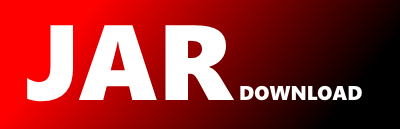
net.jini.lease.RenewalFailureEvent Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.jini.lease;
import java.io.IOException;
import java.rmi.MarshalledObject;
import net.jini.core.event.RemoteEvent;
import net.jini.core.lease.Lease;
import org.apache.river.api.io.AtomicSerial;
import org.apache.river.api.io.AtomicSerial.GetArg;
/**
* Event generated by a lease renewal set when it can't renew a lease it
* is responsible for.
*
* RenewalFailureEvent
is a subclass of
* RemoteEvent
, adding two additional items of abstract
* state: the client lease that could not be renewed before expiration
* and the Throwable
object that was thrown by the last
* renewal attempt (if any).
*
* The methods of this interface are declared to allow implementations
* to defer the unmarshalling of the lease and Throwable
* until the client explicitly asks for them.
*
* @author Sun Microsystems, Inc.
* @see LeaseRenewalSet
*/
@AtomicSerial
public abstract class RenewalFailureEvent extends RemoteEvent {
private static final long serialVersionUID = 8891457049195932943L;
/**
* Simple constructor. Note event id is fixed to
* LeaseRenewalSet.RENEWAL_FAILURE_EVENT_ID
.
*
* @param source the LeaseRenewalSet
that generated the
* event
* @param seqNum the sequence number of this event
* @param handback the client handback
*/
public RenewalFailureEvent(LeaseRenewalSet source,
long seqNum,
MarshalledObject handback)
{
super(source, LeaseRenewalSet.RENEWAL_FAILURE_EVENT_ID, seqNum,
handback);
}
/**
* Can only be called by subclasses that implement @AtomicSerial
* @see AtomicSerial
* @param arg
* @throws IOException
*/
protected RenewalFailureEvent(GetArg arg)
throws IOException, ClassNotFoundException{
super(arg);
}
/**
* Returns the lease that could not be renewed. This method may
* cause the lease to be unmarshalled. If it does and unmarshalling
* fails, future calls will attempt to re-unmarshal the lease. Once
* this method succeeds, subsequent calls must return the same
* object.
*
* If the renewal service was able to renew the lease before the
* event occurred, the expiration time of the Lease
* object returned by this method will reflect the result of the
* last successful renewal call. This time may be distorted by clock
* skew between hosts if it is currently set to use the
* Lease.ABSOLUTE
serial format. If the
* Lease
object is using the
* Lease.DURATION
serial format, and the implementation
* only unmarshals the lease when getLease
is called,
* the expiration time may be distorted if a long time has passed
* between the time the event was generated by the renewal service
* and when the client called getLease
. When a renewal
* failure event is generated for a given lease, that lease is
* removed from the set.
*
* @return the lease that could not be renewed
* @throws IOException if there are difficulties unmarshalling the
* lease, usually this will be some sort of class mismatch
* @throws ClassNotFoundException if there are difficulties
* unmarshalling the lease, usually this will indicate one
* of the classes associated with the lease's implementation
* could not be loaded
*/
public abstract Lease getLease()
throws IOException, ClassNotFoundException;
/**
* Returns the Throwable
(if any) that was thrown by
* the last renewal attempt. If null
is returned it can
* be assumed ether the last renewal attempt succeeded, or that the
* renewal service was unable to make a renewal attempt before the
* lease expired.
*
* This method may cause the Throwable
to be
* unmarshalled. If it does and unmarshalling fails, future calls
* will attempt to re-unmarshal the Throwable
. Once
* this method succeeds, subsequent calls must return the same
* object.
*
* @return the Throwable
(if any) that was thrown by
* the last renewal attempt
* @throws IOException if there are difficulties unmarshalling the
* Throwable
, usually this will be some sort of
* class mismatch
* @throws ClassNotFoundException if there are difficulties
* unmarshalling the Throwable
, usually this
* will indicate one of the classes associated with the
* implementation of the Throwable
could not be
* loaded
*/
public abstract Throwable getThrowable()
throws IOException, ClassNotFoundException;
}