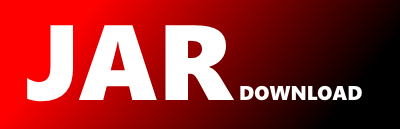
net.jini.lease.LeaseUnmarshalException Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.jini.lease;
import java.io.IOException;
import java.io.InvalidObjectException;
import java.rmi.MarshalledObject;
import net.jini.core.lease.Lease;
import org.apache.river.api.io.AtomicException;
import org.apache.river.api.io.AtomicSerial;
import org.apache.river.api.io.AtomicSerial.GetArg;
import org.apache.river.api.io.Valid;
/**
* Exception thrown when a lease renewal set can't unmarshal one or more
* leases being returned by a getLeases
call.
*
* When unmarshalling an instance of MarshalledObject
, one
* of the following checked exceptions is possible: an
* IOException
can occur while deserializing the object
* from its internal representation; and a
* ClassNotFoundException
can occur if, while deserializing
* the object from its internal representation, either the class file of
* the object cannot be found, or the class file of either an interface
* or a class referenced by the object being deserialized cannot be
* found. Typically, a ClassNotFoundException
occurs when
* the codebase from which to retrieve the needed class file is not
* currently available.
*
* This class provides a mechanism that clients of the lease renewal
* service may use for efficient handling of the exceptions that may
* occur when unmarshalling elements of a set of marshalled
* Lease
objects. When elements in such a set are
* unmarshalled, the LeaseUnmarshalException
class may be
* used to collect and report pertinent information generated when
* failure occurs while unmarshalling the elements of the set.
*
* The information that may be of interest to entities that receive this
* exception class is contained in the following fields: a set of
* Lease
objects in which each element is the result of a
* successful unmarshalling attempt, a set of marshalled
* Lease
objects in which each element could not be
* successfully unmarshalled, and a set of exceptions
* (IOException
, ClassNotFoundException
, or
* some unchecked exception) in which each element corresponds to one of
* the unmarshalling failures.
*
* Thus, when exceptional conditions occur while unmarshalling a set of
* marshalled Lease
objects, this class can be used not
* only to indicate that an exceptional condition has occurred, but also
* to provide information that can be used to perform error handling
* activities such as: determining if it is feasible to continue with
* processing, reporting errors, attempting recovery, and debugging.
*
* Note that this exception class should be used only to report
* exceptional conditions occurring when unmarshalling a set of
* marshalled Lease
objects.
*
* @author Sun Microsystems, Inc.
* @see Lease
*/
@AtomicSerial
public class LeaseUnmarshalException extends AtomicException {
private static final long serialVersionUID = -6736107321698417489L;
/**
* Array containing the set of instances of Lease
that
* were successfully unmarshalled during the process in which at
* least one failure occurred.
*
* @serial
*/
final private Lease[] unmarshalledLeases;
/**
* Array containing the set of Lease
instances that
* could not be unmarshalled. This set should contain at least one
* element.
*
* @serial
*/
final private MarshalledObject[] stillMarshalledLeases;
/**
* Array containing the set of exceptions that occurred during the
* unmarshalling process. Each element in this set should be an
* instance of IOException
,
* ClassNotFoundException
, or some unchecked
* exception. Furthermore, there should be a one-to-one
* correspondence between each element in this set and each element
* in the set of still-to-be-unmarshalled Lease
* instances. That is, the element of this set corresponding to
* index i should be an instance of the exception that occurred
* while attempting to unmarshal the element at index i of
* stillMarshalledLeases
.
*
* @serial
*/
final private Throwable[] exceptions;
/**
* Constructs a new instance of LeaseUnmarshalException
* with a specified message.
*
* @param leases array containing the set of instances of
* Lease
that were successfully unmarshalled
* @param marshalledLeases array containing the set of marshalled
* Lease
instances that could not be
* unmarshalled
* @param exceptions array containing the set of exceptions that
* occurred during the unmarshalling process. Each element in
* this set should be an instance of
* IOException
,
* ClassNotFoundException
, or some unchecked
* exception. Furthermore, there should be a one-to-one
* correspondence between each element in this set and each
* element in the marshalledLeases
argument.
*
* That is, the element of this set corresponding to index i
* should be an instance of the exception that occurred while
* attempting to unmarshal the element at index i of the
* marshalledLeases
argument.
* @param message the detail message
* @throws IllegalArgumentException when the number of elements in
* the exceptions
argument is not equal to the
* number of elements in the marshalledLeases
* argument
*/
public LeaseUnmarshalException(String message,
Lease[] leases,
MarshalledObject[] marshalledLeases,
Throwable[] exceptions)
{
this(message,
leases,
marshalledLeases,
exceptions,
validate(marshalledLeases, exceptions)
);
}
/**
* Constructs a new instance of LeaseUnmarshalException
.
*
* @param leases array containing the set of instances of
* Lease
that were successfully unmarshalled
* @param marshalledLeases array containing the set of marshalled
* Lease
instances that could not be
* unmarshalled
* @param exceptions array containing the set of exceptions that
* occurred during the unmarshalling process. Each element in
* this set should be an instance of
* IOException
,
* ClassNotFoundException
, or some unchecked
* exception. Furthermore, there should be a one-to-one
* correspondence between each element in this set and each
* element in the marshalledLeases
argument.
*
* That is, the element of this set corresponding to index i
* should be an instance of the exception that occurred while
* attempting to unmarshal the element at index i of the
* marshalledLeases
argument.
* @throws IllegalArgumentException when the number of elements in
* the exceptions
argument is not equal to the
* number of elements in the marshalledLeases
* argument
*/
public LeaseUnmarshalException(Lease[] leases,
MarshalledObject[] marshalledLeases,
Throwable[] exceptions)
{
this(leases,
marshalledLeases,
exceptions,
validate(marshalledLeases, exceptions)
);
}
private LeaseUnmarshalException(Lease[] leases,
MarshalledObject[] marshalledLeases,
Throwable[] exceptions,
boolean check)
{
this.unmarshalledLeases = leases;
this.stillMarshalledLeases = marshalledLeases;
this.exceptions = exceptions;
}
private LeaseUnmarshalException(String message,
Lease[] leases,
MarshalledObject[] marshalledLeases,
Throwable[] exceptions,
boolean check)
{
super(message);
this.unmarshalledLeases = leases;
this.stillMarshalledLeases = marshalledLeases;
this.exceptions = exceptions;
}
public LeaseUnmarshalException(GetArg arg) throws IOException, ClassNotFoundException
{
super(validateSerial(arg));
unmarshalledLeases
= Valid.copy(arg.get("unmarshalledLeases", null, Lease[].class));
stillMarshalledLeases
= Valid.copy(arg.get("stillMarshalledLeases", null, MarshalledObject[].class));
exceptions
= Valid.copy(arg.get("exceptions", null, Throwable[].class));
}
private static GetArg validateSerial(GetArg arg) throws IOException, ClassNotFoundException{
MarshalledObject[] marshalledLeases
= arg.get("stillMarshalledLeases", null, MarshalledObject[].class);
Throwable[] exceptions = arg.get("exceptions", null, Throwable[].class);
try {
validate(marshalledLeases, exceptions);
} catch (IllegalArgumentException e){
InvalidObjectException ex = new InvalidObjectException("validation failed");
ex.initCause(e);
throw ex;
} catch (NullPointerException e){
InvalidObjectException ex = new InvalidObjectException("validation failed");
ex.initCause(e);
throw ex;
}
return arg;
}
/**
* Helper method for constructors. Throws an
* IllegalArgumentException
if the length of
* marshalledLeases
does not match the length of
* exceptions
.
*/
private static boolean validate(MarshalledObject[] marshalledLeases,
Throwable[] exceptions)
{
/*
* If the number of exceptions does not equal number of leases
* that could not be unmarshalled, throw exception.
*/
if(exceptions.length != marshalledLeases.length) {
throw new IllegalArgumentException
("exceptions.length ("+exceptions.length
+") is not equal to marshalledLeases.length ("
+marshalledLeases.length +")");
}
return true;
}
/**
* Accessor method that returns an array consisting of instances of
* Lease
, where each element of the array corresponds
* to a successfully unmarshalled object. Note that a copy is returned
* on each invocation of this method.
*
* @return array of instances of Lease
, where each
* element corresponds to a successfully unmarshalled object
*/
public Lease[] getLeases() {
return unmarshalledLeases.clone();
}
/**
* Accessor method that returns an array consisting of instances of
* MarshalledObject
, where each element of the array is
* a marshalled instance of the Lease
interface, and
* corresponds to an object that could not be successfully
* unmarshalled. Note that a copy is returned on each
* invocation of this method.
*
* @return array of marshalled instances of Lease
,
* where each element corresponds to an object in which
* failure occurred while attempting to unmarshal the object
*/
public MarshalledObject[] getMarshalledLeases() {
return stillMarshalledLeases.clone();
}
/**
* Accessor method that returns an array consisting of instances of
* Throwable
, where each element of the array
* corresponds to one of the exceptions that occurred during the
* unmarshalling process. Note a copy is returned on
* each invocation of this method.
*
* Each element in the return set should be an instance of
* IOException
, ClassNotFoundException
, or
* some unchecked exception. Additionally, there should be a
* one-to-one correspondence between each element in the array
* returned by this method and the array returned by the
* getMarshalledLeases
method. That is, the i-th
* element of the set returned by this method should be an instance
* of the exception that occurred while attempting to unmarshal the
* i-th element of the set returned by the
* getMarshalledLeases
method.
*
* @return array of instances of Throwable
, where each
* element corresponds to one of the exceptions that
* occurred during the unmarshalling process
*/
public Throwable[] getExceptions() {
return exceptions.clone();
}
}