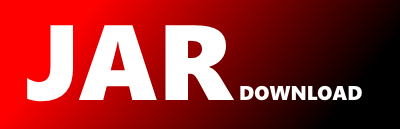
au.csiro.pathling.api.EncodeMapPartitionFunc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of encoders Show documentation
Show all versions of encoders Show documentation
Encoders for transforming FHIR data into Spark Datasets.
/*
* This is a modified version of the Bunsen library, originally published at
* https://github.com/cerner/bunsen.
*
* Bunsen is copyright 2017 Cerner Innovation, Inc., and is licensed under
* the Apache License, version 2.0 (http://www.apache.org/licenses/LICENSE-2.0).
*
* These modifications are copyright © 2018-2022, Commonwealth Scientific
* and Industrial Research Organisation (CSIRO) ABN 41 687 119 230. Licensed
* under the CSIRO Open Source Software Licence Agreement.
*
*/
package au.csiro.pathling.api;
import static au.csiro.pathling.api.FhirMimeTypes.FHIR_JSON;
import static au.csiro.pathling.api.FhirMimeTypes.FHIR_XML;
import au.csiro.pathling.encoders.FhirEncoders;
import ca.uhn.fhir.context.FhirContext;
import ca.uhn.fhir.context.FhirVersionEnum;
import ca.uhn.fhir.parser.IParser;
import java.util.Iterator;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import javax.annotation.Nonnull;
import org.apache.spark.api.java.function.MapPartitionsFunction;
import org.hl7.fhir.instance.model.api.IBaseResource;
abstract class EncodeMapPartitionsFunc implements
MapPartitionsFunction {
private static final long serialVersionUID = -189338116652852324L;
protected FhirVersionEnum fhirVersion;
protected final String inputMimeType;
protected final Class resourceClass;
protected EncodeMapPartitionsFunc(FhirVersionEnum fhirVersion, final String inputMimeType,
Class resourceClass) {
this.fhirVersion = fhirVersion;
this.inputMimeType = inputMimeType;
this.resourceClass = resourceClass;
}
/**
* Converts the parsed input resouces to the final stream of requested resources. May include
* filtering, extracting from bundles etc.
*
* @param resources the stream of all input resources
* @return the stream of desired resources
*/
@Nonnull
protected abstract Stream processResources(
@Nonnull final Stream resources);
@Override
@Nonnull
public Iterator call(@Nonnull final Iterator iterator) {
final IParser parser = createParser(inputMimeType);
final Iterable iterable = () -> iterator;
final Stream parsedResources = StreamSupport.stream(iterable.spliterator(),
false)
.map(parser::parseResource);
//noinspection unchecked
return (Iterator) processResources(parsedResources).iterator();
}
@Nonnull
protected IParser createParser(@Nonnull final String mimeType) {
final FhirContext fhirContext = FhirEncoders.contextFor(fhirVersion);
switch (mimeType) {
case FHIR_JSON:
return fhirContext.newJsonParser();
case FHIR_XML:
return fhirContext.newXmlParser();
default:
throw new IllegalArgumentException("Cannot create FHIR parser for mime type: " + mimeType);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy