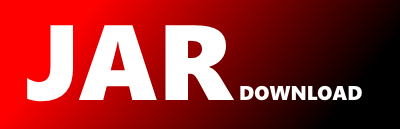
au.csiro.ontology.importer.rf2.VersionRows Maven / Gradle / Ivy
/**
* Copyright CSIRO Australian e-Health Research Centre (http://aehrc.com).
* All rights reserved. Use is subject to license terms and conditions.
*/
package au.csiro.ontology.importer.rf2;
import java.util.ArrayList;
import java.util.Collection;
import au.csiro.ontology.snomed.refset.rf2.RefsetRow;
/**
* This class represents a collection of rows from the RF2 concepts,
* descriptions, and relationships tables that correspond to a logical version.
*
* @author Alejandro Metke
*
*/
public class VersionRows {
/**
* The concept rows in this version.
*/
protected final Collection conceptRows;
/**
* The relationship rows in this version.
*/
protected final Collection relationshipRows;
/**
* The concrete domains reference set rows in this version.
*/
protected final Collection concreteDomainRows;
/**
* The attribute domains reference set rows in this version.
*/
protected final Collection attributeDomainRows;
/**
* The OWL reference set rows in this version.
*/
protected final Collection owlRows;
/**
* Builds a new VersionRows.
*/
public VersionRows() {
this(new ArrayList(), new ArrayList(), new ArrayList(), new ArrayList(), new ArrayList());
}
public VersionRows(Collection conceptRows, Collection relationshipRows,
Collection concreteDomainRows, Collection attributeDomainRows,
Collection owlRows) {
this.conceptRows = conceptRows;
this.relationshipRows = relationshipRows;
this.concreteDomainRows = concreteDomainRows;
this.attributeDomainRows = attributeDomainRows;
this.owlRows = owlRows;
}
/**
* @return the conceptRows
*/
public Collection getConceptRows() {
return conceptRows;
}
/**
* @return the relationshipRows
*/
public Collection getRelationshipRows() {
return relationshipRows;
}
/**
*
* @return the concrete domain refsetRows (includes current inactive rows)
*/
public Collection getConcreteDomainRows() {
return concreteDomainRows;
}
/**
*
* @return the attribute domain refsetRows (includes current inactive rows)
*/
public Collection getAttributeDomainRows() {
return attributeDomainRows;
}
/**
*
* @return the owl refsetRows (includes current inactive rows)
*/
public Collection getOwlRows() {
return owlRows;
}
/**
* Merges another {@link VersionRows} into this.
*
* @param other
*/
public void merge(VersionRows other) {
conceptRows.addAll(other.conceptRows);
relationshipRows.addAll(other.relationshipRows);
concreteDomainRows.addAll(other.getConcreteDomainRows());
attributeDomainRows.addAll(other.getAttributeDomainRows());
owlRows.addAll(other.getConcreteDomainRows());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy