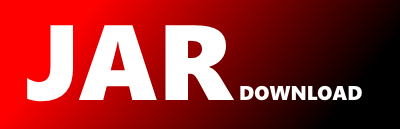
net.jini.lookup.entry.UIDescriptorBean Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.jini.lookup.entry;
import java.beans.ConstructorProperties;
import java.io.Serializable;
import java.rmi.MarshalledObject;
import java.util.Set;
import net.jini.core.entry.Entry;
/**
* A JavaBeans(TM) component that encapsulates a UIDescriptor
object.
*
* @see UIDescriptor
*/
public class UIDescriptorBean implements EntryBean, Serializable {
private static final long serialVersionUID = -3185268510626360709L;
/**
* @serial The UIDescriptor
object associated with this JavaBeans component.
*/
protected UIDescriptor assoc;
/**
* Construct a new JavaBeans component, linked to a new empty UIDescriptor
object.
*/
public UIDescriptorBean() {
assoc = new UIDescriptor();
}
@ConstructorProperties({"role", "toolkit", "attributes", "factory"})
public UIDescriptorBean(String role, String toolkit,
Set attributes, MarshalledObject factory)
{
assoc = new UIDescriptor(role, toolkit, attributes, factory);
}
/**
* Make a link to a UIDescriptor
object.
*
* @param e the Entry
object, which must be a UIDescriptor
, to which to link
* @exception java.lang.ClassCastException the Entry
is not
* a UIDescriptor
, the correct type for this JavaBeans component
*/
public void makeLink(Entry e) {
assoc = (UIDescriptor) e;
}
/**
* Return the UIDescriptor
linked to by this JavaBeans component.
*/
public Entry followLink() {
return assoc;
}
/**
* Return the value of the role
field in the object linked to by
* this JavaBeans component.
*
* @return a String
representing the role value
* @see #setRole
*/
public String getRole() {
return assoc.role;
}
/**
* Set the value of the role
field in the object linked to by this
* JavaBeans component.
*
* @param role a String
specifying the role value
* @see #getRole
*/
public void setRole(String role) {
assoc.role = role;
}
/**
* Return the value of the toolkit
field in the object linked to by
* this JavaBeans component.
*
* @return a String
representing the toolkit value
* @see #setToolkit
*/
public String getToolkit() {
return assoc.toolkit;
}
/**
* Set the value of the toolkit
field in the object linked to by this
* JavaBeans component.
*
* @param toolkit a String
specifying the toolkit value
* @see #getToolkit
*/
public void setToolkit(String toolkit) {
assoc.toolkit = toolkit;
}
/**
* Return the value of the attributes
field in the object linked to by
* this JavaBeans component.
*
* @return a Set
representing the attributes value
* @see #setAttributes
*/
public Set getAttributes() {
return assoc.attributes;
}
/**
* Set the value of the attributes
field in the object linked to by this
* JavaBeans component.
*
* @param attributes a Set
specifying the attributes value
* @see #getAttributes
*/
public void setAttributes(Set attributes) {
assoc.attributes = attributes;
}
/**
* Return the value of the factory
field in the object linked to by
* this JavaBeans component.
*
* @return a MarshalledObject
representing the factory value
* @see #setFactory
*/
public MarshalledObject getFactory() {
return assoc.factory;
}
/**
* Set the value of the factory
field in the object linked to by this
* JavaBeans component.
*
* @param factory a MarshalledObject
specifying the factory value
* @see #getFactory
*/
public void setFactory(MarshalledObject factory) {
assoc.factory = factory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy