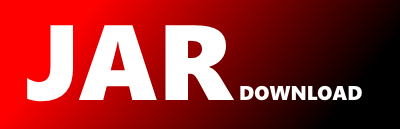
au.net.causal.shoelaces.jersey.common.JacksonJsonStringReaderProvider Maven / Gradle / Ivy
The newest version!
package au.net.causal.shoelaces.jersey.common;
import jakarta.ws.rs.Consumes;
import jakarta.ws.rs.WebApplicationException;
import jakarta.ws.rs.core.Context;
import jakarta.ws.rs.core.MediaType;
import jakarta.ws.rs.core.MultivaluedMap;
import jakarta.ws.rs.ext.MessageBodyReader;
import jakarta.ws.rs.ext.Providers;
import org.glassfish.jersey.jackson.internal.jackson.jaxrs.base.ProviderBase;
import java.io.IOException;
import java.io.InputStream;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
@Consumes(MediaType.APPLICATION_JSON)
public class JacksonJsonStringReaderProvider implements MessageBodyReader
{
@Context
protected Providers workers;
/**
* Finds an already-registered delegate for handling the specified media type for arbitrary objects.
* This should typically find the Jackson provider, however if another has been registered then this will
* be picked up.
*
* @param annotations an array of the annotations attached to the message entity instance.
* @param mediaType the media type of the HTTP entity. This will usually be {@link MediaType#APPLICATION_JSON_TYPE} since this is what this class is annotated
* to handle, but subclasses may change this.
*
* @return a delegate writer for the given media type.
*
* @throws RuntimeException if no writer could be found for the given media type. This provider should only be used when there is a known provider for handled
* media type already registered, such as Jackson for application/json.
*/
protected MessageBodyReader
© 2015 - 2025 Weber Informatics LLC | Privacy Policy