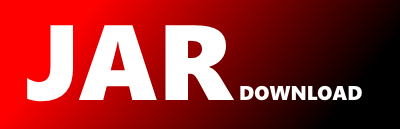
org.apache.river.admin.AdminIterator Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.river.admin;
import java.rmi.RemoteException;
import net.jini.core.entry.Entry;
import net.jini.core.entry.UnusableEntryException;
import net.jini.space.JavaSpace05;
import net.jini.space.MatchSet;
/**
* Interface for the iterators returned by the contents()
* method of JavaSpaceAdmin
. Note
* AdminIterator
s do not survive restarts of the
* underlying space.
*
*
* @deprecated Use {@link MatchSet} instead. MatchSet
s
* can be obtained using the
* {@link JavaSpace05#contents JavaSpace05.contents}
* method.
*
* @author Sun Microsystems, Inc.
*
* @see JavaSpaceAdmin
*/
public interface AdminIterator {
/**
* Return the next entry in the sequence. Returns null
* if there are no more matching entries in the space.
*
* This method is idempotent in the face of RemoteException
s.
*
* @return next Entry in the sequence.
* @throws UnusableEntryException if the field of next entry in
* sequence can't be deserialized (usually this is because the class
* in question could not be loaded).
* @throws java.rmi.RemoteException if connection problem occurs.
*/
public Entry next() throws UnusableEntryException, RemoteException;
/**
* The effect of this call depends on the most recent call to
* next()
:
*
* - If the last call to
next()
returned an
* Entry
that entry will be removed from the space.
*
* - If the last call to
next()
threw a
* UnusableEntryException
the Entry
that
* could not be deserialized will be removed from the space.
*
* - If the last call to
next()
returned
* null
, threw a RemoteException
, or
* next()
has not yet been called on this iterator a
* IllegalStateException
will be thrown and no entry will
* be removed.
*
*
* This method is idempotent in the face of
* RemoteException
.
*
* @throws java.rmi.RemoteException if connection problem occurs.
* @throws IllegalStateException if next()
has not be
* called on this iterator, or the last invocation of
* next()
returned null
or threw
* RemoteException
.
*/
public void delete() throws RemoteException;
/**
* Tell the server that this iterator is no longer in use. All
* operations on a closed iterator have undefined results, except
* the close()
method.
*
* This method is idempotent in the face of RemoteException
.
* @throws java.rmi.RemoteException if connection problem occurs, or if
* connection already closed.
*/
public void close() throws RemoteException;
}