_0 <= AUTOMATED)||LA177_0==CDECL||LA177_0==CONTAINS||(LA177_0 >= DEFAULT && LA177_0 <= DEREFERENCE)||LA177_0==DISPID||LA177_0==DYNAMIC||(LA177_0 >= EXPERIMENTAL && LA177_0 <= EXPORT)||LA177_0==EXTERNAL||(LA177_0 >= FAR && LA177_0 <= FINAL)||LA177_0==FORWARD||LA177_0==HELPER||LA177_0==IMPLEMENTS||(LA177_0 >= INDEX && LA177_0 <= INHERITED)||LA177_0==LABEL||LA177_0==LOCAL||(LA177_0 >= MESSAGE && LA177_0 <= MINUS)||(LA177_0 >= NAME && LA177_0 <= NOT)||(LA177_0 >= ON && LA177_0 <= OPERATOR)||(LA177_0 >= OUT && LA177_0 <= OVERRIDE)||LA177_0==PACKAGE||LA177_0==PAREN_BRACKET_LEFT||LA177_0==PAREN_LEFT||(LA177_0 >= PASCAL && LA177_0 <= PRIVATE)||(LA177_0 >= PROTECTED && LA177_0 <= PUBLISHED)||(LA177_0 >= READ && LA177_0 <= READONLY)||(LA177_0 >= REFERENCE && LA177_0 <= REINTRODUCE)||(LA177_0 >= REQUIRES && LA177_0 <= RESIDENT)||(LA177_0 >= SAFECALL && LA177_0 <= SEALED)||LA177_0==SQUARE_BRACKET_LEFT||(LA177_0 >= STATIC && LA177_0 <= STRING)||LA177_0==TkBinaryNumber||LA177_0==TkCharacterEscapeCode||(LA177_0 >= TkHexNumber && LA177_0 <= TkIntNumber)||LA177_0==TkMultilineString||(LA177_0 >= TkQuotedString && LA177_0 <= TkRealNumber)||LA177_0==UNSAFE||(LA177_0 >= VARARGS && LA177_0 <= VIRTUAL)||LA177_0==WINAPI||(LA177_0 >= WRITE && LA177_0 <= WRITEONLY)) ) {
alt177=1;
}
else if ( (LA177_0==FUNCTION||LA177_0==PROCEDURE) ) {
alt177=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 177, 0, input);
throw nvae;
}
switch (alt177) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:860:32: relationalExpression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_relationalExpression_in_expression15198);
relationalExpression526=relationalExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, relationalExpression526.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:861:32: anonymousMethod
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_anonymousMethod_in_expression15231);
anonymousMethod527=anonymousMethod();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, anonymousMethod527.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 137, expression_StartIndex); }
}
return retval;
}
// $ANTLR end "expression"
public static class relationalExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "relationalExpression"
// au/com/integradev/delphi/antlr/Delphi.g:868:1: relationalExpression : additiveExpression ( relationalOperator ^ additiveExpression )* ;
public final DelphiParser.relationalExpression_return relationalExpression() throws RecognitionException {
DelphiParser.relationalExpression_return retval = new DelphiParser.relationalExpression_return();
retval.start = input.LT(1);
int relationalExpression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope additiveExpression528 =null;
ParserRuleReturnScope relationalOperator529 =null;
ParserRuleReturnScope additiveExpression530 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 138) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:868:30: ( additiveExpression ( relationalOperator ^ additiveExpression )* )
// au/com/integradev/delphi/antlr/Delphi.g:868:32: additiveExpression ( relationalOperator ^ additiveExpression )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_additiveExpression_in_relationalExpression15281);
additiveExpression528=additiveExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, additiveExpression528.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:868:51: ( relationalOperator ^ additiveExpression )*
loop178:
while (true) {
int alt178=2;
alt178 = dfa178.predict(input);
switch (alt178) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:868:52: relationalOperator ^ additiveExpression
{
pushFollow(FOLLOW_relationalOperator_in_relationalExpression15284);
relationalOperator529=relationalOperator();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(relationalOperator529.getTree(), root_0);
pushFollow(FOLLOW_additiveExpression_in_relationalExpression15287);
additiveExpression530=additiveExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, additiveExpression530.getTree());
}
break;
default :
break loop178;
}
}
if ( state.backtracking==0 ) { resetBinaryExpressionTokens(root_0); }
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 138, relationalExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "relationalExpression"
public static class additiveExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "additiveExpression"
// au/com/integradev/delphi/antlr/Delphi.g:870:1: additiveExpression : multiplicativeExpression ( addOperator ^ multiplicativeExpression )* ;
public final DelphiParser.additiveExpression_return additiveExpression() throws RecognitionException {
DelphiParser.additiveExpression_return retval = new DelphiParser.additiveExpression_return();
retval.start = input.LT(1);
int additiveExpression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope multiplicativeExpression531 =null;
ParserRuleReturnScope addOperator532 =null;
ParserRuleReturnScope multiplicativeExpression533 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 139) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:870:30: ( multiplicativeExpression ( addOperator ^ multiplicativeExpression )* )
// au/com/integradev/delphi/antlr/Delphi.g:870:32: multiplicativeExpression ( addOperator ^ multiplicativeExpression )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_multiplicativeExpression_in_additiveExpression15338);
multiplicativeExpression531=multiplicativeExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, multiplicativeExpression531.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:870:57: ( addOperator ^ multiplicativeExpression )*
loop179:
while (true) {
int alt179=2;
alt179 = dfa179.predict(input);
switch (alt179) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:870:58: addOperator ^ multiplicativeExpression
{
pushFollow(FOLLOW_addOperator_in_additiveExpression15341);
addOperator532=addOperator();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(addOperator532.getTree(), root_0);
pushFollow(FOLLOW_multiplicativeExpression_in_additiveExpression15344);
multiplicativeExpression533=multiplicativeExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, multiplicativeExpression533.getTree());
}
break;
default :
break loop179;
}
}
if ( state.backtracking==0 ) { resetBinaryExpressionTokens(root_0); }
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 139, additiveExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "additiveExpression"
public static class multiplicativeExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "multiplicativeExpression"
// au/com/integradev/delphi/antlr/Delphi.g:872:1: multiplicativeExpression : unaryExpression ( multOperator ^ unaryExpression )* ;
public final DelphiParser.multiplicativeExpression_return multiplicativeExpression() throws RecognitionException {
DelphiParser.multiplicativeExpression_return retval = new DelphiParser.multiplicativeExpression_return();
retval.start = input.LT(1);
int multiplicativeExpression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope unaryExpression534 =null;
ParserRuleReturnScope multOperator535 =null;
ParserRuleReturnScope unaryExpression536 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 140) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:872:30: ( unaryExpression ( multOperator ^ unaryExpression )* )
// au/com/integradev/delphi/antlr/Delphi.g:872:32: unaryExpression ( multOperator ^ unaryExpression )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unaryExpression_in_multiplicativeExpression15389);
unaryExpression534=unaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unaryExpression534.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:872:48: ( multOperator ^ unaryExpression )*
loop180:
while (true) {
int alt180=2;
int LA180_0 = input.LA(1);
if ( (LA180_0==AND||LA180_0==AS||(LA180_0 >= DIV && LA180_0 <= DIVIDE)||(LA180_0 >= MOD && LA180_0 <= MULTIPLY)||(LA180_0 >= SHL && LA180_0 <= SHR)) ) {
alt180=1;
}
switch (alt180) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:872:49: multOperator ^ unaryExpression
{
pushFollow(FOLLOW_multOperator_in_multiplicativeExpression15392);
multOperator535=multOperator();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(multOperator535.getTree(), root_0);
pushFollow(FOLLOW_unaryExpression_in_multiplicativeExpression15395);
unaryExpression536=unaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unaryExpression536.getTree());
}
break;
default :
break loop180;
}
}
if ( state.backtracking==0 ) { resetBinaryExpressionTokens(root_0); }
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 140, multiplicativeExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "multiplicativeExpression"
public static class unaryExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unaryExpression"
// au/com/integradev/delphi/antlr/Delphi.g:874:1: unaryExpression : ( unaryOperator ^ unaryExpression | primaryExpression );
public final DelphiParser.unaryExpression_return unaryExpression() throws RecognitionException {
DelphiParser.unaryExpression_return retval = new DelphiParser.unaryExpression_return();
retval.start = input.LT(1);
int unaryExpression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope unaryOperator537 =null;
ParserRuleReturnScope unaryExpression538 =null;
ParserRuleReturnScope primaryExpression539 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 141) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:874:30: ( unaryOperator ^ unaryExpression | primaryExpression )
int alt181=2;
int LA181_0 = input.LA(1);
if ( (LA181_0==ADDRESS||LA181_0==MINUS||LA181_0==NOT||LA181_0==PLUS) ) {
alt181=1;
}
else if ( ((LA181_0 >= ABSOLUTE && LA181_0 <= ABSTRACT)||LA181_0==ALIGN||(LA181_0 >= ASSEMBLER && LA181_0 <= ASSEMBLY)||(LA181_0 >= AT && LA181_0 <= AUTOMATED)||LA181_0==CDECL||LA181_0==CONTAINS||(LA181_0 >= DEFAULT && LA181_0 <= DEREFERENCE)||LA181_0==DISPID||LA181_0==DYNAMIC||(LA181_0 >= EXPERIMENTAL && LA181_0 <= EXPORT)||LA181_0==EXTERNAL||(LA181_0 >= FAR && LA181_0 <= FINAL)||LA181_0==FORWARD||LA181_0==HELPER||LA181_0==IMPLEMENTS||(LA181_0 >= INDEX && LA181_0 <= INHERITED)||LA181_0==LABEL||LA181_0==LOCAL||LA181_0==MESSAGE||(LA181_0 >= NAME && LA181_0 <= NODEFAULT)||(LA181_0 >= ON && LA181_0 <= OPERATOR)||(LA181_0 >= OUT && LA181_0 <= OVERRIDE)||LA181_0==PACKAGE||LA181_0==PAREN_BRACKET_LEFT||LA181_0==PAREN_LEFT||(LA181_0 >= PASCAL && LA181_0 <= PLATFORM)||LA181_0==PRIVATE||(LA181_0 >= PROTECTED && LA181_0 <= PUBLISHED)||(LA181_0 >= READ && LA181_0 <= READONLY)||(LA181_0 >= REFERENCE && LA181_0 <= REINTRODUCE)||(LA181_0 >= REQUIRES && LA181_0 <= RESIDENT)||(LA181_0 >= SAFECALL && LA181_0 <= SEALED)||LA181_0==SQUARE_BRACKET_LEFT||(LA181_0 >= STATIC && LA181_0 <= STRING)||LA181_0==TkBinaryNumber||LA181_0==TkCharacterEscapeCode||(LA181_0 >= TkHexNumber && LA181_0 <= TkIntNumber)||LA181_0==TkMultilineString||(LA181_0 >= TkQuotedString && LA181_0 <= TkRealNumber)||LA181_0==UNSAFE||(LA181_0 >= VARARGS && LA181_0 <= VIRTUAL)||LA181_0==WINAPI||(LA181_0 >= WRITE && LA181_0 <= WRITEONLY)) ) {
alt181=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 181, 0, input);
throw nvae;
}
switch (alt181) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:874:32: unaryOperator ^ unaryExpression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_unaryOperator_in_unaryExpression15449);
unaryOperator537=unaryOperator();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (Object)adaptor.becomeRoot(unaryOperator537.getTree(), root_0);
pushFollow(FOLLOW_unaryExpression_in_unaryExpression15452);
unaryExpression538=unaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unaryExpression538.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:875:32: primaryExpression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_primaryExpression_in_unaryExpression15485);
primaryExpression539=primaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, primaryExpression539.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 141, unaryExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "unaryExpression"
public static class primaryExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "primaryExpression"
// au/com/integradev/delphi/antlr/Delphi.g:877:1: primaryExpression : ( atom -> ^( TkPrimaryExpression atom ) | parenthesizedExpression | INHERITED ( ( nameReference )? ( particleItem )* )? -> ^( TkPrimaryExpression INHERITED ( ( nameReference )? ( particleItem )* )? ) );
public final DelphiParser.primaryExpression_return primaryExpression() throws RecognitionException {
DelphiParser.primaryExpression_return retval = new DelphiParser.primaryExpression_return();
retval.start = input.LT(1);
int primaryExpression_StartIndex = input.index();
Object root_0 = null;
Token INHERITED542=null;
ParserRuleReturnScope atom540 =null;
ParserRuleReturnScope parenthesizedExpression541 =null;
ParserRuleReturnScope nameReference543 =null;
ParserRuleReturnScope particleItem544 =null;
Object INHERITED542_tree=null;
RewriteRuleTokenStream stream_INHERITED=new RewriteRuleTokenStream(adaptor,"token INHERITED");
RewriteRuleSubtreeStream stream_particleItem=new RewriteRuleSubtreeStream(adaptor,"rule particleItem");
RewriteRuleSubtreeStream stream_nameReference=new RewriteRuleSubtreeStream(adaptor,"rule nameReference");
RewriteRuleSubtreeStream stream_atom=new RewriteRuleSubtreeStream(adaptor,"rule atom");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 142) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:877:30: ( atom -> ^( TkPrimaryExpression atom ) | parenthesizedExpression | INHERITED ( ( nameReference )? ( particleItem )* )? -> ^( TkPrimaryExpression INHERITED ( ( nameReference )? ( particleItem )* )? ) )
int alt185=3;
switch ( input.LA(1) ) {
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DEREFERENCE:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FILE:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NIL:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PAREN_BRACKET_LEFT:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case SQUARE_BRACKET_LEFT:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case STRING:
case TkBinaryNumber:
case TkCharacterEscapeCode:
case TkHexNumber:
case TkIdentifier:
case TkIntNumber:
case TkMultilineString:
case TkQuotedString:
case TkRealNumber:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt185=1;
}
break;
case PAREN_LEFT:
{
int LA185_15 = input.LA(2);
if ( (synpred254_Delphi()) ) {
alt185=1;
}
else if ( (synpred255_Delphi()) ) {
alt185=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 185, 15, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case INHERITED:
{
alt185=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 185, 0, input);
throw nvae;
}
switch (alt185) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:877:32: atom
{
pushFollow(FOLLOW_atom_in_primaryExpression15533);
atom540=atom();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_atom.add(atom540.getTree());
// AST REWRITE
// elements: atom
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 877:37: -> ^( TkPrimaryExpression atom )
{
// au/com/integradev/delphi/antlr/Delphi.g:877:40: ^( TkPrimaryExpression atom )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new PrimaryExpressionNodeImpl(TkPrimaryExpression), root_1);
adaptor.addChild(root_1, stream_atom.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:878:32: parenthesizedExpression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_parenthesizedExpression_in_primaryExpression15577);
parenthesizedExpression541=parenthesizedExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, parenthesizedExpression541.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:879:32: INHERITED ( ( nameReference )? ( particleItem )* )?
{
INHERITED542=(Token)match(input,INHERITED,FOLLOW_INHERITED_in_primaryExpression15610); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_INHERITED.add(INHERITED542);
// au/com/integradev/delphi/antlr/Delphi.g:879:42: ( ( nameReference )? ( particleItem )* )?
int alt184=2;
alt184 = dfa184.predict(input);
switch (alt184) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:879:43: ( nameReference )? ( particleItem )*
{
// au/com/integradev/delphi/antlr/Delphi.g:879:43: ( nameReference )?
int alt182=2;
switch ( input.LA(1) ) {
case TkIdentifier:
{
int LA182_1 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case DEFAULT:
{
int LA182_2 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case READ:
{
int LA182_4 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case WRITE:
{
int LA182_5 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case READONLY:
{
int LA182_6 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case WRITEONLY:
{
int LA182_7 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case DISPID:
{
int LA182_8 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case IMPLEMENTS:
{
int LA182_9 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case INDEX:
{
int LA182_10 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case STORED:
{
int LA182_11 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case NODEFAULT:
{
int LA182_12 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case DEPRECATED:
{
int LA182_13 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case EXPERIMENTAL:
{
int LA182_14 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case PLATFORM:
{
int LA182_15 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case NAME:
{
int LA182_16 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case RESIDENT:
{
int LA182_17 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case ABSOLUTE:
{
int LA182_18 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case REFERENCE:
{
int LA182_19 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case STRICT:
{
int LA182_20 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case PROTECTED:
{
int LA182_21 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case PRIVATE:
{
int LA182_22 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case PUBLIC:
{
int LA182_23 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case PUBLISHED:
{
int LA182_24 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case AUTOMATED:
{
int LA182_25 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case OPERATOR:
{
int LA182_26 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case ON:
{
int LA182_27 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case AT:
{
int LA182_28 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case OVERLOAD:
{
int LA182_29 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case REINTRODUCE:
{
int LA182_30 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case MESSAGE:
{
int LA182_31 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case STATIC:
{
int LA182_32 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case DYNAMIC:
{
int LA182_33 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case OVERRIDE:
{
int LA182_34 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case VIRTUAL:
{
int LA182_35 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case ABSTRACT:
case FINAL:
{
int LA182_36 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case ASSEMBLER:
{
int LA182_37 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case CDECL:
case EXPORT:
case PASCAL:
case REGISTER:
case SAFECALL:
case STDCALL:
case WINAPI:
{
int LA182_38 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case FAR:
case LOCAL:
case NEAR:
{
int LA182_39 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case VARARGS:
{
int LA182_40 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case UNSAFE:
{
int LA182_41 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case LABEL:
{
int LA182_42 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case EXTERNAL:
{
int LA182_43 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case FORWARD:
{
int LA182_44 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case DELAYED:
{
int LA182_45 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
case ALIGN:
case ASSEMBLY:
case CONTAINS:
case HELPER:
case OUT:
case PACKAGE:
case REQUIRES:
case SEALED:
{
int LA182_46 = input.LA(2);
if ( (synpred256_Delphi()) ) {
alt182=1;
}
}
break;
}
switch (alt182) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:879:43: nameReference
{
pushFollow(FOLLOW_nameReference_in_primaryExpression15613);
nameReference543=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameReference.add(nameReference543.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:879:58: ( particleItem )*
loop183:
while (true) {
int alt183=2;
alt183 = dfa183.predict(input);
switch (alt183) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:879:58: particleItem
{
pushFollow(FOLLOW_particleItem_in_primaryExpression15616);
particleItem544=particleItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_particleItem.add(particleItem544.getTree());
}
break;
default :
break loop183;
}
}
}
break;
}
// AST REWRITE
// elements: nameReference, particleItem, INHERITED
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 879:74: -> ^( TkPrimaryExpression INHERITED ( ( nameReference )? ( particleItem )* )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:879:77: ^( TkPrimaryExpression INHERITED ( ( nameReference )? ( particleItem )* )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new PrimaryExpressionNodeImpl(TkPrimaryExpression), root_1);
adaptor.addChild(root_1, stream_INHERITED.nextNode());
// au/com/integradev/delphi/antlr/Delphi.g:879:136: ( ( nameReference )? ( particleItem )* )?
if ( stream_nameReference.hasNext()||stream_particleItem.hasNext() ) {
// au/com/integradev/delphi/antlr/Delphi.g:879:137: ( nameReference )?
if ( stream_nameReference.hasNext() ) {
adaptor.addChild(root_1, stream_nameReference.nextTree());
}
stream_nameReference.reset();
// au/com/integradev/delphi/antlr/Delphi.g:879:152: ( particleItem )*
while ( stream_particleItem.hasNext() ) {
adaptor.addChild(root_1, stream_particleItem.nextTree());
}
stream_particleItem.reset();
}
stream_nameReference.reset();
stream_particleItem.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 142, primaryExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "primaryExpression"
public static class parenthesizedExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "parenthesizedExpression"
// au/com/integradev/delphi/antlr/Delphi.g:881:1: parenthesizedExpression : '(' expression ')' -> ^( TkNestedExpression '(' expression ')' ) ;
public final DelphiParser.parenthesizedExpression_return parenthesizedExpression() throws RecognitionException {
DelphiParser.parenthesizedExpression_return retval = new DelphiParser.parenthesizedExpression_return();
retval.start = input.LT(1);
int parenthesizedExpression_StartIndex = input.index();
Object root_0 = null;
Token char_literal545=null;
Token char_literal547=null;
ParserRuleReturnScope expression546 =null;
Object char_literal545_tree=null;
Object char_literal547_tree=null;
RewriteRuleTokenStream stream_PAREN_RIGHT=new RewriteRuleTokenStream(adaptor,"token PAREN_RIGHT");
RewriteRuleTokenStream stream_PAREN_LEFT=new RewriteRuleTokenStream(adaptor,"token PAREN_LEFT");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 143) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:881:30: ( '(' expression ')' -> ^( TkNestedExpression '(' expression ')' ) )
// au/com/integradev/delphi/antlr/Delphi.g:881:32: '(' expression ')'
{
char_literal545=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_parenthesizedExpression15681); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_LEFT.add(char_literal545);
pushFollow(FOLLOW_expression_in_parenthesizedExpression15683);
expression546=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression546.getTree());
char_literal547=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_parenthesizedExpression15685); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PAREN_RIGHT.add(char_literal547);
// AST REWRITE
// elements: PAREN_RIGHT, PAREN_LEFT, expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 881:51: -> ^( TkNestedExpression '(' expression ')' )
{
// au/com/integradev/delphi/antlr/Delphi.g:881:54: ^( TkNestedExpression '(' expression ')' )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ParenthesizedExpressionNodeImpl(TkNestedExpression), root_1);
adaptor.addChild(root_1, stream_PAREN_LEFT.nextNode());
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_1, stream_PAREN_RIGHT.nextNode());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 143, parenthesizedExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "parenthesizedExpression"
public static class atom_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "atom"
// au/com/integradev/delphi/antlr/Delphi.g:883:1: atom : particle ( particleItem )* ;
public final DelphiParser.atom_return atom() throws RecognitionException {
DelphiParser.atom_return retval = new DelphiParser.atom_return();
retval.start = input.LT(1);
int atom_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope particle548 =null;
ParserRuleReturnScope particleItem549 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 144) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:883:30: ( particle ( particleItem )* )
// au/com/integradev/delphi/antlr/Delphi.g:883:32: particle ( particleItem )*
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_particle_in_atom15761);
particle548=particle();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, particle548.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:883:41: ( particleItem )*
loop186:
while (true) {
int alt186=2;
alt186 = dfa186.predict(input);
switch (alt186) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:883:41: particleItem
{
pushFollow(FOLLOW_particleItem_in_atom15763);
particleItem549=particleItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, particleItem549.getTree());
}
break;
default :
break loop186;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 144, atom_StartIndex); }
}
return retval;
}
// $ANTLR end "atom"
public static class particle_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "particle"
// au/com/integradev/delphi/antlr/Delphi.g:885:1: particle : ( intNum | realNum | textLiteral | nilLiteral | nameReference | arrayConstructor | STRING | FILE | parenthesizedExpression ( particleItem )+ );
public final DelphiParser.particle_return particle() throws RecognitionException {
DelphiParser.particle_return retval = new DelphiParser.particle_return();
retval.start = input.LT(1);
int particle_StartIndex = input.index();
Object root_0 = null;
Token STRING556=null;
Token FILE557=null;
ParserRuleReturnScope intNum550 =null;
ParserRuleReturnScope realNum551 =null;
ParserRuleReturnScope textLiteral552 =null;
ParserRuleReturnScope nilLiteral553 =null;
ParserRuleReturnScope nameReference554 =null;
ParserRuleReturnScope arrayConstructor555 =null;
ParserRuleReturnScope parenthesizedExpression558 =null;
ParserRuleReturnScope particleItem559 =null;
Object STRING556_tree=null;
Object FILE557_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 145) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:885:30: ( intNum | realNum | textLiteral | nilLiteral | nameReference | arrayConstructor | STRING | FILE | parenthesizedExpression ( particleItem )+ )
int alt188=9;
switch ( input.LA(1) ) {
case TkBinaryNumber:
case TkHexNumber:
case TkIntNumber:
{
alt188=1;
}
break;
case TkRealNumber:
{
alt188=2;
}
break;
case DEREFERENCE:
case TkCharacterEscapeCode:
case TkMultilineString:
case TkQuotedString:
{
alt188=3;
}
break;
case NIL:
{
alt188=4;
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt188=5;
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
alt188=6;
}
break;
case STRING:
{
alt188=7;
}
break;
case FILE:
{
alt188=8;
}
break;
case PAREN_LEFT:
{
alt188=9;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 188, 0, input);
throw nvae;
}
switch (alt188) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:885:32: intNum
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_intNum_in_particle15821);
intNum550=intNum();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, intNum550.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:886:32: realNum
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_realNum_in_particle15854);
realNum551=realNum();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, realNum551.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:887:32: textLiteral
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_textLiteral_in_particle15887);
textLiteral552=textLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, textLiteral552.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:888:32: nilLiteral
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_nilLiteral_in_particle15920);
nilLiteral553=nilLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, nilLiteral553.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:889:32: nameReference
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_nameReference_in_particle15953);
nameReference554=nameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, nameReference554.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:890:32: arrayConstructor
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_arrayConstructor_in_particle15986);
arrayConstructor555=arrayConstructor();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayConstructor555.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:891:32: STRING
{
root_0 = (Object)adaptor.nil();
STRING556=(Token)match(input,STRING,FOLLOW_STRING_in_particle16019); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STRING556_tree = (Object)adaptor.create(STRING556);
adaptor.addChild(root_0, STRING556_tree);
}
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:892:32: FILE
{
root_0 = (Object)adaptor.nil();
FILE557=(Token)match(input,FILE,FOLLOW_FILE_in_particle16052); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FILE557_tree = (Object)adaptor.create(FILE557);
adaptor.addChild(root_0, FILE557_tree);
}
}
break;
case 9 :
// au/com/integradev/delphi/antlr/Delphi.g:893:32: parenthesizedExpression ( particleItem )+
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_parenthesizedExpression_in_particle16085);
parenthesizedExpression558=parenthesizedExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, parenthesizedExpression558.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:893:56: ( particleItem )+
int cnt187=0;
loop187:
while (true) {
int alt187=2;
alt187 = dfa187.predict(input);
switch (alt187) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:893:56: particleItem
{
pushFollow(FOLLOW_particleItem_in_particle16087);
particleItem559=particleItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, particleItem559.getTree());
}
break;
default :
if ( cnt187 >= 1 ) break loop187;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(187, input);
throw eee;
}
cnt187++;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 145, particle_StartIndex); }
}
return retval;
}
// $ANTLR end "particle"
public static class particleItem_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "particleItem"
// au/com/integradev/delphi/antlr/Delphi.g:897:1: particleItem : ( '.' extendedNameReference | argumentList | arrayAccessor | '^' );
public final DelphiParser.particleItem_return particleItem() throws RecognitionException {
DelphiParser.particleItem_return retval = new DelphiParser.particleItem_return();
retval.start = input.LT(1);
int particleItem_StartIndex = input.index();
Object root_0 = null;
Token char_literal560=null;
Token char_literal564=null;
ParserRuleReturnScope extendedNameReference561 =null;
ParserRuleReturnScope argumentList562 =null;
ParserRuleReturnScope arrayAccessor563 =null;
Object char_literal560_tree=null;
Object char_literal564_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 146) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:897:30: ( '.' extendedNameReference | argumentList | arrayAccessor | '^' )
int alt189=4;
switch ( input.LA(1) ) {
case DOT:
{
alt189=1;
}
break;
case PAREN_LEFT:
{
alt189=2;
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
alt189=3;
}
break;
case DEREFERENCE:
{
alt189=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 189, 0, input);
throw nvae;
}
switch (alt189) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:897:32: '.' extendedNameReference
{
root_0 = (Object)adaptor.nil();
char_literal560=(Token)match(input,DOT,FOLLOW_DOT_in_particleItem16206); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal560_tree = (Object)adaptor.create(char_literal560);
adaptor.addChild(root_0, char_literal560_tree);
}
pushFollow(FOLLOW_extendedNameReference_in_particleItem16208);
extendedNameReference561=extendedNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, extendedNameReference561.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:898:32: argumentList
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_argumentList_in_particleItem16241);
argumentList562=argumentList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, argumentList562.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:899:32: arrayAccessor
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_arrayAccessor_in_particleItem16274);
arrayAccessor563=arrayAccessor();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayAccessor563.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:900:32: '^'
{
root_0 = (Object)adaptor.nil();
char_literal564=(Token)match(input,DEREFERENCE,FOLLOW_DEREFERENCE_in_particleItem16307); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal564_tree = (Object)adaptor.create(char_literal564);
adaptor.addChild(root_0, char_literal564_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 146, particleItem_StartIndex); }
}
return retval;
}
// $ANTLR end "particleItem"
public static class arrayAccessor_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayAccessor"
// au/com/integradev/delphi/antlr/Delphi.g:902:1: arrayAccessor : lbrack expressionList rbrack -> ^( TkArrayAccessorNode expressionList ) ;
public final DelphiParser.arrayAccessor_return arrayAccessor() throws RecognitionException {
DelphiParser.arrayAccessor_return retval = new DelphiParser.arrayAccessor_return();
retval.start = input.LT(1);
int arrayAccessor_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope lbrack565 =null;
ParserRuleReturnScope expressionList566 =null;
ParserRuleReturnScope rbrack567 =null;
RewriteRuleSubtreeStream stream_expressionList=new RewriteRuleSubtreeStream(adaptor,"rule expressionList");
RewriteRuleSubtreeStream stream_rbrack=new RewriteRuleSubtreeStream(adaptor,"rule rbrack");
RewriteRuleSubtreeStream stream_lbrack=new RewriteRuleSubtreeStream(adaptor,"rule lbrack");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 147) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:902:30: ( lbrack expressionList rbrack -> ^( TkArrayAccessorNode expressionList ) )
// au/com/integradev/delphi/antlr/Delphi.g:902:32: lbrack expressionList rbrack
{
pushFollow(FOLLOW_lbrack_in_arrayAccessor16359);
lbrack565=lbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_lbrack.add(lbrack565.getTree());
pushFollow(FOLLOW_expressionList_in_arrayAccessor16361);
expressionList566=expressionList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expressionList.add(expressionList566.getTree());
pushFollow(FOLLOW_rbrack_in_arrayAccessor16363);
rbrack567=rbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_rbrack.add(rbrack567.getTree());
// AST REWRITE
// elements: expressionList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 903:30: -> ^( TkArrayAccessorNode expressionList )
{
// au/com/integradev/delphi/antlr/Delphi.g:903:33: ^( TkArrayAccessorNode expressionList )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ArrayAccessorNodeImpl(TkArrayAccessorNode), root_1);
adaptor.addChild(root_1, stream_expressionList.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 147, arrayAccessor_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayAccessor"
public static class argumentList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "argumentList"
// au/com/integradev/delphi/antlr/Delphi.g:905:1: argumentList : '(' ^ ( argument ( ',' argument )* ( ',' )? )? ')' ;
public final DelphiParser.argumentList_return argumentList() throws RecognitionException {
DelphiParser.argumentList_return retval = new DelphiParser.argumentList_return();
retval.start = input.LT(1);
int argumentList_StartIndex = input.index();
Object root_0 = null;
Token char_literal568=null;
Token char_literal570=null;
Token char_literal572=null;
Token char_literal573=null;
ParserRuleReturnScope argument569 =null;
ParserRuleReturnScope argument571 =null;
Object char_literal568_tree=null;
Object char_literal570_tree=null;
Object char_literal572_tree=null;
Object char_literal573_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 148) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:905:30: ( '(' ^ ( argument ( ',' argument )* ( ',' )? )? ')' )
// au/com/integradev/delphi/antlr/Delphi.g:905:32: '(' ^ ( argument ( ',' argument )* ( ',' )? )? ')'
{
root_0 = (Object)adaptor.nil();
char_literal568=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_argumentList16456); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal568_tree = new ArgumentListNodeImpl(char_literal568) ;
root_0 = (Object)adaptor.becomeRoot(char_literal568_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:905:59: ( argument ( ',' argument )* ( ',' )? )?
int alt192=2;
int LA192_0 = input.LA(1);
if ( ((LA192_0 >= ABSOLUTE && LA192_0 <= ALIGN)||(LA192_0 >= AND && LA192_0 <= ASSEMBLY)||(LA192_0 >= AT && LA192_0 <= AUTOMATED)||LA192_0==BEGIN||(LA192_0 >= CASE && LA192_0 <= CLASS)||(LA192_0 >= CONST && LA192_0 <= CONTAINS)||(LA192_0 >= DEFAULT && LA192_0 <= DIV)||LA192_0==DO||(LA192_0 >= DOWNTO && LA192_0 <= DYNAMIC)||(LA192_0 >= ELSE && LA192_0 <= END)||(LA192_0 >= EXCEPT && LA192_0 <= EXTERNAL)||(LA192_0 >= FAR && LA192_0 <= FUNCTION)||LA192_0==GOTO||LA192_0==HELPER||(LA192_0 >= IF && LA192_0 <= IS)||LA192_0==LABEL||(LA192_0 >= LIBRARY && LA192_0 <= LOCAL)||(LA192_0 >= MESSAGE && LA192_0 <= MOD)||(LA192_0 >= NAME && LA192_0 <= NOT)||(LA192_0 >= OBJECT && LA192_0 <= OVERRIDE)||(LA192_0 >= PACKAGE && LA192_0 <= PAREN_BRACKET_LEFT)||LA192_0==PAREN_LEFT||(LA192_0 >= PASCAL && LA192_0 <= PUBLISHED)||(LA192_0 >= RAISE && LA192_0 <= RESOURCESTRING)||(LA192_0 >= SAFECALL && LA192_0 <= SEALED)||(LA192_0 >= SET && LA192_0 <= SQUARE_BRACKET_LEFT)||(LA192_0 >= STATIC && LA192_0 <= STRING)||(LA192_0 >= THEN && LA192_0 <= TYPE)||LA192_0==TkBinaryNumber||LA192_0==TkCharacterEscapeCode||(LA192_0 >= TkHexNumber && LA192_0 <= TkIntNumber)||LA192_0==TkMultilineString||(LA192_0 >= TkQuotedString && LA192_0 <= TkRealNumber)||(LA192_0 >= UNIT && LA192_0 <= USES)||(LA192_0 >= VAR && LA192_0 <= VIRTUAL)||LA192_0==WHILE||(LA192_0 >= WINAPI && LA192_0 <= WRITEONLY)||LA192_0==XOR) ) {
alt192=1;
}
switch (alt192) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:905:60: argument ( ',' argument )* ( ',' )?
{
pushFollow(FOLLOW_argument_in_argumentList16463);
argument569=argument();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, argument569.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:905:69: ( ',' argument )*
loop190:
while (true) {
int alt190=2;
int LA190_0 = input.LA(1);
if ( (LA190_0==COMMA) ) {
int LA190_1 = input.LA(2);
if ( ((LA190_1 >= ABSOLUTE && LA190_1 <= ALIGN)||(LA190_1 >= AND && LA190_1 <= ASSEMBLY)||(LA190_1 >= AT && LA190_1 <= AUTOMATED)||LA190_1==BEGIN||(LA190_1 >= CASE && LA190_1 <= CLASS)||(LA190_1 >= CONST && LA190_1 <= CONTAINS)||(LA190_1 >= DEFAULT && LA190_1 <= DIV)||LA190_1==DO||(LA190_1 >= DOWNTO && LA190_1 <= DYNAMIC)||(LA190_1 >= ELSE && LA190_1 <= END)||(LA190_1 >= EXCEPT && LA190_1 <= EXTERNAL)||(LA190_1 >= FAR && LA190_1 <= FUNCTION)||LA190_1==GOTO||LA190_1==HELPER||(LA190_1 >= IF && LA190_1 <= IS)||LA190_1==LABEL||(LA190_1 >= LIBRARY && LA190_1 <= LOCAL)||(LA190_1 >= MESSAGE && LA190_1 <= MOD)||(LA190_1 >= NAME && LA190_1 <= NOT)||(LA190_1 >= OBJECT && LA190_1 <= OVERRIDE)||(LA190_1 >= PACKAGE && LA190_1 <= PAREN_BRACKET_LEFT)||LA190_1==PAREN_LEFT||(LA190_1 >= PASCAL && LA190_1 <= PUBLISHED)||(LA190_1 >= RAISE && LA190_1 <= RESOURCESTRING)||(LA190_1 >= SAFECALL && LA190_1 <= SEALED)||(LA190_1 >= SET && LA190_1 <= SQUARE_BRACKET_LEFT)||(LA190_1 >= STATIC && LA190_1 <= STRING)||(LA190_1 >= THEN && LA190_1 <= TYPE)||LA190_1==TkBinaryNumber||LA190_1==TkCharacterEscapeCode||(LA190_1 >= TkHexNumber && LA190_1 <= TkIntNumber)||LA190_1==TkMultilineString||(LA190_1 >= TkQuotedString && LA190_1 <= TkRealNumber)||(LA190_1 >= UNIT && LA190_1 <= USES)||(LA190_1 >= VAR && LA190_1 <= VIRTUAL)||LA190_1==WHILE||(LA190_1 >= WINAPI && LA190_1 <= WRITEONLY)||LA190_1==XOR) ) {
alt190=1;
}
}
switch (alt190) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:905:70: ',' argument
{
char_literal570=(Token)match(input,COMMA,FOLLOW_COMMA_in_argumentList16466); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal570_tree = (Object)adaptor.create(char_literal570);
adaptor.addChild(root_0, char_literal570_tree);
}
pushFollow(FOLLOW_argument_in_argumentList16468);
argument571=argument();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, argument571.getTree());
}
break;
default :
break loop190;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:905:85: ( ',' )?
int alt191=2;
int LA191_0 = input.LA(1);
if ( (LA191_0==COMMA) ) {
alt191=1;
}
switch (alt191) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:905:85: ','
{
char_literal572=(Token)match(input,COMMA,FOLLOW_COMMA_in_argumentList16472); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal572_tree = (Object)adaptor.create(char_literal572);
adaptor.addChild(root_0, char_literal572_tree);
}
}
break;
}
}
break;
}
char_literal573=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_argumentList16477); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal573_tree = (Object)adaptor.create(char_literal573);
adaptor.addChild(root_0, char_literal573_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 148, argumentList_StartIndex); }
}
return retval;
}
// $ANTLR end "argumentList"
public static class argument_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "argument"
// au/com/integradev/delphi/antlr/Delphi.g:907:1: argument : ( argumentName )? argumentExpression -> ^( TkArgument ( argumentName )? argumentExpression ) ;
public final DelphiParser.argument_return argument() throws RecognitionException {
DelphiParser.argument_return retval = new DelphiParser.argument_return();
retval.start = input.LT(1);
int argument_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope argumentName574 =null;
ParserRuleReturnScope argumentExpression575 =null;
RewriteRuleSubtreeStream stream_argumentExpression=new RewriteRuleSubtreeStream(adaptor,"rule argumentExpression");
RewriteRuleSubtreeStream stream_argumentName=new RewriteRuleSubtreeStream(adaptor,"rule argumentName");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 149) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:907:30: ( ( argumentName )? argumentExpression -> ^( TkArgument ( argumentName )? argumentExpression ) )
// au/com/integradev/delphi/antlr/Delphi.g:907:32: ( argumentName )? argumentExpression
{
// au/com/integradev/delphi/antlr/Delphi.g:907:32: ( argumentName )?
int alt193=2;
switch ( input.LA(1) ) {
case TkIdentifier:
{
int LA193_1 = input.LA(2);
if ( (LA193_1==ASSIGN) ) {
alt193=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA193_2 = input.LA(2);
if ( (LA193_2==ASSIGN) ) {
alt193=1;
}
}
break;
case NOT:
{
int LA193_3 = input.LA(2);
if ( (LA193_3==ASSIGN) ) {
alt193=1;
}
}
break;
case NIL:
{
int LA193_4 = input.LA(2);
if ( (LA193_4==ASSIGN) ) {
alt193=1;
}
}
break;
case STRING:
{
int LA193_6 = input.LA(2);
if ( (LA193_6==ASSIGN) ) {
alt193=1;
}
}
break;
case FILE:
{
int LA193_7 = input.LA(2);
if ( (LA193_7==ASSIGN) ) {
alt193=1;
}
}
break;
case INHERITED:
{
int LA193_8 = input.LA(2);
if ( (LA193_8==ASSIGN) ) {
alt193=1;
}
}
break;
case PROCEDURE:
{
int LA193_9 = input.LA(2);
if ( (LA193_9==ASSIGN) ) {
alt193=1;
}
}
break;
case FUNCTION:
{
int LA193_10 = input.LA(2);
if ( (LA193_10==ASSIGN) ) {
alt193=1;
}
}
break;
case AND:
case ARRAY:
case AS:
case ASM:
case BEGIN:
case CASE:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case DISPINTERFACE:
case DIV:
case DO:
case DOWNTO:
case ELSE:
case END:
case EXCEPT:
case EXPORTS:
case FINALIZATION:
case FINALLY:
case FOR:
case GOTO:
case IF:
case IMPLEMENTATION:
case IN:
case INITIALIZATION:
case INLINE:
case INTERFACE:
case IS:
case LIBRARY:
case MOD:
case OBJECT:
case OF:
case OR:
case PACKED:
case PROGRAM:
case PROPERTY:
case RAISE:
case RECORD:
case REPEAT:
case RESOURCESTRING:
case SET:
case SHL:
case SHR:
case THEN:
case THREADVAR:
case TO:
case TRY:
case TYPE:
case UNIT:
case UNTIL:
case USES:
case VAR:
case WHILE:
case WITH:
case XOR:
{
alt193=1;
}
break;
}
switch (alt193) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:907:32: argumentName
{
pushFollow(FOLLOW_argumentName_in_argument16534);
argumentName574=argumentName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_argumentName.add(argumentName574.getTree());
}
break;
}
pushFollow(FOLLOW_argumentExpression_in_argument16537);
argumentExpression575=argumentExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_argumentExpression.add(argumentExpression575.getTree());
// AST REWRITE
// elements: argumentName, argumentExpression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 908:30: -> ^( TkArgument ( argumentName )? argumentExpression )
{
// au/com/integradev/delphi/antlr/Delphi.g:908:33: ^( TkArgument ( argumentName )? argumentExpression )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ArgumentNodeImpl(TkArgument), root_1);
// au/com/integradev/delphi/antlr/Delphi.g:908:64: ( argumentName )?
if ( stream_argumentName.hasNext() ) {
adaptor.addChild(root_1, stream_argumentName.nextTree());
}
stream_argumentName.reset();
adaptor.addChild(root_1, stream_argumentExpression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 149, argument_StartIndex); }
}
return retval;
}
// $ANTLR end "argument"
public static class argumentName_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "argumentName"
// au/com/integradev/delphi/antlr/Delphi.g:910:1: argumentName : ( ident ':=' !| keywords ':=' -> ^() );
public final DelphiParser.argumentName_return argumentName() throws RecognitionException {
DelphiParser.argumentName_return retval = new DelphiParser.argumentName_return();
retval.start = input.LT(1);
int argumentName_StartIndex = input.index();
Object root_0 = null;
Token string_literal577=null;
Token string_literal579=null;
ParserRuleReturnScope ident576 =null;
ParserRuleReturnScope keywords578 =null;
Object string_literal577_tree=null;
Object string_literal579_tree=null;
RewriteRuleTokenStream stream_ASSIGN=new RewriteRuleTokenStream(adaptor,"token ASSIGN");
RewriteRuleSubtreeStream stream_keywords=new RewriteRuleSubtreeStream(adaptor,"rule keywords");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 150) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:910:30: ( ident ':=' !| keywords ':=' -> ^() )
int alt194=2;
switch ( input.LA(1) ) {
case TkIdentifier:
{
alt194=1;
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA194_2 = input.LA(2);
if ( (LA194_2==ASSIGN) ) {
int LA194_4 = input.LA(3);
if ( (synpred276_Delphi()) ) {
alt194=1;
}
else if ( (true) ) {
alt194=2;
}
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 194, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case AND:
case ARRAY:
case AS:
case ASM:
case BEGIN:
case CASE:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case DISPINTERFACE:
case DIV:
case DO:
case DOWNTO:
case ELSE:
case END:
case EXCEPT:
case EXPORTS:
case FILE:
case FINALIZATION:
case FINALLY:
case FOR:
case FUNCTION:
case GOTO:
case IF:
case IMPLEMENTATION:
case IN:
case INHERITED:
case INITIALIZATION:
case INLINE:
case INTERFACE:
case IS:
case LIBRARY:
case MOD:
case NIL:
case NOT:
case OBJECT:
case OF:
case OR:
case PACKED:
case PROCEDURE:
case PROGRAM:
case PROPERTY:
case RAISE:
case RECORD:
case REPEAT:
case RESOURCESTRING:
case SET:
case SHL:
case SHR:
case STRING:
case THEN:
case THREADVAR:
case TO:
case TRY:
case TYPE:
case UNIT:
case UNTIL:
case USES:
case VAR:
case WHILE:
case WITH:
case XOR:
{
alt194=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 194, 0, input);
throw nvae;
}
switch (alt194) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:910:32: ident ':=' !
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_ident_in_argumentName16633);
ident576=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident576.getTree());
string_literal577=(Token)match(input,ASSIGN,FOLLOW_ASSIGN_in_argumentName16635); if (state.failed) return retval;
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:911:32: keywords ':='
{
pushFollow(FOLLOW_keywords_in_argumentName16669);
keywords578=keywords();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_keywords.add(keywords578.getTree());
string_literal579=(Token)match(input,ASSIGN,FOLLOW_ASSIGN_in_argumentName16671); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ASSIGN.add(string_literal579);
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 911:46: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:911:49: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier, -2), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 150, argumentName_StartIndex); }
}
return retval;
}
// $ANTLR end "argumentName"
public static class argumentExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "argumentExpression"
// au/com/integradev/delphi/antlr/Delphi.g:913:1: argumentExpression : expression ( writeArguments )? ;
public final DelphiParser.argumentExpression_return argumentExpression() throws RecognitionException {
DelphiParser.argumentExpression_return retval = new DelphiParser.argumentExpression_return();
retval.start = input.LT(1);
int argumentExpression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope expression580 =null;
ParserRuleReturnScope writeArguments581 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 151) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:913:30: ( expression ( writeArguments )? )
// au/com/integradev/delphi/antlr/Delphi.g:913:32: expression ( writeArguments )?
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_expression_in_argumentExpression16724);
expression580=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression580.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:913:43: ( writeArguments )?
int alt195=2;
int LA195_0 = input.LA(1);
if ( (LA195_0==COLON) ) {
alt195=1;
}
switch (alt195) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:913:43: writeArguments
{
pushFollow(FOLLOW_writeArguments_in_argumentExpression16726);
writeArguments581=writeArguments();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, writeArguments581.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 151, argumentExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "argumentExpression"
public static class writeArguments_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "writeArguments"
// au/com/integradev/delphi/antlr/Delphi.g:915:1: writeArguments : ':' ! expression ( ':' ! expression )? ;
public final DelphiParser.writeArguments_return writeArguments() throws RecognitionException {
DelphiParser.writeArguments_return retval = new DelphiParser.writeArguments_return();
retval.start = input.LT(1);
int writeArguments_StartIndex = input.index();
Object root_0 = null;
Token char_literal582=null;
Token char_literal584=null;
ParserRuleReturnScope expression583 =null;
ParserRuleReturnScope expression585 =null;
Object char_literal582_tree=null;
Object char_literal584_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 152) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:915:30: ( ':' ! expression ( ':' ! expression )? )
// au/com/integradev/delphi/antlr/Delphi.g:915:32: ':' ! expression ( ':' ! expression )?
{
root_0 = (Object)adaptor.nil();
char_literal582=(Token)match(input,COLON,FOLLOW_COLON_in_writeArguments16778); if (state.failed) return retval;
pushFollow(FOLLOW_expression_in_writeArguments16781);
expression583=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression583.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:915:48: ( ':' ! expression )?
int alt196=2;
int LA196_0 = input.LA(1);
if ( (LA196_0==COLON) ) {
alt196=1;
}
switch (alt196) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:915:49: ':' ! expression
{
char_literal584=(Token)match(input,COLON,FOLLOW_COLON_in_writeArguments16784); if (state.failed) return retval;
pushFollow(FOLLOW_expression_in_writeArguments16787);
expression585=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression585.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 152, writeArguments_StartIndex); }
}
return retval;
}
// $ANTLR end "writeArguments"
public static class anonymousMethod_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "anonymousMethod"
// au/com/integradev/delphi/antlr/Delphi.g:917:1: anonymousMethod : anonymousMethodHeading block -> ^( TkAnonymousMethod anonymousMethodHeading block ) ;
public final DelphiParser.anonymousMethod_return anonymousMethod() throws RecognitionException {
DelphiParser.anonymousMethod_return retval = new DelphiParser.anonymousMethod_return();
retval.start = input.LT(1);
int anonymousMethod_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope anonymousMethodHeading586 =null;
ParserRuleReturnScope block587 =null;
RewriteRuleSubtreeStream stream_anonymousMethodHeading=new RewriteRuleSubtreeStream(adaptor,"rule anonymousMethodHeading");
RewriteRuleSubtreeStream stream_block=new RewriteRuleSubtreeStream(adaptor,"rule block");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 153) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:917:30: ( anonymousMethodHeading block -> ^( TkAnonymousMethod anonymousMethodHeading block ) )
// au/com/integradev/delphi/antlr/Delphi.g:917:32: anonymousMethodHeading block
{
pushFollow(FOLLOW_anonymousMethodHeading_in_anonymousMethod16840);
anonymousMethodHeading586=anonymousMethodHeading();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_anonymousMethodHeading.add(anonymousMethodHeading586.getTree());
pushFollow(FOLLOW_block_in_anonymousMethod16842);
block587=block();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_block.add(block587.getTree());
// AST REWRITE
// elements: block, anonymousMethodHeading
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 917:61: -> ^( TkAnonymousMethod anonymousMethodHeading block )
{
// au/com/integradev/delphi/antlr/Delphi.g:917:64: ^( TkAnonymousMethod anonymousMethodHeading block )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AnonymousMethodNodeImpl(TkAnonymousMethod), root_1);
adaptor.addChild(root_1, stream_anonymousMethodHeading.nextTree());
adaptor.addChild(root_1, stream_block.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 153, anonymousMethod_StartIndex); }
}
return retval;
}
// $ANTLR end "anonymousMethod"
public static class anonymousMethodHeading_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "anonymousMethodHeading"
// au/com/integradev/delphi/antlr/Delphi.g:919:1: anonymousMethodHeading : ( PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )* -> ^( TkAnonymousMethodHeading PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )* ) | FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )* -> ^( TkAnonymousMethodHeading FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )* ) );
public final DelphiParser.anonymousMethodHeading_return anonymousMethodHeading() throws RecognitionException {
DelphiParser.anonymousMethodHeading_return retval = new DelphiParser.anonymousMethodHeading_return();
retval.start = input.LT(1);
int anonymousMethodHeading_StartIndex = input.index();
Object root_0 = null;
Token PROCEDURE588=null;
Token char_literal590=null;
Token FUNCTION592=null;
Token char_literal595=null;
ParserRuleReturnScope routineParameters589 =null;
ParserRuleReturnScope interfaceDirective591 =null;
ParserRuleReturnScope routineParameters593 =null;
ParserRuleReturnScope routineReturnType594 =null;
ParserRuleReturnScope interfaceDirective596 =null;
Object PROCEDURE588_tree=null;
Object char_literal590_tree=null;
Object FUNCTION592_tree=null;
Object char_literal595_tree=null;
RewriteRuleTokenStream stream_PROCEDURE=new RewriteRuleTokenStream(adaptor,"token PROCEDURE");
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_FUNCTION=new RewriteRuleTokenStream(adaptor,"token FUNCTION");
RewriteRuleSubtreeStream stream_routineReturnType=new RewriteRuleSubtreeStream(adaptor,"rule routineReturnType");
RewriteRuleSubtreeStream stream_interfaceDirective=new RewriteRuleSubtreeStream(adaptor,"rule interfaceDirective");
RewriteRuleSubtreeStream stream_routineParameters=new RewriteRuleSubtreeStream(adaptor,"rule routineParameters");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 154) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:919:30: ( PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )* -> ^( TkAnonymousMethodHeading PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )* ) | FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )* -> ^( TkAnonymousMethodHeading FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )* ) )
int alt203=2;
int LA203_0 = input.LA(1);
if ( (LA203_0==PROCEDURE) ) {
alt203=1;
}
else if ( (LA203_0==FUNCTION) ) {
alt203=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 203, 0, input);
throw nvae;
}
switch (alt203) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:919:32: PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )*
{
PROCEDURE588=(Token)match(input,PROCEDURE,FOLLOW_PROCEDURE_in_anonymousMethodHeading16898); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_PROCEDURE.add(PROCEDURE588);
// au/com/integradev/delphi/antlr/Delphi.g:919:42: ( routineParameters )?
int alt197=2;
int LA197_0 = input.LA(1);
if ( (LA197_0==PAREN_LEFT) ) {
alt197=1;
}
switch (alt197) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:919:42: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_anonymousMethodHeading16900);
routineParameters589=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineParameters.add(routineParameters589.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:919:61: ( ( ';' )? interfaceDirective )*
loop199:
while (true) {
int alt199=2;
int LA199_0 = input.LA(1);
if ( (LA199_0==ABSTRACT||LA199_0==ASSEMBLER||LA199_0==CDECL||LA199_0==DEPRECATED||LA199_0==DISPID||LA199_0==DYNAMIC||(LA199_0 >= EXPERIMENTAL && LA199_0 <= EXPORT)||LA199_0==EXTERNAL||LA199_0==FAR||LA199_0==FINAL||LA199_0==FORWARD||LA199_0==INLINE||(LA199_0 >= LIBRARY && LA199_0 <= LOCAL)||LA199_0==MESSAGE||LA199_0==NEAR||(LA199_0 >= OVERLOAD && LA199_0 <= OVERRIDE)||(LA199_0 >= PASCAL && LA199_0 <= PLATFORM)||(LA199_0 >= REGISTER && LA199_0 <= REINTRODUCE)||LA199_0==SAFECALL||LA199_0==SEMICOLON||(LA199_0 >= STATIC && LA199_0 <= STDCALL)||LA199_0==UNSAFE||(LA199_0 >= VARARGS && LA199_0 <= VIRTUAL)||LA199_0==WINAPI) ) {
alt199=1;
}
switch (alt199) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:919:62: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:919:62: ( ';' )?
int alt198=2;
int LA198_0 = input.LA(1);
if ( (LA198_0==SEMICOLON) ) {
alt198=1;
}
switch (alt198) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:919:63: ';'
{
char_literal590=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_anonymousMethodHeading16905); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal590);
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_anonymousMethodHeading16909);
interfaceDirective591=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_interfaceDirective.add(interfaceDirective591.getTree());
}
break;
default :
break loop199;
}
}
// AST REWRITE
// elements: routineParameters, interfaceDirective, PROCEDURE, SEMICOLON
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 920:30: -> ^( TkAnonymousMethodHeading PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:920:33: ^( TkAnonymousMethodHeading PROCEDURE ( routineParameters )? ( ( ';' )? interfaceDirective )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AnonymousMethodHeadingNodeImpl(TkAnonymousMethodHeading), root_1);
adaptor.addChild(root_1, stream_PROCEDURE.nextNode());
// au/com/integradev/delphi/antlr/Delphi.g:920:102: ( routineParameters )?
if ( stream_routineParameters.hasNext() ) {
adaptor.addChild(root_1, stream_routineParameters.nextTree());
}
stream_routineParameters.reset();
// au/com/integradev/delphi/antlr/Delphi.g:920:121: ( ( ';' )? interfaceDirective )*
while ( stream_interfaceDirective.hasNext() ) {
// au/com/integradev/delphi/antlr/Delphi.g:920:122: ( ';' )?
if ( stream_SEMICOLON.hasNext() ) {
adaptor.addChild(root_1, stream_SEMICOLON.nextNode());
}
stream_SEMICOLON.reset();
adaptor.addChild(root_1, stream_interfaceDirective.nextTree());
}
stream_interfaceDirective.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:921:32: FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )*
{
FUNCTION592=(Token)match(input,FUNCTION,FOLLOW_FUNCTION_in_anonymousMethodHeading16997); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_FUNCTION.add(FUNCTION592);
// au/com/integradev/delphi/antlr/Delphi.g:921:41: ( routineParameters )?
int alt200=2;
int LA200_0 = input.LA(1);
if ( (LA200_0==PAREN_LEFT) ) {
alt200=1;
}
switch (alt200) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:921:41: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_anonymousMethodHeading16999);
routineParameters593=routineParameters();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineParameters.add(routineParameters593.getTree());
}
break;
}
pushFollow(FOLLOW_routineReturnType_in_anonymousMethodHeading17002);
routineReturnType594=routineReturnType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineReturnType.add(routineReturnType594.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:921:78: ( ( ';' )? interfaceDirective )*
loop202:
while (true) {
int alt202=2;
int LA202_0 = input.LA(1);
if ( (LA202_0==ABSTRACT||LA202_0==ASSEMBLER||LA202_0==CDECL||LA202_0==DEPRECATED||LA202_0==DISPID||LA202_0==DYNAMIC||(LA202_0 >= EXPERIMENTAL && LA202_0 <= EXPORT)||LA202_0==EXTERNAL||LA202_0==FAR||LA202_0==FINAL||LA202_0==FORWARD||LA202_0==INLINE||(LA202_0 >= LIBRARY && LA202_0 <= LOCAL)||LA202_0==MESSAGE||LA202_0==NEAR||(LA202_0 >= OVERLOAD && LA202_0 <= OVERRIDE)||(LA202_0 >= PASCAL && LA202_0 <= PLATFORM)||(LA202_0 >= REGISTER && LA202_0 <= REINTRODUCE)||LA202_0==SAFECALL||LA202_0==SEMICOLON||(LA202_0 >= STATIC && LA202_0 <= STDCALL)||LA202_0==UNSAFE||(LA202_0 >= VARARGS && LA202_0 <= VIRTUAL)||LA202_0==WINAPI) ) {
alt202=1;
}
switch (alt202) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:921:79: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:921:79: ( ';' )?
int alt201=2;
int LA201_0 = input.LA(1);
if ( (LA201_0==SEMICOLON) ) {
alt201=1;
}
switch (alt201) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:921:80: ';'
{
char_literal595=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_anonymousMethodHeading17006); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal595);
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_anonymousMethodHeading17010);
interfaceDirective596=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_interfaceDirective.add(interfaceDirective596.getTree());
}
break;
default :
break loop202;
}
}
// AST REWRITE
// elements: routineReturnType, interfaceDirective, routineParameters, FUNCTION, SEMICOLON
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 922:30: -> ^( TkAnonymousMethodHeading FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:922:33: ^( TkAnonymousMethodHeading FUNCTION ( routineParameters )? routineReturnType ( ( ';' )? interfaceDirective )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new AnonymousMethodHeadingNodeImpl(TkAnonymousMethodHeading), root_1);
adaptor.addChild(root_1, stream_FUNCTION.nextNode());
// au/com/integradev/delphi/antlr/Delphi.g:922:101: ( routineParameters )?
if ( stream_routineParameters.hasNext() ) {
adaptor.addChild(root_1, stream_routineParameters.nextTree());
}
stream_routineParameters.reset();
adaptor.addChild(root_1, stream_routineReturnType.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:922:138: ( ( ';' )? interfaceDirective )*
while ( stream_interfaceDirective.hasNext() ) {
// au/com/integradev/delphi/antlr/Delphi.g:922:139: ( ';' )?
if ( stream_SEMICOLON.hasNext() ) {
adaptor.addChild(root_1, stream_SEMICOLON.nextNode());
}
stream_SEMICOLON.reset();
adaptor.addChild(root_1, stream_interfaceDirective.nextTree());
}
stream_interfaceDirective.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 154, anonymousMethodHeading_StartIndex); }
}
return retval;
}
// $ANTLR end "anonymousMethodHeading"
public static class expressionOrRange_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "expressionOrRange"
// au/com/integradev/delphi/antlr/Delphi.g:924:1: expressionOrRange : expression ( '..' ^ expression )? ;
public final DelphiParser.expressionOrRange_return expressionOrRange() throws RecognitionException {
DelphiParser.expressionOrRange_return retval = new DelphiParser.expressionOrRange_return();
retval.start = input.LT(1);
int expressionOrRange_StartIndex = input.index();
Object root_0 = null;
Token string_literal598=null;
ParserRuleReturnScope expression597 =null;
ParserRuleReturnScope expression599 =null;
Object string_literal598_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 155) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:924:30: ( expression ( '..' ^ expression )? )
// au/com/integradev/delphi/antlr/Delphi.g:924:32: expression ( '..' ^ expression )?
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_expression_in_expressionOrRange17115);
expression597=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression597.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:924:43: ( '..' ^ expression )?
int alt204=2;
int LA204_0 = input.LA(1);
if ( (LA204_0==DOT_DOT) ) {
alt204=1;
}
switch (alt204) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:924:44: '..' ^ expression
{
string_literal598=(Token)match(input,DOT_DOT,FOLLOW_DOT_DOT_in_expressionOrRange17118); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal598_tree = new RangeExpressionNodeImpl(string_literal598) ;
root_0 = (Object)adaptor.becomeRoot(string_literal598_tree, root_0);
}
pushFollow(FOLLOW_expression_in_expressionOrRange17124);
expression599=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression599.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 155, expressionOrRange_StartIndex); }
}
return retval;
}
// $ANTLR end "expressionOrRange"
public static class expressionList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "expressionList"
// au/com/integradev/delphi/antlr/Delphi.g:926:1: expressionList : ( expression ( ',' !)? )+ ;
public final DelphiParser.expressionList_return expressionList() throws RecognitionException {
DelphiParser.expressionList_return retval = new DelphiParser.expressionList_return();
retval.start = input.LT(1);
int expressionList_StartIndex = input.index();
Object root_0 = null;
Token char_literal601=null;
ParserRuleReturnScope expression600 =null;
Object char_literal601_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 156) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:926:30: ( ( expression ( ',' !)? )+ )
// au/com/integradev/delphi/antlr/Delphi.g:926:32: ( expression ( ',' !)? )+
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:926:32: ( expression ( ',' !)? )+
int cnt206=0;
loop206:
while (true) {
int alt206=2;
int LA206_0 = input.LA(1);
if ( ((LA206_0 >= ABSOLUTE && LA206_0 <= ALIGN)||(LA206_0 >= ASSEMBLER && LA206_0 <= ASSEMBLY)||(LA206_0 >= AT && LA206_0 <= AUTOMATED)||LA206_0==CDECL||LA206_0==CONTAINS||(LA206_0 >= DEFAULT && LA206_0 <= DEREFERENCE)||LA206_0==DISPID||LA206_0==DYNAMIC||(LA206_0 >= EXPERIMENTAL && LA206_0 <= EXPORT)||LA206_0==EXTERNAL||(LA206_0 >= FAR && LA206_0 <= FINAL)||(LA206_0 >= FORWARD && LA206_0 <= FUNCTION)||LA206_0==HELPER||LA206_0==IMPLEMENTS||(LA206_0 >= INDEX && LA206_0 <= INHERITED)||LA206_0==LABEL||LA206_0==LOCAL||(LA206_0 >= MESSAGE && LA206_0 <= MINUS)||(LA206_0 >= NAME && LA206_0 <= NOT)||(LA206_0 >= ON && LA206_0 <= OPERATOR)||(LA206_0 >= OUT && LA206_0 <= OVERRIDE)||LA206_0==PACKAGE||LA206_0==PAREN_BRACKET_LEFT||LA206_0==PAREN_LEFT||(LA206_0 >= PASCAL && LA206_0 <= PROCEDURE)||(LA206_0 >= PROTECTED && LA206_0 <= PUBLISHED)||(LA206_0 >= READ && LA206_0 <= READONLY)||(LA206_0 >= REFERENCE && LA206_0 <= REINTRODUCE)||(LA206_0 >= REQUIRES && LA206_0 <= RESIDENT)||(LA206_0 >= SAFECALL && LA206_0 <= SEALED)||LA206_0==SQUARE_BRACKET_LEFT||(LA206_0 >= STATIC && LA206_0 <= STRING)||LA206_0==TkBinaryNumber||LA206_0==TkCharacterEscapeCode||(LA206_0 >= TkHexNumber && LA206_0 <= TkIntNumber)||LA206_0==TkMultilineString||(LA206_0 >= TkQuotedString && LA206_0 <= TkRealNumber)||LA206_0==UNSAFE||(LA206_0 >= VARARGS && LA206_0 <= VIRTUAL)||LA206_0==WINAPI||(LA206_0 >= WRITE && LA206_0 <= WRITEONLY)) ) {
alt206=1;
}
switch (alt206) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:926:33: expression ( ',' !)?
{
pushFollow(FOLLOW_expression_in_expressionList17178);
expression600=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression600.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:926:44: ( ',' !)?
int alt205=2;
int LA205_0 = input.LA(1);
if ( (LA205_0==COMMA) ) {
alt205=1;
}
switch (alt205) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:926:45: ',' !
{
char_literal601=(Token)match(input,COMMA,FOLLOW_COMMA_in_expressionList17181); if (state.failed) return retval;
}
break;
}
}
break;
default :
if ( cnt206 >= 1 ) break loop206;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(206, input);
throw eee;
}
cnt206++;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 156, expressionList_StartIndex); }
}
return retval;
}
// $ANTLR end "expressionList"
public static class expressionOrRangeList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "expressionOrRangeList"
// au/com/integradev/delphi/antlr/Delphi.g:928:1: expressionOrRangeList : ( expressionOrRange ( ',' !)? )+ ;
public final DelphiParser.expressionOrRangeList_return expressionOrRangeList() throws RecognitionException {
DelphiParser.expressionOrRangeList_return retval = new DelphiParser.expressionOrRangeList_return();
retval.start = input.LT(1);
int expressionOrRangeList_StartIndex = input.index();
Object root_0 = null;
Token char_literal603=null;
ParserRuleReturnScope expressionOrRange602 =null;
Object char_literal603_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 157) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:928:30: ( ( expressionOrRange ( ',' !)? )+ )
// au/com/integradev/delphi/antlr/Delphi.g:928:32: ( expressionOrRange ( ',' !)? )+
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:928:32: ( expressionOrRange ( ',' !)? )+
int cnt208=0;
loop208:
while (true) {
int alt208=2;
int LA208_0 = input.LA(1);
if ( ((LA208_0 >= ABSOLUTE && LA208_0 <= ALIGN)||(LA208_0 >= ASSEMBLER && LA208_0 <= ASSEMBLY)||(LA208_0 >= AT && LA208_0 <= AUTOMATED)||LA208_0==CDECL||LA208_0==CONTAINS||(LA208_0 >= DEFAULT && LA208_0 <= DEREFERENCE)||LA208_0==DISPID||LA208_0==DYNAMIC||(LA208_0 >= EXPERIMENTAL && LA208_0 <= EXPORT)||LA208_0==EXTERNAL||(LA208_0 >= FAR && LA208_0 <= FINAL)||(LA208_0 >= FORWARD && LA208_0 <= FUNCTION)||LA208_0==HELPER||LA208_0==IMPLEMENTS||(LA208_0 >= INDEX && LA208_0 <= INHERITED)||LA208_0==LABEL||LA208_0==LOCAL||(LA208_0 >= MESSAGE && LA208_0 <= MINUS)||(LA208_0 >= NAME && LA208_0 <= NOT)||(LA208_0 >= ON && LA208_0 <= OPERATOR)||(LA208_0 >= OUT && LA208_0 <= OVERRIDE)||LA208_0==PACKAGE||LA208_0==PAREN_BRACKET_LEFT||LA208_0==PAREN_LEFT||(LA208_0 >= PASCAL && LA208_0 <= PROCEDURE)||(LA208_0 >= PROTECTED && LA208_0 <= PUBLISHED)||(LA208_0 >= READ && LA208_0 <= READONLY)||(LA208_0 >= REFERENCE && LA208_0 <= REINTRODUCE)||(LA208_0 >= REQUIRES && LA208_0 <= RESIDENT)||(LA208_0 >= SAFECALL && LA208_0 <= SEALED)||LA208_0==SQUARE_BRACKET_LEFT||(LA208_0 >= STATIC && LA208_0 <= STRING)||LA208_0==TkBinaryNumber||LA208_0==TkCharacterEscapeCode||(LA208_0 >= TkHexNumber && LA208_0 <= TkIntNumber)||LA208_0==TkMultilineString||(LA208_0 >= TkQuotedString && LA208_0 <= TkRealNumber)||LA208_0==UNSAFE||(LA208_0 >= VARARGS && LA208_0 <= VIRTUAL)||LA208_0==WINAPI||(LA208_0 >= WRITE && LA208_0 <= WRITEONLY)) ) {
alt208=1;
}
switch (alt208) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:928:33: expressionOrRange ( ',' !)?
{
pushFollow(FOLLOW_expressionOrRange_in_expressionOrRangeList17231);
expressionOrRange602=expressionOrRange();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expressionOrRange602.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:928:51: ( ',' !)?
int alt207=2;
int LA207_0 = input.LA(1);
if ( (LA207_0==COMMA) ) {
alt207=1;
}
switch (alt207) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:928:52: ',' !
{
char_literal603=(Token)match(input,COMMA,FOLLOW_COMMA_in_expressionOrRangeList17234); if (state.failed) return retval;
}
break;
}
}
break;
default :
if ( cnt208 >= 1 ) break loop208;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(208, input);
throw eee;
}
cnt208++;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 157, expressionOrRangeList_StartIndex); }
}
return retval;
}
// $ANTLR end "expressionOrRangeList"
public static class textLiteral_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "textLiteral"
// au/com/integradev/delphi/antlr/Delphi.g:930:1: textLiteral : ( singleLineTextLiteral -> ^( TkTextLiteral singleLineTextLiteral ) | multilineTextLiteral -> ^( TkTextLiteral multilineTextLiteral ) );
public final DelphiParser.textLiteral_return textLiteral() throws RecognitionException {
DelphiParser.textLiteral_return retval = new DelphiParser.textLiteral_return();
retval.start = input.LT(1);
int textLiteral_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope singleLineTextLiteral604 =null;
ParserRuleReturnScope multilineTextLiteral605 =null;
RewriteRuleSubtreeStream stream_multilineTextLiteral=new RewriteRuleSubtreeStream(adaptor,"rule multilineTextLiteral");
RewriteRuleSubtreeStream stream_singleLineTextLiteral=new RewriteRuleSubtreeStream(adaptor,"rule singleLineTextLiteral");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 158) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:930:30: ( singleLineTextLiteral -> ^( TkTextLiteral singleLineTextLiteral ) | multilineTextLiteral -> ^( TkTextLiteral multilineTextLiteral ) )
int alt209=2;
int LA209_0 = input.LA(1);
if ( (LA209_0==DEREFERENCE||LA209_0==TkCharacterEscapeCode||LA209_0==TkQuotedString) ) {
alt209=1;
}
else if ( (LA209_0==TkMultilineString) ) {
alt209=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 209, 0, input);
throw nvae;
}
switch (alt209) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:930:32: singleLineTextLiteral
{
pushFollow(FOLLOW_singleLineTextLiteral_in_textLiteral17293);
singleLineTextLiteral604=singleLineTextLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_singleLineTextLiteral.add(singleLineTextLiteral604.getTree());
// AST REWRITE
// elements: singleLineTextLiteral
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 930:54: -> ^( TkTextLiteral singleLineTextLiteral )
{
// au/com/integradev/delphi/antlr/Delphi.g:930:57: ^( TkTextLiteral singleLineTextLiteral )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new TextLiteralNodeImpl(TkTextLiteral), root_1);
adaptor.addChild(root_1, stream_singleLineTextLiteral.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:931:32: multilineTextLiteral
{
pushFollow(FOLLOW_multilineTextLiteral_in_textLiteral17337);
multilineTextLiteral605=multilineTextLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_multilineTextLiteral.add(multilineTextLiteral605.getTree());
// AST REWRITE
// elements: multilineTextLiteral
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 931:53: -> ^( TkTextLiteral multilineTextLiteral )
{
// au/com/integradev/delphi/antlr/Delphi.g:931:56: ^( TkTextLiteral multilineTextLiteral )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new TextLiteralNodeImpl(TkTextLiteral), root_1);
adaptor.addChild(root_1, stream_multilineTextLiteral.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 158, textLiteral_StartIndex); }
}
return retval;
}
// $ANTLR end "textLiteral"
public static class singleLineTextLiteral_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "singleLineTextLiteral"
// au/com/integradev/delphi/antlr/Delphi.g:933:1: singleLineTextLiteral : ( TkQuotedString ( ( escapedCharacter )+ TkQuotedString )* ( escapedCharacter )* | ( escapedCharacter )+ ( TkQuotedString ( escapedCharacter )+ )* ( TkQuotedString )? );
public final DelphiParser.singleLineTextLiteral_return singleLineTextLiteral() throws RecognitionException {
DelphiParser.singleLineTextLiteral_return retval = new DelphiParser.singleLineTextLiteral_return();
retval.start = input.LT(1);
int singleLineTextLiteral_StartIndex = input.index();
Object root_0 = null;
Token TkQuotedString606=null;
Token TkQuotedString608=null;
Token TkQuotedString611=null;
Token TkQuotedString613=null;
ParserRuleReturnScope escapedCharacter607 =null;
ParserRuleReturnScope escapedCharacter609 =null;
ParserRuleReturnScope escapedCharacter610 =null;
ParserRuleReturnScope escapedCharacter612 =null;
Object TkQuotedString606_tree=null;
Object TkQuotedString608_tree=null;
Object TkQuotedString611_tree=null;
Object TkQuotedString613_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 159) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:933:30: ( TkQuotedString ( ( escapedCharacter )+ TkQuotedString )* ( escapedCharacter )* | ( escapedCharacter )+ ( TkQuotedString ( escapedCharacter )+ )* ( TkQuotedString )? )
int alt217=2;
int LA217_0 = input.LA(1);
if ( (LA217_0==TkQuotedString) ) {
alt217=1;
}
else if ( (LA217_0==DEREFERENCE||LA217_0==TkCharacterEscapeCode) ) {
alt217=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 217, 0, input);
throw nvae;
}
switch (alt217) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:933:32: TkQuotedString ( ( escapedCharacter )+ TkQuotedString )* ( escapedCharacter )*
{
root_0 = (Object)adaptor.nil();
TkQuotedString606=(Token)match(input,TkQuotedString,FOLLOW_TkQuotedString_in_singleLineTextLiteral17392); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkQuotedString606_tree = (Object)adaptor.create(TkQuotedString606);
adaptor.addChild(root_0, TkQuotedString606_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:933:47: ( ( escapedCharacter )+ TkQuotedString )*
loop211:
while (true) {
int alt211=2;
alt211 = dfa211.predict(input);
switch (alt211) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:933:48: ( escapedCharacter )+ TkQuotedString
{
// au/com/integradev/delphi/antlr/Delphi.g:933:48: ( escapedCharacter )+
int cnt210=0;
loop210:
while (true) {
int alt210=2;
int LA210_0 = input.LA(1);
if ( (LA210_0==DEREFERENCE||LA210_0==TkCharacterEscapeCode) ) {
alt210=1;
}
switch (alt210) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:933:48: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_singleLineTextLiteral17395);
escapedCharacter607=escapedCharacter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, escapedCharacter607.getTree());
}
break;
default :
if ( cnt210 >= 1 ) break loop210;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(210, input);
throw eee;
}
cnt210++;
}
TkQuotedString608=(Token)match(input,TkQuotedString,FOLLOW_TkQuotedString_in_singleLineTextLiteral17398); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkQuotedString608_tree = (Object)adaptor.create(TkQuotedString608);
adaptor.addChild(root_0, TkQuotedString608_tree);
}
}
break;
default :
break loop211;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:933:83: ( escapedCharacter )*
loop212:
while (true) {
int alt212=2;
int LA212_0 = input.LA(1);
if ( (LA212_0==DEREFERENCE) ) {
switch ( input.LA(2) ) {
case TkIntNumber:
{
int LA212_4 = input.LA(3);
if ( (synpred294_Delphi()) ) {
alt212=1;
}
}
break;
case TkIdentifier:
{
int LA212_5 = input.LA(3);
if ( (synpred294_Delphi()) ) {
alt212=1;
}
}
break;
case TkAnyChar:
{
int LA212_6 = input.LA(3);
if ( (synpred294_Delphi()) ) {
alt212=1;
}
}
break;
}
}
else if ( (LA212_0==TkCharacterEscapeCode) ) {
int LA212_3 = input.LA(2);
if ( (synpred294_Delphi()) ) {
alt212=1;
}
}
switch (alt212) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:933:83: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_singleLineTextLiteral17402);
escapedCharacter609=escapedCharacter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, escapedCharacter609.getTree());
}
break;
default :
break loop212;
}
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:934:32: ( escapedCharacter )+ ( TkQuotedString ( escapedCharacter )+ )* ( TkQuotedString )?
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:934:32: ( escapedCharacter )+
int cnt213=0;
loop213:
while (true) {
int alt213=2;
int LA213_0 = input.LA(1);
if ( (LA213_0==DEREFERENCE) ) {
int LA213_2 = input.LA(2);
if ( (synpred296_Delphi()) ) {
alt213=1;
}
}
else if ( (LA213_0==TkCharacterEscapeCode) ) {
int LA213_3 = input.LA(2);
if ( (synpred296_Delphi()) ) {
alt213=1;
}
}
switch (alt213) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:934:32: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_singleLineTextLiteral17436);
escapedCharacter610=escapedCharacter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, escapedCharacter610.getTree());
}
break;
default :
if ( cnt213 >= 1 ) break loop213;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(213, input);
throw eee;
}
cnt213++;
}
// au/com/integradev/delphi/antlr/Delphi.g:934:50: ( TkQuotedString ( escapedCharacter )+ )*
loop215:
while (true) {
int alt215=2;
int LA215_0 = input.LA(1);
if ( (LA215_0==TkQuotedString) ) {
int LA215_1 = input.LA(2);
if ( (LA215_1==DEREFERENCE) ) {
switch ( input.LA(3) ) {
case TkIntNumber:
{
int LA215_5 = input.LA(4);
if ( (synpred298_Delphi()) ) {
alt215=1;
}
}
break;
case TkIdentifier:
{
int LA215_6 = input.LA(4);
if ( (synpred298_Delphi()) ) {
alt215=1;
}
}
break;
case TkAnyChar:
{
int LA215_7 = input.LA(4);
if ( (synpred298_Delphi()) ) {
alt215=1;
}
}
break;
}
}
else if ( (LA215_1==TkCharacterEscapeCode) ) {
int LA215_4 = input.LA(3);
if ( (synpred298_Delphi()) ) {
alt215=1;
}
}
}
switch (alt215) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:934:51: TkQuotedString ( escapedCharacter )+
{
TkQuotedString611=(Token)match(input,TkQuotedString,FOLLOW_TkQuotedString_in_singleLineTextLiteral17440); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkQuotedString611_tree = (Object)adaptor.create(TkQuotedString611);
adaptor.addChild(root_0, TkQuotedString611_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:934:66: ( escapedCharacter )+
int cnt214=0;
loop214:
while (true) {
int alt214=2;
int LA214_0 = input.LA(1);
if ( (LA214_0==DEREFERENCE) ) {
switch ( input.LA(2) ) {
case TkIntNumber:
{
int LA214_4 = input.LA(3);
if ( (synpred297_Delphi()) ) {
alt214=1;
}
}
break;
case TkIdentifier:
{
int LA214_5 = input.LA(3);
if ( (synpred297_Delphi()) ) {
alt214=1;
}
}
break;
case TkAnyChar:
{
int LA214_6 = input.LA(3);
if ( (synpred297_Delphi()) ) {
alt214=1;
}
}
break;
}
}
else if ( (LA214_0==TkCharacterEscapeCode) ) {
int LA214_3 = input.LA(2);
if ( (synpred297_Delphi()) ) {
alt214=1;
}
}
switch (alt214) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:934:66: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_singleLineTextLiteral17442);
escapedCharacter612=escapedCharacter();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, escapedCharacter612.getTree());
}
break;
default :
if ( cnt214 >= 1 ) break loop214;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(214, input);
throw eee;
}
cnt214++;
}
}
break;
default :
break loop215;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:934:86: ( TkQuotedString )?
int alt216=2;
int LA216_0 = input.LA(1);
if ( (LA216_0==TkQuotedString) ) {
int LA216_1 = input.LA(2);
if ( (synpred299_Delphi()) ) {
alt216=1;
}
}
switch (alt216) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:934:86: TkQuotedString
{
TkQuotedString613=(Token)match(input,TkQuotedString,FOLLOW_TkQuotedString_in_singleLineTextLiteral17447); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkQuotedString613_tree = (Object)adaptor.create(TkQuotedString613);
adaptor.addChild(root_0, TkQuotedString613_tree);
}
}
break;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 159, singleLineTextLiteral_StartIndex); }
}
return retval;
}
// $ANTLR end "singleLineTextLiteral"
public static class multilineTextLiteral_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "multilineTextLiteral"
// au/com/integradev/delphi/antlr/Delphi.g:936:1: multilineTextLiteral : TkMultilineString ;
public final DelphiParser.multilineTextLiteral_return multilineTextLiteral() throws RecognitionException {
DelphiParser.multilineTextLiteral_return retval = new DelphiParser.multilineTextLiteral_return();
retval.start = input.LT(1);
int multilineTextLiteral_StartIndex = input.index();
Object root_0 = null;
Token TkMultilineString614=null;
Object TkMultilineString614_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 160) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:936:30: ( TkMultilineString )
// au/com/integradev/delphi/antlr/Delphi.g:936:32: TkMultilineString
{
root_0 = (Object)adaptor.nil();
TkMultilineString614=(Token)match(input,TkMultilineString,FOLLOW_TkMultilineString_in_multilineTextLiteral17493); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkMultilineString614_tree = (Object)adaptor.create(TkMultilineString614);
adaptor.addChild(root_0, TkMultilineString614_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 160, multilineTextLiteral_StartIndex); }
}
return retval;
}
// $ANTLR end "multilineTextLiteral"
public static class escapedCharacter_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "escapedCharacter"
// au/com/integradev/delphi/antlr/Delphi.g:938:1: escapedCharacter : ( TkCharacterEscapeCode | '^' ( TkIdentifier | TkIntNumber | TkAnyChar ) -> ^() );
public final DelphiParser.escapedCharacter_return escapedCharacter() throws RecognitionException {
DelphiParser.escapedCharacter_return retval = new DelphiParser.escapedCharacter_return();
retval.start = input.LT(1);
int escapedCharacter_StartIndex = input.index();
Object root_0 = null;
Token TkCharacterEscapeCode615=null;
Token char_literal616=null;
Token TkIdentifier617=null;
Token TkIntNumber618=null;
Token TkAnyChar619=null;
Object TkCharacterEscapeCode615_tree=null;
Object char_literal616_tree=null;
Object TkIdentifier617_tree=null;
Object TkIntNumber618_tree=null;
Object TkAnyChar619_tree=null;
RewriteRuleTokenStream stream_TkIntNumber=new RewriteRuleTokenStream(adaptor,"token TkIntNumber");
RewriteRuleTokenStream stream_DEREFERENCE=new RewriteRuleTokenStream(adaptor,"token DEREFERENCE");
RewriteRuleTokenStream stream_TkIdentifier=new RewriteRuleTokenStream(adaptor,"token TkIdentifier");
RewriteRuleTokenStream stream_TkAnyChar=new RewriteRuleTokenStream(adaptor,"token TkAnyChar");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 161) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:938:30: ( TkCharacterEscapeCode | '^' ( TkIdentifier | TkIntNumber | TkAnyChar ) -> ^() )
int alt219=2;
int LA219_0 = input.LA(1);
if ( (LA219_0==TkCharacterEscapeCode) ) {
alt219=1;
}
else if ( (LA219_0==DEREFERENCE) ) {
alt219=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 219, 0, input);
throw nvae;
}
switch (alt219) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:938:32: TkCharacterEscapeCode
{
root_0 = (Object)adaptor.nil();
TkCharacterEscapeCode615=(Token)match(input,TkCharacterEscapeCode,FOLLOW_TkCharacterEscapeCode_in_escapedCharacter17542); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkCharacterEscapeCode615_tree = (Object)adaptor.create(TkCharacterEscapeCode615);
adaptor.addChild(root_0, TkCharacterEscapeCode615_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:939:32: '^' ( TkIdentifier | TkIntNumber | TkAnyChar )
{
char_literal616=(Token)match(input,DEREFERENCE,FOLLOW_DEREFERENCE_in_escapedCharacter17575); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DEREFERENCE.add(char_literal616);
// au/com/integradev/delphi/antlr/Delphi.g:939:36: ( TkIdentifier | TkIntNumber | TkAnyChar )
int alt218=3;
switch ( input.LA(1) ) {
case TkIdentifier:
{
alt218=1;
}
break;
case TkIntNumber:
{
alt218=2;
}
break;
case TkAnyChar:
{
alt218=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 218, 0, input);
throw nvae;
}
switch (alt218) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:939:37: TkIdentifier
{
TkIdentifier617=(Token)match(input,TkIdentifier,FOLLOW_TkIdentifier_in_escapedCharacter17578); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_TkIdentifier.add(TkIdentifier617);
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:939:52: TkIntNumber
{
TkIntNumber618=(Token)match(input,TkIntNumber,FOLLOW_TkIntNumber_in_escapedCharacter17582); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_TkIntNumber.add(TkIntNumber618);
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:939:66: TkAnyChar
{
TkAnyChar619=(Token)match(input,TkAnyChar,FOLLOW_TkAnyChar_in_escapedCharacter17586); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_TkAnyChar.add(TkAnyChar619);
}
break;
}
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 939:77: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:939:80: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkEscapedCharacter), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 161, escapedCharacter_StartIndex); }
}
return retval;
}
// $ANTLR end "escapedCharacter"
public static class nilLiteral_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "nilLiteral"
// au/com/integradev/delphi/antlr/Delphi.g:941:1: nilLiteral : NIL ;
public final DelphiParser.nilLiteral_return nilLiteral() throws RecognitionException {
DelphiParser.nilLiteral_return retval = new DelphiParser.nilLiteral_return();
retval.start = input.LT(1);
int nilLiteral_StartIndex = input.index();
Object root_0 = null;
Token NIL620=null;
Object NIL620_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 162) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:941:30: ( NIL )
// au/com/integradev/delphi/antlr/Delphi.g:941:32: NIL
{
root_0 = (Object)adaptor.nil();
NIL620=(Token)match(input,NIL,FOLLOW_NIL_in_nilLiteral17648); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NIL620_tree = new NilLiteralNodeImpl(NIL620) ;
adaptor.addChild(root_0, NIL620_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 162, nilLiteral_StartIndex); }
}
return retval;
}
// $ANTLR end "nilLiteral"
public static class arrayConstructor_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayConstructor"
// au/com/integradev/delphi/antlr/Delphi.g:943:1: arrayConstructor : lbrack ( expressionOrRangeList )? rbrack -> ^( TkArrayConstructor lbrack ( expressionOrRangeList )? rbrack ) ;
public final DelphiParser.arrayConstructor_return arrayConstructor() throws RecognitionException {
DelphiParser.arrayConstructor_return retval = new DelphiParser.arrayConstructor_return();
retval.start = input.LT(1);
int arrayConstructor_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope lbrack621 =null;
ParserRuleReturnScope expressionOrRangeList622 =null;
ParserRuleReturnScope rbrack623 =null;
RewriteRuleSubtreeStream stream_rbrack=new RewriteRuleSubtreeStream(adaptor,"rule rbrack");
RewriteRuleSubtreeStream stream_lbrack=new RewriteRuleSubtreeStream(adaptor,"rule lbrack");
RewriteRuleSubtreeStream stream_expressionOrRangeList=new RewriteRuleSubtreeStream(adaptor,"rule expressionOrRangeList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 163) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:943:30: ( lbrack ( expressionOrRangeList )? rbrack -> ^( TkArrayConstructor lbrack ( expressionOrRangeList )? rbrack ) )
// au/com/integradev/delphi/antlr/Delphi.g:943:32: lbrack ( expressionOrRangeList )? rbrack
{
pushFollow(FOLLOW_lbrack_in_arrayConstructor17700);
lbrack621=lbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_lbrack.add(lbrack621.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:943:39: ( expressionOrRangeList )?
int alt220=2;
int LA220_0 = input.LA(1);
if ( ((LA220_0 >= ABSOLUTE && LA220_0 <= ALIGN)||(LA220_0 >= ASSEMBLER && LA220_0 <= ASSEMBLY)||(LA220_0 >= AT && LA220_0 <= AUTOMATED)||LA220_0==CDECL||LA220_0==CONTAINS||(LA220_0 >= DEFAULT && LA220_0 <= DEREFERENCE)||LA220_0==DISPID||LA220_0==DYNAMIC||(LA220_0 >= EXPERIMENTAL && LA220_0 <= EXPORT)||LA220_0==EXTERNAL||(LA220_0 >= FAR && LA220_0 <= FINAL)||(LA220_0 >= FORWARD && LA220_0 <= FUNCTION)||LA220_0==HELPER||LA220_0==IMPLEMENTS||(LA220_0 >= INDEX && LA220_0 <= INHERITED)||LA220_0==LABEL||LA220_0==LOCAL||(LA220_0 >= MESSAGE && LA220_0 <= MINUS)||(LA220_0 >= NAME && LA220_0 <= NOT)||(LA220_0 >= ON && LA220_0 <= OPERATOR)||(LA220_0 >= OUT && LA220_0 <= OVERRIDE)||LA220_0==PACKAGE||LA220_0==PAREN_BRACKET_LEFT||LA220_0==PAREN_LEFT||(LA220_0 >= PASCAL && LA220_0 <= PROCEDURE)||(LA220_0 >= PROTECTED && LA220_0 <= PUBLISHED)||(LA220_0 >= READ && LA220_0 <= READONLY)||(LA220_0 >= REFERENCE && LA220_0 <= REINTRODUCE)||(LA220_0 >= REQUIRES && LA220_0 <= RESIDENT)||(LA220_0 >= SAFECALL && LA220_0 <= SEALED)||LA220_0==SQUARE_BRACKET_LEFT||(LA220_0 >= STATIC && LA220_0 <= STRING)||LA220_0==TkBinaryNumber||LA220_0==TkCharacterEscapeCode||(LA220_0 >= TkHexNumber && LA220_0 <= TkIntNumber)||LA220_0==TkMultilineString||(LA220_0 >= TkQuotedString && LA220_0 <= TkRealNumber)||LA220_0==UNSAFE||(LA220_0 >= VARARGS && LA220_0 <= VIRTUAL)||LA220_0==WINAPI||(LA220_0 >= WRITE && LA220_0 <= WRITEONLY)) ) {
alt220=1;
}
switch (alt220) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:943:39: expressionOrRangeList
{
pushFollow(FOLLOW_expressionOrRangeList_in_arrayConstructor17702);
expressionOrRangeList622=expressionOrRangeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expressionOrRangeList.add(expressionOrRangeList622.getTree());
}
break;
}
pushFollow(FOLLOW_rbrack_in_arrayConstructor17705);
rbrack623=rbrack();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_rbrack.add(rbrack623.getTree());
// AST REWRITE
// elements: lbrack, expressionOrRangeList, rbrack
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 944:30: -> ^( TkArrayConstructor lbrack ( expressionOrRangeList )? rbrack )
{
// au/com/integradev/delphi/antlr/Delphi.g:944:33: ^( TkArrayConstructor lbrack ( expressionOrRangeList )? rbrack )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ArrayConstructorNodeImpl(TkArrayConstructor), root_1);
adaptor.addChild(root_1, stream_lbrack.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:944:87: ( expressionOrRangeList )?
if ( stream_expressionOrRangeList.hasNext() ) {
adaptor.addChild(root_1, stream_expressionOrRangeList.nextTree());
}
stream_expressionOrRangeList.reset();
adaptor.addChild(root_1, stream_rbrack.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 163, arrayConstructor_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayConstructor"
public static class addOperator_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "addOperator"
// au/com/integradev/delphi/antlr/Delphi.g:946:1: addOperator : ( '+' | '-' | OR | XOR );
public final DelphiParser.addOperator_return addOperator() throws RecognitionException {
DelphiParser.addOperator_return retval = new DelphiParser.addOperator_return();
retval.start = input.LT(1);
int addOperator_StartIndex = input.index();
Object root_0 = null;
Token char_literal624=null;
Token char_literal625=null;
Token OR626=null;
Token XOR627=null;
Object char_literal624_tree=null;
Object char_literal625_tree=null;
Object OR626_tree=null;
Object XOR627_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 164) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:946:30: ( '+' | '-' | OR | XOR )
int alt221=4;
switch ( input.LA(1) ) {
case PLUS:
{
alt221=1;
}
break;
case MINUS:
{
alt221=2;
}
break;
case OR:
{
alt221=3;
}
break;
case XOR:
{
alt221=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 221, 0, input);
throw nvae;
}
switch (alt221) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:946:32: '+'
{
root_0 = (Object)adaptor.nil();
char_literal624=(Token)match(input,PLUS,FOLLOW_PLUS_in_addOperator17804); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal624_tree = new BinaryExpressionNodeImpl(char_literal624) ;
adaptor.addChild(root_0, char_literal624_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:947:32: '-'
{
root_0 = (Object)adaptor.nil();
char_literal625=(Token)match(input,MINUS,FOLLOW_MINUS_in_addOperator17840); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal625_tree = new BinaryExpressionNodeImpl(char_literal625) ;
adaptor.addChild(root_0, char_literal625_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:948:32: OR
{
root_0 = (Object)adaptor.nil();
OR626=(Token)match(input,OR,FOLLOW_OR_in_addOperator17876); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OR626_tree = new BinaryExpressionNodeImpl(OR626) ;
adaptor.addChild(root_0, OR626_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:949:32: XOR
{
root_0 = (Object)adaptor.nil();
XOR627=(Token)match(input,XOR,FOLLOW_XOR_in_addOperator17912); if (state.failed) return retval;
if ( state.backtracking==0 ) {
XOR627_tree = new BinaryExpressionNodeImpl(XOR627) ;
adaptor.addChild(root_0, XOR627_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 164, addOperator_StartIndex); }
}
return retval;
}
// $ANTLR end "addOperator"
public static class multOperator_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "multOperator"
// au/com/integradev/delphi/antlr/Delphi.g:951:1: multOperator : ( '*' | '/' | DIV | MOD | AND | SHL | SHR | AS );
public final DelphiParser.multOperator_return multOperator() throws RecognitionException {
DelphiParser.multOperator_return retval = new DelphiParser.multOperator_return();
retval.start = input.LT(1);
int multOperator_StartIndex = input.index();
Object root_0 = null;
Token char_literal628=null;
Token char_literal629=null;
Token DIV630=null;
Token MOD631=null;
Token AND632=null;
Token SHL633=null;
Token SHR634=null;
Token AS635=null;
Object char_literal628_tree=null;
Object char_literal629_tree=null;
Object DIV630_tree=null;
Object MOD631_tree=null;
Object AND632_tree=null;
Object SHL633_tree=null;
Object SHR634_tree=null;
Object AS635_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 165) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:951:30: ( '*' | '/' | DIV | MOD | AND | SHL | SHR | AS )
int alt222=8;
switch ( input.LA(1) ) {
case MULTIPLY:
{
alt222=1;
}
break;
case DIVIDE:
{
alt222=2;
}
break;
case DIV:
{
alt222=3;
}
break;
case MOD:
{
alt222=4;
}
break;
case AND:
{
alt222=5;
}
break;
case SHL:
{
alt222=6;
}
break;
case SHR:
{
alt222=7;
}
break;
case AS:
{
alt222=8;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 222, 0, input);
throw nvae;
}
switch (alt222) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:951:32: '*'
{
root_0 = (Object)adaptor.nil();
char_literal628=(Token)match(input,MULTIPLY,FOLLOW_MULTIPLY_in_multOperator17968); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal628_tree = new BinaryExpressionNodeImpl(char_literal628) ;
adaptor.addChild(root_0, char_literal628_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:952:32: '/'
{
root_0 = (Object)adaptor.nil();
char_literal629=(Token)match(input,DIVIDE,FOLLOW_DIVIDE_in_multOperator18004); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal629_tree = new BinaryExpressionNodeImpl(char_literal629) ;
adaptor.addChild(root_0, char_literal629_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:953:32: DIV
{
root_0 = (Object)adaptor.nil();
DIV630=(Token)match(input,DIV,FOLLOW_DIV_in_multOperator18040); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DIV630_tree = new BinaryExpressionNodeImpl(DIV630) ;
adaptor.addChild(root_0, DIV630_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:954:32: MOD
{
root_0 = (Object)adaptor.nil();
MOD631=(Token)match(input,MOD,FOLLOW_MOD_in_multOperator18076); if (state.failed) return retval;
if ( state.backtracking==0 ) {
MOD631_tree = new BinaryExpressionNodeImpl(MOD631) ;
adaptor.addChild(root_0, MOD631_tree);
}
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:955:32: AND
{
root_0 = (Object)adaptor.nil();
AND632=(Token)match(input,AND,FOLLOW_AND_in_multOperator18112); if (state.failed) return retval;
if ( state.backtracking==0 ) {
AND632_tree = new BinaryExpressionNodeImpl(AND632) ;
adaptor.addChild(root_0, AND632_tree);
}
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:956:32: SHL
{
root_0 = (Object)adaptor.nil();
SHL633=(Token)match(input,SHL,FOLLOW_SHL_in_multOperator18148); if (state.failed) return retval;
if ( state.backtracking==0 ) {
SHL633_tree = new BinaryExpressionNodeImpl(SHL633) ;
adaptor.addChild(root_0, SHL633_tree);
}
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:957:32: SHR
{
root_0 = (Object)adaptor.nil();
SHR634=(Token)match(input,SHR,FOLLOW_SHR_in_multOperator18184); if (state.failed) return retval;
if ( state.backtracking==0 ) {
SHR634_tree = new BinaryExpressionNodeImpl(SHR634) ;
adaptor.addChild(root_0, SHR634_tree);
}
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:958:32: AS
{
root_0 = (Object)adaptor.nil();
AS635=(Token)match(input,AS,FOLLOW_AS_in_multOperator18220); if (state.failed) return retval;
if ( state.backtracking==0 ) {
AS635_tree = new BinaryExpressionNodeImpl(AS635) ;
adaptor.addChild(root_0, AS635_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 165, multOperator_StartIndex); }
}
return retval;
}
// $ANTLR end "multOperator"
public static class unaryOperator_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "unaryOperator"
// au/com/integradev/delphi/antlr/Delphi.g:960:1: unaryOperator : ( NOT | '+' | '-' | '@' );
public final DelphiParser.unaryOperator_return unaryOperator() throws RecognitionException {
DelphiParser.unaryOperator_return retval = new DelphiParser.unaryOperator_return();
retval.start = input.LT(1);
int unaryOperator_StartIndex = input.index();
Object root_0 = null;
Token NOT636=null;
Token char_literal637=null;
Token char_literal638=null;
Token char_literal639=null;
Object NOT636_tree=null;
Object char_literal637_tree=null;
Object char_literal638_tree=null;
Object char_literal639_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 166) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:960:30: ( NOT | '+' | '-' | '@' )
int alt223=4;
switch ( input.LA(1) ) {
case NOT:
{
alt223=1;
}
break;
case PLUS:
{
alt223=2;
}
break;
case MINUS:
{
alt223=3;
}
break;
case ADDRESS:
{
alt223=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 223, 0, input);
throw nvae;
}
switch (alt223) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:960:32: NOT
{
root_0 = (Object)adaptor.nil();
NOT636=(Token)match(input,NOT,FOLLOW_NOT_in_unaryOperator18275); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NOT636_tree = new UnaryExpressionNodeImpl(NOT636) ;
adaptor.addChild(root_0, NOT636_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:961:32: '+'
{
root_0 = (Object)adaptor.nil();
char_literal637=(Token)match(input,PLUS,FOLLOW_PLUS_in_unaryOperator18311); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal637_tree = new UnaryExpressionNodeImpl(char_literal637) ;
adaptor.addChild(root_0, char_literal637_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:962:32: '-'
{
root_0 = (Object)adaptor.nil();
char_literal638=(Token)match(input,MINUS,FOLLOW_MINUS_in_unaryOperator18347); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal638_tree = new UnaryExpressionNodeImpl(char_literal638) ;
adaptor.addChild(root_0, char_literal638_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:963:32: '@'
{
root_0 = (Object)adaptor.nil();
char_literal639=(Token)match(input,ADDRESS,FOLLOW_ADDRESS_in_unaryOperator18383); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal639_tree = new UnaryExpressionNodeImpl(char_literal639) ;
adaptor.addChild(root_0, char_literal639_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 166, unaryOperator_StartIndex); }
}
return retval;
}
// $ANTLR end "unaryOperator"
public static class relationalOperator_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "relationalOperator"
// au/com/integradev/delphi/antlr/Delphi.g:965:1: relationalOperator : ( '=' | '>' | '<' | '<=' | '>=' | '<>' | IN | IS );
public final DelphiParser.relationalOperator_return relationalOperator() throws RecognitionException {
DelphiParser.relationalOperator_return retval = new DelphiParser.relationalOperator_return();
retval.start = input.LT(1);
int relationalOperator_StartIndex = input.index();
Object root_0 = null;
Token char_literal640=null;
Token char_literal641=null;
Token char_literal642=null;
Token string_literal643=null;
Token string_literal644=null;
Token string_literal645=null;
Token IN646=null;
Token IS647=null;
Object char_literal640_tree=null;
Object char_literal641_tree=null;
Object char_literal642_tree=null;
Object string_literal643_tree=null;
Object string_literal644_tree=null;
Object string_literal645_tree=null;
Object IN646_tree=null;
Object IS647_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 167) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:965:30: ( '=' | '>' | '<' | '<=' | '>=' | '<>' | IN | IS )
int alt224=8;
switch ( input.LA(1) ) {
case EQUAL:
{
alt224=1;
}
break;
case GREATER_THAN:
{
alt224=2;
}
break;
case LESS_THAN:
{
alt224=3;
}
break;
case LESS_THAN_EQUAL:
{
alt224=4;
}
break;
case GREATER_THAN_EQUAL:
{
alt224=5;
}
break;
case NOT_EQUAL:
{
alt224=6;
}
break;
case IN:
{
alt224=7;
}
break;
case IS:
{
alt224=8;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 224, 0, input);
throw nvae;
}
switch (alt224) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:965:32: '='
{
root_0 = (Object)adaptor.nil();
char_literal640=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_relationalOperator18433); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal640_tree = new BinaryExpressionNodeImpl(char_literal640) ;
adaptor.addChild(root_0, char_literal640_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:966:32: '>'
{
root_0 = (Object)adaptor.nil();
char_literal641=(Token)match(input,GREATER_THAN,FOLLOW_GREATER_THAN_in_relationalOperator18469); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal641_tree = new BinaryExpressionNodeImpl(char_literal641) ;
adaptor.addChild(root_0, char_literal641_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:967:32: '<'
{
root_0 = (Object)adaptor.nil();
char_literal642=(Token)match(input,LESS_THAN,FOLLOW_LESS_THAN_in_relationalOperator18505); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal642_tree = new BinaryExpressionNodeImpl(char_literal642) ;
adaptor.addChild(root_0, char_literal642_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:968:32: '<='
{
root_0 = (Object)adaptor.nil();
string_literal643=(Token)match(input,LESS_THAN_EQUAL,FOLLOW_LESS_THAN_EQUAL_in_relationalOperator18541); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal643_tree = new BinaryExpressionNodeImpl(string_literal643) ;
adaptor.addChild(root_0, string_literal643_tree);
}
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:969:32: '>='
{
root_0 = (Object)adaptor.nil();
string_literal644=(Token)match(input,GREATER_THAN_EQUAL,FOLLOW_GREATER_THAN_EQUAL_in_relationalOperator18577); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal644_tree = new BinaryExpressionNodeImpl(string_literal644) ;
adaptor.addChild(root_0, string_literal644_tree);
}
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:970:32: '<>'
{
root_0 = (Object)adaptor.nil();
string_literal645=(Token)match(input,NOT_EQUAL,FOLLOW_NOT_EQUAL_in_relationalOperator18613); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal645_tree = new BinaryExpressionNodeImpl(string_literal645) ;
adaptor.addChild(root_0, string_literal645_tree);
}
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:971:32: IN
{
root_0 = (Object)adaptor.nil();
IN646=(Token)match(input,IN,FOLLOW_IN_in_relationalOperator18649); if (state.failed) return retval;
if ( state.backtracking==0 ) {
IN646_tree = new BinaryExpressionNodeImpl(IN646) ;
adaptor.addChild(root_0, IN646_tree);
}
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:972:32: IS
{
root_0 = (Object)adaptor.nil();
IS647=(Token)match(input,IS,FOLLOW_IS_in_relationalOperator18685); if (state.failed) return retval;
if ( state.backtracking==0 ) {
IS647_tree = new BinaryExpressionNodeImpl(IS647) ;
adaptor.addChild(root_0, IS647_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 167, relationalOperator_StartIndex); }
}
return retval;
}
// $ANTLR end "relationalOperator"
public static class constExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "constExpression"
// au/com/integradev/delphi/antlr/Delphi.g:974:1: constExpression : ( expression | recordExpression | arrayExpression );
public final DelphiParser.constExpression_return constExpression() throws RecognitionException {
DelphiParser.constExpression_return retval = new DelphiParser.constExpression_return();
retval.start = input.LT(1);
int constExpression_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope expression648 =null;
ParserRuleReturnScope recordExpression649 =null;
ParserRuleReturnScope arrayExpression650 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 168) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:974:30: ( expression | recordExpression | arrayExpression )
int alt225=3;
int LA225_0 = input.LA(1);
if ( ((LA225_0 >= ABSOLUTE && LA225_0 <= ALIGN)||(LA225_0 >= ASSEMBLER && LA225_0 <= ASSEMBLY)||(LA225_0 >= AT && LA225_0 <= AUTOMATED)||LA225_0==CDECL||LA225_0==CONTAINS||(LA225_0 >= DEFAULT && LA225_0 <= DEREFERENCE)||LA225_0==DISPID||LA225_0==DYNAMIC||(LA225_0 >= EXPERIMENTAL && LA225_0 <= EXPORT)||LA225_0==EXTERNAL||(LA225_0 >= FAR && LA225_0 <= FINAL)||(LA225_0 >= FORWARD && LA225_0 <= FUNCTION)||LA225_0==HELPER||LA225_0==IMPLEMENTS||(LA225_0 >= INDEX && LA225_0 <= INHERITED)||LA225_0==LABEL||LA225_0==LOCAL||(LA225_0 >= MESSAGE && LA225_0 <= MINUS)||(LA225_0 >= NAME && LA225_0 <= NOT)||(LA225_0 >= ON && LA225_0 <= OPERATOR)||(LA225_0 >= OUT && LA225_0 <= OVERRIDE)||LA225_0==PACKAGE||LA225_0==PAREN_BRACKET_LEFT||(LA225_0 >= PASCAL && LA225_0 <= PROCEDURE)||(LA225_0 >= PROTECTED && LA225_0 <= PUBLISHED)||(LA225_0 >= READ && LA225_0 <= READONLY)||(LA225_0 >= REFERENCE && LA225_0 <= REINTRODUCE)||(LA225_0 >= REQUIRES && LA225_0 <= RESIDENT)||(LA225_0 >= SAFECALL && LA225_0 <= SEALED)||LA225_0==SQUARE_BRACKET_LEFT||(LA225_0 >= STATIC && LA225_0 <= STRING)||LA225_0==TkBinaryNumber||LA225_0==TkCharacterEscapeCode||(LA225_0 >= TkHexNumber && LA225_0 <= TkIntNumber)||LA225_0==TkMultilineString||(LA225_0 >= TkQuotedString && LA225_0 <= TkRealNumber)||LA225_0==UNSAFE||(LA225_0 >= VARARGS && LA225_0 <= VIRTUAL)||LA225_0==WINAPI||(LA225_0 >= WRITE && LA225_0 <= WRITEONLY)) ) {
alt225=1;
}
else if ( (LA225_0==PAREN_LEFT) ) {
int LA225_19 = input.LA(2);
if ( (synpred324_Delphi()) ) {
alt225=1;
}
else if ( (synpred325_Delphi()) ) {
alt225=2;
}
else if ( (true) ) {
alt225=3;
}
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 225, 0, input);
throw nvae;
}
switch (alt225) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:974:32: expression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_expression_in_constExpression18738);
expression648=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression648.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:975:32: recordExpression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_recordExpression_in_constExpression18771);
recordExpression649=recordExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordExpression649.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:976:32: arrayExpression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_arrayExpression_in_constExpression18804);
arrayExpression650=arrayExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, arrayExpression650.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 168, constExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "constExpression"
public static class recordExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordExpression"
// au/com/integradev/delphi/antlr/Delphi.g:978:1: recordExpression : '(' ^ ( recordExpressionItem ( ';' )? )+ ')' ;
public final DelphiParser.recordExpression_return recordExpression() throws RecognitionException {
DelphiParser.recordExpression_return retval = new DelphiParser.recordExpression_return();
retval.start = input.LT(1);
int recordExpression_StartIndex = input.index();
Object root_0 = null;
Token char_literal651=null;
Token char_literal653=null;
Token char_literal654=null;
ParserRuleReturnScope recordExpressionItem652 =null;
Object char_literal651_tree=null;
Object char_literal653_tree=null;
Object char_literal654_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 169) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:978:30: ( '(' ^ ( recordExpressionItem ( ';' )? )+ ')' )
// au/com/integradev/delphi/antlr/Delphi.g:978:32: '(' ^ ( recordExpressionItem ( ';' )? )+ ')'
{
root_0 = (Object)adaptor.nil();
char_literal651=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_recordExpression18853); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal651_tree = new RecordExpressionNodeImpl(char_literal651) ;
root_0 = (Object)adaptor.becomeRoot(char_literal651_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:978:63: ( recordExpressionItem ( ';' )? )+
int cnt227=0;
loop227:
while (true) {
int alt227=2;
int LA227_0 = input.LA(1);
if ( ((LA227_0 >= ABSOLUTE && LA227_0 <= ABSTRACT)||LA227_0==ALIGN||(LA227_0 >= ASSEMBLER && LA227_0 <= ASSEMBLY)||(LA227_0 >= AT && LA227_0 <= AUTOMATED)||LA227_0==CDECL||LA227_0==CONTAINS||(LA227_0 >= DEFAULT && LA227_0 <= DEPRECATED)||LA227_0==DISPID||LA227_0==DYNAMIC||(LA227_0 >= EXPERIMENTAL && LA227_0 <= EXPORT)||LA227_0==EXTERNAL||LA227_0==FAR||LA227_0==FINAL||LA227_0==FORWARD||LA227_0==HELPER||LA227_0==IMPLEMENTS||LA227_0==INDEX||LA227_0==LABEL||LA227_0==LOCAL||LA227_0==MESSAGE||(LA227_0 >= NAME && LA227_0 <= NEAR)||LA227_0==NODEFAULT||(LA227_0 >= ON && LA227_0 <= OPERATOR)||(LA227_0 >= OUT && LA227_0 <= OVERRIDE)||LA227_0==PACKAGE||(LA227_0 >= PASCAL && LA227_0 <= PLATFORM)||LA227_0==PRIVATE||(LA227_0 >= PROTECTED && LA227_0 <= PUBLISHED)||(LA227_0 >= READ && LA227_0 <= READONLY)||(LA227_0 >= REFERENCE && LA227_0 <= REINTRODUCE)||(LA227_0 >= REQUIRES && LA227_0 <= RESIDENT)||(LA227_0 >= SAFECALL && LA227_0 <= SEALED)||(LA227_0 >= STATIC && LA227_0 <= STRICT)||LA227_0==TkIdentifier||LA227_0==UNSAFE||(LA227_0 >= VARARGS && LA227_0 <= VIRTUAL)||LA227_0==WINAPI||(LA227_0 >= WRITE && LA227_0 <= WRITEONLY)) ) {
alt227=1;
}
switch (alt227) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:978:64: recordExpressionItem ( ';' )?
{
pushFollow(FOLLOW_recordExpressionItem_in_recordExpression18860);
recordExpressionItem652=recordExpressionItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, recordExpressionItem652.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:978:85: ( ';' )?
int alt226=2;
int LA226_0 = input.LA(1);
if ( (LA226_0==SEMICOLON) ) {
alt226=1;
}
switch (alt226) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:978:86: ';'
{
char_literal653=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_recordExpression18863); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal653_tree = (Object)adaptor.create(char_literal653);
adaptor.addChild(root_0, char_literal653_tree);
}
}
break;
}
}
break;
default :
if ( cnt227 >= 1 ) break loop227;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(227, input);
throw eee;
}
cnt227++;
}
char_literal654=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_recordExpression18869); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal654_tree = (Object)adaptor.create(char_literal654);
adaptor.addChild(root_0, char_literal654_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 169, recordExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "recordExpression"
public static class recordExpressionItem_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "recordExpressionItem"
// au/com/integradev/delphi/antlr/Delphi.g:980:1: recordExpressionItem : ident ':' constExpression -> ^( TkRecordExpressionItem ident constExpression ) ;
public final DelphiParser.recordExpressionItem_return recordExpressionItem() throws RecognitionException {
DelphiParser.recordExpressionItem_return retval = new DelphiParser.recordExpressionItem_return();
retval.start = input.LT(1);
int recordExpressionItem_StartIndex = input.index();
Object root_0 = null;
Token char_literal656=null;
ParserRuleReturnScope ident655 =null;
ParserRuleReturnScope constExpression657 =null;
Object char_literal656_tree=null;
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
RewriteRuleSubtreeStream stream_constExpression=new RewriteRuleSubtreeStream(adaptor,"rule constExpression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 170) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:980:30: ( ident ':' constExpression -> ^( TkRecordExpressionItem ident constExpression ) )
// au/com/integradev/delphi/antlr/Delphi.g:980:32: ident ':' constExpression
{
pushFollow(FOLLOW_ident_in_recordExpressionItem18914);
ident655=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident655.getTree());
char_literal656=(Token)match(input,COLON,FOLLOW_COLON_in_recordExpressionItem18916); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal656);
pushFollow(FOLLOW_constExpression_in_recordExpressionItem18918);
constExpression657=constExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_constExpression.add(constExpression657.getTree());
// AST REWRITE
// elements: ident, constExpression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 981:30: -> ^( TkRecordExpressionItem ident constExpression )
{
// au/com/integradev/delphi/antlr/Delphi.g:981:33: ^( TkRecordExpressionItem ident constExpression )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new RecordExpressionItemNodeImpl(TkRecordExpressionItem), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
adaptor.addChild(root_1, stream_constExpression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 170, recordExpressionItem_StartIndex); }
}
return retval;
}
// $ANTLR end "recordExpressionItem"
public static class arrayExpression_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "arrayExpression"
// au/com/integradev/delphi/antlr/Delphi.g:983:1: arrayExpression : '(' ^ ( constExpression ( ',' !)? )* ')' ;
public final DelphiParser.arrayExpression_return arrayExpression() throws RecognitionException {
DelphiParser.arrayExpression_return retval = new DelphiParser.arrayExpression_return();
retval.start = input.LT(1);
int arrayExpression_StartIndex = input.index();
Object root_0 = null;
Token char_literal658=null;
Token char_literal660=null;
Token char_literal661=null;
ParserRuleReturnScope constExpression659 =null;
Object char_literal658_tree=null;
Object char_literal660_tree=null;
Object char_literal661_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 171) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:983:30: ( '(' ^ ( constExpression ( ',' !)? )* ')' )
// au/com/integradev/delphi/antlr/Delphi.g:983:32: '(' ^ ( constExpression ( ',' !)? )* ')'
{
root_0 = (Object)adaptor.nil();
char_literal658=(Token)match(input,PAREN_LEFT,FOLLOW_PAREN_LEFT_in_arrayExpression19010); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal658_tree = new ArrayExpressionNodeImpl(char_literal658) ;
root_0 = (Object)adaptor.becomeRoot(char_literal658_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:983:62: ( constExpression ( ',' !)? )*
loop229:
while (true) {
int alt229=2;
int LA229_0 = input.LA(1);
if ( ((LA229_0 >= ABSOLUTE && LA229_0 <= ALIGN)||(LA229_0 >= ASSEMBLER && LA229_0 <= ASSEMBLY)||(LA229_0 >= AT && LA229_0 <= AUTOMATED)||LA229_0==CDECL||LA229_0==CONTAINS||(LA229_0 >= DEFAULT && LA229_0 <= DEREFERENCE)||LA229_0==DISPID||LA229_0==DYNAMIC||(LA229_0 >= EXPERIMENTAL && LA229_0 <= EXPORT)||LA229_0==EXTERNAL||(LA229_0 >= FAR && LA229_0 <= FINAL)||(LA229_0 >= FORWARD && LA229_0 <= FUNCTION)||LA229_0==HELPER||LA229_0==IMPLEMENTS||(LA229_0 >= INDEX && LA229_0 <= INHERITED)||LA229_0==LABEL||LA229_0==LOCAL||(LA229_0 >= MESSAGE && LA229_0 <= MINUS)||(LA229_0 >= NAME && LA229_0 <= NOT)||(LA229_0 >= ON && LA229_0 <= OPERATOR)||(LA229_0 >= OUT && LA229_0 <= OVERRIDE)||LA229_0==PACKAGE||LA229_0==PAREN_BRACKET_LEFT||LA229_0==PAREN_LEFT||(LA229_0 >= PASCAL && LA229_0 <= PROCEDURE)||(LA229_0 >= PROTECTED && LA229_0 <= PUBLISHED)||(LA229_0 >= READ && LA229_0 <= READONLY)||(LA229_0 >= REFERENCE && LA229_0 <= REINTRODUCE)||(LA229_0 >= REQUIRES && LA229_0 <= RESIDENT)||(LA229_0 >= SAFECALL && LA229_0 <= SEALED)||LA229_0==SQUARE_BRACKET_LEFT||(LA229_0 >= STATIC && LA229_0 <= STRING)||LA229_0==TkBinaryNumber||LA229_0==TkCharacterEscapeCode||(LA229_0 >= TkHexNumber && LA229_0 <= TkIntNumber)||LA229_0==TkMultilineString||(LA229_0 >= TkQuotedString && LA229_0 <= TkRealNumber)||LA229_0==UNSAFE||(LA229_0 >= VARARGS && LA229_0 <= VIRTUAL)||LA229_0==WINAPI||(LA229_0 >= WRITE && LA229_0 <= WRITEONLY)) ) {
alt229=1;
}
switch (alt229) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:983:63: constExpression ( ',' !)?
{
pushFollow(FOLLOW_constExpression_in_arrayExpression19017);
constExpression659=constExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constExpression659.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:983:79: ( ',' !)?
int alt228=2;
int LA228_0 = input.LA(1);
if ( (LA228_0==COMMA) ) {
alt228=1;
}
switch (alt228) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:983:80: ',' !
{
char_literal660=(Token)match(input,COMMA,FOLLOW_COMMA_in_arrayExpression19020); if (state.failed) return retval;
}
break;
}
}
break;
default :
break loop229;
}
}
char_literal661=(Token)match(input,PAREN_RIGHT,FOLLOW_PAREN_RIGHT_in_arrayExpression19027); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal661_tree = (Object)adaptor.create(char_literal661);
adaptor.addChild(root_0, char_literal661_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 171, arrayExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "arrayExpression"
public static class statement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "statement"
// au/com/integradev/delphi/antlr/Delphi.g:989:1: statement : ( ifStatement | varStatement | constStatement | caseStatement | repeatStatement | whileStatement | forStatement | withStatement | tryStatement | raiseStatement | assemblerStatement | compoundStatement | labelStatement | assignmentStatement | expressionStatement | gotoStatement );
public final DelphiParser.statement_return statement() throws RecognitionException {
DelphiParser.statement_return retval = new DelphiParser.statement_return();
retval.start = input.LT(1);
int statement_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope ifStatement662 =null;
ParserRuleReturnScope varStatement663 =null;
ParserRuleReturnScope constStatement664 =null;
ParserRuleReturnScope caseStatement665 =null;
ParserRuleReturnScope repeatStatement666 =null;
ParserRuleReturnScope whileStatement667 =null;
ParserRuleReturnScope forStatement668 =null;
ParserRuleReturnScope withStatement669 =null;
ParserRuleReturnScope tryStatement670 =null;
ParserRuleReturnScope raiseStatement671 =null;
ParserRuleReturnScope assemblerStatement672 =null;
ParserRuleReturnScope compoundStatement673 =null;
ParserRuleReturnScope labelStatement674 =null;
ParserRuleReturnScope assignmentStatement675 =null;
ParserRuleReturnScope expressionStatement676 =null;
ParserRuleReturnScope gotoStatement677 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 172) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:989:30: ( ifStatement | varStatement | constStatement | caseStatement | repeatStatement | whileStatement | forStatement | withStatement | tryStatement | raiseStatement | assemblerStatement | compoundStatement | labelStatement | assignmentStatement | expressionStatement | gotoStatement )
int alt230=16;
switch ( input.LA(1) ) {
case IF:
{
alt230=1;
}
break;
case VAR:
{
alt230=2;
}
break;
case CONST:
{
alt230=3;
}
break;
case CASE:
{
alt230=4;
}
break;
case REPEAT:
{
alt230=5;
}
break;
case WHILE:
{
alt230=6;
}
break;
case FOR:
{
alt230=7;
}
break;
case WITH:
{
alt230=8;
}
break;
case TRY:
{
alt230=9;
}
break;
case RAISE:
{
alt230=10;
}
break;
case ASM:
{
alt230=11;
}
break;
case BEGIN:
{
alt230=12;
}
break;
case TkIdentifier:
{
int LA230_13 = input.LA(2);
if ( (((input.LA(2) == COLON)&&synpred342_Delphi())) ) {
alt230=13;
}
else if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 13, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA230_14 = input.LA(2);
if ( (((input.LA(2) == COLON)&&synpred342_Delphi())) ) {
alt230=13;
}
else if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 14, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkIntNumber:
{
int LA230_15 = input.LA(2);
if ( (((input.LA(2) == COLON)&&synpred342_Delphi())) ) {
alt230=13;
}
else if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 15, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkHexNumber:
{
int LA230_16 = input.LA(2);
if ( (((input.LA(2) == COLON)&&synpred342_Delphi())) ) {
alt230=13;
}
else if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 16, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkBinaryNumber:
{
int LA230_17 = input.LA(2);
if ( (((input.LA(2) == COLON)&&synpred342_Delphi())) ) {
alt230=13;
}
else if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 17, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case NOT:
{
int LA230_18 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 18, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PLUS:
{
int LA230_19 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 19, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case MINUS:
{
int LA230_20 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 20, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case ADDRESS:
{
int LA230_21 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 21, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkRealNumber:
{
int LA230_22 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 22, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkQuotedString:
{
int LA230_23 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 23, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkCharacterEscapeCode:
{
int LA230_24 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 24, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case DEREFERENCE:
{
int LA230_25 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 25, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case TkMultilineString:
{
int LA230_26 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 26, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case NIL:
{
int LA230_27 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 27, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA230_28 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 28, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case STRING:
{
int LA230_29 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 29, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FILE:
{
int LA230_30 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 30, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PAREN_LEFT:
{
int LA230_31 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 31, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case INHERITED:
{
int LA230_32 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 32, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case PROCEDURE:
{
int LA230_33 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 33, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FUNCTION:
{
int LA230_34 = input.LA(2);
if ( (synpred343_Delphi()) ) {
alt230=14;
}
else if ( (synpred344_Delphi()) ) {
alt230=15;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 230, 34, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case GOTO:
{
alt230=16;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 230, 0, input);
throw nvae;
}
switch (alt230) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:989:32: ifStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_ifStatement_in_statement19087);
ifStatement662=ifStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ifStatement662.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:990:32: varStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_varStatement_in_statement19120);
varStatement663=varStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, varStatement663.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:991:32: constStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_constStatement_in_statement19153);
constStatement664=constStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, constStatement664.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:992:32: caseStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_caseStatement_in_statement19186);
caseStatement665=caseStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, caseStatement665.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:993:32: repeatStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_repeatStatement_in_statement19219);
repeatStatement666=repeatStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, repeatStatement666.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:994:32: whileStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_whileStatement_in_statement19252);
whileStatement667=whileStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, whileStatement667.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:995:32: forStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_forStatement_in_statement19285);
forStatement668=forStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, forStatement668.getTree());
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:996:32: withStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_withStatement_in_statement19318);
withStatement669=withStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, withStatement669.getTree());
}
break;
case 9 :
// au/com/integradev/delphi/antlr/Delphi.g:997:32: tryStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_tryStatement_in_statement19351);
tryStatement670=tryStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, tryStatement670.getTree());
}
break;
case 10 :
// au/com/integradev/delphi/antlr/Delphi.g:998:32: raiseStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_raiseStatement_in_statement19384);
raiseStatement671=raiseStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, raiseStatement671.getTree());
}
break;
case 11 :
// au/com/integradev/delphi/antlr/Delphi.g:999:32: assemblerStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_assemblerStatement_in_statement19417);
assemblerStatement672=assemblerStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, assemblerStatement672.getTree());
}
break;
case 12 :
// au/com/integradev/delphi/antlr/Delphi.g:1000:32: compoundStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_compoundStatement_in_statement19450);
compoundStatement673=compoundStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, compoundStatement673.getTree());
}
break;
case 13 :
// au/com/integradev/delphi/antlr/Delphi.g:1001:32: labelStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_labelStatement_in_statement19483);
labelStatement674=labelStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, labelStatement674.getTree());
}
break;
case 14 :
// au/com/integradev/delphi/antlr/Delphi.g:1002:32: assignmentStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_assignmentStatement_in_statement19516);
assignmentStatement675=assignmentStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, assignmentStatement675.getTree());
}
break;
case 15 :
// au/com/integradev/delphi/antlr/Delphi.g:1003:32: expressionStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_expressionStatement_in_statement19549);
expressionStatement676=expressionStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expressionStatement676.getTree());
}
break;
case 16 :
// au/com/integradev/delphi/antlr/Delphi.g:1004:32: gotoStatement
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_gotoStatement_in_statement19582);
gotoStatement677=gotoStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, gotoStatement677.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 172, statement_StartIndex); }
}
return retval;
}
// $ANTLR end "statement"
public static class ifStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "ifStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1006:1: ifStatement : IF ^ expression THEN ( statement )? ( ELSE ( statement )? )? ;
public final DelphiParser.ifStatement_return ifStatement() throws RecognitionException {
DelphiParser.ifStatement_return retval = new DelphiParser.ifStatement_return();
retval.start = input.LT(1);
int ifStatement_StartIndex = input.index();
Object root_0 = null;
Token IF678=null;
Token THEN680=null;
Token ELSE682=null;
ParserRuleReturnScope expression679 =null;
ParserRuleReturnScope statement681 =null;
ParserRuleReturnScope statement683 =null;
Object IF678_tree=null;
Object THEN680_tree=null;
Object ELSE682_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 173) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1006:30: ( IF ^ expression THEN ( statement )? ( ELSE ( statement )? )? )
// au/com/integradev/delphi/antlr/Delphi.g:1006:32: IF ^ expression THEN ( statement )? ( ELSE ( statement )? )?
{
root_0 = (Object)adaptor.nil();
IF678=(Token)match(input,IF,FOLLOW_IF_in_ifStatement19636); if (state.failed) return retval;
if ( state.backtracking==0 ) {
IF678_tree = new IfStatementNodeImpl(IF678) ;
root_0 = (Object)adaptor.becomeRoot(IF678_tree, root_0);
}
pushFollow(FOLLOW_expression_in_ifStatement19642);
expression679=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression679.getTree());
THEN680=(Token)match(input,THEN,FOLLOW_THEN_in_ifStatement19644); if (state.failed) return retval;
if ( state.backtracking==0 ) {
THEN680_tree = (Object)adaptor.create(THEN680);
adaptor.addChild(root_0, THEN680_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1006:73: ( statement )?
int alt231=2;
switch ( input.LA(1) ) {
case IF:
{
int LA231_1 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case VAR:
{
int LA231_2 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case CONST:
{
int LA231_3 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case CASE:
{
int LA231_4 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case REPEAT:
{
int LA231_5 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case WHILE:
{
int LA231_6 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case FOR:
{
int LA231_7 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case WITH:
{
int LA231_8 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TRY:
{
int LA231_9 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case RAISE:
{
int LA231_10 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case ASM:
{
int LA231_11 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case BEGIN:
{
int LA231_12 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkIdentifier:
{
int LA231_13 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case ON:
{
int LA231_14 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkIntNumber:
{
int LA231_15 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkHexNumber:
{
int LA231_16 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkBinaryNumber:
{
int LA231_17 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case NOT:
{
int LA231_18 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case PLUS:
{
int LA231_19 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case MINUS:
{
int LA231_20 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case ADDRESS:
{
int LA231_21 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkRealNumber:
{
int LA231_22 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkQuotedString:
{
int LA231_23 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA231_24 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case DEREFERENCE:
{
int LA231_25 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case TkMultilineString:
{
int LA231_26 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case NIL:
{
int LA231_27 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA231_28 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case STRING:
{
int LA231_29 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case FILE:
{
int LA231_30 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case PAREN_LEFT:
{
int LA231_31 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case INHERITED:
{
int LA231_32 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case PROCEDURE:
{
int LA231_33 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case FUNCTION:
{
int LA231_34 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case GOTO:
{
int LA231_35 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA231_44 = input.LA(2);
if ( (synpred345_Delphi()) ) {
alt231=1;
}
}
break;
}
switch (alt231) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1006:73: statement
{
pushFollow(FOLLOW_statement_in_ifStatement19646);
statement681=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement681.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1006:84: ( ELSE ( statement )? )?
int alt233=2;
int LA233_0 = input.LA(1);
if ( (LA233_0==ELSE) ) {
int LA233_1 = input.LA(2);
if ( (synpred347_Delphi()) ) {
alt233=1;
}
}
switch (alt233) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1006:85: ELSE ( statement )?
{
ELSE682=(Token)match(input,ELSE,FOLLOW_ELSE_in_ifStatement19650); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ELSE682_tree = (Object)adaptor.create(ELSE682);
adaptor.addChild(root_0, ELSE682_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1006:90: ( statement )?
int alt232=2;
switch ( input.LA(1) ) {
case IF:
{
int LA232_1 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case VAR:
{
int LA232_2 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case CONST:
{
int LA232_3 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case CASE:
{
int LA232_4 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case REPEAT:
{
int LA232_5 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case WHILE:
{
int LA232_6 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case FOR:
{
int LA232_7 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case WITH:
{
int LA232_8 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TRY:
{
int LA232_9 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case RAISE:
{
int LA232_10 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case ASM:
{
int LA232_11 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case BEGIN:
{
int LA232_12 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkIdentifier:
{
int LA232_13 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case ON:
{
int LA232_14 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkIntNumber:
{
int LA232_15 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkHexNumber:
{
int LA232_16 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkBinaryNumber:
{
int LA232_17 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case NOT:
{
int LA232_18 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case PLUS:
{
int LA232_19 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case MINUS:
{
int LA232_20 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case ADDRESS:
{
int LA232_21 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkRealNumber:
{
int LA232_22 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkQuotedString:
{
int LA232_23 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA232_24 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case DEREFERENCE:
{
int LA232_25 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case TkMultilineString:
{
int LA232_26 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case NIL:
{
int LA232_27 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA232_28 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case STRING:
{
int LA232_29 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case FILE:
{
int LA232_30 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case PAREN_LEFT:
{
int LA232_31 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case INHERITED:
{
int LA232_32 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case PROCEDURE:
{
int LA232_33 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case FUNCTION:
{
int LA232_34 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case GOTO:
{
int LA232_35 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA232_44 = input.LA(2);
if ( (synpred346_Delphi()) ) {
alt232=1;
}
}
break;
}
switch (alt232) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1006:90: statement
{
pushFollow(FOLLOW_statement_in_ifStatement19652);
statement683=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement683.getTree());
}
break;
}
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 173, ifStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "ifStatement"
public static class varStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "varStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1008:1: varStatement : VAR ( attributeList )? nameDeclarationList ( ':' varType )? ( ':=' expression )? -> ^( VAR nameDeclarationList ( ':' varType )? ( ':=' expression )? ( attributeList )? ) ;
public final DelphiParser.varStatement_return varStatement() throws RecognitionException {
DelphiParser.varStatement_return retval = new DelphiParser.varStatement_return();
retval.start = input.LT(1);
int varStatement_StartIndex = input.index();
Object root_0 = null;
Token VAR684=null;
Token char_literal687=null;
Token string_literal689=null;
ParserRuleReturnScope attributeList685 =null;
ParserRuleReturnScope nameDeclarationList686 =null;
ParserRuleReturnScope varType688 =null;
ParserRuleReturnScope expression690 =null;
Object VAR684_tree=null;
Object char_literal687_tree=null;
Object string_literal689_tree=null;
RewriteRuleTokenStream stream_VAR=new RewriteRuleTokenStream(adaptor,"token VAR");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleTokenStream stream_ASSIGN=new RewriteRuleTokenStream(adaptor,"token ASSIGN");
RewriteRuleSubtreeStream stream_nameDeclarationList=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclarationList");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 174) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1008:30: ( VAR ( attributeList )? nameDeclarationList ( ':' varType )? ( ':=' expression )? -> ^( VAR nameDeclarationList ( ':' varType )? ( ':=' expression )? ( attributeList )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1008:32: VAR ( attributeList )? nameDeclarationList ( ':' varType )? ( ':=' expression )?
{
VAR684=(Token)match(input,VAR,FOLLOW_VAR_in_varStatement19708); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_VAR.add(VAR684);
// au/com/integradev/delphi/antlr/Delphi.g:1008:36: ( attributeList )?
int alt234=2;
int LA234_0 = input.LA(1);
if ( (LA234_0==PAREN_BRACKET_LEFT||LA234_0==SQUARE_BRACKET_LEFT) ) {
alt234=1;
}
switch (alt234) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1008:36: attributeList
{
pushFollow(FOLLOW_attributeList_in_varStatement19710);
attributeList685=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList685.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclarationList_in_varStatement19713);
nameDeclarationList686=nameDeclarationList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclarationList.add(nameDeclarationList686.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1008:71: ( ':' varType )?
int alt235=2;
int LA235_0 = input.LA(1);
if ( (LA235_0==COLON) ) {
alt235=1;
}
switch (alt235) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1008:72: ':' varType
{
char_literal687=(Token)match(input,COLON,FOLLOW_COLON_in_varStatement19716); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal687);
pushFollow(FOLLOW_varType_in_varStatement19718);
varType688=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType688.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1008:86: ( ':=' expression )?
int alt236=2;
int LA236_0 = input.LA(1);
if ( (LA236_0==ASSIGN) ) {
alt236=1;
}
switch (alt236) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1008:87: ':=' expression
{
string_literal689=(Token)match(input,ASSIGN,FOLLOW_ASSIGN_in_varStatement19723); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ASSIGN.add(string_literal689);
pushFollow(FOLLOW_expression_in_varStatement19725);
expression690=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression690.getTree());
}
break;
}
// AST REWRITE
// elements: VAR, nameDeclarationList, COLON, ASSIGN, expression, varType, attributeList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1009:30: -> ^( VAR nameDeclarationList ( ':' varType )? ( ':=' expression )? ( attributeList )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1009:33: ^( VAR nameDeclarationList ( ':' varType )? ( ':=' expression )? ( attributeList )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new VarStatementNodeImpl(stream_VAR.nextToken()), root_1);
adaptor.addChild(root_1, stream_nameDeclarationList.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1009:81: ( ':' varType )?
if ( stream_COLON.hasNext()||stream_varType.hasNext() ) {
adaptor.addChild(root_1, stream_COLON.nextNode());
adaptor.addChild(root_1, stream_varType.nextTree());
}
stream_COLON.reset();
stream_varType.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1009:96: ( ':=' expression )?
if ( stream_ASSIGN.hasNext()||stream_expression.hasNext() ) {
adaptor.addChild(root_1, stream_ASSIGN.nextNode());
adaptor.addChild(root_1, stream_expression.nextTree());
}
stream_ASSIGN.reset();
stream_expression.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1009:115: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 174, varStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "varStatement"
public static class constStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "constStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1011:1: constStatement : CONST ( attributeList )? nameDeclaration ( ':' varType )? '=' expression -> ^( CONST nameDeclaration ( ':' varType )? '=' expression ( attributeList )? ) ;
public final DelphiParser.constStatement_return constStatement() throws RecognitionException {
DelphiParser.constStatement_return retval = new DelphiParser.constStatement_return();
retval.start = input.LT(1);
int constStatement_StartIndex = input.index();
Object root_0 = null;
Token CONST691=null;
Token char_literal694=null;
Token char_literal696=null;
ParserRuleReturnScope attributeList692 =null;
ParserRuleReturnScope nameDeclaration693 =null;
ParserRuleReturnScope varType695 =null;
ParserRuleReturnScope expression697 =null;
Object CONST691_tree=null;
Object char_literal694_tree=null;
Object char_literal696_tree=null;
RewriteRuleTokenStream stream_EQUAL=new RewriteRuleTokenStream(adaptor,"token EQUAL");
RewriteRuleTokenStream stream_CONST=new RewriteRuleTokenStream(adaptor,"token CONST");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
RewriteRuleSubtreeStream stream_attributeList=new RewriteRuleSubtreeStream(adaptor,"rule attributeList");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 175) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1011:30: ( CONST ( attributeList )? nameDeclaration ( ':' varType )? '=' expression -> ^( CONST nameDeclaration ( ':' varType )? '=' expression ( attributeList )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1011:32: CONST ( attributeList )? nameDeclaration ( ':' varType )? '=' expression
{
CONST691=(Token)match(input,CONST,FOLLOW_CONST_in_constStatement19835); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CONST.add(CONST691);
// au/com/integradev/delphi/antlr/Delphi.g:1011:38: ( attributeList )?
int alt237=2;
int LA237_0 = input.LA(1);
if ( (LA237_0==PAREN_BRACKET_LEFT||LA237_0==SQUARE_BRACKET_LEFT) ) {
alt237=1;
}
switch (alt237) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1011:38: attributeList
{
pushFollow(FOLLOW_attributeList_in_constStatement19837);
attributeList692=attributeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_attributeList.add(attributeList692.getTree());
}
break;
}
pushFollow(FOLLOW_nameDeclaration_in_constStatement19840);
nameDeclaration693=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration693.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1011:69: ( ':' varType )?
int alt238=2;
int LA238_0 = input.LA(1);
if ( (LA238_0==COLON) ) {
alt238=1;
}
switch (alt238) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1011:70: ':' varType
{
char_literal694=(Token)match(input,COLON,FOLLOW_COLON_in_constStatement19843); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal694);
pushFollow(FOLLOW_varType_in_constStatement19845);
varType695=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType695.getTree());
}
break;
}
char_literal696=(Token)match(input,EQUAL,FOLLOW_EQUAL_in_constStatement19849); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQUAL.add(char_literal696);
pushFollow(FOLLOW_expression_in_constStatement19851);
expression697=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression697.getTree());
// AST REWRITE
// elements: expression, varType, nameDeclaration, COLON, EQUAL, CONST, attributeList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1012:30: -> ^( CONST nameDeclaration ( ':' varType )? '=' expression ( attributeList )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1012:33: ^( CONST nameDeclaration ( ':' varType )? '=' expression ( attributeList )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ConstStatementNodeImpl(stream_CONST.nextToken()), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1012:81: ( ':' varType )?
if ( stream_varType.hasNext()||stream_COLON.hasNext() ) {
adaptor.addChild(root_1, stream_COLON.nextNode());
adaptor.addChild(root_1, stream_varType.nextTree());
}
stream_varType.reset();
stream_COLON.reset();
adaptor.addChild(root_1, stream_EQUAL.nextNode());
adaptor.addChild(root_1, stream_expression.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1012:111: ( attributeList )?
if ( stream_attributeList.hasNext() ) {
adaptor.addChild(root_1, stream_attributeList.nextTree());
}
stream_attributeList.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 175, constStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "constStatement"
public static class caseStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "caseStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1014:1: caseStatement : CASE ^ expression OF ( caseItem )* ( elseBlock )? END ;
public final DelphiParser.caseStatement_return caseStatement() throws RecognitionException {
DelphiParser.caseStatement_return retval = new DelphiParser.caseStatement_return();
retval.start = input.LT(1);
int caseStatement_StartIndex = input.index();
Object root_0 = null;
Token CASE698=null;
Token OF700=null;
Token END703=null;
ParserRuleReturnScope expression699 =null;
ParserRuleReturnScope caseItem701 =null;
ParserRuleReturnScope elseBlock702 =null;
Object CASE698_tree=null;
Object OF700_tree=null;
Object END703_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 176) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1014:30: ( CASE ^ expression OF ( caseItem )* ( elseBlock )? END )
// au/com/integradev/delphi/antlr/Delphi.g:1014:32: CASE ^ expression OF ( caseItem )* ( elseBlock )? END
{
root_0 = (Object)adaptor.nil();
CASE698=(Token)match(input,CASE,FOLLOW_CASE_in_caseStatement19957); if (state.failed) return retval;
if ( state.backtracking==0 ) {
CASE698_tree = new CaseStatementNodeImpl(CASE698) ;
root_0 = (Object)adaptor.becomeRoot(CASE698_tree, root_0);
}
pushFollow(FOLLOW_expression_in_caseStatement19963);
expression699=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression699.getTree());
OF700=(Token)match(input,OF,FOLLOW_OF_in_caseStatement19965); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OF700_tree = (Object)adaptor.create(OF700);
adaptor.addChild(root_0, OF700_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1014:75: ( caseItem )*
loop239:
while (true) {
int alt239=2;
int LA239_0 = input.LA(1);
if ( ((LA239_0 >= ABSOLUTE && LA239_0 <= ALIGN)||(LA239_0 >= ASSEMBLER && LA239_0 <= ASSEMBLY)||(LA239_0 >= AT && LA239_0 <= AUTOMATED)||LA239_0==CDECL||LA239_0==CONTAINS||(LA239_0 >= DEFAULT && LA239_0 <= DEREFERENCE)||LA239_0==DISPID||LA239_0==DYNAMIC||(LA239_0 >= EXPERIMENTAL && LA239_0 <= EXPORT)||LA239_0==EXTERNAL||(LA239_0 >= FAR && LA239_0 <= FINAL)||(LA239_0 >= FORWARD && LA239_0 <= FUNCTION)||LA239_0==HELPER||LA239_0==IMPLEMENTS||(LA239_0 >= INDEX && LA239_0 <= INHERITED)||LA239_0==LABEL||LA239_0==LOCAL||(LA239_0 >= MESSAGE && LA239_0 <= MINUS)||(LA239_0 >= NAME && LA239_0 <= NOT)||(LA239_0 >= ON && LA239_0 <= OPERATOR)||(LA239_0 >= OUT && LA239_0 <= OVERRIDE)||LA239_0==PACKAGE||LA239_0==PAREN_BRACKET_LEFT||LA239_0==PAREN_LEFT||(LA239_0 >= PASCAL && LA239_0 <= PROCEDURE)||(LA239_0 >= PROTECTED && LA239_0 <= PUBLISHED)||(LA239_0 >= READ && LA239_0 <= READONLY)||(LA239_0 >= REFERENCE && LA239_0 <= REINTRODUCE)||(LA239_0 >= REQUIRES && LA239_0 <= RESIDENT)||(LA239_0 >= SAFECALL && LA239_0 <= SEALED)||LA239_0==SQUARE_BRACKET_LEFT||(LA239_0 >= STATIC && LA239_0 <= STRING)||LA239_0==TkBinaryNumber||LA239_0==TkCharacterEscapeCode||(LA239_0 >= TkHexNumber && LA239_0 <= TkIntNumber)||LA239_0==TkMultilineString||(LA239_0 >= TkQuotedString && LA239_0 <= TkRealNumber)||LA239_0==UNSAFE||(LA239_0 >= VARARGS && LA239_0 <= VIRTUAL)||LA239_0==WINAPI||(LA239_0 >= WRITE && LA239_0 <= WRITEONLY)) ) {
alt239=1;
}
switch (alt239) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1014:75: caseItem
{
pushFollow(FOLLOW_caseItem_in_caseStatement19967);
caseItem701=caseItem();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, caseItem701.getTree());
}
break;
default :
break loop239;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:1014:85: ( elseBlock )?
int alt240=2;
int LA240_0 = input.LA(1);
if ( (LA240_0==ELSE) ) {
alt240=1;
}
switch (alt240) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1014:85: elseBlock
{
pushFollow(FOLLOW_elseBlock_in_caseStatement19970);
elseBlock702=elseBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, elseBlock702.getTree());
}
break;
}
END703=(Token)match(input,END,FOLLOW_END_in_caseStatement19973); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END703_tree = (Object)adaptor.create(END703);
adaptor.addChild(root_0, END703_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 176, caseStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "caseStatement"
public static class elseBlock_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "elseBlock"
// au/com/integradev/delphi/antlr/Delphi.g:1016:1: elseBlock : ELSE ^ statementList ;
public final DelphiParser.elseBlock_return elseBlock() throws RecognitionException {
DelphiParser.elseBlock_return retval = new DelphiParser.elseBlock_return();
retval.start = input.LT(1);
int elseBlock_StartIndex = input.index();
Object root_0 = null;
Token ELSE704=null;
ParserRuleReturnScope statementList705 =null;
Object ELSE704_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 177) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1016:30: ( ELSE ^ statementList )
// au/com/integradev/delphi/antlr/Delphi.g:1016:32: ELSE ^ statementList
{
root_0 = (Object)adaptor.nil();
ELSE704=(Token)match(input,ELSE,FOLLOW_ELSE_in_elseBlock20029); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ELSE704_tree = new ElseBlockNodeImpl(ELSE704) ;
root_0 = (Object)adaptor.becomeRoot(ELSE704_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_elseBlock20035);
statementList705=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList705.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 177, elseBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "elseBlock"
public static class caseItem_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "caseItem"
// au/com/integradev/delphi/antlr/Delphi.g:1018:1: caseItem : expressionOrRangeList ':' ( statement )? ( ';' )? -> ^( TkCaseItem expressionOrRangeList ( statement )? ( ';' )? ) ;
public final DelphiParser.caseItem_return caseItem() throws RecognitionException {
DelphiParser.caseItem_return retval = new DelphiParser.caseItem_return();
retval.start = input.LT(1);
int caseItem_StartIndex = input.index();
Object root_0 = null;
Token char_literal707=null;
Token char_literal709=null;
ParserRuleReturnScope expressionOrRangeList706 =null;
ParserRuleReturnScope statement708 =null;
Object char_literal707_tree=null;
Object char_literal709_tree=null;
RewriteRuleTokenStream stream_SEMICOLON=new RewriteRuleTokenStream(adaptor,"token SEMICOLON");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_expressionOrRangeList=new RewriteRuleSubtreeStream(adaptor,"rule expressionOrRangeList");
RewriteRuleSubtreeStream stream_statement=new RewriteRuleSubtreeStream(adaptor,"rule statement");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 178) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1018:30: ( expressionOrRangeList ':' ( statement )? ( ';' )? -> ^( TkCaseItem expressionOrRangeList ( statement )? ( ';' )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1018:32: expressionOrRangeList ':' ( statement )? ( ';' )?
{
pushFollow(FOLLOW_expressionOrRangeList_in_caseItem20092);
expressionOrRangeList706=expressionOrRangeList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expressionOrRangeList.add(expressionOrRangeList706.getTree());
char_literal707=(Token)match(input,COLON,FOLLOW_COLON_in_caseItem20094); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal707);
// au/com/integradev/delphi/antlr/Delphi.g:1018:58: ( statement )?
int alt241=2;
switch ( input.LA(1) ) {
case ASM:
case BEGIN:
case CASE:
case CONST:
case FOR:
case GOTO:
case IF:
case RAISE:
case REPEAT:
case TRY:
case VAR:
case WHILE:
case WITH:
{
alt241=1;
}
break;
case TkIdentifier:
{
int LA241_13 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA241_14 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkIntNumber:
{
int LA241_15 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkHexNumber:
{
int LA241_16 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkBinaryNumber:
{
int LA241_17 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case NOT:
{
int LA241_18 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case PLUS:
{
int LA241_19 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case MINUS:
{
int LA241_20 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case ADDRESS:
{
int LA241_21 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkRealNumber:
{
int LA241_22 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkQuotedString:
{
int LA241_23 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA241_24 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case DEREFERENCE:
{
int LA241_25 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case TkMultilineString:
{
int LA241_26 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case NIL:
{
int LA241_27 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA241_28 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case STRING:
{
int LA241_29 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case FILE:
{
int LA241_30 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case PAREN_LEFT:
{
int LA241_31 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case INHERITED:
{
int LA241_32 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case PROCEDURE:
{
int LA241_33 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
case FUNCTION:
{
int LA241_34 = input.LA(2);
if ( (synpred355_Delphi()) ) {
alt241=1;
}
}
break;
}
switch (alt241) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1018:59: statement
{
pushFollow(FOLLOW_statement_in_caseItem20097);
statement708=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(statement708.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1018:71: ( ';' )?
int alt242=2;
int LA242_0 = input.LA(1);
if ( (LA242_0==SEMICOLON) ) {
alt242=1;
}
switch (alt242) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1018:72: ';'
{
char_literal709=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_caseItem20102); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMICOLON.add(char_literal709);
}
break;
}
// AST REWRITE
// elements: expressionOrRangeList, SEMICOLON, statement
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1018:78: -> ^( TkCaseItem expressionOrRangeList ( statement )? ( ';' )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1018:81: ^( TkCaseItem expressionOrRangeList ( statement )? ( ';' )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new CaseItemStatementNodeImpl(TkCaseItem), root_1);
adaptor.addChild(root_1, stream_expressionOrRangeList.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1018:143: ( statement )?
if ( stream_statement.hasNext() ) {
adaptor.addChild(root_1, stream_statement.nextTree());
}
stream_statement.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1018:156: ( ';' )?
if ( stream_SEMICOLON.hasNext() ) {
adaptor.addChild(root_1, stream_SEMICOLON.nextNode());
}
stream_SEMICOLON.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 178, caseItem_StartIndex); }
}
return retval;
}
// $ANTLR end "caseItem"
public static class repeatStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "repeatStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1020:1: repeatStatement : REPEAT ^ statementList UNTIL expression ;
public final DelphiParser.repeatStatement_return repeatStatement() throws RecognitionException {
DelphiParser.repeatStatement_return retval = new DelphiParser.repeatStatement_return();
retval.start = input.LT(1);
int repeatStatement_StartIndex = input.index();
Object root_0 = null;
Token REPEAT710=null;
Token UNTIL712=null;
ParserRuleReturnScope statementList711 =null;
ParserRuleReturnScope expression713 =null;
Object REPEAT710_tree=null;
Object UNTIL712_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 179) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1020:30: ( REPEAT ^ statementList UNTIL expression )
// au/com/integradev/delphi/antlr/Delphi.g:1020:32: REPEAT ^ statementList UNTIL expression
{
root_0 = (Object)adaptor.nil();
REPEAT710=(Token)match(input,REPEAT,FOLLOW_REPEAT_in_repeatStatement20176); if (state.failed) return retval;
if ( state.backtracking==0 ) {
REPEAT710_tree = new RepeatStatementNodeImpl(REPEAT710) ;
root_0 = (Object)adaptor.becomeRoot(REPEAT710_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_repeatStatement20182);
statementList711=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList711.getTree());
UNTIL712=(Token)match(input,UNTIL,FOLLOW_UNTIL_in_repeatStatement20184); if (state.failed) return retval;
if ( state.backtracking==0 ) {
UNTIL712_tree = (Object)adaptor.create(UNTIL712);
adaptor.addChild(root_0, UNTIL712_tree);
}
pushFollow(FOLLOW_expression_in_repeatStatement20186);
expression713=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression713.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 179, repeatStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "repeatStatement"
public static class whileStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "whileStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1022:1: whileStatement : WHILE ^ expression DO ( statement )? ;
public final DelphiParser.whileStatement_return whileStatement() throws RecognitionException {
DelphiParser.whileStatement_return retval = new DelphiParser.whileStatement_return();
retval.start = input.LT(1);
int whileStatement_StartIndex = input.index();
Object root_0 = null;
Token WHILE714=null;
Token DO716=null;
ParserRuleReturnScope expression715 =null;
ParserRuleReturnScope statement717 =null;
Object WHILE714_tree=null;
Object DO716_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 180) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1022:30: ( WHILE ^ expression DO ( statement )? )
// au/com/integradev/delphi/antlr/Delphi.g:1022:32: WHILE ^ expression DO ( statement )?
{
root_0 = (Object)adaptor.nil();
WHILE714=(Token)match(input,WHILE,FOLLOW_WHILE_in_whileStatement20237); if (state.failed) return retval;
if ( state.backtracking==0 ) {
WHILE714_tree = new WhileStatementNodeImpl(WHILE714) ;
root_0 = (Object)adaptor.becomeRoot(WHILE714_tree, root_0);
}
pushFollow(FOLLOW_expression_in_whileStatement20243);
expression715=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression715.getTree());
DO716=(Token)match(input,DO,FOLLOW_DO_in_whileStatement20245); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DO716_tree = (Object)adaptor.create(DO716);
adaptor.addChild(root_0, DO716_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1022:77: ( statement )?
int alt243=2;
switch ( input.LA(1) ) {
case IF:
{
int LA243_1 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case VAR:
{
int LA243_2 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case CONST:
{
int LA243_3 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case CASE:
{
int LA243_4 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case REPEAT:
{
int LA243_5 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case WHILE:
{
int LA243_6 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case FOR:
{
int LA243_7 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case WITH:
{
int LA243_8 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TRY:
{
int LA243_9 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case RAISE:
{
int LA243_10 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case ASM:
{
int LA243_11 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case BEGIN:
{
int LA243_12 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkIdentifier:
{
int LA243_13 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case ON:
{
int LA243_14 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkIntNumber:
{
int LA243_15 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkHexNumber:
{
int LA243_16 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkBinaryNumber:
{
int LA243_17 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case NOT:
{
int LA243_18 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case PLUS:
{
int LA243_19 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case MINUS:
{
int LA243_20 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case ADDRESS:
{
int LA243_21 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkRealNumber:
{
int LA243_22 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkQuotedString:
{
int LA243_23 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA243_24 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case DEREFERENCE:
{
int LA243_25 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case TkMultilineString:
{
int LA243_26 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case NIL:
{
int LA243_27 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA243_28 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case STRING:
{
int LA243_29 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case FILE:
{
int LA243_30 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case PAREN_LEFT:
{
int LA243_31 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case INHERITED:
{
int LA243_32 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case PROCEDURE:
{
int LA243_33 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case FUNCTION:
{
int LA243_34 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case GOTO:
{
int LA243_35 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA243_44 = input.LA(2);
if ( (synpred357_Delphi()) ) {
alt243=1;
}
}
break;
}
switch (alt243) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1022:77: statement
{
pushFollow(FOLLOW_statement_in_whileStatement20247);
statement717=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement717.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 180, whileStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "whileStatement"
public static class forStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "forStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1024:1: forStatement : ( FOR ^ forVar ':=' expression TO expression DO ( statement )? | FOR ^ forVar ':=' expression DOWNTO expression DO ( statement )? | FOR ^ forVar IN expression DO ( statement )? );
public final DelphiParser.forStatement_return forStatement() throws RecognitionException {
DelphiParser.forStatement_return retval = new DelphiParser.forStatement_return();
retval.start = input.LT(1);
int forStatement_StartIndex = input.index();
Object root_0 = null;
Token FOR718=null;
Token string_literal720=null;
Token TO722=null;
Token DO724=null;
Token FOR726=null;
Token string_literal728=null;
Token DOWNTO730=null;
Token DO732=null;
Token FOR734=null;
Token IN736=null;
Token DO738=null;
ParserRuleReturnScope forVar719 =null;
ParserRuleReturnScope expression721 =null;
ParserRuleReturnScope expression723 =null;
ParserRuleReturnScope statement725 =null;
ParserRuleReturnScope forVar727 =null;
ParserRuleReturnScope expression729 =null;
ParserRuleReturnScope expression731 =null;
ParserRuleReturnScope statement733 =null;
ParserRuleReturnScope forVar735 =null;
ParserRuleReturnScope expression737 =null;
ParserRuleReturnScope statement739 =null;
Object FOR718_tree=null;
Object string_literal720_tree=null;
Object TO722_tree=null;
Object DO724_tree=null;
Object FOR726_tree=null;
Object string_literal728_tree=null;
Object DOWNTO730_tree=null;
Object DO732_tree=null;
Object FOR734_tree=null;
Object IN736_tree=null;
Object DO738_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 181) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1024:30: ( FOR ^ forVar ':=' expression TO expression DO ( statement )? | FOR ^ forVar ':=' expression DOWNTO expression DO ( statement )? | FOR ^ forVar IN expression DO ( statement )? )
int alt247=3;
int LA247_0 = input.LA(1);
if ( (LA247_0==FOR) ) {
int LA247_1 = input.LA(2);
if ( (synpred359_Delphi()) ) {
alt247=1;
}
else if ( (synpred361_Delphi()) ) {
alt247=2;
}
else if ( (true) ) {
alt247=3;
}
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 247, 0, input);
throw nvae;
}
switch (alt247) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1024:32: FOR ^ forVar ':=' expression TO expression DO ( statement )?
{
root_0 = (Object)adaptor.nil();
FOR718=(Token)match(input,FOR,FOLLOW_FOR_in_forStatement20301); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FOR718_tree = new ForToStatementNodeImpl(FOR718) ;
root_0 = (Object)adaptor.becomeRoot(FOR718_tree, root_0);
}
pushFollow(FOLLOW_forVar_in_forStatement20307);
forVar719=forVar();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, forVar719.getTree());
string_literal720=(Token)match(input,ASSIGN,FOLLOW_ASSIGN_in_forStatement20309); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal720_tree = (Object)adaptor.create(string_literal720);
adaptor.addChild(root_0, string_literal720_tree);
}
pushFollow(FOLLOW_expression_in_forStatement20311);
expression721=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression721.getTree());
TO722=(Token)match(input,TO,FOLLOW_TO_in_forStatement20313); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TO722_tree = (Object)adaptor.create(TO722);
adaptor.addChild(root_0, TO722_tree);
}
pushFollow(FOLLOW_expression_in_forStatement20315);
expression723=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression723.getTree());
DO724=(Token)match(input,DO,FOLLOW_DO_in_forStatement20317); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DO724_tree = (Object)adaptor.create(DO724);
adaptor.addChild(root_0, DO724_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1024:101: ( statement )?
int alt244=2;
switch ( input.LA(1) ) {
case IF:
{
int LA244_1 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case VAR:
{
int LA244_2 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case CONST:
{
int LA244_3 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case CASE:
{
int LA244_4 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case REPEAT:
{
int LA244_5 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case WHILE:
{
int LA244_6 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case FOR:
{
int LA244_7 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case WITH:
{
int LA244_8 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TRY:
{
int LA244_9 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case RAISE:
{
int LA244_10 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case ASM:
{
int LA244_11 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case BEGIN:
{
int LA244_12 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkIdentifier:
{
int LA244_13 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case ON:
{
int LA244_14 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkIntNumber:
{
int LA244_15 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkHexNumber:
{
int LA244_16 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkBinaryNumber:
{
int LA244_17 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case NOT:
{
int LA244_18 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case PLUS:
{
int LA244_19 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case MINUS:
{
int LA244_20 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case ADDRESS:
{
int LA244_21 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkRealNumber:
{
int LA244_22 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkQuotedString:
{
int LA244_23 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA244_24 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case DEREFERENCE:
{
int LA244_25 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case TkMultilineString:
{
int LA244_26 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case NIL:
{
int LA244_27 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA244_28 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case STRING:
{
int LA244_29 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case FILE:
{
int LA244_30 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case PAREN_LEFT:
{
int LA244_31 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case INHERITED:
{
int LA244_32 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case PROCEDURE:
{
int LA244_33 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case FUNCTION:
{
int LA244_34 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case GOTO:
{
int LA244_35 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA244_44 = input.LA(2);
if ( (synpred358_Delphi()) ) {
alt244=1;
}
}
break;
}
switch (alt244) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1024:101: statement
{
pushFollow(FOLLOW_statement_in_forStatement20319);
statement725=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement725.getTree());
}
break;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1025:32: FOR ^ forVar ':=' expression DOWNTO expression DO ( statement )?
{
root_0 = (Object)adaptor.nil();
FOR726=(Token)match(input,FOR,FOLLOW_FOR_in_forStatement20353); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FOR726_tree = new ForToStatementNodeImpl(FOR726) ;
root_0 = (Object)adaptor.becomeRoot(FOR726_tree, root_0);
}
pushFollow(FOLLOW_forVar_in_forStatement20359);
forVar727=forVar();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, forVar727.getTree());
string_literal728=(Token)match(input,ASSIGN,FOLLOW_ASSIGN_in_forStatement20361); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal728_tree = (Object)adaptor.create(string_literal728);
adaptor.addChild(root_0, string_literal728_tree);
}
pushFollow(FOLLOW_expression_in_forStatement20363);
expression729=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression729.getTree());
DOWNTO730=(Token)match(input,DOWNTO,FOLLOW_DOWNTO_in_forStatement20365); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DOWNTO730_tree = (Object)adaptor.create(DOWNTO730);
adaptor.addChild(root_0, DOWNTO730_tree);
}
pushFollow(FOLLOW_expression_in_forStatement20367);
expression731=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression731.getTree());
DO732=(Token)match(input,DO,FOLLOW_DO_in_forStatement20369); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DO732_tree = (Object)adaptor.create(DO732);
adaptor.addChild(root_0, DO732_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1025:105: ( statement )?
int alt245=2;
switch ( input.LA(1) ) {
case IF:
{
int LA245_1 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case VAR:
{
int LA245_2 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case CONST:
{
int LA245_3 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case CASE:
{
int LA245_4 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case REPEAT:
{
int LA245_5 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case WHILE:
{
int LA245_6 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case FOR:
{
int LA245_7 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case WITH:
{
int LA245_8 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TRY:
{
int LA245_9 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case RAISE:
{
int LA245_10 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case ASM:
{
int LA245_11 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case BEGIN:
{
int LA245_12 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkIdentifier:
{
int LA245_13 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case ON:
{
int LA245_14 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkIntNumber:
{
int LA245_15 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkHexNumber:
{
int LA245_16 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkBinaryNumber:
{
int LA245_17 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case NOT:
{
int LA245_18 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case PLUS:
{
int LA245_19 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case MINUS:
{
int LA245_20 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case ADDRESS:
{
int LA245_21 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkRealNumber:
{
int LA245_22 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkQuotedString:
{
int LA245_23 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA245_24 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case DEREFERENCE:
{
int LA245_25 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case TkMultilineString:
{
int LA245_26 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case NIL:
{
int LA245_27 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA245_28 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case STRING:
{
int LA245_29 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case FILE:
{
int LA245_30 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case PAREN_LEFT:
{
int LA245_31 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case INHERITED:
{
int LA245_32 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case PROCEDURE:
{
int LA245_33 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case FUNCTION:
{
int LA245_34 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case GOTO:
{
int LA245_35 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA245_44 = input.LA(2);
if ( (synpred360_Delphi()) ) {
alt245=1;
}
}
break;
}
switch (alt245) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1025:105: statement
{
pushFollow(FOLLOW_statement_in_forStatement20371);
statement733=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement733.getTree());
}
break;
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1026:32: FOR ^ forVar IN expression DO ( statement )?
{
root_0 = (Object)adaptor.nil();
FOR734=(Token)match(input,FOR,FOLLOW_FOR_in_forStatement20405); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FOR734_tree = new ForInStatementNodeImpl(FOR734) ;
root_0 = (Object)adaptor.becomeRoot(FOR734_tree, root_0);
}
pushFollow(FOLLOW_forVar_in_forStatement20411);
forVar735=forVar();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, forVar735.getTree());
IN736=(Token)match(input,IN,FOLLOW_IN_in_forStatement20413); if (state.failed) return retval;
if ( state.backtracking==0 ) {
IN736_tree = (Object)adaptor.create(IN736);
adaptor.addChild(root_0, IN736_tree);
}
pushFollow(FOLLOW_expression_in_forStatement20415);
expression737=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression737.getTree());
DO738=(Token)match(input,DO,FOLLOW_DO_in_forStatement20417); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DO738_tree = (Object)adaptor.create(DO738);
adaptor.addChild(root_0, DO738_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1026:85: ( statement )?
int alt246=2;
switch ( input.LA(1) ) {
case IF:
{
int LA246_1 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case VAR:
{
int LA246_2 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case CONST:
{
int LA246_3 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case CASE:
{
int LA246_4 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case REPEAT:
{
int LA246_5 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case WHILE:
{
int LA246_6 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case FOR:
{
int LA246_7 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case WITH:
{
int LA246_8 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TRY:
{
int LA246_9 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case RAISE:
{
int LA246_10 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case ASM:
{
int LA246_11 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case BEGIN:
{
int LA246_12 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkIdentifier:
{
int LA246_13 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case ON:
{
int LA246_14 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkIntNumber:
{
int LA246_15 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkHexNumber:
{
int LA246_16 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkBinaryNumber:
{
int LA246_17 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case NOT:
{
int LA246_18 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case PLUS:
{
int LA246_19 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case MINUS:
{
int LA246_20 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case ADDRESS:
{
int LA246_21 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkRealNumber:
{
int LA246_22 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkQuotedString:
{
int LA246_23 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA246_24 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case DEREFERENCE:
{
int LA246_25 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case TkMultilineString:
{
int LA246_26 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case NIL:
{
int LA246_27 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA246_28 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case STRING:
{
int LA246_29 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case FILE:
{
int LA246_30 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case PAREN_LEFT:
{
int LA246_31 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case INHERITED:
{
int LA246_32 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case PROCEDURE:
{
int LA246_33 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case FUNCTION:
{
int LA246_34 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case GOTO:
{
int LA246_35 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA246_44 = input.LA(2);
if ( (synpred362_Delphi()) ) {
alt246=1;
}
}
break;
}
switch (alt246) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1026:85: statement
{
pushFollow(FOLLOW_statement_in_forStatement20419);
statement739=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement739.getTree());
}
break;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 181, forStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "forStatement"
public static class forVar_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "forVar"
// au/com/integradev/delphi/antlr/Delphi.g:1028:1: forVar : ( VAR nameDeclaration ( ':' varType )? -> ^( TkForLoopVar nameDeclaration ( varType )? ) | simpleNameReference -> ^( TkForLoopVar simpleNameReference ) );
public final DelphiParser.forVar_return forVar() throws RecognitionException {
DelphiParser.forVar_return retval = new DelphiParser.forVar_return();
retval.start = input.LT(1);
int forVar_StartIndex = input.index();
Object root_0 = null;
Token VAR740=null;
Token char_literal742=null;
ParserRuleReturnScope nameDeclaration741 =null;
ParserRuleReturnScope varType743 =null;
ParserRuleReturnScope simpleNameReference744 =null;
Object VAR740_tree=null;
Object char_literal742_tree=null;
RewriteRuleTokenStream stream_VAR=new RewriteRuleTokenStream(adaptor,"token VAR");
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_varType=new RewriteRuleSubtreeStream(adaptor,"rule varType");
RewriteRuleSubtreeStream stream_simpleNameReference=new RewriteRuleSubtreeStream(adaptor,"rule simpleNameReference");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 182) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1028:30: ( VAR nameDeclaration ( ':' varType )? -> ^( TkForLoopVar nameDeclaration ( varType )? ) | simpleNameReference -> ^( TkForLoopVar simpleNameReference ) )
int alt249=2;
int LA249_0 = input.LA(1);
if ( (LA249_0==VAR) ) {
alt249=1;
}
else if ( ((LA249_0 >= ABSOLUTE && LA249_0 <= ABSTRACT)||LA249_0==ALIGN||(LA249_0 >= ASSEMBLER && LA249_0 <= ASSEMBLY)||(LA249_0 >= AT && LA249_0 <= AUTOMATED)||LA249_0==CDECL||LA249_0==CONTAINS||(LA249_0 >= DEFAULT && LA249_0 <= DEPRECATED)||LA249_0==DISPID||LA249_0==DYNAMIC||(LA249_0 >= EXPERIMENTAL && LA249_0 <= EXPORT)||LA249_0==EXTERNAL||LA249_0==FAR||LA249_0==FINAL||LA249_0==FORWARD||LA249_0==HELPER||LA249_0==IMPLEMENTS||LA249_0==INDEX||LA249_0==LABEL||LA249_0==LOCAL||LA249_0==MESSAGE||(LA249_0 >= NAME && LA249_0 <= NEAR)||LA249_0==NODEFAULT||(LA249_0 >= ON && LA249_0 <= OPERATOR)||(LA249_0 >= OUT && LA249_0 <= OVERRIDE)||LA249_0==PACKAGE||(LA249_0 >= PASCAL && LA249_0 <= PLATFORM)||LA249_0==PRIVATE||(LA249_0 >= PROTECTED && LA249_0 <= PUBLISHED)||(LA249_0 >= READ && LA249_0 <= READONLY)||(LA249_0 >= REFERENCE && LA249_0 <= REINTRODUCE)||(LA249_0 >= REQUIRES && LA249_0 <= RESIDENT)||(LA249_0 >= SAFECALL && LA249_0 <= SEALED)||(LA249_0 >= STATIC && LA249_0 <= STRICT)||LA249_0==TkIdentifier||LA249_0==UNSAFE||(LA249_0 >= VARARGS && LA249_0 <= VIRTUAL)||LA249_0==WINAPI||(LA249_0 >= WRITE && LA249_0 <= WRITEONLY)) ) {
alt249=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 249, 0, input);
throw nvae;
}
switch (alt249) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1028:32: VAR nameDeclaration ( ':' varType )?
{
VAR740=(Token)match(input,VAR,FOLLOW_VAR_in_forVar20479); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_VAR.add(VAR740);
pushFollow(FOLLOW_nameDeclaration_in_forVar20481);
nameDeclaration741=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration741.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1028:52: ( ':' varType )?
int alt248=2;
int LA248_0 = input.LA(1);
if ( (LA248_0==COLON) ) {
alt248=1;
}
switch (alt248) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1028:53: ':' varType
{
char_literal742=(Token)match(input,COLON,FOLLOW_COLON_in_forVar20484); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal742);
pushFollow(FOLLOW_varType_in_forVar20486);
varType743=varType();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varType.add(varType743.getTree());
}
break;
}
// AST REWRITE
// elements: nameDeclaration, varType
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1028:67: -> ^( TkForLoopVar nameDeclaration ( varType )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1028:70: ^( TkForLoopVar nameDeclaration ( varType )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ForLoopVarDeclarationNodeImpl(TkForLoopVar), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1028:132: ( varType )?
if ( stream_varType.hasNext() ) {
adaptor.addChild(root_1, stream_varType.nextTree());
}
stream_varType.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1029:32: simpleNameReference
{
pushFollow(FOLLOW_simpleNameReference_in_forVar20535);
simpleNameReference744=simpleNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_simpleNameReference.add(simpleNameReference744.getTree());
// AST REWRITE
// elements: simpleNameReference
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1029:52: -> ^( TkForLoopVar simpleNameReference )
{
// au/com/integradev/delphi/antlr/Delphi.g:1029:55: ^( TkForLoopVar simpleNameReference )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ForLoopVarReferenceNodeImpl(TkForLoopVar), root_1);
adaptor.addChild(root_1, stream_simpleNameReference.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 182, forVar_StartIndex); }
}
return retval;
}
// $ANTLR end "forVar"
public static class withStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "withStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1031:1: withStatement : WITH ^ expressionList DO ( statement )? ;
public final DelphiParser.withStatement_return withStatement() throws RecognitionException {
DelphiParser.withStatement_return retval = new DelphiParser.withStatement_return();
retval.start = input.LT(1);
int withStatement_StartIndex = input.index();
Object root_0 = null;
Token WITH745=null;
Token DO747=null;
ParserRuleReturnScope expressionList746 =null;
ParserRuleReturnScope statement748 =null;
Object WITH745_tree=null;
Object DO747_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 183) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1031:30: ( WITH ^ expressionList DO ( statement )? )
// au/com/integradev/delphi/antlr/Delphi.g:1031:32: WITH ^ expressionList DO ( statement )?
{
root_0 = (Object)adaptor.nil();
WITH745=(Token)match(input,WITH,FOLLOW_WITH_in_withStatement20598); if (state.failed) return retval;
if ( state.backtracking==0 ) {
WITH745_tree = new WithStatementNodeImpl(WITH745) ;
root_0 = (Object)adaptor.becomeRoot(WITH745_tree, root_0);
}
pushFollow(FOLLOW_expressionList_in_withStatement20604);
expressionList746=expressionList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expressionList746.getTree());
DO747=(Token)match(input,DO,FOLLOW_DO_in_withStatement20606); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DO747_tree = (Object)adaptor.create(DO747);
adaptor.addChild(root_0, DO747_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1031:79: ( statement )?
int alt250=2;
switch ( input.LA(1) ) {
case IF:
{
int LA250_1 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case VAR:
{
int LA250_2 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case CONST:
{
int LA250_3 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case CASE:
{
int LA250_4 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case REPEAT:
{
int LA250_5 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case WHILE:
{
int LA250_6 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case FOR:
{
int LA250_7 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case WITH:
{
int LA250_8 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TRY:
{
int LA250_9 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case RAISE:
{
int LA250_10 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case ASM:
{
int LA250_11 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case BEGIN:
{
int LA250_12 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkIdentifier:
{
int LA250_13 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case ON:
{
int LA250_14 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkIntNumber:
{
int LA250_15 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkHexNumber:
{
int LA250_16 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkBinaryNumber:
{
int LA250_17 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case NOT:
{
int LA250_18 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case PLUS:
{
int LA250_19 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case MINUS:
{
int LA250_20 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case ADDRESS:
{
int LA250_21 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkRealNumber:
{
int LA250_22 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkQuotedString:
{
int LA250_23 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA250_24 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case DEREFERENCE:
{
int LA250_25 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case TkMultilineString:
{
int LA250_26 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case NIL:
{
int LA250_27 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA250_28 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case STRING:
{
int LA250_29 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case FILE:
{
int LA250_30 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case PAREN_LEFT:
{
int LA250_31 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case INHERITED:
{
int LA250_32 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case PROCEDURE:
{
int LA250_33 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case FUNCTION:
{
int LA250_34 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case GOTO:
{
int LA250_35 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA250_44 = input.LA(2);
if ( (synpred365_Delphi()) ) {
alt250=1;
}
}
break;
}
switch (alt250) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1031:79: statement
{
pushFollow(FOLLOW_statement_in_withStatement20608);
statement748=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement748.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 183, withStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "withStatement"
public static class compoundStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "compoundStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1033:1: compoundStatement : BEGIN ^ statementList END ;
public final DelphiParser.compoundStatement_return compoundStatement() throws RecognitionException {
DelphiParser.compoundStatement_return retval = new DelphiParser.compoundStatement_return();
retval.start = input.LT(1);
int compoundStatement_StartIndex = input.index();
Object root_0 = null;
Token BEGIN749=null;
Token END751=null;
ParserRuleReturnScope statementList750 =null;
Object BEGIN749_tree=null;
Object END751_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 184) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1033:30: ( BEGIN ^ statementList END )
// au/com/integradev/delphi/antlr/Delphi.g:1033:32: BEGIN ^ statementList END
{
root_0 = (Object)adaptor.nil();
BEGIN749=(Token)match(input,BEGIN,FOLLOW_BEGIN_in_compoundStatement20657); if (state.failed) return retval;
if ( state.backtracking==0 ) {
BEGIN749_tree = new CompoundStatementNodeImpl(BEGIN749) ;
root_0 = (Object)adaptor.becomeRoot(BEGIN749_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_compoundStatement20663);
statementList750=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList750.getTree());
END751=(Token)match(input,END,FOLLOW_END_in_compoundStatement20665); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END751_tree = (Object)adaptor.create(END751);
adaptor.addChild(root_0, END751_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 184, compoundStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "compoundStatement"
public static class statementList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "statementList"
// au/com/integradev/delphi/antlr/Delphi.g:1035:1: statementList : ( delimitedStatements )? -> ^( TkStatementList ( delimitedStatements )? ) ;
public final DelphiParser.statementList_return statementList() throws RecognitionException {
DelphiParser.statementList_return retval = new DelphiParser.statementList_return();
retval.start = input.LT(1);
int statementList_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope delimitedStatements752 =null;
RewriteRuleSubtreeStream stream_delimitedStatements=new RewriteRuleSubtreeStream(adaptor,"rule delimitedStatements");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 185) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1035:30: ( ( delimitedStatements )? -> ^( TkStatementList ( delimitedStatements )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1035:32: ( delimitedStatements )?
{
// au/com/integradev/delphi/antlr/Delphi.g:1035:32: ( delimitedStatements )?
int alt251=2;
int LA251_0 = input.LA(1);
if ( ((LA251_0 >= ABSOLUTE && LA251_0 <= ALIGN)||(LA251_0 >= ASM && LA251_0 <= ASSEMBLY)||(LA251_0 >= AT && LA251_0 <= AUTOMATED)||LA251_0==BEGIN||(LA251_0 >= CASE && LA251_0 <= CDECL)||LA251_0==CONST||LA251_0==CONTAINS||(LA251_0 >= DEFAULT && LA251_0 <= DEREFERENCE)||LA251_0==DISPID||LA251_0==DYNAMIC||(LA251_0 >= EXPERIMENTAL && LA251_0 <= EXPORT)||LA251_0==EXTERNAL||(LA251_0 >= FAR && LA251_0 <= FINAL)||(LA251_0 >= FOR && LA251_0 <= FUNCTION)||LA251_0==GOTO||LA251_0==HELPER||LA251_0==IF||LA251_0==IMPLEMENTS||(LA251_0 >= INDEX && LA251_0 <= INHERITED)||LA251_0==LABEL||LA251_0==LOCAL||(LA251_0 >= MESSAGE && LA251_0 <= MINUS)||(LA251_0 >= NAME && LA251_0 <= NOT)||(LA251_0 >= ON && LA251_0 <= OPERATOR)||(LA251_0 >= OUT && LA251_0 <= OVERRIDE)||LA251_0==PACKAGE||LA251_0==PAREN_BRACKET_LEFT||LA251_0==PAREN_LEFT||(LA251_0 >= PASCAL && LA251_0 <= PROCEDURE)||(LA251_0 >= PROTECTED && LA251_0 <= PUBLISHED)||(LA251_0 >= RAISE && LA251_0 <= READONLY)||(LA251_0 >= REFERENCE && LA251_0 <= RESIDENT)||(LA251_0 >= SAFECALL && LA251_0 <= SEMICOLON)||LA251_0==SQUARE_BRACKET_LEFT||(LA251_0 >= STATIC && LA251_0 <= STRING)||LA251_0==TRY||LA251_0==TkBinaryNumber||LA251_0==TkCharacterEscapeCode||(LA251_0 >= TkHexNumber && LA251_0 <= TkIntNumber)||LA251_0==TkMultilineString||(LA251_0 >= TkQuotedString && LA251_0 <= TkRealNumber)||LA251_0==UNSAFE||(LA251_0 >= VAR && LA251_0 <= VIRTUAL)||LA251_0==WHILE||(LA251_0 >= WINAPI && LA251_0 <= WRITEONLY)) ) {
alt251=1;
}
switch (alt251) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1035:32: delimitedStatements
{
pushFollow(FOLLOW_delimitedStatements_in_statementList20717);
delimitedStatements752=delimitedStatements();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_delimitedStatements.add(delimitedStatements752.getTree());
}
break;
}
// AST REWRITE
// elements: delimitedStatements
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1035:53: -> ^( TkStatementList ( delimitedStatements )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1035:56: ^( TkStatementList ( delimitedStatements )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new StatementListNodeImpl(TkStatementList), root_1);
// au/com/integradev/delphi/antlr/Delphi.g:1035:97: ( delimitedStatements )?
if ( stream_delimitedStatements.hasNext() ) {
adaptor.addChild(root_1, stream_delimitedStatements.nextTree());
}
stream_delimitedStatements.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 185, statementList_StartIndex); }
}
return retval;
}
// $ANTLR end "statementList"
public static class delimitedStatements_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "delimitedStatements"
// au/com/integradev/delphi/antlr/Delphi.g:1037:1: delimitedStatements : ( statement | ';' )+ ;
public final DelphiParser.delimitedStatements_return delimitedStatements() throws RecognitionException {
DelphiParser.delimitedStatements_return retval = new DelphiParser.delimitedStatements_return();
retval.start = input.LT(1);
int delimitedStatements_StartIndex = input.index();
Object root_0 = null;
Token char_literal754=null;
ParserRuleReturnScope statement753 =null;
Object char_literal754_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 186) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1037:30: ( ( statement | ';' )+ )
// au/com/integradev/delphi/antlr/Delphi.g:1037:32: ( statement | ';' )+
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1037:32: ( statement | ';' )+
int cnt252=0;
loop252:
while (true) {
int alt252=3;
int LA252_0 = input.LA(1);
if ( ((LA252_0 >= ABSOLUTE && LA252_0 <= ALIGN)||(LA252_0 >= ASM && LA252_0 <= ASSEMBLY)||(LA252_0 >= AT && LA252_0 <= AUTOMATED)||LA252_0==BEGIN||(LA252_0 >= CASE && LA252_0 <= CDECL)||LA252_0==CONST||LA252_0==CONTAINS||(LA252_0 >= DEFAULT && LA252_0 <= DEREFERENCE)||LA252_0==DISPID||LA252_0==DYNAMIC||(LA252_0 >= EXPERIMENTAL && LA252_0 <= EXPORT)||LA252_0==EXTERNAL||(LA252_0 >= FAR && LA252_0 <= FINAL)||(LA252_0 >= FOR && LA252_0 <= FUNCTION)||LA252_0==GOTO||LA252_0==HELPER||LA252_0==IF||LA252_0==IMPLEMENTS||(LA252_0 >= INDEX && LA252_0 <= INHERITED)||LA252_0==LABEL||LA252_0==LOCAL||(LA252_0 >= MESSAGE && LA252_0 <= MINUS)||(LA252_0 >= NAME && LA252_0 <= NOT)||(LA252_0 >= ON && LA252_0 <= OPERATOR)||(LA252_0 >= OUT && LA252_0 <= OVERRIDE)||LA252_0==PACKAGE||LA252_0==PAREN_BRACKET_LEFT||LA252_0==PAREN_LEFT||(LA252_0 >= PASCAL && LA252_0 <= PROCEDURE)||(LA252_0 >= PROTECTED && LA252_0 <= PUBLISHED)||(LA252_0 >= RAISE && LA252_0 <= READONLY)||(LA252_0 >= REFERENCE && LA252_0 <= RESIDENT)||(LA252_0 >= SAFECALL && LA252_0 <= SEALED)||LA252_0==SQUARE_BRACKET_LEFT||(LA252_0 >= STATIC && LA252_0 <= STRING)||LA252_0==TRY||LA252_0==TkBinaryNumber||LA252_0==TkCharacterEscapeCode||(LA252_0 >= TkHexNumber && LA252_0 <= TkIntNumber)||LA252_0==TkMultilineString||(LA252_0 >= TkQuotedString && LA252_0 <= TkRealNumber)||LA252_0==UNSAFE||(LA252_0 >= VAR && LA252_0 <= VIRTUAL)||LA252_0==WHILE||(LA252_0 >= WINAPI && LA252_0 <= WRITEONLY)) ) {
alt252=1;
}
else if ( (LA252_0==SEMICOLON) ) {
alt252=2;
}
switch (alt252) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1037:33: statement
{
pushFollow(FOLLOW_statement_in_delimitedStatements20777);
statement753=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement753.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1037:45: ';'
{
char_literal754=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_delimitedStatements20781); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal754_tree = (Object)adaptor.create(char_literal754);
adaptor.addChild(root_0, char_literal754_tree);
}
}
break;
default :
if ( cnt252 >= 1 ) break loop252;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(252, input);
throw eee;
}
cnt252++;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 186, delimitedStatements_StartIndex); }
}
return retval;
}
// $ANTLR end "delimitedStatements"
public static class labelStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "labelStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1039:1: labelStatement :{...}? => labelNameReference ':' ( statement )? -> ^( TkLabelStatement labelNameReference ':' ( statement )? ) ;
public final DelphiParser.labelStatement_return labelStatement() throws RecognitionException {
DelphiParser.labelStatement_return retval = new DelphiParser.labelStatement_return();
retval.start = input.LT(1);
int labelStatement_StartIndex = input.index();
Object root_0 = null;
Token char_literal756=null;
ParserRuleReturnScope labelNameReference755 =null;
ParserRuleReturnScope statement757 =null;
Object char_literal756_tree=null;
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_labelNameReference=new RewriteRuleSubtreeStream(adaptor,"rule labelNameReference");
RewriteRuleSubtreeStream stream_statement=new RewriteRuleSubtreeStream(adaptor,"rule statement");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 187) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1039:30: ({...}? => labelNameReference ':' ( statement )? -> ^( TkLabelStatement labelNameReference ':' ( statement )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1039:32: {...}? => labelNameReference ':' ( statement )?
{
if ( !((input.LA(2) == COLON)) ) {
if (state.backtracking>0) {state.failed=true; return retval;}
throw new FailedPredicateException(input, "labelStatement", "input.LA(2) == COLON");
}
pushFollow(FOLLOW_labelNameReference_in_labelStatement20838);
labelNameReference755=labelNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_labelNameReference.add(labelNameReference755.getTree());
char_literal756=(Token)match(input,COLON,FOLLOW_COLON_in_labelStatement20840); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(char_literal756);
// au/com/integradev/delphi/antlr/Delphi.g:1039:82: ( statement )?
int alt253=2;
switch ( input.LA(1) ) {
case IF:
{
int LA253_1 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case VAR:
{
int LA253_2 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case CONST:
{
int LA253_3 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case CASE:
{
int LA253_4 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case REPEAT:
{
int LA253_5 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case WHILE:
{
int LA253_6 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case FOR:
{
int LA253_7 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case WITH:
{
int LA253_8 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TRY:
{
int LA253_9 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case RAISE:
{
int LA253_10 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case ASM:
{
int LA253_11 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case BEGIN:
{
int LA253_12 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkIdentifier:
{
int LA253_13 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case ON:
{
int LA253_14 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkIntNumber:
{
int LA253_15 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkHexNumber:
{
int LA253_16 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkBinaryNumber:
{
int LA253_17 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case NOT:
{
int LA253_18 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case PLUS:
{
int LA253_19 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case MINUS:
{
int LA253_20 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case ADDRESS:
{
int LA253_21 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkRealNumber:
{
int LA253_22 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkQuotedString:
{
int LA253_23 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA253_24 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case DEREFERENCE:
{
int LA253_25 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case TkMultilineString:
{
int LA253_26 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case NIL:
{
int LA253_27 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA253_28 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case STRING:
{
int LA253_29 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case FILE:
{
int LA253_30 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case PAREN_LEFT:
{
int LA253_31 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case INHERITED:
{
int LA253_32 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case PROCEDURE:
{
int LA253_33 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case FUNCTION:
{
int LA253_34 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case GOTO:
{
int LA253_35 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA253_44 = input.LA(2);
if ( (synpred369_Delphi()) ) {
alt253=1;
}
}
break;
}
switch (alt253) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1039:82: statement
{
pushFollow(FOLLOW_statement_in_labelStatement20842);
statement757=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(statement757.getTree());
}
break;
}
// AST REWRITE
// elements: statement, labelNameReference, COLON
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1040:30: -> ^( TkLabelStatement labelNameReference ':' ( statement )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1040:33: ^( TkLabelStatement labelNameReference ':' ( statement )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new LabelStatementNodeImpl(TkLabelStatement), root_1);
adaptor.addChild(root_1, stream_labelNameReference.nextTree());
adaptor.addChild(root_1, stream_COLON.nextNode());
// au/com/integradev/delphi/antlr/Delphi.g:1040:99: ( statement )?
if ( stream_statement.hasNext() ) {
adaptor.addChild(root_1, stream_statement.nextTree());
}
stream_statement.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 187, labelStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "labelStatement"
public static class assignmentStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "assignmentStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1042:1: assignmentStatement : expression ':=' ^ expression ;
public final DelphiParser.assignmentStatement_return assignmentStatement() throws RecognitionException {
DelphiParser.assignmentStatement_return retval = new DelphiParser.assignmentStatement_return();
retval.start = input.LT(1);
int assignmentStatement_StartIndex = input.index();
Object root_0 = null;
Token string_literal759=null;
ParserRuleReturnScope expression758 =null;
ParserRuleReturnScope expression760 =null;
Object string_literal759_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 188) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1042:30: ( expression ':=' ^ expression )
// au/com/integradev/delphi/antlr/Delphi.g:1042:32: expression ':=' ^ expression
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_expression_in_assignmentStatement20934);
expression758=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression758.getTree());
string_literal759=(Token)match(input,ASSIGN,FOLLOW_ASSIGN_in_assignmentStatement20936); if (state.failed) return retval;
if ( state.backtracking==0 ) {
string_literal759_tree = new AssignmentStatementNodeImpl(string_literal759) ;
root_0 = (Object)adaptor.becomeRoot(string_literal759_tree, root_0);
}
pushFollow(FOLLOW_expression_in_assignmentStatement20942);
expression760=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression760.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 188, assignmentStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "assignmentStatement"
public static class expressionStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "expressionStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1044:1: expressionStatement : expression -> ^( TkExpressionStatement expression ) ;
public final DelphiParser.expressionStatement_return expressionStatement() throws RecognitionException {
DelphiParser.expressionStatement_return retval = new DelphiParser.expressionStatement_return();
retval.start = input.LT(1);
int expressionStatement_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope expression761 =null;
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 189) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1044:30: ( expression -> ^( TkExpressionStatement expression ) )
// au/com/integradev/delphi/antlr/Delphi.g:1044:32: expression
{
pushFollow(FOLLOW_expression_in_expressionStatement20988);
expression761=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression761.getTree());
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1044:43: -> ^( TkExpressionStatement expression )
{
// au/com/integradev/delphi/antlr/Delphi.g:1044:46: ^( TkExpressionStatement expression )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new ExpressionStatementNodeImpl(TkExpressionStatement), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 189, expressionStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "expressionStatement"
public static class gotoStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "gotoStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1046:1: gotoStatement : GOTO ^ labelNameReference ;
public final DelphiParser.gotoStatement_return gotoStatement() throws RecognitionException {
DelphiParser.gotoStatement_return retval = new DelphiParser.gotoStatement_return();
retval.start = input.LT(1);
int gotoStatement_StartIndex = input.index();
Object root_0 = null;
Token GOTO762=null;
ParserRuleReturnScope labelNameReference763 =null;
Object GOTO762_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 190) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1046:30: ( GOTO ^ labelNameReference )
// au/com/integradev/delphi/antlr/Delphi.g:1046:32: GOTO ^ labelNameReference
{
root_0 = (Object)adaptor.nil();
GOTO762=(Token)match(input,GOTO,FOLLOW_GOTO_in_gotoStatement21051); if (state.failed) return retval;
if ( state.backtracking==0 ) {
GOTO762_tree = new GotoStatementNodeImpl(GOTO762) ;
root_0 = (Object)adaptor.becomeRoot(GOTO762_tree, root_0);
}
pushFollow(FOLLOW_labelNameReference_in_gotoStatement21057);
labelNameReference763=labelNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, labelNameReference763.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 190, gotoStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "gotoStatement"
public static class tryStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "tryStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1048:1: tryStatement : TRY ^ statementList ( exceptBlock | finallyBlock ) END ;
public final DelphiParser.tryStatement_return tryStatement() throws RecognitionException {
DelphiParser.tryStatement_return retval = new DelphiParser.tryStatement_return();
retval.start = input.LT(1);
int tryStatement_StartIndex = input.index();
Object root_0 = null;
Token TRY764=null;
Token END768=null;
ParserRuleReturnScope statementList765 =null;
ParserRuleReturnScope exceptBlock766 =null;
ParserRuleReturnScope finallyBlock767 =null;
Object TRY764_tree=null;
Object END768_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 191) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1048:30: ( TRY ^ statementList ( exceptBlock | finallyBlock ) END )
// au/com/integradev/delphi/antlr/Delphi.g:1048:32: TRY ^ statementList ( exceptBlock | finallyBlock ) END
{
root_0 = (Object)adaptor.nil();
TRY764=(Token)match(input,TRY,FOLLOW_TRY_in_tryStatement21110); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TRY764_tree = new TryStatementNodeImpl(TRY764) ;
root_0 = (Object)adaptor.becomeRoot(TRY764_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_tryStatement21116);
statementList765=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList765.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1048:73: ( exceptBlock | finallyBlock )
int alt254=2;
int LA254_0 = input.LA(1);
if ( (LA254_0==EXCEPT) ) {
alt254=1;
}
else if ( (LA254_0==FINALLY) ) {
alt254=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 254, 0, input);
throw nvae;
}
switch (alt254) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1048:74: exceptBlock
{
pushFollow(FOLLOW_exceptBlock_in_tryStatement21119);
exceptBlock766=exceptBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, exceptBlock766.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1048:88: finallyBlock
{
pushFollow(FOLLOW_finallyBlock_in_tryStatement21123);
finallyBlock767=finallyBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, finallyBlock767.getTree());
}
break;
}
END768=(Token)match(input,END,FOLLOW_END_in_tryStatement21126); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END768_tree = (Object)adaptor.create(END768);
adaptor.addChild(root_0, END768_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 191, tryStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "tryStatement"
public static class exceptBlock_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "exceptBlock"
// au/com/integradev/delphi/antlr/Delphi.g:1050:1: exceptBlock : EXCEPT ^ handlerList ;
public final DelphiParser.exceptBlock_return exceptBlock() throws RecognitionException {
DelphiParser.exceptBlock_return retval = new DelphiParser.exceptBlock_return();
retval.start = input.LT(1);
int exceptBlock_StartIndex = input.index();
Object root_0 = null;
Token EXCEPT769=null;
ParserRuleReturnScope handlerList770 =null;
Object EXCEPT769_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 192) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1050:30: ( EXCEPT ^ handlerList )
// au/com/integradev/delphi/antlr/Delphi.g:1050:32: EXCEPT ^ handlerList
{
root_0 = (Object)adaptor.nil();
EXCEPT769=(Token)match(input,EXCEPT,FOLLOW_EXCEPT_in_exceptBlock21180); if (state.failed) return retval;
if ( state.backtracking==0 ) {
EXCEPT769_tree = new ExceptBlockNodeImpl(EXCEPT769) ;
root_0 = (Object)adaptor.becomeRoot(EXCEPT769_tree, root_0);
}
pushFollow(FOLLOW_handlerList_in_exceptBlock21186);
handlerList770=handlerList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, handlerList770.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 192, exceptBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "exceptBlock"
public static class finallyBlock_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "finallyBlock"
// au/com/integradev/delphi/antlr/Delphi.g:1052:1: finallyBlock : FINALLY ^ statementList ;
public final DelphiParser.finallyBlock_return finallyBlock() throws RecognitionException {
DelphiParser.finallyBlock_return retval = new DelphiParser.finallyBlock_return();
retval.start = input.LT(1);
int finallyBlock_StartIndex = input.index();
Object root_0 = null;
Token FINALLY771=null;
ParserRuleReturnScope statementList772 =null;
Object FINALLY771_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 193) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1052:30: ( FINALLY ^ statementList )
// au/com/integradev/delphi/antlr/Delphi.g:1052:32: FINALLY ^ statementList
{
root_0 = (Object)adaptor.nil();
FINALLY771=(Token)match(input,FINALLY,FOLLOW_FINALLY_in_finallyBlock21239); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FINALLY771_tree = new FinallyBlockNodeImpl(FINALLY771) ;
root_0 = (Object)adaptor.becomeRoot(FINALLY771_tree, root_0);
}
pushFollow(FOLLOW_statementList_in_finallyBlock21245);
statementList772=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList772.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 193, finallyBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "finallyBlock"
public static class handlerList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "handlerList"
// au/com/integradev/delphi/antlr/Delphi.g:1054:1: handlerList : ( ( handler )+ ( elseBlock )? | statementList );
public final DelphiParser.handlerList_return handlerList() throws RecognitionException {
DelphiParser.handlerList_return retval = new DelphiParser.handlerList_return();
retval.start = input.LT(1);
int handlerList_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope handler773 =null;
ParserRuleReturnScope elseBlock774 =null;
ParserRuleReturnScope statementList775 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 194) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1054:30: ( ( handler )+ ( elseBlock )? | statementList )
int alt257=2;
int LA257_0 = input.LA(1);
if ( (LA257_0==ON) ) {
int LA257_1 = input.LA(2);
if ( (synpred373_Delphi()) ) {
alt257=1;
}
else if ( (true) ) {
alt257=2;
}
}
else if ( (LA257_0==EOF||(LA257_0 >= ABSOLUTE && LA257_0 <= ALIGN)||(LA257_0 >= ASM && LA257_0 <= ASSEMBLY)||(LA257_0 >= AT && LA257_0 <= AUTOMATED)||LA257_0==BEGIN||(LA257_0 >= CASE && LA257_0 <= CDECL)||LA257_0==CONST||LA257_0==CONTAINS||(LA257_0 >= DEFAULT && LA257_0 <= DEREFERENCE)||LA257_0==DISPID||LA257_0==DYNAMIC||LA257_0==END||(LA257_0 >= EXPERIMENTAL && LA257_0 <= EXPORT)||LA257_0==EXTERNAL||(LA257_0 >= FAR && LA257_0 <= FINAL)||(LA257_0 >= FOR && LA257_0 <= FUNCTION)||LA257_0==GOTO||LA257_0==HELPER||LA257_0==IF||LA257_0==IMPLEMENTS||(LA257_0 >= INDEX && LA257_0 <= INHERITED)||LA257_0==LABEL||LA257_0==LOCAL||(LA257_0 >= MESSAGE && LA257_0 <= MINUS)||(LA257_0 >= NAME && LA257_0 <= NOT)||LA257_0==OPERATOR||(LA257_0 >= OUT && LA257_0 <= OVERRIDE)||LA257_0==PACKAGE||LA257_0==PAREN_BRACKET_LEFT||LA257_0==PAREN_LEFT||(LA257_0 >= PASCAL && LA257_0 <= PROCEDURE)||(LA257_0 >= PROTECTED && LA257_0 <= PUBLISHED)||(LA257_0 >= RAISE && LA257_0 <= READONLY)||(LA257_0 >= REFERENCE && LA257_0 <= RESIDENT)||(LA257_0 >= SAFECALL && LA257_0 <= SEMICOLON)||LA257_0==SQUARE_BRACKET_LEFT||(LA257_0 >= STATIC && LA257_0 <= STRING)||LA257_0==TRY||LA257_0==TkBinaryNumber||LA257_0==TkCharacterEscapeCode||(LA257_0 >= TkHexNumber && LA257_0 <= TkIntNumber)||LA257_0==TkMultilineString||(LA257_0 >= TkQuotedString && LA257_0 <= TkRealNumber)||LA257_0==UNSAFE||(LA257_0 >= VAR && LA257_0 <= VIRTUAL)||LA257_0==WHILE||(LA257_0 >= WINAPI && LA257_0 <= WRITEONLY)) ) {
alt257=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 257, 0, input);
throw nvae;
}
switch (alt257) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: ( handler )+ ( elseBlock )?
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: ( handler )+
int cnt255=0;
loop255:
while (true) {
int alt255=2;
int LA255_0 = input.LA(1);
if ( (LA255_0==ON) ) {
alt255=1;
}
switch (alt255) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: handler
{
pushFollow(FOLLOW_handler_in_handlerList21299);
handler773=handler();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, handler773.getTree());
}
break;
default :
if ( cnt255 >= 1 ) break loop255;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(255, input);
throw eee;
}
cnt255++;
}
// au/com/integradev/delphi/antlr/Delphi.g:1054:41: ( elseBlock )?
int alt256=2;
int LA256_0 = input.LA(1);
if ( (LA256_0==ELSE) ) {
alt256=1;
}
switch (alt256) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1054:41: elseBlock
{
pushFollow(FOLLOW_elseBlock_in_handlerList21302);
elseBlock774=elseBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, elseBlock774.getTree());
}
break;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1055:32: statementList
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_statementList_in_handlerList21336);
statementList775=statementList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statementList775.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 194, handlerList_StartIndex); }
}
return retval;
}
// $ANTLR end "handlerList"
public static class handler_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "handler"
// au/com/integradev/delphi/antlr/Delphi.g:1057:1: handler : ON ^ ( nameDeclaration ':' !)? typeReference DO ( statement )? ( ';' )? ;
public final DelphiParser.handler_return handler() throws RecognitionException {
DelphiParser.handler_return retval = new DelphiParser.handler_return();
retval.start = input.LT(1);
int handler_StartIndex = input.index();
Object root_0 = null;
Token ON776=null;
Token char_literal778=null;
Token DO780=null;
Token char_literal782=null;
ParserRuleReturnScope nameDeclaration777 =null;
ParserRuleReturnScope typeReference779 =null;
ParserRuleReturnScope statement781 =null;
Object ON776_tree=null;
Object char_literal778_tree=null;
Object DO780_tree=null;
Object char_literal782_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 195) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1057:30: ( ON ^ ( nameDeclaration ':' !)? typeReference DO ( statement )? ( ';' )? )
// au/com/integradev/delphi/antlr/Delphi.g:1057:32: ON ^ ( nameDeclaration ':' !)? typeReference DO ( statement )? ( ';' )?
{
root_0 = (Object)adaptor.nil();
ON776=(Token)match(input,ON,FOLLOW_ON_in_handler21394); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ON776_tree = new ExceptItemNodeImpl(ON776) ;
root_0 = (Object)adaptor.becomeRoot(ON776_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:1057:56: ( nameDeclaration ':' !)?
int alt258=2;
int LA258_0 = input.LA(1);
if ( (LA258_0==TkIdentifier) ) {
int LA258_1 = input.LA(2);
if ( (LA258_1==COLON) ) {
alt258=1;
}
}
else if ( ((LA258_0 >= ABSOLUTE && LA258_0 <= ABSTRACT)||LA258_0==ALIGN||(LA258_0 >= ASSEMBLER && LA258_0 <= ASSEMBLY)||(LA258_0 >= AT && LA258_0 <= AUTOMATED)||LA258_0==CDECL||LA258_0==CONTAINS||(LA258_0 >= DEFAULT && LA258_0 <= DEPRECATED)||LA258_0==DISPID||LA258_0==DYNAMIC||(LA258_0 >= EXPERIMENTAL && LA258_0 <= EXPORT)||LA258_0==EXTERNAL||LA258_0==FAR||LA258_0==FINAL||LA258_0==FORWARD||LA258_0==HELPER||LA258_0==IMPLEMENTS||LA258_0==INDEX||LA258_0==LABEL||LA258_0==LOCAL||LA258_0==MESSAGE||(LA258_0 >= NAME && LA258_0 <= NEAR)||LA258_0==NODEFAULT||(LA258_0 >= ON && LA258_0 <= OPERATOR)||(LA258_0 >= OUT && LA258_0 <= OVERRIDE)||LA258_0==PACKAGE||(LA258_0 >= PASCAL && LA258_0 <= PLATFORM)||LA258_0==PRIVATE||(LA258_0 >= PROTECTED && LA258_0 <= PUBLISHED)||(LA258_0 >= READ && LA258_0 <= READONLY)||(LA258_0 >= REFERENCE && LA258_0 <= REINTRODUCE)||(LA258_0 >= REQUIRES && LA258_0 <= RESIDENT)||(LA258_0 >= SAFECALL && LA258_0 <= SEALED)||(LA258_0 >= STATIC && LA258_0 <= STRICT)||LA258_0==UNSAFE||(LA258_0 >= VARARGS && LA258_0 <= VIRTUAL)||LA258_0==WINAPI||(LA258_0 >= WRITE && LA258_0 <= WRITEONLY)) ) {
int LA258_2 = input.LA(2);
if ( (LA258_2==COLON) ) {
alt258=1;
}
}
switch (alt258) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1057:57: nameDeclaration ':' !
{
pushFollow(FOLLOW_nameDeclaration_in_handler21401);
nameDeclaration777=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, nameDeclaration777.getTree());
char_literal778=(Token)match(input,COLON,FOLLOW_COLON_in_handler21403); if (state.failed) return retval;
}
break;
}
pushFollow(FOLLOW_typeReference_in_handler21408);
typeReference779=typeReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, typeReference779.getTree());
DO780=(Token)match(input,DO,FOLLOW_DO_in_handler21410); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DO780_tree = (Object)adaptor.create(DO780);
adaptor.addChild(root_0, DO780_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1057:97: ( statement )?
int alt259=2;
int LA259_0 = input.LA(1);
if ( ((LA259_0 >= ABSOLUTE && LA259_0 <= ALIGN)||(LA259_0 >= ASM && LA259_0 <= ASSEMBLY)||(LA259_0 >= AT && LA259_0 <= AUTOMATED)||LA259_0==BEGIN||(LA259_0 >= CASE && LA259_0 <= CDECL)||LA259_0==CONST||LA259_0==CONTAINS||(LA259_0 >= DEFAULT && LA259_0 <= DEREFERENCE)||LA259_0==DISPID||LA259_0==DYNAMIC||(LA259_0 >= EXPERIMENTAL && LA259_0 <= EXPORT)||LA259_0==EXTERNAL||(LA259_0 >= FAR && LA259_0 <= FINAL)||(LA259_0 >= FOR && LA259_0 <= FUNCTION)||LA259_0==GOTO||LA259_0==HELPER||LA259_0==IF||LA259_0==IMPLEMENTS||(LA259_0 >= INDEX && LA259_0 <= INHERITED)||LA259_0==LABEL||LA259_0==LOCAL||(LA259_0 >= MESSAGE && LA259_0 <= MINUS)||(LA259_0 >= NAME && LA259_0 <= NOT)||LA259_0==OPERATOR||(LA259_0 >= OUT && LA259_0 <= OVERRIDE)||LA259_0==PACKAGE||LA259_0==PAREN_BRACKET_LEFT||LA259_0==PAREN_LEFT||(LA259_0 >= PASCAL && LA259_0 <= PROCEDURE)||(LA259_0 >= PROTECTED && LA259_0 <= PUBLISHED)||(LA259_0 >= RAISE && LA259_0 <= READONLY)||(LA259_0 >= REFERENCE && LA259_0 <= RESIDENT)||(LA259_0 >= SAFECALL && LA259_0 <= SEALED)||LA259_0==SQUARE_BRACKET_LEFT||(LA259_0 >= STATIC && LA259_0 <= STRING)||LA259_0==TRY||LA259_0==TkBinaryNumber||LA259_0==TkCharacterEscapeCode||(LA259_0 >= TkHexNumber && LA259_0 <= TkIntNumber)||LA259_0==TkMultilineString||(LA259_0 >= TkQuotedString && LA259_0 <= TkRealNumber)||LA259_0==UNSAFE||(LA259_0 >= VAR && LA259_0 <= VIRTUAL)||LA259_0==WHILE||(LA259_0 >= WINAPI && LA259_0 <= WRITEONLY)) ) {
alt259=1;
}
else if ( (LA259_0==ON) ) {
int LA259_2 = input.LA(2);
if ( (LA259_2==EOF||LA259_2==AND||LA259_2==AS||LA259_2==ASSIGN||LA259_2==COLON||LA259_2==DEREFERENCE||(LA259_2 >= DIV && LA259_2 <= DIVIDE)||LA259_2==DOT||(LA259_2 >= ELSE && LA259_2 <= EQUAL)||(LA259_2 >= GREATER_THAN && LA259_2 <= GREATER_THAN_EQUAL)||LA259_2==IN||LA259_2==IS||(LA259_2 >= LESS_THAN && LA259_2 <= LESS_THAN_EQUAL)||(LA259_2 >= MINUS && LA259_2 <= MULTIPLY)||LA259_2==NOT_EQUAL||LA259_2==OR||LA259_2==PAREN_BRACKET_LEFT||LA259_2==PAREN_LEFT||LA259_2==PLUS||LA259_2==SEMICOLON||(LA259_2 >= SHL && LA259_2 <= SQUARE_BRACKET_LEFT)||LA259_2==XOR) ) {
alt259=1;
}
else if ( (LA259_2==ON) ) {
int LA259_4 = input.LA(3);
if ( ((LA259_4 >= ABSOLUTE && LA259_4 <= ABSTRACT)||LA259_4==ALIGN||(LA259_4 >= ASSEMBLER && LA259_4 <= ASSEMBLY)||(LA259_4 >= AT && LA259_4 <= AUTOMATED)||LA259_4==CDECL||LA259_4==CONTAINS||(LA259_4 >= DEFAULT && LA259_4 <= DEPRECATED)||LA259_4==DISPID||LA259_4==DYNAMIC||(LA259_4 >= EXPERIMENTAL && LA259_4 <= EXPORT)||LA259_4==EXTERNAL||LA259_4==FAR||LA259_4==FINAL||LA259_4==FORWARD||LA259_4==HELPER||LA259_4==IMPLEMENTS||LA259_4==INDEX||LA259_4==LABEL||LA259_4==LOCAL||LA259_4==MESSAGE||(LA259_4 >= NAME && LA259_4 <= NEAR)||LA259_4==NODEFAULT||(LA259_4 >= ON && LA259_4 <= OPERATOR)||(LA259_4 >= OUT && LA259_4 <= OVERRIDE)||LA259_4==PACKAGE||(LA259_4 >= PASCAL && LA259_4 <= PLATFORM)||LA259_4==PRIVATE||(LA259_4 >= PROTECTED && LA259_4 <= PUBLISHED)||(LA259_4 >= READ && LA259_4 <= READONLY)||(LA259_4 >= REFERENCE && LA259_4 <= REINTRODUCE)||(LA259_4 >= REQUIRES && LA259_4 <= RESIDENT)||(LA259_4 >= SAFECALL && LA259_4 <= SEALED)||(LA259_4 >= STATIC && LA259_4 <= STRICT)||LA259_4==TkIdentifier||LA259_4==UNSAFE||(LA259_4 >= VARARGS && LA259_4 <= VIRTUAL)||LA259_4==WINAPI||(LA259_4 >= WRITE && LA259_4 <= WRITEONLY)) ) {
alt259=1;
}
}
}
switch (alt259) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1057:97: statement
{
pushFollow(FOLLOW_statement_in_handler21412);
statement781=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement781.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1057:108: ( ';' )?
int alt260=2;
int LA260_0 = input.LA(1);
if ( (LA260_0==SEMICOLON) ) {
alt260=1;
}
switch (alt260) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1057:109: ';'
{
char_literal782=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_handler21416); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal782_tree = (Object)adaptor.create(char_literal782);
adaptor.addChild(root_0, char_literal782_tree);
}
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 195, handler_StartIndex); }
}
return retval;
}
// $ANTLR end "handler"
public static class raiseStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "raiseStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1059:1: raiseStatement : RAISE ^ ( expression )? ( AT ! expression )? ;
public final DelphiParser.raiseStatement_return raiseStatement() throws RecognitionException {
DelphiParser.raiseStatement_return retval = new DelphiParser.raiseStatement_return();
retval.start = input.LT(1);
int raiseStatement_StartIndex = input.index();
Object root_0 = null;
Token RAISE783=null;
Token AT785=null;
ParserRuleReturnScope expression784 =null;
ParserRuleReturnScope expression786 =null;
Object RAISE783_tree=null;
Object AT785_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 196) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1059:30: ( RAISE ^ ( expression )? ( AT ! expression )? )
// au/com/integradev/delphi/antlr/Delphi.g:1059:32: RAISE ^ ( expression )? ( AT ! expression )?
{
root_0 = (Object)adaptor.nil();
RAISE783=(Token)match(input,RAISE,FOLLOW_RAISE_in_raiseStatement21469); if (state.failed) return retval;
if ( state.backtracking==0 ) {
RAISE783_tree = new RaiseStatementNodeImpl(RAISE783) ;
root_0 = (Object)adaptor.becomeRoot(RAISE783_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:1059:63: ( expression )?
int alt261=2;
switch ( input.LA(1) ) {
case NOT:
{
int LA261_1 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case PLUS:
{
int LA261_2 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case MINUS:
{
int LA261_3 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case ADDRESS:
{
int LA261_4 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkIntNumber:
{
int LA261_5 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkHexNumber:
{
int LA261_6 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkBinaryNumber:
{
int LA261_7 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkRealNumber:
{
int LA261_8 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkQuotedString:
{
int LA261_9 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA261_10 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case DEREFERENCE:
{
int LA261_11 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkMultilineString:
{
int LA261_12 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case NIL:
{
int LA261_13 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case TkIdentifier:
{
int LA261_14 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case AT:
{
int LA261_15 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case PAREN_BRACKET_LEFT:
case SQUARE_BRACKET_LEFT:
{
int LA261_16 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case STRING:
{
int LA261_17 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case FILE:
{
int LA261_18 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case PAREN_LEFT:
{
int LA261_19 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case INHERITED:
{
int LA261_20 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case PROCEDURE:
{
int LA261_21 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case FUNCTION:
{
int LA261_22 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case ON:
{
int LA261_23 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA261_45 = input.LA(2);
if ( (synpred377_Delphi()) ) {
alt261=1;
}
}
break;
}
switch (alt261) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1059:63: expression
{
pushFollow(FOLLOW_expression_in_raiseStatement21475);
expression784=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression784.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1059:75: ( AT ! expression )?
int alt262=2;
int LA262_0 = input.LA(1);
if ( (LA262_0==AT) ) {
int LA262_1 = input.LA(2);
if ( (synpred378_Delphi()) ) {
alt262=1;
}
}
switch (alt262) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1059:76: AT ! expression
{
AT785=(Token)match(input,AT,FOLLOW_AT_in_raiseStatement21479); if (state.failed) return retval;
pushFollow(FOLLOW_expression_in_raiseStatement21482);
expression786=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression786.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 196, raiseStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "raiseStatement"
public static class assemblerStatement_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "assemblerStatement"
// au/com/integradev/delphi/antlr/Delphi.g:1061:1: assemblerStatement : ASM ^ assemblerInstructions END ;
public final DelphiParser.assemblerStatement_return assemblerStatement() throws RecognitionException {
DelphiParser.assemblerStatement_return retval = new DelphiParser.assemblerStatement_return();
retval.start = input.LT(1);
int assemblerStatement_StartIndex = input.index();
Object root_0 = null;
Token ASM787=null;
Token END789=null;
ParserRuleReturnScope assemblerInstructions788 =null;
Object ASM787_tree=null;
Object END789_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 197) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1061:30: ( ASM ^ assemblerInstructions END )
// au/com/integradev/delphi/antlr/Delphi.g:1061:32: ASM ^ assemblerInstructions END
{
root_0 = (Object)adaptor.nil();
ASM787=(Token)match(input,ASM,FOLLOW_ASM_in_assemblerStatement21531); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ASM787_tree = new AsmStatementNodeImpl(ASM787) ;
root_0 = (Object)adaptor.becomeRoot(ASM787_tree, root_0);
}
pushFollow(FOLLOW_assemblerInstructions_in_assemblerStatement21537);
assemblerInstructions788=assemblerInstructions();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, assemblerInstructions788.getTree());
END789=(Token)match(input,END,FOLLOW_END_in_assemblerStatement21539); if (state.failed) return retval;
if ( state.backtracking==0 ) {
END789_tree = (Object)adaptor.create(END789);
adaptor.addChild(root_0, END789_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 197, assemblerStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "assemblerStatement"
public static class assemblerInstructions_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "assemblerInstructions"
// au/com/integradev/delphi/antlr/Delphi.g:1063:1: assemblerInstructions : (~ ( END ) )* ;
public final DelphiParser.assemblerInstructions_return assemblerInstructions() throws RecognitionException {
DelphiParser.assemblerInstructions_return retval = new DelphiParser.assemblerInstructions_return();
retval.start = input.LT(1);
int assemblerInstructions_StartIndex = input.index();
Object root_0 = null;
Token set790=null;
Object set790_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 198) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1063:30: ( (~ ( END ) )* )
// au/com/integradev/delphi/antlr/Delphi.g:1063:32: (~ ( END ) )*
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1063:32: (~ ( END ) )*
loop263:
while (true) {
int alt263=2;
int LA263_0 = input.LA(1);
if ( ((LA263_0 >= A && LA263_0 <= ELSE)||(LA263_0 >= EQUAL && LA263_0 <= Z)) ) {
alt263=1;
}
switch (alt263) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:
{
set790=input.LT(1);
if ( (input.LA(1) >= A && input.LA(1) <= ELSE)||(input.LA(1) >= EQUAL && input.LA(1) <= Z) ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set790));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
break;
default :
break loop263;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 198, assemblerInstructions_StartIndex); }
}
return retval;
}
// $ANTLR end "assemblerInstructions"
public static class implDirectiveSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "implDirectiveSection"
// au/com/integradev/delphi/antlr/Delphi.g:1069:1: implDirectiveSection : ( ( ( ';' )? implDirective )* ';' | ( ';' implDirective )+ );
public final DelphiParser.implDirectiveSection_return implDirectiveSection() throws RecognitionException {
DelphiParser.implDirectiveSection_return retval = new DelphiParser.implDirectiveSection_return();
retval.start = input.LT(1);
int implDirectiveSection_StartIndex = input.index();
Object root_0 = null;
Token char_literal791=null;
Token char_literal793=null;
Token char_literal794=null;
ParserRuleReturnScope implDirective792 =null;
ParserRuleReturnScope implDirective795 =null;
Object char_literal791_tree=null;
Object char_literal793_tree=null;
Object char_literal794_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 199) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1069:30: ( ( ( ';' )? implDirective )* ';' | ( ';' implDirective )+ )
int alt267=2;
int LA267_0 = input.LA(1);
if ( (LA267_0==SEMICOLON) ) {
int LA267_1 = input.LA(2);
if ( (synpred383_Delphi()) ) {
alt267=1;
}
else if ( (true) ) {
alt267=2;
}
}
else if ( (LA267_0==ABSTRACT||LA267_0==ASSEMBLER||LA267_0==CDECL||LA267_0==DEPRECATED||LA267_0==DISPID||LA267_0==DYNAMIC||(LA267_0 >= EXPERIMENTAL && LA267_0 <= EXPORT)||LA267_0==FAR||LA267_0==FINAL||LA267_0==INLINE||(LA267_0 >= LIBRARY && LA267_0 <= LOCAL)||LA267_0==MESSAGE||LA267_0==NEAR||(LA267_0 >= OVERLOAD && LA267_0 <= OVERRIDE)||(LA267_0 >= PASCAL && LA267_0 <= PLATFORM)||(LA267_0 >= REGISTER && LA267_0 <= REINTRODUCE)||LA267_0==SAFECALL||(LA267_0 >= STATIC && LA267_0 <= STDCALL)||LA267_0==UNSAFE||(LA267_0 >= VARARGS && LA267_0 <= VIRTUAL)||LA267_0==WINAPI) ) {
alt267=1;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 267, 0, input);
throw nvae;
}
switch (alt267) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1069:32: ( ( ';' )? implDirective )* ';'
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1069:32: ( ( ';' )? implDirective )*
loop265:
while (true) {
int alt265=2;
int LA265_0 = input.LA(1);
if ( (LA265_0==SEMICOLON) ) {
int LA265_1 = input.LA(2);
if ( (LA265_1==ABSTRACT||LA265_1==ASSEMBLER||LA265_1==CDECL||LA265_1==DEPRECATED||LA265_1==DISPID||LA265_1==DYNAMIC||(LA265_1 >= EXPERIMENTAL && LA265_1 <= EXPORT)||LA265_1==FAR||LA265_1==FINAL||LA265_1==INLINE||(LA265_1 >= LIBRARY && LA265_1 <= LOCAL)||LA265_1==MESSAGE||LA265_1==NEAR||(LA265_1 >= OVERLOAD && LA265_1 <= OVERRIDE)||(LA265_1 >= PASCAL && LA265_1 <= PLATFORM)||(LA265_1 >= REGISTER && LA265_1 <= REINTRODUCE)||LA265_1==SAFECALL||(LA265_1 >= STATIC && LA265_1 <= STDCALL)||LA265_1==UNSAFE||(LA265_1 >= VARARGS && LA265_1 <= VIRTUAL)||LA265_1==WINAPI) ) {
alt265=1;
}
}
else if ( (LA265_0==ABSTRACT||LA265_0==ASSEMBLER||LA265_0==CDECL||LA265_0==DEPRECATED||LA265_0==DISPID||LA265_0==DYNAMIC||(LA265_0 >= EXPERIMENTAL && LA265_0 <= EXPORT)||LA265_0==FAR||LA265_0==FINAL||LA265_0==INLINE||(LA265_0 >= LIBRARY && LA265_0 <= LOCAL)||LA265_0==MESSAGE||LA265_0==NEAR||(LA265_0 >= OVERLOAD && LA265_0 <= OVERRIDE)||(LA265_0 >= PASCAL && LA265_0 <= PLATFORM)||(LA265_0 >= REGISTER && LA265_0 <= REINTRODUCE)||LA265_0==SAFECALL||(LA265_0 >= STATIC && LA265_0 <= STDCALL)||LA265_0==UNSAFE||(LA265_0 >= VARARGS && LA265_0 <= VIRTUAL)||LA265_0==WINAPI) ) {
alt265=1;
}
switch (alt265) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1069:33: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1069:33: ( ';' )?
int alt264=2;
int LA264_0 = input.LA(1);
if ( (LA264_0==SEMICOLON) ) {
alt264=1;
}
switch (alt264) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1069:33: ';'
{
char_literal791=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_implDirectiveSection21638); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal791_tree = (Object)adaptor.create(char_literal791);
adaptor.addChild(root_0, char_literal791_tree);
}
}
break;
}
pushFollow(FOLLOW_implDirective_in_implDirectiveSection21641);
implDirective792=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective792.getTree());
}
break;
default :
break loop265;
}
}
char_literal793=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_implDirectiveSection21645); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal793_tree = (Object)adaptor.create(char_literal793);
adaptor.addChild(root_0, char_literal793_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1070:32: ( ';' implDirective )+
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1070:32: ( ';' implDirective )+
int cnt266=0;
loop266:
while (true) {
int alt266=2;
int LA266_0 = input.LA(1);
if ( (LA266_0==SEMICOLON) ) {
alt266=1;
}
switch (alt266) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1070:33: ';' implDirective
{
char_literal794=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_implDirectiveSection21679); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal794_tree = (Object)adaptor.create(char_literal794);
adaptor.addChild(root_0, char_literal794_tree);
}
pushFollow(FOLLOW_implDirective_in_implDirectiveSection21681);
implDirective795=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective795.getTree());
}
break;
default :
if ( cnt266 >= 1 ) break loop266;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(266, input);
throw eee;
}
cnt266++;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 199, implDirectiveSection_StartIndex); }
}
return retval;
}
// $ANTLR end "implDirectiveSection"
public static class interfaceDirectiveSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceDirectiveSection"
// au/com/integradev/delphi/antlr/Delphi.g:1072:1: interfaceDirectiveSection : ( ( ( ';' )? interfaceDirective )* ';' | ( ';' interfaceDirective )+ );
public final DelphiParser.interfaceDirectiveSection_return interfaceDirectiveSection() throws RecognitionException {
DelphiParser.interfaceDirectiveSection_return retval = new DelphiParser.interfaceDirectiveSection_return();
retval.start = input.LT(1);
int interfaceDirectiveSection_StartIndex = input.index();
Object root_0 = null;
Token char_literal796=null;
Token char_literal798=null;
Token char_literal799=null;
ParserRuleReturnScope interfaceDirective797 =null;
ParserRuleReturnScope interfaceDirective800 =null;
Object char_literal796_tree=null;
Object char_literal798_tree=null;
Object char_literal799_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 200) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1072:30: ( ( ( ';' )? interfaceDirective )* ';' | ( ';' interfaceDirective )+ )
int alt271=2;
int LA271_0 = input.LA(1);
if ( (LA271_0==SEMICOLON) ) {
int LA271_1 = input.LA(2);
if ( (synpred387_Delphi()) ) {
alt271=1;
}
else if ( (true) ) {
alt271=2;
}
}
else if ( (LA271_0==ABSTRACT||LA271_0==ASSEMBLER||LA271_0==CDECL||LA271_0==DEPRECATED||LA271_0==DISPID||LA271_0==DYNAMIC||(LA271_0 >= EXPERIMENTAL && LA271_0 <= EXPORT)||LA271_0==EXTERNAL||LA271_0==FAR||LA271_0==FINAL||LA271_0==FORWARD||LA271_0==INLINE||(LA271_0 >= LIBRARY && LA271_0 <= LOCAL)||LA271_0==MESSAGE||LA271_0==NEAR||(LA271_0 >= OVERLOAD && LA271_0 <= OVERRIDE)||(LA271_0 >= PASCAL && LA271_0 <= PLATFORM)||(LA271_0 >= REGISTER && LA271_0 <= REINTRODUCE)||LA271_0==SAFECALL||(LA271_0 >= STATIC && LA271_0 <= STDCALL)||LA271_0==UNSAFE||(LA271_0 >= VARARGS && LA271_0 <= VIRTUAL)||LA271_0==WINAPI) ) {
alt271=1;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 271, 0, input);
throw nvae;
}
switch (alt271) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1072:32: ( ( ';' )? interfaceDirective )* ';'
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1072:32: ( ( ';' )? interfaceDirective )*
loop269:
while (true) {
int alt269=2;
int LA269_0 = input.LA(1);
if ( (LA269_0==SEMICOLON) ) {
switch ( input.LA(2) ) {
case FORWARD:
{
int LA269_4 = input.LA(3);
if ( (LA269_4==ABSTRACT||LA269_4==ASSEMBLER||LA269_4==CDECL||LA269_4==DEPRECATED||LA269_4==DISPID||LA269_4==DYNAMIC||(LA269_4 >= EXPERIMENTAL && LA269_4 <= EXPORT)||LA269_4==EXTERNAL||LA269_4==FAR||LA269_4==FINAL||LA269_4==FORWARD||LA269_4==INLINE||(LA269_4 >= LIBRARY && LA269_4 <= LOCAL)||LA269_4==MESSAGE||LA269_4==NEAR||(LA269_4 >= OVERLOAD && LA269_4 <= OVERRIDE)||(LA269_4 >= PASCAL && LA269_4 <= PLATFORM)||(LA269_4 >= REGISTER && LA269_4 <= REINTRODUCE)||LA269_4==SAFECALL||LA269_4==SEMICOLON||(LA269_4 >= STATIC && LA269_4 <= STDCALL)||LA269_4==UNSAFE||(LA269_4 >= VARARGS && LA269_4 <= VIRTUAL)||LA269_4==WINAPI) ) {
alt269=1;
}
}
break;
case EXTERNAL:
{
int LA269_5 = input.LA(3);
if ( ((LA269_5 >= ABSOLUTE && LA269_5 <= ALIGN)||(LA269_5 >= ASSEMBLER && LA269_5 <= ASSEMBLY)||(LA269_5 >= AT && LA269_5 <= AUTOMATED)||LA269_5==CDECL||LA269_5==CONTAINS||(LA269_5 >= DEFAULT && LA269_5 <= DEREFERENCE)||LA269_5==DISPID||LA269_5==DYNAMIC||(LA269_5 >= EXPERIMENTAL && LA269_5 <= EXPORT)||LA269_5==EXTERNAL||(LA269_5 >= FAR && LA269_5 <= FINAL)||(LA269_5 >= FORWARD && LA269_5 <= FUNCTION)||LA269_5==HELPER||LA269_5==IMPLEMENTS||(LA269_5 >= INDEX && LA269_5 <= INHERITED)||LA269_5==INLINE||LA269_5==LABEL||(LA269_5 >= LIBRARY && LA269_5 <= LOCAL)||(LA269_5 >= MESSAGE && LA269_5 <= MINUS)||(LA269_5 >= NAME && LA269_5 <= NOT)||(LA269_5 >= ON && LA269_5 <= OPERATOR)||(LA269_5 >= OUT && LA269_5 <= OVERRIDE)||LA269_5==PACKAGE||LA269_5==PAREN_BRACKET_LEFT||LA269_5==PAREN_LEFT||(LA269_5 >= PASCAL && LA269_5 <= PROCEDURE)||(LA269_5 >= PROTECTED && LA269_5 <= PUBLISHED)||(LA269_5 >= READ && LA269_5 <= READONLY)||(LA269_5 >= REFERENCE && LA269_5 <= REINTRODUCE)||(LA269_5 >= REQUIRES && LA269_5 <= RESIDENT)||(LA269_5 >= SAFECALL && LA269_5 <= SEMICOLON)||LA269_5==SQUARE_BRACKET_LEFT||(LA269_5 >= STATIC && LA269_5 <= STRING)||LA269_5==TkBinaryNumber||LA269_5==TkCharacterEscapeCode||(LA269_5 >= TkHexNumber && LA269_5 <= TkIntNumber)||LA269_5==TkMultilineString||(LA269_5 >= TkQuotedString && LA269_5 <= TkRealNumber)||LA269_5==UNSAFE||(LA269_5 >= VARARGS && LA269_5 <= VIRTUAL)||LA269_5==WINAPI||(LA269_5 >= WRITE && LA269_5 <= WRITEONLY)) ) {
alt269=1;
}
}
break;
case OVERLOAD:
{
int LA269_6 = input.LA(3);
if ( (LA269_6==ABSTRACT||LA269_6==ASSEMBLER||LA269_6==CDECL||LA269_6==DEPRECATED||LA269_6==DISPID||LA269_6==DYNAMIC||(LA269_6 >= EXPERIMENTAL && LA269_6 <= EXPORT)||LA269_6==EXTERNAL||LA269_6==FAR||LA269_6==FINAL||LA269_6==FORWARD||LA269_6==INLINE||(LA269_6 >= LIBRARY && LA269_6 <= LOCAL)||LA269_6==MESSAGE||LA269_6==NEAR||(LA269_6 >= OVERLOAD && LA269_6 <= OVERRIDE)||(LA269_6 >= PASCAL && LA269_6 <= PLATFORM)||(LA269_6 >= REGISTER && LA269_6 <= REINTRODUCE)||LA269_6==SAFECALL||LA269_6==SEMICOLON||(LA269_6 >= STATIC && LA269_6 <= STDCALL)||LA269_6==UNSAFE||(LA269_6 >= VARARGS && LA269_6 <= VIRTUAL)||LA269_6==WINAPI) ) {
alt269=1;
}
}
break;
case REINTRODUCE:
{
int LA269_7 = input.LA(3);
if ( (LA269_7==ABSTRACT||LA269_7==ASSEMBLER||LA269_7==CDECL||LA269_7==DEPRECATED||LA269_7==DISPID||LA269_7==DYNAMIC||(LA269_7 >= EXPERIMENTAL && LA269_7 <= EXPORT)||LA269_7==EXTERNAL||LA269_7==FAR||LA269_7==FINAL||LA269_7==FORWARD||LA269_7==INLINE||(LA269_7 >= LIBRARY && LA269_7 <= LOCAL)||LA269_7==MESSAGE||LA269_7==NEAR||(LA269_7 >= OVERLOAD && LA269_7 <= OVERRIDE)||(LA269_7 >= PASCAL && LA269_7 <= PLATFORM)||(LA269_7 >= REGISTER && LA269_7 <= REINTRODUCE)||LA269_7==SAFECALL||LA269_7==SEMICOLON||(LA269_7 >= STATIC && LA269_7 <= STDCALL)||LA269_7==UNSAFE||(LA269_7 >= VARARGS && LA269_7 <= VIRTUAL)||LA269_7==WINAPI) ) {
alt269=1;
}
}
break;
case MESSAGE:
{
int LA269_8 = input.LA(3);
if ( ((LA269_8 >= ABSOLUTE && LA269_8 <= ALIGN)||(LA269_8 >= ASSEMBLER && LA269_8 <= ASSEMBLY)||(LA269_8 >= AT && LA269_8 <= AUTOMATED)||LA269_8==CDECL||LA269_8==CONTAINS||(LA269_8 >= DEFAULT && LA269_8 <= DEREFERENCE)||LA269_8==DISPID||LA269_8==DYNAMIC||(LA269_8 >= EXPERIMENTAL && LA269_8 <= EXPORT)||LA269_8==EXTERNAL||(LA269_8 >= FAR && LA269_8 <= FINAL)||(LA269_8 >= FORWARD && LA269_8 <= FUNCTION)||LA269_8==HELPER||LA269_8==IMPLEMENTS||(LA269_8 >= INDEX && LA269_8 <= INHERITED)||LA269_8==LABEL||LA269_8==LOCAL||(LA269_8 >= MESSAGE && LA269_8 <= MINUS)||(LA269_8 >= NAME && LA269_8 <= NOT)||(LA269_8 >= ON && LA269_8 <= OPERATOR)||(LA269_8 >= OUT && LA269_8 <= OVERRIDE)||LA269_8==PACKAGE||LA269_8==PAREN_BRACKET_LEFT||LA269_8==PAREN_LEFT||(LA269_8 >= PASCAL && LA269_8 <= PROCEDURE)||(LA269_8 >= PROTECTED && LA269_8 <= PUBLISHED)||(LA269_8 >= READ && LA269_8 <= READONLY)||(LA269_8 >= REFERENCE && LA269_8 <= REINTRODUCE)||(LA269_8 >= REQUIRES && LA269_8 <= RESIDENT)||(LA269_8 >= SAFECALL && LA269_8 <= SEALED)||LA269_8==SQUARE_BRACKET_LEFT||(LA269_8 >= STATIC && LA269_8 <= STRING)||LA269_8==TkBinaryNumber||LA269_8==TkCharacterEscapeCode||(LA269_8 >= TkHexNumber && LA269_8 <= TkIntNumber)||LA269_8==TkMultilineString||(LA269_8 >= TkQuotedString && LA269_8 <= TkRealNumber)||LA269_8==UNSAFE||(LA269_8 >= VARARGS && LA269_8 <= VIRTUAL)||LA269_8==WINAPI||(LA269_8 >= WRITE && LA269_8 <= WRITEONLY)) ) {
alt269=1;
}
}
break;
case STATIC:
{
int LA269_9 = input.LA(3);
if ( (LA269_9==ABSTRACT||LA269_9==ASSEMBLER||LA269_9==CDECL||LA269_9==DEPRECATED||LA269_9==DISPID||LA269_9==DYNAMIC||(LA269_9 >= EXPERIMENTAL && LA269_9 <= EXPORT)||LA269_9==EXTERNAL||LA269_9==FAR||LA269_9==FINAL||LA269_9==FORWARD||LA269_9==INLINE||(LA269_9 >= LIBRARY && LA269_9 <= LOCAL)||LA269_9==MESSAGE||LA269_9==NEAR||(LA269_9 >= OVERLOAD && LA269_9 <= OVERRIDE)||(LA269_9 >= PASCAL && LA269_9 <= PLATFORM)||(LA269_9 >= REGISTER && LA269_9 <= REINTRODUCE)||LA269_9==SAFECALL||LA269_9==SEMICOLON||(LA269_9 >= STATIC && LA269_9 <= STDCALL)||LA269_9==UNSAFE||(LA269_9 >= VARARGS && LA269_9 <= VIRTUAL)||LA269_9==WINAPI) ) {
alt269=1;
}
}
break;
case DYNAMIC:
{
int LA269_10 = input.LA(3);
if ( (LA269_10==ABSTRACT||LA269_10==ASSEMBLER||LA269_10==CDECL||LA269_10==DEPRECATED||LA269_10==DISPID||LA269_10==DYNAMIC||(LA269_10 >= EXPERIMENTAL && LA269_10 <= EXPORT)||LA269_10==EXTERNAL||LA269_10==FAR||LA269_10==FINAL||LA269_10==FORWARD||LA269_10==INLINE||(LA269_10 >= LIBRARY && LA269_10 <= LOCAL)||LA269_10==MESSAGE||LA269_10==NEAR||(LA269_10 >= OVERLOAD && LA269_10 <= OVERRIDE)||(LA269_10 >= PASCAL && LA269_10 <= PLATFORM)||(LA269_10 >= REGISTER && LA269_10 <= REINTRODUCE)||LA269_10==SAFECALL||LA269_10==SEMICOLON||(LA269_10 >= STATIC && LA269_10 <= STDCALL)||LA269_10==UNSAFE||(LA269_10 >= VARARGS && LA269_10 <= VIRTUAL)||LA269_10==WINAPI) ) {
alt269=1;
}
}
break;
case OVERRIDE:
{
int LA269_11 = input.LA(3);
if ( (LA269_11==ABSTRACT||LA269_11==ASSEMBLER||LA269_11==CDECL||LA269_11==DEPRECATED||LA269_11==DISPID||LA269_11==DYNAMIC||(LA269_11 >= EXPERIMENTAL && LA269_11 <= EXPORT)||LA269_11==EXTERNAL||LA269_11==FAR||LA269_11==FINAL||LA269_11==FORWARD||LA269_11==INLINE||(LA269_11 >= LIBRARY && LA269_11 <= LOCAL)||LA269_11==MESSAGE||LA269_11==NEAR||(LA269_11 >= OVERLOAD && LA269_11 <= OVERRIDE)||(LA269_11 >= PASCAL && LA269_11 <= PLATFORM)||(LA269_11 >= REGISTER && LA269_11 <= REINTRODUCE)||LA269_11==SAFECALL||LA269_11==SEMICOLON||(LA269_11 >= STATIC && LA269_11 <= STDCALL)||LA269_11==UNSAFE||(LA269_11 >= VARARGS && LA269_11 <= VIRTUAL)||LA269_11==WINAPI) ) {
alt269=1;
}
}
break;
case VIRTUAL:
{
int LA269_12 = input.LA(3);
if ( (LA269_12==ABSTRACT||LA269_12==ASSEMBLER||LA269_12==CDECL||LA269_12==DEPRECATED||LA269_12==DISPID||LA269_12==DYNAMIC||(LA269_12 >= EXPERIMENTAL && LA269_12 <= EXPORT)||LA269_12==EXTERNAL||LA269_12==FAR||LA269_12==FINAL||LA269_12==FORWARD||LA269_12==INLINE||(LA269_12 >= LIBRARY && LA269_12 <= LOCAL)||LA269_12==MESSAGE||LA269_12==NEAR||(LA269_12 >= OVERLOAD && LA269_12 <= OVERRIDE)||(LA269_12 >= PASCAL && LA269_12 <= PLATFORM)||(LA269_12 >= REGISTER && LA269_12 <= REINTRODUCE)||LA269_12==SAFECALL||LA269_12==SEMICOLON||(LA269_12 >= STATIC && LA269_12 <= STDCALL)||LA269_12==UNSAFE||(LA269_12 >= VARARGS && LA269_12 <= VIRTUAL)||LA269_12==WINAPI) ) {
alt269=1;
}
}
break;
case ABSTRACT:
case FINAL:
{
int LA269_13 = input.LA(3);
if ( (LA269_13==ABSTRACT||LA269_13==ASSEMBLER||LA269_13==CDECL||LA269_13==DEPRECATED||LA269_13==DISPID||LA269_13==DYNAMIC||(LA269_13 >= EXPERIMENTAL && LA269_13 <= EXPORT)||LA269_13==EXTERNAL||LA269_13==FAR||LA269_13==FINAL||LA269_13==FORWARD||LA269_13==INLINE||(LA269_13 >= LIBRARY && LA269_13 <= LOCAL)||LA269_13==MESSAGE||LA269_13==NEAR||(LA269_13 >= OVERLOAD && LA269_13 <= OVERRIDE)||(LA269_13 >= PASCAL && LA269_13 <= PLATFORM)||(LA269_13 >= REGISTER && LA269_13 <= REINTRODUCE)||LA269_13==SAFECALL||LA269_13==SEMICOLON||(LA269_13 >= STATIC && LA269_13 <= STDCALL)||LA269_13==UNSAFE||(LA269_13 >= VARARGS && LA269_13 <= VIRTUAL)||LA269_13==WINAPI) ) {
alt269=1;
}
}
break;
case ASSEMBLER:
{
int LA269_14 = input.LA(3);
if ( (LA269_14==ABSTRACT||LA269_14==ASSEMBLER||LA269_14==CDECL||LA269_14==DEPRECATED||LA269_14==DISPID||LA269_14==DYNAMIC||(LA269_14 >= EXPERIMENTAL && LA269_14 <= EXPORT)||LA269_14==EXTERNAL||LA269_14==FAR||LA269_14==FINAL||LA269_14==FORWARD||LA269_14==INLINE||(LA269_14 >= LIBRARY && LA269_14 <= LOCAL)||LA269_14==MESSAGE||LA269_14==NEAR||(LA269_14 >= OVERLOAD && LA269_14 <= OVERRIDE)||(LA269_14 >= PASCAL && LA269_14 <= PLATFORM)||(LA269_14 >= REGISTER && LA269_14 <= REINTRODUCE)||LA269_14==SAFECALL||LA269_14==SEMICOLON||(LA269_14 >= STATIC && LA269_14 <= STDCALL)||LA269_14==UNSAFE||(LA269_14 >= VARARGS && LA269_14 <= VIRTUAL)||LA269_14==WINAPI) ) {
alt269=1;
}
}
break;
case CDECL:
case EXPORT:
case PASCAL:
case REGISTER:
case SAFECALL:
case STDCALL:
case WINAPI:
{
int LA269_15 = input.LA(3);
if ( (LA269_15==ABSTRACT||LA269_15==ASSEMBLER||LA269_15==CDECL||LA269_15==DEPRECATED||LA269_15==DISPID||LA269_15==DYNAMIC||(LA269_15 >= EXPERIMENTAL && LA269_15 <= EXPORT)||LA269_15==EXTERNAL||LA269_15==FAR||LA269_15==FINAL||LA269_15==FORWARD||LA269_15==INLINE||(LA269_15 >= LIBRARY && LA269_15 <= LOCAL)||LA269_15==MESSAGE||LA269_15==NEAR||(LA269_15 >= OVERLOAD && LA269_15 <= OVERRIDE)||(LA269_15 >= PASCAL && LA269_15 <= PLATFORM)||(LA269_15 >= REGISTER && LA269_15 <= REINTRODUCE)||LA269_15==SAFECALL||LA269_15==SEMICOLON||(LA269_15 >= STATIC && LA269_15 <= STDCALL)||LA269_15==UNSAFE||(LA269_15 >= VARARGS && LA269_15 <= VIRTUAL)||LA269_15==WINAPI) ) {
alt269=1;
}
}
break;
case INLINE:
case LIBRARY:
{
alt269=1;
}
break;
case DEPRECATED:
{
int LA269_16 = input.LA(3);
if ( (LA269_16==ABSTRACT||LA269_16==ASSEMBLER||LA269_16==CDECL||(LA269_16 >= DEPRECATED && LA269_16 <= DEREFERENCE)||LA269_16==DISPID||LA269_16==DYNAMIC||(LA269_16 >= EXPERIMENTAL && LA269_16 <= EXPORT)||LA269_16==EXTERNAL||LA269_16==FAR||LA269_16==FINAL||LA269_16==FORWARD||LA269_16==INLINE||(LA269_16 >= LIBRARY && LA269_16 <= LOCAL)||LA269_16==MESSAGE||LA269_16==NEAR||(LA269_16 >= OVERLOAD && LA269_16 <= OVERRIDE)||(LA269_16 >= PASCAL && LA269_16 <= PLATFORM)||(LA269_16 >= REGISTER && LA269_16 <= REINTRODUCE)||LA269_16==SAFECALL||LA269_16==SEMICOLON||(LA269_16 >= STATIC && LA269_16 <= STDCALL)||LA269_16==TkCharacterEscapeCode||LA269_16==TkMultilineString||LA269_16==TkQuotedString||LA269_16==UNSAFE||(LA269_16 >= VARARGS && LA269_16 <= VIRTUAL)||LA269_16==WINAPI) ) {
alt269=1;
}
}
break;
case EXPERIMENTAL:
{
int LA269_17 = input.LA(3);
if ( (LA269_17==ABSTRACT||LA269_17==ASSEMBLER||LA269_17==CDECL||LA269_17==DEPRECATED||LA269_17==DISPID||LA269_17==DYNAMIC||(LA269_17 >= EXPERIMENTAL && LA269_17 <= EXPORT)||LA269_17==EXTERNAL||LA269_17==FAR||LA269_17==FINAL||LA269_17==FORWARD||LA269_17==INLINE||(LA269_17 >= LIBRARY && LA269_17 <= LOCAL)||LA269_17==MESSAGE||LA269_17==NEAR||(LA269_17 >= OVERLOAD && LA269_17 <= OVERRIDE)||(LA269_17 >= PASCAL && LA269_17 <= PLATFORM)||(LA269_17 >= REGISTER && LA269_17 <= REINTRODUCE)||LA269_17==SAFECALL||LA269_17==SEMICOLON||(LA269_17 >= STATIC && LA269_17 <= STDCALL)||LA269_17==UNSAFE||(LA269_17 >= VARARGS && LA269_17 <= VIRTUAL)||LA269_17==WINAPI) ) {
alt269=1;
}
}
break;
case PLATFORM:
{
int LA269_18 = input.LA(3);
if ( (LA269_18==ABSTRACT||LA269_18==ASSEMBLER||LA269_18==CDECL||LA269_18==DEPRECATED||LA269_18==DISPID||LA269_18==DYNAMIC||(LA269_18 >= EXPERIMENTAL && LA269_18 <= EXPORT)||LA269_18==EXTERNAL||LA269_18==FAR||LA269_18==FINAL||LA269_18==FORWARD||LA269_18==INLINE||(LA269_18 >= LIBRARY && LA269_18 <= LOCAL)||LA269_18==MESSAGE||LA269_18==NEAR||(LA269_18 >= OVERLOAD && LA269_18 <= OVERRIDE)||(LA269_18 >= PASCAL && LA269_18 <= PLATFORM)||(LA269_18 >= REGISTER && LA269_18 <= REINTRODUCE)||LA269_18==SAFECALL||LA269_18==SEMICOLON||(LA269_18 >= STATIC && LA269_18 <= STDCALL)||LA269_18==UNSAFE||(LA269_18 >= VARARGS && LA269_18 <= VIRTUAL)||LA269_18==WINAPI) ) {
alt269=1;
}
}
break;
case FAR:
case LOCAL:
case NEAR:
{
int LA269_19 = input.LA(3);
if ( (LA269_19==ABSTRACT||LA269_19==ASSEMBLER||LA269_19==CDECL||LA269_19==DEPRECATED||LA269_19==DISPID||LA269_19==DYNAMIC||(LA269_19 >= EXPERIMENTAL && LA269_19 <= EXPORT)||LA269_19==EXTERNAL||LA269_19==FAR||LA269_19==FINAL||LA269_19==FORWARD||LA269_19==INLINE||(LA269_19 >= LIBRARY && LA269_19 <= LOCAL)||LA269_19==MESSAGE||LA269_19==NEAR||(LA269_19 >= OVERLOAD && LA269_19 <= OVERRIDE)||(LA269_19 >= PASCAL && LA269_19 <= PLATFORM)||(LA269_19 >= REGISTER && LA269_19 <= REINTRODUCE)||LA269_19==SAFECALL||LA269_19==SEMICOLON||(LA269_19 >= STATIC && LA269_19 <= STDCALL)||LA269_19==UNSAFE||(LA269_19 >= VARARGS && LA269_19 <= VIRTUAL)||LA269_19==WINAPI) ) {
alt269=1;
}
}
break;
case DISPID:
{
int LA269_20 = input.LA(3);
if ( ((LA269_20 >= ABSOLUTE && LA269_20 <= ALIGN)||(LA269_20 >= ASSEMBLER && LA269_20 <= ASSEMBLY)||(LA269_20 >= AT && LA269_20 <= AUTOMATED)||LA269_20==CDECL||LA269_20==CONTAINS||(LA269_20 >= DEFAULT && LA269_20 <= DEREFERENCE)||LA269_20==DISPID||LA269_20==DYNAMIC||(LA269_20 >= EXPERIMENTAL && LA269_20 <= EXPORT)||LA269_20==EXTERNAL||(LA269_20 >= FAR && LA269_20 <= FINAL)||(LA269_20 >= FORWARD && LA269_20 <= FUNCTION)||LA269_20==HELPER||LA269_20==IMPLEMENTS||(LA269_20 >= INDEX && LA269_20 <= INHERITED)||LA269_20==LABEL||LA269_20==LOCAL||(LA269_20 >= MESSAGE && LA269_20 <= MINUS)||(LA269_20 >= NAME && LA269_20 <= NOT)||(LA269_20 >= ON && LA269_20 <= OPERATOR)||(LA269_20 >= OUT && LA269_20 <= OVERRIDE)||LA269_20==PACKAGE||LA269_20==PAREN_BRACKET_LEFT||LA269_20==PAREN_LEFT||(LA269_20 >= PASCAL && LA269_20 <= PROCEDURE)||(LA269_20 >= PROTECTED && LA269_20 <= PUBLISHED)||(LA269_20 >= READ && LA269_20 <= READONLY)||(LA269_20 >= REFERENCE && LA269_20 <= REINTRODUCE)||(LA269_20 >= REQUIRES && LA269_20 <= RESIDENT)||(LA269_20 >= SAFECALL && LA269_20 <= SEALED)||LA269_20==SQUARE_BRACKET_LEFT||(LA269_20 >= STATIC && LA269_20 <= STRING)||LA269_20==TkBinaryNumber||LA269_20==TkCharacterEscapeCode||(LA269_20 >= TkHexNumber && LA269_20 <= TkIntNumber)||LA269_20==TkMultilineString||(LA269_20 >= TkQuotedString && LA269_20 <= TkRealNumber)||LA269_20==UNSAFE||(LA269_20 >= VARARGS && LA269_20 <= VIRTUAL)||LA269_20==WINAPI||(LA269_20 >= WRITE && LA269_20 <= WRITEONLY)) ) {
alt269=1;
}
}
break;
case VARARGS:
{
int LA269_21 = input.LA(3);
if ( (LA269_21==ABSTRACT||LA269_21==ASSEMBLER||LA269_21==CDECL||LA269_21==DEPRECATED||LA269_21==DISPID||LA269_21==DYNAMIC||(LA269_21 >= EXPERIMENTAL && LA269_21 <= EXPORT)||LA269_21==EXTERNAL||LA269_21==FAR||LA269_21==FINAL||LA269_21==FORWARD||LA269_21==INLINE||(LA269_21 >= LIBRARY && LA269_21 <= LOCAL)||LA269_21==MESSAGE||LA269_21==NEAR||(LA269_21 >= OVERLOAD && LA269_21 <= OVERRIDE)||(LA269_21 >= PASCAL && LA269_21 <= PLATFORM)||(LA269_21 >= REGISTER && LA269_21 <= REINTRODUCE)||LA269_21==SAFECALL||LA269_21==SEMICOLON||(LA269_21 >= STATIC && LA269_21 <= STDCALL)||LA269_21==UNSAFE||(LA269_21 >= VARARGS && LA269_21 <= VIRTUAL)||LA269_21==WINAPI) ) {
alt269=1;
}
}
break;
case UNSAFE:
{
int LA269_22 = input.LA(3);
if ( (LA269_22==ABSTRACT||LA269_22==ASSEMBLER||LA269_22==CDECL||LA269_22==DEPRECATED||LA269_22==DISPID||LA269_22==DYNAMIC||(LA269_22 >= EXPERIMENTAL && LA269_22 <= EXPORT)||LA269_22==EXTERNAL||LA269_22==FAR||LA269_22==FINAL||LA269_22==FORWARD||LA269_22==INLINE||(LA269_22 >= LIBRARY && LA269_22 <= LOCAL)||LA269_22==MESSAGE||LA269_22==NEAR||(LA269_22 >= OVERLOAD && LA269_22 <= OVERRIDE)||(LA269_22 >= PASCAL && LA269_22 <= PLATFORM)||(LA269_22 >= REGISTER && LA269_22 <= REINTRODUCE)||LA269_22==SAFECALL||LA269_22==SEMICOLON||(LA269_22 >= STATIC && LA269_22 <= STDCALL)||LA269_22==UNSAFE||(LA269_22 >= VARARGS && LA269_22 <= VIRTUAL)||LA269_22==WINAPI) ) {
alt269=1;
}
}
break;
}
}
else if ( (LA269_0==ABSTRACT||LA269_0==ASSEMBLER||LA269_0==CDECL||LA269_0==DEPRECATED||LA269_0==DISPID||LA269_0==DYNAMIC||(LA269_0 >= EXPERIMENTAL && LA269_0 <= EXPORT)||LA269_0==EXTERNAL||LA269_0==FAR||LA269_0==FINAL||LA269_0==FORWARD||LA269_0==INLINE||(LA269_0 >= LIBRARY && LA269_0 <= LOCAL)||LA269_0==MESSAGE||LA269_0==NEAR||(LA269_0 >= OVERLOAD && LA269_0 <= OVERRIDE)||(LA269_0 >= PASCAL && LA269_0 <= PLATFORM)||(LA269_0 >= REGISTER && LA269_0 <= REINTRODUCE)||LA269_0==SAFECALL||(LA269_0 >= STATIC && LA269_0 <= STDCALL)||LA269_0==UNSAFE||(LA269_0 >= VARARGS && LA269_0 <= VIRTUAL)||LA269_0==WINAPI) ) {
alt269=1;
}
switch (alt269) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1072:33: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1072:33: ( ';' )?
int alt268=2;
int LA268_0 = input.LA(1);
if ( (LA268_0==SEMICOLON) ) {
alt268=1;
}
switch (alt268) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1072:33: ';'
{
char_literal796=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_interfaceDirectiveSection21724); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal796_tree = (Object)adaptor.create(char_literal796);
adaptor.addChild(root_0, char_literal796_tree);
}
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_interfaceDirectiveSection21727);
interfaceDirective797=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceDirective797.getTree());
}
break;
default :
break loop269;
}
}
char_literal798=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_interfaceDirectiveSection21731); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal798_tree = (Object)adaptor.create(char_literal798);
adaptor.addChild(root_0, char_literal798_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1073:32: ( ';' interfaceDirective )+
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1073:32: ( ';' interfaceDirective )+
int cnt270=0;
loop270:
while (true) {
int alt270=2;
int LA270_0 = input.LA(1);
if ( (LA270_0==SEMICOLON) ) {
alt270=1;
}
switch (alt270) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1073:33: ';' interfaceDirective
{
char_literal799=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_interfaceDirectiveSection21765); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal799_tree = (Object)adaptor.create(char_literal799);
adaptor.addChild(root_0, char_literal799_tree);
}
pushFollow(FOLLOW_interfaceDirective_in_interfaceDirectiveSection21767);
interfaceDirective800=interfaceDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, interfaceDirective800.getTree());
}
break;
default :
if ( cnt270 >= 1 ) break loop270;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee = new EarlyExitException(270, input);
throw eee;
}
cnt270++;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 200, interfaceDirectiveSection_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceDirectiveSection"
public static class externalDirectiveSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "externalDirectiveSection"
// au/com/integradev/delphi/antlr/Delphi.g:1075:1: externalDirectiveSection : ( ( ( ';' )? implDirective )* ( ';' )? externalDirective ( ( ';' )? implDirective )* ';' | ( ';' implDirective )* ';' externalDirective ( ';' implDirective )* );
public final DelphiParser.externalDirectiveSection_return externalDirectiveSection() throws RecognitionException {
DelphiParser.externalDirectiveSection_return retval = new DelphiParser.externalDirectiveSection_return();
retval.start = input.LT(1);
int externalDirectiveSection_StartIndex = input.index();
Object root_0 = null;
Token char_literal801=null;
Token char_literal803=null;
Token char_literal805=null;
Token char_literal807=null;
Token char_literal808=null;
Token char_literal810=null;
Token char_literal812=null;
ParserRuleReturnScope implDirective802 =null;
ParserRuleReturnScope externalDirective804 =null;
ParserRuleReturnScope implDirective806 =null;
ParserRuleReturnScope implDirective809 =null;
ParserRuleReturnScope externalDirective811 =null;
ParserRuleReturnScope implDirective813 =null;
Object char_literal801_tree=null;
Object char_literal803_tree=null;
Object char_literal805_tree=null;
Object char_literal807_tree=null;
Object char_literal808_tree=null;
Object char_literal810_tree=null;
Object char_literal812_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 201) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1075:31: ( ( ( ';' )? implDirective )* ( ';' )? externalDirective ( ( ';' )? implDirective )* ';' | ( ';' implDirective )* ';' externalDirective ( ';' implDirective )* )
int alt279=2;
int LA279_0 = input.LA(1);
if ( (LA279_0==SEMICOLON) ) {
int LA279_1 = input.LA(2);
if ( (synpred394_Delphi()) ) {
alt279=1;
}
else if ( (true) ) {
alt279=2;
}
}
else if ( (LA279_0==ABSTRACT||LA279_0==ASSEMBLER||LA279_0==CDECL||LA279_0==DEPRECATED||LA279_0==DISPID||LA279_0==DYNAMIC||(LA279_0 >= EXPERIMENTAL && LA279_0 <= EXPORT)||LA279_0==EXTERNAL||LA279_0==FAR||LA279_0==FINAL||LA279_0==INLINE||(LA279_0 >= LIBRARY && LA279_0 <= LOCAL)||LA279_0==MESSAGE||LA279_0==NEAR||(LA279_0 >= OVERLOAD && LA279_0 <= OVERRIDE)||(LA279_0 >= PASCAL && LA279_0 <= PLATFORM)||(LA279_0 >= REGISTER && LA279_0 <= REINTRODUCE)||LA279_0==SAFECALL||(LA279_0 >= STATIC && LA279_0 <= STDCALL)||LA279_0==UNSAFE||(LA279_0 >= VARARGS && LA279_0 <= VIRTUAL)||LA279_0==WINAPI) ) {
alt279=1;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 279, 0, input);
throw nvae;
}
switch (alt279) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:33: ( ( ';' )? implDirective )* ( ';' )? externalDirective ( ( ';' )? implDirective )* ';'
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1075:33: ( ( ';' )? implDirective )*
loop273:
while (true) {
int alt273=2;
int LA273_0 = input.LA(1);
if ( (LA273_0==SEMICOLON) ) {
int LA273_1 = input.LA(2);
if ( (LA273_1==ABSTRACT||LA273_1==ASSEMBLER||LA273_1==CDECL||LA273_1==DEPRECATED||LA273_1==DISPID||LA273_1==DYNAMIC||(LA273_1 >= EXPERIMENTAL && LA273_1 <= EXPORT)||LA273_1==FAR||LA273_1==FINAL||LA273_1==INLINE||(LA273_1 >= LIBRARY && LA273_1 <= LOCAL)||LA273_1==MESSAGE||LA273_1==NEAR||(LA273_1 >= OVERLOAD && LA273_1 <= OVERRIDE)||(LA273_1 >= PASCAL && LA273_1 <= PLATFORM)||(LA273_1 >= REGISTER && LA273_1 <= REINTRODUCE)||LA273_1==SAFECALL||(LA273_1 >= STATIC && LA273_1 <= STDCALL)||LA273_1==UNSAFE||(LA273_1 >= VARARGS && LA273_1 <= VIRTUAL)||LA273_1==WINAPI) ) {
alt273=1;
}
}
else if ( (LA273_0==ABSTRACT||LA273_0==ASSEMBLER||LA273_0==CDECL||LA273_0==DEPRECATED||LA273_0==DISPID||LA273_0==DYNAMIC||(LA273_0 >= EXPERIMENTAL && LA273_0 <= EXPORT)||LA273_0==FAR||LA273_0==FINAL||LA273_0==INLINE||(LA273_0 >= LIBRARY && LA273_0 <= LOCAL)||LA273_0==MESSAGE||LA273_0==NEAR||(LA273_0 >= OVERLOAD && LA273_0 <= OVERRIDE)||(LA273_0 >= PASCAL && LA273_0 <= PLATFORM)||(LA273_0 >= REGISTER && LA273_0 <= REINTRODUCE)||LA273_0==SAFECALL||(LA273_0 >= STATIC && LA273_0 <= STDCALL)||LA273_0==UNSAFE||(LA273_0 >= VARARGS && LA273_0 <= VIRTUAL)||LA273_0==WINAPI) ) {
alt273=1;
}
switch (alt273) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:34: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1075:34: ( ';' )?
int alt272=2;
int LA272_0 = input.LA(1);
if ( (LA272_0==SEMICOLON) ) {
alt272=1;
}
switch (alt272) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:34: ';'
{
char_literal801=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21812); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal801_tree = (Object)adaptor.create(char_literal801);
adaptor.addChild(root_0, char_literal801_tree);
}
}
break;
}
pushFollow(FOLLOW_implDirective_in_externalDirectiveSection21815);
implDirective802=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective802.getTree());
}
break;
default :
break loop273;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:1075:55: ( ';' )?
int alt274=2;
int LA274_0 = input.LA(1);
if ( (LA274_0==SEMICOLON) ) {
alt274=1;
}
switch (alt274) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:55: ';'
{
char_literal803=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21819); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal803_tree = (Object)adaptor.create(char_literal803);
adaptor.addChild(root_0, char_literal803_tree);
}
}
break;
}
pushFollow(FOLLOW_externalDirective_in_externalDirectiveSection21822);
externalDirective804=externalDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, externalDirective804.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1075:78: ( ( ';' )? implDirective )*
loop276:
while (true) {
int alt276=2;
int LA276_0 = input.LA(1);
if ( (LA276_0==SEMICOLON) ) {
int LA276_1 = input.LA(2);
if ( (LA276_1==ABSTRACT||LA276_1==ASSEMBLER||LA276_1==CDECL||LA276_1==DEPRECATED||LA276_1==DISPID||LA276_1==DYNAMIC||(LA276_1 >= EXPERIMENTAL && LA276_1 <= EXPORT)||LA276_1==FAR||LA276_1==FINAL||LA276_1==INLINE||(LA276_1 >= LIBRARY && LA276_1 <= LOCAL)||LA276_1==MESSAGE||LA276_1==NEAR||(LA276_1 >= OVERLOAD && LA276_1 <= OVERRIDE)||(LA276_1 >= PASCAL && LA276_1 <= PLATFORM)||(LA276_1 >= REGISTER && LA276_1 <= REINTRODUCE)||LA276_1==SAFECALL||(LA276_1 >= STATIC && LA276_1 <= STDCALL)||LA276_1==UNSAFE||(LA276_1 >= VARARGS && LA276_1 <= VIRTUAL)||LA276_1==WINAPI) ) {
alt276=1;
}
}
else if ( (LA276_0==ABSTRACT||LA276_0==ASSEMBLER||LA276_0==CDECL||LA276_0==DEPRECATED||LA276_0==DISPID||LA276_0==DYNAMIC||(LA276_0 >= EXPERIMENTAL && LA276_0 <= EXPORT)||LA276_0==FAR||LA276_0==FINAL||LA276_0==INLINE||(LA276_0 >= LIBRARY && LA276_0 <= LOCAL)||LA276_0==MESSAGE||LA276_0==NEAR||(LA276_0 >= OVERLOAD && LA276_0 <= OVERRIDE)||(LA276_0 >= PASCAL && LA276_0 <= PLATFORM)||(LA276_0 >= REGISTER && LA276_0 <= REINTRODUCE)||LA276_0==SAFECALL||(LA276_0 >= STATIC && LA276_0 <= STDCALL)||LA276_0==UNSAFE||(LA276_0 >= VARARGS && LA276_0 <= VIRTUAL)||LA276_0==WINAPI) ) {
alt276=1;
}
switch (alt276) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:79: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1075:79: ( ';' )?
int alt275=2;
int LA275_0 = input.LA(1);
if ( (LA275_0==SEMICOLON) ) {
alt275=1;
}
switch (alt275) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:79: ';'
{
char_literal805=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21825); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal805_tree = (Object)adaptor.create(char_literal805);
adaptor.addChild(root_0, char_literal805_tree);
}
}
break;
}
pushFollow(FOLLOW_implDirective_in_externalDirectiveSection21828);
implDirective806=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective806.getTree());
}
break;
default :
break loop276;
}
}
char_literal807=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21831); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal807_tree = (Object)adaptor.create(char_literal807);
adaptor.addChild(root_0, char_literal807_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1076:32: ( ';' implDirective )* ';' externalDirective ( ';' implDirective )*
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1076:32: ( ';' implDirective )*
loop277:
while (true) {
int alt277=2;
int LA277_0 = input.LA(1);
if ( (LA277_0==SEMICOLON) ) {
int LA277_1 = input.LA(2);
if ( (LA277_1==ABSTRACT||LA277_1==ASSEMBLER||LA277_1==CDECL||LA277_1==DEPRECATED||LA277_1==DISPID||LA277_1==DYNAMIC||(LA277_1 >= EXPERIMENTAL && LA277_1 <= EXPORT)||LA277_1==FAR||LA277_1==FINAL||LA277_1==INLINE||(LA277_1 >= LIBRARY && LA277_1 <= LOCAL)||LA277_1==MESSAGE||LA277_1==NEAR||(LA277_1 >= OVERLOAD && LA277_1 <= OVERRIDE)||(LA277_1 >= PASCAL && LA277_1 <= PLATFORM)||(LA277_1 >= REGISTER && LA277_1 <= REINTRODUCE)||LA277_1==SAFECALL||(LA277_1 >= STATIC && LA277_1 <= STDCALL)||LA277_1==UNSAFE||(LA277_1 >= VARARGS && LA277_1 <= VIRTUAL)||LA277_1==WINAPI) ) {
alt277=1;
}
}
switch (alt277) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1076:33: ';' implDirective
{
char_literal808=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21865); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal808_tree = (Object)adaptor.create(char_literal808);
adaptor.addChild(root_0, char_literal808_tree);
}
pushFollow(FOLLOW_implDirective_in_externalDirectiveSection21867);
implDirective809=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective809.getTree());
}
break;
default :
break loop277;
}
}
char_literal810=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21871); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal810_tree = (Object)adaptor.create(char_literal810);
adaptor.addChild(root_0, char_literal810_tree);
}
pushFollow(FOLLOW_externalDirective_in_externalDirectiveSection21873);
externalDirective811=externalDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, externalDirective811.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1076:75: ( ';' implDirective )*
loop278:
while (true) {
int alt278=2;
int LA278_0 = input.LA(1);
if ( (LA278_0==SEMICOLON) ) {
alt278=1;
}
switch (alt278) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1076:76: ';' implDirective
{
char_literal812=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_externalDirectiveSection21876); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal812_tree = (Object)adaptor.create(char_literal812);
adaptor.addChild(root_0, char_literal812_tree);
}
pushFollow(FOLLOW_implDirective_in_externalDirectiveSection21878);
implDirective813=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective813.getTree());
}
break;
default :
break loop278;
}
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 201, externalDirectiveSection_StartIndex); }
}
return retval;
}
// $ANTLR end "externalDirectiveSection"
public static class forwardDirectiveSection_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "forwardDirectiveSection"
// au/com/integradev/delphi/antlr/Delphi.g:1078:1: forwardDirectiveSection : ( ( ( ';' )? implDirective )* ( ';' )? FORWARD ( ( ';' )? implDirective )* ';' | ( ';' implDirective )* ';' FORWARD ( ';' implDirective )* );
public final DelphiParser.forwardDirectiveSection_return forwardDirectiveSection() throws RecognitionException {
DelphiParser.forwardDirectiveSection_return retval = new DelphiParser.forwardDirectiveSection_return();
retval.start = input.LT(1);
int forwardDirectiveSection_StartIndex = input.index();
Object root_0 = null;
Token char_literal814=null;
Token char_literal816=null;
Token FORWARD817=null;
Token char_literal818=null;
Token char_literal820=null;
Token char_literal821=null;
Token char_literal823=null;
Token FORWARD824=null;
Token char_literal825=null;
ParserRuleReturnScope implDirective815 =null;
ParserRuleReturnScope implDirective819 =null;
ParserRuleReturnScope implDirective822 =null;
ParserRuleReturnScope implDirective826 =null;
Object char_literal814_tree=null;
Object char_literal816_tree=null;
Object FORWARD817_tree=null;
Object char_literal818_tree=null;
Object char_literal820_tree=null;
Object char_literal821_tree=null;
Object char_literal823_tree=null;
Object FORWARD824_tree=null;
Object char_literal825_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 202) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1078:30: ( ( ( ';' )? implDirective )* ( ';' )? FORWARD ( ( ';' )? implDirective )* ';' | ( ';' implDirective )* ';' FORWARD ( ';' implDirective )* )
int alt287=2;
int LA287_0 = input.LA(1);
if ( (LA287_0==SEMICOLON) ) {
int LA287_1 = input.LA(2);
if ( (synpred402_Delphi()) ) {
alt287=1;
}
else if ( (true) ) {
alt287=2;
}
}
else if ( (LA287_0==ABSTRACT||LA287_0==ASSEMBLER||LA287_0==CDECL||LA287_0==DEPRECATED||LA287_0==DISPID||LA287_0==DYNAMIC||(LA287_0 >= EXPERIMENTAL && LA287_0 <= EXPORT)||LA287_0==FAR||LA287_0==FINAL||LA287_0==FORWARD||LA287_0==INLINE||(LA287_0 >= LIBRARY && LA287_0 <= LOCAL)||LA287_0==MESSAGE||LA287_0==NEAR||(LA287_0 >= OVERLOAD && LA287_0 <= OVERRIDE)||(LA287_0 >= PASCAL && LA287_0 <= PLATFORM)||(LA287_0 >= REGISTER && LA287_0 <= REINTRODUCE)||LA287_0==SAFECALL||(LA287_0 >= STATIC && LA287_0 <= STDCALL)||LA287_0==UNSAFE||(LA287_0 >= VARARGS && LA287_0 <= VIRTUAL)||LA287_0==WINAPI) ) {
alt287=1;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 287, 0, input);
throw nvae;
}
switch (alt287) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:32: ( ( ';' )? implDirective )* ( ';' )? FORWARD ( ( ';' )? implDirective )* ';'
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1078:32: ( ( ';' )? implDirective )*
loop281:
while (true) {
int alt281=2;
int LA281_0 = input.LA(1);
if ( (LA281_0==SEMICOLON) ) {
int LA281_1 = input.LA(2);
if ( (LA281_1==ABSTRACT||LA281_1==ASSEMBLER||LA281_1==CDECL||LA281_1==DEPRECATED||LA281_1==DISPID||LA281_1==DYNAMIC||(LA281_1 >= EXPERIMENTAL && LA281_1 <= EXPORT)||LA281_1==FAR||LA281_1==FINAL||LA281_1==INLINE||(LA281_1 >= LIBRARY && LA281_1 <= LOCAL)||LA281_1==MESSAGE||LA281_1==NEAR||(LA281_1 >= OVERLOAD && LA281_1 <= OVERRIDE)||(LA281_1 >= PASCAL && LA281_1 <= PLATFORM)||(LA281_1 >= REGISTER && LA281_1 <= REINTRODUCE)||LA281_1==SAFECALL||(LA281_1 >= STATIC && LA281_1 <= STDCALL)||LA281_1==UNSAFE||(LA281_1 >= VARARGS && LA281_1 <= VIRTUAL)||LA281_1==WINAPI) ) {
alt281=1;
}
}
else if ( (LA281_0==ABSTRACT||LA281_0==ASSEMBLER||LA281_0==CDECL||LA281_0==DEPRECATED||LA281_0==DISPID||LA281_0==DYNAMIC||(LA281_0 >= EXPERIMENTAL && LA281_0 <= EXPORT)||LA281_0==FAR||LA281_0==FINAL||LA281_0==INLINE||(LA281_0 >= LIBRARY && LA281_0 <= LOCAL)||LA281_0==MESSAGE||LA281_0==NEAR||(LA281_0 >= OVERLOAD && LA281_0 <= OVERRIDE)||(LA281_0 >= PASCAL && LA281_0 <= PLATFORM)||(LA281_0 >= REGISTER && LA281_0 <= REINTRODUCE)||LA281_0==SAFECALL||(LA281_0 >= STATIC && LA281_0 <= STDCALL)||LA281_0==UNSAFE||(LA281_0 >= VARARGS && LA281_0 <= VIRTUAL)||LA281_0==WINAPI) ) {
alt281=1;
}
switch (alt281) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:33: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1078:33: ( ';' )?
int alt280=2;
int LA280_0 = input.LA(1);
if ( (LA280_0==SEMICOLON) ) {
alt280=1;
}
switch (alt280) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:33: ';'
{
char_literal814=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21923); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal814_tree = (Object)adaptor.create(char_literal814);
adaptor.addChild(root_0, char_literal814_tree);
}
}
break;
}
pushFollow(FOLLOW_implDirective_in_forwardDirectiveSection21926);
implDirective815=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective815.getTree());
}
break;
default :
break loop281;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:1078:54: ( ';' )?
int alt282=2;
int LA282_0 = input.LA(1);
if ( (LA282_0==SEMICOLON) ) {
alt282=1;
}
switch (alt282) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:54: ';'
{
char_literal816=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21930); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal816_tree = (Object)adaptor.create(char_literal816);
adaptor.addChild(root_0, char_literal816_tree);
}
}
break;
}
FORWARD817=(Token)match(input,FORWARD,FOLLOW_FORWARD_in_forwardDirectiveSection21933); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FORWARD817_tree = (Object)adaptor.create(FORWARD817);
adaptor.addChild(root_0, FORWARD817_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1078:67: ( ( ';' )? implDirective )*
loop284:
while (true) {
int alt284=2;
int LA284_0 = input.LA(1);
if ( (LA284_0==SEMICOLON) ) {
int LA284_1 = input.LA(2);
if ( (LA284_1==ABSTRACT||LA284_1==ASSEMBLER||LA284_1==CDECL||LA284_1==DEPRECATED||LA284_1==DISPID||LA284_1==DYNAMIC||(LA284_1 >= EXPERIMENTAL && LA284_1 <= EXPORT)||LA284_1==FAR||LA284_1==FINAL||LA284_1==INLINE||(LA284_1 >= LIBRARY && LA284_1 <= LOCAL)||LA284_1==MESSAGE||LA284_1==NEAR||(LA284_1 >= OVERLOAD && LA284_1 <= OVERRIDE)||(LA284_1 >= PASCAL && LA284_1 <= PLATFORM)||(LA284_1 >= REGISTER && LA284_1 <= REINTRODUCE)||LA284_1==SAFECALL||(LA284_1 >= STATIC && LA284_1 <= STDCALL)||LA284_1==UNSAFE||(LA284_1 >= VARARGS && LA284_1 <= VIRTUAL)||LA284_1==WINAPI) ) {
alt284=1;
}
}
else if ( (LA284_0==ABSTRACT||LA284_0==ASSEMBLER||LA284_0==CDECL||LA284_0==DEPRECATED||LA284_0==DISPID||LA284_0==DYNAMIC||(LA284_0 >= EXPERIMENTAL && LA284_0 <= EXPORT)||LA284_0==FAR||LA284_0==FINAL||LA284_0==INLINE||(LA284_0 >= LIBRARY && LA284_0 <= LOCAL)||LA284_0==MESSAGE||LA284_0==NEAR||(LA284_0 >= OVERLOAD && LA284_0 <= OVERRIDE)||(LA284_0 >= PASCAL && LA284_0 <= PLATFORM)||(LA284_0 >= REGISTER && LA284_0 <= REINTRODUCE)||LA284_0==SAFECALL||(LA284_0 >= STATIC && LA284_0 <= STDCALL)||LA284_0==UNSAFE||(LA284_0 >= VARARGS && LA284_0 <= VIRTUAL)||LA284_0==WINAPI) ) {
alt284=1;
}
switch (alt284) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:68: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1078:68: ( ';' )?
int alt283=2;
int LA283_0 = input.LA(1);
if ( (LA283_0==SEMICOLON) ) {
alt283=1;
}
switch (alt283) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:68: ';'
{
char_literal818=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21936); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal818_tree = (Object)adaptor.create(char_literal818);
adaptor.addChild(root_0, char_literal818_tree);
}
}
break;
}
pushFollow(FOLLOW_implDirective_in_forwardDirectiveSection21939);
implDirective819=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective819.getTree());
}
break;
default :
break loop284;
}
}
char_literal820=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21942); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal820_tree = (Object)adaptor.create(char_literal820);
adaptor.addChild(root_0, char_literal820_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1079:32: ( ';' implDirective )* ';' FORWARD ( ';' implDirective )*
{
root_0 = (Object)adaptor.nil();
// au/com/integradev/delphi/antlr/Delphi.g:1079:32: ( ';' implDirective )*
loop285:
while (true) {
int alt285=2;
int LA285_0 = input.LA(1);
if ( (LA285_0==SEMICOLON) ) {
int LA285_1 = input.LA(2);
if ( (LA285_1==ABSTRACT||LA285_1==ASSEMBLER||LA285_1==CDECL||LA285_1==DEPRECATED||LA285_1==DISPID||LA285_1==DYNAMIC||(LA285_1 >= EXPERIMENTAL && LA285_1 <= EXPORT)||LA285_1==FAR||LA285_1==FINAL||LA285_1==INLINE||(LA285_1 >= LIBRARY && LA285_1 <= LOCAL)||LA285_1==MESSAGE||LA285_1==NEAR||(LA285_1 >= OVERLOAD && LA285_1 <= OVERRIDE)||(LA285_1 >= PASCAL && LA285_1 <= PLATFORM)||(LA285_1 >= REGISTER && LA285_1 <= REINTRODUCE)||LA285_1==SAFECALL||(LA285_1 >= STATIC && LA285_1 <= STDCALL)||LA285_1==UNSAFE||(LA285_1 >= VARARGS && LA285_1 <= VIRTUAL)||LA285_1==WINAPI) ) {
alt285=1;
}
}
switch (alt285) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1079:33: ';' implDirective
{
char_literal821=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21976); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal821_tree = (Object)adaptor.create(char_literal821);
adaptor.addChild(root_0, char_literal821_tree);
}
pushFollow(FOLLOW_implDirective_in_forwardDirectiveSection21978);
implDirective822=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective822.getTree());
}
break;
default :
break loop285;
}
}
char_literal823=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21982); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal823_tree = (Object)adaptor.create(char_literal823);
adaptor.addChild(root_0, char_literal823_tree);
}
FORWARD824=(Token)match(input,FORWARD,FOLLOW_FORWARD_in_forwardDirectiveSection21984); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FORWARD824_tree = (Object)adaptor.create(FORWARD824);
adaptor.addChild(root_0, FORWARD824_tree);
}
// au/com/integradev/delphi/antlr/Delphi.g:1079:65: ( ';' implDirective )*
loop286:
while (true) {
int alt286=2;
int LA286_0 = input.LA(1);
if ( (LA286_0==SEMICOLON) ) {
alt286=1;
}
switch (alt286) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1079:66: ';' implDirective
{
char_literal825=(Token)match(input,SEMICOLON,FOLLOW_SEMICOLON_in_forwardDirectiveSection21987); if (state.failed) return retval;
if ( state.backtracking==0 ) {
char_literal825_tree = (Object)adaptor.create(char_literal825);
adaptor.addChild(root_0, char_literal825_tree);
}
pushFollow(FOLLOW_implDirective_in_forwardDirectiveSection21989);
implDirective826=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective826.getTree());
}
break;
default :
break loop286;
}
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 202, forwardDirectiveSection_StartIndex); }
}
return retval;
}
// $ANTLR end "forwardDirectiveSection"
public static class implDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "implDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1081:1: implDirective : ( OVERLOAD | REINTRODUCE | bindingDirective | abstractDirective | inlineDirective | callConvention | portabilityDirective | oldCallConventionDirective | dispIDDirective | VARARGS | UNSAFE );
public final DelphiParser.implDirective_return implDirective() throws RecognitionException {
DelphiParser.implDirective_return retval = new DelphiParser.implDirective_return();
retval.start = input.LT(1);
int implDirective_StartIndex = input.index();
Object root_0 = null;
Token OVERLOAD827=null;
Token REINTRODUCE828=null;
Token VARARGS836=null;
Token UNSAFE837=null;
ParserRuleReturnScope bindingDirective829 =null;
ParserRuleReturnScope abstractDirective830 =null;
ParserRuleReturnScope inlineDirective831 =null;
ParserRuleReturnScope callConvention832 =null;
ParserRuleReturnScope portabilityDirective833 =null;
ParserRuleReturnScope oldCallConventionDirective834 =null;
ParserRuleReturnScope dispIDDirective835 =null;
Object OVERLOAD827_tree=null;
Object REINTRODUCE828_tree=null;
Object VARARGS836_tree=null;
Object UNSAFE837_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 203) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1081:30: ( OVERLOAD | REINTRODUCE | bindingDirective | abstractDirective | inlineDirective | callConvention | portabilityDirective | oldCallConventionDirective | dispIDDirective | VARARGS | UNSAFE )
int alt288=11;
switch ( input.LA(1) ) {
case OVERLOAD:
{
alt288=1;
}
break;
case REINTRODUCE:
{
alt288=2;
}
break;
case DYNAMIC:
case MESSAGE:
case OVERRIDE:
case STATIC:
case VIRTUAL:
{
alt288=3;
}
break;
case ABSTRACT:
case FINAL:
{
alt288=4;
}
break;
case ASSEMBLER:
case INLINE:
{
alt288=5;
}
break;
case CDECL:
case EXPORT:
case PASCAL:
case REGISTER:
case SAFECALL:
case STDCALL:
case WINAPI:
{
alt288=6;
}
break;
case DEPRECATED:
case EXPERIMENTAL:
case LIBRARY:
case PLATFORM:
{
alt288=7;
}
break;
case FAR:
case LOCAL:
case NEAR:
{
alt288=8;
}
break;
case DISPID:
{
alt288=9;
}
break;
case VARARGS:
{
alt288=10;
}
break;
case UNSAFE:
{
alt288=11;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 288, 0, input);
throw nvae;
}
switch (alt288) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1081:32: OVERLOAD
{
root_0 = (Object)adaptor.nil();
OVERLOAD827=(Token)match(input,OVERLOAD,FOLLOW_OVERLOAD_in_implDirective22043); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OVERLOAD827_tree = (Object)adaptor.create(OVERLOAD827);
adaptor.addChild(root_0, OVERLOAD827_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1082:32: REINTRODUCE
{
root_0 = (Object)adaptor.nil();
REINTRODUCE828=(Token)match(input,REINTRODUCE,FOLLOW_REINTRODUCE_in_implDirective22076); if (state.failed) return retval;
if ( state.backtracking==0 ) {
REINTRODUCE828_tree = (Object)adaptor.create(REINTRODUCE828);
adaptor.addChild(root_0, REINTRODUCE828_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1083:32: bindingDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_bindingDirective_in_implDirective22109);
bindingDirective829=bindingDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, bindingDirective829.getTree());
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:1084:32: abstractDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_abstractDirective_in_implDirective22142);
abstractDirective830=abstractDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, abstractDirective830.getTree());
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:1085:32: inlineDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_inlineDirective_in_implDirective22175);
inlineDirective831=inlineDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, inlineDirective831.getTree());
}
break;
case 6 :
// au/com/integradev/delphi/antlr/Delphi.g:1086:32: callConvention
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_callConvention_in_implDirective22208);
callConvention832=callConvention();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, callConvention832.getTree());
}
break;
case 7 :
// au/com/integradev/delphi/antlr/Delphi.g:1087:32: portabilityDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_portabilityDirective_in_implDirective22241);
portabilityDirective833=portabilityDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, portabilityDirective833.getTree());
}
break;
case 8 :
// au/com/integradev/delphi/antlr/Delphi.g:1088:32: oldCallConventionDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_oldCallConventionDirective_in_implDirective22274);
oldCallConventionDirective834=oldCallConventionDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, oldCallConventionDirective834.getTree());
}
break;
case 9 :
// au/com/integradev/delphi/antlr/Delphi.g:1089:32: dispIDDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_dispIDDirective_in_implDirective22307);
dispIDDirective835=dispIDDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, dispIDDirective835.getTree());
}
break;
case 10 :
// au/com/integradev/delphi/antlr/Delphi.g:1090:32: VARARGS
{
root_0 = (Object)adaptor.nil();
VARARGS836=(Token)match(input,VARARGS,FOLLOW_VARARGS_in_implDirective22340); if (state.failed) return retval;
if ( state.backtracking==0 ) {
VARARGS836_tree = (Object)adaptor.create(VARARGS836);
adaptor.addChild(root_0, VARARGS836_tree);
}
}
break;
case 11 :
// au/com/integradev/delphi/antlr/Delphi.g:1091:32: UNSAFE
{
root_0 = (Object)adaptor.nil();
UNSAFE837=(Token)match(input,UNSAFE,FOLLOW_UNSAFE_in_implDirective22374); if (state.failed) return retval;
if ( state.backtracking==0 ) {
UNSAFE837_tree = (Object)adaptor.create(UNSAFE837);
adaptor.addChild(root_0, UNSAFE837_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 203, implDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "implDirective"
public static class interfaceDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "interfaceDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1093:1: interfaceDirective : ( FORWARD | externalDirective | implDirective );
public final DelphiParser.interfaceDirective_return interfaceDirective() throws RecognitionException {
DelphiParser.interfaceDirective_return retval = new DelphiParser.interfaceDirective_return();
retval.start = input.LT(1);
int interfaceDirective_StartIndex = input.index();
Object root_0 = null;
Token FORWARD838=null;
ParserRuleReturnScope externalDirective839 =null;
ParserRuleReturnScope implDirective840 =null;
Object FORWARD838_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 204) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1093:30: ( FORWARD | externalDirective | implDirective )
int alt289=3;
switch ( input.LA(1) ) {
case FORWARD:
{
alt289=1;
}
break;
case EXTERNAL:
{
alt289=2;
}
break;
case ABSTRACT:
case ASSEMBLER:
case CDECL:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case FAR:
case FINAL:
case INLINE:
case LIBRARY:
case LOCAL:
case MESSAGE:
case NEAR:
case OVERLOAD:
case OVERRIDE:
case PASCAL:
case PLATFORM:
case REGISTER:
case REINTRODUCE:
case SAFECALL:
case STATIC:
case STDCALL:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
{
alt289=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 289, 0, input);
throw nvae;
}
switch (alt289) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1093:32: FORWARD
{
root_0 = (Object)adaptor.nil();
FORWARD838=(Token)match(input,FORWARD,FOLLOW_FORWARD_in_interfaceDirective22422); if (state.failed) return retval;
if ( state.backtracking==0 ) {
FORWARD838_tree = (Object)adaptor.create(FORWARD838);
adaptor.addChild(root_0, FORWARD838_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1094:32: externalDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_externalDirective_in_interfaceDirective22455);
externalDirective839=externalDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, externalDirective839.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1095:32: implDirective
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_implDirective_in_interfaceDirective22488);
implDirective840=implDirective();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, implDirective840.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 204, interfaceDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "interfaceDirective"
public static class bindingDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "bindingDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1097:1: bindingDirective : ( MESSAGE expression | STATIC | DYNAMIC | OVERRIDE | VIRTUAL );
public final DelphiParser.bindingDirective_return bindingDirective() throws RecognitionException {
DelphiParser.bindingDirective_return retval = new DelphiParser.bindingDirective_return();
retval.start = input.LT(1);
int bindingDirective_StartIndex = input.index();
Object root_0 = null;
Token MESSAGE841=null;
Token STATIC843=null;
Token DYNAMIC844=null;
Token OVERRIDE845=null;
Token VIRTUAL846=null;
ParserRuleReturnScope expression842 =null;
Object MESSAGE841_tree=null;
Object STATIC843_tree=null;
Object DYNAMIC844_tree=null;
Object OVERRIDE845_tree=null;
Object VIRTUAL846_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 205) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1097:30: ( MESSAGE expression | STATIC | DYNAMIC | OVERRIDE | VIRTUAL )
int alt290=5;
switch ( input.LA(1) ) {
case MESSAGE:
{
alt290=1;
}
break;
case STATIC:
{
alt290=2;
}
break;
case DYNAMIC:
{
alt290=3;
}
break;
case OVERRIDE:
{
alt290=4;
}
break;
case VIRTUAL:
{
alt290=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 290, 0, input);
throw nvae;
}
switch (alt290) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1097:32: MESSAGE expression
{
root_0 = (Object)adaptor.nil();
MESSAGE841=(Token)match(input,MESSAGE,FOLLOW_MESSAGE_in_bindingDirective22537); if (state.failed) return retval;
if ( state.backtracking==0 ) {
MESSAGE841_tree = (Object)adaptor.create(MESSAGE841);
adaptor.addChild(root_0, MESSAGE841_tree);
}
pushFollow(FOLLOW_expression_in_bindingDirective22539);
expression842=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression842.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1098:32: STATIC
{
root_0 = (Object)adaptor.nil();
STATIC843=(Token)match(input,STATIC,FOLLOW_STATIC_in_bindingDirective22572); if (state.failed) return retval;
if ( state.backtracking==0 ) {
STATIC843_tree = (Object)adaptor.create(STATIC843);
adaptor.addChild(root_0, STATIC843_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1099:32: DYNAMIC
{
root_0 = (Object)adaptor.nil();
DYNAMIC844=(Token)match(input,DYNAMIC,FOLLOW_DYNAMIC_in_bindingDirective22605); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DYNAMIC844_tree = (Object)adaptor.create(DYNAMIC844);
adaptor.addChild(root_0, DYNAMIC844_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:1100:32: OVERRIDE
{
root_0 = (Object)adaptor.nil();
OVERRIDE845=(Token)match(input,OVERRIDE,FOLLOW_OVERRIDE_in_bindingDirective22638); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OVERRIDE845_tree = (Object)adaptor.create(OVERRIDE845);
adaptor.addChild(root_0, OVERRIDE845_tree);
}
}
break;
case 5 :
// au/com/integradev/delphi/antlr/Delphi.g:1101:32: VIRTUAL
{
root_0 = (Object)adaptor.nil();
VIRTUAL846=(Token)match(input,VIRTUAL,FOLLOW_VIRTUAL_in_bindingDirective22671); if (state.failed) return retval;
if ( state.backtracking==0 ) {
VIRTUAL846_tree = (Object)adaptor.create(VIRTUAL846);
adaptor.addChild(root_0, VIRTUAL846_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 205, bindingDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "bindingDirective"
public static class abstractDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "abstractDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1103:1: abstractDirective : ( ABSTRACT | FINAL );
public final DelphiParser.abstractDirective_return abstractDirective() throws RecognitionException {
DelphiParser.abstractDirective_return retval = new DelphiParser.abstractDirective_return();
retval.start = input.LT(1);
int abstractDirective_StartIndex = input.index();
Object root_0 = null;
Token set847=null;
Object set847_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 206) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1103:30: ( ABSTRACT | FINAL )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set847=input.LT(1);
if ( input.LA(1)==ABSTRACT||input.LA(1)==FINAL ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set847));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 206, abstractDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "abstractDirective"
public static class inlineDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "inlineDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1106:1: inlineDirective : ( INLINE | ASSEMBLER );
public final DelphiParser.inlineDirective_return inlineDirective() throws RecognitionException {
DelphiParser.inlineDirective_return retval = new DelphiParser.inlineDirective_return();
retval.start = input.LT(1);
int inlineDirective_StartIndex = input.index();
Object root_0 = null;
Token set848=null;
Object set848_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 207) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1106:30: ( INLINE | ASSEMBLER )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set848=input.LT(1);
if ( input.LA(1)==ASSEMBLER||input.LA(1)==INLINE ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set848));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 207, inlineDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "inlineDirective"
public static class callConvention_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "callConvention"
// au/com/integradev/delphi/antlr/Delphi.g:1109:1: callConvention : ( CDECL | PASCAL | REGISTER | SAFECALL | STDCALL | WINAPI | EXPORT );
public final DelphiParser.callConvention_return callConvention() throws RecognitionException {
DelphiParser.callConvention_return retval = new DelphiParser.callConvention_return();
retval.start = input.LT(1);
int callConvention_StartIndex = input.index();
Object root_0 = null;
Token set849=null;
Object set849_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 208) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1109:30: ( CDECL | PASCAL | REGISTER | SAFECALL | STDCALL | WINAPI | EXPORT )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set849=input.LT(1);
if ( input.LA(1)==CDECL||input.LA(1)==EXPORT||input.LA(1)==PASCAL||input.LA(1)==REGISTER||input.LA(1)==SAFECALL||input.LA(1)==STDCALL||input.LA(1)==WINAPI ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set849));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 208, callConvention_StartIndex); }
}
return retval;
}
// $ANTLR end "callConvention"
public static class oldCallConventionDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "oldCallConventionDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1117:1: oldCallConventionDirective : ( FAR | LOCAL | NEAR );
public final DelphiParser.oldCallConventionDirective_return oldCallConventionDirective() throws RecognitionException {
DelphiParser.oldCallConventionDirective_return retval = new DelphiParser.oldCallConventionDirective_return();
retval.start = input.LT(1);
int oldCallConventionDirective_StartIndex = input.index();
Object root_0 = null;
Token set850=null;
Object set850_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 209) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1117:30: ( FAR | LOCAL | NEAR )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set850=input.LT(1);
if ( input.LA(1)==FAR||input.LA(1)==LOCAL||input.LA(1)==NEAR ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set850));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 209, oldCallConventionDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "oldCallConventionDirective"
public static class portabilityDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "portabilityDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1121:1: portabilityDirective : ( DEPRECATED ^ ( textLiteral )? | EXPERIMENTAL | PLATFORM | LIBRARY );
public final DelphiParser.portabilityDirective_return portabilityDirective() throws RecognitionException {
DelphiParser.portabilityDirective_return retval = new DelphiParser.portabilityDirective_return();
retval.start = input.LT(1);
int portabilityDirective_StartIndex = input.index();
Object root_0 = null;
Token DEPRECATED851=null;
Token EXPERIMENTAL853=null;
Token PLATFORM854=null;
Token LIBRARY855=null;
ParserRuleReturnScope textLiteral852 =null;
Object DEPRECATED851_tree=null;
Object EXPERIMENTAL853_tree=null;
Object PLATFORM854_tree=null;
Object LIBRARY855_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 210) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1121:30: ( DEPRECATED ^ ( textLiteral )? | EXPERIMENTAL | PLATFORM | LIBRARY )
int alt292=4;
switch ( input.LA(1) ) {
case DEPRECATED:
{
alt292=1;
}
break;
case EXPERIMENTAL:
{
alt292=2;
}
break;
case PLATFORM:
{
alt292=3;
}
break;
case LIBRARY:
{
alt292=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 292, 0, input);
throw nvae;
}
switch (alt292) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1121:32: DEPRECATED ^ ( textLiteral )?
{
root_0 = (Object)adaptor.nil();
DEPRECATED851=(Token)match(input,DEPRECATED,FOLLOW_DEPRECATED_in_portabilityDirective23239); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DEPRECATED851_tree = (Object)adaptor.create(DEPRECATED851);
root_0 = (Object)adaptor.becomeRoot(DEPRECATED851_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:1121:44: ( textLiteral )?
int alt291=2;
switch ( input.LA(1) ) {
case TkQuotedString:
{
int LA291_1 = input.LA(2);
if ( (synpred431_Delphi()) ) {
alt291=1;
}
}
break;
case TkCharacterEscapeCode:
{
int LA291_2 = input.LA(2);
if ( (synpred431_Delphi()) ) {
alt291=1;
}
}
break;
case DEREFERENCE:
{
switch ( input.LA(2) ) {
case TkIdentifier:
{
int LA291_7 = input.LA(3);
if ( (synpred431_Delphi()) ) {
alt291=1;
}
}
break;
case TkIntNumber:
{
int LA291_8 = input.LA(3);
if ( (synpred431_Delphi()) ) {
alt291=1;
}
}
break;
case TkAnyChar:
{
int LA291_9 = input.LA(3);
if ( (synpred431_Delphi()) ) {
alt291=1;
}
}
break;
}
}
break;
case TkMultilineString:
{
int LA291_4 = input.LA(2);
if ( (synpred431_Delphi()) ) {
alt291=1;
}
}
break;
}
switch (alt291) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1121:44: textLiteral
{
pushFollow(FOLLOW_textLiteral_in_portabilityDirective23242);
textLiteral852=textLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, textLiteral852.getTree());
}
break;
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1122:32: EXPERIMENTAL
{
root_0 = (Object)adaptor.nil();
EXPERIMENTAL853=(Token)match(input,EXPERIMENTAL,FOLLOW_EXPERIMENTAL_in_portabilityDirective23276); if (state.failed) return retval;
if ( state.backtracking==0 ) {
EXPERIMENTAL853_tree = (Object)adaptor.create(EXPERIMENTAL853);
adaptor.addChild(root_0, EXPERIMENTAL853_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1123:32: PLATFORM
{
root_0 = (Object)adaptor.nil();
PLATFORM854=(Token)match(input,PLATFORM,FOLLOW_PLATFORM_in_portabilityDirective23309); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PLATFORM854_tree = (Object)adaptor.create(PLATFORM854);
adaptor.addChild(root_0, PLATFORM854_tree);
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:1124:32: LIBRARY
{
root_0 = (Object)adaptor.nil();
LIBRARY855=(Token)match(input,LIBRARY,FOLLOW_LIBRARY_in_portabilityDirective23342); if (state.failed) return retval;
if ( state.backtracking==0 ) {
LIBRARY855_tree = (Object)adaptor.create(LIBRARY855);
adaptor.addChild(root_0, LIBRARY855_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 210, portabilityDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "portabilityDirective"
public static class externalDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "externalDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1126:1: externalDirective : EXTERNAL ^ ( dllName )? ( externalSpecifier )* ;
public final DelphiParser.externalDirective_return externalDirective() throws RecognitionException {
DelphiParser.externalDirective_return retval = new DelphiParser.externalDirective_return();
retval.start = input.LT(1);
int externalDirective_StartIndex = input.index();
Object root_0 = null;
Token EXTERNAL856=null;
ParserRuleReturnScope dllName857 =null;
ParserRuleReturnScope externalSpecifier858 =null;
Object EXTERNAL856_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 211) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1126:30: ( EXTERNAL ^ ( dllName )? ( externalSpecifier )* )
// au/com/integradev/delphi/antlr/Delphi.g:1126:32: EXTERNAL ^ ( dllName )? ( externalSpecifier )*
{
root_0 = (Object)adaptor.nil();
EXTERNAL856=(Token)match(input,EXTERNAL,FOLLOW_EXTERNAL_in_externalDirective23390); if (state.failed) return retval;
if ( state.backtracking==0 ) {
EXTERNAL856_tree = (Object)adaptor.create(EXTERNAL856);
root_0 = (Object)adaptor.becomeRoot(EXTERNAL856_tree, root_0);
}
// au/com/integradev/delphi/antlr/Delphi.g:1126:42: ( dllName )?
int alt293=2;
alt293 = dfa293.predict(input);
switch (alt293) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1126:42: dllName
{
pushFollow(FOLLOW_dllName_in_externalDirective23393);
dllName857=dllName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, dllName857.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1126:51: ( externalSpecifier )*
loop294:
while (true) {
int alt294=2;
alt294 = dfa294.predict(input);
switch (alt294) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1126:51: externalSpecifier
{
pushFollow(FOLLOW_externalSpecifier_in_externalDirective23396);
externalSpecifier858=externalSpecifier();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, externalSpecifier858.getTree());
}
break;
default :
break loop294;
}
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 211, externalDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "externalDirective"
public static class dllName_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "dllName"
// au/com/integradev/delphi/antlr/Delphi.g:1128:1: dllName :{...}? expression ;
public final DelphiParser.dllName_return dllName() throws RecognitionException {
DelphiParser.dllName_return retval = new DelphiParser.dllName_return();
retval.start = input.LT(1);
int dllName_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope expression859 =null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 212) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1128:30: ({...}? expression )
// au/com/integradev/delphi/antlr/Delphi.g:1128:32: {...}? expression
{
root_0 = (Object)adaptor.nil();
if ( !((!input.LT(1).getText().equals("name"))) ) {
if (state.backtracking>0) {state.failed=true; return retval;}
throw new FailedPredicateException(input, "dllName", "!input.LT(1).getText().equals(\"name\")");
}
pushFollow(FOLLOW_expression_in_dllName23457);
expression859=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression859.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 212, dllName_StartIndex); }
}
return retval;
}
// $ANTLR end "dllName"
public static class externalSpecifier_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "externalSpecifier"
// au/com/integradev/delphi/antlr/Delphi.g:1130:1: externalSpecifier : ( NAME ^ expression | INDEX ^ expression | DELAYED );
public final DelphiParser.externalSpecifier_return externalSpecifier() throws RecognitionException {
DelphiParser.externalSpecifier_return retval = new DelphiParser.externalSpecifier_return();
retval.start = input.LT(1);
int externalSpecifier_StartIndex = input.index();
Object root_0 = null;
Token NAME860=null;
Token INDEX862=null;
Token DELAYED864=null;
ParserRuleReturnScope expression861 =null;
ParserRuleReturnScope expression863 =null;
Object NAME860_tree=null;
Object INDEX862_tree=null;
Object DELAYED864_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 213) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1130:30: ( NAME ^ expression | INDEX ^ expression | DELAYED )
int alt295=3;
switch ( input.LA(1) ) {
case NAME:
{
alt295=1;
}
break;
case INDEX:
{
alt295=2;
}
break;
case DELAYED:
{
alt295=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 295, 0, input);
throw nvae;
}
switch (alt295) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1130:32: NAME ^ expression
{
root_0 = (Object)adaptor.nil();
NAME860=(Token)match(input,NAME,FOLLOW_NAME_in_externalSpecifier23505); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NAME860_tree = (Object)adaptor.create(NAME860);
root_0 = (Object)adaptor.becomeRoot(NAME860_tree, root_0);
}
pushFollow(FOLLOW_expression_in_externalSpecifier23508);
expression861=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression861.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1131:32: INDEX ^ expression
{
root_0 = (Object)adaptor.nil();
INDEX862=(Token)match(input,INDEX,FOLLOW_INDEX_in_externalSpecifier23541); if (state.failed) return retval;
if ( state.backtracking==0 ) {
INDEX862_tree = (Object)adaptor.create(INDEX862);
root_0 = (Object)adaptor.becomeRoot(INDEX862_tree, root_0);
}
pushFollow(FOLLOW_expression_in_externalSpecifier23544);
expression863=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression863.getTree());
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1132:32: DELAYED
{
root_0 = (Object)adaptor.nil();
DELAYED864=(Token)match(input,DELAYED,FOLLOW_DELAYED_in_externalSpecifier23578); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DELAYED864_tree = (Object)adaptor.create(DELAYED864);
adaptor.addChild(root_0, DELAYED864_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 213, externalSpecifier_StartIndex); }
}
return retval;
}
// $ANTLR end "externalSpecifier"
public static class dispIDDirective_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "dispIDDirective"
// au/com/integradev/delphi/antlr/Delphi.g:1134:1: dispIDDirective : DISPID expression ;
public final DelphiParser.dispIDDirective_return dispIDDirective() throws RecognitionException {
DelphiParser.dispIDDirective_return retval = new DelphiParser.dispIDDirective_return();
retval.start = input.LT(1);
int dispIDDirective_StartIndex = input.index();
Object root_0 = null;
Token DISPID865=null;
ParserRuleReturnScope expression866 =null;
Object DISPID865_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 214) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1134:30: ( DISPID expression )
// au/com/integradev/delphi/antlr/Delphi.g:1134:32: DISPID expression
{
root_0 = (Object)adaptor.nil();
DISPID865=(Token)match(input,DISPID,FOLLOW_DISPID_in_dispIDDirective23629); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DISPID865_tree = (Object)adaptor.create(DISPID865);
adaptor.addChild(root_0, DISPID865_tree);
}
pushFollow(FOLLOW_expression_in_dispIDDirective23631);
expression866=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression866.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 214, dispIDDirective_StartIndex); }
}
return retval;
}
// $ANTLR end "dispIDDirective"
public static class ident_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "ident"
// au/com/integradev/delphi/antlr/Delphi.g:1140:1: ident : ( TkIdentifier | keywordsUsedAsNames -> ^() );
public final DelphiParser.ident_return ident() throws RecognitionException {
DelphiParser.ident_return retval = new DelphiParser.ident_return();
retval.start = input.LT(1);
int ident_StartIndex = input.index();
Object root_0 = null;
Token TkIdentifier867=null;
ParserRuleReturnScope keywordsUsedAsNames868 =null;
Object TkIdentifier867_tree=null;
RewriteRuleSubtreeStream stream_keywordsUsedAsNames=new RewriteRuleSubtreeStream(adaptor,"rule keywordsUsedAsNames");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 215) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1140:30: ( TkIdentifier | keywordsUsedAsNames -> ^() )
int alt296=2;
int LA296_0 = input.LA(1);
if ( (LA296_0==TkIdentifier) ) {
alt296=1;
}
else if ( ((LA296_0 >= ABSOLUTE && LA296_0 <= ABSTRACT)||LA296_0==ALIGN||(LA296_0 >= ASSEMBLER && LA296_0 <= ASSEMBLY)||(LA296_0 >= AT && LA296_0 <= AUTOMATED)||LA296_0==CDECL||LA296_0==CONTAINS||(LA296_0 >= DEFAULT && LA296_0 <= DEPRECATED)||LA296_0==DISPID||LA296_0==DYNAMIC||(LA296_0 >= EXPERIMENTAL && LA296_0 <= EXPORT)||LA296_0==EXTERNAL||LA296_0==FAR||LA296_0==FINAL||LA296_0==FORWARD||LA296_0==HELPER||LA296_0==IMPLEMENTS||LA296_0==INDEX||LA296_0==LABEL||LA296_0==LOCAL||LA296_0==MESSAGE||(LA296_0 >= NAME && LA296_0 <= NEAR)||LA296_0==NODEFAULT||(LA296_0 >= ON && LA296_0 <= OPERATOR)||(LA296_0 >= OUT && LA296_0 <= OVERRIDE)||LA296_0==PACKAGE||(LA296_0 >= PASCAL && LA296_0 <= PLATFORM)||LA296_0==PRIVATE||(LA296_0 >= PROTECTED && LA296_0 <= PUBLISHED)||(LA296_0 >= READ && LA296_0 <= READONLY)||(LA296_0 >= REFERENCE && LA296_0 <= REINTRODUCE)||(LA296_0 >= REQUIRES && LA296_0 <= RESIDENT)||(LA296_0 >= SAFECALL && LA296_0 <= SEALED)||(LA296_0 >= STATIC && LA296_0 <= STRICT)||LA296_0==UNSAFE||(LA296_0 >= VARARGS && LA296_0 <= VIRTUAL)||LA296_0==WINAPI||(LA296_0 >= WRITE && LA296_0 <= WRITEONLY)) ) {
alt296=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 296, 0, input);
throw nvae;
}
switch (alt296) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1140:32: TkIdentifier
{
root_0 = (Object)adaptor.nil();
TkIdentifier867=(Token)match(input,TkIdentifier,FOLLOW_TkIdentifier_in_ident23695); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkIdentifier867_tree = (Object)adaptor.create(TkIdentifier867);
adaptor.addChild(root_0, TkIdentifier867_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1141:32: keywordsUsedAsNames
{
pushFollow(FOLLOW_keywordsUsedAsNames_in_ident23728);
keywordsUsedAsNames868=keywordsUsedAsNames();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_keywordsUsedAsNames.add(keywordsUsedAsNames868.getTree());
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1141:52: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:1141:55: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 215, ident_StartIndex); }
}
return retval;
}
// $ANTLR end "ident"
public static class keywordsUsedAsNames_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "keywordsUsedAsNames"
// au/com/integradev/delphi/antlr/Delphi.g:1143:1: keywordsUsedAsNames : ( ( ABSOLUTE | ABSTRACT | ALIGN | ASSEMBLER | ASSEMBLY | AT | AUTOMATED | CDECL ) | ( CONTAINS | DEFAULT | DELAYED | DEPRECATED | DISPID | DYNAMIC | EXPERIMENTAL | EXPORT ) | ( EXTERNAL | FAR | FINAL | FORWARD | HELPER | IMPLEMENTS | INDEX | LOCAL | MESSAGE | NAME ) | ( NEAR | NODEFAULT | ON | OPERATOR | OUT | OVERLOAD | OVERRIDE | PACKAGE | PASCAL | PLATFORM ) | ( PRIVATE | PROTECTED | PUBLIC | PUBLISHED | READ | READONLY | REFERENCE | REGISTER | REINTRODUCE ) | ( REQUIRES | RESIDENT | SAFECALL | SEALED | STATIC | STDCALL | STORED | STRICT | UNSAFE ) | ( VARARGS | VIRTUAL | WINAPI | WRITE | WRITEONLY ) | ( LABEL ) );
public final DelphiParser.keywordsUsedAsNames_return keywordsUsedAsNames() throws RecognitionException {
DelphiParser.keywordsUsedAsNames_return retval = new DelphiParser.keywordsUsedAsNames_return();
retval.start = input.LT(1);
int keywordsUsedAsNames_StartIndex = input.index();
Object root_0 = null;
Token set869=null;
Object set869_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 216) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1143:30: ( ( ABSOLUTE | ABSTRACT | ALIGN | ASSEMBLER | ASSEMBLY | AT | AUTOMATED | CDECL ) | ( CONTAINS | DEFAULT | DELAYED | DEPRECATED | DISPID | DYNAMIC | EXPERIMENTAL | EXPORT ) | ( EXTERNAL | FAR | FINAL | FORWARD | HELPER | IMPLEMENTS | INDEX | LOCAL | MESSAGE | NAME ) | ( NEAR | NODEFAULT | ON | OPERATOR | OUT | OVERLOAD | OVERRIDE | PACKAGE | PASCAL | PLATFORM ) | ( PRIVATE | PROTECTED | PUBLIC | PUBLISHED | READ | READONLY | REFERENCE | REGISTER | REINTRODUCE ) | ( REQUIRES | RESIDENT | SAFECALL | SEALED | STATIC | STDCALL | STORED | STRICT | UNSAFE ) | ( VARARGS | VIRTUAL | WINAPI | WRITE | WRITEONLY ) | ( LABEL ) )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set869=input.LT(1);
if ( (input.LA(1) >= ABSOLUTE && input.LA(1) <= ABSTRACT)||input.LA(1)==ALIGN||(input.LA(1) >= ASSEMBLER && input.LA(1) <= ASSEMBLY)||(input.LA(1) >= AT && input.LA(1) <= AUTOMATED)||input.LA(1)==CDECL||input.LA(1)==CONTAINS||(input.LA(1) >= DEFAULT && input.LA(1) <= DEPRECATED)||input.LA(1)==DISPID||input.LA(1)==DYNAMIC||(input.LA(1) >= EXPERIMENTAL && input.LA(1) <= EXPORT)||input.LA(1)==EXTERNAL||input.LA(1)==FAR||input.LA(1)==FINAL||input.LA(1)==FORWARD||input.LA(1)==HELPER||input.LA(1)==IMPLEMENTS||input.LA(1)==INDEX||input.LA(1)==LABEL||input.LA(1)==LOCAL||input.LA(1)==MESSAGE||(input.LA(1) >= NAME && input.LA(1) <= NEAR)||input.LA(1)==NODEFAULT||(input.LA(1) >= ON && input.LA(1) <= OPERATOR)||(input.LA(1) >= OUT && input.LA(1) <= OVERRIDE)||input.LA(1)==PACKAGE||(input.LA(1) >= PASCAL && input.LA(1) <= PLATFORM)||input.LA(1)==PRIVATE||(input.LA(1) >= PROTECTED && input.LA(1) <= PUBLISHED)||(input.LA(1) >= READ && input.LA(1) <= READONLY)||(input.LA(1) >= REFERENCE && input.LA(1) <= REINTRODUCE)||(input.LA(1) >= REQUIRES && input.LA(1) <= RESIDENT)||(input.LA(1) >= SAFECALL && input.LA(1) <= SEALED)||(input.LA(1) >= STATIC && input.LA(1) <= STRICT)||input.LA(1)==UNSAFE||(input.LA(1) >= VARARGS && input.LA(1) <= VIRTUAL)||input.LA(1)==WINAPI||(input.LA(1) >= WRITE && input.LA(1) <= WRITEONLY) ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set869));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 216, keywordsUsedAsNames_StartIndex); }
}
return retval;
}
// $ANTLR end "keywordsUsedAsNames"
public static class keywords_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "keywords"
// au/com/integradev/delphi/antlr/Delphi.g:1152:1: keywords : ( ( ABSOLUTE | ABSTRACT | AND | ALIGN | ARRAY | AS | ASM | ASSEMBLER | ASSEMBLY ) | ( AT | AUTOMATED | BEGIN | CASE | CDECL | CLASS | CONST | CONSTRUCTOR | CONTAINS | DEFAULT ) | ( DELAYED | DEPRECATED | DESTRUCTOR | DISPID | DISPINTERFACE | DIV | DO | DOWNTO | DYNAMIC ) | ( ELSE | END | EXCEPT | EXPERIMENTAL | EXPORT | EXPORTS | EXTERNAL | FAR | FILE | FINAL ) | ( FINALIZATION | FINALLY | FOR | FORWARD | FUNCTION | GOTO | HELPER | IF | IMPLEMENTATION ) | ( IMPLEMENTS | IN | INDEX | INHERITED | INITIALIZATION | INLINE | INTERFACE | IS | LABEL ) | ( LIBRARY | LOCAL | MESSAGE | MOD | NAME | NEAR | NIL | NODEFAULT | NOT | OBJECT | OF | ON ) | ( OPERATOR | OR | OUT | OVERLOAD | OVERRIDE | PACKAGE | PACKED | PASCAL | PLATFORM | PRIVATE ) | ( PROCEDURE | PROGRAM | PROPERTY | PROTECTED | PUBLIC | PUBLISHED | RAISE | READ | READONLY ) | ( RECORD | REFERENCE | REGISTER | REINTRODUCE | REPEAT | REQUIRES | RESIDENT ) | ( RESOURCESTRING | SAFECALL | SEALED | SET | SHL | SHR | STATIC | STDCALL | STORED | STRICT ) | ( STRING | THEN | THREADVAR | TO | TRY | TYPE | UNIT | UNSAFE | UNTIL | USES | VAR | VARARGS ) | ( VIRTUAL | WHILE | WINAPI | WITH | WRITE | WRITEONLY | XOR ) );
public final DelphiParser.keywords_return keywords() throws RecognitionException {
DelphiParser.keywords_return retval = new DelphiParser.keywords_return();
retval.start = input.LT(1);
int keywords_StartIndex = input.index();
Object root_0 = null;
Token set870=null;
Object set870_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 217) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1152:30: ( ( ABSOLUTE | ABSTRACT | AND | ALIGN | ARRAY | AS | ASM | ASSEMBLER | ASSEMBLY ) | ( AT | AUTOMATED | BEGIN | CASE | CDECL | CLASS | CONST | CONSTRUCTOR | CONTAINS | DEFAULT ) | ( DELAYED | DEPRECATED | DESTRUCTOR | DISPID | DISPINTERFACE | DIV | DO | DOWNTO | DYNAMIC ) | ( ELSE | END | EXCEPT | EXPERIMENTAL | EXPORT | EXPORTS | EXTERNAL | FAR | FILE | FINAL ) | ( FINALIZATION | FINALLY | FOR | FORWARD | FUNCTION | GOTO | HELPER | IF | IMPLEMENTATION ) | ( IMPLEMENTS | IN | INDEX | INHERITED | INITIALIZATION | INLINE | INTERFACE | IS | LABEL ) | ( LIBRARY | LOCAL | MESSAGE | MOD | NAME | NEAR | NIL | NODEFAULT | NOT | OBJECT | OF | ON ) | ( OPERATOR | OR | OUT | OVERLOAD | OVERRIDE | PACKAGE | PACKED | PASCAL | PLATFORM | PRIVATE ) | ( PROCEDURE | PROGRAM | PROPERTY | PROTECTED | PUBLIC | PUBLISHED | RAISE | READ | READONLY ) | ( RECORD | REFERENCE | REGISTER | REINTRODUCE | REPEAT | REQUIRES | RESIDENT ) | ( RESOURCESTRING | SAFECALL | SEALED | SET | SHL | SHR | STATIC | STDCALL | STORED | STRICT ) | ( STRING | THEN | THREADVAR | TO | TRY | TYPE | UNIT | UNSAFE | UNTIL | USES | VAR | VARARGS ) | ( VIRTUAL | WHILE | WINAPI | WITH | WRITE | WRITEONLY | XOR ) )
// au/com/integradev/delphi/antlr/Delphi.g:
{
root_0 = (Object)adaptor.nil();
set870=input.LT(1);
if ( (input.LA(1) >= ABSOLUTE && input.LA(1) <= ABSTRACT)||input.LA(1)==ALIGN||(input.LA(1) >= AND && input.LA(1) <= ASSEMBLY)||(input.LA(1) >= AT && input.LA(1) <= AUTOMATED)||input.LA(1)==BEGIN||(input.LA(1) >= CASE && input.LA(1) <= CLASS)||(input.LA(1) >= CONST && input.LA(1) <= CONTAINS)||(input.LA(1) >= DEFAULT && input.LA(1) <= DEPRECATED)||(input.LA(1) >= DESTRUCTOR && input.LA(1) <= DIV)||input.LA(1)==DO||(input.LA(1) >= DOWNTO && input.LA(1) <= DYNAMIC)||(input.LA(1) >= ELSE && input.LA(1) <= END)||(input.LA(1) >= EXCEPT && input.LA(1) <= EXTERNAL)||(input.LA(1) >= FAR && input.LA(1) <= FUNCTION)||input.LA(1)==GOTO||input.LA(1)==HELPER||(input.LA(1) >= IF && input.LA(1) <= IS)||input.LA(1)==LABEL||(input.LA(1) >= LIBRARY && input.LA(1) <= LOCAL)||input.LA(1)==MESSAGE||input.LA(1)==MOD||(input.LA(1) >= NAME && input.LA(1) <= NOT)||(input.LA(1) >= OBJECT && input.LA(1) <= OVERRIDE)||(input.LA(1) >= PACKAGE && input.LA(1) <= PACKED)||(input.LA(1) >= PASCAL && input.LA(1) <= PLATFORM)||(input.LA(1) >= PRIVATE && input.LA(1) <= PUBLISHED)||(input.LA(1) >= RAISE && input.LA(1) <= RESOURCESTRING)||(input.LA(1) >= SAFECALL && input.LA(1) <= SEALED)||(input.LA(1) >= SET && input.LA(1) <= SHR)||(input.LA(1) >= STATIC && input.LA(1) <= STRING)||(input.LA(1) >= THEN && input.LA(1) <= TYPE)||(input.LA(1) >= UNIT && input.LA(1) <= USES)||(input.LA(1) >= VAR && input.LA(1) <= VIRTUAL)||input.LA(1)==WHILE||(input.LA(1) >= WINAPI && input.LA(1) <= WRITEONLY)||input.LA(1)==XOR ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (Object)adaptor.create(set870));
state.errorRecovery=false;
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 217, keywords_StartIndex); }
}
return retval;
}
// $ANTLR end "keywords"
public static class nameDeclarationList_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "nameDeclarationList"
// au/com/integradev/delphi/antlr/Delphi.g:1166:1: nameDeclarationList : nameDeclaration ( ',' nameDeclaration )* -> ^( TkNameDeclarationList nameDeclaration ( ',' nameDeclaration )* ) ;
public final DelphiParser.nameDeclarationList_return nameDeclarationList() throws RecognitionException {
DelphiParser.nameDeclarationList_return retval = new DelphiParser.nameDeclarationList_return();
retval.start = input.LT(1);
int nameDeclarationList_StartIndex = input.index();
Object root_0 = null;
Token char_literal872=null;
ParserRuleReturnScope nameDeclaration871 =null;
ParserRuleReturnScope nameDeclaration873 =null;
Object char_literal872_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleSubtreeStream stream_nameDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule nameDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 218) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1166:30: ( nameDeclaration ( ',' nameDeclaration )* -> ^( TkNameDeclarationList nameDeclaration ( ',' nameDeclaration )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:1166:32: nameDeclaration ( ',' nameDeclaration )*
{
pushFollow(FOLLOW_nameDeclaration_in_nameDeclarationList25200);
nameDeclaration871=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration871.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1166:48: ( ',' nameDeclaration )*
loop297:
while (true) {
int alt297=2;
int LA297_0 = input.LA(1);
if ( (LA297_0==COMMA) ) {
alt297=1;
}
switch (alt297) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1166:49: ',' nameDeclaration
{
char_literal872=(Token)match(input,COMMA,FOLLOW_COMMA_in_nameDeclarationList25203); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(char_literal872);
pushFollow(FOLLOW_nameDeclaration_in_nameDeclarationList25205);
nameDeclaration873=nameDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_nameDeclaration.add(nameDeclaration873.getTree());
}
break;
default :
break loop297;
}
}
// AST REWRITE
// elements: nameDeclaration, nameDeclaration, COMMA
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1166:71: -> ^( TkNameDeclarationList nameDeclaration ( ',' nameDeclaration )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:1166:74: ^( TkNameDeclarationList nameDeclaration ( ',' nameDeclaration )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameDeclarationListNodeImpl(TkNameDeclarationList), root_1);
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1166:143: ( ',' nameDeclaration )*
while ( stream_nameDeclaration.hasNext()||stream_COMMA.hasNext() ) {
adaptor.addChild(root_1, stream_COMMA.nextNode());
adaptor.addChild(root_1, stream_nameDeclaration.nextTree());
}
stream_nameDeclaration.reset();
stream_COMMA.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 218, nameDeclarationList_StartIndex); }
}
return retval;
}
// $ANTLR end "nameDeclarationList"
public static class nameDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "nameDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:1168:1: nameDeclaration : ident -> ^( TkNameDeclaration ident ) ;
public final DelphiParser.nameDeclaration_return nameDeclaration() throws RecognitionException {
DelphiParser.nameDeclaration_return retval = new DelphiParser.nameDeclaration_return();
retval.start = input.LT(1);
int nameDeclaration_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope ident874 =null;
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 219) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1168:30: ( ident -> ^( TkNameDeclaration ident ) )
// au/com/integradev/delphi/antlr/Delphi.g:1168:32: ident
{
pushFollow(FOLLOW_ident_in_nameDeclaration25275);
ident874=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident874.getTree());
// AST REWRITE
// elements: ident
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1168:38: -> ^( TkNameDeclaration ident )
{
// au/com/integradev/delphi/antlr/Delphi.g:1168:41: ^( TkNameDeclaration ident )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new SimpleNameDeclarationNodeImpl(TkNameDeclaration), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 219, nameDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "nameDeclaration"
public static class genericNameDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "genericNameDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:1170:1: genericNameDeclaration : ident ( '.' extendedIdent )* ( genericDefinition )? -> ^( TkNameDeclaration ident ( extendedIdent )* ( genericDefinition )? ) ;
public final DelphiParser.genericNameDeclaration_return genericNameDeclaration() throws RecognitionException {
DelphiParser.genericNameDeclaration_return retval = new DelphiParser.genericNameDeclaration_return();
retval.start = input.LT(1);
int genericNameDeclaration_StartIndex = input.index();
Object root_0 = null;
Token char_literal876=null;
ParserRuleReturnScope ident875 =null;
ParserRuleReturnScope extendedIdent877 =null;
ParserRuleReturnScope genericDefinition878 =null;
Object char_literal876_tree=null;
RewriteRuleTokenStream stream_DOT=new RewriteRuleTokenStream(adaptor,"token DOT");
RewriteRuleSubtreeStream stream_genericDefinition=new RewriteRuleSubtreeStream(adaptor,"rule genericDefinition");
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
RewriteRuleSubtreeStream stream_extendedIdent=new RewriteRuleSubtreeStream(adaptor,"rule extendedIdent");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 220) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1170:30: ( ident ( '.' extendedIdent )* ( genericDefinition )? -> ^( TkNameDeclaration ident ( extendedIdent )* ( genericDefinition )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1170:32: ident ( '.' extendedIdent )* ( genericDefinition )?
{
pushFollow(FOLLOW_ident_in_genericNameDeclaration25329);
ident875=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident875.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1170:38: ( '.' extendedIdent )*
loop298:
while (true) {
int alt298=2;
int LA298_0 = input.LA(1);
if ( (LA298_0==DOT) ) {
alt298=1;
}
switch (alt298) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1170:39: '.' extendedIdent
{
char_literal876=(Token)match(input,DOT,FOLLOW_DOT_in_genericNameDeclaration25332); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DOT.add(char_literal876);
pushFollow(FOLLOW_extendedIdent_in_genericNameDeclaration25334);
extendedIdent877=extendedIdent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedIdent.add(extendedIdent877.getTree());
}
break;
default :
break loop298;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:1170:59: ( genericDefinition )?
int alt299=2;
int LA299_0 = input.LA(1);
if ( (LA299_0==LESS_THAN) ) {
alt299=1;
}
switch (alt299) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1170:59: genericDefinition
{
pushFollow(FOLLOW_genericDefinition_in_genericNameDeclaration25338);
genericDefinition878=genericDefinition();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericDefinition.add(genericDefinition878.getTree());
}
break;
}
// AST REWRITE
// elements: extendedIdent, genericDefinition, ident
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1171:30: -> ^( TkNameDeclaration ident ( extendedIdent )* ( genericDefinition )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1171:33: ^( TkNameDeclaration ident ( extendedIdent )* ( genericDefinition )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new SimpleNameDeclarationNodeImpl(TkNameDeclaration), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1171:90: ( extendedIdent )*
while ( stream_extendedIdent.hasNext() ) {
adaptor.addChild(root_1, stream_extendedIdent.nextTree());
}
stream_extendedIdent.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1171:105: ( genericDefinition )?
if ( stream_genericDefinition.hasNext() ) {
adaptor.addChild(root_1, stream_genericDefinition.nextTree());
}
stream_genericDefinition.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 220, genericNameDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "genericNameDeclaration"
public static class qualifiedNameDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "qualifiedNameDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:1173:1: qualifiedNameDeclaration : ident ( '.' extendedIdent )* -> ^( TkNameDeclaration ident ( extendedIdent )* ) ;
public final DelphiParser.qualifiedNameDeclaration_return qualifiedNameDeclaration() throws RecognitionException {
DelphiParser.qualifiedNameDeclaration_return retval = new DelphiParser.qualifiedNameDeclaration_return();
retval.start = input.LT(1);
int qualifiedNameDeclaration_StartIndex = input.index();
Object root_0 = null;
Token char_literal880=null;
ParserRuleReturnScope ident879 =null;
ParserRuleReturnScope extendedIdent881 =null;
Object char_literal880_tree=null;
RewriteRuleTokenStream stream_DOT=new RewriteRuleTokenStream(adaptor,"token DOT");
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
RewriteRuleSubtreeStream stream_extendedIdent=new RewriteRuleSubtreeStream(adaptor,"rule extendedIdent");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 221) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1173:30: ( ident ( '.' extendedIdent )* -> ^( TkNameDeclaration ident ( extendedIdent )* ) )
// au/com/integradev/delphi/antlr/Delphi.g:1173:32: ident ( '.' extendedIdent )*
{
pushFollow(FOLLOW_ident_in_qualifiedNameDeclaration25426);
ident879=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident879.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1173:38: ( '.' extendedIdent )*
loop300:
while (true) {
int alt300=2;
int LA300_0 = input.LA(1);
if ( (LA300_0==DOT) ) {
alt300=1;
}
switch (alt300) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1173:39: '.' extendedIdent
{
char_literal880=(Token)match(input,DOT,FOLLOW_DOT_in_qualifiedNameDeclaration25429); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DOT.add(char_literal880);
pushFollow(FOLLOW_extendedIdent_in_qualifiedNameDeclaration25431);
extendedIdent881=extendedIdent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedIdent.add(extendedIdent881.getTree());
}
break;
default :
break loop300;
}
}
// AST REWRITE
// elements: extendedIdent, ident
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1174:30: -> ^( TkNameDeclaration ident ( extendedIdent )* )
{
// au/com/integradev/delphi/antlr/Delphi.g:1174:33: ^( TkNameDeclaration ident ( extendedIdent )* )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new QualifiedNameDeclarationNodeImpl(TkNameDeclaration), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1174:93: ( extendedIdent )*
while ( stream_extendedIdent.hasNext() ) {
adaptor.addChild(root_1, stream_extendedIdent.nextTree());
}
stream_extendedIdent.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 221, qualifiedNameDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "qualifiedNameDeclaration"
public static class specialOpNameDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "specialOpNameDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:1176:1: specialOpNameDeclaration : specialOperatorName -> ^( TkNameDeclaration specialOperatorName ) ;
public final DelphiParser.specialOpNameDeclaration_return specialOpNameDeclaration() throws RecognitionException {
DelphiParser.specialOpNameDeclaration_return retval = new DelphiParser.specialOpNameDeclaration_return();
retval.start = input.LT(1);
int specialOpNameDeclaration_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope specialOperatorName882 =null;
RewriteRuleSubtreeStream stream_specialOperatorName=new RewriteRuleSubtreeStream(adaptor,"rule specialOperatorName");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 222) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1176:30: ( specialOperatorName -> ^( TkNameDeclaration specialOperatorName ) )
// au/com/integradev/delphi/antlr/Delphi.g:1176:32: specialOperatorName
{
pushFollow(FOLLOW_specialOperatorName_in_specialOpNameDeclaration25517);
specialOperatorName882=specialOperatorName();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_specialOperatorName.add(specialOperatorName882.getTree());
// AST REWRITE
// elements: specialOperatorName
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1177:30: -> ^( TkNameDeclaration specialOperatorName )
{
// au/com/integradev/delphi/antlr/Delphi.g:1177:33: ^( TkNameDeclaration specialOperatorName )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new SimpleNameDeclarationNodeImpl(TkNameDeclaration), root_1);
adaptor.addChild(root_1, stream_specialOperatorName.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 222, specialOpNameDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "specialOpNameDeclaration"
public static class specialOperatorName_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "specialOperatorName"
// au/com/integradev/delphi/antlr/Delphi.g:1179:1: specialOperatorName : IN -> ^() ;
public final DelphiParser.specialOperatorName_return specialOperatorName() throws RecognitionException {
DelphiParser.specialOperatorName_return retval = new DelphiParser.specialOperatorName_return();
retval.start = input.LT(1);
int specialOperatorName_StartIndex = input.index();
Object root_0 = null;
Token IN883=null;
Object IN883_tree=null;
RewriteRuleTokenStream stream_IN=new RewriteRuleTokenStream(adaptor,"token IN");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 223) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1179:30: ( IN -> ^() )
// au/com/integradev/delphi/antlr/Delphi.g:1179:32: IN
{
IN883=(Token)match(input,IN,FOLLOW_IN_in_specialOperatorName25603); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_IN.add(IN883);
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1179:35: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:1179:38: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 223, specialOperatorName_StartIndex); }
}
return retval;
}
// $ANTLR end "specialOperatorName"
public static class nameReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "nameReference"
// au/com/integradev/delphi/antlr/Delphi.g:1181:1: nameReference : ident ( genericArguments )? ( '.' extendedNameReference )? -> ^( TkNameReference ident ( genericArguments )? ( '.' extendedNameReference )? ) ;
public final DelphiParser.nameReference_return nameReference() throws RecognitionException {
DelphiParser.nameReference_return retval = new DelphiParser.nameReference_return();
retval.start = input.LT(1);
int nameReference_StartIndex = input.index();
Object root_0 = null;
Token char_literal886=null;
ParserRuleReturnScope ident884 =null;
ParserRuleReturnScope genericArguments885 =null;
ParserRuleReturnScope extendedNameReference887 =null;
Object char_literal886_tree=null;
RewriteRuleTokenStream stream_DOT=new RewriteRuleTokenStream(adaptor,"token DOT");
RewriteRuleSubtreeStream stream_extendedNameReference=new RewriteRuleSubtreeStream(adaptor,"rule extendedNameReference");
RewriteRuleSubtreeStream stream_genericArguments=new RewriteRuleSubtreeStream(adaptor,"rule genericArguments");
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 224) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1181:30: ( ident ( genericArguments )? ( '.' extendedNameReference )? -> ^( TkNameReference ident ( genericArguments )? ( '.' extendedNameReference )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1181:32: ident ( genericArguments )? ( '.' extendedNameReference )?
{
pushFollow(FOLLOW_ident_in_nameReference25661);
ident884=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident884.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1181:38: ( genericArguments )?
int alt301=2;
alt301 = dfa301.predict(input);
switch (alt301) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1181:38: genericArguments
{
pushFollow(FOLLOW_genericArguments_in_nameReference25663);
genericArguments885=genericArguments();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericArguments.add(genericArguments885.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1181:56: ( '.' extendedNameReference )?
int alt302=2;
int LA302_0 = input.LA(1);
if ( (LA302_0==DOT) ) {
switch ( input.LA(2) ) {
case TkIdentifier:
{
int LA302_3 = input.LA(3);
if ( (synpred626_Delphi()) ) {
alt302=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA302_4 = input.LA(3);
if ( (synpred626_Delphi()) ) {
alt302=1;
}
}
break;
case AND:
case ARRAY:
case AS:
case ASM:
case BEGIN:
case CASE:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case DISPINTERFACE:
case DIV:
case DO:
case DOWNTO:
case ELSE:
case END:
case EXCEPT:
case EXPORTS:
case FILE:
case FINALIZATION:
case FINALLY:
case FOR:
case FUNCTION:
case GOTO:
case IF:
case IMPLEMENTATION:
case IN:
case INHERITED:
case INITIALIZATION:
case INLINE:
case INTERFACE:
case IS:
case LIBRARY:
case MOD:
case NIL:
case NOT:
case OBJECT:
case OF:
case OR:
case PACKED:
case PROCEDURE:
case PROGRAM:
case PROPERTY:
case RAISE:
case RECORD:
case REPEAT:
case RESOURCESTRING:
case SET:
case SHL:
case SHR:
case STRING:
case THEN:
case THREADVAR:
case TO:
case TRY:
case TYPE:
case UNIT:
case UNTIL:
case USES:
case VAR:
case WHILE:
case WITH:
case XOR:
{
int LA302_5 = input.LA(3);
if ( (synpred626_Delphi()) ) {
alt302=1;
}
}
break;
}
}
switch (alt302) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1181:57: '.' extendedNameReference
{
char_literal886=(Token)match(input,DOT,FOLLOW_DOT_in_nameReference25667); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DOT.add(char_literal886);
pushFollow(FOLLOW_extendedNameReference_in_nameReference25669);
extendedNameReference887=extendedNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedNameReference.add(extendedNameReference887.getTree());
}
break;
}
// AST REWRITE
// elements: ident, extendedNameReference, genericArguments, DOT
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1182:30: -> ^( TkNameReference ident ( genericArguments )? ( '.' extendedNameReference )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1182:33: ^( TkNameReference ident ( genericArguments )? ( '.' extendedNameReference )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameReferenceNodeImpl(TkNameReference), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1182:80: ( genericArguments )?
if ( stream_genericArguments.hasNext() ) {
adaptor.addChild(root_1, stream_genericArguments.nextTree());
}
stream_genericArguments.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1182:98: ( '.' extendedNameReference )?
if ( stream_extendedNameReference.hasNext()||stream_DOT.hasNext() ) {
adaptor.addChild(root_1, stream_DOT.nextNode());
adaptor.addChild(root_1, stream_extendedNameReference.nextTree());
}
stream_extendedNameReference.reset();
stream_DOT.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 224, nameReference_StartIndex); }
}
return retval;
}
// $ANTLR end "nameReference"
public static class simpleNameReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "simpleNameReference"
// au/com/integradev/delphi/antlr/Delphi.g:1184:1: simpleNameReference : ident -> ^( TkNameReference ident ) ;
public final DelphiParser.simpleNameReference_return simpleNameReference() throws RecognitionException {
DelphiParser.simpleNameReference_return retval = new DelphiParser.simpleNameReference_return();
retval.start = input.LT(1);
int simpleNameReference_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope ident888 =null;
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 225) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1184:30: ( ident -> ^( TkNameReference ident ) )
// au/com/integradev/delphi/antlr/Delphi.g:1184:32: ident
{
pushFollow(FOLLOW_ident_in_simpleNameReference25767);
ident888=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident888.getTree());
// AST REWRITE
// elements: ident
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1185:30: -> ^( TkNameReference ident )
{
// au/com/integradev/delphi/antlr/Delphi.g:1185:33: ^( TkNameReference ident )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameReferenceNodeImpl(TkNameReference), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 225, simpleNameReference_StartIndex); }
}
return retval;
}
// $ANTLR end "simpleNameReference"
public static class extendedNameReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "extendedNameReference"
// au/com/integradev/delphi/antlr/Delphi.g:1187:1: extendedNameReference : extendedIdent ( genericArguments )? ( '.' extendedNameReference )? -> ^( TkNameReference extendedIdent ( genericArguments )? ( '.' extendedNameReference )? ) ;
public final DelphiParser.extendedNameReference_return extendedNameReference() throws RecognitionException {
DelphiParser.extendedNameReference_return retval = new DelphiParser.extendedNameReference_return();
retval.start = input.LT(1);
int extendedNameReference_StartIndex = input.index();
Object root_0 = null;
Token char_literal891=null;
ParserRuleReturnScope extendedIdent889 =null;
ParserRuleReturnScope genericArguments890 =null;
ParserRuleReturnScope extendedNameReference892 =null;
Object char_literal891_tree=null;
RewriteRuleTokenStream stream_DOT=new RewriteRuleTokenStream(adaptor,"token DOT");
RewriteRuleSubtreeStream stream_extendedNameReference=new RewriteRuleSubtreeStream(adaptor,"rule extendedNameReference");
RewriteRuleSubtreeStream stream_genericArguments=new RewriteRuleSubtreeStream(adaptor,"rule genericArguments");
RewriteRuleSubtreeStream stream_extendedIdent=new RewriteRuleSubtreeStream(adaptor,"rule extendedIdent");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 226) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1187:30: ( extendedIdent ( genericArguments )? ( '.' extendedNameReference )? -> ^( TkNameReference extendedIdent ( genericArguments )? ( '.' extendedNameReference )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1187:32: extendedIdent ( genericArguments )? ( '.' extendedNameReference )?
{
pushFollow(FOLLOW_extendedIdent_in_extendedNameReference25851);
extendedIdent889=extendedIdent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedIdent.add(extendedIdent889.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1187:46: ( genericArguments )?
int alt303=2;
alt303 = dfa303.predict(input);
switch (alt303) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1187:46: genericArguments
{
pushFollow(FOLLOW_genericArguments_in_extendedNameReference25853);
genericArguments890=genericArguments();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_genericArguments.add(genericArguments890.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1187:64: ( '.' extendedNameReference )?
int alt304=2;
int LA304_0 = input.LA(1);
if ( (LA304_0==DOT) ) {
switch ( input.LA(2) ) {
case TkIdentifier:
{
int LA304_3 = input.LA(3);
if ( (synpred628_Delphi()) ) {
alt304=1;
}
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA304_4 = input.LA(3);
if ( (synpred628_Delphi()) ) {
alt304=1;
}
}
break;
case AND:
case ARRAY:
case AS:
case ASM:
case BEGIN:
case CASE:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case DISPINTERFACE:
case DIV:
case DO:
case DOWNTO:
case ELSE:
case END:
case EXCEPT:
case EXPORTS:
case FILE:
case FINALIZATION:
case FINALLY:
case FOR:
case FUNCTION:
case GOTO:
case IF:
case IMPLEMENTATION:
case IN:
case INHERITED:
case INITIALIZATION:
case INLINE:
case INTERFACE:
case IS:
case LIBRARY:
case MOD:
case NIL:
case NOT:
case OBJECT:
case OF:
case OR:
case PACKED:
case PROCEDURE:
case PROGRAM:
case PROPERTY:
case RAISE:
case RECORD:
case REPEAT:
case RESOURCESTRING:
case SET:
case SHL:
case SHR:
case STRING:
case THEN:
case THREADVAR:
case TO:
case TRY:
case TYPE:
case UNIT:
case UNTIL:
case USES:
case VAR:
case WHILE:
case WITH:
case XOR:
{
int LA304_5 = input.LA(3);
if ( (synpred628_Delphi()) ) {
alt304=1;
}
}
break;
}
}
switch (alt304) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1187:65: '.' extendedNameReference
{
char_literal891=(Token)match(input,DOT,FOLLOW_DOT_in_extendedNameReference25857); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DOT.add(char_literal891);
pushFollow(FOLLOW_extendedNameReference_in_extendedNameReference25859);
extendedNameReference892=extendedNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedNameReference.add(extendedNameReference892.getTree());
}
break;
}
// AST REWRITE
// elements: extendedIdent, extendedNameReference, DOT, genericArguments
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1188:30: -> ^( TkNameReference extendedIdent ( genericArguments )? ( '.' extendedNameReference )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1188:33: ^( TkNameReference extendedIdent ( genericArguments )? ( '.' extendedNameReference )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameReferenceNodeImpl(TkNameReference), root_1);
adaptor.addChild(root_1, stream_extendedIdent.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1188:88: ( genericArguments )?
if ( stream_genericArguments.hasNext() ) {
adaptor.addChild(root_1, stream_genericArguments.nextTree());
}
stream_genericArguments.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1188:106: ( '.' extendedNameReference )?
if ( stream_extendedNameReference.hasNext()||stream_DOT.hasNext() ) {
adaptor.addChild(root_1, stream_DOT.nextNode());
adaptor.addChild(root_1, stream_extendedNameReference.nextTree());
}
stream_extendedNameReference.reset();
stream_DOT.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 226, extendedNameReference_StartIndex); }
}
return retval;
}
// $ANTLR end "extendedNameReference"
public static class routineNameReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "routineNameReference"
// au/com/integradev/delphi/antlr/Delphi.g:1190:1: routineNameReference : ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? -> ^( TkNameReference ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? ) ;
public final DelphiParser.routineNameReference_return routineNameReference() throws RecognitionException {
DelphiParser.routineNameReference_return retval = new DelphiParser.routineNameReference_return();
retval.start = input.LT(1);
int routineNameReference_StartIndex = input.index();
Object root_0 = null;
Token char_literal895=null;
ParserRuleReturnScope ident893 =null;
ParserRuleReturnScope routineNameGenericArguments894 =null;
ParserRuleReturnScope extendedRoutineNameReference896 =null;
Object char_literal895_tree=null;
RewriteRuleTokenStream stream_DOT=new RewriteRuleTokenStream(adaptor,"token DOT");
RewriteRuleSubtreeStream stream_ident=new RewriteRuleSubtreeStream(adaptor,"rule ident");
RewriteRuleSubtreeStream stream_routineNameGenericArguments=new RewriteRuleSubtreeStream(adaptor,"rule routineNameGenericArguments");
RewriteRuleSubtreeStream stream_extendedRoutineNameReference=new RewriteRuleSubtreeStream(adaptor,"rule extendedRoutineNameReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 227) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1190:30: ( ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? -> ^( TkNameReference ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1190:32: ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )?
{
pushFollow(FOLLOW_ident_in_routineNameReference25956);
ident893=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ident.add(ident893.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1190:38: ( routineNameGenericArguments )?
int alt305=2;
int LA305_0 = input.LA(1);
if ( (LA305_0==LESS_THAN) ) {
alt305=1;
}
switch (alt305) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1190:38: routineNameGenericArguments
{
pushFollow(FOLLOW_routineNameGenericArguments_in_routineNameReference25958);
routineNameGenericArguments894=routineNameGenericArguments();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineNameGenericArguments.add(routineNameGenericArguments894.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1190:67: ( '.' extendedRoutineNameReference )?
int alt306=2;
int LA306_0 = input.LA(1);
if ( (LA306_0==DOT) ) {
alt306=1;
}
switch (alt306) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1190:68: '.' extendedRoutineNameReference
{
char_literal895=(Token)match(input,DOT,FOLLOW_DOT_in_routineNameReference25962); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DOT.add(char_literal895);
pushFollow(FOLLOW_extendedRoutineNameReference_in_routineNameReference25964);
extendedRoutineNameReference896=extendedRoutineNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedRoutineNameReference.add(extendedRoutineNameReference896.getTree());
}
break;
}
// AST REWRITE
// elements: ident, extendedRoutineNameReference, routineNameGenericArguments, DOT
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1191:30: -> ^( TkNameReference ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1191:33: ^( TkNameReference ident ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameReferenceNodeImpl(TkNameReference), root_1);
adaptor.addChild(root_1, stream_ident.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1191:80: ( routineNameGenericArguments )?
if ( stream_routineNameGenericArguments.hasNext() ) {
adaptor.addChild(root_1, stream_routineNameGenericArguments.nextTree());
}
stream_routineNameGenericArguments.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1191:109: ( '.' extendedRoutineNameReference )?
if ( stream_extendedRoutineNameReference.hasNext()||stream_DOT.hasNext() ) {
adaptor.addChild(root_1, stream_DOT.nextNode());
adaptor.addChild(root_1, stream_extendedRoutineNameReference.nextTree());
}
stream_extendedRoutineNameReference.reset();
stream_DOT.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 227, routineNameReference_StartIndex); }
}
return retval;
}
// $ANTLR end "routineNameReference"
public static class extendedRoutineNameReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "extendedRoutineNameReference"
// au/com/integradev/delphi/antlr/Delphi.g:1193:1: extendedRoutineNameReference : extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? -> ^( TkNameReference extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? ) ;
public final DelphiParser.extendedRoutineNameReference_return extendedRoutineNameReference() throws RecognitionException {
DelphiParser.extendedRoutineNameReference_return retval = new DelphiParser.extendedRoutineNameReference_return();
retval.start = input.LT(1);
int extendedRoutineNameReference_StartIndex = input.index();
Object root_0 = null;
Token char_literal899=null;
ParserRuleReturnScope extendedIdent897 =null;
ParserRuleReturnScope routineNameGenericArguments898 =null;
ParserRuleReturnScope extendedRoutineNameReference900 =null;
Object char_literal899_tree=null;
RewriteRuleTokenStream stream_DOT=new RewriteRuleTokenStream(adaptor,"token DOT");
RewriteRuleSubtreeStream stream_extendedIdent=new RewriteRuleSubtreeStream(adaptor,"rule extendedIdent");
RewriteRuleSubtreeStream stream_routineNameGenericArguments=new RewriteRuleSubtreeStream(adaptor,"rule routineNameGenericArguments");
RewriteRuleSubtreeStream stream_extendedRoutineNameReference=new RewriteRuleSubtreeStream(adaptor,"rule extendedRoutineNameReference");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 228) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1193:30: ( extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? -> ^( TkNameReference extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? ) )
// au/com/integradev/delphi/antlr/Delphi.g:1193:32: extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )?
{
pushFollow(FOLLOW_extendedIdent_in_extendedRoutineNameReference26053);
extendedIdent897=extendedIdent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedIdent.add(extendedIdent897.getTree());
// au/com/integradev/delphi/antlr/Delphi.g:1193:46: ( routineNameGenericArguments )?
int alt307=2;
int LA307_0 = input.LA(1);
if ( (LA307_0==LESS_THAN) ) {
alt307=1;
}
switch (alt307) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1193:46: routineNameGenericArguments
{
pushFollow(FOLLOW_routineNameGenericArguments_in_extendedRoutineNameReference26055);
routineNameGenericArguments898=routineNameGenericArguments();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_routineNameGenericArguments.add(routineNameGenericArguments898.getTree());
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:1193:75: ( '.' extendedRoutineNameReference )?
int alt308=2;
int LA308_0 = input.LA(1);
if ( (LA308_0==DOT) ) {
alt308=1;
}
switch (alt308) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1193:76: '.' extendedRoutineNameReference
{
char_literal899=(Token)match(input,DOT,FOLLOW_DOT_in_extendedRoutineNameReference26059); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_DOT.add(char_literal899);
pushFollow(FOLLOW_extendedRoutineNameReference_in_extendedRoutineNameReference26061);
extendedRoutineNameReference900=extendedRoutineNameReference();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_extendedRoutineNameReference.add(extendedRoutineNameReference900.getTree());
}
break;
}
// AST REWRITE
// elements: extendedIdent, extendedRoutineNameReference, DOT, routineNameGenericArguments
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1194:30: -> ^( TkNameReference extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? )
{
// au/com/integradev/delphi/antlr/Delphi.g:1194:33: ^( TkNameReference extendedIdent ( routineNameGenericArguments )? ( '.' extendedRoutineNameReference )? )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameReferenceNodeImpl(TkNameReference), root_1);
adaptor.addChild(root_1, stream_extendedIdent.nextTree());
// au/com/integradev/delphi/antlr/Delphi.g:1194:88: ( routineNameGenericArguments )?
if ( stream_routineNameGenericArguments.hasNext() ) {
adaptor.addChild(root_1, stream_routineNameGenericArguments.nextTree());
}
stream_routineNameGenericArguments.reset();
// au/com/integradev/delphi/antlr/Delphi.g:1194:117: ( '.' extendedRoutineNameReference )?
if ( stream_extendedRoutineNameReference.hasNext()||stream_DOT.hasNext() ) {
adaptor.addChild(root_1, stream_DOT.nextNode());
adaptor.addChild(root_1, stream_extendedRoutineNameReference.nextTree());
}
stream_extendedRoutineNameReference.reset();
stream_DOT.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 228, extendedRoutineNameReference_StartIndex); }
}
return retval;
}
// $ANTLR end "extendedRoutineNameReference"
public static class extendedIdent_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "extendedIdent"
// au/com/integradev/delphi/antlr/Delphi.g:1196:1: extendedIdent : ( ident | keywords -> ^() );
public final DelphiParser.extendedIdent_return extendedIdent() throws RecognitionException {
DelphiParser.extendedIdent_return retval = new DelphiParser.extendedIdent_return();
retval.start = input.LT(1);
int extendedIdent_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope ident901 =null;
ParserRuleReturnScope keywords902 =null;
RewriteRuleSubtreeStream stream_keywords=new RewriteRuleSubtreeStream(adaptor,"rule keywords");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 229) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1196:30: ( ident | keywords -> ^() )
int alt309=2;
switch ( input.LA(1) ) {
case TkIdentifier:
{
alt309=1;
}
break;
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
int LA309_2 = input.LA(2);
if ( (synpred633_Delphi()) ) {
alt309=1;
}
else if ( (true) ) {
alt309=2;
}
}
break;
case AND:
case ARRAY:
case AS:
case ASM:
case BEGIN:
case CASE:
case CLASS:
case CONST:
case CONSTRUCTOR:
case DESTRUCTOR:
case DISPINTERFACE:
case DIV:
case DO:
case DOWNTO:
case ELSE:
case END:
case EXCEPT:
case EXPORTS:
case FILE:
case FINALIZATION:
case FINALLY:
case FOR:
case FUNCTION:
case GOTO:
case IF:
case IMPLEMENTATION:
case IN:
case INHERITED:
case INITIALIZATION:
case INLINE:
case INTERFACE:
case IS:
case LIBRARY:
case MOD:
case NIL:
case NOT:
case OBJECT:
case OF:
case OR:
case PACKED:
case PROCEDURE:
case PROGRAM:
case PROPERTY:
case RAISE:
case RECORD:
case REPEAT:
case RESOURCESTRING:
case SET:
case SHL:
case SHR:
case STRING:
case THEN:
case THREADVAR:
case TO:
case TRY:
case TYPE:
case UNIT:
case UNTIL:
case USES:
case VAR:
case WHILE:
case WITH:
case XOR:
{
alt309=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 309, 0, input);
throw nvae;
}
switch (alt309) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1196:32: ident
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_ident_in_extendedIdent26165);
ident901=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident901.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1197:32: keywords
{
pushFollow(FOLLOW_keywords_in_extendedIdent26198);
keywords902=keywords();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_keywords.add(keywords902.getTree());
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1197:41: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:1197:44: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 229, extendedIdent_StartIndex); }
}
return retval;
}
// $ANTLR end "extendedIdent"
public static class labelIdent_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "labelIdent"
// au/com/integradev/delphi/antlr/Delphi.g:1199:1: labelIdent : ( ident | TkIntNumber -> ^() | TkHexNumber -> ^() | TkBinaryNumber -> ^() );
public final DelphiParser.labelIdent_return labelIdent() throws RecognitionException {
DelphiParser.labelIdent_return retval = new DelphiParser.labelIdent_return();
retval.start = input.LT(1);
int labelIdent_StartIndex = input.index();
Object root_0 = null;
Token TkIntNumber904=null;
Token TkHexNumber905=null;
Token TkBinaryNumber906=null;
ParserRuleReturnScope ident903 =null;
Object TkIntNumber904_tree=null;
Object TkHexNumber905_tree=null;
Object TkBinaryNumber906_tree=null;
RewriteRuleTokenStream stream_TkHexNumber=new RewriteRuleTokenStream(adaptor,"token TkHexNumber");
RewriteRuleTokenStream stream_TkIntNumber=new RewriteRuleTokenStream(adaptor,"token TkIntNumber");
RewriteRuleTokenStream stream_TkBinaryNumber=new RewriteRuleTokenStream(adaptor,"token TkBinaryNumber");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 230) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1199:30: ( ident | TkIntNumber -> ^() | TkHexNumber -> ^() | TkBinaryNumber -> ^() )
int alt310=4;
switch ( input.LA(1) ) {
case ABSOLUTE:
case ABSTRACT:
case ALIGN:
case ASSEMBLER:
case ASSEMBLY:
case AT:
case AUTOMATED:
case CDECL:
case CONTAINS:
case DEFAULT:
case DELAYED:
case DEPRECATED:
case DISPID:
case DYNAMIC:
case EXPERIMENTAL:
case EXPORT:
case EXTERNAL:
case FAR:
case FINAL:
case FORWARD:
case HELPER:
case IMPLEMENTS:
case INDEX:
case LABEL:
case LOCAL:
case MESSAGE:
case NAME:
case NEAR:
case NODEFAULT:
case ON:
case OPERATOR:
case OUT:
case OVERLOAD:
case OVERRIDE:
case PACKAGE:
case PASCAL:
case PLATFORM:
case PRIVATE:
case PROTECTED:
case PUBLIC:
case PUBLISHED:
case READ:
case READONLY:
case REFERENCE:
case REGISTER:
case REINTRODUCE:
case REQUIRES:
case RESIDENT:
case SAFECALL:
case SEALED:
case STATIC:
case STDCALL:
case STORED:
case STRICT:
case TkIdentifier:
case UNSAFE:
case VARARGS:
case VIRTUAL:
case WINAPI:
case WRITE:
case WRITEONLY:
{
alt310=1;
}
break;
case TkIntNumber:
{
alt310=2;
}
break;
case TkHexNumber:
{
alt310=3;
}
break;
case TkBinaryNumber:
{
alt310=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 310, 0, input);
throw nvae;
}
switch (alt310) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1199:32: ident
{
root_0 = (Object)adaptor.nil();
pushFollow(FOLLOW_ident_in_labelIdent26259);
ident903=ident();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ident903.getTree());
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1200:32: TkIntNumber
{
TkIntNumber904=(Token)match(input,TkIntNumber,FOLLOW_TkIntNumber_in_labelIdent26292); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_TkIntNumber.add(TkIntNumber904);
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1200:44: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:1200:47: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1201:32: TkHexNumber
{
TkHexNumber905=(Token)match(input,TkHexNumber,FOLLOW_TkHexNumber_in_labelIdent26331); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_TkHexNumber.add(TkHexNumber905);
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1201:44: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:1201:47: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
case 4 :
// au/com/integradev/delphi/antlr/Delphi.g:1202:32: TkBinaryNumber
{
TkBinaryNumber906=(Token)match(input,TkBinaryNumber,FOLLOW_TkBinaryNumber_in_labelIdent26370); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_TkBinaryNumber.add(TkBinaryNumber906);
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1202:47: -> ^()
{
// au/com/integradev/delphi/antlr/Delphi.g:1202:50: ^()
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(changeTokenType(TkIdentifier), root_1);
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 230, labelIdent_StartIndex); }
}
return retval;
}
// $ANTLR end "labelIdent"
public static class labelNameDeclaration_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "labelNameDeclaration"
// au/com/integradev/delphi/antlr/Delphi.g:1204:1: labelNameDeclaration : labelIdent -> ^( TkNameDeclaration labelIdent ) ;
public final DelphiParser.labelNameDeclaration_return labelNameDeclaration() throws RecognitionException {
DelphiParser.labelNameDeclaration_return retval = new DelphiParser.labelNameDeclaration_return();
retval.start = input.LT(1);
int labelNameDeclaration_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope labelIdent907 =null;
RewriteRuleSubtreeStream stream_labelIdent=new RewriteRuleSubtreeStream(adaptor,"rule labelIdent");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 231) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1204:30: ( labelIdent -> ^( TkNameDeclaration labelIdent ) )
// au/com/integradev/delphi/antlr/Delphi.g:1204:32: labelIdent
{
pushFollow(FOLLOW_labelIdent_in_labelNameDeclaration26421);
labelIdent907=labelIdent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_labelIdent.add(labelIdent907.getTree());
// AST REWRITE
// elements: labelIdent
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1204:43: -> ^( TkNameDeclaration labelIdent )
{
// au/com/integradev/delphi/antlr/Delphi.g:1204:46: ^( TkNameDeclaration labelIdent )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new SimpleNameDeclarationNodeImpl(TkNameDeclaration), root_1);
adaptor.addChild(root_1, stream_labelIdent.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 231, labelNameDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "labelNameDeclaration"
public static class labelNameReference_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "labelNameReference"
// au/com/integradev/delphi/antlr/Delphi.g:1206:1: labelNameReference : labelIdent -> ^( TkNameReference labelIdent ) ;
public final DelphiParser.labelNameReference_return labelNameReference() throws RecognitionException {
DelphiParser.labelNameReference_return retval = new DelphiParser.labelNameReference_return();
retval.start = input.LT(1);
int labelNameReference_StartIndex = input.index();
Object root_0 = null;
ParserRuleReturnScope labelIdent908 =null;
RewriteRuleSubtreeStream stream_labelIdent=new RewriteRuleSubtreeStream(adaptor,"rule labelIdent");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 232) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1206:30: ( labelIdent -> ^( TkNameReference labelIdent ) )
// au/com/integradev/delphi/antlr/Delphi.g:1206:32: labelIdent
{
pushFollow(FOLLOW_labelIdent_in_labelNameReference26479);
labelIdent908=labelIdent();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_labelIdent.add(labelIdent908.getTree());
// AST REWRITE
// elements: labelIdent
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.getTree():null);
root_0 = (Object)adaptor.nil();
// 1206:43: -> ^( TkNameReference labelIdent )
{
// au/com/integradev/delphi/antlr/Delphi.g:1206:46: ^( TkNameReference labelIdent )
{
Object root_1 = (Object)adaptor.nil();
root_1 = (Object)adaptor.becomeRoot(new NameReferenceNodeImpl(TkNameReference), root_1);
adaptor.addChild(root_1, stream_labelIdent.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 232, labelNameReference_StartIndex); }
}
return retval;
}
// $ANTLR end "labelNameReference"
public static class intNum_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "intNum"
// au/com/integradev/delphi/antlr/Delphi.g:1211:1: intNum : ( TkIntNumber | TkHexNumber | TkBinaryNumber );
public final DelphiParser.intNum_return intNum() throws RecognitionException {
DelphiParser.intNum_return retval = new DelphiParser.intNum_return();
retval.start = input.LT(1);
int intNum_StartIndex = input.index();
Object root_0 = null;
Token TkIntNumber909=null;
Token TkHexNumber910=null;
Token TkBinaryNumber911=null;
Object TkIntNumber909_tree=null;
Object TkHexNumber910_tree=null;
Object TkBinaryNumber911_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 233) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1211:30: ( TkIntNumber | TkHexNumber | TkBinaryNumber )
int alt311=3;
switch ( input.LA(1) ) {
case TkIntNumber:
{
alt311=1;
}
break;
case TkHexNumber:
{
alt311=2;
}
break;
case TkBinaryNumber:
{
alt311=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 311, 0, input);
throw nvae;
}
switch (alt311) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1211:32: TkIntNumber
{
root_0 = (Object)adaptor.nil();
TkIntNumber909=(Token)match(input,TkIntNumber,FOLLOW_TkIntNumber_in_intNum26552); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkIntNumber909_tree = new IntegerLiteralNodeImpl(TkIntNumber909) ;
adaptor.addChild(root_0, TkIntNumber909_tree);
}
}
break;
case 2 :
// au/com/integradev/delphi/antlr/Delphi.g:1212:32: TkHexNumber
{
root_0 = (Object)adaptor.nil();
TkHexNumber910=(Token)match(input,TkHexNumber,FOLLOW_TkHexNumber_in_intNum26588); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkHexNumber910_tree = new IntegerLiteralNodeImpl(TkHexNumber910) ;
adaptor.addChild(root_0, TkHexNumber910_tree);
}
}
break;
case 3 :
// au/com/integradev/delphi/antlr/Delphi.g:1213:32: TkBinaryNumber
{
root_0 = (Object)adaptor.nil();
TkBinaryNumber911=(Token)match(input,TkBinaryNumber,FOLLOW_TkBinaryNumber_in_intNum26624); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkBinaryNumber911_tree = new IntegerLiteralNodeImpl(TkBinaryNumber911) ;
adaptor.addChild(root_0, TkBinaryNumber911_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 233, intNum_StartIndex); }
}
return retval;
}
// $ANTLR end "intNum"
public static class realNum_return extends ParserRuleReturnScope {
Object tree;
@Override
public Object getTree() { return tree; }
};
// $ANTLR start "realNum"
// au/com/integradev/delphi/antlr/Delphi.g:1215:1: realNum : TkRealNumber ;
public final DelphiParser.realNum_return realNum() throws RecognitionException {
DelphiParser.realNum_return retval = new DelphiParser.realNum_return();
retval.start = input.LT(1);
int realNum_StartIndex = input.index();
Object root_0 = null;
Token TkRealNumber912=null;
Object TkRealNumber912_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 234) ) { return retval; }
// au/com/integradev/delphi/antlr/Delphi.g:1215:30: ( TkRealNumber )
// au/com/integradev/delphi/antlr/Delphi.g:1215:32: TkRealNumber
{
root_0 = (Object)adaptor.nil();
TkRealNumber912=(Token)match(input,TkRealNumber,FOLLOW_TkRealNumber_in_realNum26685); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TkRealNumber912_tree = new RealLiteralNodeImpl(TkRealNumber912) ;
adaptor.addChild(root_0, TkRealNumber912_tree);
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (Object)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (Object)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
// do for sure before leaving
if ( state.backtracking>0 ) { memoize(input, 234, realNum_StartIndex); }
}
return retval;
}
// $ANTLR end "realNum"
// $ANTLR start synpred37_Delphi
public final void synpred37_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:455:32: ( routineImplementation )
// au/com/integradev/delphi/antlr/Delphi.g:455:32: routineImplementation
{
pushFollow(FOLLOW_routineImplementation_in_synpred37_Delphi2487);
routineImplementation();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred37_Delphi
// $ANTLR start synpred43_Delphi
public final void synpred43_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:463:32: ( routineInterface )
// au/com/integradev/delphi/antlr/Delphi.g:463:32: routineInterface
{
pushFollow(FOLLOW_routineInterface_in_synpred43_Delphi2737);
routineInterface();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred43_Delphi
// $ANTLR start synpred46_Delphi
public final void synpred46_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:468:103: ( constDeclaration )
// au/com/integradev/delphi/antlr/Delphi.g:468:103: constDeclaration
{
pushFollow(FOLLOW_constDeclaration_in_synpred46_Delphi2903);
constDeclaration();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred46_Delphi
// $ANTLR start synpred49_Delphi
public final void synpred49_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:473:32: ( ( attributeList )? nameDeclaration ':' fixedArrayType '=' arrayExpression ( portabilityDirective )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:473:32: ( attributeList )? nameDeclaration ':' fixedArrayType '=' arrayExpression ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:473:32: ( attributeList )?
int alt314=2;
int LA314_0 = input.LA(1);
if ( (LA314_0==PAREN_BRACKET_LEFT||LA314_0==SQUARE_BRACKET_LEFT) ) {
alt314=1;
}
switch (alt314) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:473:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_synpred49_Delphi3043);
attributeList();
state._fsp--;
if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_nameDeclaration_in_synpred49_Delphi3046);
nameDeclaration();
state._fsp--;
if (state.failed) return;
match(input,COLON,FOLLOW_COLON_in_synpred49_Delphi3048); if (state.failed) return;
pushFollow(FOLLOW_fixedArrayType_in_synpred49_Delphi3050);
fixedArrayType();
state._fsp--;
if (state.failed) return;
match(input,EQUAL,FOLLOW_EQUAL_in_synpred49_Delphi3052); if (state.failed) return;
pushFollow(FOLLOW_arrayExpression_in_synpred49_Delphi3054);
arrayExpression();
state._fsp--;
if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:473:102: ( portabilityDirective )*
loop315:
while (true) {
int alt315=2;
int LA315_0 = input.LA(1);
if ( (LA315_0==DEPRECATED||LA315_0==EXPERIMENTAL||LA315_0==LIBRARY||LA315_0==PLATFORM) ) {
alt315=1;
}
switch (alt315) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:473:102: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_synpred49_Delphi3056);
portabilityDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop315;
}
}
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred49_Delphi3059); if (state.failed) return;
}
}
// $ANTLR end synpred49_Delphi
// $ANTLR start synpred53_Delphi
public final void synpred53_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:478:59: ( typeDeclaration )
// au/com/integradev/delphi/antlr/Delphi.g:478:59: typeDeclaration
{
pushFollow(FOLLOW_typeDeclaration_in_synpred53_Delphi3272);
typeDeclaration();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred53_Delphi
// $ANTLR start synpred54_Delphi
public final void synpred54_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:480:59: ( typeDeclaration )
// au/com/integradev/delphi/antlr/Delphi.g:480:59: typeDeclaration
{
pushFollow(FOLLOW_typeDeclaration_in_synpred54_Delphi3328);
typeDeclaration();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred54_Delphi
// $ANTLR start synpred58_Delphi
public final void synpred58_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:485:107: ( varDeclaration )
// au/com/integradev/delphi/antlr/Delphi.g:485:107: varDeclaration
{
pushFollow(FOLLOW_varDeclaration_in_synpred58_Delphi3512);
varDeclaration();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred58_Delphi
// $ANTLR start synpred60_Delphi
public final void synpred60_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:487:86: ( portabilityDirective )
// au/com/integradev/delphi/antlr/Delphi.g:487:86: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_synpred60_Delphi3573);
portabilityDirective();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred60_Delphi
// $ANTLR start synpred63_Delphi
public final void synpred63_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:487:32: ( ( attributeList )? nameDeclarationList ':' fixedArrayType ( portabilityDirective )* ( arrayVarValueSpec )? ( portabilityDirective )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:487:32: ( attributeList )? nameDeclarationList ':' fixedArrayType ( portabilityDirective )* ( arrayVarValueSpec )? ( portabilityDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:487:32: ( attributeList )?
int alt316=2;
int LA316_0 = input.LA(1);
if ( (LA316_0==PAREN_BRACKET_LEFT||LA316_0==SQUARE_BRACKET_LEFT) ) {
alt316=1;
}
switch (alt316) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:32: attributeList
{
pushFollow(FOLLOW_attributeList_in_synpred63_Delphi3564);
attributeList();
state._fsp--;
if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_nameDeclarationList_in_synpred63_Delphi3567);
nameDeclarationList();
state._fsp--;
if (state.failed) return;
match(input,COLON,FOLLOW_COLON_in_synpred63_Delphi3569); if (state.failed) return;
pushFollow(FOLLOW_fixedArrayType_in_synpred63_Delphi3571);
fixedArrayType();
state._fsp--;
if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:487:86: ( portabilityDirective )*
loop317:
while (true) {
int alt317=2;
switch ( input.LA(1) ) {
case DEPRECATED:
{
int LA317_2 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt317=1;
}
}
break;
case EXPERIMENTAL:
{
int LA317_3 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt317=1;
}
}
break;
case PLATFORM:
{
int LA317_4 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt317=1;
}
}
break;
case LIBRARY:
{
int LA317_5 = input.LA(2);
if ( (synpred60_Delphi()) ) {
alt317=1;
}
}
break;
}
switch (alt317) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:86: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_synpred63_Delphi3573);
portabilityDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop317;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:487:108: ( arrayVarValueSpec )?
int alt318=2;
int LA318_0 = input.LA(1);
if ( (LA318_0==ABSOLUTE||LA318_0==EQUAL) ) {
alt318=1;
}
switch (alt318) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:108: arrayVarValueSpec
{
pushFollow(FOLLOW_arrayVarValueSpec_in_synpred63_Delphi3576);
arrayVarValueSpec();
state._fsp--;
if (state.failed) return;
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:487:127: ( portabilityDirective )*
loop319:
while (true) {
int alt319=2;
int LA319_0 = input.LA(1);
if ( (LA319_0==DEPRECATED||LA319_0==EXPERIMENTAL||LA319_0==LIBRARY||LA319_0==PLATFORM) ) {
alt319=1;
}
switch (alt319) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:487:127: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_synpred63_Delphi3579);
portabilityDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop319;
}
}
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred63_Delphi3582); if (state.failed) return;
}
}
// $ANTLR end synpred63_Delphi
// $ANTLR start synpred65_Delphi
public final void synpred65_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:489:79: ( portabilityDirective )
// au/com/integradev/delphi/antlr/Delphi.g:489:79: portabilityDirective
{
pushFollow(FOLLOW_portabilityDirective_in_synpred65_Delphi3672);
portabilityDirective();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred65_Delphi
// $ANTLR start synpred77_Delphi
public final void synpred77_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:508:32: ( fullFileType )
// au/com/integradev/delphi/antlr/Delphi.g:508:32: fullFileType
{
pushFollow(FOLLOW_fullFileType_in_synpred77_Delphi4178);
fullFileType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred77_Delphi
// $ANTLR start synpred78_Delphi
public final void synpred78_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:509:32: ( classHelperType )
// au/com/integradev/delphi/antlr/Delphi.g:509:32: classHelperType
{
pushFollow(FOLLOW_classHelperType_in_synpred78_Delphi4211);
classHelperType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred78_Delphi
// $ANTLR start synpred79_Delphi
public final void synpred79_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:510:32: ( classReferenceType )
// au/com/integradev/delphi/antlr/Delphi.g:510:32: classReferenceType
{
pushFollow(FOLLOW_classReferenceType_in_synpred79_Delphi4244);
classReferenceType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred79_Delphi
// $ANTLR start synpred80_Delphi
public final void synpred80_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:511:32: ( classType )
// au/com/integradev/delphi/antlr/Delphi.g:511:32: classType
{
pushFollow(FOLLOW_classType_in_synpred80_Delphi4277);
classType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred80_Delphi
// $ANTLR start synpred83_Delphi
public final void synpred83_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:514:32: ( recordType )
// au/com/integradev/delphi/antlr/Delphi.g:514:32: recordType
{
pushFollow(FOLLOW_recordType_in_synpred83_Delphi4376);
recordType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred83_Delphi
// $ANTLR start synpred84_Delphi
public final void synpred84_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:515:32: ( recordHelperType )
// au/com/integradev/delphi/antlr/Delphi.g:515:32: recordHelperType
{
pushFollow(FOLLOW_recordHelperType_in_synpred84_Delphi4409);
recordHelperType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred84_Delphi
// $ANTLR start synpred85_Delphi
public final void synpred85_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:516:32: ( pointerType )
// au/com/integradev/delphi/antlr/Delphi.g:516:32: pointerType
{
pushFollow(FOLLOW_pointerType_in_synpred85_Delphi4442);
pointerType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred85_Delphi
// $ANTLR start synpred86_Delphi
public final void synpred86_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:517:32: ( fullStringType )
// au/com/integradev/delphi/antlr/Delphi.g:517:32: fullStringType
{
pushFollow(FOLLOW_fullStringType_in_synpred86_Delphi4475);
fullStringType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred86_Delphi
// $ANTLR start synpred87_Delphi
public final void synpred87_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:518:32: ( procedureType )
// au/com/integradev/delphi/antlr/Delphi.g:518:32: procedureType
{
pushFollow(FOLLOW_procedureType_in_synpred87_Delphi4508);
procedureType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred87_Delphi
// $ANTLR start synpred88_Delphi
public final void synpred88_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:519:32: ( subRangeType )
// au/com/integradev/delphi/antlr/Delphi.g:519:32: subRangeType
{
pushFollow(FOLLOW_subRangeType_in_synpred88_Delphi4541);
subRangeType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred88_Delphi
// $ANTLR start synpred89_Delphi
public final void synpred89_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:520:32: ( typeOfType )
// au/com/integradev/delphi/antlr/Delphi.g:520:32: typeOfType
{
pushFollow(FOLLOW_typeOfType_in_synpred89_Delphi4574);
typeOfType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred89_Delphi
// $ANTLR start synpred90_Delphi
public final void synpred90_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:521:32: ( strongAliasType )
// au/com/integradev/delphi/antlr/Delphi.g:521:32: strongAliasType
{
pushFollow(FOLLOW_strongAliasType_in_synpred90_Delphi4607);
strongAliasType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred90_Delphi
// $ANTLR start synpred91_Delphi
public final void synpred91_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:522:32: ( weakAliasType )
// au/com/integradev/delphi/antlr/Delphi.g:522:32: weakAliasType
{
pushFollow(FOLLOW_weakAliasType_in_synpred91_Delphi4640);
weakAliasType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred91_Delphi
// $ANTLR start synpred92_Delphi
public final void synpred92_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:523:32: ( enumType )
// au/com/integradev/delphi/antlr/Delphi.g:523:32: enumType
{
pushFollow(FOLLOW_enumType_in_synpred92_Delphi4673);
enumType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred92_Delphi
// $ANTLR start synpred95_Delphi
public final void synpred95_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:528:32: ( fullStringType )
// au/com/integradev/delphi/antlr/Delphi.g:528:32: fullStringType
{
pushFollow(FOLLOW_fullStringType_in_synpred95_Delphi4833);
fullStringType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred95_Delphi
// $ANTLR start synpred96_Delphi
public final void synpred96_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:529:32: ( fullFileType )
// au/com/integradev/delphi/antlr/Delphi.g:529:32: fullFileType
{
pushFollow(FOLLOW_fullFileType_in_synpred96_Delphi4866);
fullFileType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred96_Delphi
// $ANTLR start synpred98_Delphi
public final void synpred98_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:531:32: ( pointerType )
// au/com/integradev/delphi/antlr/Delphi.g:531:32: pointerType
{
pushFollow(FOLLOW_pointerType_in_synpred98_Delphi4932);
pointerType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred98_Delphi
// $ANTLR start synpred99_Delphi
public final void synpred99_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:532:32: ( procedureType )
// au/com/integradev/delphi/antlr/Delphi.g:532:32: procedureType
{
pushFollow(FOLLOW_procedureType_in_synpred99_Delphi4965);
procedureType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred99_Delphi
// $ANTLR start synpred100_Delphi
public final void synpred100_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:533:32: ( subRangeType )
// au/com/integradev/delphi/antlr/Delphi.g:533:32: subRangeType
{
pushFollow(FOLLOW_subRangeType_in_synpred100_Delphi4998);
subRangeType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred100_Delphi
// $ANTLR start synpred101_Delphi
public final void synpred101_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:534:32: ( typeReference )
// au/com/integradev/delphi/antlr/Delphi.g:534:32: typeReference
{
pushFollow(FOLLOW_typeReference_in_synpred101_Delphi5031);
typeReference();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred101_Delphi
// $ANTLR start synpred102_Delphi
public final void synpred102_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:535:32: ( enumType )
// au/com/integradev/delphi/antlr/Delphi.g:535:32: enumType
{
pushFollow(FOLLOW_enumType_in_synpred102_Delphi5064);
enumType();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred102_Delphi
// $ANTLR start synpred114_Delphi
public final void synpred114_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:572:61: ( lbrack expression rbrack )
// au/com/integradev/delphi/antlr/Delphi.g:572:61: lbrack expression rbrack
{
pushFollow(FOLLOW_lbrack_in_synpred114_Delphi6249);
lbrack();
state._fsp--;
if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred114_Delphi6251);
expression();
state._fsp--;
if (state.failed) return;
pushFollow(FOLLOW_rbrack_in_synpred114_Delphi6253);
rbrack();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred114_Delphi
// $ANTLR start synpred115_Delphi
public final void synpred115_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:574:32: ( procedureOfObject )
// au/com/integradev/delphi/antlr/Delphi.g:574:32: procedureOfObject
{
pushFollow(FOLLOW_procedureOfObject_in_synpred115_Delphi6307);
procedureOfObject();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred115_Delphi
// $ANTLR start synpred118_Delphi
public final void synpred118_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:578:96: ( ( ';' )? interfaceDirective )
// au/com/integradev/delphi/antlr/Delphi.g:578:96: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:578:96: ( ';' )?
int alt321=2;
int LA321_0 = input.LA(1);
if ( (LA321_0==SEMICOLON) ) {
alt321=1;
}
switch (alt321) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:578:97: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred118_Delphi6433); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_synpred118_Delphi6437);
interfaceDirective();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred118_Delphi
// $ANTLR start synpred119_Delphi
public final void synpred119_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:584:72: ( routineParameters )
// au/com/integradev/delphi/antlr/Delphi.g:584:72: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_synpred119_Delphi6603);
routineParameters();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred119_Delphi
// $ANTLR start synpred122_Delphi
public final void synpred122_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:584:111: ( ( ';' )? interfaceDirective )
// au/com/integradev/delphi/antlr/Delphi.g:584:111: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:584:111: ( ';' )?
int alt322=2;
int LA322_0 = input.LA(1);
if ( (LA322_0==SEMICOLON) ) {
alt322=1;
}
switch (alt322) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:584:112: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred122_Delphi6611); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_synpred122_Delphi6615);
interfaceDirective();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred122_Delphi
// $ANTLR start synpred124_Delphi
public final void synpred124_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:585:73: ( routineParameters )
// au/com/integradev/delphi/antlr/Delphi.g:585:73: routineParameters
{
pushFollow(FOLLOW_routineParameters_in_synpred124_Delphi6656);
routineParameters();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred124_Delphi
// $ANTLR start synpred126_Delphi
public final void synpred126_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:585:93: ( ( ';' )? interfaceDirective )
// au/com/integradev/delphi/antlr/Delphi.g:585:93: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:585:93: ( ';' )?
int alt327=2;
int LA327_0 = input.LA(1);
if ( (LA327_0==SEMICOLON) ) {
alt327=1;
}
switch (alt327) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:585:94: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred126_Delphi6661); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_synpred126_Delphi6665);
interfaceDirective();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred126_Delphi
// $ANTLR start synpred140_Delphi
public final void synpred140_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:627:43: ( visibilitySectionItem )
// au/com/integradev/delphi/antlr/Delphi.g:627:43: visibilitySectionItem
{
pushFollow(FOLLOW_visibilitySectionItem_in_synpred140_Delphi7840);
visibilitySectionItem();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred140_Delphi
// $ANTLR start synpred142_Delphi
public final void synpred142_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:628:32: ( visibilitySectionItem )
// au/com/integradev/delphi/antlr/Delphi.g:628:32: visibilitySectionItem
{
pushFollow(FOLLOW_visibilitySectionItem_in_synpred142_Delphi7874);
visibilitySectionItem();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred142_Delphi
// $ANTLR start synpred143_Delphi
public final void synpred143_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:630:32: ( fieldSection )
// au/com/integradev/delphi/antlr/Delphi.g:630:32: fieldSection
{
pushFollow(FOLLOW_fieldSection_in_synpred143_Delphi7919);
fieldSection();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred143_Delphi
// $ANTLR start synpred144_Delphi
public final void synpred144_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:631:32: ( routineInterface )
// au/com/integradev/delphi/antlr/Delphi.g:631:32: routineInterface
{
pushFollow(FOLLOW_routineInterface_in_synpred144_Delphi7952);
routineInterface();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred144_Delphi
// $ANTLR start synpred145_Delphi
public final void synpred145_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:632:32: ( methodResolutionClause )
// au/com/integradev/delphi/antlr/Delphi.g:632:32: methodResolutionClause
{
pushFollow(FOLLOW_methodResolutionClause_in_synpred145_Delphi7985);
methodResolutionClause();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred145_Delphi
// $ANTLR start synpred146_Delphi
public final void synpred146_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:633:32: ( property )
// au/com/integradev/delphi/antlr/Delphi.g:633:32: property
{
pushFollow(FOLLOW_property_in_synpred146_Delphi8018);
property();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred146_Delphi
// $ANTLR start synpred150_Delphi
public final void synpred150_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:640:55: ( fieldDecl )
// au/com/integradev/delphi/antlr/Delphi.g:640:55: fieldDecl
{
pushFollow(FOLLOW_fieldDecl_in_synpred150_Delphi8225);
fieldDecl();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred150_Delphi
// $ANTLR start synpred152_Delphi
public final void synpred152_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:641:32: ( fieldDecl )
// au/com/integradev/delphi/antlr/Delphi.g:641:32: fieldDecl
{
pushFollow(FOLLOW_fieldDecl_in_synpred152_Delphi8276);
fieldDecl();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred152_Delphi
// $ANTLR start synpred160_Delphi
public final void synpred160_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:648:122: ( interfaceGuid )
// au/com/integradev/delphi/antlr/Delphi.g:648:122: interfaceGuid
{
pushFollow(FOLLOW_interfaceGuid_in_synpred160_Delphi8549);
interfaceGuid();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred160_Delphi
// $ANTLR start synpred164_Delphi
public final void synpred164_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:654:32: ( routineInterface )
// au/com/integradev/delphi/antlr/Delphi.g:654:32: routineInterface
{
pushFollow(FOLLOW_routineInterface_in_synpred164_Delphi8740);
routineInterface();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred164_Delphi
// $ANTLR start synpred169_Delphi
public final void synpred169_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:659:106: ( ALIGN expression )
// au/com/integradev/delphi/antlr/Delphi.g:659:106: ALIGN expression
{
match(input,ALIGN,FOLLOW_ALIGN_in_synpred169_Delphi8976); if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred169_Delphi8978);
expression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred169_Delphi
// $ANTLR start synpred209_Delphi
public final void synpred209_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:748:32: ( fullRoutineImplementation )
// au/com/integradev/delphi/antlr/Delphi.g:748:32: fullRoutineImplementation
{
pushFollow(FOLLOW_fullRoutineImplementation_in_synpred209_Delphi11627);
fullRoutineImplementation();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred209_Delphi
// $ANTLR start synpred210_Delphi
public final void synpred210_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:749:32: ( externalRoutine )
// au/com/integradev/delphi/antlr/Delphi.g:749:32: externalRoutine
{
pushFollow(FOLLOW_externalRoutine_in_synpred210_Delphi11660);
externalRoutine();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred210_Delphi
// $ANTLR start synpred242_Delphi
public final void synpred242_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:847:32: ( attributeGroup )
// au/com/integradev/delphi/antlr/Delphi.g:847:32: attributeGroup
{
pushFollow(FOLLOW_attributeGroup_in_synpred242_Delphi14863);
attributeGroup();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred242_Delphi
// $ANTLR start synpred245_Delphi
public final void synpred245_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:853:33: ( ASSEMBLY ':' )
// au/com/integradev/delphi/antlr/Delphi.g:853:33: ASSEMBLY ':'
{
match(input,ASSEMBLY,FOLLOW_ASSEMBLY_in_synpred245_Delphi15064); if (state.failed) return;
match(input,COLON,FOLLOW_COLON_in_synpred245_Delphi15066); if (state.failed) return;
}
}
// $ANTLR end synpred245_Delphi
// $ANTLR start synpred250_Delphi
public final void synpred250_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:868:52: ( relationalOperator additiveExpression )
// au/com/integradev/delphi/antlr/Delphi.g:868:52: relationalOperator additiveExpression
{
pushFollow(FOLLOW_relationalOperator_in_synpred250_Delphi15284);
relationalOperator();
state._fsp--;
if (state.failed) return;
pushFollow(FOLLOW_additiveExpression_in_synpred250_Delphi15287);
additiveExpression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred250_Delphi
// $ANTLR start synpred251_Delphi
public final void synpred251_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:870:58: ( addOperator multiplicativeExpression )
// au/com/integradev/delphi/antlr/Delphi.g:870:58: addOperator multiplicativeExpression
{
pushFollow(FOLLOW_addOperator_in_synpred251_Delphi15341);
addOperator();
state._fsp--;
if (state.failed) return;
pushFollow(FOLLOW_multiplicativeExpression_in_synpred251_Delphi15344);
multiplicativeExpression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred251_Delphi
// $ANTLR start synpred254_Delphi
public final void synpred254_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:877:32: ( atom )
// au/com/integradev/delphi/antlr/Delphi.g:877:32: atom
{
pushFollow(FOLLOW_atom_in_synpred254_Delphi15533);
atom();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred254_Delphi
// $ANTLR start synpred255_Delphi
public final void synpred255_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:878:32: ( parenthesizedExpression )
// au/com/integradev/delphi/antlr/Delphi.g:878:32: parenthesizedExpression
{
pushFollow(FOLLOW_parenthesizedExpression_in_synpred255_Delphi15577);
parenthesizedExpression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred255_Delphi
// $ANTLR start synpred256_Delphi
public final void synpred256_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:879:43: ( nameReference )
// au/com/integradev/delphi/antlr/Delphi.g:879:43: nameReference
{
pushFollow(FOLLOW_nameReference_in_synpred256_Delphi15613);
nameReference();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred256_Delphi
// $ANTLR start synpred257_Delphi
public final void synpred257_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:879:58: ( particleItem )
// au/com/integradev/delphi/antlr/Delphi.g:879:58: particleItem
{
pushFollow(FOLLOW_particleItem_in_synpred257_Delphi15616);
particleItem();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred257_Delphi
// $ANTLR start synpred258_Delphi
public final void synpred258_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:879:43: ( ( nameReference )? ( particleItem )* )
// au/com/integradev/delphi/antlr/Delphi.g:879:43: ( nameReference )? ( particleItem )*
{
// au/com/integradev/delphi/antlr/Delphi.g:879:43: ( nameReference )?
int alt341=2;
int LA341_0 = input.LA(1);
if ( ((LA341_0 >= ABSOLUTE && LA341_0 <= ABSTRACT)||LA341_0==ALIGN||(LA341_0 >= ASSEMBLER && LA341_0 <= ASSEMBLY)||(LA341_0 >= AT && LA341_0 <= AUTOMATED)||LA341_0==CDECL||LA341_0==CONTAINS||(LA341_0 >= DEFAULT && LA341_0 <= DEPRECATED)||LA341_0==DISPID||LA341_0==DYNAMIC||(LA341_0 >= EXPERIMENTAL && LA341_0 <= EXPORT)||LA341_0==EXTERNAL||LA341_0==FAR||LA341_0==FINAL||LA341_0==FORWARD||LA341_0==HELPER||LA341_0==IMPLEMENTS||LA341_0==INDEX||LA341_0==LABEL||LA341_0==LOCAL||LA341_0==MESSAGE||(LA341_0 >= NAME && LA341_0 <= NEAR)||LA341_0==NODEFAULT||(LA341_0 >= ON && LA341_0 <= OPERATOR)||(LA341_0 >= OUT && LA341_0 <= OVERRIDE)||LA341_0==PACKAGE||(LA341_0 >= PASCAL && LA341_0 <= PLATFORM)||LA341_0==PRIVATE||(LA341_0 >= PROTECTED && LA341_0 <= PUBLISHED)||(LA341_0 >= READ && LA341_0 <= READONLY)||(LA341_0 >= REFERENCE && LA341_0 <= REINTRODUCE)||(LA341_0 >= REQUIRES && LA341_0 <= RESIDENT)||(LA341_0 >= SAFECALL && LA341_0 <= SEALED)||(LA341_0 >= STATIC && LA341_0 <= STRICT)||LA341_0==TkIdentifier||LA341_0==UNSAFE||(LA341_0 >= VARARGS && LA341_0 <= VIRTUAL)||LA341_0==WINAPI||(LA341_0 >= WRITE && LA341_0 <= WRITEONLY)) ) {
alt341=1;
}
switch (alt341) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:879:43: nameReference
{
pushFollow(FOLLOW_nameReference_in_synpred258_Delphi15613);
nameReference();
state._fsp--;
if (state.failed) return;
}
break;
}
// au/com/integradev/delphi/antlr/Delphi.g:879:58: ( particleItem )*
loop342:
while (true) {
int alt342=2;
int LA342_0 = input.LA(1);
if ( (LA342_0==DEREFERENCE||LA342_0==DOT||LA342_0==PAREN_BRACKET_LEFT||LA342_0==PAREN_LEFT||LA342_0==SQUARE_BRACKET_LEFT) ) {
alt342=1;
}
switch (alt342) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:879:58: particleItem
{
pushFollow(FOLLOW_particleItem_in_synpred258_Delphi15616);
particleItem();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop342;
}
}
}
}
// $ANTLR end synpred258_Delphi
// $ANTLR start synpred259_Delphi
public final void synpred259_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:883:41: ( particleItem )
// au/com/integradev/delphi/antlr/Delphi.g:883:41: particleItem
{
pushFollow(FOLLOW_particleItem_in_synpred259_Delphi15763);
particleItem();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred259_Delphi
// $ANTLR start synpred268_Delphi
public final void synpred268_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:893:56: ( particleItem )
// au/com/integradev/delphi/antlr/Delphi.g:893:56: particleItem
{
pushFollow(FOLLOW_particleItem_in_synpred268_Delphi16087);
particleItem();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred268_Delphi
// $ANTLR start synpred276_Delphi
public final void synpred276_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:910:32: ( ident ':=' )
// au/com/integradev/delphi/antlr/Delphi.g:910:32: ident ':='
{
pushFollow(FOLLOW_ident_in_synpred276_Delphi16633);
ident();
state._fsp--;
if (state.failed) return;
match(input,ASSIGN,FOLLOW_ASSIGN_in_synpred276_Delphi16635); if (state.failed) return;
}
}
// $ANTLR end synpred276_Delphi
// $ANTLR start synpred293_Delphi
public final void synpred293_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:933:48: ( ( escapedCharacter )+ TkQuotedString )
// au/com/integradev/delphi/antlr/Delphi.g:933:48: ( escapedCharacter )+ TkQuotedString
{
// au/com/integradev/delphi/antlr/Delphi.g:933:48: ( escapedCharacter )+
int cnt352=0;
loop352:
while (true) {
int alt352=2;
int LA352_0 = input.LA(1);
if ( (LA352_0==DEREFERENCE||LA352_0==TkCharacterEscapeCode) ) {
alt352=1;
}
switch (alt352) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:933:48: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_synpred293_Delphi17395);
escapedCharacter();
state._fsp--;
if (state.failed) return;
}
break;
default :
if ( cnt352 >= 1 ) break loop352;
if (state.backtracking>0) {state.failed=true; return;}
EarlyExitException eee = new EarlyExitException(352, input);
throw eee;
}
cnt352++;
}
match(input,TkQuotedString,FOLLOW_TkQuotedString_in_synpred293_Delphi17398); if (state.failed) return;
}
}
// $ANTLR end synpred293_Delphi
// $ANTLR start synpred294_Delphi
public final void synpred294_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:933:83: ( escapedCharacter )
// au/com/integradev/delphi/antlr/Delphi.g:933:83: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_synpred294_Delphi17402);
escapedCharacter();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred294_Delphi
// $ANTLR start synpred296_Delphi
public final void synpred296_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:934:32: ( escapedCharacter )
// au/com/integradev/delphi/antlr/Delphi.g:934:32: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_synpred296_Delphi17436);
escapedCharacter();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred296_Delphi
// $ANTLR start synpred297_Delphi
public final void synpred297_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:934:66: ( escapedCharacter )
// au/com/integradev/delphi/antlr/Delphi.g:934:66: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_synpred297_Delphi17442);
escapedCharacter();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred297_Delphi
// $ANTLR start synpred298_Delphi
public final void synpred298_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:934:51: ( TkQuotedString ( escapedCharacter )+ )
// au/com/integradev/delphi/antlr/Delphi.g:934:51: TkQuotedString ( escapedCharacter )+
{
match(input,TkQuotedString,FOLLOW_TkQuotedString_in_synpred298_Delphi17440); if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:934:66: ( escapedCharacter )+
int cnt356=0;
loop356:
while (true) {
int alt356=2;
int LA356_0 = input.LA(1);
if ( (LA356_0==DEREFERENCE||LA356_0==TkCharacterEscapeCode) ) {
alt356=1;
}
switch (alt356) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:934:66: escapedCharacter
{
pushFollow(FOLLOW_escapedCharacter_in_synpred298_Delphi17442);
escapedCharacter();
state._fsp--;
if (state.failed) return;
}
break;
default :
if ( cnt356 >= 1 ) break loop356;
if (state.backtracking>0) {state.failed=true; return;}
EarlyExitException eee = new EarlyExitException(356, input);
throw eee;
}
cnt356++;
}
}
}
// $ANTLR end synpred298_Delphi
// $ANTLR start synpred299_Delphi
public final void synpred299_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:934:86: ( TkQuotedString )
// au/com/integradev/delphi/antlr/Delphi.g:934:86: TkQuotedString
{
match(input,TkQuotedString,FOLLOW_TkQuotedString_in_synpred299_Delphi17447); if (state.failed) return;
}
}
// $ANTLR end synpred299_Delphi
// $ANTLR start synpred324_Delphi
public final void synpred324_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:974:32: ( expression )
// au/com/integradev/delphi/antlr/Delphi.g:974:32: expression
{
pushFollow(FOLLOW_expression_in_synpred324_Delphi18738);
expression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred324_Delphi
// $ANTLR start synpred325_Delphi
public final void synpred325_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:975:32: ( recordExpression )
// au/com/integradev/delphi/antlr/Delphi.g:975:32: recordExpression
{
pushFollow(FOLLOW_recordExpression_in_synpred325_Delphi18771);
recordExpression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred325_Delphi
// $ANTLR start synpred342_Delphi
public final void synpred342_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1001:32: ( labelStatement )
// au/com/integradev/delphi/antlr/Delphi.g:1001:32: labelStatement
{
pushFollow(FOLLOW_labelStatement_in_synpred342_Delphi19483);
labelStatement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred342_Delphi
// $ANTLR start synpred343_Delphi
public final void synpred343_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1002:32: ( assignmentStatement )
// au/com/integradev/delphi/antlr/Delphi.g:1002:32: assignmentStatement
{
pushFollow(FOLLOW_assignmentStatement_in_synpred343_Delphi19516);
assignmentStatement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred343_Delphi
// $ANTLR start synpred344_Delphi
public final void synpred344_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1003:32: ( expressionStatement )
// au/com/integradev/delphi/antlr/Delphi.g:1003:32: expressionStatement
{
pushFollow(FOLLOW_expressionStatement_in_synpred344_Delphi19549);
expressionStatement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred344_Delphi
// $ANTLR start synpred345_Delphi
public final void synpred345_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1006:73: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1006:73: statement
{
pushFollow(FOLLOW_statement_in_synpred345_Delphi19646);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred345_Delphi
// $ANTLR start synpred346_Delphi
public final void synpred346_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1006:90: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1006:90: statement
{
pushFollow(FOLLOW_statement_in_synpred346_Delphi19652);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred346_Delphi
// $ANTLR start synpred347_Delphi
public final void synpred347_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1006:85: ( ELSE ( statement )? )
// au/com/integradev/delphi/antlr/Delphi.g:1006:85: ELSE ( statement )?
{
match(input,ELSE,FOLLOW_ELSE_in_synpred347_Delphi19650); if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:1006:90: ( statement )?
int alt359=2;
int LA359_0 = input.LA(1);
if ( ((LA359_0 >= ABSOLUTE && LA359_0 <= ALIGN)||(LA359_0 >= ASM && LA359_0 <= ASSEMBLY)||(LA359_0 >= AT && LA359_0 <= AUTOMATED)||LA359_0==BEGIN||(LA359_0 >= CASE && LA359_0 <= CDECL)||LA359_0==CONST||LA359_0==CONTAINS||(LA359_0 >= DEFAULT && LA359_0 <= DEREFERENCE)||LA359_0==DISPID||LA359_0==DYNAMIC||(LA359_0 >= EXPERIMENTAL && LA359_0 <= EXPORT)||LA359_0==EXTERNAL||(LA359_0 >= FAR && LA359_0 <= FINAL)||(LA359_0 >= FOR && LA359_0 <= FUNCTION)||LA359_0==GOTO||LA359_0==HELPER||LA359_0==IF||LA359_0==IMPLEMENTS||(LA359_0 >= INDEX && LA359_0 <= INHERITED)||LA359_0==LABEL||LA359_0==LOCAL||(LA359_0 >= MESSAGE && LA359_0 <= MINUS)||(LA359_0 >= NAME && LA359_0 <= NOT)||(LA359_0 >= ON && LA359_0 <= OPERATOR)||(LA359_0 >= OUT && LA359_0 <= OVERRIDE)||LA359_0==PACKAGE||LA359_0==PAREN_BRACKET_LEFT||LA359_0==PAREN_LEFT||(LA359_0 >= PASCAL && LA359_0 <= PROCEDURE)||(LA359_0 >= PROTECTED && LA359_0 <= PUBLISHED)||(LA359_0 >= RAISE && LA359_0 <= READONLY)||(LA359_0 >= REFERENCE && LA359_0 <= RESIDENT)||(LA359_0 >= SAFECALL && LA359_0 <= SEALED)||LA359_0==SQUARE_BRACKET_LEFT||(LA359_0 >= STATIC && LA359_0 <= STRING)||LA359_0==TRY||LA359_0==TkBinaryNumber||LA359_0==TkCharacterEscapeCode||(LA359_0 >= TkHexNumber && LA359_0 <= TkIntNumber)||LA359_0==TkMultilineString||(LA359_0 >= TkQuotedString && LA359_0 <= TkRealNumber)||LA359_0==UNSAFE||(LA359_0 >= VAR && LA359_0 <= VIRTUAL)||LA359_0==WHILE||(LA359_0 >= WINAPI && LA359_0 <= WRITEONLY)) ) {
alt359=1;
}
switch (alt359) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1006:90: statement
{
pushFollow(FOLLOW_statement_in_synpred347_Delphi19652);
statement();
state._fsp--;
if (state.failed) return;
}
break;
}
}
}
// $ANTLR end synpred347_Delphi
// $ANTLR start synpred355_Delphi
public final void synpred355_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1018:59: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1018:59: statement
{
pushFollow(FOLLOW_statement_in_synpred355_Delphi20097);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred355_Delphi
// $ANTLR start synpred357_Delphi
public final void synpred357_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1022:77: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1022:77: statement
{
pushFollow(FOLLOW_statement_in_synpred357_Delphi20247);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred357_Delphi
// $ANTLR start synpred358_Delphi
public final void synpred358_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1024:101: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1024:101: statement
{
pushFollow(FOLLOW_statement_in_synpred358_Delphi20319);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred358_Delphi
// $ANTLR start synpred359_Delphi
public final void synpred359_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1024:32: ( FOR forVar ':=' expression TO expression DO ( statement )? )
// au/com/integradev/delphi/antlr/Delphi.g:1024:32: FOR forVar ':=' expression TO expression DO ( statement )?
{
match(input,FOR,FOLLOW_FOR_in_synpred359_Delphi20301); if (state.failed) return;
pushFollow(FOLLOW_forVar_in_synpred359_Delphi20307);
forVar();
state._fsp--;
if (state.failed) return;
match(input,ASSIGN,FOLLOW_ASSIGN_in_synpred359_Delphi20309); if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred359_Delphi20311);
expression();
state._fsp--;
if (state.failed) return;
match(input,TO,FOLLOW_TO_in_synpred359_Delphi20313); if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred359_Delphi20315);
expression();
state._fsp--;
if (state.failed) return;
match(input,DO,FOLLOW_DO_in_synpred359_Delphi20317); if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:1024:101: ( statement )?
int alt360=2;
int LA360_0 = input.LA(1);
if ( ((LA360_0 >= ABSOLUTE && LA360_0 <= ALIGN)||(LA360_0 >= ASM && LA360_0 <= ASSEMBLY)||(LA360_0 >= AT && LA360_0 <= AUTOMATED)||LA360_0==BEGIN||(LA360_0 >= CASE && LA360_0 <= CDECL)||LA360_0==CONST||LA360_0==CONTAINS||(LA360_0 >= DEFAULT && LA360_0 <= DEREFERENCE)||LA360_0==DISPID||LA360_0==DYNAMIC||(LA360_0 >= EXPERIMENTAL && LA360_0 <= EXPORT)||LA360_0==EXTERNAL||(LA360_0 >= FAR && LA360_0 <= FINAL)||(LA360_0 >= FOR && LA360_0 <= FUNCTION)||LA360_0==GOTO||LA360_0==HELPER||LA360_0==IF||LA360_0==IMPLEMENTS||(LA360_0 >= INDEX && LA360_0 <= INHERITED)||LA360_0==LABEL||LA360_0==LOCAL||(LA360_0 >= MESSAGE && LA360_0 <= MINUS)||(LA360_0 >= NAME && LA360_0 <= NOT)||(LA360_0 >= ON && LA360_0 <= OPERATOR)||(LA360_0 >= OUT && LA360_0 <= OVERRIDE)||LA360_0==PACKAGE||LA360_0==PAREN_BRACKET_LEFT||LA360_0==PAREN_LEFT||(LA360_0 >= PASCAL && LA360_0 <= PROCEDURE)||(LA360_0 >= PROTECTED && LA360_0 <= PUBLISHED)||(LA360_0 >= RAISE && LA360_0 <= READONLY)||(LA360_0 >= REFERENCE && LA360_0 <= RESIDENT)||(LA360_0 >= SAFECALL && LA360_0 <= SEALED)||LA360_0==SQUARE_BRACKET_LEFT||(LA360_0 >= STATIC && LA360_0 <= STRING)||LA360_0==TRY||LA360_0==TkBinaryNumber||LA360_0==TkCharacterEscapeCode||(LA360_0 >= TkHexNumber && LA360_0 <= TkIntNumber)||LA360_0==TkMultilineString||(LA360_0 >= TkQuotedString && LA360_0 <= TkRealNumber)||LA360_0==UNSAFE||(LA360_0 >= VAR && LA360_0 <= VIRTUAL)||LA360_0==WHILE||(LA360_0 >= WINAPI && LA360_0 <= WRITEONLY)) ) {
alt360=1;
}
switch (alt360) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1024:101: statement
{
pushFollow(FOLLOW_statement_in_synpred359_Delphi20319);
statement();
state._fsp--;
if (state.failed) return;
}
break;
}
}
}
// $ANTLR end synpred359_Delphi
// $ANTLR start synpred360_Delphi
public final void synpred360_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1025:105: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1025:105: statement
{
pushFollow(FOLLOW_statement_in_synpred360_Delphi20371);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred360_Delphi
// $ANTLR start synpred361_Delphi
public final void synpred361_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1025:32: ( FOR forVar ':=' expression DOWNTO expression DO ( statement )? )
// au/com/integradev/delphi/antlr/Delphi.g:1025:32: FOR forVar ':=' expression DOWNTO expression DO ( statement )?
{
match(input,FOR,FOLLOW_FOR_in_synpred361_Delphi20353); if (state.failed) return;
pushFollow(FOLLOW_forVar_in_synpred361_Delphi20359);
forVar();
state._fsp--;
if (state.failed) return;
match(input,ASSIGN,FOLLOW_ASSIGN_in_synpred361_Delphi20361); if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred361_Delphi20363);
expression();
state._fsp--;
if (state.failed) return;
match(input,DOWNTO,FOLLOW_DOWNTO_in_synpred361_Delphi20365); if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred361_Delphi20367);
expression();
state._fsp--;
if (state.failed) return;
match(input,DO,FOLLOW_DO_in_synpred361_Delphi20369); if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:1025:105: ( statement )?
int alt361=2;
int LA361_0 = input.LA(1);
if ( ((LA361_0 >= ABSOLUTE && LA361_0 <= ALIGN)||(LA361_0 >= ASM && LA361_0 <= ASSEMBLY)||(LA361_0 >= AT && LA361_0 <= AUTOMATED)||LA361_0==BEGIN||(LA361_0 >= CASE && LA361_0 <= CDECL)||LA361_0==CONST||LA361_0==CONTAINS||(LA361_0 >= DEFAULT && LA361_0 <= DEREFERENCE)||LA361_0==DISPID||LA361_0==DYNAMIC||(LA361_0 >= EXPERIMENTAL && LA361_0 <= EXPORT)||LA361_0==EXTERNAL||(LA361_0 >= FAR && LA361_0 <= FINAL)||(LA361_0 >= FOR && LA361_0 <= FUNCTION)||LA361_0==GOTO||LA361_0==HELPER||LA361_0==IF||LA361_0==IMPLEMENTS||(LA361_0 >= INDEX && LA361_0 <= INHERITED)||LA361_0==LABEL||LA361_0==LOCAL||(LA361_0 >= MESSAGE && LA361_0 <= MINUS)||(LA361_0 >= NAME && LA361_0 <= NOT)||(LA361_0 >= ON && LA361_0 <= OPERATOR)||(LA361_0 >= OUT && LA361_0 <= OVERRIDE)||LA361_0==PACKAGE||LA361_0==PAREN_BRACKET_LEFT||LA361_0==PAREN_LEFT||(LA361_0 >= PASCAL && LA361_0 <= PROCEDURE)||(LA361_0 >= PROTECTED && LA361_0 <= PUBLISHED)||(LA361_0 >= RAISE && LA361_0 <= READONLY)||(LA361_0 >= REFERENCE && LA361_0 <= RESIDENT)||(LA361_0 >= SAFECALL && LA361_0 <= SEALED)||LA361_0==SQUARE_BRACKET_LEFT||(LA361_0 >= STATIC && LA361_0 <= STRING)||LA361_0==TRY||LA361_0==TkBinaryNumber||LA361_0==TkCharacterEscapeCode||(LA361_0 >= TkHexNumber && LA361_0 <= TkIntNumber)||LA361_0==TkMultilineString||(LA361_0 >= TkQuotedString && LA361_0 <= TkRealNumber)||LA361_0==UNSAFE||(LA361_0 >= VAR && LA361_0 <= VIRTUAL)||LA361_0==WHILE||(LA361_0 >= WINAPI && LA361_0 <= WRITEONLY)) ) {
alt361=1;
}
switch (alt361) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1025:105: statement
{
pushFollow(FOLLOW_statement_in_synpred361_Delphi20371);
statement();
state._fsp--;
if (state.failed) return;
}
break;
}
}
}
// $ANTLR end synpred361_Delphi
// $ANTLR start synpred362_Delphi
public final void synpred362_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1026:85: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1026:85: statement
{
pushFollow(FOLLOW_statement_in_synpred362_Delphi20419);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred362_Delphi
// $ANTLR start synpred365_Delphi
public final void synpred365_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1031:79: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1031:79: statement
{
pushFollow(FOLLOW_statement_in_synpred365_Delphi20608);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred365_Delphi
// $ANTLR start synpred369_Delphi
public final void synpred369_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1039:82: ( statement )
// au/com/integradev/delphi/antlr/Delphi.g:1039:82: statement
{
pushFollow(FOLLOW_statement_in_synpred369_Delphi20842);
statement();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred369_Delphi
// $ANTLR start synpred373_Delphi
public final void synpred373_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: ( ( handler )+ ( elseBlock )? )
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: ( handler )+ ( elseBlock )?
{
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: ( handler )+
int cnt363=0;
loop363:
while (true) {
int alt363=2;
int LA363_0 = input.LA(1);
if ( (LA363_0==ON) ) {
alt363=1;
}
switch (alt363) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1054:32: handler
{
pushFollow(FOLLOW_handler_in_synpred373_Delphi21299);
handler();
state._fsp--;
if (state.failed) return;
}
break;
default :
if ( cnt363 >= 1 ) break loop363;
if (state.backtracking>0) {state.failed=true; return;}
EarlyExitException eee = new EarlyExitException(363, input);
throw eee;
}
cnt363++;
}
// au/com/integradev/delphi/antlr/Delphi.g:1054:41: ( elseBlock )?
int alt364=2;
int LA364_0 = input.LA(1);
if ( (LA364_0==ELSE) ) {
alt364=1;
}
switch (alt364) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1054:41: elseBlock
{
pushFollow(FOLLOW_elseBlock_in_synpred373_Delphi21302);
elseBlock();
state._fsp--;
if (state.failed) return;
}
break;
}
}
}
// $ANTLR end synpred373_Delphi
// $ANTLR start synpred377_Delphi
public final void synpred377_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1059:63: ( expression )
// au/com/integradev/delphi/antlr/Delphi.g:1059:63: expression
{
pushFollow(FOLLOW_expression_in_synpred377_Delphi21475);
expression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred377_Delphi
// $ANTLR start synpred378_Delphi
public final void synpred378_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1059:76: ( AT expression )
// au/com/integradev/delphi/antlr/Delphi.g:1059:76: AT expression
{
match(input,AT,FOLLOW_AT_in_synpred378_Delphi21479); if (state.failed) return;
pushFollow(FOLLOW_expression_in_synpred378_Delphi21482);
expression();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred378_Delphi
// $ANTLR start synpred383_Delphi
public final void synpred383_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1069:32: ( ( ( ';' )? implDirective )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:1069:32: ( ( ';' )? implDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:1069:32: ( ( ';' )? implDirective )*
loop367:
while (true) {
int alt367=2;
int LA367_0 = input.LA(1);
if ( (LA367_0==SEMICOLON) ) {
int LA367_1 = input.LA(2);
if ( (LA367_1==ABSTRACT||LA367_1==ASSEMBLER||LA367_1==CDECL||LA367_1==DEPRECATED||LA367_1==DISPID||LA367_1==DYNAMIC||(LA367_1 >= EXPERIMENTAL && LA367_1 <= EXPORT)||LA367_1==FAR||LA367_1==FINAL||LA367_1==INLINE||(LA367_1 >= LIBRARY && LA367_1 <= LOCAL)||LA367_1==MESSAGE||LA367_1==NEAR||(LA367_1 >= OVERLOAD && LA367_1 <= OVERRIDE)||(LA367_1 >= PASCAL && LA367_1 <= PLATFORM)||(LA367_1 >= REGISTER && LA367_1 <= REINTRODUCE)||LA367_1==SAFECALL||(LA367_1 >= STATIC && LA367_1 <= STDCALL)||LA367_1==UNSAFE||(LA367_1 >= VARARGS && LA367_1 <= VIRTUAL)||LA367_1==WINAPI) ) {
alt367=1;
}
}
else if ( (LA367_0==ABSTRACT||LA367_0==ASSEMBLER||LA367_0==CDECL||LA367_0==DEPRECATED||LA367_0==DISPID||LA367_0==DYNAMIC||(LA367_0 >= EXPERIMENTAL && LA367_0 <= EXPORT)||LA367_0==FAR||LA367_0==FINAL||LA367_0==INLINE||(LA367_0 >= LIBRARY && LA367_0 <= LOCAL)||LA367_0==MESSAGE||LA367_0==NEAR||(LA367_0 >= OVERLOAD && LA367_0 <= OVERRIDE)||(LA367_0 >= PASCAL && LA367_0 <= PLATFORM)||(LA367_0 >= REGISTER && LA367_0 <= REINTRODUCE)||LA367_0==SAFECALL||(LA367_0 >= STATIC && LA367_0 <= STDCALL)||LA367_0==UNSAFE||(LA367_0 >= VARARGS && LA367_0 <= VIRTUAL)||LA367_0==WINAPI) ) {
alt367=1;
}
switch (alt367) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1069:33: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1069:33: ( ';' )?
int alt366=2;
int LA366_0 = input.LA(1);
if ( (LA366_0==SEMICOLON) ) {
alt366=1;
}
switch (alt366) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1069:33: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred383_Delphi21638); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_implDirective_in_synpred383_Delphi21641);
implDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop367;
}
}
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred383_Delphi21645); if (state.failed) return;
}
}
// $ANTLR end synpred383_Delphi
// $ANTLR start synpred387_Delphi
public final void synpred387_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1072:32: ( ( ( ';' )? interfaceDirective )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:1072:32: ( ( ';' )? interfaceDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:1072:32: ( ( ';' )? interfaceDirective )*
loop370:
while (true) {
int alt370=2;
int LA370_0 = input.LA(1);
if ( (LA370_0==SEMICOLON) ) {
int LA370_1 = input.LA(2);
if ( (LA370_1==ABSTRACT||LA370_1==ASSEMBLER||LA370_1==CDECL||LA370_1==DEPRECATED||LA370_1==DISPID||LA370_1==DYNAMIC||(LA370_1 >= EXPERIMENTAL && LA370_1 <= EXPORT)||LA370_1==EXTERNAL||LA370_1==FAR||LA370_1==FINAL||LA370_1==FORWARD||LA370_1==INLINE||(LA370_1 >= LIBRARY && LA370_1 <= LOCAL)||LA370_1==MESSAGE||LA370_1==NEAR||(LA370_1 >= OVERLOAD && LA370_1 <= OVERRIDE)||(LA370_1 >= PASCAL && LA370_1 <= PLATFORM)||(LA370_1 >= REGISTER && LA370_1 <= REINTRODUCE)||LA370_1==SAFECALL||(LA370_1 >= STATIC && LA370_1 <= STDCALL)||LA370_1==UNSAFE||(LA370_1 >= VARARGS && LA370_1 <= VIRTUAL)||LA370_1==WINAPI) ) {
alt370=1;
}
}
else if ( (LA370_0==ABSTRACT||LA370_0==ASSEMBLER||LA370_0==CDECL||LA370_0==DEPRECATED||LA370_0==DISPID||LA370_0==DYNAMIC||(LA370_0 >= EXPERIMENTAL && LA370_0 <= EXPORT)||LA370_0==EXTERNAL||LA370_0==FAR||LA370_0==FINAL||LA370_0==FORWARD||LA370_0==INLINE||(LA370_0 >= LIBRARY && LA370_0 <= LOCAL)||LA370_0==MESSAGE||LA370_0==NEAR||(LA370_0 >= OVERLOAD && LA370_0 <= OVERRIDE)||(LA370_0 >= PASCAL && LA370_0 <= PLATFORM)||(LA370_0 >= REGISTER && LA370_0 <= REINTRODUCE)||LA370_0==SAFECALL||(LA370_0 >= STATIC && LA370_0 <= STDCALL)||LA370_0==UNSAFE||(LA370_0 >= VARARGS && LA370_0 <= VIRTUAL)||LA370_0==WINAPI) ) {
alt370=1;
}
switch (alt370) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1072:33: ( ';' )? interfaceDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1072:33: ( ';' )?
int alt369=2;
int LA369_0 = input.LA(1);
if ( (LA369_0==SEMICOLON) ) {
alt369=1;
}
switch (alt369) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1072:33: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred387_Delphi21724); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_interfaceDirective_in_synpred387_Delphi21727);
interfaceDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop370;
}
}
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred387_Delphi21731); if (state.failed) return;
}
}
// $ANTLR end synpred387_Delphi
// $ANTLR start synpred394_Delphi
public final void synpred394_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1075:33: ( ( ( ';' )? implDirective )* ( ';' )? externalDirective ( ( ';' )? implDirective )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:1075:33: ( ( ';' )? implDirective )* ( ';' )? externalDirective ( ( ';' )? implDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:1075:33: ( ( ';' )? implDirective )*
loop374:
while (true) {
int alt374=2;
int LA374_0 = input.LA(1);
if ( (LA374_0==SEMICOLON) ) {
int LA374_1 = input.LA(2);
if ( (LA374_1==ABSTRACT||LA374_1==ASSEMBLER||LA374_1==CDECL||LA374_1==DEPRECATED||LA374_1==DISPID||LA374_1==DYNAMIC||(LA374_1 >= EXPERIMENTAL && LA374_1 <= EXPORT)||LA374_1==FAR||LA374_1==FINAL||LA374_1==INLINE||(LA374_1 >= LIBRARY && LA374_1 <= LOCAL)||LA374_1==MESSAGE||LA374_1==NEAR||(LA374_1 >= OVERLOAD && LA374_1 <= OVERRIDE)||(LA374_1 >= PASCAL && LA374_1 <= PLATFORM)||(LA374_1 >= REGISTER && LA374_1 <= REINTRODUCE)||LA374_1==SAFECALL||(LA374_1 >= STATIC && LA374_1 <= STDCALL)||LA374_1==UNSAFE||(LA374_1 >= VARARGS && LA374_1 <= VIRTUAL)||LA374_1==WINAPI) ) {
alt374=1;
}
}
else if ( (LA374_0==ABSTRACT||LA374_0==ASSEMBLER||LA374_0==CDECL||LA374_0==DEPRECATED||LA374_0==DISPID||LA374_0==DYNAMIC||(LA374_0 >= EXPERIMENTAL && LA374_0 <= EXPORT)||LA374_0==FAR||LA374_0==FINAL||LA374_0==INLINE||(LA374_0 >= LIBRARY && LA374_0 <= LOCAL)||LA374_0==MESSAGE||LA374_0==NEAR||(LA374_0 >= OVERLOAD && LA374_0 <= OVERRIDE)||(LA374_0 >= PASCAL && LA374_0 <= PLATFORM)||(LA374_0 >= REGISTER && LA374_0 <= REINTRODUCE)||LA374_0==SAFECALL||(LA374_0 >= STATIC && LA374_0 <= STDCALL)||LA374_0==UNSAFE||(LA374_0 >= VARARGS && LA374_0 <= VIRTUAL)||LA374_0==WINAPI) ) {
alt374=1;
}
switch (alt374) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:34: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1075:34: ( ';' )?
int alt373=2;
int LA373_0 = input.LA(1);
if ( (LA373_0==SEMICOLON) ) {
alt373=1;
}
switch (alt373) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:34: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred394_Delphi21812); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_implDirective_in_synpred394_Delphi21815);
implDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop374;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:1075:55: ( ';' )?
int alt375=2;
int LA375_0 = input.LA(1);
if ( (LA375_0==SEMICOLON) ) {
alt375=1;
}
switch (alt375) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:55: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred394_Delphi21819); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_externalDirective_in_synpred394_Delphi21822);
externalDirective();
state._fsp--;
if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:1075:78: ( ( ';' )? implDirective )*
loop377:
while (true) {
int alt377=2;
int LA377_0 = input.LA(1);
if ( (LA377_0==SEMICOLON) ) {
int LA377_1 = input.LA(2);
if ( (LA377_1==ABSTRACT||LA377_1==ASSEMBLER||LA377_1==CDECL||LA377_1==DEPRECATED||LA377_1==DISPID||LA377_1==DYNAMIC||(LA377_1 >= EXPERIMENTAL && LA377_1 <= EXPORT)||LA377_1==FAR||LA377_1==FINAL||LA377_1==INLINE||(LA377_1 >= LIBRARY && LA377_1 <= LOCAL)||LA377_1==MESSAGE||LA377_1==NEAR||(LA377_1 >= OVERLOAD && LA377_1 <= OVERRIDE)||(LA377_1 >= PASCAL && LA377_1 <= PLATFORM)||(LA377_1 >= REGISTER && LA377_1 <= REINTRODUCE)||LA377_1==SAFECALL||(LA377_1 >= STATIC && LA377_1 <= STDCALL)||LA377_1==UNSAFE||(LA377_1 >= VARARGS && LA377_1 <= VIRTUAL)||LA377_1==WINAPI) ) {
alt377=1;
}
}
else if ( (LA377_0==ABSTRACT||LA377_0==ASSEMBLER||LA377_0==CDECL||LA377_0==DEPRECATED||LA377_0==DISPID||LA377_0==DYNAMIC||(LA377_0 >= EXPERIMENTAL && LA377_0 <= EXPORT)||LA377_0==FAR||LA377_0==FINAL||LA377_0==INLINE||(LA377_0 >= LIBRARY && LA377_0 <= LOCAL)||LA377_0==MESSAGE||LA377_0==NEAR||(LA377_0 >= OVERLOAD && LA377_0 <= OVERRIDE)||(LA377_0 >= PASCAL && LA377_0 <= PLATFORM)||(LA377_0 >= REGISTER && LA377_0 <= REINTRODUCE)||LA377_0==SAFECALL||(LA377_0 >= STATIC && LA377_0 <= STDCALL)||LA377_0==UNSAFE||(LA377_0 >= VARARGS && LA377_0 <= VIRTUAL)||LA377_0==WINAPI) ) {
alt377=1;
}
switch (alt377) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:79: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1075:79: ( ';' )?
int alt376=2;
int LA376_0 = input.LA(1);
if ( (LA376_0==SEMICOLON) ) {
alt376=1;
}
switch (alt376) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1075:79: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred394_Delphi21825); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_implDirective_in_synpred394_Delphi21828);
implDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop377;
}
}
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred394_Delphi21831); if (state.failed) return;
}
}
// $ANTLR end synpred394_Delphi
// $ANTLR start synpred402_Delphi
public final void synpred402_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1078:32: ( ( ( ';' )? implDirective )* ( ';' )? FORWARD ( ( ';' )? implDirective )* ';' )
// au/com/integradev/delphi/antlr/Delphi.g:1078:32: ( ( ';' )? implDirective )* ( ';' )? FORWARD ( ( ';' )? implDirective )* ';'
{
// au/com/integradev/delphi/antlr/Delphi.g:1078:32: ( ( ';' )? implDirective )*
loop381:
while (true) {
int alt381=2;
int LA381_0 = input.LA(1);
if ( (LA381_0==SEMICOLON) ) {
int LA381_1 = input.LA(2);
if ( (LA381_1==ABSTRACT||LA381_1==ASSEMBLER||LA381_1==CDECL||LA381_1==DEPRECATED||LA381_1==DISPID||LA381_1==DYNAMIC||(LA381_1 >= EXPERIMENTAL && LA381_1 <= EXPORT)||LA381_1==FAR||LA381_1==FINAL||LA381_1==INLINE||(LA381_1 >= LIBRARY && LA381_1 <= LOCAL)||LA381_1==MESSAGE||LA381_1==NEAR||(LA381_1 >= OVERLOAD && LA381_1 <= OVERRIDE)||(LA381_1 >= PASCAL && LA381_1 <= PLATFORM)||(LA381_1 >= REGISTER && LA381_1 <= REINTRODUCE)||LA381_1==SAFECALL||(LA381_1 >= STATIC && LA381_1 <= STDCALL)||LA381_1==UNSAFE||(LA381_1 >= VARARGS && LA381_1 <= VIRTUAL)||LA381_1==WINAPI) ) {
alt381=1;
}
}
else if ( (LA381_0==ABSTRACT||LA381_0==ASSEMBLER||LA381_0==CDECL||LA381_0==DEPRECATED||LA381_0==DISPID||LA381_0==DYNAMIC||(LA381_0 >= EXPERIMENTAL && LA381_0 <= EXPORT)||LA381_0==FAR||LA381_0==FINAL||LA381_0==INLINE||(LA381_0 >= LIBRARY && LA381_0 <= LOCAL)||LA381_0==MESSAGE||LA381_0==NEAR||(LA381_0 >= OVERLOAD && LA381_0 <= OVERRIDE)||(LA381_0 >= PASCAL && LA381_0 <= PLATFORM)||(LA381_0 >= REGISTER && LA381_0 <= REINTRODUCE)||LA381_0==SAFECALL||(LA381_0 >= STATIC && LA381_0 <= STDCALL)||LA381_0==UNSAFE||(LA381_0 >= VARARGS && LA381_0 <= VIRTUAL)||LA381_0==WINAPI) ) {
alt381=1;
}
switch (alt381) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:33: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1078:33: ( ';' )?
int alt380=2;
int LA380_0 = input.LA(1);
if ( (LA380_0==SEMICOLON) ) {
alt380=1;
}
switch (alt380) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:33: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred402_Delphi21923); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_implDirective_in_synpred402_Delphi21926);
implDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop381;
}
}
// au/com/integradev/delphi/antlr/Delphi.g:1078:54: ( ';' )?
int alt382=2;
int LA382_0 = input.LA(1);
if ( (LA382_0==SEMICOLON) ) {
alt382=1;
}
switch (alt382) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:54: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred402_Delphi21930); if (state.failed) return;
}
break;
}
match(input,FORWARD,FOLLOW_FORWARD_in_synpred402_Delphi21933); if (state.failed) return;
// au/com/integradev/delphi/antlr/Delphi.g:1078:67: ( ( ';' )? implDirective )*
loop384:
while (true) {
int alt384=2;
int LA384_0 = input.LA(1);
if ( (LA384_0==SEMICOLON) ) {
int LA384_1 = input.LA(2);
if ( (LA384_1==ABSTRACT||LA384_1==ASSEMBLER||LA384_1==CDECL||LA384_1==DEPRECATED||LA384_1==DISPID||LA384_1==DYNAMIC||(LA384_1 >= EXPERIMENTAL && LA384_1 <= EXPORT)||LA384_1==FAR||LA384_1==FINAL||LA384_1==INLINE||(LA384_1 >= LIBRARY && LA384_1 <= LOCAL)||LA384_1==MESSAGE||LA384_1==NEAR||(LA384_1 >= OVERLOAD && LA384_1 <= OVERRIDE)||(LA384_1 >= PASCAL && LA384_1 <= PLATFORM)||(LA384_1 >= REGISTER && LA384_1 <= REINTRODUCE)||LA384_1==SAFECALL||(LA384_1 >= STATIC && LA384_1 <= STDCALL)||LA384_1==UNSAFE||(LA384_1 >= VARARGS && LA384_1 <= VIRTUAL)||LA384_1==WINAPI) ) {
alt384=1;
}
}
else if ( (LA384_0==ABSTRACT||LA384_0==ASSEMBLER||LA384_0==CDECL||LA384_0==DEPRECATED||LA384_0==DISPID||LA384_0==DYNAMIC||(LA384_0 >= EXPERIMENTAL && LA384_0 <= EXPORT)||LA384_0==FAR||LA384_0==FINAL||LA384_0==INLINE||(LA384_0 >= LIBRARY && LA384_0 <= LOCAL)||LA384_0==MESSAGE||LA384_0==NEAR||(LA384_0 >= OVERLOAD && LA384_0 <= OVERRIDE)||(LA384_0 >= PASCAL && LA384_0 <= PLATFORM)||(LA384_0 >= REGISTER && LA384_0 <= REINTRODUCE)||LA384_0==SAFECALL||(LA384_0 >= STATIC && LA384_0 <= STDCALL)||LA384_0==UNSAFE||(LA384_0 >= VARARGS && LA384_0 <= VIRTUAL)||LA384_0==WINAPI) ) {
alt384=1;
}
switch (alt384) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:68: ( ';' )? implDirective
{
// au/com/integradev/delphi/antlr/Delphi.g:1078:68: ( ';' )?
int alt383=2;
int LA383_0 = input.LA(1);
if ( (LA383_0==SEMICOLON) ) {
alt383=1;
}
switch (alt383) {
case 1 :
// au/com/integradev/delphi/antlr/Delphi.g:1078:68: ';'
{
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred402_Delphi21936); if (state.failed) return;
}
break;
}
pushFollow(FOLLOW_implDirective_in_synpred402_Delphi21939);
implDirective();
state._fsp--;
if (state.failed) return;
}
break;
default :
break loop384;
}
}
match(input,SEMICOLON,FOLLOW_SEMICOLON_in_synpred402_Delphi21942); if (state.failed) return;
}
}
// $ANTLR end synpred402_Delphi
// $ANTLR start synpred431_Delphi
public final void synpred431_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1121:44: ( textLiteral )
// au/com/integradev/delphi/antlr/Delphi.g:1121:44: textLiteral
{
pushFollow(FOLLOW_textLiteral_in_synpred431_Delphi23242);
textLiteral();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred431_Delphi
// $ANTLR start synpred435_Delphi
public final void synpred435_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1126:42: ( dllName )
// au/com/integradev/delphi/antlr/Delphi.g:1126:42: dllName
{
pushFollow(FOLLOW_dllName_in_synpred435_Delphi23393);
dllName();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred435_Delphi
// $ANTLR start synpred436_Delphi
public final void synpred436_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1126:51: ( externalSpecifier )
// au/com/integradev/delphi/antlr/Delphi.g:1126:51: externalSpecifier
{
pushFollow(FOLLOW_externalSpecifier_in_synpred436_Delphi23396);
externalSpecifier();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred436_Delphi
// $ANTLR start synpred625_Delphi
public final void synpred625_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1181:38: ( genericArguments )
// au/com/integradev/delphi/antlr/Delphi.g:1181:38: genericArguments
{
pushFollow(FOLLOW_genericArguments_in_synpred625_Delphi25663);
genericArguments();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred625_Delphi
// $ANTLR start synpred626_Delphi
public final void synpred626_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1181:57: ( '.' extendedNameReference )
// au/com/integradev/delphi/antlr/Delphi.g:1181:57: '.' extendedNameReference
{
match(input,DOT,FOLLOW_DOT_in_synpred626_Delphi25667); if (state.failed) return;
pushFollow(FOLLOW_extendedNameReference_in_synpred626_Delphi25669);
extendedNameReference();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred626_Delphi
// $ANTLR start synpred627_Delphi
public final void synpred627_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1187:46: ( genericArguments )
// au/com/integradev/delphi/antlr/Delphi.g:1187:46: genericArguments
{
pushFollow(FOLLOW_genericArguments_in_synpred627_Delphi25853);
genericArguments();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred627_Delphi
// $ANTLR start synpred628_Delphi
public final void synpred628_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1187:65: ( '.' extendedNameReference )
// au/com/integradev/delphi/antlr/Delphi.g:1187:65: '.' extendedNameReference
{
match(input,DOT,FOLLOW_DOT_in_synpred628_Delphi25857); if (state.failed) return;
pushFollow(FOLLOW_extendedNameReference_in_synpred628_Delphi25859);
extendedNameReference();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred628_Delphi
// $ANTLR start synpred633_Delphi
public final void synpred633_Delphi_fragment() throws RecognitionException {
// au/com/integradev/delphi/antlr/Delphi.g:1196:32: ( ident )
// au/com/integradev/delphi/antlr/Delphi.g:1196:32: ident
{
pushFollow(FOLLOW_ident_in_synpred633_Delphi26165);
ident();
state._fsp--;
if (state.failed) return;
}
}
// $ANTLR end synpred633_Delphi
// Delegated rules
public final boolean synpred355_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred355_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred357_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred357_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred358_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred358_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred169_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred169_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred394_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred394_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred164_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred164_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred299_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred299_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred633_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred633_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred297_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred297_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred296_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred296_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred298_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred298_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred293_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred293_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred160_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred160_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred256_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred256_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred258_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred258_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred254_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred254_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred77_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred77_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred79_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred79_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred402_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred402_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred387_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred387_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred37_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred37_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred361_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred361_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred346_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred346_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred43_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred43_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred383_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred383_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred210_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred210_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred80_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred80_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred251_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred251_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred345_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred345_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred342_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred342_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred83_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred83_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred84_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred84_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred324_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred324_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred628_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred628_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred625_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred625_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred124_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred124_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred150_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred150_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred98_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred98_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred436_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred436_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred87_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred87_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred255_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred255_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred259_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred259_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred58_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred58_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred102_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred102_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred65_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred65_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred54_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred54_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred360_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred360_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred91_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred91_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred92_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred92_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred90_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred90_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred95_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred95_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred145_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred145_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred143_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred143_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred144_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred144_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred146_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred146_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred142_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred142_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred99_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred99_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred276_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred276_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred435_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred435_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred88_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred88_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred86_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred86_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred431_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred431_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred114_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred114_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred118_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred118_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred101_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred101_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred378_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred378_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred209_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred209_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred245_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred245_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred46_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred46_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred53_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred53_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred365_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred365_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred369_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred369_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred347_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred347_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred362_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred362_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred60_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred60_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred343_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred343_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred250_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred250_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred344_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred344_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred325_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred325_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred626_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred626_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred627_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred627_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred140_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred140_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred268_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred268_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred89_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred89_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred294_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred294_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred122_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred122_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred126_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred126_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred152_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred152_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred96_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred96_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred78_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred78_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred85_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred85_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred115_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred115_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred119_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred119_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred257_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred257_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred49_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred49_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred100_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred100_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred242_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred242_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred63_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred63_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred359_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred359_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred377_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred377_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred373_Delphi() {
state.backtracking++;
int start = input.mark();
try {
synpred373_Delphi_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
protected DFA70 dfa70 = new DFA70(this);
protected DFA73 dfa73 = new DFA73(this);
protected DFA74 dfa74 = new DFA74(this);
protected DFA77 dfa77 = new DFA77(this);
protected DFA78 dfa78 = new DFA78(this);
protected DFA80 dfa80 = new DFA80(this);
protected DFA117 dfa117 = new DFA117(this);
protected DFA178 dfa178 = new DFA178(this);
protected DFA179 dfa179 = new DFA179(this);
protected DFA184 dfa184 = new DFA184(this);
protected DFA183 dfa183 = new DFA183(this);
protected DFA186 dfa186 = new DFA186(this);
protected DFA187 dfa187 = new DFA187(this);
protected DFA211 dfa211 = new DFA211(this);
protected DFA293 dfa293 = new DFA293(this);
protected DFA294 dfa294 = new DFA294(this);
protected DFA301 dfa301 = new DFA301(this);
protected DFA303 dfa303 = new DFA303(this);
static final String DFA70_eotS =
"\125\uffff";
static final String DFA70_eofS =
"\1\2\124\uffff";
static final String DFA70_minS =
"\1\5\1\0\123\uffff";
static final String DFA70_maxS =
"\1\u00fa\1\0\123\uffff";
static final String DFA70_acceptS =
"\2\uffff\1\2\121\uffff\1\1";
static final String DFA70_specialS =
"\1\uffff\1\0\123\uffff}>";
static final String[] DFA70_transitionS = {
"\4\2\2\uffff\1\2\1\uffff\6\2\2\uffff\1\2\3\uffff\3\2\1\uffff\1\2\1\uffff"+
"\3\2\1\uffff\6\2\7\uffff\1\2\3\uffff\6\2\1\uffff\1\2\1\uffff\10\2\2\uffff"+
"\1\2\3\uffff\1\2\3\uffff\1\2\1\uffff\4\2\7\uffff\1\2\2\uffff\2\2\1\uffff"+
"\2\2\3\uffff\5\2\4\uffff\2\2\1\uffff\3\2\1\uffff\2\2\1\1\10\2\1\uffff"+
"\4\2\2\uffff\13\2\1\uffff\4\2\2\uffff\1\1\6\2\3\uffff\1\2\1\uffff\2\2"+
"\15\uffff\1\2\1\uffff\1\2\16\uffff\3\2\3\uffff\1\2\6\uffff\2\2\27\uffff"+
"\2\2\2\uffff\3\2\1\uffff\1\2\1\uffff\4\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA70_eot = DFA.unpackEncodedString(DFA70_eotS);
static final short[] DFA70_eof = DFA.unpackEncodedString(DFA70_eofS);
static final char[] DFA70_min = DFA.unpackEncodedStringToUnsignedChars(DFA70_minS);
static final char[] DFA70_max = DFA.unpackEncodedStringToUnsignedChars(DFA70_maxS);
static final short[] DFA70_accept = DFA.unpackEncodedString(DFA70_acceptS);
static final short[] DFA70_special = DFA.unpackEncodedString(DFA70_specialS);
static final short[][] DFA70_transition;
static {
int numStates = DFA70_transitionS.length;
DFA70_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 70, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA73_eotS =
"\144\uffff";
static final String DFA73_eofS =
"\1\6\143\uffff";
static final String DFA73_minS =
"\1\5\5\0\65\uffff\1\0\26\uffff\1\0\1\uffff\13\0\1\uffff\3\0\1\uffff";
static final String DFA73_maxS =
"\1\u00fa\5\0\65\uffff\1\0\26\uffff\1\0\1\uffff\13\0\1\uffff\3\0\1\uffff";
static final String DFA73_acceptS =
"\6\uffff\1\2\130\uffff\1\1\4\uffff";
static final String DFA73_specialS =
"\1\uffff\1\0\1\1\1\2\1\3\1\4\65\uffff\1\5\26\uffff\1\6\1\uffff\1\7\1\10"+
"\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\uffff\1\22\1\23\1\24\1"+
"\uffff}>";
static final String[] DFA73_transitionS = {
"\1\6\1\134\2\6\2\uffff\1\6\1\uffff\1\6\1\135\4\6\2\uffff\1\6\3\uffff"+
"\1\6\1\136\1\6\1\uffff\1\6\1\uffff\3\6\1\uffff\2\6\1\1\2\6\1\73\7\uffff"+
"\1\131\3\uffff\4\6\1\2\1\136\1\uffff\1\124\1\uffff\1\140\1\6\1\134\3"+
"\6\1\122\1\6\2\uffff\1\6\3\uffff\1\6\3\uffff\1\6\1\uffff\4\6\1\uffff"+
"\1\137\5\uffff\1\6\2\uffff\1\4\1\140\1\uffff\1\127\1\6\3\uffff\1\6\1"+
"\140\3\6\4\uffff\2\6\1\uffff\1\6\1\125\1\132\1\uffff\6\6\1\136\1\3\3"+
"\6\1\uffff\4\6\2\uffff\5\6\1\136\1\126\4\6\1\uffff\1\136\1\6\1\5\1\6"+
"\2\uffff\2\6\1\130\1\136\3\6\3\uffff\1\6\1\uffff\2\6\15\uffff\1\6\1\uffff"+
"\1\6\16\uffff\3\6\3\uffff\1\6\6\uffff\2\6\27\uffff\1\142\1\6\2\uffff"+
"\1\6\1\141\1\133\1\uffff\1\6\1\uffff\1\136\3\6",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
""
};
static final short[] DFA73_eot = DFA.unpackEncodedString(DFA73_eotS);
static final short[] DFA73_eof = DFA.unpackEncodedString(DFA73_eofS);
static final char[] DFA73_min = DFA.unpackEncodedStringToUnsignedChars(DFA73_minS);
static final char[] DFA73_max = DFA.unpackEncodedStringToUnsignedChars(DFA73_maxS);
static final short[] DFA73_accept = DFA.unpackEncodedString(DFA73_acceptS);
static final short[] DFA73_special = DFA.unpackEncodedString(DFA73_specialS);
static final short[][] DFA73_transition;
static {
int numStates = DFA73_transitionS.length;
DFA73_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA73_2 = input.LA(1);
int index73_2 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_2);
if ( s>=0 ) return s;
break;
case 2 :
int LA73_3 = input.LA(1);
int index73_3 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_3);
if ( s>=0 ) return s;
break;
case 3 :
int LA73_4 = input.LA(1);
int index73_4 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_4);
if ( s>=0 ) return s;
break;
case 4 :
int LA73_5 = input.LA(1);
int index73_5 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_5);
if ( s>=0 ) return s;
break;
case 5 :
int LA73_59 = input.LA(1);
int index73_59 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_59);
if ( s>=0 ) return s;
break;
case 6 :
int LA73_82 = input.LA(1);
int index73_82 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_82);
if ( s>=0 ) return s;
break;
case 7 :
int LA73_84 = input.LA(1);
int index73_84 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_84);
if ( s>=0 ) return s;
break;
case 8 :
int LA73_85 = input.LA(1);
int index73_85 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_85);
if ( s>=0 ) return s;
break;
case 9 :
int LA73_86 = input.LA(1);
int index73_86 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_86);
if ( s>=0 ) return s;
break;
case 10 :
int LA73_87 = input.LA(1);
int index73_87 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_87);
if ( s>=0 ) return s;
break;
case 11 :
int LA73_88 = input.LA(1);
int index73_88 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_88);
if ( s>=0 ) return s;
break;
case 12 :
int LA73_89 = input.LA(1);
int index73_89 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_89);
if ( s>=0 ) return s;
break;
case 13 :
int LA73_90 = input.LA(1);
int index73_90 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_90);
if ( s>=0 ) return s;
break;
case 14 :
int LA73_91 = input.LA(1);
int index73_91 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_91);
if ( s>=0 ) return s;
break;
case 15 :
int LA73_92 = input.LA(1);
int index73_92 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_92);
if ( s>=0 ) return s;
break;
case 16 :
int LA73_93 = input.LA(1);
int index73_93 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_93);
if ( s>=0 ) return s;
break;
case 17 :
int LA73_94 = input.LA(1);
int index73_94 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_94);
if ( s>=0 ) return s;
break;
case 18 :
int LA73_96 = input.LA(1);
int index73_96 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_96);
if ( s>=0 ) return s;
break;
case 19 :
int LA73_97 = input.LA(1);
int index73_97 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_97);
if ( s>=0 ) return s;
break;
case 20 :
int LA73_98 = input.LA(1);
int index73_98 = input.index();
input.rewind();
s = -1;
if ( (synpred118_Delphi()) ) {s = 95;}
else if ( (true) ) {s = 6;}
input.seek(index73_98);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 73, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA74_eotS =
"\147\uffff";
static final String DFA74_eofS =
"\1\2\146\uffff";
static final String DFA74_minS =
"\1\5\1\0\145\uffff";
static final String DFA74_maxS =
"\1\u00fa\1\0\145\uffff";
static final String DFA74_acceptS =
"\2\uffff\1\2\143\uffff\1\1";
static final String DFA74_specialS =
"\1\uffff\1\0\145\uffff}>";
static final String[] DFA74_transitionS = {
"\4\2\2\uffff\1\2\1\uffff\6\2\2\uffff\1\2\3\uffff\5\2\1\uffff\3\2\1\uffff"+
"\6\2\7\uffff\1\2\3\uffff\6\2\1\uffff\1\2\1\uffff\10\2\2\uffff\1\2\3\uffff"+
"\1\2\3\uffff\1\2\1\uffff\4\2\1\uffff\1\2\5\uffff\1\2\2\uffff\2\2\1\uffff"+
"\2\2\3\uffff\5\2\3\uffff\3\2\1\uffff\3\2\1\uffff\4\2\1\1\6\2\1\uffff"+
"\4\2\2\uffff\13\2\1\uffff\4\2\2\uffff\7\2\3\uffff\1\2\1\uffff\2\2\15"+
"\uffff\1\2\1\uffff\1\2\16\uffff\3\2\3\uffff\1\2\6\uffff\2\2\27\uffff"+
"\2\2\2\uffff\3\2\1\uffff\1\2\1\uffff\4\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA74_eot = DFA.unpackEncodedString(DFA74_eotS);
static final short[] DFA74_eof = DFA.unpackEncodedString(DFA74_eofS);
static final char[] DFA74_min = DFA.unpackEncodedStringToUnsignedChars(DFA74_minS);
static final char[] DFA74_max = DFA.unpackEncodedStringToUnsignedChars(DFA74_maxS);
static final short[] DFA74_accept = DFA.unpackEncodedString(DFA74_acceptS);
static final short[] DFA74_special = DFA.unpackEncodedString(DFA74_specialS);
static final short[][] DFA74_transition;
static {
int numStates = DFA74_transitionS.length;
DFA74_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 74, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA77_eotS =
"\145\uffff";
static final String DFA77_eofS =
"\1\1\144\uffff";
static final String DFA77_minS =
"\1\5\1\uffff\5\0\65\uffff\1\0\26\uffff\1\0\1\uffff\13\0\1\uffff\3\0\1"+
"\uffff";
static final String DFA77_maxS =
"\1\u00fa\1\uffff\5\0\65\uffff\1\0\26\uffff\1\0\1\uffff\13\0\1\uffff\3"+
"\0\1\uffff";
static final String DFA77_acceptS =
"\1\uffff\1\2\136\uffff\1\1\4\uffff";
static final String DFA77_specialS =
"\2\uffff\1\0\1\1\1\2\1\3\1\4\65\uffff\1\5\26\uffff\1\6\1\uffff\1\7\1\10"+
"\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\uffff\1\22\1\23\1\24\1"+
"\uffff}>";
static final String[] DFA77_transitionS = {
"\1\1\1\135\2\1\2\uffff\1\1\1\uffff\1\1\1\136\4\1\2\uffff\1\1\3\uffff"+
"\1\1\1\137\1\1\1\uffff\1\1\1\uffff\3\1\1\uffff\2\1\1\2\2\1\1\74\7\uffff"+
"\1\132\3\uffff\4\1\1\3\1\137\1\uffff\1\125\1\uffff\1\141\1\1\1\135\3"+
"\1\1\123\1\1\2\uffff\1\1\3\uffff\1\1\3\uffff\1\1\1\uffff\4\1\1\uffff"+
"\1\140\5\uffff\1\1\2\uffff\1\5\1\141\1\uffff\1\130\1\1\3\uffff\1\1\1"+
"\141\3\1\3\uffff\3\1\1\uffff\1\1\1\126\1\133\1\uffff\6\1\1\137\1\4\3"+
"\1\1\uffff\4\1\2\uffff\5\1\1\137\1\127\4\1\1\uffff\1\137\1\1\1\6\1\1"+
"\2\uffff\2\1\1\131\1\137\3\1\3\uffff\1\1\1\uffff\2\1\15\uffff\1\1\1\uffff"+
"\1\1\16\uffff\3\1\3\uffff\1\1\6\uffff\2\1\27\uffff\1\143\1\1\2\uffff"+
"\1\1\1\142\1\134\1\uffff\1\1\1\uffff\1\137\3\1",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
""
};
static final short[] DFA77_eot = DFA.unpackEncodedString(DFA77_eotS);
static final short[] DFA77_eof = DFA.unpackEncodedString(DFA77_eofS);
static final char[] DFA77_min = DFA.unpackEncodedStringToUnsignedChars(DFA77_minS);
static final char[] DFA77_max = DFA.unpackEncodedStringToUnsignedChars(DFA77_maxS);
static final short[] DFA77_accept = DFA.unpackEncodedString(DFA77_acceptS);
static final short[] DFA77_special = DFA.unpackEncodedString(DFA77_specialS);
static final short[][] DFA77_transition;
static {
int numStates = DFA77_transitionS.length;
DFA77_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA77_3 = input.LA(1);
int index77_3 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_3);
if ( s>=0 ) return s;
break;
case 2 :
int LA77_4 = input.LA(1);
int index77_4 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_4);
if ( s>=0 ) return s;
break;
case 3 :
int LA77_5 = input.LA(1);
int index77_5 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_5);
if ( s>=0 ) return s;
break;
case 4 :
int LA77_6 = input.LA(1);
int index77_6 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_6);
if ( s>=0 ) return s;
break;
case 5 :
int LA77_60 = input.LA(1);
int index77_60 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_60);
if ( s>=0 ) return s;
break;
case 6 :
int LA77_83 = input.LA(1);
int index77_83 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_83);
if ( s>=0 ) return s;
break;
case 7 :
int LA77_85 = input.LA(1);
int index77_85 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_85);
if ( s>=0 ) return s;
break;
case 8 :
int LA77_86 = input.LA(1);
int index77_86 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_86);
if ( s>=0 ) return s;
break;
case 9 :
int LA77_87 = input.LA(1);
int index77_87 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_87);
if ( s>=0 ) return s;
break;
case 10 :
int LA77_88 = input.LA(1);
int index77_88 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_88);
if ( s>=0 ) return s;
break;
case 11 :
int LA77_89 = input.LA(1);
int index77_89 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_89);
if ( s>=0 ) return s;
break;
case 12 :
int LA77_90 = input.LA(1);
int index77_90 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_90);
if ( s>=0 ) return s;
break;
case 13 :
int LA77_91 = input.LA(1);
int index77_91 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_91);
if ( s>=0 ) return s;
break;
case 14 :
int LA77_92 = input.LA(1);
int index77_92 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_92);
if ( s>=0 ) return s;
break;
case 15 :
int LA77_93 = input.LA(1);
int index77_93 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_93);
if ( s>=0 ) return s;
break;
case 16 :
int LA77_94 = input.LA(1);
int index77_94 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_94);
if ( s>=0 ) return s;
break;
case 17 :
int LA77_95 = input.LA(1);
int index77_95 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_95);
if ( s>=0 ) return s;
break;
case 18 :
int LA77_97 = input.LA(1);
int index77_97 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_97);
if ( s>=0 ) return s;
break;
case 19 :
int LA77_98 = input.LA(1);
int index77_98 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_98);
if ( s>=0 ) return s;
break;
case 20 :
int LA77_99 = input.LA(1);
int index77_99 = input.index();
input.rewind();
s = -1;
if ( (synpred122_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index77_99);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 77, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA78_eotS =
"\146\uffff";
static final String DFA78_eofS =
"\1\2\145\uffff";
static final String DFA78_minS =
"\1\5\1\0\144\uffff";
static final String DFA78_maxS =
"\1\u00fa\1\0\144\uffff";
static final String DFA78_acceptS =
"\2\uffff\1\2\142\uffff\1\1";
static final String DFA78_specialS =
"\1\uffff\1\0\144\uffff}>";
static final String[] DFA78_transitionS = {
"\4\2\2\uffff\1\2\1\uffff\6\2\2\uffff\1\2\3\uffff\3\2\1\uffff\1\2\1\uffff"+
"\3\2\1\uffff\6\2\7\uffff\1\2\3\uffff\6\2\1\uffff\1\2\1\uffff\10\2\2\uffff"+
"\1\2\3\uffff\1\2\3\uffff\1\2\1\uffff\4\2\1\uffff\1\2\5\uffff\1\2\2\uffff"+
"\2\2\1\uffff\2\2\3\uffff\5\2\3\uffff\3\2\1\uffff\3\2\1\uffff\4\2\1\1"+
"\6\2\1\uffff\4\2\2\uffff\13\2\1\uffff\4\2\2\uffff\7\2\3\uffff\1\2\1\uffff"+
"\2\2\15\uffff\1\2\1\uffff\1\2\16\uffff\3\2\3\uffff\1\2\6\uffff\2\2\27"+
"\uffff\2\2\2\uffff\3\2\1\uffff\1\2\1\uffff\4\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA78_eot = DFA.unpackEncodedString(DFA78_eotS);
static final short[] DFA78_eof = DFA.unpackEncodedString(DFA78_eofS);
static final char[] DFA78_min = DFA.unpackEncodedStringToUnsignedChars(DFA78_minS);
static final char[] DFA78_max = DFA.unpackEncodedStringToUnsignedChars(DFA78_maxS);
static final short[] DFA78_accept = DFA.unpackEncodedString(DFA78_acceptS);
static final short[] DFA78_special = DFA.unpackEncodedString(DFA78_specialS);
static final short[][] DFA78_transition;
static {
int numStates = DFA78_transitionS.length;
DFA78_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 78, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA80_eotS =
"\145\uffff";
static final String DFA80_eofS =
"\1\1\144\uffff";
static final String DFA80_minS =
"\1\5\1\uffff\5\0\65\uffff\1\0\26\uffff\1\0\1\uffff\13\0\1\uffff\3\0\1"+
"\uffff";
static final String DFA80_maxS =
"\1\u00fa\1\uffff\5\0\65\uffff\1\0\26\uffff\1\0\1\uffff\13\0\1\uffff\3"+
"\0\1\uffff";
static final String DFA80_acceptS =
"\1\uffff\1\2\136\uffff\1\1\4\uffff";
static final String DFA80_specialS =
"\2\uffff\1\0\1\1\1\2\1\3\1\4\65\uffff\1\5\26\uffff\1\6\1\uffff\1\7\1\10"+
"\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\uffff\1\22\1\23\1\24\1"+
"\uffff}>";
static final String[] DFA80_transitionS = {
"\1\1\1\135\2\1\2\uffff\1\1\1\uffff\1\1\1\136\4\1\2\uffff\1\1\3\uffff"+
"\1\1\1\137\1\1\1\uffff\1\1\1\uffff\3\1\1\uffff\2\1\1\2\2\1\1\74\7\uffff"+
"\1\132\3\uffff\4\1\1\3\1\137\1\uffff\1\125\1\uffff\1\141\1\1\1\135\3"+
"\1\1\123\1\1\2\uffff\1\1\3\uffff\1\1\3\uffff\1\1\1\uffff\4\1\1\uffff"+
"\1\140\5\uffff\1\1\2\uffff\1\5\1\141\1\uffff\1\130\1\1\3\uffff\1\1\1"+
"\141\3\1\3\uffff\3\1\1\uffff\1\1\1\126\1\133\1\uffff\6\1\1\137\1\4\3"+
"\1\1\uffff\4\1\2\uffff\5\1\1\137\1\127\4\1\1\uffff\1\137\1\1\1\6\1\1"+
"\2\uffff\2\1\1\131\1\137\3\1\3\uffff\1\1\1\uffff\2\1\15\uffff\1\1\1\uffff"+
"\1\1\16\uffff\3\1\3\uffff\1\1\6\uffff\2\1\27\uffff\1\143\1\1\2\uffff"+
"\1\1\1\142\1\134\1\uffff\1\1\1\uffff\1\137\3\1",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
""
};
static final short[] DFA80_eot = DFA.unpackEncodedString(DFA80_eotS);
static final short[] DFA80_eof = DFA.unpackEncodedString(DFA80_eofS);
static final char[] DFA80_min = DFA.unpackEncodedStringToUnsignedChars(DFA80_minS);
static final char[] DFA80_max = DFA.unpackEncodedStringToUnsignedChars(DFA80_maxS);
static final short[] DFA80_accept = DFA.unpackEncodedString(DFA80_acceptS);
static final short[] DFA80_special = DFA.unpackEncodedString(DFA80_specialS);
static final short[][] DFA80_transition;
static {
int numStates = DFA80_transitionS.length;
DFA80_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA80_3 = input.LA(1);
int index80_3 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_3);
if ( s>=0 ) return s;
break;
case 2 :
int LA80_4 = input.LA(1);
int index80_4 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_4);
if ( s>=0 ) return s;
break;
case 3 :
int LA80_5 = input.LA(1);
int index80_5 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_5);
if ( s>=0 ) return s;
break;
case 4 :
int LA80_6 = input.LA(1);
int index80_6 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_6);
if ( s>=0 ) return s;
break;
case 5 :
int LA80_60 = input.LA(1);
int index80_60 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_60);
if ( s>=0 ) return s;
break;
case 6 :
int LA80_83 = input.LA(1);
int index80_83 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_83);
if ( s>=0 ) return s;
break;
case 7 :
int LA80_85 = input.LA(1);
int index80_85 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_85);
if ( s>=0 ) return s;
break;
case 8 :
int LA80_86 = input.LA(1);
int index80_86 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_86);
if ( s>=0 ) return s;
break;
case 9 :
int LA80_87 = input.LA(1);
int index80_87 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_87);
if ( s>=0 ) return s;
break;
case 10 :
int LA80_88 = input.LA(1);
int index80_88 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_88);
if ( s>=0 ) return s;
break;
case 11 :
int LA80_89 = input.LA(1);
int index80_89 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_89);
if ( s>=0 ) return s;
break;
case 12 :
int LA80_90 = input.LA(1);
int index80_90 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_90);
if ( s>=0 ) return s;
break;
case 13 :
int LA80_91 = input.LA(1);
int index80_91 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_91);
if ( s>=0 ) return s;
break;
case 14 :
int LA80_92 = input.LA(1);
int index80_92 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_92);
if ( s>=0 ) return s;
break;
case 15 :
int LA80_93 = input.LA(1);
int index80_93 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_93);
if ( s>=0 ) return s;
break;
case 16 :
int LA80_94 = input.LA(1);
int index80_94 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_94);
if ( s>=0 ) return s;
break;
case 17 :
int LA80_95 = input.LA(1);
int index80_95 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_95);
if ( s>=0 ) return s;
break;
case 18 :
int LA80_97 = input.LA(1);
int index80_97 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_97);
if ( s>=0 ) return s;
break;
case 19 :
int LA80_98 = input.LA(1);
int index80_98 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_98);
if ( s>=0 ) return s;
break;
case 20 :
int LA80_99 = input.LA(1);
int index80_99 = input.index();
input.rewind();
s = -1;
if ( (synpred126_Delphi()) ) {s = 96;}
else if ( (true) ) {s = 1;}
input.seek(index80_99);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 80, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA117_eotS =
"\126\uffff";
static final String DFA117_eofS =
"\1\2\125\uffff";
static final String DFA117_minS =
"\1\5\1\0\124\uffff";
static final String DFA117_maxS =
"\1\u00fa\1\0\124\uffff";
static final String DFA117_acceptS =
"\2\uffff\1\2\122\uffff\1\1";
static final String DFA117_specialS =
"\1\uffff\1\0\124\uffff}>";
static final String[] DFA117_transitionS = {
"\3\2\1\1\2\uffff\1\2\1\uffff\6\2\2\uffff\1\2\3\uffff\3\2\1\uffff\1\2"+
"\1\uffff\3\2\1\uffff\6\2\7\uffff\1\2\3\uffff\6\2\1\uffff\1\2\1\uffff"+
"\10\2\2\uffff\1\2\3\uffff\1\2\3\uffff\1\2\1\uffff\4\2\7\uffff\1\2\2\uffff"+
"\2\2\1\uffff\2\2\3\uffff\5\2\4\uffff\2\2\1\uffff\3\2\1\uffff\13\2\1\uffff"+
"\4\2\2\uffff\13\2\1\uffff\4\2\2\uffff\7\2\3\uffff\1\2\1\uffff\2\2\15"+
"\uffff\1\2\1\uffff\1\2\16\uffff\3\2\3\uffff\1\2\6\uffff\2\2\27\uffff"+
"\2\2\2\uffff\3\2\1\uffff\1\2\1\uffff\4\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA117_eot = DFA.unpackEncodedString(DFA117_eotS);
static final short[] DFA117_eof = DFA.unpackEncodedString(DFA117_eofS);
static final char[] DFA117_min = DFA.unpackEncodedStringToUnsignedChars(DFA117_minS);
static final char[] DFA117_max = DFA.unpackEncodedStringToUnsignedChars(DFA117_maxS);
static final short[] DFA117_accept = DFA.unpackEncodedString(DFA117_acceptS);
static final short[] DFA117_special = DFA.unpackEncodedString(DFA117_specialS);
static final short[][] DFA117_transition;
static {
int numStates = DFA117_transitionS.length;
DFA117_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 117, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA178_eotS =
"\171\uffff";
static final String DFA178_eofS =
"\1\1\170\uffff";
static final String DFA178_minS =
"\1\5\14\uffff\1\0\110\uffff\1\0\42\uffff";
static final String DFA178_maxS =
"\1\u00fa\14\uffff\1\0\110\uffff\1\0\42\uffff";
static final String DFA178_acceptS =
"\1\uffff\1\2\161\uffff\1\1\5\uffff";
static final String DFA178_specialS =
"\15\uffff\1\0\110\uffff\1\1\42\uffff}>";
static final String[] DFA178_transitionS = {
"\4\1\2\uffff\1\1\1\uffff\6\1\2\uffff\1\1\3\uffff\5\1\1\uffff\3\1\1\uffff"+
"\6\1\3\uffff\1\1\1\uffff\3\1\3\uffff\2\1\1\15\5\1\1\uffff\10\1\2\uffff"+
"\1\1\2\163\1\uffff\1\1\3\uffff\3\1\1\126\4\1\1\uffff\1\163\3\uffff\1"+
"\1\2\163\2\1\1\uffff\2\1\3\uffff\5\1\1\163\2\uffff\3\1\1\uffff\3\1\1"+
"\uffff\13\1\1\uffff\4\1\2\uffff\13\1\1\uffff\4\1\2\uffff\7\1\2\uffff"+
"\5\1\15\uffff\1\1\1\uffff\1\1\16\uffff\3\1\3\uffff\1\1\6\uffff\2\1\27"+
"\uffff\2\1\2\uffff\3\1\1\uffff\1\1\1\uffff\4\1",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA178_eot = DFA.unpackEncodedString(DFA178_eotS);
static final short[] DFA178_eof = DFA.unpackEncodedString(DFA178_eofS);
static final char[] DFA178_min = DFA.unpackEncodedStringToUnsignedChars(DFA178_minS);
static final char[] DFA178_max = DFA.unpackEncodedStringToUnsignedChars(DFA178_maxS);
static final short[] DFA178_accept = DFA.unpackEncodedString(DFA178_acceptS);
static final short[] DFA178_special = DFA.unpackEncodedString(DFA178_specialS);
static final short[][] DFA178_transition;
static {
int numStates = DFA178_transitionS.length;
DFA178_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA178_86 = input.LA(1);
int index178_86 = input.index();
input.rewind();
s = -1;
if ( (synpred250_Delphi()) ) {s = 115;}
else if ( (true) ) {s = 1;}
input.seek(index178_86);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 178, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA179_eotS =
"\173\uffff";
static final String DFA179_eofS =
"\1\1\172\uffff";
static final String DFA179_minS =
"\1\5\37\uffff\2\0\131\uffff";
static final String DFA179_maxS =
"\1\u00fc\37\uffff\2\0\131\uffff";
static final String DFA179_acceptS =
"\1\uffff\1\2\167\uffff\1\1\1\uffff";
static final String DFA179_specialS =
"\40\uffff\1\0\1\1\131\uffff}>";
static final String[] DFA179_transitionS = {
"\4\1\2\uffff\1\1\1\uffff\6\1\2\uffff\1\1\3\uffff\5\1\1\uffff\3\1\1\uffff"+
"\6\1\3\uffff\1\1\1\uffff\3\1\3\uffff\10\1\1\uffff\10\1\2\uffff\3\1\1"+
"\uffff\1\1\3\uffff\10\1\1\uffff\1\1\3\uffff\5\1\1\uffff\1\1\1\41\3\uffff"+
"\6\1\2\uffff\3\1\1\171\3\1\1\uffff\10\1\1\40\2\1\1\uffff\4\1\2\uffff"+
"\13\1\1\uffff\4\1\2\uffff\7\1\2\uffff\5\1\15\uffff\1\1\1\uffff\1\1\16"+
"\uffff\3\1\3\uffff\1\1\6\uffff\2\1\27\uffff\2\1\2\uffff\3\1\1\uffff\1"+
"\1\1\uffff\4\1\1\uffff\1\171",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA179_eot = DFA.unpackEncodedString(DFA179_eotS);
static final short[] DFA179_eof = DFA.unpackEncodedString(DFA179_eofS);
static final char[] DFA179_min = DFA.unpackEncodedStringToUnsignedChars(DFA179_minS);
static final char[] DFA179_max = DFA.unpackEncodedStringToUnsignedChars(DFA179_maxS);
static final short[] DFA179_accept = DFA.unpackEncodedString(DFA179_acceptS);
static final short[] DFA179_special = DFA.unpackEncodedString(DFA179_specialS);
static final short[][] DFA179_transition;
static {
int numStates = DFA179_transitionS.length;
DFA179_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA179_33 = input.LA(1);
int index179_33 = input.index();
input.rewind();
s = -1;
if ( (synpred251_Delphi()) ) {s = 121;}
else if ( (true) ) {s = 1;}
input.seek(index179_33);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 179, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA184_eotS =
"\u0085\uffff";
static final String DFA184_eofS =
"\1\22\u0084\uffff";
static final String DFA184_minS =
"\1\5\2\0\1\uffff\u0080\0\1\uffff";
static final String DFA184_maxS =
"\1\u00fc\2\0\1\uffff\u0080\0\1\uffff";
static final String DFA184_acceptS =
"\3\uffff\1\1\u0080\uffff\1\2";
static final String DFA184_specialS =
"\1\uffff\1\0\1\1\1\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1"+
"\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\31\1"+
"\32\1\33\1\34\1\35\1\36\1\37\1\40\1\41\1\42\1\43\1\44\1\45\1\46\1\47\1"+
"\50\1\51\1\52\1\53\1\54\1\55\1\56\1\57\1\60\1\61\1\62\1\63\1\64\1\65\1"+
"\66\1\67\1\70\1\71\1\72\1\73\1\74\1\75\1\76\1\77\1\100\1\101\1\102\1\103"+
"\1\104\1\105\1\106\1\107\1\110\1\111\1\112\1\113\1\114\1\115\1\116\1\117"+
"\1\120\1\121\1\122\1\123\1\124\1\125\1\126\1\127\1\130\1\131\1\132\1\133"+
"\1\134\1\135\1\136\1\137\1\140\1\141\1\142\1\143\1\144\1\145\1\146\1\147"+
"\1\150\1\151\1\152\1\153\1\154\1\155\1\156\1\157\1\160\1\161\1\162\1\163"+
"\1\164\1\165\1\166\1\167\1\170\1\171\1\172\1\173\1\174\1\175\1\176\1\177"+
"\1\u0080\1\u0081\1\uffff}>";
static final String[] DFA184_transitionS = {
"\1\54\1\165\1\73\1\u0083\1\uffff\1\27\1\62\1\32\1\144\1\166\1\u0083\1"+
"\126\1\147\1\113\2\uffff\1\145\3\uffff\1\125\1\167\1\115\1\150\1\55\1"+
"\uffff\1\122\1\120\1\u0083\1\uffff\1\2\1\u0081\1\21\1\6\1\120\1\14\1"+
"\uffff\1\25\1\24\1\151\1\3\1\60\1\155\1\162\3\uffff\1\127\1\106\1\37"+
"\1\132\1\47\1\167\1\176\1\175\1\uffff\1\171\1\65\1\165\1\130\1\133\1"+
"\140\1\177\1\67\2\uffff\1\146\1\40\1\43\1\uffff\1\u0083\3\uffff\1\134"+
"\1\u0082\1\15\1\45\1\16\1\104\1\u0080\1\170\1\uffff\1\46\3\uffff\1\174"+
"\1\41\1\42\1\52\1\171\1\uffff\1\160\1\34\1\26\1\23\1\uffff\1\51\1\171"+
"\1\103\1\20\1\72\1\44\2\uffff\1\153\1\117\1\114\1\35\1\u0083\1\156\1"+
"\163\1\uffff\1\u0083\1\105\1\5\1\56\1\4\1\57\1\167\1\50\1\33\1\110\1"+
"\70\1\uffff\1\121\1\107\1\111\1\112\2\uffff\1\143\1\10\1\12\1\66\1\61"+
"\1\167\1\157\1\136\1\u0083\1\53\1\123\1\uffff\1\167\1\u0083\1\7\1\63"+
"\1\30\1\31\1\5\1\56\1\161\1\167\1\17\1\71\1\64\2\uffff\1\152\1\135\1"+
"\154\1\142\1\124\15\uffff\1\76\1\uffff\1\101\16\uffff\1\75\1\1\1\74\3"+
"\uffff\1\102\6\uffff\1\100\1\77\27\uffff\1\173\1\131\2\uffff\1\116\1"+
"\172\1\164\1\uffff\1\137\1\uffff\1\167\1\141\1\11\1\13\1\uffff\1\36",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
""
};
static final short[] DFA184_eot = DFA.unpackEncodedString(DFA184_eotS);
static final short[] DFA184_eof = DFA.unpackEncodedString(DFA184_eofS);
static final char[] DFA184_min = DFA.unpackEncodedStringToUnsignedChars(DFA184_minS);
static final char[] DFA184_max = DFA.unpackEncodedStringToUnsignedChars(DFA184_maxS);
static final short[] DFA184_accept = DFA.unpackEncodedString(DFA184_acceptS);
static final short[] DFA184_special = DFA.unpackEncodedString(DFA184_specialS);
static final short[][] DFA184_transition;
static {
int numStates = DFA184_transitionS.length;
DFA184_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA184_2 = input.LA(1);
int index184_2 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_2);
if ( s>=0 ) return s;
break;
case 2 :
int LA184_4 = input.LA(1);
int index184_4 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_4);
if ( s>=0 ) return s;
break;
case 3 :
int LA184_5 = input.LA(1);
int index184_5 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_5);
if ( s>=0 ) return s;
break;
case 4 :
int LA184_6 = input.LA(1);
int index184_6 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_6);
if ( s>=0 ) return s;
break;
case 5 :
int LA184_7 = input.LA(1);
int index184_7 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_7);
if ( s>=0 ) return s;
break;
case 6 :
int LA184_8 = input.LA(1);
int index184_8 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_8);
if ( s>=0 ) return s;
break;
case 7 :
int LA184_9 = input.LA(1);
int index184_9 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_9);
if ( s>=0 ) return s;
break;
case 8 :
int LA184_10 = input.LA(1);
int index184_10 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_10);
if ( s>=0 ) return s;
break;
case 9 :
int LA184_11 = input.LA(1);
int index184_11 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_11);
if ( s>=0 ) return s;
break;
case 10 :
int LA184_12 = input.LA(1);
int index184_12 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_12);
if ( s>=0 ) return s;
break;
case 11 :
int LA184_13 = input.LA(1);
int index184_13 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_13);
if ( s>=0 ) return s;
break;
case 12 :
int LA184_14 = input.LA(1);
int index184_14 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_14);
if ( s>=0 ) return s;
break;
case 13 :
int LA184_15 = input.LA(1);
int index184_15 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_15);
if ( s>=0 ) return s;
break;
case 14 :
int LA184_16 = input.LA(1);
int index184_16 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_16);
if ( s>=0 ) return s;
break;
case 15 :
int LA184_17 = input.LA(1);
int index184_17 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_17);
if ( s>=0 ) return s;
break;
case 16 :
int LA184_18 = input.LA(1);
int index184_18 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_18);
if ( s>=0 ) return s;
break;
case 17 :
int LA184_19 = input.LA(1);
int index184_19 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_19);
if ( s>=0 ) return s;
break;
case 18 :
int LA184_20 = input.LA(1);
int index184_20 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_20);
if ( s>=0 ) return s;
break;
case 19 :
int LA184_21 = input.LA(1);
int index184_21 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_21);
if ( s>=0 ) return s;
break;
case 20 :
int LA184_22 = input.LA(1);
int index184_22 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_22);
if ( s>=0 ) return s;
break;
case 21 :
int LA184_23 = input.LA(1);
int index184_23 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_23);
if ( s>=0 ) return s;
break;
case 22 :
int LA184_24 = input.LA(1);
int index184_24 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_24);
if ( s>=0 ) return s;
break;
case 23 :
int LA184_25 = input.LA(1);
int index184_25 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_25);
if ( s>=0 ) return s;
break;
case 24 :
int LA184_26 = input.LA(1);
int index184_26 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_26);
if ( s>=0 ) return s;
break;
case 25 :
int LA184_27 = input.LA(1);
int index184_27 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_27);
if ( s>=0 ) return s;
break;
case 26 :
int LA184_28 = input.LA(1);
int index184_28 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_28);
if ( s>=0 ) return s;
break;
case 27 :
int LA184_29 = input.LA(1);
int index184_29 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_29);
if ( s>=0 ) return s;
break;
case 28 :
int LA184_30 = input.LA(1);
int index184_30 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_30);
if ( s>=0 ) return s;
break;
case 29 :
int LA184_31 = input.LA(1);
int index184_31 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_31);
if ( s>=0 ) return s;
break;
case 30 :
int LA184_32 = input.LA(1);
int index184_32 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_32);
if ( s>=0 ) return s;
break;
case 31 :
int LA184_33 = input.LA(1);
int index184_33 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_33);
if ( s>=0 ) return s;
break;
case 32 :
int LA184_34 = input.LA(1);
int index184_34 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_34);
if ( s>=0 ) return s;
break;
case 33 :
int LA184_35 = input.LA(1);
int index184_35 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_35);
if ( s>=0 ) return s;
break;
case 34 :
int LA184_36 = input.LA(1);
int index184_36 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_36);
if ( s>=0 ) return s;
break;
case 35 :
int LA184_37 = input.LA(1);
int index184_37 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_37);
if ( s>=0 ) return s;
break;
case 36 :
int LA184_38 = input.LA(1);
int index184_38 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_38);
if ( s>=0 ) return s;
break;
case 37 :
int LA184_39 = input.LA(1);
int index184_39 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_39);
if ( s>=0 ) return s;
break;
case 38 :
int LA184_40 = input.LA(1);
int index184_40 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_40);
if ( s>=0 ) return s;
break;
case 39 :
int LA184_41 = input.LA(1);
int index184_41 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_41);
if ( s>=0 ) return s;
break;
case 40 :
int LA184_42 = input.LA(1);
int index184_42 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_42);
if ( s>=0 ) return s;
break;
case 41 :
int LA184_43 = input.LA(1);
int index184_43 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_43);
if ( s>=0 ) return s;
break;
case 42 :
int LA184_44 = input.LA(1);
int index184_44 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_44);
if ( s>=0 ) return s;
break;
case 43 :
int LA184_45 = input.LA(1);
int index184_45 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_45);
if ( s>=0 ) return s;
break;
case 44 :
int LA184_46 = input.LA(1);
int index184_46 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_46);
if ( s>=0 ) return s;
break;
case 45 :
int LA184_47 = input.LA(1);
int index184_47 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_47);
if ( s>=0 ) return s;
break;
case 46 :
int LA184_48 = input.LA(1);
int index184_48 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_48);
if ( s>=0 ) return s;
break;
case 47 :
int LA184_49 = input.LA(1);
int index184_49 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_49);
if ( s>=0 ) return s;
break;
case 48 :
int LA184_50 = input.LA(1);
int index184_50 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_50);
if ( s>=0 ) return s;
break;
case 49 :
int LA184_51 = input.LA(1);
int index184_51 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_51);
if ( s>=0 ) return s;
break;
case 50 :
int LA184_52 = input.LA(1);
int index184_52 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_52);
if ( s>=0 ) return s;
break;
case 51 :
int LA184_53 = input.LA(1);
int index184_53 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_53);
if ( s>=0 ) return s;
break;
case 52 :
int LA184_54 = input.LA(1);
int index184_54 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_54);
if ( s>=0 ) return s;
break;
case 53 :
int LA184_55 = input.LA(1);
int index184_55 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_55);
if ( s>=0 ) return s;
break;
case 54 :
int LA184_56 = input.LA(1);
int index184_56 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_56);
if ( s>=0 ) return s;
break;
case 55 :
int LA184_57 = input.LA(1);
int index184_57 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_57);
if ( s>=0 ) return s;
break;
case 56 :
int LA184_58 = input.LA(1);
int index184_58 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_58);
if ( s>=0 ) return s;
break;
case 57 :
int LA184_59 = input.LA(1);
int index184_59 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_59);
if ( s>=0 ) return s;
break;
case 58 :
int LA184_60 = input.LA(1);
int index184_60 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_60);
if ( s>=0 ) return s;
break;
case 59 :
int LA184_61 = input.LA(1);
int index184_61 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_61);
if ( s>=0 ) return s;
break;
case 60 :
int LA184_62 = input.LA(1);
int index184_62 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_62);
if ( s>=0 ) return s;
break;
case 61 :
int LA184_63 = input.LA(1);
int index184_63 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_63);
if ( s>=0 ) return s;
break;
case 62 :
int LA184_64 = input.LA(1);
int index184_64 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_64);
if ( s>=0 ) return s;
break;
case 63 :
int LA184_65 = input.LA(1);
int index184_65 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_65);
if ( s>=0 ) return s;
break;
case 64 :
int LA184_66 = input.LA(1);
int index184_66 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_66);
if ( s>=0 ) return s;
break;
case 65 :
int LA184_67 = input.LA(1);
int index184_67 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_67);
if ( s>=0 ) return s;
break;
case 66 :
int LA184_68 = input.LA(1);
int index184_68 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_68);
if ( s>=0 ) return s;
break;
case 67 :
int LA184_69 = input.LA(1);
int index184_69 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_69);
if ( s>=0 ) return s;
break;
case 68 :
int LA184_70 = input.LA(1);
int index184_70 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_70);
if ( s>=0 ) return s;
break;
case 69 :
int LA184_71 = input.LA(1);
int index184_71 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_71);
if ( s>=0 ) return s;
break;
case 70 :
int LA184_72 = input.LA(1);
int index184_72 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_72);
if ( s>=0 ) return s;
break;
case 71 :
int LA184_73 = input.LA(1);
int index184_73 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_73);
if ( s>=0 ) return s;
break;
case 72 :
int LA184_74 = input.LA(1);
int index184_74 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_74);
if ( s>=0 ) return s;
break;
case 73 :
int LA184_75 = input.LA(1);
int index184_75 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_75);
if ( s>=0 ) return s;
break;
case 74 :
int LA184_76 = input.LA(1);
int index184_76 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_76);
if ( s>=0 ) return s;
break;
case 75 :
int LA184_77 = input.LA(1);
int index184_77 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_77);
if ( s>=0 ) return s;
break;
case 76 :
int LA184_78 = input.LA(1);
int index184_78 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_78);
if ( s>=0 ) return s;
break;
case 77 :
int LA184_79 = input.LA(1);
int index184_79 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_79);
if ( s>=0 ) return s;
break;
case 78 :
int LA184_80 = input.LA(1);
int index184_80 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_80);
if ( s>=0 ) return s;
break;
case 79 :
int LA184_81 = input.LA(1);
int index184_81 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_81);
if ( s>=0 ) return s;
break;
case 80 :
int LA184_82 = input.LA(1);
int index184_82 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_82);
if ( s>=0 ) return s;
break;
case 81 :
int LA184_83 = input.LA(1);
int index184_83 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_83);
if ( s>=0 ) return s;
break;
case 82 :
int LA184_84 = input.LA(1);
int index184_84 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_84);
if ( s>=0 ) return s;
break;
case 83 :
int LA184_85 = input.LA(1);
int index184_85 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_85);
if ( s>=0 ) return s;
break;
case 84 :
int LA184_86 = input.LA(1);
int index184_86 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_86);
if ( s>=0 ) return s;
break;
case 85 :
int LA184_87 = input.LA(1);
int index184_87 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_87);
if ( s>=0 ) return s;
break;
case 86 :
int LA184_88 = input.LA(1);
int index184_88 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_88);
if ( s>=0 ) return s;
break;
case 87 :
int LA184_89 = input.LA(1);
int index184_89 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_89);
if ( s>=0 ) return s;
break;
case 88 :
int LA184_90 = input.LA(1);
int index184_90 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_90);
if ( s>=0 ) return s;
break;
case 89 :
int LA184_91 = input.LA(1);
int index184_91 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_91);
if ( s>=0 ) return s;
break;
case 90 :
int LA184_92 = input.LA(1);
int index184_92 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_92);
if ( s>=0 ) return s;
break;
case 91 :
int LA184_93 = input.LA(1);
int index184_93 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_93);
if ( s>=0 ) return s;
break;
case 92 :
int LA184_94 = input.LA(1);
int index184_94 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_94);
if ( s>=0 ) return s;
break;
case 93 :
int LA184_95 = input.LA(1);
int index184_95 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_95);
if ( s>=0 ) return s;
break;
case 94 :
int LA184_96 = input.LA(1);
int index184_96 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_96);
if ( s>=0 ) return s;
break;
case 95 :
int LA184_97 = input.LA(1);
int index184_97 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_97);
if ( s>=0 ) return s;
break;
case 96 :
int LA184_98 = input.LA(1);
int index184_98 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_98);
if ( s>=0 ) return s;
break;
case 97 :
int LA184_99 = input.LA(1);
int index184_99 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_99);
if ( s>=0 ) return s;
break;
case 98 :
int LA184_100 = input.LA(1);
int index184_100 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_100);
if ( s>=0 ) return s;
break;
case 99 :
int LA184_101 = input.LA(1);
int index184_101 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_101);
if ( s>=0 ) return s;
break;
case 100 :
int LA184_102 = input.LA(1);
int index184_102 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_102);
if ( s>=0 ) return s;
break;
case 101 :
int LA184_103 = input.LA(1);
int index184_103 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_103);
if ( s>=0 ) return s;
break;
case 102 :
int LA184_104 = input.LA(1);
int index184_104 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_104);
if ( s>=0 ) return s;
break;
case 103 :
int LA184_105 = input.LA(1);
int index184_105 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_105);
if ( s>=0 ) return s;
break;
case 104 :
int LA184_106 = input.LA(1);
int index184_106 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_106);
if ( s>=0 ) return s;
break;
case 105 :
int LA184_107 = input.LA(1);
int index184_107 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_107);
if ( s>=0 ) return s;
break;
case 106 :
int LA184_108 = input.LA(1);
int index184_108 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_108);
if ( s>=0 ) return s;
break;
case 107 :
int LA184_109 = input.LA(1);
int index184_109 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_109);
if ( s>=0 ) return s;
break;
case 108 :
int LA184_110 = input.LA(1);
int index184_110 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_110);
if ( s>=0 ) return s;
break;
case 109 :
int LA184_111 = input.LA(1);
int index184_111 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_111);
if ( s>=0 ) return s;
break;
case 110 :
int LA184_112 = input.LA(1);
int index184_112 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_112);
if ( s>=0 ) return s;
break;
case 111 :
int LA184_113 = input.LA(1);
int index184_113 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_113);
if ( s>=0 ) return s;
break;
case 112 :
int LA184_114 = input.LA(1);
int index184_114 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_114);
if ( s>=0 ) return s;
break;
case 113 :
int LA184_115 = input.LA(1);
int index184_115 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_115);
if ( s>=0 ) return s;
break;
case 114 :
int LA184_116 = input.LA(1);
int index184_116 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_116);
if ( s>=0 ) return s;
break;
case 115 :
int LA184_117 = input.LA(1);
int index184_117 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_117);
if ( s>=0 ) return s;
break;
case 116 :
int LA184_118 = input.LA(1);
int index184_118 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_118);
if ( s>=0 ) return s;
break;
case 117 :
int LA184_119 = input.LA(1);
int index184_119 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_119);
if ( s>=0 ) return s;
break;
case 118 :
int LA184_120 = input.LA(1);
int index184_120 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_120);
if ( s>=0 ) return s;
break;
case 119 :
int LA184_121 = input.LA(1);
int index184_121 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_121);
if ( s>=0 ) return s;
break;
case 120 :
int LA184_122 = input.LA(1);
int index184_122 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_122);
if ( s>=0 ) return s;
break;
case 121 :
int LA184_123 = input.LA(1);
int index184_123 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_123);
if ( s>=0 ) return s;
break;
case 122 :
int LA184_124 = input.LA(1);
int index184_124 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_124);
if ( s>=0 ) return s;
break;
case 123 :
int LA184_125 = input.LA(1);
int index184_125 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_125);
if ( s>=0 ) return s;
break;
case 124 :
int LA184_126 = input.LA(1);
int index184_126 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_126);
if ( s>=0 ) return s;
break;
case 125 :
int LA184_127 = input.LA(1);
int index184_127 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_127);
if ( s>=0 ) return s;
break;
case 126 :
int LA184_128 = input.LA(1);
int index184_128 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_128);
if ( s>=0 ) return s;
break;
case 127 :
int LA184_129 = input.LA(1);
int index184_129 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_129);
if ( s>=0 ) return s;
break;
case 128 :
int LA184_130 = input.LA(1);
int index184_130 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_130);
if ( s>=0 ) return s;
break;
case 129 :
int LA184_131 = input.LA(1);
int index184_131 = input.index();
input.rewind();
s = -1;
if ( (synpred258_Delphi()) ) {s = 3;}
else if ( (true) ) {s = 132;}
input.seek(index184_131);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 184, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA183_eotS =
"\u0084\uffff";
static final String DFA183_eofS =
"\1\1\u0083\uffff";
static final String DFA183_minS =
"\1\5\60\uffff\1\0\17\uffff\2\0\101\uffff";
static final String DFA183_maxS =
"\1\u00fc\60\uffff\1\0\17\uffff\2\0\101\uffff";
static final String DFA183_acceptS =
"\1\uffff\1\2\u0081\uffff\1\1";
static final String DFA183_specialS =
"\61\uffff\1\0\17\uffff\1\1\1\2\101\uffff}>";
static final String[] DFA183_transitionS = {
"\4\1\1\uffff\11\1\2\uffff\1\1\3\uffff\5\1\1\uffff\3\1\1\uffff\3\1\1\61"+
"\2\1\1\uffff\3\1\1\u0083\3\1\3\uffff\10\1\1\uffff\10\1\2\uffff\3\1\1"+
"\uffff\1\1\3\uffff\10\1\1\uffff\1\1\3\uffff\5\1\1\uffff\4\1\1\uffff\6"+
"\1\2\uffff\7\1\1\uffff\2\1\1\101\1\1\1\102\6\1\1\uffff\4\1\2\uffff\13"+
"\1\1\uffff\6\1\1\101\6\1\2\uffff\5\1\15\uffff\1\1\1\uffff\1\1\16\uffff"+
"\3\1\3\uffff\1\1\6\uffff\2\1\27\uffff\2\1\2\uffff\3\1\1\uffff\1\1\1\uffff"+
"\4\1\1\uffff\1\1",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA183_eot = DFA.unpackEncodedString(DFA183_eotS);
static final short[] DFA183_eof = DFA.unpackEncodedString(DFA183_eofS);
static final char[] DFA183_min = DFA.unpackEncodedStringToUnsignedChars(DFA183_minS);
static final char[] DFA183_max = DFA.unpackEncodedStringToUnsignedChars(DFA183_maxS);
static final short[] DFA183_accept = DFA.unpackEncodedString(DFA183_acceptS);
static final short[] DFA183_special = DFA.unpackEncodedString(DFA183_specialS);
static final short[][] DFA183_transition;
static {
int numStates = DFA183_transitionS.length;
DFA183_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA183_65 = input.LA(1);
int index183_65 = input.index();
input.rewind();
s = -1;
if ( (synpred257_Delphi()) ) {s = 131;}
else if ( (true) ) {s = 1;}
input.seek(index183_65);
if ( s>=0 ) return s;
break;
case 2 :
int LA183_66 = input.LA(1);
int index183_66 = input.index();
input.rewind();
s = -1;
if ( (synpred257_Delphi()) ) {s = 131;}
else if ( (true) ) {s = 1;}
input.seek(index183_66);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 183, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA186_eotS =
"\u0084\uffff";
static final String DFA186_eofS =
"\1\1\u0083\uffff";
static final String DFA186_minS =
"\1\5\60\uffff\1\0\17\uffff\2\0\101\uffff";
static final String DFA186_maxS =
"\1\u00fc\60\uffff\1\0\17\uffff\2\0\101\uffff";
static final String DFA186_acceptS =
"\1\uffff\1\2\u0081\uffff\1\1";
static final String DFA186_specialS =
"\61\uffff\1\0\17\uffff\1\1\1\2\101\uffff}>";
static final String[] DFA186_transitionS = {
"\4\1\1\uffff\11\1\2\uffff\1\1\3\uffff\5\1\1\uffff\3\1\1\uffff\3\1\1\61"+
"\2\1\1\uffff\3\1\1\u0083\3\1\3\uffff\10\1\1\uffff\10\1\2\uffff\3\1\1"+
"\uffff\1\1\3\uffff\10\1\1\uffff\1\1\3\uffff\5\1\1\uffff\4\1\1\uffff\6"+
"\1\2\uffff\7\1\1\uffff\2\1\1\101\1\1\1\102\6\1\1\uffff\4\1\2\uffff\13"+
"\1\1\uffff\6\1\1\101\6\1\2\uffff\5\1\15\uffff\1\1\1\uffff\1\1\16\uffff"+
"\3\1\3\uffff\1\1\6\uffff\2\1\27\uffff\2\1\2\uffff\3\1\1\uffff\1\1\1\uffff"+
"\4\1\1\uffff\1\1",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA186_eot = DFA.unpackEncodedString(DFA186_eotS);
static final short[] DFA186_eof = DFA.unpackEncodedString(DFA186_eofS);
static final char[] DFA186_min = DFA.unpackEncodedStringToUnsignedChars(DFA186_minS);
static final char[] DFA186_max = DFA.unpackEncodedStringToUnsignedChars(DFA186_maxS);
static final short[] DFA186_accept = DFA.unpackEncodedString(DFA186_acceptS);
static final short[] DFA186_special = DFA.unpackEncodedString(DFA186_specialS);
static final short[][] DFA186_transition;
static {
int numStates = DFA186_transitionS.length;
DFA186_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA186_65 = input.LA(1);
int index186_65 = input.index();
input.rewind();
s = -1;
if ( (synpred259_Delphi()) ) {s = 131;}
else if ( (true) ) {s = 1;}
input.seek(index186_65);
if ( s>=0 ) return s;
break;
case 2 :
int LA186_66 = input.LA(1);
int index186_66 = input.index();
input.rewind();
s = -1;
if ( (synpred259_Delphi()) ) {s = 131;}
else if ( (true) ) {s = 1;}
input.seek(index186_66);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 186, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA187_eotS =
"\u0085\uffff";
static final String DFA187_eofS =
"\1\5\u0084\uffff";
static final String DFA187_minS =
"\1\5\4\0\u0080\uffff";
static final String DFA187_maxS =
"\1\u00fc\4\0\u0080\uffff";
static final String DFA187_acceptS =
"\5\uffff\1\2\176\uffff\1\1";
static final String DFA187_specialS =
"\1\uffff\1\0\1\1\1\2\1\3\u0080\uffff}>";
static final String[] DFA187_transitionS = {
"\4\5\1\uffff\11\5\2\uffff\1\5\3\uffff\5\5\1\uffff\3\5\1\uffff\3\5\1\4"+
"\2\5\1\uffff\3\5\1\1\3\5\3\uffff\10\5\1\uffff\10\5\2\uffff\3\5\1\uffff"+
"\1\5\3\uffff\10\5\1\uffff\1\5\3\uffff\5\5\1\uffff\4\5\1\uffff\6\5\2\uffff"+
"\7\5\1\uffff\2\5\1\3\1\5\1\2\6\5\1\uffff\4\5\2\uffff\13\5\1\uffff\6\5"+
"\1\3\6\5\2\uffff\5\5\15\uffff\1\5\1\uffff\1\5\16\uffff\3\5\3\uffff\1"+
"\5\6\uffff\2\5\27\uffff\2\5\2\uffff\3\5\1\uffff\1\5\1\uffff\4\5\1\uffff"+
"\1\5",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA187_eot = DFA.unpackEncodedString(DFA187_eotS);
static final short[] DFA187_eof = DFA.unpackEncodedString(DFA187_eofS);
static final char[] DFA187_min = DFA.unpackEncodedStringToUnsignedChars(DFA187_minS);
static final char[] DFA187_max = DFA.unpackEncodedStringToUnsignedChars(DFA187_maxS);
static final short[] DFA187_accept = DFA.unpackEncodedString(DFA187_acceptS);
static final short[] DFA187_special = DFA.unpackEncodedString(DFA187_specialS);
static final short[][] DFA187_transition;
static {
int numStates = DFA187_transitionS.length;
DFA187_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA187_2 = input.LA(1);
int index187_2 = input.index();
input.rewind();
s = -1;
if ( (synpred268_Delphi()) ) {s = 132;}
else if ( (true) ) {s = 5;}
input.seek(index187_2);
if ( s>=0 ) return s;
break;
case 2 :
int LA187_3 = input.LA(1);
int index187_3 = input.index();
input.rewind();
s = -1;
if ( (synpred268_Delphi()) ) {s = 132;}
else if ( (true) ) {s = 5;}
input.seek(index187_3);
if ( s>=0 ) return s;
break;
case 3 :
int LA187_4 = input.LA(1);
int index187_4 = input.index();
input.rewind();
s = -1;
if ( (synpred268_Delphi()) ) {s = 132;}
else if ( (true) ) {s = 5;}
input.seek(index187_4);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 187, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA211_eotS =
"\16\uffff";
static final String DFA211_eofS =
"\3\3\1\uffff\1\3\1\uffff\3\3\1\uffff\1\3\3\uffff";
static final String DFA211_minS =
"\3\5\1\uffff\1\5\1\0\3\5\1\uffff\1\5\3\0";
static final String DFA211_maxS =
"\3\u00fc\1\uffff\1\u00fc\1\0\3\u00fc\1\uffff\1\u00fc\3\0";
static final String DFA211_acceptS =
"\3\uffff\1\2\5\uffff\1\1\4\uffff";
static final String DFA211_specialS =
"\5\uffff\1\0\5\uffff\1\1\1\2\1\3}>";
static final String[] DFA211_transitionS = {
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\3\3\1\2"+
"\2\3\1\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3\3"+
"\uffff\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff\7"+
"\3\1\uffff\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\15"+
"\uffff\1\3\1\uffff\1\1\16\uffff\3\3\3\uffff\1\3\6\uffff\2\3\27\uffff"+
"\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\3\3\1"+
"\4\2\3\1\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3"+
"\3\uffff\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff"+
"\7\3\1\uffff\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\15"+
"\uffff\1\3\1\uffff\1\1\16\uffff\3\3\3\uffff\1\3\6\uffff\1\5\1\3\27\uffff"+
"\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\6\3\1"+
"\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3\3\uffff"+
"\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff\7\3\1\uffff"+
"\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\2\uffff\1\10"+
"\12\uffff\1\3\1\uffff\1\3\16\uffff\1\3\1\6\1\7\3\uffff\1\3\6\uffff\2"+
"\3\27\uffff\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\6\3\1"+
"\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3\3\uffff"+
"\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff\7\3\1\uffff"+
"\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\2\uffff\1\10"+
"\12\uffff\1\3\1\uffff\1\3\16\uffff\1\3\1\6\1\7\3\uffff\1\3\6\uffff\2"+
"\3\27\uffff\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"\1\uffff",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\3\3\1"+
"\12\2\3\1\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3"+
"\3\uffff\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff"+
"\7\3\1\uffff\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\15"+
"\uffff\1\3\1\uffff\1\1\16\uffff\3\3\3\uffff\1\3\6\uffff\1\13\1\3\27\uffff"+
"\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\3\3\1"+
"\12\2\3\1\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3"+
"\3\uffff\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff"+
"\7\3\1\uffff\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\15"+
"\uffff\1\3\1\uffff\1\1\16\uffff\3\3\3\uffff\1\3\6\uffff\1\14\1\3\27\uffff"+
"\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\3\3\1"+
"\4\2\3\1\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3"+
"\3\uffff\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff"+
"\7\3\1\uffff\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\15"+
"\uffff\1\3\1\uffff\1\1\16\uffff\3\3\3\uffff\1\3\6\uffff\1\15\1\3\27\uffff"+
"\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"",
"\4\3\1\uffff\11\3\2\uffff\1\3\3\uffff\5\3\1\uffff\3\3\1\uffff\6\3\1"+
"\uffff\7\3\3\uffff\10\3\1\uffff\10\3\2\uffff\3\3\1\uffff\1\3\3\uffff"+
"\10\3\1\uffff\1\3\3\uffff\5\3\1\uffff\4\3\1\uffff\6\3\2\uffff\7\3\1\uffff"+
"\13\3\1\uffff\4\3\2\uffff\13\3\1\uffff\15\3\2\uffff\5\3\2\uffff\1\10"+
"\12\uffff\1\3\1\uffff\1\3\16\uffff\1\3\1\6\1\7\3\uffff\1\3\6\uffff\2"+
"\3\27\uffff\2\3\2\uffff\3\3\1\uffff\1\3\1\uffff\4\3\1\uffff\1\3",
"\1\uffff",
"\1\uffff",
"\1\uffff"
};
static final short[] DFA211_eot = DFA.unpackEncodedString(DFA211_eotS);
static final short[] DFA211_eof = DFA.unpackEncodedString(DFA211_eofS);
static final char[] DFA211_min = DFA.unpackEncodedStringToUnsignedChars(DFA211_minS);
static final char[] DFA211_max = DFA.unpackEncodedStringToUnsignedChars(DFA211_maxS);
static final short[] DFA211_accept = DFA.unpackEncodedString(DFA211_acceptS);
static final short[] DFA211_special = DFA.unpackEncodedString(DFA211_specialS);
static final short[][] DFA211_transition;
static {
int numStates = DFA211_transitionS.length;
DFA211_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA211_11 = input.LA(1);
int index211_11 = input.index();
input.rewind();
s = -1;
if ( (synpred293_Delphi()) ) {s = 9;}
else if ( (true) ) {s = 3;}
input.seek(index211_11);
if ( s>=0 ) return s;
break;
case 2 :
int LA211_12 = input.LA(1);
int index211_12 = input.index();
input.rewind();
s = -1;
if ( (synpred293_Delphi()) ) {s = 9;}
else if ( (true) ) {s = 3;}
input.seek(index211_12);
if ( s>=0 ) return s;
break;
case 3 :
int LA211_13 = input.LA(1);
int index211_13 = input.index();
input.rewind();
s = -1;
if ( (synpred293_Delphi()) ) {s = 9;}
else if ( (true) ) {s = 3;}
input.seek(index211_13);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 211, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA293_eotS =
"\154\uffff";
static final String DFA293_eofS =
"\1\32\153\uffff";
static final String DFA293_minS =
"\1\5\31\0\1\uffff\11\0\1\uffff\4\0\1\uffff\4\0\3\uffff\1\0\6\uffff\1\0"+
"\5\uffff\1\0\5\uffff\1\0\2\uffff\6\0\2\uffff\10\0\16\uffff\1\0\1\uffff"+
"\2\0\3\uffff";
static final String DFA293_maxS =
"\1\u00fa\31\0\1\uffff\11\0\1\uffff\4\0\1\uffff\4\0\3\uffff\1\0\6\uffff"+
"\1\0\5\uffff\1\0\5\uffff\1\0\2\uffff\6\0\2\uffff\10\0\16\uffff\1\0\1\uffff"+
"\2\0\3\uffff";
static final String DFA293_acceptS =
"\32\uffff\1\2\120\uffff\1\1";
static final String DFA293_specialS =
"\1\uffff\1\0\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15"+
"\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\uffff\1\31\1"+
"\32\1\33\1\34\1\35\1\36\1\37\1\40\1\41\1\uffff\1\42\1\43\1\44\1\45\1\uffff"+
"\1\46\1\47\1\50\1\51\3\uffff\1\52\6\uffff\1\53\5\uffff\1\54\5\uffff\1"+
"\55\2\uffff\1\56\1\57\1\60\1\61\1\62\1\63\2\uffff\1\64\1\65\1\66\1\67"+
"\1\70\1\71\1\72\1\73\16\uffff\1\74\1\uffff\1\75\1\76\3\uffff}>";
static final String[] DFA293_transitionS = {
"\1\70\1\41\1\4\1\150\2\uffff\1\32\1\uffff\1\32\1\42\1\150\1\32\1\150"+
"\1\113\2\uffff\1\32\3\uffff\1\32\1\43\1\32\1\uffff\1\32\1\uffff\2\32"+
"\1\150\1\uffff\1\114\1\30\1\45\1\13\1\32\1\52\7\uffff\1\36\3\uffff\4"+
"\32\1\46\1\43\1\32\1\147\1\uffff\1\50\1\22\1\41\3\32\1\145\1\26\2\uffff"+
"\1\32\3\uffff\1\150\3\uffff\2\32\1\123\1\32\1\27\1\24\2\32\5\uffff\1"+
"\55\2\uffff\1\32\1\50\1\uffff\1\34\1\3\3\uffff\1\17\1\50\1\15\1\125\1"+
"\1\3\uffff\1\32\1\126\1\61\1\uffff\1\150\1\31\1\37\1\uffff\1\150\1\32"+
"\1\20\1\32\1\23\1\32\1\43\1\47\1\2\1\110\1\25\1\uffff\1\32\1\107\1\111"+
"\1\112\2\uffff\1\32\1\117\1\121\1\32\1\76\1\43\1\33\1\32\2\150\1\32\1"+
"\uffff\1\43\1\150\2\32\2\uffff\1\20\1\32\1\35\1\43\1\124\1\104\1\21\3"+
"\uffff\1\32\1\uffff\2\32\15\uffff\1\7\1\uffff\1\12\16\uffff\1\6\1\16"+
"\1\5\3\uffff\1\14\6\uffff\1\11\1\10\27\uffff\1\54\1\32\2\uffff\1\32\1"+
"\53\1\40\1\uffff\1\32\1\uffff\1\43\1\32\1\120\1\122",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"\1\uffff",
"\1\uffff",
"",
"",
""
};
static final short[] DFA293_eot = DFA.unpackEncodedString(DFA293_eotS);
static final short[] DFA293_eof = DFA.unpackEncodedString(DFA293_eofS);
static final char[] DFA293_min = DFA.unpackEncodedStringToUnsignedChars(DFA293_minS);
static final char[] DFA293_max = DFA.unpackEncodedStringToUnsignedChars(DFA293_maxS);
static final short[] DFA293_accept = DFA.unpackEncodedString(DFA293_acceptS);
static final short[] DFA293_special = DFA.unpackEncodedString(DFA293_specialS);
static final short[][] DFA293_transition;
static {
int numStates = DFA293_transitionS.length;
DFA293_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA293_2 = input.LA(1);
int index293_2 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_2);
if ( s>=0 ) return s;
break;
case 2 :
int LA293_3 = input.LA(1);
int index293_3 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_3);
if ( s>=0 ) return s;
break;
case 3 :
int LA293_4 = input.LA(1);
int index293_4 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_4);
if ( s>=0 ) return s;
break;
case 4 :
int LA293_5 = input.LA(1);
int index293_5 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_5);
if ( s>=0 ) return s;
break;
case 5 :
int LA293_6 = input.LA(1);
int index293_6 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_6);
if ( s>=0 ) return s;
break;
case 6 :
int LA293_7 = input.LA(1);
int index293_7 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_7);
if ( s>=0 ) return s;
break;
case 7 :
int LA293_8 = input.LA(1);
int index293_8 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_8);
if ( s>=0 ) return s;
break;
case 8 :
int LA293_9 = input.LA(1);
int index293_9 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_9);
if ( s>=0 ) return s;
break;
case 9 :
int LA293_10 = input.LA(1);
int index293_10 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_10);
if ( s>=0 ) return s;
break;
case 10 :
int LA293_11 = input.LA(1);
int index293_11 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_11);
if ( s>=0 ) return s;
break;
case 11 :
int LA293_12 = input.LA(1);
int index293_12 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_12);
if ( s>=0 ) return s;
break;
case 12 :
int LA293_13 = input.LA(1);
int index293_13 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_13);
if ( s>=0 ) return s;
break;
case 13 :
int LA293_14 = input.LA(1);
int index293_14 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_14);
if ( s>=0 ) return s;
break;
case 14 :
int LA293_15 = input.LA(1);
int index293_15 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_15);
if ( s>=0 ) return s;
break;
case 15 :
int LA293_16 = input.LA(1);
int index293_16 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_16);
if ( s>=0 ) return s;
break;
case 16 :
int LA293_17 = input.LA(1);
int index293_17 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_17);
if ( s>=0 ) return s;
break;
case 17 :
int LA293_18 = input.LA(1);
int index293_18 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_18);
if ( s>=0 ) return s;
break;
case 18 :
int LA293_19 = input.LA(1);
int index293_19 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_19);
if ( s>=0 ) return s;
break;
case 19 :
int LA293_20 = input.LA(1);
int index293_20 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_20);
if ( s>=0 ) return s;
break;
case 20 :
int LA293_21 = input.LA(1);
int index293_21 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_21);
if ( s>=0 ) return s;
break;
case 21 :
int LA293_22 = input.LA(1);
int index293_22 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_22);
if ( s>=0 ) return s;
break;
case 22 :
int LA293_23 = input.LA(1);
int index293_23 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_23);
if ( s>=0 ) return s;
break;
case 23 :
int LA293_24 = input.LA(1);
int index293_24 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_24);
if ( s>=0 ) return s;
break;
case 24 :
int LA293_25 = input.LA(1);
int index293_25 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_25);
if ( s>=0 ) return s;
break;
case 25 :
int LA293_27 = input.LA(1);
int index293_27 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_27);
if ( s>=0 ) return s;
break;
case 26 :
int LA293_28 = input.LA(1);
int index293_28 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_28);
if ( s>=0 ) return s;
break;
case 27 :
int LA293_29 = input.LA(1);
int index293_29 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_29);
if ( s>=0 ) return s;
break;
case 28 :
int LA293_30 = input.LA(1);
int index293_30 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_30);
if ( s>=0 ) return s;
break;
case 29 :
int LA293_31 = input.LA(1);
int index293_31 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_31);
if ( s>=0 ) return s;
break;
case 30 :
int LA293_32 = input.LA(1);
int index293_32 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_32);
if ( s>=0 ) return s;
break;
case 31 :
int LA293_33 = input.LA(1);
int index293_33 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_33);
if ( s>=0 ) return s;
break;
case 32 :
int LA293_34 = input.LA(1);
int index293_34 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_34);
if ( s>=0 ) return s;
break;
case 33 :
int LA293_35 = input.LA(1);
int index293_35 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_35);
if ( s>=0 ) return s;
break;
case 34 :
int LA293_37 = input.LA(1);
int index293_37 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_37);
if ( s>=0 ) return s;
break;
case 35 :
int LA293_38 = input.LA(1);
int index293_38 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_38);
if ( s>=0 ) return s;
break;
case 36 :
int LA293_39 = input.LA(1);
int index293_39 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_39);
if ( s>=0 ) return s;
break;
case 37 :
int LA293_40 = input.LA(1);
int index293_40 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_40);
if ( s>=0 ) return s;
break;
case 38 :
int LA293_42 = input.LA(1);
int index293_42 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_42);
if ( s>=0 ) return s;
break;
case 39 :
int LA293_43 = input.LA(1);
int index293_43 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_43);
if ( s>=0 ) return s;
break;
case 40 :
int LA293_44 = input.LA(1);
int index293_44 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_44);
if ( s>=0 ) return s;
break;
case 41 :
int LA293_45 = input.LA(1);
int index293_45 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_45);
if ( s>=0 ) return s;
break;
case 42 :
int LA293_49 = input.LA(1);
int index293_49 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_49);
if ( s>=0 ) return s;
break;
case 43 :
int LA293_56 = input.LA(1);
int index293_56 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_56);
if ( s>=0 ) return s;
break;
case 44 :
int LA293_62 = input.LA(1);
int index293_62 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_62);
if ( s>=0 ) return s;
break;
case 45 :
int LA293_68 = input.LA(1);
int index293_68 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_68);
if ( s>=0 ) return s;
break;
case 46 :
int LA293_71 = input.LA(1);
int index293_71 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_71);
if ( s>=0 ) return s;
break;
case 47 :
int LA293_72 = input.LA(1);
int index293_72 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_72);
if ( s>=0 ) return s;
break;
case 48 :
int LA293_73 = input.LA(1);
int index293_73 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_73);
if ( s>=0 ) return s;
break;
case 49 :
int LA293_74 = input.LA(1);
int index293_74 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_74);
if ( s>=0 ) return s;
break;
case 50 :
int LA293_75 = input.LA(1);
int index293_75 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_75);
if ( s>=0 ) return s;
break;
case 51 :
int LA293_76 = input.LA(1);
int index293_76 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_76);
if ( s>=0 ) return s;
break;
case 52 :
int LA293_79 = input.LA(1);
int index293_79 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_79);
if ( s>=0 ) return s;
break;
case 53 :
int LA293_80 = input.LA(1);
int index293_80 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_80);
if ( s>=0 ) return s;
break;
case 54 :
int LA293_81 = input.LA(1);
int index293_81 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_81);
if ( s>=0 ) return s;
break;
case 55 :
int LA293_82 = input.LA(1);
int index293_82 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_82);
if ( s>=0 ) return s;
break;
case 56 :
int LA293_83 = input.LA(1);
int index293_83 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_83);
if ( s>=0 ) return s;
break;
case 57 :
int LA293_84 = input.LA(1);
int index293_84 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_84);
if ( s>=0 ) return s;
break;
case 58 :
int LA293_85 = input.LA(1);
int index293_85 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_85);
if ( s>=0 ) return s;
break;
case 59 :
int LA293_86 = input.LA(1);
int index293_86 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_86);
if ( s>=0 ) return s;
break;
case 60 :
int LA293_101 = input.LA(1);
int index293_101 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_101);
if ( s>=0 ) return s;
break;
case 61 :
int LA293_103 = input.LA(1);
int index293_103 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_103);
if ( s>=0 ) return s;
break;
case 62 :
int LA293_104 = input.LA(1);
int index293_104 = input.index();
input.rewind();
s = -1;
if ( (((!input.LT(1).getText().equals("name"))&&synpred435_Delphi())) ) {s = 107;}
else if ( (true) ) {s = 26;}
input.seek(index293_104);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 293, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA294_eotS =
"\154\uffff";
static final String DFA294_eofS =
"\1\1\153\uffff";
static final String DFA294_minS =
"\1\5\120\uffff\1\0\24\uffff\1\0\2\uffff\1\0\2\uffff";
static final String DFA294_maxS =
"\1\u00fa\120\uffff\1\0\24\uffff\1\0\2\uffff\1\0\2\uffff";
static final String DFA294_acceptS =
"\1\uffff\1\2\151\uffff\1\1";
static final String DFA294_specialS =
"\121\uffff\1\0\24\uffff\1\1\2\uffff\1\2\2\uffff}>";
static final String[] DFA294_transitionS = {
"\4\1\2\uffff\1\1\1\uffff\6\1\2\uffff\1\1\3\uffff\3\1\1\uffff\1\1\1\uffff"+
"\3\1\1\uffff\1\1\1\151\4\1\7\uffff\1\1\3\uffff\10\1\1\uffff\10\1\2\uffff"+
"\1\1\3\uffff\1\1\3\uffff\4\1\1\121\3\1\5\uffff\1\1\2\uffff\2\1\1\uffff"+
"\2\1\3\uffff\1\146\4\1\3\uffff\3\1\1\uffff\3\1\1\uffff\13\1\1\uffff\4"+
"\1\2\uffff\13\1\1\uffff\4\1\2\uffff\7\1\3\uffff\1\1\1\uffff\2\1\15\uffff"+
"\1\1\1\uffff\1\1\16\uffff\3\1\3\uffff\1\1\6\uffff\2\1\27\uffff\2\1\2"+
"\uffff\3\1\1\uffff\1\1\1\uffff\4\1",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
"",
"\1\uffff",
"",
""
};
static final short[] DFA294_eot = DFA.unpackEncodedString(DFA294_eotS);
static final short[] DFA294_eof = DFA.unpackEncodedString(DFA294_eofS);
static final char[] DFA294_min = DFA.unpackEncodedStringToUnsignedChars(DFA294_minS);
static final char[] DFA294_max = DFA.unpackEncodedStringToUnsignedChars(DFA294_maxS);
static final short[] DFA294_accept = DFA.unpackEncodedString(DFA294_acceptS);
static final short[] DFA294_special = DFA.unpackEncodedString(DFA294_specialS);
static final short[][] DFA294_transition;
static {
int numStates = DFA294_transitionS.length;
DFA294_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA294_102 = input.LA(1);
int index294_102 = input.index();
input.rewind();
s = -1;
if ( (synpred436_Delphi()) ) {s = 107;}
else if ( (true) ) {s = 1;}
input.seek(index294_102);
if ( s>=0 ) return s;
break;
case 2 :
int LA294_105 = input.LA(1);
int index294_105 = input.index();
input.rewind();
s = -1;
if ( (synpred436_Delphi()) ) {s = 107;}
else if ( (true) ) {s = 1;}
input.seek(index294_105);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 294, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA301_eotS =
"\u0086\uffff";
static final String DFA301_eofS =
"\1\2\u0085\uffff";
static final String DFA301_minS =
"\1\5\1\0\u0084\uffff";
static final String DFA301_maxS =
"\1\u00fc\1\0\u0084\uffff";
static final String DFA301_acceptS =
"\2\uffff\1\2\u0082\uffff\1\1";
static final String DFA301_specialS =
"\1\uffff\1\0\u0084\uffff}>";
static final String[] DFA301_transitionS = {
"\4\2\1\uffff\11\2\2\uffff\1\2\3\uffff\5\2\1\uffff\3\2\1\uffff\6\2\1\uffff"+
"\7\2\3\uffff\10\2\1\uffff\10\2\2\uffff\3\2\1\uffff\1\2\3\uffff\10\2\1"+
"\uffff\1\2\3\uffff\1\2\1\1\3\2\1\uffff\4\2\1\uffff\6\2\2\uffff\7\2\1"+
"\uffff\13\2\1\uffff\4\2\2\uffff\13\2\1\uffff\15\2\2\uffff\5\2\15\uffff"+
"\1\2\1\uffff\1\2\16\uffff\3\2\3\uffff\1\2\6\uffff\2\2\27\uffff\2\2\2"+
"\uffff\3\2\1\uffff\1\2\1\uffff\4\2\1\uffff\1\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA301_eot = DFA.unpackEncodedString(DFA301_eotS);
static final short[] DFA301_eof = DFA.unpackEncodedString(DFA301_eofS);
static final char[] DFA301_min = DFA.unpackEncodedStringToUnsignedChars(DFA301_minS);
static final char[] DFA301_max = DFA.unpackEncodedStringToUnsignedChars(DFA301_maxS);
static final short[] DFA301_accept = DFA.unpackEncodedString(DFA301_acceptS);
static final short[] DFA301_special = DFA.unpackEncodedString(DFA301_specialS);
static final short[][] DFA301_transition;
static {
int numStates = DFA301_transitionS.length;
DFA301_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 301, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA303_eotS =
"\u0086\uffff";
static final String DFA303_eofS =
"\1\2\u0085\uffff";
static final String DFA303_minS =
"\1\5\1\0\u0084\uffff";
static final String DFA303_maxS =
"\1\u00fc\1\0\u0084\uffff";
static final String DFA303_acceptS =
"\2\uffff\1\2\u0082\uffff\1\1";
static final String DFA303_specialS =
"\1\uffff\1\0\u0084\uffff}>";
static final String[] DFA303_transitionS = {
"\4\2\1\uffff\11\2\2\uffff\1\2\3\uffff\5\2\1\uffff\3\2\1\uffff\6\2\1\uffff"+
"\7\2\3\uffff\10\2\1\uffff\10\2\2\uffff\3\2\1\uffff\1\2\3\uffff\10\2\1"+
"\uffff\1\2\3\uffff\1\2\1\1\3\2\1\uffff\4\2\1\uffff\6\2\2\uffff\7\2\1"+
"\uffff\13\2\1\uffff\4\2\2\uffff\13\2\1\uffff\15\2\2\uffff\5\2\15\uffff"+
"\1\2\1\uffff\1\2\16\uffff\3\2\3\uffff\1\2\6\uffff\2\2\27\uffff\2\2\2"+
"\uffff\3\2\1\uffff\1\2\1\uffff\4\2\1\uffff\1\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA303_eot = DFA.unpackEncodedString(DFA303_eotS);
static final short[] DFA303_eof = DFA.unpackEncodedString(DFA303_eofS);
static final char[] DFA303_min = DFA.unpackEncodedStringToUnsignedChars(DFA303_minS);
static final char[] DFA303_max = DFA.unpackEncodedStringToUnsignedChars(DFA303_maxS);
static final short[] DFA303_accept = DFA.unpackEncodedString(DFA303_acceptS);
static final short[] DFA303_special = DFA.unpackEncodedString(DFA303_specialS);
static final short[][] DFA303_transition;
static {
int numStates = DFA303_transitionS.length;
DFA303_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 303, _s, input);
error(nvae);
throw nvae;
}
}
public static final BitSet FOLLOW_program_in_file405 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_library_in_file409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_unit_in_file413 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_package__in_file417 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_program_in_fileWithoutImplementation457 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_library_in_fileWithoutImplementation461 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_unitWithoutImplementation_in_fileWithoutImplementation465 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_package__in_fileWithoutImplementation469 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_programHead_in_program532 = new BitSet(new long[]{0x0420008188200000L,0x0202000010000010L,0x0000012008080002L,0x0002800000000000L});
public static final BitSet FOLLOW_usesFileClause_in_program535 = new BitSet(new long[]{0x0420008188200000L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_programBody_in_program538 = new BitSet(new long[]{0x0000200000000000L});
public static final BitSet FOLLOW_DOT_in_program540 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PROGRAM_in_programHead594 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_qualifiedNameDeclaration_in_programHead600 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_programParameters_in_programHead602 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_programHead605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_programParameters654 = new BitSet(new long[]{0xAB01013A0406C160L,0x70BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_ident_in_programParameters657 = new BitSet(new long[]{0x0000000020000000L,0x1000000000000000L});
public static final BitSet FOLLOW_COMMA_in_programParameters660 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_ident_in_programParameters662 = new BitSet(new long[]{0x0000000020000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_programParameters669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_localDeclSection_in_programBody724 = new BitSet(new long[]{0x0020000000200000L});
public static final BitSet FOLLOW_compoundStatement_in_programBody728 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_END_in_programBody732 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_libraryHead_in_library791 = new BitSet(new long[]{0x0420008188200000L,0x0202000010000010L,0x0000012008080002L,0x0002800000000000L});
public static final BitSet FOLLOW_usesFileClause_in_library793 = new BitSet(new long[]{0x0420008188200000L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_programBody_in_library796 = new BitSet(new long[]{0x0000200000000000L});
public static final BitSet FOLLOW_DOT_in_library798 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LIBRARY_in_libraryHead852 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_qualifiedNameDeclaration_in_libraryHead858 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_libraryHead861 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_libraryHead866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_packageHead_in_package_924 = new BitSet(new long[]{0x0020000200000000L,0x0200000000000000L,0x0000000008020000L});
public static final BitSet FOLLOW_requiresClause_in_package_926 = new BitSet(new long[]{0x0020000200000000L,0x0200000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_containsClause_in_package_929 = new BitSet(new long[]{0x0020000000000000L,0x0200000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_attributeList_in_package_932 = new BitSet(new long[]{0x0020000000000000L,0x0200000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_END_in_package_935 = new BitSet(new long[]{0x0000200000000000L});
public static final BitSet FOLLOW_DOT_in_package_937 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PACKAGE_in_packageHead991 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_qualifiedNameDeclaration_in_packageHead997 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_packageHead999 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_unitHead_in_unit1061 = new BitSet(new long[]{0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_unitInterface_in_unit1063 = new BitSet(new long[]{0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_unitImplementation_in_unit1065 = new BitSet(new long[]{0x0020000000200000L,0x0000000000200000L});
public static final BitSet FOLLOW_unitBlock_in_unit1067 = new BitSet(new long[]{0x0000200000000000L});
public static final BitSet FOLLOW_DOT_in_unit1069 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_unitHead_in_unitWithoutImplementation1109 = new BitSet(new long[]{0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_unitInterface_in_unitWithoutImplementation1111 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_UNIT_in_unitHead1168 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_qualifiedNameDeclaration_in_unitHead1174 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_unitHead1176 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_unitHead1179 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INTERFACE_in_unitInterface1232 = new BitSet(new long[]{0x0400008188000002L,0x0202000000000010L,0x0000012008080002L,0x0002800000000000L});
public static final BitSet FOLLOW_usesClause_in_unitInterface1238 = new BitSet(new long[]{0x0400008188000002L,0x0202000000000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_interfaceDecl_in_unitInterface1241 = new BitSet(new long[]{0x0400008188000002L,0x0202000000000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_IMPLEMENTATION_in_unitImplementation1289 = new BitSet(new long[]{0x0400008188000002L,0x0202000010000010L,0x0000012008080002L,0x0002800000000000L});
public static final BitSet FOLLOW_usesClause_in_unitImplementation1295 = new BitSet(new long[]{0x0400008188000002L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_declSection_in_unitImplementation1298 = new BitSet(new long[]{0x0400008188000002L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_initializationFinalization_in_unitBlock1355 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_unitBlock1358 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_compoundStatement_in_unitBlock1391 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_initializationSection_in_initializationFinalization1430 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000001L});
public static final BitSet FOLLOW_finalizationSection_in_initializationFinalization1432 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INITIALIZATION_in_initializationSection1477 = new BitSet(new long[]{0xEB01017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statementList_in_initializationSection1483 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FINALIZATION_in_finalizationSection1529 = new BitSet(new long[]{0xEB01017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statementList_in_finalizationSection1535 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONTAINS_in_containsClause1590 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_unitInFileImportList_in_containsClause1596 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_REQUIRES_in_requiresClause1647 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_unitImportList_in_requiresClause1653 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_USES_in_usesClause1708 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_unitImportList_in_usesClause1714 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_USES_in_usesFileClause1765 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_unitInFileImportList_in_usesFileClause1771 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_unitInFileImport_in_unitInFileImportList1816 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_COMMA_in_unitInFileImportList1819 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_unitInFileImport_in_unitInFileImportList1821 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_unitInFileImportList1825 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_unitImport_in_unitImportList1876 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_COMMA_in_unitImportList1879 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_unitImport_in_unitImportList1881 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_unitImportList1885 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_qualifiedNameDeclaration_in_unitImport1940 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_qualifiedNameDeclaration_in_unitInFileImport2029 = new BitSet(new long[]{0x0000000000000002L,0x0000000000040000L});
public static final BitSet FOLLOW_IN_in_unitInFileImport2032 = new BitSet(new long[]{0x0000004000000000L,0x0000000000000000L,0x0100000000000000L,0x0000000000102000L});
public static final BitSet FOLLOW_textLiteral_in_unitInFileImport2034 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_localDeclSection_in_block2147 = new BitSet(new long[]{0x0000000000202000L});
public static final BitSet FOLLOW_blockBody_in_block2150 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_declSection_in_localDeclSection2199 = new BitSet(new long[]{0x0400008188000002L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_compoundStatement_in_blockBody2268 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_assemblerStatement_in_blockBody2301 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_labelDeclSection_in_declSection2355 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_constSection_in_declSection2388 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeSection_in_declSection2421 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_varSection_in_declSection2454 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineImplementation_in_declSection2487 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_exportsSection_in_declSection2520 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_declSection2553 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_constSection_in_interfaceDecl2605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeSection_in_interfaceDecl2638 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_varSection_in_interfaceDecl2671 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_exportsSection_in_interfaceDecl2704 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineInterface_in_interfaceDecl2737 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_interfaceDecl2770 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LABEL_in_labelDeclSection2819 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00400001E066EC71L,0x068C200000000380L});
public static final BitSet FOLLOW_labelNameDeclaration_in_labelDeclSection2825 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_COMMA_in_labelDeclSection2828 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00400001E066EC71L,0x068C200000000380L});
public static final BitSet FOLLOW_labelNameDeclaration_in_labelDeclSection2830 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_labelDeclSection2834 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONST_in_constSection2888 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_RESOURCESTRING_in_constSection2896 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_constDeclaration_in_constSection2903 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_constDeclaration3043 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_constDeclaration3046 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_constDeclaration3048 = new BitSet(new long[]{0x0000000000000800L});
public static final BitSet FOLLOW_fixedArrayType_in_constDeclaration3050 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_EQUAL_in_constDeclaration3052 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L});
public static final BitSet FOLLOW_arrayExpression_in_constDeclaration3054 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_constDeclaration3056 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_constDeclaration3059 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_constDeclaration3142 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_constDeclaration3145 = new BitSet(new long[]{0x0040000010000000L});
public static final BitSet FOLLOW_COLON_in_constDeclaration3148 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_constDeclaration3150 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_EQUAL_in_constDeclaration3154 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_constExpression_in_constDeclaration3156 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_constDeclaration3158 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_constDeclaration3161 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TYPE_in_typeSection3266 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeDeclaration_in_typeSection3272 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_TYPE_in_innerTypeSection3322 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeDeclaration_in_innerTypeSection3328 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_typeDeclaration3379 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_genericNameDeclaration_in_typeDeclaration3382 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_EQUAL_in_typeDeclaration3384 = new BitSet(new long[]{0xEB01037A0C06C9E0L,0xEBBB4F8D109A0818L,0x01400103E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_typeDecl_in_typeDeclaration3386 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_typeDeclaration3388 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_typeDeclaration3391 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_in_varSection3495 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_THREADVAR_in_varSection3503 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_varDeclaration_in_varSection3510 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_varDeclaration_in_varSection3512 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_varDeclaration3564 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclarationList_in_varDeclaration3567 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_varDeclaration3569 = new BitSet(new long[]{0x0000000000000800L});
public static final BitSet FOLLOW_fixedArrayType_in_varDeclaration3571 = new BitSet(new long[]{0x0140002000000020L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_varDeclaration3573 = new BitSet(new long[]{0x0140002000000020L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_arrayVarValueSpec_in_varDeclaration3576 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_varDeclaration3579 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_varDeclaration3582 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_varDeclaration3663 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclarationList_in_varDeclaration3666 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_varDeclaration3668 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_varDeclaration3670 = new BitSet(new long[]{0x0140002000000020L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_varDeclaration3672 = new BitSet(new long[]{0x0140002000000020L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_varValueSpec_in_varDeclaration3675 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_varDeclaration3678 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_varDeclaration3681 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ABSOLUTE_in_arrayVarValueSpec3777 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_arrayVarValueSpec3779 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EQUAL_in_arrayVarValueSpec3812 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L});
public static final BitSet FOLLOW_arrayExpression_in_arrayVarValueSpec3814 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ABSOLUTE_in_varValueSpec3867 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_varValueSpec3869 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EQUAL_in_varValueSpec3902 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_constExpression_in_varValueSpec3904 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EXPORTS_in_exportsSection3955 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_ident_in_exportsSection3957 = new BitSet(new long[]{0x0000000020000000L,0x0800008000080000L,0x0000000000840000L});
public static final BitSet FOLLOW_exportItem_in_exportsSection3959 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_COMMA_in_exportsSection3962 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_ident_in_exportsSection3964 = new BitSet(new long[]{0x0000000020000000L,0x0800008000080000L,0x0000000000840000L});
public static final BitSet FOLLOW_exportItem_in_exportsSection3966 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_exportsSection3970 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_exportItem4026 = new BitSet(new long[]{0xAB01013A8406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_formalParameterList_in_exportItem4028 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_exportItem4030 = new BitSet(new long[]{0x0000000000000002L,0x0000008000080000L,0x0000000000040000L});
public static final BitSet FOLLOW_INDEX_in_exportItem4035 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_exportItem4037 = new BitSet(new long[]{0x0000000000000002L,0x0000008000000000L,0x0000000000040000L});
public static final BitSet FOLLOW_NAME_in_exportItem4042 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_exportItem4044 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000040000L});
public static final BitSet FOLLOW_RESIDENT_in_exportItem4049 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arrayType_in_typeDecl4112 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_setType_in_typeDecl4145 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullFileType_in_typeDecl4178 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_classHelperType_in_typeDecl4211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_classReferenceType_in_typeDecl4244 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_classType_in_typeDecl4277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_interfaceType_in_typeDecl4310 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_objectType_in_typeDecl4343 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordType_in_typeDecl4376 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordHelperType_in_typeDecl4409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_pointerType_in_typeDecl4442 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullStringType_in_typeDecl4475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureType_in_typeDecl4508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_subRangeType_in_typeDecl4541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeOfType_in_typeDecl4574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_strongAliasType_in_typeDecl4607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_weakAliasType_in_typeDecl4640 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_enumType_in_typeDecl4673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PACKED_in_typeDecl4706 = new BitSet(new long[]{0xEB01037A0C06C9E0L,0xEBBB4F8D109A0818L,0x01400103E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_typeDecl_in_typeDecl4708 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arrayType_in_varType4767 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_setType_in_varType4800 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullStringType_in_varType4833 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullFileType_in_varType4866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordType_in_varType4899 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_pointerType_in_varType4932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureType_in_varType4965 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_subRangeType_in_varType4998 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReference_in_varType5031 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_enumType_in_varType5064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PACKED_in_varType5097 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_varType5099 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_stringType_in_parameterType5152 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fileType_in_parameterType5185 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arrayType_in_parameterType5218 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReference_in_parameterType5251 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PACKED_in_parameterType5284 = new BitSet(new long[]{0xEB01013A0406C960L,0x61BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_parameterType_in_parameterType5286 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ARRAY_in_fixedArrayType5338 = new BitSet(new long[]{0x0000000000000000L,0x0200000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_arrayIndices_in_fixedArrayType5340 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_fixedArrayType5342 = new BitSet(new long[]{0xEB01017A8406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_arrayElementType_in_fixedArrayType5344 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ARRAY_in_arrayType5446 = new BitSet(new long[]{0x0000000000000000L,0x0200800000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_arrayIndices_in_arrayType5448 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_arrayType5451 = new BitSet(new long[]{0xEB01017A8406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_arrayElementType_in_arrayType5453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_lbrack_in_arrayIndices5736 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_arrayIndices5739 = new BitSet(new long[]{0xEB01017A2406C9E0L,0xEFBB0F8D101A0818L,0x01400003F966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_COMMA_in_arrayIndices5741 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEFBB0F8D101A0818L,0x01400003F966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_rbrack_in_arrayIndices5746 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONST_in_arrayElementType5845 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_varType_in_arrayElementType5881 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FILE_in_fileType5938 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FILE_in_fullFileType5995 = new BitSet(new long[]{0x0000000000000002L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_fullFileType6002 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_fullFileType6004 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SET_in_setType6064 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_setType6070 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_setType6072 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEREFERENCE_in_pointerType6126 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_pointerType6132 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRING_in_stringType6187 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRING_in_fullStringType6242 = new BitSet(new long[]{0x0000000000000002L,0x0200000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_lbrack_in_fullStringType6249 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_fullStringType6251 = new BitSet(new long[]{0x0000000000000000L,0x0400000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rbrack_in_fullStringType6253 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureOfObject_in_procedureType6307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureReference_in_procedureType6340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_simpleProcedureType_in_procedureType6373 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureTypeHeading_in_procedureOfObject6421 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_procedureOfObject6423 = new BitSet(new long[]{0x0000000000000000L,0x0000400000000000L});
public static final BitSet FOLLOW_OBJECT_in_procedureOfObject6425 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_procedureOfObject6433 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_procedureOfObject6437 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_REFERENCE_in_procedureReference6486 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000004000000000L});
public static final BitSet FOLLOW_TO_in_procedureReference6492 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_procedureTypeHeading_in_procedureReference6495 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureTypeHeading_in_simpleProcedureType6541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FUNCTION_in_procedureTypeHeading6597 = new BitSet(new long[]{0xAB01012014004042L,0x6830010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_procedureTypeHeading6603 = new BitSet(new long[]{0xAB01012014004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineReturnType_in_procedureTypeHeading6606 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_procedureTypeHeading6611 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_procedureTypeHeading6615 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_PROCEDURE_in_procedureTypeHeading6650 = new BitSet(new long[]{0xAB01012004004042L,0x6830010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_procedureTypeHeading6656 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_procedureTypeHeading6661 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_procedureTypeHeading6665 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_TYPE_in_typeOfType6722 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_typeOfType6728 = new BitSet(new long[]{0xEB01037A0C06C9E0L,0xEBBB4F8D109A0818L,0x01400103E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_typeDecl_in_typeOfType6730 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TYPE_in_strongAliasType6780 = new BitSet(new long[]{0xEB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReferenceOrStringOrFile_in_strongAliasType6786 = new BitSet(new long[]{0x0000000000000002L,0x0800000000000000L});
public static final BitSet FOLLOW_codePageExpression_in_strongAliasType6788 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_codePageExpression6836 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_codePageExpression6839 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_codePageExpression6841 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReference_in_weakAliasType6894 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_subRangeType6958 = new BitSet(new long[]{0x0000400000000000L});
public static final BitSet FOLLOW_DOT_DOT_in_subRangeType6960 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_subRangeType6966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_enumType7023 = new BitSet(new long[]{0xAB01013A0406C160L,0x70BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_enumTypeElement_in_enumType7030 = new BitSet(new long[]{0xAB01013A2406C160L,0x70BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_COMMA_in_enumType7033 = new BitSet(new long[]{0xAB01013A0406C160L,0x70BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_enumType7039 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nameDeclaration_in_enumTypeElement7090 = new BitSet(new long[]{0x0040000000000002L});
public static final BitSet FOLLOW_EQUAL_in_enumTypeElement7093 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_enumTypeElement7095 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nameReference_in_typeReference7163 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_stringType_in_typeReferenceOrString7218 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReference_in_typeReferenceOrString7251 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_stringType_in_typeReferenceOrStringOrFile7289 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fileType_in_typeReferenceOrStringOrFile7322 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReference_in_typeReferenceOrStringOrFile7355 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_classReferenceType7406 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_classReferenceType7412 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_classReferenceType7414 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_classType7470 = new BitSet(new long[]{0xAB2101BB8C06C162L,0x6ABB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_classState_in_classType7472 = new BitSet(new long[]{0xAB2101BB8C06C162L,0x6ABB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_classParent_in_classType7475 = new BitSet(new long[]{0xAB2101BB8C06C162L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySection_in_classType7479 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_END_in_classType7482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_classParent7678 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_classParent7680 = new BitSet(new long[]{0x0000000020000000L,0x1000000000000000L});
public static final BitSet FOLLOW_COMMA_in_classParent7683 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_classParent7685 = new BitSet(new long[]{0x0000000020000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_classParent7689 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_visibilitySection__in_visibilitySection7780 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_visibility_in_visibilitySection_7838 = new BitSet(new long[]{0xAB0101BB8C06C162L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySectionItem_in_visibilitySection_7840 = new BitSet(new long[]{0xAB0101BB8C06C162L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySectionItem_in_visibilitySection_7874 = new BitSet(new long[]{0xAB0101BB8C06C162L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_fieldSection_in_visibilitySectionItem7919 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineInterface_in_visibilitySectionItem7952 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_methodResolutionClause_in_visibilitySectionItem7985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_property_in_visibilitySectionItem8018 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_constSection_in_visibilitySectionItem8051 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_innerTypeSection_in_visibilitySectionItem8084 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_fieldSection8220 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000002000000000L,0x0002000000000000L});
public static final BitSet FOLLOW_fieldSectionKey_in_fieldSection8223 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_fieldDecl_in_fieldSection8225 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_fieldDecl_in_fieldSection8276 = new BitSet(new long[]{0xAB01013A0406C162L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_fieldDecl8345 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclarationList_in_fieldDecl8348 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_fieldDecl8350 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_fieldDecl8352 = new BitSet(new long[]{0x0100002000000002L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_fieldDecl8354 = new BitSet(new long[]{0x0100002000000002L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_fieldDecl8357 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_classHelperType8459 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000800L});
public static final BitSet FOLLOW_HELPER_in_classHelperType8465 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000004L});
public static final BitSet FOLLOW_classParent_in_classHelperType8467 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000004L});
public static final BitSet FOLLOW_FOR_in_classHelperType8470 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_classHelperType8472 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySection_in_classHelperType8474 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_END_in_classHelperType8477 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INTERFACE_in_interfaceType8530 = new BitSet(new long[]{0x0020008108000002L,0x0A02000000000010L,0x000000000800000AL});
public static final BitSet FOLLOW_DISPINTERFACE_in_interfaceType8538 = new BitSet(new long[]{0x0020008108000002L,0x0A02000000000010L,0x000000000800000AL});
public static final BitSet FOLLOW_classParent_in_interfaceType8545 = new BitSet(new long[]{0x0020008108000002L,0x0202000000000010L,0x000000000800000AL});
public static final BitSet FOLLOW_interfaceGuid_in_interfaceType8549 = new BitSet(new long[]{0x0020008108000000L,0x0202000000000010L,0x000000000800000AL});
public static final BitSet FOLLOW_interfaceItems_in_interfaceType8552 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_interfaceType8555 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_lbrack_in_interfaceGuid8609 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_interfaceGuid8611 = new BitSet(new long[]{0x0000000000000000L,0x0400000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rbrack_in_interfaceGuid8613 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_interfaceItem_in_interfaceItems8675 = new BitSet(new long[]{0x0000008108000002L,0x0202000000000010L,0x000000000800000AL});
public static final BitSet FOLLOW_routineInterface_in_interfaceItem8740 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_property_in_interfaceItem8773 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_OBJECT_in_objectType8828 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x6ABB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_classParent_in_objectType8834 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySection_in_objectType8837 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_END_in_objectType8840 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RECORD_in_recordType8961 = new BitSet(new long[]{0xAB2101BB8E06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySection_in_recordType8967 = new BitSet(new long[]{0xAB2101BB8E06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_recordVariantSection_in_recordType8970 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_recordType8973 = new BitSet(new long[]{0x0000000000000102L});
public static final BitSet FOLLOW_ALIGN_in_recordType8976 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_recordType8978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CASE_in_recordVariantSection9025 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_recordVariantTag_in_recordVariantSection9031 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_recordVariantSection9033 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_recordVariant_in_recordVariantSection9035 = new BitSet(new long[]{0xEB01017A0406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_nameDeclaration_in_recordVariantTag9086 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_recordVariantTag9088 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_recordVariantTag9092 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expressionList_in_recordVariant9187 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_recordVariant9189 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L});
public static final BitSet FOLLOW_PAREN_LEFT_in_recordVariant9191 = new BitSet(new long[]{0xAB01013A0606C160L,0x72BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_fieldDecl_in_recordVariant9193 = new BitSet(new long[]{0xAB01013A0606C160L,0x72BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_recordVariantSection_in_recordVariant9196 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_recordVariant9199 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_recordVariant9201 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RECORD_in_recordHelperType9300 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000800L});
public static final BitSet FOLLOW_HELPER_in_recordHelperType9306 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000004L});
public static final BitSet FOLLOW_FOR_in_recordHelperType9308 = new BitSet(new long[]{0xEB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReferenceOrStringOrFile_in_recordHelperType9310 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_visibilitySection_in_recordHelperType9312 = new BitSet(new long[]{0xAB2101BB8C06C160L,0x62BB0585100A0818L,0x00000121E86EEC7BL,0x068E200000000100L});
public static final BitSet FOLLOW_END_in_recordHelperType9315 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_property9372 = new BitSet(new long[]{0x0000000008000000L,0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_CLASS_in_property9375 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_PROPERTY_in_property9378 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_property9380 = new BitSet(new long[]{0x0000010810000000L,0x02000400000A0000L,0x0000000088800C00L,0x0600000000000000L});
public static final BitSet FOLLOW_propertyArray_in_property9382 = new BitSet(new long[]{0x0000010810000000L,0x00000400000A0000L,0x0000000080800C00L,0x0600000000000000L});
public static final BitSet FOLLOW_COLON_in_property9386 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_property9388 = new BitSet(new long[]{0x0000010800000000L,0x00000400000A0000L,0x0000000080800C00L,0x0600000000000000L});
public static final BitSet FOLLOW_propertyDirective_in_property9393 = new BitSet(new long[]{0x0000010800000000L,0x00000400000A0000L,0x0000000080800C00L,0x0600000000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_property9397 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_lbrack_in_propertyArray9504 = new BitSet(new long[]{0xAB01013A8406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_formalParameterList_in_propertyArray9507 = new BitSet(new long[]{0x0000000000000000L,0x0400000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rbrack_in_propertyArray9509 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_propertyDirective9558 = new BitSet(new long[]{0x0000000800000000L});
public static final BitSet FOLLOW_propertyDefaultNoExpression_in_propertyDirective9560 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_propertyDefault_in_propertyDirective9593 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_propertyReadWrite_in_propertyDirective9626 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_propertyDispInterface_in_propertyDirective9659 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_propertyImplements_in_propertyDirective9692 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_propertyIndex_in_propertyDirective9725 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_propertyStored_in_propertyDirective9758 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NODEFAULT_in_propertyDirective9791 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEFAULT_in_propertyDefaultNoExpression9829 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEFAULT_in_propertyDefault9883 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_propertyDefault9889 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_READ_in_propertyReadWrite9938 = new BitSet(new long[]{0xEB01017A0406C160L,0x6ABB0785101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_WRITE_in_propertyReadWrite9946 = new BitSet(new long[]{0xEB01017A0406C160L,0x6ABB0785101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_primaryExpression_in_propertyReadWrite9953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IMPLEMENTS_in_propertyImplements10000 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_propertyImplements10006 = new BitSet(new long[]{0x0000000020000002L});
public static final BitSet FOLLOW_COMMA_in_propertyImplements10009 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_propertyImplements10011 = new BitSet(new long[]{0x0000000020000002L});
public static final BitSet FOLLOW_INDEX_in_propertyIndex10065 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_propertyIndex10071 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STORED_in_propertyStored10122 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_propertyStored10128 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_READONLY_in_propertyDispInterface10172 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WRITEONLY_in_propertyDispInterface10205 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_dispIDDirective_in_propertyDispInterface10238 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRICT_in_visibility10293 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000010L});
public static final BitSet FOLLOW_PROTECTED_in_visibility10296 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRICT_in_visibility10333 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000001L});
public static final BitSet FOLLOW_PRIVATE_in_visibility10336 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PUBLIC_in_visibility10373 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PUBLISHED_in_visibility10409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_AUTOMATED_in_visibility10445 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LESS_THAN_in_genericDefinition10532 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeParameterList_in_genericDefinition10534 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000100L});
public static final BitSet FOLLOW_GREATER_THAN_in_genericDefinition10536 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeParameter_in_typeParameterList10624 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_typeParameterList10627 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeParameter_in_typeParameterList10630 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_nameDeclaration_in_typeParameter10685 = new BitSet(new long[]{0x0000000030000002L});
public static final BitSet FOLLOW_COMMA_in_typeParameter10688 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_typeParameter10690 = new BitSet(new long[]{0x0000000030000002L});
public static final BitSet FOLLOW_COLON_in_typeParameter10695 = new BitSet(new long[]{0xAB01013B0C06C160L,0x60BB0585100A0808L,0x00000001E066FC71L,0x068C200000000100L});
public static final BitSet FOLLOW_genericConstraint_in_typeParameter10697 = new BitSet(new long[]{0x0000000020000002L});
public static final BitSet FOLLOW_COMMA_in_typeParameter10700 = new BitSet(new long[]{0xAB01013B0C06C160L,0x60BB0585100A0808L,0x00000001E066FC71L,0x068C200000000100L});
public static final BitSet FOLLOW_genericConstraint_in_typeParameter10702 = new BitSet(new long[]{0x0000000020000002L});
public static final BitSet FOLLOW_typeReference_in_genericConstraint10877 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RECORD_in_genericConstraint10910 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_genericConstraint10943 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONSTRUCTOR_in_genericConstraint10976 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LESS_THAN_in_genericArguments11025 = new BitSet(new long[]{0xEB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReferenceOrStringOrFile_in_genericArguments11027 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000100L});
public static final BitSet FOLLOW_COMMA_in_genericArguments11030 = new BitSet(new long[]{0xEB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReferenceOrStringOrFile_in_genericArguments11032 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000100L});
public static final BitSet FOLLOW_GREATER_THAN_in_genericArguments11036 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LESS_THAN_in_routineNameGenericArguments11125 = new BitSet(new long[]{0xEB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReferenceOrStringOrFile_in_routineNameGenericArguments11127 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000100L,0x0000000000800000L});
public static final BitSet FOLLOW_commaOrSemicolon_in_routineNameGenericArguments11130 = new BitSet(new long[]{0xEB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReferenceOrStringOrFile_in_routineNameGenericArguments11132 = new BitSet(new long[]{0x0000000020000000L,0x0000000000000100L,0x0000000000800000L});
public static final BitSet FOLLOW_GREATER_THAN_in_routineNameGenericArguments11136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FUNCTION_in_methodResolutionClause11290 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_PROCEDURE_in_methodResolutionClause11294 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameReference_in_methodResolutionClause11299 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_EQUAL_in_methodResolutionClause11301 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameReference_in_methodResolutionClause11305 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_methodResolutionClause11307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineInterfaceHeading_in_routineInterface11473 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullRoutineImplementation_in_routineImplementation11627 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_externalRoutine_in_routineImplementation11660 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_forwardRoutine_in_routineImplementation11693 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineImplementationHeading_in_fullRoutineImplementation11733 = new BitSet(new long[]{0x0400008188202000L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_routineBody_in_fullRoutineImplementation11735 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_externalRoutineHeading_in_externalRoutine11933 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_forwardRoutineHeading_in_forwardRoutine12094 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_routineInterfaceHeading12246 = new BitSet(new long[]{0x0000008108000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_routineInterfaceHeading12249 = new BitSet(new long[]{0x0000008100000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_routineKey_in_routineInterfaceHeading12252 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100E0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_routineDeclarationName_in_routineInterfaceHeading12254 = new BitSet(new long[]{0xAB01012014004040L,0x6830010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_routineInterfaceHeading12256 = new BitSet(new long[]{0xAB01012014004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineReturnType_in_routineInterfaceHeading12259 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirectiveSection_in_routineInterfaceHeading12262 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_routineImplementationHeading12641 = new BitSet(new long[]{0x0000008108000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_routineImplementationHeading12644 = new BitSet(new long[]{0x0000008100000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_routineKey_in_routineImplementationHeading12647 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_routineImplementationName_in_routineImplementationHeading12649 = new BitSet(new long[]{0xA301012014004040L,0x6830010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_routineImplementationHeading12651 = new BitSet(new long[]{0xA301012014004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineReturnType_in_routineImplementationHeading12654 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirectiveSection_in_routineImplementationHeading12657 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_externalRoutineHeading13042 = new BitSet(new long[]{0x0000008108000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_externalRoutineHeading13045 = new BitSet(new long[]{0x0000008100000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_routineKey_in_externalRoutineHeading13048 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_routineImplementationName_in_externalRoutineHeading13050 = new BitSet(new long[]{0xAB01012014004040L,0x6830010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_externalRoutineHeading13052 = new BitSet(new long[]{0xAB01012014004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineReturnType_in_externalRoutineHeading13055 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_externalDirectiveSection_in_externalRoutineHeading13058 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_forwardRoutineHeading13444 = new BitSet(new long[]{0x0000008108000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_CLASS_in_forwardRoutineHeading13447 = new BitSet(new long[]{0x0000008100000000L,0x0002000000000010L,0x0000000000000002L});
public static final BitSet FOLLOW_routineKey_in_forwardRoutineHeading13450 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100E0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_routineDeclarationName_in_forwardRoutineHeading13452 = new BitSet(new long[]{0xA301012014004040L,0x6830010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_forwardRoutineHeading13454 = new BitSet(new long[]{0xA301012014004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineReturnType_in_forwardRoutineHeading13457 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_forwardDirectiveSection_in_forwardRoutineHeading13460 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_genericNameDeclaration_in_routineDeclarationName13882 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_specialOpNameDeclaration_in_routineDeclarationName13919 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineNameReference_in_routineImplementationName14031 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_COLON_in_routineReturnType14277 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000003E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_routineReturnType14279 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_returnType_in_routineReturnType14282 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReferenceOrString_in_returnType14351 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_routineParameters14399 = new BitSet(new long[]{0xAB01013A8406C160L,0x72BB0585100A0808L,0x00000001E866EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_formalParameterList_in_routineParameters14401 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_routineParameters14404 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_formalParameter_in_formalParameterList14466 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_formalParameterList14469 = new BitSet(new long[]{0xAB01013A8406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_formalParameter_in_formalParameterList14471 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_attributeList_in_formalParameter14539 = new BitSet(new long[]{0xAB01013A8406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_paramSpecifier_in_formalParameter14543 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_formalParameter14547 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclarationList_in_formalParameter14552 = new BitSet(new long[]{0x0040000010000002L});
public static final BitSet FOLLOW_COLON_in_formalParameter14555 = new BitSet(new long[]{0xEB01013A0406C960L,0x61BB0585100A0808L,0x00000003E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_parameterType_in_formalParameter14557 = new BitSet(new long[]{0x0040000000000002L});
public static final BitSet FOLLOW_EQUAL_in_formalParameter14562 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_formalParameter14564 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_block_in_routineBody14794 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_routineBody14796 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeGroup_in_attributeList14863 = new BitSet(new long[]{0x0000000000000002L,0x0200000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_lbrack_in_attributeGroup14956 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attribute_in_attributeGroup14959 = new BitSet(new long[]{0xAB01013A2406C160L,0x64BB0585100A0808L,0x00000001F066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_COMMA_in_attributeGroup14961 = new BitSet(new long[]{0xAB01013A0406C160L,0x64BB0585100A0808L,0x00000001F066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_rbrack_in_attributeGroup14966 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ASSEMBLY_in_attribute15064 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_attribute15066 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameReference_in_attribute15070 = new BitSet(new long[]{0x0000000010000002L,0x0800000000000000L});
public static final BitSet FOLLOW_argumentList_in_attribute15072 = new BitSet(new long[]{0x0000000010000002L});
public static final BitSet FOLLOW_COLON_in_attribute15076 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameReference_in_attribute15078 = new BitSet(new long[]{0x0000000010000002L,0x0800000000000000L});
public static final BitSet FOLLOW_argumentList_in_attribute15080 = new BitSet(new long[]{0x0000000010000002L});
public static final BitSet FOLLOW_relationalExpression_in_expression15198 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_anonymousMethod_in_expression15231 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_additiveExpression_in_relationalExpression15281 = new BitSet(new long[]{0x0040000000000002L,0x0000100061040300L});
public static final BitSet FOLLOW_relationalOperator_in_relationalExpression15284 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_additiveExpression_in_relationalExpression15287 = new BitSet(new long[]{0x0040000000000002L,0x0000100061040300L});
public static final BitSet FOLLOW_multiplicativeExpression_in_additiveExpression15338 = new BitSet(new long[]{0x0000000000000002L,0x8004000800000000L,0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_addOperator_in_additiveExpression15341 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_multiplicativeExpression_in_additiveExpression15344 = new BitSet(new long[]{0x0000000000000002L,0x8004000800000000L,0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_unaryExpression_in_multiplicativeExpression15389 = new BitSet(new long[]{0x00000C0000001402L,0x0000003000000000L,0x0000000006000000L});
public static final BitSet FOLLOW_multOperator_in_multiplicativeExpression15392 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_unaryExpression_in_multiplicativeExpression15395 = new BitSet(new long[]{0x00000C0000001402L,0x0000003000000000L,0x0000000006000000L});
public static final BitSet FOLLOW_unaryOperator_in_unaryExpression15449 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_unaryExpression_in_unaryExpression15452 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_primaryExpression_in_unaryExpression15485 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_atom_in_primaryExpression15533 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_parenthesizedExpression_in_primaryExpression15577 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INHERITED_in_primaryExpression15610 = new BitSet(new long[]{0xAB01217A0406C162L,0x6ABB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameReference_in_primaryExpression15613 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_particleItem_in_primaryExpression15616 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_PAREN_LEFT_in_parenthesizedExpression15681 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_parenthesizedExpression15683 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_parenthesizedExpression15685 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_particle_in_atom15761 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_particleItem_in_atom15763 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_intNum_in_particle15821 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_realNum_in_particle15854 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_textLiteral_in_particle15887 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nilLiteral_in_particle15920 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nameReference_in_particle15953 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arrayConstructor_in_particle15986 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRING_in_particle16019 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FILE_in_particle16052 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_parenthesizedExpression_in_particle16085 = new BitSet(new long[]{0x0000204000000000L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_particleItem_in_particle16087 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_DOT_in_particleItem16206 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedNameReference_in_particleItem16208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_argumentList_in_particleItem16241 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arrayAccessor_in_particleItem16274 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEREFERENCE_in_particleItem16307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_lbrack_in_arrayAccessor16359 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expressionList_in_arrayAccessor16361 = new BitSet(new long[]{0x0000000000000000L,0x0400000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rbrack_in_arrayAccessor16363 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_argumentList16456 = new BitSet(new long[]{0xEFB197FB8E26FDE0L,0xFBBFCF9D91FF889FL,0x014001F3EF6FFE7FL,0x17AEF00000302380L});
public static final BitSet FOLLOW_argument_in_argumentList16463 = new BitSet(new long[]{0x0000000020000000L,0x1000000000000000L});
public static final BitSet FOLLOW_COMMA_in_argumentList16466 = new BitSet(new long[]{0xEFB197FB8E26FDE0L,0xEBBFCF9D91FF889FL,0x014001F3EF6FFE7FL,0x17AEF00000302380L});
public static final BitSet FOLLOW_argument_in_argumentList16468 = new BitSet(new long[]{0x0000000020000000L,0x1000000000000000L});
public static final BitSet FOLLOW_COMMA_in_argumentList16472 = new BitSet(new long[]{0x0000000000000000L,0x1000000000000000L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_argumentList16477 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_argumentName_in_argument16534 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_argumentExpression_in_argument16537 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_argumentName16633 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_argumentName16635 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_keywords_in_argumentName16669 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_argumentName16671 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_argumentExpression16724 = new BitSet(new long[]{0x0000000010000002L});
public static final BitSet FOLLOW_writeArguments_in_argumentExpression16726 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_COLON_in_writeArguments16778 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_writeArguments16781 = new BitSet(new long[]{0x0000000010000002L});
public static final BitSet FOLLOW_COLON_in_writeArguments16784 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_writeArguments16787 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_anonymousMethodHeading_in_anonymousMethod16840 = new BitSet(new long[]{0x0400008188202000L,0x0202000010000010L,0x0000012008080002L,0x0002000000000000L});
public static final BitSet FOLLOW_block_in_anonymousMethod16842 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PROCEDURE_in_anonymousMethodHeading16898 = new BitSet(new long[]{0xAB01012004004042L,0x6830010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_routineParameters_in_anonymousMethodHeading16900 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_anonymousMethodHeading16905 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_anonymousMethodHeading16909 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_FUNCTION_in_anonymousMethodHeading16997 = new BitSet(new long[]{0x0000000010000000L,0x0800000000000000L});
public static final BitSet FOLLOW_routineParameters_in_anonymousMethodHeading16999 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_routineReturnType_in_anonymousMethodHeading17002 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_anonymousMethodHeading17006 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_anonymousMethodHeading17010 = new BitSet(new long[]{0xAB01012004004042L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_expression_in_expressionOrRange17115 = new BitSet(new long[]{0x0000400000000002L});
public static final BitSet FOLLOW_DOT_DOT_in_expressionOrRange17118 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_expressionOrRange17124 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_expressionList17178 = new BitSet(new long[]{0xEB01017A2406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_COMMA_in_expressionList17181 = new BitSet(new long[]{0xEB01017A0406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expressionOrRange_in_expressionOrRangeList17231 = new BitSet(new long[]{0xEB01017A2406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_COMMA_in_expressionOrRangeList17234 = new BitSet(new long[]{0xEB01017A0406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_singleLineTextLiteral_in_textLiteral17293 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_multilineTextLiteral_in_textLiteral17337 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkQuotedString_in_singleLineTextLiteral17392 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_escapedCharacter_in_singleLineTextLiteral17395 = new BitSet(new long[]{0x0000004000000000L,0x0000000000000000L,0x0100000000000000L,0x0000000000100000L});
public static final BitSet FOLLOW_TkQuotedString_in_singleLineTextLiteral17398 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_escapedCharacter_in_singleLineTextLiteral17402 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_escapedCharacter_in_singleLineTextLiteral17436 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L,0x0000000000100000L});
public static final BitSet FOLLOW_TkQuotedString_in_singleLineTextLiteral17440 = new BitSet(new long[]{0x0000004000000000L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_escapedCharacter_in_singleLineTextLiteral17442 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L,0x0000000000100000L});
public static final BitSet FOLLOW_TkQuotedString_in_singleLineTextLiteral17447 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkMultilineString_in_multilineTextLiteral17493 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkCharacterEscapeCode_in_escapedCharacter17542 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEREFERENCE_in_escapedCharacter17575 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000080000000000L,0x0000000000000300L});
public static final BitSet FOLLOW_TkIdentifier_in_escapedCharacter17578 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkIntNumber_in_escapedCharacter17582 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkAnyChar_in_escapedCharacter17586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NIL_in_nilLiteral17648 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_lbrack_in_arrayConstructor17700 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEEBB0F8D101A0818L,0x01400003F866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expressionOrRangeList_in_arrayConstructor17702 = new BitSet(new long[]{0x0000000000000000L,0x0400000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rbrack_in_arrayConstructor17705 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PLUS_in_addOperator17804 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MINUS_in_addOperator17840 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_OR_in_addOperator17876 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_XOR_in_addOperator17912 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MULTIPLY_in_multOperator17968 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DIVIDE_in_multOperator18004 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DIV_in_multOperator18040 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MOD_in_multOperator18076 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_AND_in_multOperator18112 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SHL_in_multOperator18148 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SHR_in_multOperator18184 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_AS_in_multOperator18220 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NOT_in_unaryOperator18275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PLUS_in_unaryOperator18311 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MINUS_in_unaryOperator18347 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ADDRESS_in_unaryOperator18383 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EQUAL_in_relationalOperator18433 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_GREATER_THAN_in_relationalOperator18469 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LESS_THAN_in_relationalOperator18505 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LESS_THAN_EQUAL_in_relationalOperator18541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_GREATER_THAN_EQUAL_in_relationalOperator18577 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NOT_EQUAL_in_relationalOperator18613 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IN_in_relationalOperator18649 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IS_in_relationalOperator18685 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_constExpression18738 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordExpression_in_constExpression18771 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_arrayExpression_in_constExpression18804 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_recordExpression18853 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_recordExpressionItem_in_recordExpression18860 = new BitSet(new long[]{0xAB01013A0406C160L,0x70BB0585100A0808L,0x00000001E0E6EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_SEMICOLON_in_recordExpression18863 = new BitSet(new long[]{0xAB01013A0406C160L,0x70BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_recordExpression18869 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_recordExpressionItem18914 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_recordExpressionItem18916 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_constExpression_in_recordExpressionItem18918 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PAREN_LEFT_in_arrayExpression19010 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xFABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_constExpression_in_arrayExpression19017 = new BitSet(new long[]{0xEB01017A2406C1E0L,0xFABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_COMMA_in_arrayExpression19020 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xFABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_PAREN_RIGHT_in_arrayExpression19027 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ifStatement_in_statement19087 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_varStatement_in_statement19120 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_constStatement_in_statement19153 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_caseStatement_in_statement19186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_repeatStatement_in_statement19219 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_whileStatement_in_statement19252 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_forStatement_in_statement19285 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_withStatement_in_statement19318 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_tryStatement_in_statement19351 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_raiseStatement_in_statement19384 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_assemblerStatement_in_statement19417 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_compoundStatement_in_statement19450 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_labelStatement_in_statement19483 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_assignmentStatement_in_statement19516 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expressionStatement_in_statement19549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_gotoStatement_in_statement19582 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IF_in_ifStatement19636 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_ifStatement19642 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000001000000000L});
public static final BitSet FOLLOW_THEN_in_ifStatement19644 = new BitSet(new long[]{0xEB11017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_ifStatement19646 = new BitSet(new long[]{0x0010000000000002L});
public static final BitSet FOLLOW_ELSE_in_ifStatement19650 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_ifStatement19652 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_in_varStatement19708 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_varStatement19710 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclarationList_in_varStatement19713 = new BitSet(new long[]{0x0000000010010002L});
public static final BitSet FOLLOW_COLON_in_varStatement19716 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_varStatement19718 = new BitSet(new long[]{0x0000000000010002L});
public static final BitSet FOLLOW_ASSIGN_in_varStatement19723 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_varStatement19725 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONST_in_constStatement19835 = new BitSet(new long[]{0xAB01013A0406C160L,0x62BB0585100A0808L,0x00000001E866EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_attributeList_in_constStatement19837 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_constStatement19840 = new BitSet(new long[]{0x0040000010000000L});
public static final BitSet FOLLOW_COLON_in_constStatement19843 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_constStatement19845 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_EQUAL_in_constStatement19849 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_constStatement19851 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CASE_in_caseStatement19957 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_caseStatement19963 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_OF_in_caseStatement19965 = new BitSet(new long[]{0xEB31017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_caseItem_in_caseStatement19967 = new BitSet(new long[]{0xEB31017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_elseBlock_in_caseStatement19970 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_caseStatement19973 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ELSE_in_elseBlock20029 = new BitSet(new long[]{0xEB01017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statementList_in_elseBlock20035 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expressionOrRangeList_in_caseItem20092 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_caseItem20094 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_caseItem20097 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_caseItem20102 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_REPEAT_in_repeatStatement20176 = new BitSet(new long[]{0xEB01017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE600000302380L});
public static final BitSet FOLLOW_statementList_in_repeatStatement20182 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000000L,0x0000400000000000L});
public static final BitSet FOLLOW_UNTIL_in_repeatStatement20184 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_repeatStatement20186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WHILE_in_whileStatement20237 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_whileStatement20243 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_whileStatement20245 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_whileStatement20247 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FOR_in_forStatement20301 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_forVar_in_forStatement20307 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_forStatement20309 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_forStatement20311 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000004000000000L});
public static final BitSet FOLLOW_TO_in_forStatement20313 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_forStatement20315 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_forStatement20317 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_forStatement20319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FOR_in_forStatement20353 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_forVar_in_forStatement20359 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_forStatement20361 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_forStatement20363 = new BitSet(new long[]{0x0000800000000000L});
public static final BitSet FOLLOW_DOWNTO_in_forStatement20365 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_forStatement20367 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_forStatement20369 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_forStatement20371 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FOR_in_forStatement20405 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_forVar_in_forStatement20411 = new BitSet(new long[]{0x0000000000000000L,0x0000000000040000L});
public static final BitSet FOLLOW_IN_in_forStatement20413 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_forStatement20415 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_forStatement20417 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_forStatement20419 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_in_forVar20479 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_forVar20481 = new BitSet(new long[]{0x0000000010000002L});
public static final BitSet FOLLOW_COLON_in_forVar20484 = new BitSet(new long[]{0xEB01017A0406C9E0L,0xEBBB0F8D101A0818L,0x01400003E966FC73L,0x068C200000302380L});
public static final BitSet FOLLOW_varType_in_forVar20486 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_simpleNameReference_in_forVar20535 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WITH_in_withStatement20598 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expressionList_in_withStatement20604 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_withStatement20606 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_withStatement20608 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_BEGIN_in_compoundStatement20657 = new BitSet(new long[]{0xEB21017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statementList_in_compoundStatement20663 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_compoundStatement20665 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_delimitedStatements_in_statementList20717 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_delimitedStatements20777 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_SEMICOLON_in_delimitedStatements20781 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_labelNameReference_in_labelStatement20838 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_labelStatement20840 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_labelStatement20842 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_assignmentStatement20934 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_assignmentStatement20936 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_assignmentStatement20942 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_expressionStatement20988 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_GOTO_in_gotoStatement21051 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00400001E066EC71L,0x068C200000000380L});
public static final BitSet FOLLOW_labelNameReference_in_gotoStatement21057 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TRY_in_tryStatement21110 = new BitSet(new long[]{0xEB81017A8626E1E0L,0xEABB0F8D101A889EL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statementList_in_tryStatement21116 = new BitSet(new long[]{0x0080000000000000L,0x0000000000000002L});
public static final BitSet FOLLOW_exceptBlock_in_tryStatement21119 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_finallyBlock_in_tryStatement21123 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_tryStatement21126 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EXCEPT_in_exceptBlock21180 = new BitSet(new long[]{0xEB01017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_handlerList_in_exceptBlock21186 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FINALLY_in_finallyBlock21239 = new BitSet(new long[]{0xEB01017A8626E1E0L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statementList_in_finallyBlock21245 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_handler_in_handlerList21299 = new BitSet(new long[]{0x0010000000000002L,0x0001000000000000L});
public static final BitSet FOLLOW_elseBlock_in_handlerList21302 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statementList_in_handlerList21336 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ON_in_handler21394 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_handler21401 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_handler21403 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_typeReference_in_handler21408 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_handler21410 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E8E7EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_handler21412 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_handler21416 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_RAISE_in_raiseStatement21469 = new BitSet(new long[]{0xEB01017A0406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_raiseStatement21475 = new BitSet(new long[]{0x0000000000020002L});
public static final BitSet FOLLOW_AT_in_raiseStatement21479 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_raiseStatement21482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ASM_in_assemblerStatement21531 = new BitSet(new long[]{0xFFFFFFFFFFFFFFF0L,0xFFFFFFFFFFFFFFFFL,0xFFFFFFFFFFFFFFFFL,0x7FFFFFFFFFFFFFFFL});
public static final BitSet FOLLOW_assemblerInstructions_in_assemblerStatement21537 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_END_in_assemblerStatement21539 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_implDirectiveSection21638 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_implDirectiveSection21641 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_implDirectiveSection21645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_implDirectiveSection21679 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_implDirectiveSection21681 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_interfaceDirectiveSection21724 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_interfaceDirectiveSection21727 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_interfaceDirectiveSection21731 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_interfaceDirectiveSection21765 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_interfaceDirectiveSection21767 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21812 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_externalDirectiveSection21815 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21819 = new BitSet(new long[]{0x0800000000000000L});
public static final BitSet FOLLOW_externalDirective_in_externalDirectiveSection21822 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21825 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_externalDirectiveSection21828 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21831 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21865 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_externalDirectiveSection21867 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21871 = new BitSet(new long[]{0x0800000000000000L});
public static final BitSet FOLLOW_externalDirective_in_externalDirectiveSection21873 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_externalDirectiveSection21876 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_externalDirectiveSection21878 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21923 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_forwardDirectiveSection21926 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21930 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_FORWARD_in_forwardDirectiveSection21933 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21936 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_forwardDirectiveSection21939 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21942 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21976 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_forwardDirectiveSection21978 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21982 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_FORWARD_in_forwardDirectiveSection21984 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_forwardDirectiveSection21987 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_forwardDirectiveSection21989 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_OVERLOAD_in_implDirective22043 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_REINTRODUCE_in_implDirective22076 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_bindingDirective_in_implDirective22109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_abstractDirective_in_implDirective22142 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_inlineDirective_in_implDirective22175 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_callConvention_in_implDirective22208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_portabilityDirective_in_implDirective22241 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_oldCallConventionDirective_in_implDirective22274 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_dispIDDirective_in_implDirective22307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VARARGS_in_implDirective22340 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_UNSAFE_in_implDirective22374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FORWARD_in_interfaceDirective22422 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_externalDirective_in_interfaceDirective22455 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_implDirective_in_interfaceDirective22488 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MESSAGE_in_bindingDirective22537 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_bindingDirective22539 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STATIC_in_bindingDirective22572 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DYNAMIC_in_bindingDirective22605 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_OVERRIDE_in_bindingDirective22638 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VIRTUAL_in_bindingDirective22671 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEPRECATED_in_portabilityDirective23239 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L,0x0000000000102000L});
public static final BitSet FOLLOW_textLiteral_in_portabilityDirective23242 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EXPERIMENTAL_in_portabilityDirective23276 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PLATFORM_in_portabilityDirective23309 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LIBRARY_in_portabilityDirective23342 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EXTERNAL_in_externalDirective23390 = new BitSet(new long[]{0xEB01017A0406C1E2L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_dllName_in_externalDirective23393 = new BitSet(new long[]{0x0000001000000002L,0x0000008000080000L});
public static final BitSet FOLLOW_externalSpecifier_in_externalDirective23396 = new BitSet(new long[]{0x0000001000000002L,0x0000008000080000L});
public static final BitSet FOLLOW_expression_in_dllName23457 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NAME_in_externalSpecifier23505 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_externalSpecifier23508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INDEX_in_externalSpecifier23541 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_externalSpecifier23544 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DELAYED_in_externalSpecifier23578 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DISPID_in_dispIDDirective23629 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_dispIDDirective23631 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkIdentifier_in_ident23695 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_keywordsUsedAsNames_in_ident23728 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nameDeclaration_in_nameDeclarationList25200 = new BitSet(new long[]{0x0000000020000002L});
public static final BitSet FOLLOW_COMMA_in_nameDeclarationList25203 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_nameDeclarationList25205 = new BitSet(new long[]{0x0000000020000002L});
public static final BitSet FOLLOW_ident_in_nameDeclaration25275 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_genericNameDeclaration25329 = new BitSet(new long[]{0x0000200000000002L,0x0000000020000000L});
public static final BitSet FOLLOW_DOT_in_genericNameDeclaration25332 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedIdent_in_genericNameDeclaration25334 = new BitSet(new long[]{0x0000200000000002L,0x0000000020000000L});
public static final BitSet FOLLOW_genericDefinition_in_genericNameDeclaration25338 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_qualifiedNameDeclaration25426 = new BitSet(new long[]{0x0000200000000002L});
public static final BitSet FOLLOW_DOT_in_qualifiedNameDeclaration25429 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedIdent_in_qualifiedNameDeclaration25431 = new BitSet(new long[]{0x0000200000000002L});
public static final BitSet FOLLOW_specialOperatorName_in_specialOpNameDeclaration25517 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IN_in_specialOperatorName25603 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_nameReference25661 = new BitSet(new long[]{0x0000200000000002L,0x0000000020000000L});
public static final BitSet FOLLOW_genericArguments_in_nameReference25663 = new BitSet(new long[]{0x0000200000000002L});
public static final BitSet FOLLOW_DOT_in_nameReference25667 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedNameReference_in_nameReference25669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_simpleNameReference25767 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_extendedIdent_in_extendedNameReference25851 = new BitSet(new long[]{0x0000200000000002L,0x0000000020000000L});
public static final BitSet FOLLOW_genericArguments_in_extendedNameReference25853 = new BitSet(new long[]{0x0000200000000002L});
public static final BitSet FOLLOW_DOT_in_extendedNameReference25857 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedNameReference_in_extendedNameReference25859 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_routineNameReference25956 = new BitSet(new long[]{0x0000200000000002L,0x0000000020000000L});
public static final BitSet FOLLOW_routineNameGenericArguments_in_routineNameReference25958 = new BitSet(new long[]{0x0000200000000002L});
public static final BitSet FOLLOW_DOT_in_routineNameReference25962 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedRoutineNameReference_in_routineNameReference25964 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_extendedIdent_in_extendedRoutineNameReference26053 = new BitSet(new long[]{0x0000200000000002L,0x0000000020000000L});
public static final BitSet FOLLOW_routineNameGenericArguments_in_extendedRoutineNameReference26055 = new BitSet(new long[]{0x0000200000000002L});
public static final BitSet FOLLOW_DOT_in_extendedRoutineNameReference26059 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedRoutineNameReference_in_extendedRoutineNameReference26061 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_extendedIdent26165 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_keywords_in_extendedIdent26198 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_labelIdent26259 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkIntNumber_in_labelIdent26292 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkHexNumber_in_labelIdent26331 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkBinaryNumber_in_labelIdent26370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_labelIdent_in_labelNameDeclaration26421 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_labelIdent_in_labelNameReference26479 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkIntNumber_in_intNum26552 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkHexNumber_in_intNum26588 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkBinaryNumber_in_intNum26624 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkRealNumber_in_realNum26685 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineImplementation_in_synpred37_Delphi2487 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineInterface_in_synpred43_Delphi2737 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_constDeclaration_in_synpred46_Delphi2903 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_synpred49_Delphi3043 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclaration_in_synpred49_Delphi3046 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_synpred49_Delphi3048 = new BitSet(new long[]{0x0000000000000800L});
public static final BitSet FOLLOW_fixedArrayType_in_synpred49_Delphi3050 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_EQUAL_in_synpred49_Delphi3052 = new BitSet(new long[]{0x0000000000000000L,0x0800000000000000L});
public static final BitSet FOLLOW_arrayExpression_in_synpred49_Delphi3054 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_synpred49_Delphi3056 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred49_Delphi3059 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeDeclaration_in_synpred53_Delphi3272 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeDeclaration_in_synpred54_Delphi3328 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_varDeclaration_in_synpred58_Delphi3512 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_portabilityDirective_in_synpred60_Delphi3573 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeList_in_synpred63_Delphi3564 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068C200000000100L});
public static final BitSet FOLLOW_nameDeclarationList_in_synpred63_Delphi3567 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_synpred63_Delphi3569 = new BitSet(new long[]{0x0000000000000800L});
public static final BitSet FOLLOW_fixedArrayType_in_synpred63_Delphi3571 = new BitSet(new long[]{0x0140002000000020L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_synpred63_Delphi3573 = new BitSet(new long[]{0x0140002000000020L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_arrayVarValueSpec_in_synpred63_Delphi3576 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_portabilityDirective_in_synpred63_Delphi3579 = new BitSet(new long[]{0x0100002000000000L,0x4000000080000000L,0x0000000000800000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred63_Delphi3582 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_portabilityDirective_in_synpred65_Delphi3672 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullFileType_in_synpred77_Delphi4178 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_classHelperType_in_synpred78_Delphi4211 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_classReferenceType_in_synpred79_Delphi4244 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_classType_in_synpred80_Delphi4277 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordType_in_synpred83_Delphi4376 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordHelperType_in_synpred84_Delphi4409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_pointerType_in_synpred85_Delphi4442 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullStringType_in_synpred86_Delphi4475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureType_in_synpred87_Delphi4508 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_subRangeType_in_synpred88_Delphi4541 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeOfType_in_synpred89_Delphi4574 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_strongAliasType_in_synpred90_Delphi4607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_weakAliasType_in_synpred91_Delphi4640 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_enumType_in_synpred92_Delphi4673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullStringType_in_synpred95_Delphi4833 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullFileType_in_synpred96_Delphi4866 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_pointerType_in_synpred98_Delphi4932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureType_in_synpred99_Delphi4965 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_subRangeType_in_synpred100_Delphi4998 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_typeReference_in_synpred101_Delphi5031 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_enumType_in_synpred102_Delphi5064 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_lbrack_in_synpred114_Delphi6249 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred114_Delphi6251 = new BitSet(new long[]{0x0000000000000000L,0x0400000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_rbrack_in_synpred114_Delphi6253 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_procedureOfObject_in_synpred115_Delphi6307 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred118_Delphi6433 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_synpred118_Delphi6437 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineParameters_in_synpred119_Delphi6603 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred122_Delphi6611 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_synpred122_Delphi6615 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineParameters_in_synpred124_Delphi6656 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred126_Delphi6661 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_synpred126_Delphi6665 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_visibilitySectionItem_in_synpred140_Delphi7840 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_visibilitySectionItem_in_synpred142_Delphi7874 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fieldSection_in_synpred143_Delphi7919 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineInterface_in_synpred144_Delphi7952 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_methodResolutionClause_in_synpred145_Delphi7985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_property_in_synpred146_Delphi8018 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fieldDecl_in_synpred150_Delphi8225 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fieldDecl_in_synpred152_Delphi8276 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_interfaceGuid_in_synpred160_Delphi8549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_routineInterface_in_synpred164_Delphi8740 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ALIGN_in_synpred169_Delphi8976 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred169_Delphi8978 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_fullRoutineImplementation_in_synpred209_Delphi11627 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_externalRoutine_in_synpred210_Delphi11660 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_attributeGroup_in_synpred242_Delphi14863 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ASSEMBLY_in_synpred245_Delphi15064 = new BitSet(new long[]{0x0000000010000000L});
public static final BitSet FOLLOW_COLON_in_synpred245_Delphi15066 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_relationalOperator_in_synpred250_Delphi15284 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_additiveExpression_in_synpred250_Delphi15287 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_addOperator_in_synpred251_Delphi15341 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0808L,0x01400003E866EC71L,0x068C200000302380L});
public static final BitSet FOLLOW_multiplicativeExpression_in_synpred251_Delphi15344 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_atom_in_synpred254_Delphi15533 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_parenthesizedExpression_in_synpred255_Delphi15577 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nameReference_in_synpred256_Delphi15613 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_particleItem_in_synpred257_Delphi15616 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_nameReference_in_synpred258_Delphi15613 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_particleItem_in_synpred258_Delphi15616 = new BitSet(new long[]{0x0000204000000002L,0x0A00000000000000L,0x0000000008000000L});
public static final BitSet FOLLOW_particleItem_in_synpred259_Delphi15763 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_particleItem_in_synpred268_Delphi16087 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_synpred276_Delphi16633 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_synpred276_Delphi16635 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_escapedCharacter_in_synpred293_Delphi17395 = new BitSet(new long[]{0x0000004000000000L,0x0000000000000000L,0x0100000000000000L,0x0000000000100000L});
public static final BitSet FOLLOW_TkQuotedString_in_synpred293_Delphi17398 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_escapedCharacter_in_synpred294_Delphi17402 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_escapedCharacter_in_synpred296_Delphi17436 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_escapedCharacter_in_synpred297_Delphi17442 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TkQuotedString_in_synpred298_Delphi17440 = new BitSet(new long[]{0x0000004000000000L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_escapedCharacter_in_synpred298_Delphi17442 = new BitSet(new long[]{0x0000004000000002L,0x0000000000000000L,0x0100000000000000L});
public static final BitSet FOLLOW_TkQuotedString_in_synpred299_Delphi17447 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_synpred324_Delphi18738 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_recordExpression_in_synpred325_Delphi18771 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_labelStatement_in_synpred342_Delphi19483 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_assignmentStatement_in_synpred343_Delphi19516 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expressionStatement_in_synpred344_Delphi19549 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred345_Delphi19646 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred346_Delphi19652 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ELSE_in_synpred347_Delphi19650 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_synpred347_Delphi19652 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred355_Delphi20097 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred357_Delphi20247 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred358_Delphi20319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FOR_in_synpred359_Delphi20301 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_forVar_in_synpred359_Delphi20307 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_synpred359_Delphi20309 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred359_Delphi20311 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000004000000000L});
public static final BitSet FOLLOW_TO_in_synpred359_Delphi20313 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred359_Delphi20315 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_synpred359_Delphi20317 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_synpred359_Delphi20319 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred360_Delphi20371 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FOR_in_synpred361_Delphi20353 = new BitSet(new long[]{0xAB01013A0406C160L,0x60BB0585100A0808L,0x00000001E066EC71L,0x068E200000000100L});
public static final BitSet FOLLOW_forVar_in_synpred361_Delphi20359 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_ASSIGN_in_synpred361_Delphi20361 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred361_Delphi20363 = new BitSet(new long[]{0x0000800000000000L});
public static final BitSet FOLLOW_DOWNTO_in_synpred361_Delphi20365 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred361_Delphi20367 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_DO_in_synpred361_Delphi20369 = new BitSet(new long[]{0xEB01017A8626E1E2L,0xEABB0F8D101A889CL,0x01400083E867EE73L,0x07AE200000302380L});
public static final BitSet FOLLOW_statement_in_synpred361_Delphi20371 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred362_Delphi20419 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred365_Delphi20608 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_statement_in_synpred369_Delphi20842 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_handler_in_synpred373_Delphi21299 = new BitSet(new long[]{0x0010000000000002L,0x0001000000000000L});
public static final BitSet FOLLOW_elseBlock_in_synpred373_Delphi21302 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_synpred377_Delphi21475 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_AT_in_synpred378_Delphi21479 = new BitSet(new long[]{0xEB01017A0406C1E0L,0xEABB0F8D101A0818L,0x01400003E866EC73L,0x068C200000302380L});
public static final BitSet FOLLOW_expression_in_synpred378_Delphi21482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred383_Delphi21638 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_synpred383_Delphi21641 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred383_Delphi21645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred387_Delphi21724 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_interfaceDirective_in_synpred387_Delphi21727 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred387_Delphi21731 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred394_Delphi21812 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_synpred394_Delphi21815 = new BitSet(new long[]{0xAB01012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred394_Delphi21819 = new BitSet(new long[]{0x0800000000000000L});
public static final BitSet FOLLOW_externalDirective_in_synpred394_Delphi21822 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred394_Delphi21825 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_synpred394_Delphi21828 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred394_Delphi21831 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred402_Delphi21923 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_synpred402_Delphi21926 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400008L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred402_Delphi21930 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000008L});
public static final BitSet FOLLOW_FORWARD_in_synpred402_Delphi21933 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred402_Delphi21936 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x000000006020C000L,0x008C200000000000L});
public static final BitSet FOLLOW_implDirective_in_synpred402_Delphi21939 = new BitSet(new long[]{0xA301012004004040L,0x6030010580400000L,0x0000000060A0C000L,0x008C200000000000L});
public static final BitSet FOLLOW_SEMICOLON_in_synpred402_Delphi21942 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_textLiteral_in_synpred431_Delphi23242 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_dllName_in_synpred435_Delphi23393 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_externalSpecifier_in_synpred436_Delphi23396 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_genericArguments_in_synpred625_Delphi25663 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DOT_in_synpred626_Delphi25667 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedNameReference_in_synpred626_Delphi25669 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_genericArguments_in_synpred627_Delphi25853 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DOT_in_synpred628_Delphi25857 = new BitSet(new long[]{0xEFB197BB8E26FD60L,0x61BFCF9591FF889FL,0x000001F3E76FFE7FL,0x17AEF00000000100L});
public static final BitSet FOLLOW_extendedNameReference_in_synpred628_Delphi25859 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ident_in_synpred633_Delphi26165 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy