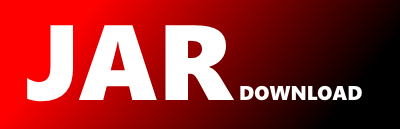
net.jini.space.JavaSpace05 Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.jini.space;
import java.rmi.MarshalledObject;
import java.rmi.MarshalException;
import java.rmi.RemoteException;
import java.util.Collection;
import java.util.List;
import net.jini.core.entry.Entry;
import net.jini.core.entry.UnusableEntryException;
import net.jini.core.event.EventRegistration;
import net.jini.core.event.RemoteEvent;
import net.jini.core.event.RemoteEventListener;
import net.jini.core.lease.Lease;
import net.jini.core.transaction.Transaction;
import net.jini.core.transaction.TransactionException;
import net.jini.entry.UnusableEntriesException;
/**
* The JavaSpace05
interface extends the {@link
* JavaSpace} interface to provide methods that allow clients to
* perform batch operations on the space (which are generally more
* efficient than performing an equivalent set of singleton
* operations) and to more easily develop applications where a
* given {@link Entry} needs to be read by multiple clients.
* Implementations of the JavaSpace
interface are not
* required to implement this interface.
*
* Unless otherwise noted, the effects of any invocation of a
* method defined by this interface must be visible to any
* operation on the space that is started after the invocation
* returns normally. Note, the effects of a method invocation that
* throws a {@link RemoteException} are not necessarily visible
* when the exception is thrown.
*
* All of the methods of this interface take one or more {@link
* Collection}s as arguments. Each such Collection
* must be treated as immutable by implementations and must not be
* changed by the client during the course of any method
* invocation to which they have been passed.
*
* This interface is not a remote interface. Each implementation of
* this interface exports a proxy object that implements this
* interface local to the client. Each method of the interface
* takes as one of its arguments a Collection
of
* Entry
instances. The entries themselves must be
* serialized in accordance with the Jini Entry
* Specification and will not be altered by the
* call. Typically, the Collection
holding the entries
* will not be serialized at all. If one of these entries can't be
* serialized, a {@link MarshalException} will be thrown. Aside
* from the handling of these Collection
of
* Entry
parameters, all methods defined by this
* interface otherwise obey normal Java(TM) Remote Method
* Invocation remote interface semantics.
*
* @see
* JavaSpaces Service Specification
* @see
* Jini Entry Specification
* @since 2.1
*/
public interface JavaSpace05 extends JavaSpace {
/**
* This method provides an overload of the {@link
* JavaSpace#write JavaSpace.write} method that allows new
* copies of multiple {@link Entry} instances to be stored in
* the space using a single call. The client may specify a
* {@link Transaction} for the operation to be performed
* under. Each Entry
to be stored in the space
* has a separate requested initial lease duration.
*
* The effect on the space of an invocation of this method
* successfully storing an Entry
is the same as if
* the Entry
had been successfully stored by a
* call to the singleton form of write
under
* txn
with the given requested initial lease
* duration. This method returns the proxies to the leases for
* each newly stored Entry
by returning a {@link
* List} of {@link Lease} instances. The i th element
* of the returned List
will be a proxy for the
* lease on the Entry
created from the i
* th element of entries
.
*
* If an invocation of this method returns normally, then a new
* copy of each element of entries
must have been
* stored in the space. A new copy of each element will be
* stored even if there are duplicates (either in terms of
* object identity or of entry equivalence) in
* entries
.
*
* The order in which the entries stored by an invocation of
* this method will become visible in the space is unspecified,
* and different observers may see the entries become visible
* in different orders.
*
* If a {@link TransactionException}, {@link
* SecurityException}, {@link IllegalArgumentException}, or
* {@link NullPointerException} is thrown, no entries will
* have been added to the space by this operation. If a {@link
* RemoteException} is thrown, either new copies of all of the
* elements of entries
will have been stored or
* no entries will have been stored; that is, in the case of a
* RemoteException
, the storing of new entries in
* the space will either fail or succeed as a unit.
*
* @param entries a {@link List} of {@link Entry} instances to
* be written to the space
* @param txn the {@link Transaction} this operation should be
* performed under, may be null
* @param leaseDurations a List
of
* {@link Long}s representing the
* requested initial lease durations
* @return a List
of {@link Lease} instances, one
* for each element of entries
, may be
* immutable. The space will not keep a reference to
* the result
* @throws TransactionException if txn
is
* non-null
and is not usable by the
* space
* @throws RemoteException if a communication error occurs
* @throws IllegalArgumentException if entries
and
* leaseDurations
are not the same length
* or are empty, if any element of entries
* is not an instance of Entry
, if any
* element of leaseDurations
is not an
* instance of Long
, or if any element of
* leaseDurations
is a negative value
* other than {@link Lease#ANY Lease.ANY}
* @throws NullPointerException if either entries
* or leaseDurations
is null
* or contains a null
value
*/
public List write(List entries,
Transaction txn,
List leaseDurations)
throws TransactionException, RemoteException;
/**
* This method provides an overload of the {@link
* JavaSpace#take JavaSpace.take} method that attempts to
* remove, optionally under a {@link Transaction}, and return
* one or more entries from the space. Each {@link Entry}
* taken will match one or more elements of the passed {@link
* Collection} of templates, and all of the taken entries will
* be visible to the passed Transaction
. If there
* are initially no matches in the space that are visible to
* the passed Transaction
, an invocation of this
* method will block for up to a specified timeout for one or
* more matches to appear.
*
* The effect on the space of an invocation of this method
* successfully taking an Entry
will be the same
* as if the Entry
had been taken using the
* singleton version of this method and passing
* txn
as the Transaction
.
*
* The tmpls
parameter must be a
* Collection
of Entry
instances to
* be used as templates. All of the entries taken must match
* one or more of these templates. The tmpls
* parameter may contain null
values and may
* contain duplicates. An Entry
is said to be
* available to an invocation of this method if the
* Entry
could have been returned by an
* invocation of the singleton take
method using
* txn
.
*
* If the method succeeds, a non-null
* Collection
will be returned. The
* Collection
will contain a copy of each
* Entry
that was taken. If no entries were taken,
* the Collection
will be empty. Each
* Entry
taken will be represented by a distinct
* Entry
instance in the returned
* Collection
, even if some of the entries are
* equivalent to others taken by the operation. There will be
* no null
elements in the returned
* Collection
.
*
* If one or more of the entries taken cannot be unmarshalled
* in the client, an {@link UnusableEntriesException} is
* thrown. The exception's {@link
* UnusableEntriesException#getEntries
* UnusableEntriesException.getEntries} method will return a
* Collection
with a copy of each
* Entry
that could be unmarshalled. The {@link
* UnusableEntriesException#getUnusableEntryExceptions
* UnusableEntriesException.getUnusableEntryExceptions} method
* will return a Collection
with an {@link
* UnusableEntryException} for each Entry
that
* could not be unmarshalled. Every Entry
taken
* by the invocation will either be represented in the
* Collection
returned by getEntries
* or in the Collection
returned by
* getUnusableEntryExceptions
.
*
* If there is at least one matching Entry
* available in the space, an invocation of this method must
* take at least one Entry
. If more than one
* matching Entry
is available, the invocation may
* take additional entries. It must not take more than
* maxEntries
, but an implementation may chose to
* take fewer entries from the space than the maximum available or
* the maximum allowed by maxEntries
. If for
* whatever reason, an invocation of this method takes fewer
* entries than the maximum number of available matching
* entries, how an implementation selects which entries should be
* taken by the invocation and which are left in the space is
* unspecified. How consumption of entries is arbitrated
* between conflicting queries is also unspecified.
*
* If there are initially no matching entries in the space, an
* invocation of this method should block for up to
* timeout
milliseconds for a match to appear. If
* one or more matches become available before
* timeout
expires, one or more of the newly
* available entries should be taken and the method should
* return without further blocking. If for some reason the
* invocation can't block for the full timeout and no entries
* have been taken, the invocation must fail with a {@link
* RemoteException} or {@link TransactionException} as
* appropriate.
*
* If an invocation of this method removes (or locks) more than
* one Entry
, the order in which the removal (or
* locking) occurs is undefined, and different observers may see
* the removal or locking of the entries in different
* orders.
*
* If a TransactionException
, {@link
* SecurityException}, {@link IllegalArgumentException}, or
* {@link NullPointerException} is thrown, no entries will
* have been taken. If a RemoteException
is
* thrown, up to maxEntries
may have been taken
* by this operation.
*
* @param tmpls a {@link Collection} of {@link Entry}
* instances, each representing a template. All
* of the entries taken by an invocation of this
* method will match one or more elements of
* tmpls
* @param txn the {@link Transaction} this operation should be
* performed under, may be null
* @param timeout if there are initially no available
* matches in the space, the maximum number of
* milliseconds to block waiting for a match to
* become available
* @param maxEntries the maximum number of entries that may be
* taken by this method
* @return a Collection
that contains a copy of each
* Entry
taken from the space by this
* method. The space will not keep a reference to
* this Collection
. May be immutable
* @throws UnusableEntriesException if one or more of the
* entries taken can't be unmarshalled in the client
* @throws TransactionException if txn
is
* non-null
and is not usable by the
* space.
* @throws RemoteException if a communication error occurs
* @throws IllegalArgumentException if any non-null
* element of tmpls
is not an instance of
* Entry
, if tmpls
is empty,
* if timeout
is negative, or if
* maxEntries
is non-positive
* @throws NullPointerException if tmpls
is null
*/
public Collection take(Collection tmpls,
Transaction txn,
long timeout,
long maxEntries)
throws UnusableEntriesException, TransactionException,
RemoteException;
/**
* Creates a {@linkplain MatchSet match set} that can be used to
* exhaustively read through all of the matching entries in
* the space that are visible to the passed {@link
* Transaction} and remain visible for the lifetime of the
* match set. May also yield additional entries that match but
* are only visible for part of the lifetime of the match
* set.
*
* The tmpls
parameter must be a {@link
* Collection} of {@link Entry} instances to be used as
* templates. All of the entries placed in the match set will
* match one or more of these templates. tmpls
may
* contain null
values and may contain
* duplicates. An Entry
is said to be
* visible to an invocation of this method if the
* Entry
could have been returned by a singleton
* {@link JavaSpace#read JavaSpace.read} using the same
* transaction.
*
* The resulting match set must initially contain all of the
* visible matching entries in the space. During the lifetime
* of the match set an Entry
may be, but is not
* required to be, added to the match set if it becomes
* visible. If the match set becomes empty, no more entries can
* be added and the match set enters the {@linkplain MatchSet
* exhausted} state.
*
* Normally there are three conditions under which an
* Entry
might be removed from the match set:
*
*
*
* - Any
Entry
yielded by an invocation of the
* {@link MatchSet#next MatchSet.next} method on the match
* set (either as the return value of a successful call or
* embedded in an {@link UnusableEntryException}) must be
* removed from the match set.
*
* - Any
Entry
that remains in the match set
* after maxEntries
entries are yielded by
* next
invocations must be removed from the
* match set. In such a case, the criteria used to select which
* entries are yielded by next
calls and which
* get removed from the set at the end is unspecified.
*
* - Any
Entry
that during the lifetime of the
* match set becomes invisible may at the discretion of the
* implementation be removed from the match set.
*
*
*
*
* An implementation may decide to remove an Entry
* from the set for other reasons. If it does so, however, it
* must {@linkplain MatchSet invalidate} the set.
*
* If txn
is non-null
and still
* active, any Entry
removed from the match set by
* a next
call must be locked as if it had been
* returned by a read operation using txn
. An
* implementation may establish the read lock on the
* Entry
any time between when the
* Entry
is added to the match set and when the
* Entry
is removed from the match set by an
* invocation of next
. These read locks are not
* released when the match set reaches either the exhausted
* state or the invalidated state. If from the space's
* perspective the txn
leaves the active state,
* the space must remove from the match set any entries in the
* match set that have not yet been read locked. This may
* require the match set to be invalidated.
*
* If the match set is leased and leaseDuration
* is positive, the initial duration of the lease must be less
* than or equal to leaseDuration
. If
* leaseDuration
is {@link Lease#ANY Lease.ANY},
* the initial duration of the lease can be any positive value
* desired by the implementation.
*
* If there are {@linkplain net.jini.core.constraint remote
* method constraints} associated with an invocation of this
* method, any remote communications performed by or on behalf
* of the match set's next
method will be
* performed in compliance with these constraints, not with the
* constraints (if any) associated with next
.
*
* @param tmpls a {@link Collection} of {@link Entry}
* instances, each representing a template. All
* the entries added to the resulting match set will
* match one or more elements of tmpls
* @param txn the {@link Transaction} this operation should be
* performed under, may be null
* @param leaseDuration the requested initial lease time on
* the resulting match set
* @param maxEntries the maximum number of entries to remove
* from the set via {@link MatchSet#next MatchSet.next}
* calls
* @return A proxy to the newly created {@linkplain MatchSet match set}
* @throws TransactionException if txn
is
* non-null
and is not usable by the
* space
* @throws RemoteException if a communication error occurs
* @throws IllegalArgumentException if any non-null
* element of tmpls
is not an instance of
* Entry
, if tmpls
is empty,
* if leaseDuration
is neither positive
* nor {@link Lease#ANY Lease.ANY}, or if
* maxEntries
is non-positive
* @throws NullPointerException if tmpls
is
* null
*/
public MatchSet contents(Collection tmpls,
Transaction txn,
long leaseDuration,
long maxEntries)
throws TransactionException, RemoteException;
/**
* Register for events triggered when a matching {@link Entry}
* transitions from unavailable to available. The resulting
* events will be instances of the {@link AvailabilityEvent}
* class and the {@link AvailabilityEvent#getEntry
* AvailabilityEvent.getEntry} method will return a copy of
* the Entry
whose transition triggered the
* event.
*
* An Entry
makes a transition from
* unavailable to available when it goes from being in
* a state where it could not be returned by a {@link
* JavaSpace#take JavaSpace.take} using txn
to a
* state where it could be returned. An Entry
* makes a transition from invisible to visible when
* it goes from being in a state where it could not be returned
* by a {@link JavaSpace#read JavaSpace.read} using
* txn
to a state where it could be
* returned. Note, any transition from invisible to visible is
* also a transition from unavailable to available, but an
* already visible entry can be unavailable and then make a
* transition from unavailable to available. Because the entry
* was already visible, this transition would not be a
* transition from invisible to visible.
*
* The tmpls
parameter must be a {@link
* Collection} of Entry
instances to be used as
* templates. Events will be generated when an
* Entry
that matches one or more of these
* templates makes an appropriate transition. A single
* transition will generate only one event per registration, in
* particular the transition of an Entry
that
* matches multiple elements of tmpls
must still
* generate exactly one event for this registration. If a given
* Entry
undergoes multiple applicable transitions
* while the registration is active, each must generate a
* separate event.
*
* Events are not generated directly by the transition of
* matching entries, but instead by an abstract observer set up
* in the space for each registration. The observer may see the
* transitions out of order and as a result the order of the
* events generated for this registration (as determined by the
* sequence numbers assigned to the events) may be different
* from the order of the transitions themselves. Additionally,
* each registration will have its own abstract observer and
* different observers may see the same sequence of transitions
* in different orders. As a result, given a set of transitions
* that trigger events for two different registrations, the
* order of the events generated for one registration may
* differ from the order of the events generated for the
* other.
*
* A non-null
{@link EventRegistration} object
* will be returned. Each registration will be assigned an
* event ID. The event ID will be unique at least with respect
* to all other active event registrations for
* AvailabilityEvent
s on this space with a
* non-equivalent set of templates, a different transaction,
* and/or a different value for the
* visibilityOnly
flag. The event ID can be
* obtained by calling the {@link EventRegistration#getID
* EventRegistration.getID} method on the returned
* EventRegistration
. The returned
* EventRegistration
object's {@link
* EventRegistration#getSource EventRegistration.getSource}
* method will return a reference to the space.
*
* Registrations are leased. leaseDurations
* represents the client's desired initial lease duration. If
* leaseDuration
is positive, the initial lease
* duration will be a positive value less than or equal to
* leaseDuration
. If leaseDuration
is
* {@link Lease#ANY Lease.ANY}, the space is free to pick any
* positive initial lease duration it desires. A proxy for the
* lease associated with the registration can be obtained by
* calling the returned EventRegistration
's {@link
* EventRegistration#getLease EventRegistration.getLease}
* method.
*
* A registration made with a non-null
value for
* txn
is implicitly dropped when the space
* observes txn
has left the active state.
*
* @param tmpls a {@link Collection} of {@link Entry}
* instances, each representing a
* template. Events for this registration will be
* generated by the transitions of entries
* matching one or more elements of
* tmpls
* @param txn the {@link Transaction} this operation should be
* performed under, may be null
* @param visibilityOnly if true
, events will
* be generated for this registration only when a
* matching Entry
transitions from
* invisible to visible, otherwise events will be
* generated when a matching Entry
* makes any transition from unavailable to
* available
* @param listener the object to which events generated for
* this registration should be delivered
* @param leaseDuration the requested initial lease time on
* the resulting event registration
* @param handback the {@link MarshalledObject} to be
* returned by the {@link
* RemoteEvent#getRegistrationObject
* RemoteEvent.getRegistrationObject} method of
* the events generated for this registration
* @return an {@link EventRegistration} object with
* information on this registration
* @throws TransactionException if txn
is
* non-null
and is not usable by the
* space
* @throws RemoteException if a communication error occurs
* @throws IllegalArgumentException if any non-null
* element of tmpls
is not an instance of
* Entry
, if tmpls
is empty,
* or if leaseDuration
is neither
* positive nor {@link Lease#ANY Lease.ANY}
* @throws NullPointerException if tmpls
or
* listener
is null
* @deprecated
*/
@Deprecated
public EventRegistration
registerForAvailabilityEvent(Collection tmpls,
Transaction txn,
boolean visibilityOnly,
RemoteEventListener listener,
long leaseDuration,
MarshalledObject handback)
throws TransactionException, RemoteException;
}